The ESP8266 is an extremely robust and versatile microcontroller, which has proven to be a powerful tool in building Internet of Things solutions. What makes the ESP8266 such a popular tool is the perfect integration between a robust 32-bit processor and a fully functional WIFI module that already includes Internet Protocols.
A simple target, but the goal here is to prepare and test the development environment and introduce the programming mode.
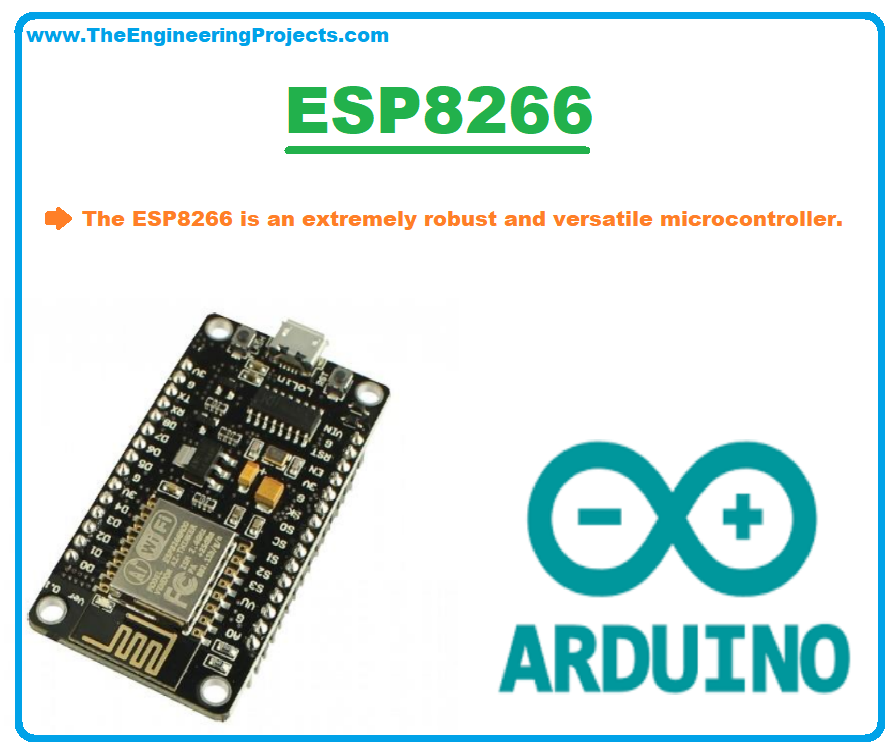
Material
- 1x Computer/notebook with the minimum requirements to install Arduino IDE;
- 1x Mini-USB cable;
- 1x NodeMCU
Arduino IDE Install
- Our first step is to download the Arduino IDE installer. It is available at this URL (https://www.arduino.cc/en/software).
- Figure 2 shows the latest stable version 1.8.15.
- It is recommended that you always have the most current version.
- So, if there’s a newer version when you download it, it’s the right for you. The Arduino community provides constant improvements to the IDE.
