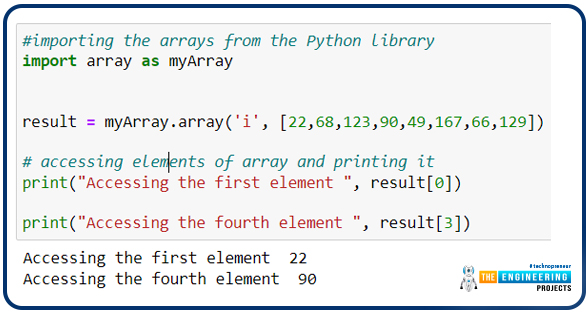
Hello Python programmers! Welcome to the engineering projects where you will find the best learning data in a precise way. We are dealing with Python nowadays, and today, the topic of discussion is the arrays in the language. We have seen different data types in Python and discussed a lot about them in detail till now. In the previous lecture, we saw the details of the procedures in dictionaries. There are certain ways to store the data in the different types of sequences, and we have highlighted a lot about almost all of them. It is time to discuss the arrays, but before this, it is better to understand the objectives of this lecture:
Introduction to arrays
Difference between contiguous and non-contiguous memory locations
One-dimensional arrays
Functions in arrays
What are Arrays in Programming Languages?
If you are from a programming background, then you have surely heard the name "arrays" and used them in your practice. Yet, if you are a beginner, you must know that arrays are present in almost all types of high-level programming languages. The array is also the way to organize and store the data in a sequential way, but it has some very basic characteristics, most of which are similar to those of other data types, but some are a little bit different, and we will work on both of these types. But before that, let's take a look at the basic definitions of an array:
“An array is a collection of items that are stored in contiguous memory locations. The idea is to group together items of the same type.”
This makes calculating the position of each element easier by simply adding an offset to a base value, i.e., the memory location of the array's first element. These are used to store a gigantic amount of data in different departments and offices, and because it is an old technique, people prefer it to other types such as sets, lists, and dictionaries.
Contiguous Locations in Array
When working with arrays, you will always notice the term "contiguous," and it makes sense because it is the best word to describe the structure of the array. It is the finite collection of data in which the elements are arranged strictly one after the other in the successive locations of memory, and in this way, a contiguous structure is obtained just like a ladder in a horizontal way. If you think it is all about knowing about the contiguous memory locations, then you are wrong. But, it is better to understand the concept by comparing it with the help of the opposite of it, that is the non-contiguous memory places.
Sr # |
Feature |
Contiguous Memory Allocation |
Non-Contiguous Memory Allocation |
1 |
Definition |
Contiguous memory locations are the type of allocation that allocates a continuous block of memory without skipping any places. |
Non-contiguous memory allocation, on the other hand, is the type in which memory is not continuous but separate blocks of memory are allocated regardless of how little space exists between them. |
2 |
Complexity |
The control of the operating system is easy in it because the memory can be smoothly be accessed. |
In this case, controlling the OS is comparatively difficult. |
3 |
Execution time |
The execution time is faster as compared to the other type, and it makes sense because the compiler does not have to sense the gap and then jump according to the length of the gap. |
In this case, the execution time is longer than in the first case because the compiler jumps from one memory location to the other. The time is determined by the gaps between the allocated memory. |
4 |
Overhead |
Because there are fewer address translations when dealing with contiguous memory allocation, there is less overhead. |
Because the address translation is greater in this case, we have more overhead. |
5 |
Special Features |
The contiguous memory allocation contains single-partition allocation as well as multi-partition allocation. |
When dealing with non-contiguous memory allocation, paging and segmentation are observed. |
6 |
Type of Fragmentation |
These include both internal and external fragmentation in the case of contiguous memory allocation. |
The internal fragmentation does not occur in this case, and we get only external fragmentation. |
7 |
Swap-in process |
It is noted that the swap-in process can only be arranged in the space that was originally allocated. |
If we talk about the non-contiguous memory allocation, the swap-in process is arranged in any memory allocation, so we get versatility in this case. |
8 |
Wastage of memory |
In this case, the wasted memory varies according to the array we are using. |
It is an advantage of this type of allocation that no memory is wasted in this case. |
9 |
Types |
There are only two types of contiguous memory allocation:
The hint for this point is also given in another feature. |
We observe five types in this case that are mentioned below:
All of this is unnecessary in our case; thus, do not go into detail about this type of situation. |
Importing the Array in Python
This is the distinguishing feature of arrays in Python when compared to other programming languages. Arrays, like many other functions and data types, are pre-defined in Python for the convenience of programmers. Because arrays are very general data types that programmers frequently use, it is simple to declare and import arrays in the same line and then begin working with the arrays. There are several ways to use arrays in Python, one of which is to import the arrays. Have a look at the details of this process:
There are different steps that are to be followed when dealing with the arrays in Python, and these are mentioned here:
Import the arrays.
