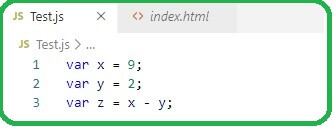
What are Variables In JavaScript
Variable means anything that can vary(change). In JavaScript, Variable holds the data value, in simple words, JavaScript variables are containers and can store the data value. Variable in JavaScript are declared with var (reserved keyword).- It is the basic unit for holding or storing the data.
- A variable value can change at any time.
- Variable must have a unique name.
var (Variable-Name) = value;
Variable declaration
- var number = 5; // variable store numeric value
- var string = "Hello" // variable store string value
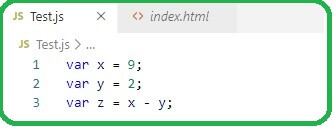
- x store the value of 9
- y store the value of 2
- z store the value of 7
- JavaScript is a dynamically typed language. In simple words, Its data types are converted automatically as needed during script execution.
- For example in c# language, we have to create an integer variable to use for numerics.
int X = 5;
- To create a string variable we use string keywords
String str = "Learning"But in JavaScript, Variable will be automatically detected and you don't have to define their data type that if they are integer or string etc.
var myVarname = 10; alert(myVarname); myVarname = "This is a string value"; alert(myVarname)Look in this above example, in the beginning, myVarname variable is treated as int numeric type and with alert. Following that, we have worked on myVarname again as a string and showed it. May you have noticed that we have not defined their data types.
How to Declare A Variable in JavaScript
Storing the value of a variable is called variable initialization. You can store the value of the variable at the time of variable creation(in other word declare) or later when you need to call that variable. Example:var name = "Justin"; var salary; salary = 10,000;You can create a variable or declare the value of the variable later as you can see in the example. Although you can also do variable initialization too. You can declare multiple variables with the single var keyword without storing the value.
var age, height, class, name;
How you can declare a variable in JavaScript with examples
// initializing variable var x = 5; var name = "Adam";
// declaring single variable var name; // declaring multiple variables var age, height, class, name;
Types of Variables
These are two types of JavaScript variables.- Local Variables - Local variable will be visible in the only function or block where you have defined it. Function parameters are local to that function and can't define anywhere in the JavaScript.
- Global Variables - Global variables are variables that will be only visible in a function where it is defined and can be defined anywhere in the javascript.
Things That You Should Keep In Mind
- Variables can only store a single value of data that can change later.
- All the variables can only be defined using a var keyword. Variables declared without var keywords becomes global variables.
- Multiple variables can be declared in a single line like var age = 10; name = "ali"; class = 3;
JavaScript Reserved Word
These are all the reserved words of JavaScript. All of them can not use as JavaScript Variable, function, loop labels, method or any other objects name.
JavaScript Reserved Word |
||||
abstract |
throws | catch | var | implements |
Else | native | final | protected | delete |
instanceof | false | new | function | volatile |
switch | case | transient | continue | return |
boolean | throw | char | typeof | if |
enum | long | finally | private | default |
int | extends | null | for | void |
synchronized | byte | true | const | public |
break | this | class | try | goto |
export | interface | float | package | debugger |
goto | public | void | default | if |
return | volatile | delete | implements | short |
while | do | import | static | with |
double | in | super |