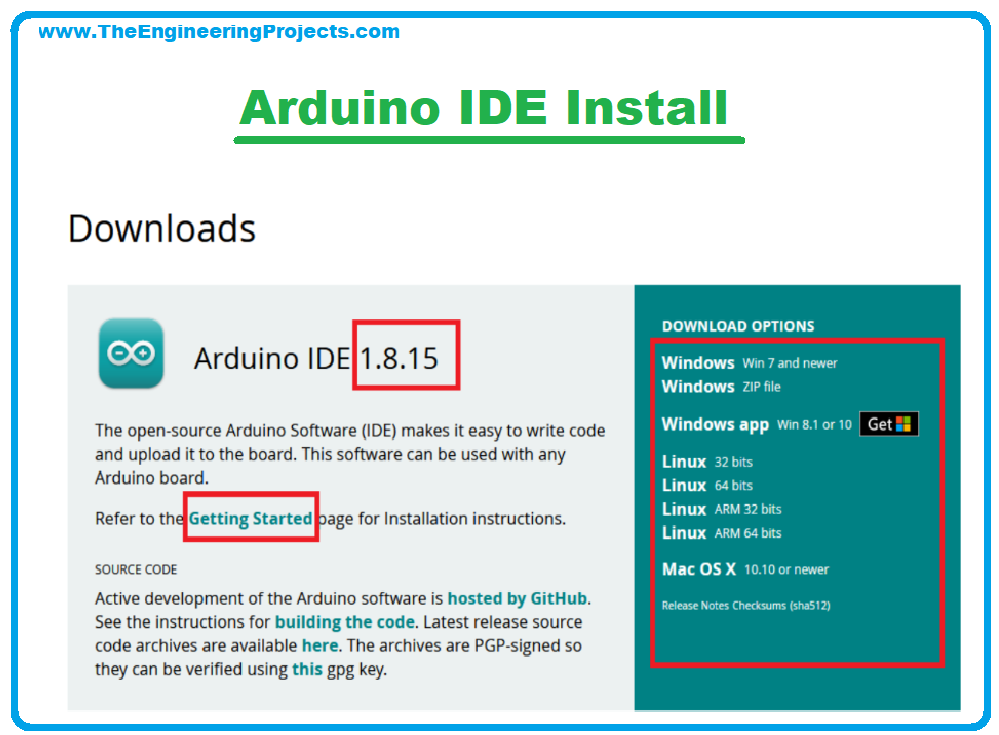
- On the right side, you can find the correct version for each Operating System and download the correct installer.
- We will not go into details here about how to install from the file. The Arduino page itself features an excellent manual on Getting Started.
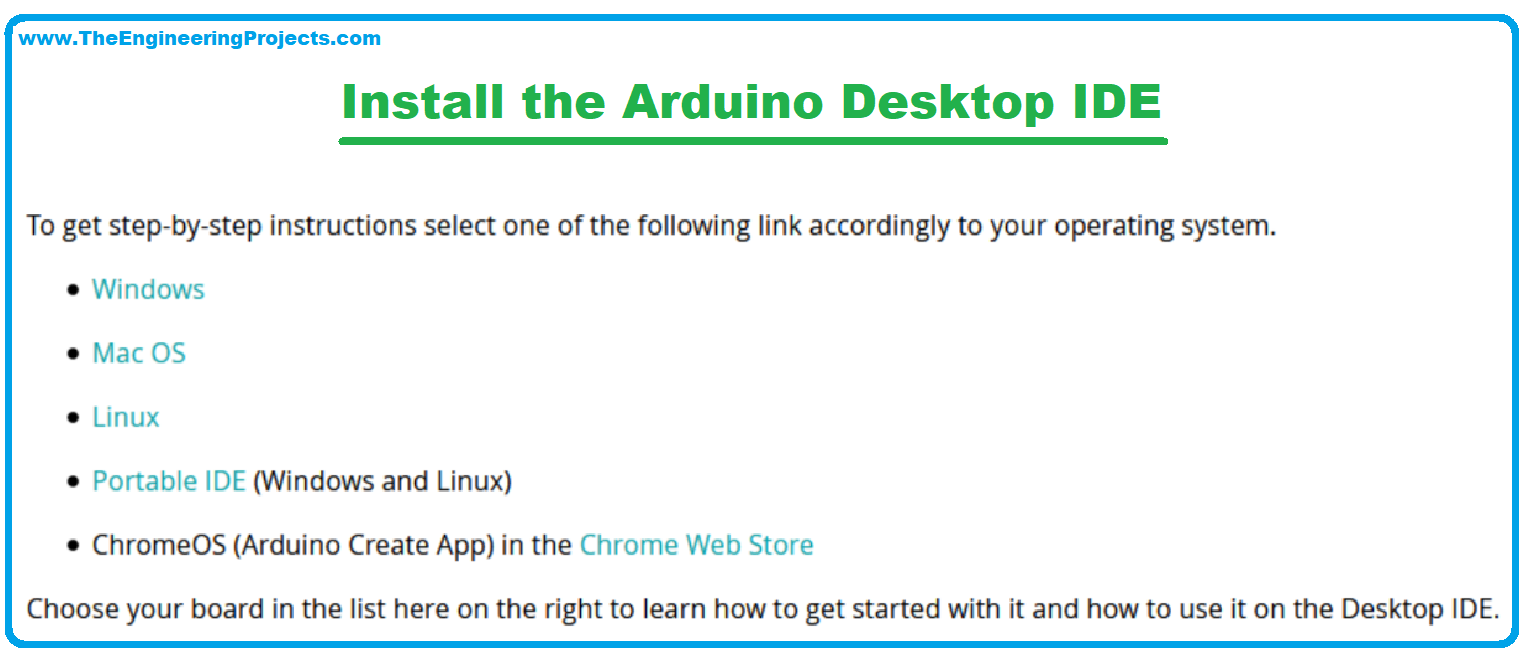
- If this is your first contact with Arduino, I recommend checking out the Learn Arduino section as well.