Declare the name.
Use the array in different operations.
Hence using these steps, the arrays are used in different ways and it is interesting to notice that the declaration and the usage of an array are very different in Python as compared to other higher-level programming languages such as C++, C#, etc.
To understand well, let us see all these steps in action, but I want to discuss the type of the arrays with you while performing all these steps so that we may understand both these concepts one after the other.
Dimensions in the Arrays
Here is the point of the array that makes it different from the dictionaries and sets we have mentioned before. The arrays can be made in three ways:
One-dimensional array
Two-dimensional array
Three-dimensional array
Now it's the choice of the programmer according to the requirement, which type of array is he or she using. The details of each of them will be shared with you in just a bit, but before that, open your Jupyter notebook so that we can apply these arrays practically side by side.
Go to the search area of the window and get the Jupyter notebook there.
A screen will appear in front of you, and you have to go to the drop-down menu that is present on the right side of the screen.
Choose python there and wait for the new tab to open on your browser.
Click on the new cell location and start coding.
One-Dimensional Array
The most basic and commonly used array is one, and it does not require much effort for you to understand its concept. The very basic structure of the array that we see in the examples and discussion The elements are arranged one after the other, and the index starts at the first place where the zero number is assigned to that location. All the elements are linearly arranged, and it is the simplest form of array that is easy to create and access. In this way, the programmer gets the collection of the same data type in a linear format. The array is then stored in a variable to be used in different ways.
#importing the arrays from the Python library
import array as myArray
result = myArray.array('i', [22,68,123,90,49,167,66,129])
# accessing elements of array and printing it
print("Accessing the first element ", result[0])
print("Accessing the fourth element ", result[3])
The following output is observed as a result:
Hence, the single elements can be accessed in this way. Have you noticed that in the array, we have mentioned the “i” before, and after that, a list is being used? It has a very special reason, and you can check this by deleting the “i” in the code. You will observe that the compiler is throwing an error about the declaration of the Unicode character. Always remember that if the programmer is using the import array method in Python, there is a rule in Python for using arrays that the first argument of the array is always a Unicode character.
Functions for the Arrays in Python
Here comes the reason why we are importing the arrays into Python. The primary reason is, it has multiple functions that make the work super easy, and the programmers do not have to write the codes again and again. I am just sharing the very basic and common functions. So let’s jump to the codes:
count() in Python using Array
Take the example in your mind that when you are dealing with a gigantic amount of data at the same array and it becomes tedious to search for the frequency of the particular item. In such cases, when the detail of the element appearing in the array is required, the count function is used. The working of this function can be understood with this example:
#Declaring our array of strings
import array as myArray
result = myArray.array('i', [22,68,123,90,49,34,67,22,90,33,7,11,8,44,11,77,23,90,28,541,490,299,611,20,561,112,3,88,167,66,129])
#Using the count function to get frequency
print(result.count(90))
So, when we check the frequency of the number 90, we get the following output:
Hence, we can easily conclude that in our array, the integer 90 appears three times.
append() in Python Array
The next function that is to be tested is the append function. For this, we add a new element at the end of the array, and doing this is very simple, as you can see in the code given next:
#Declaring our array of integers
import array as myArray
result = myArray.array('i', [22,68,123,90,49,34,67,22,90,33,7,11,8,44,
11,77,23,90,28,541,490,299,611,20,561,112,3,88,167,66,129])
#appending a new 90 at the end of array
result.append(90)
print(result)
reverse() in Python Array
The next function is reverse(), and as you can guess, the reverse of the array can be obtained with the help of a short command that we are going to test in the code next:
#Declaring our array of strings
import array as myArray
result = myArray.array('i', [22,68,123,90,49,34,67,22,90,33,7,11,8,44,
11,77,23,90,28,541,490,299,611,20,561,112,3,88,167,66,129])
result.reverse()
print(result)
As you can see, the order of all the elements is reversed in such a way that the first element is the last and vice versa.
Consequently, in this lecture, we have seen the details of arrays in a different way than when the introduction was done at the start. After that, we have seen what the workings of the contiguous memory location are and how it is different from the other types of contiguous memory locations. Different functions were also discussed in this regard, and more will be discussed in the next lecture.