Arduino IDE Presentation
- So, IDE installed, let's get to know the interface before making some important settings.
- If everything is ok, you should get the following result when running Arduino.
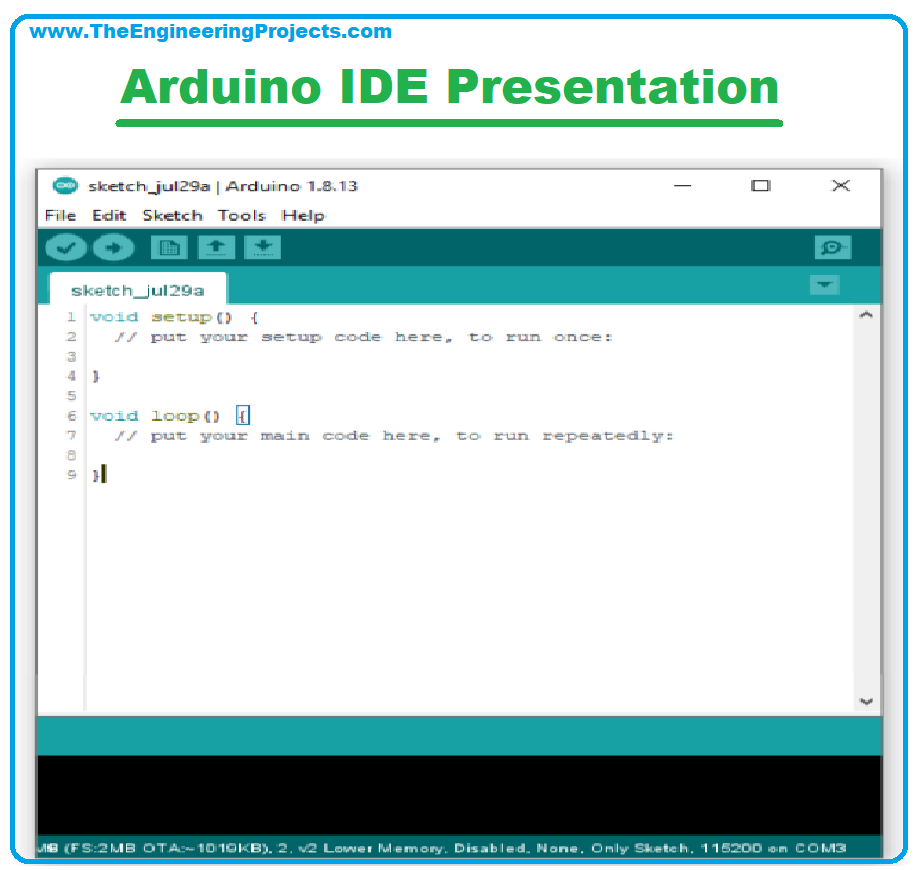
Text Editor
- The first thing that should catch your attention is that the code has already started with two functions.
- The “void setup()” and the “void loop()”.
- Arduino maintains a bootloader in the microcontroller, which is an initiator program responsible for preparing the hardware for correct operation.
- When we turn the module on power or press the reset button, the bootloader is the first program to run.
1– What do I do first?
Answer: What does the “setup()” function say to do.
2– What do I do after “setup()”?
Answer: Repeatedly execute whatever the “loop()” function tells you to do.
- By default, we use the “setup()” function to inform whether a pin will operate as input or output, set the initial state of each pin used, set serial port Baud Rate, initialize additional configuration functions.
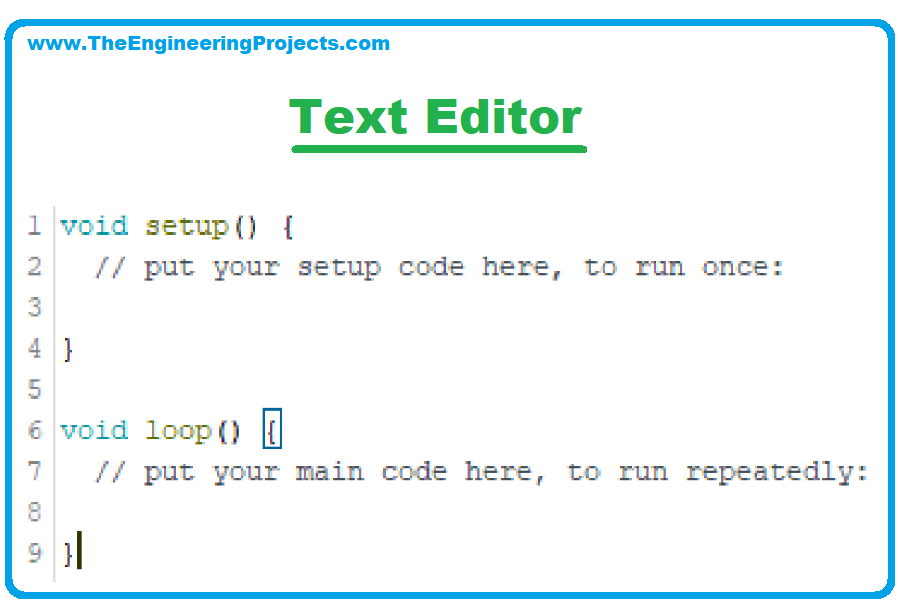
Verify and Upload Buttons
- Once our code is done, we need to check if everything is right and write the code in the microcontroller.
- The check button does a code check to identify syntax or library integration errors. Attention: logic errors are not checked; these are up to the programmer.
- The Upload button also performs the verification and then sends the code to the microcontroller via the Serial port.
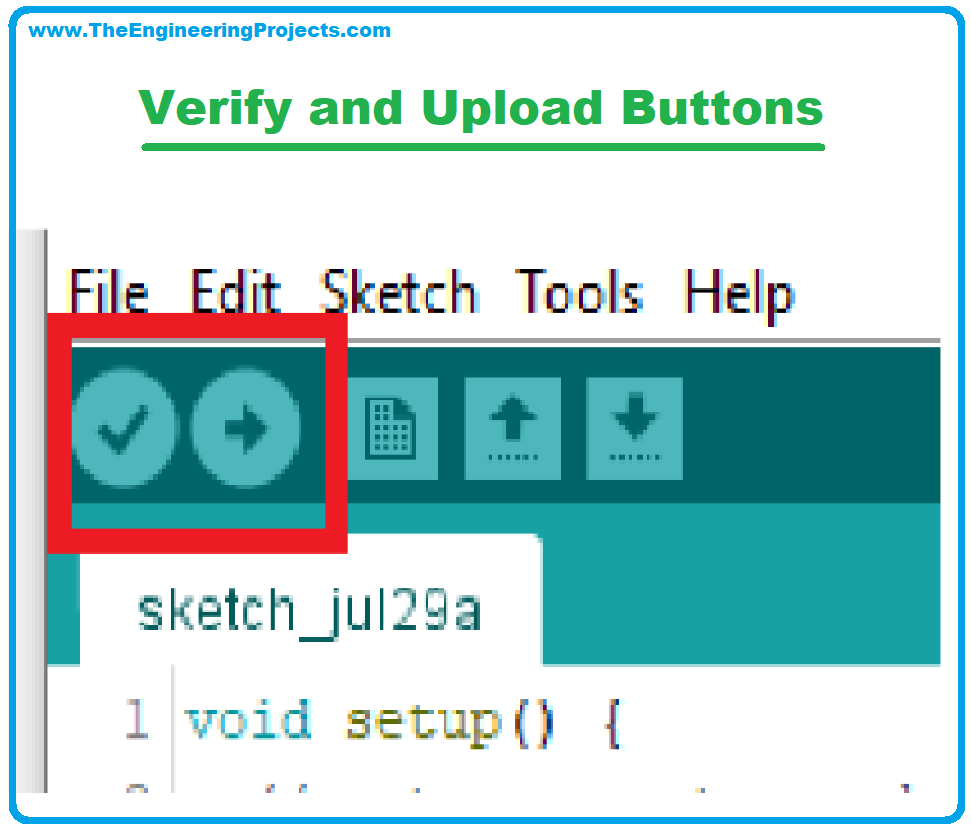
Feedback Interface
- Perhaps the most important snippet of the IDE, here it reports the status of testing and recording. This is where you see if everything is ok.
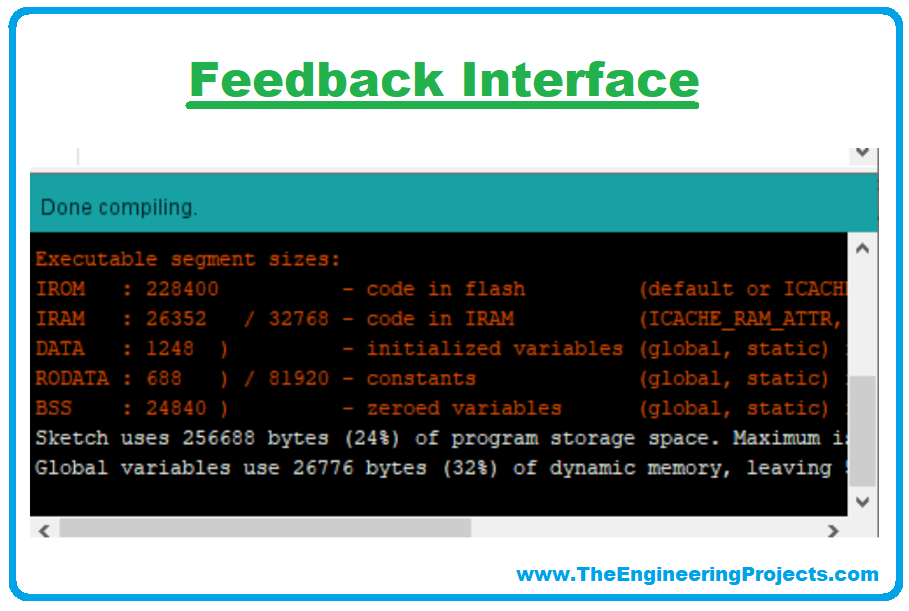
Serial Monitor
- The Arduino IDE has a tool called Serial Monitor that allows direct communication with the microcontroller from the Serial port.
- It is possible to follow what is being sent by the module and send commands directly to it.
Two points of attention
- The Communication Port (in the example COM3) must be selected in the Tools
- The Baud Rate (in this case, 115200) must be entered correctly, otherwise, the data may become illegible or simply not appear.
- Baud Rate can be set in the “setup()” function when preparing the recording
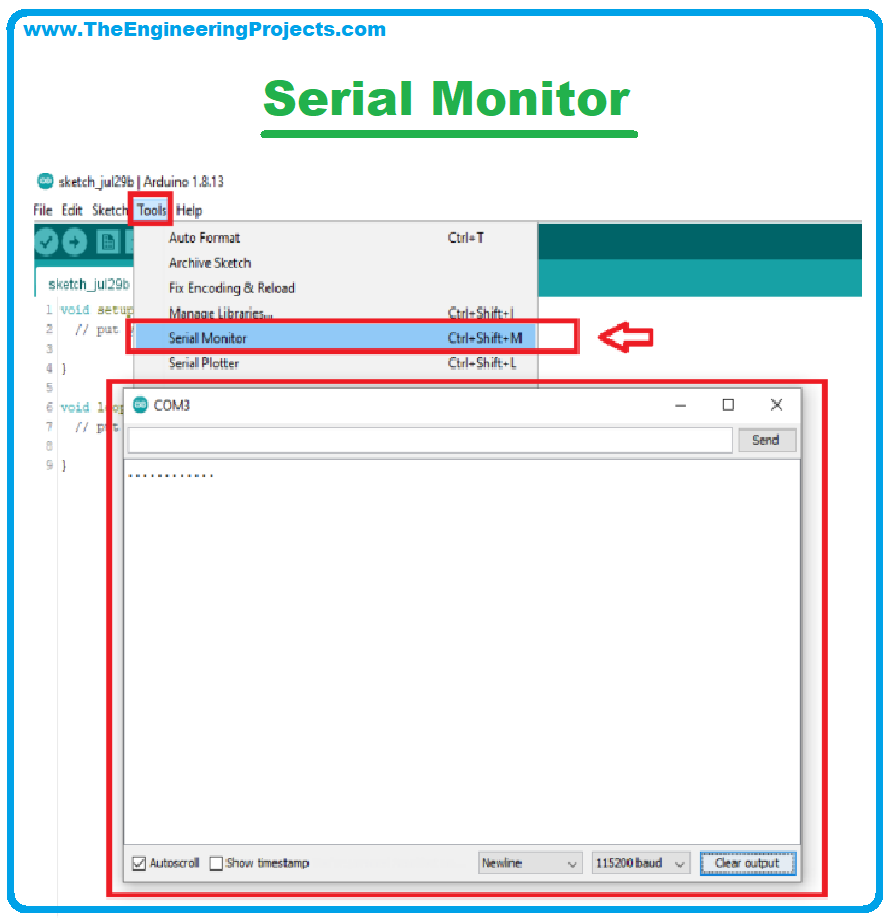
Boards Manager
- Each Arduino module has its peculiarities. Amount of memory, available pins, etc.
- Just as important as making a well-built code is telling the IDE which card model will be used.
- IDE Arduino already brings in its basic installation, the installation of boards that use ATMEGA microcontrollers.
- If you are going to use another type of board, it needs to be installed in Boards Manager (tools -> Boards Manager...).
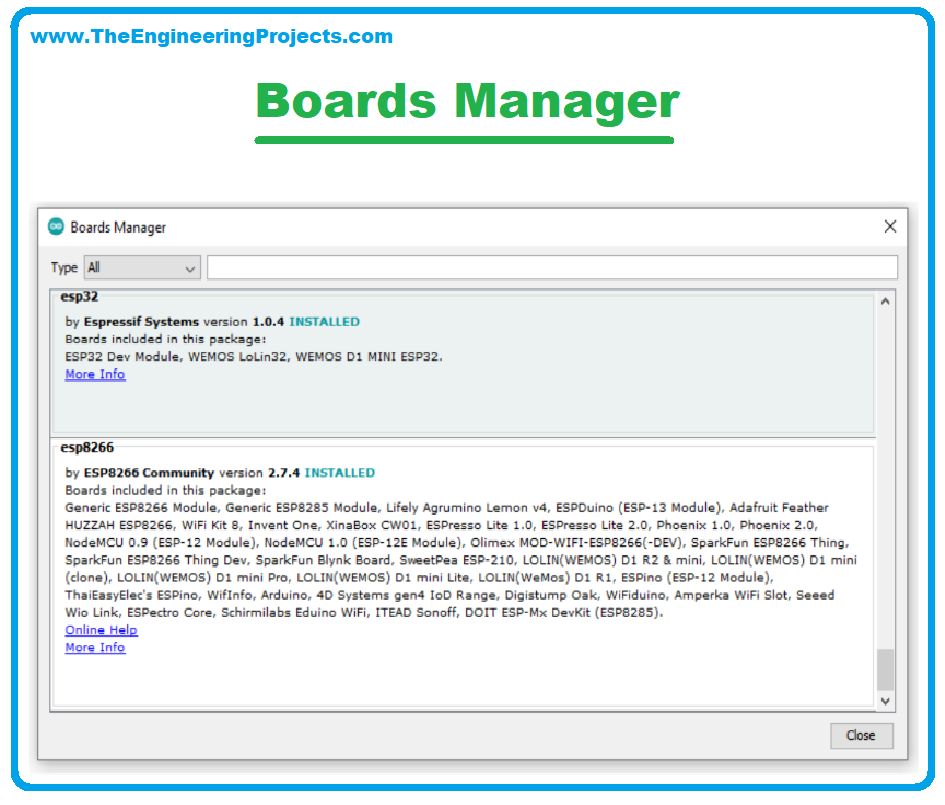
- Once installed, the board will be available and must be selected from the Tools -> Board menu.
Attention point
- Until Arduino version 1.8.15, modules using the ESP8266 do not appear available in Boards Manager.
- This is because it is not yet included in the official Arduino repository.
- For it to appear, it is necessary to add the path of a new repository.
- Do this by going to the File -> preferences menu and add http://arduino.esp8266.com/stable/package_esp8266com_index.json in the Additional Boards Manager URLs field.
- Press OK, close Arduino, and open it again.
- In Boards Manager the ESP8266 option will be available. Click install.
Once this step is completed, the main modules using the ESP8266 will be available. For our application, we will use nodeMCU 1.0.
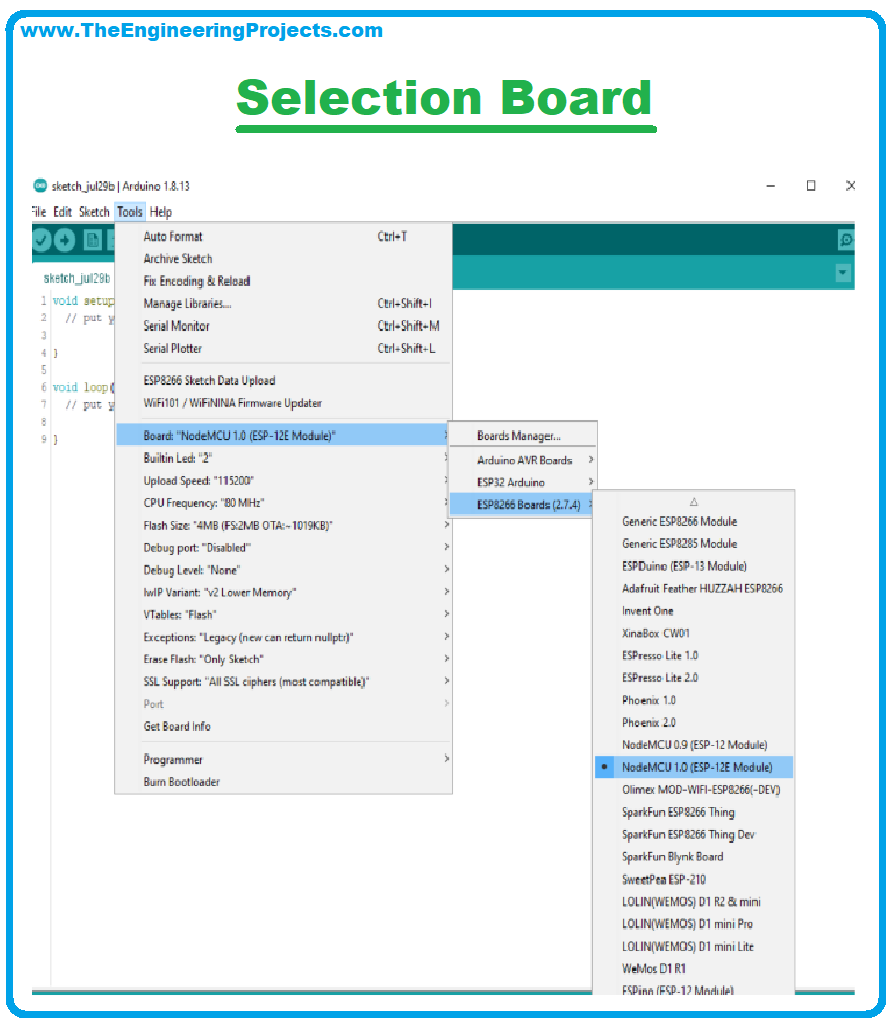
Coding NodeMCU with Arduino IDE
- After choosing the board, choosing the port (note, the port will only appear when you connect the board to the USB), it's time to make our first code.
- The nodeMCU Module has a built-in LED and we will make it blink every 1 second.
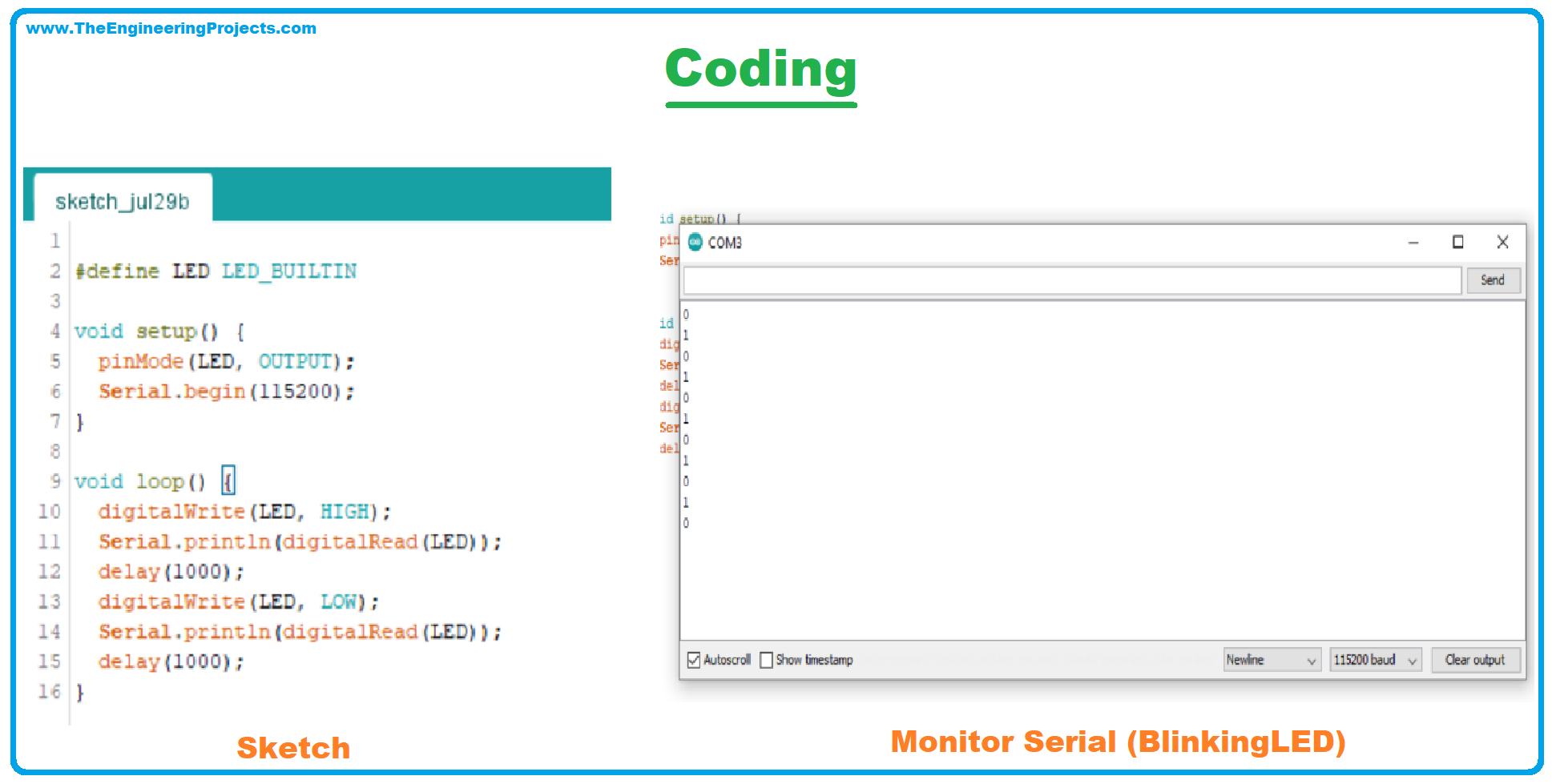
- For this sketch, we don't need to include any additional libraries.
- When we inform Arduino that we are using the NodeMCU board, it already knows who the LED_BUILTIN is and which pin it is connected to.
- We use #define LED LED_BUILTIN just to give a more practical name to our LED, it could be used to name an external pin, for example, #define LED 13, alternatively, the original name could be used in the code.
- pinMode(LED, OUTPUT) and pinMode(13, OUTPUT) would have the same effect.
- The pinMode(LED, OUTPUT), by the way, is used to inform that this pin will operate as output. Note that this configuration was done inside the setup() function, so the pin will be prepared before being used in another function.
- Serial.begin(115200) initializes communication with the serial port at baud rate 115200. In our Loop function we will follow the sequence:
- Turn off the LED, write the pin status to the serial, wait for 1 second, turn the LED on, write the pin reading to the serial, wait for 1 second and start all over again.
- Here's the video demonstration of LED Blinking with NodeMCU: