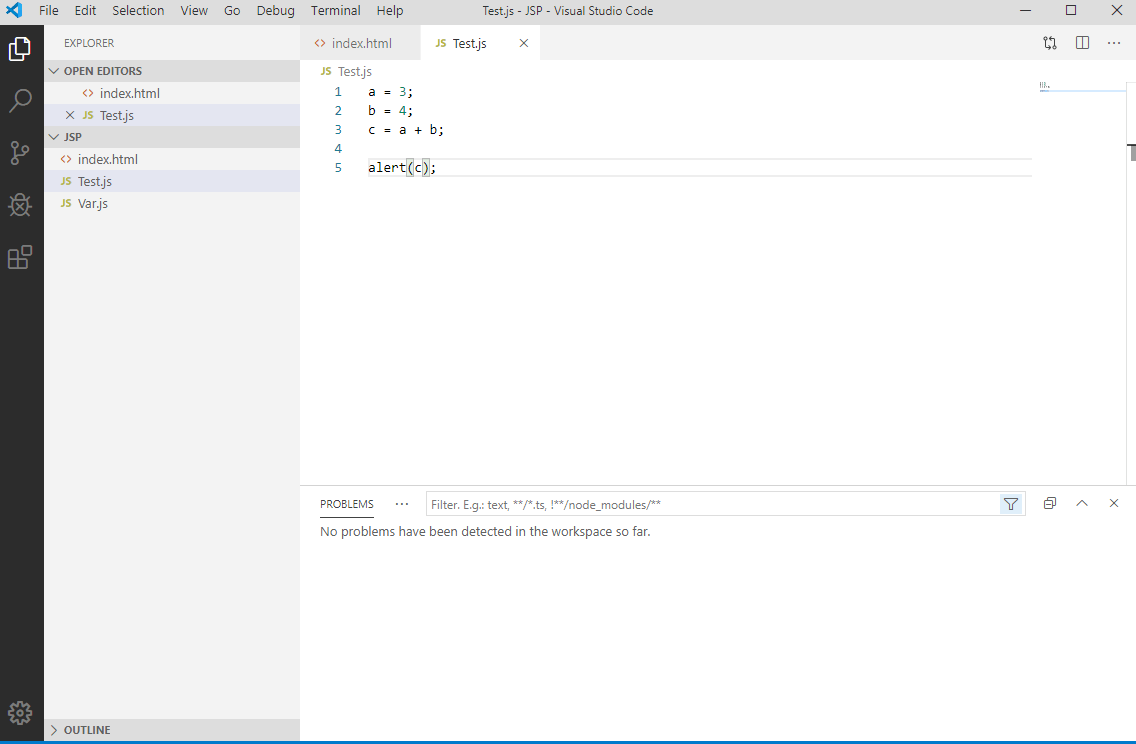
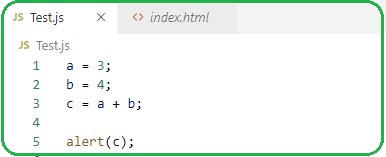
- Arithmetic operator
- Assignment operator
Arithmetic Operators in JavaScript
To perform an action between two variable or values we use mathematical operators. In the equation 3+2 = 5 (+ is a syntax). Java script has many different operators some are basic math operator and others are additionals operators particular to programming. A table of JavaScript OperatorsOperator | Description | Example | Definition |
+ | Addition | 2 + 3 | Sum of 2 and 3 |
- | Subtraction | 9 - 2 | Difference of 9 and 2 |
* | Multiplication | 3 * 2 | Product of 3 and 2 |
/ | Division | 10 / 5 | Division of 10 and 5 |
% | Modulo | 9 % 2 | Remainder of 9 and 2 |
** | Exponentiation | 9 ** 3 | 9 to the 3 power |
++ | Increment | 9++ | 9 plus one |
-- | Decrement | 9-- | 9 minus one |
Addition and Subtraction in JavaScript
Addition and subtractions are the operators from basic maths. They are used to find the sum and difference of two numerical values. JavsScript has its own built-in calculator. All these mathematical operators can be directly performed in the console. As you have seen a simple example of addition and now I am going to explain it with detail. Now I will assign numerical values to a and b and place the sum in c.- Assign the value to a and b
- Suppose: a = 3; b = 4;
- Now add a + b and assign the sum of a+b to c
- c = a + b; alert(c);
Output
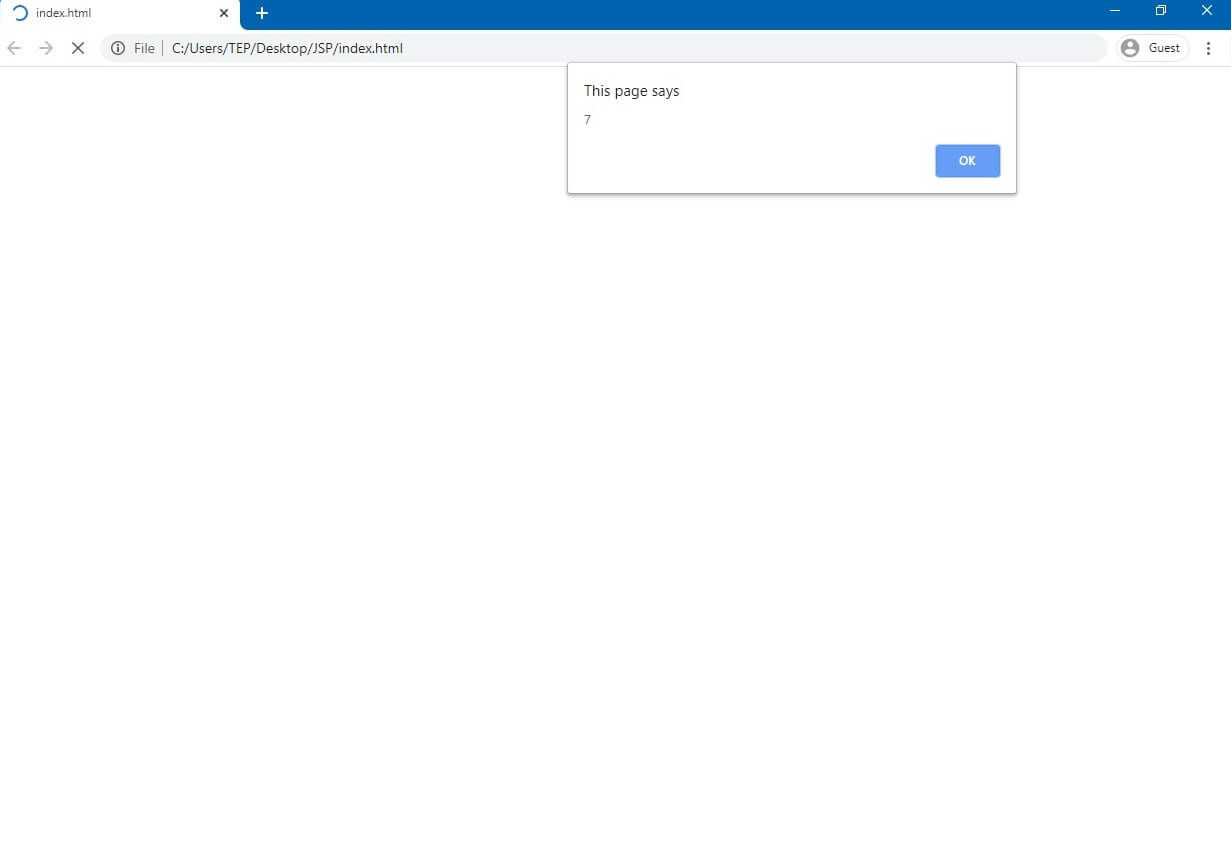
- Assign the value to a and b
- Suppose: a = 6; b = 3;
- Now subtract a - b and assign the diffrence of a-b to c
- c = a - b; alert(c);
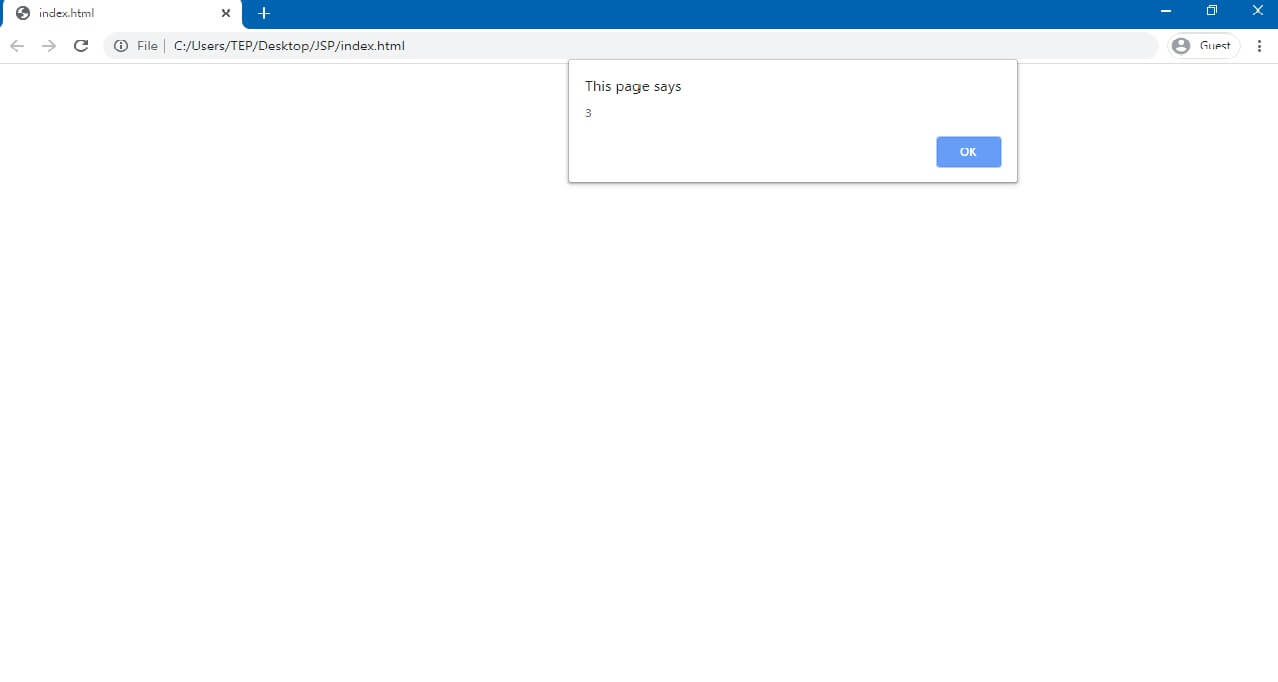
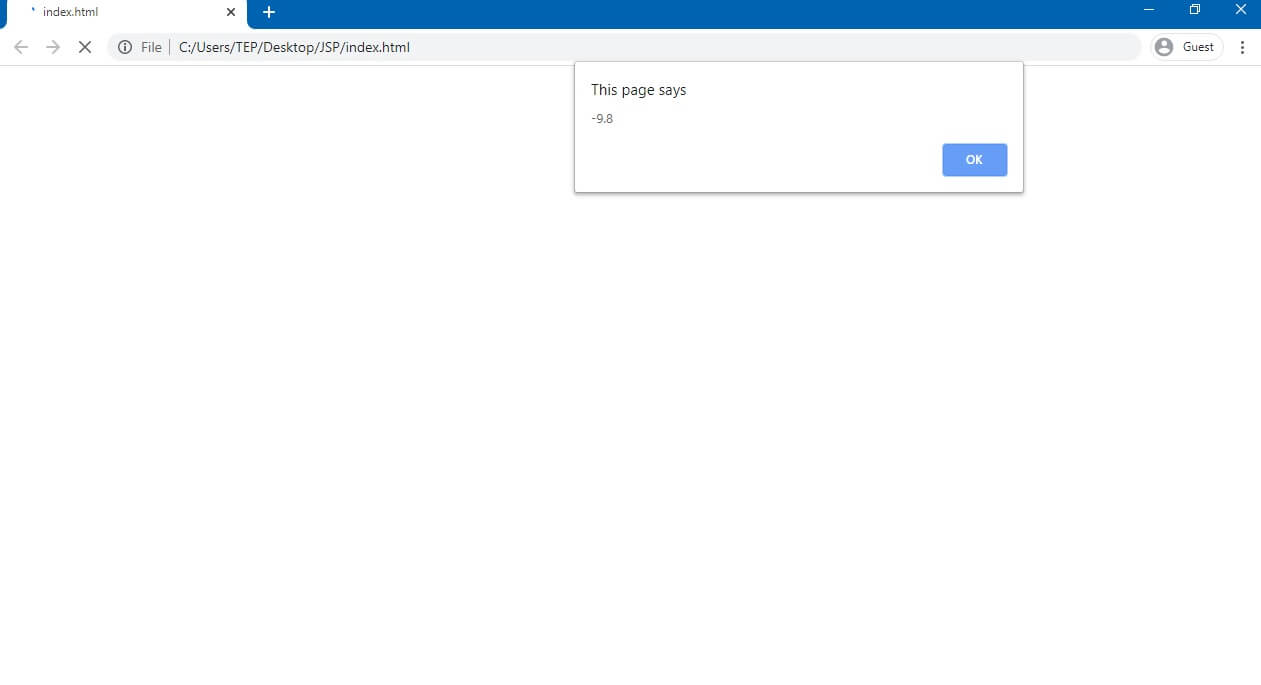
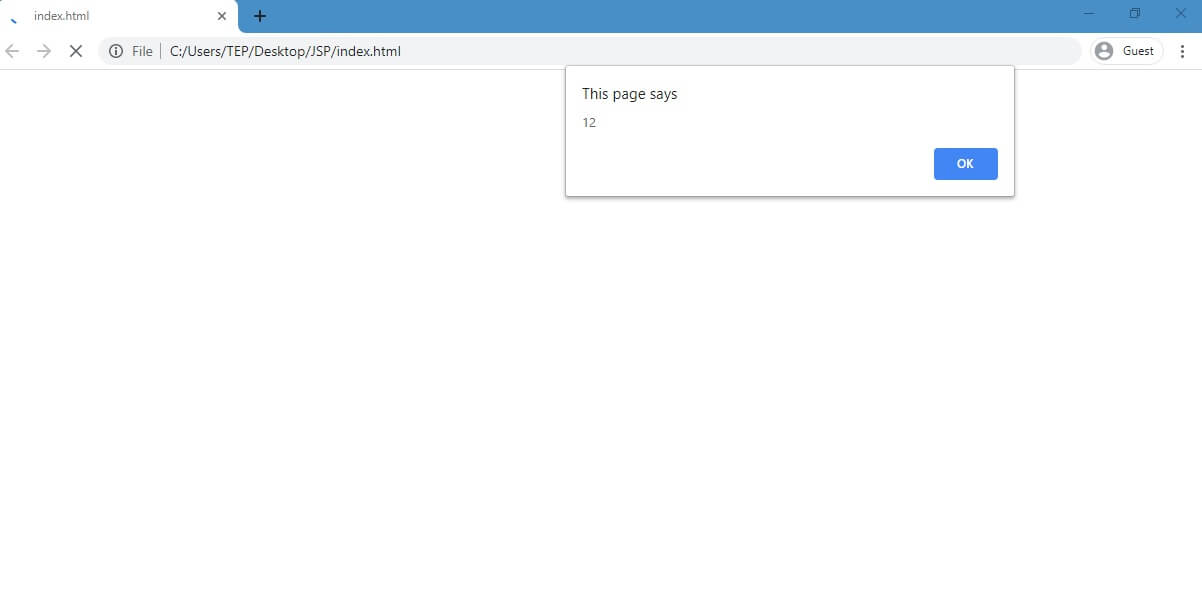
Multiplication in JavaScript
Multiplication (*) and division (/) are also used in Javascript to find the numeric values by division or multiplication. Multiplication could be used many times like calculating the price of any item etc and division help you in finding the time like the number of hours or minutes. Output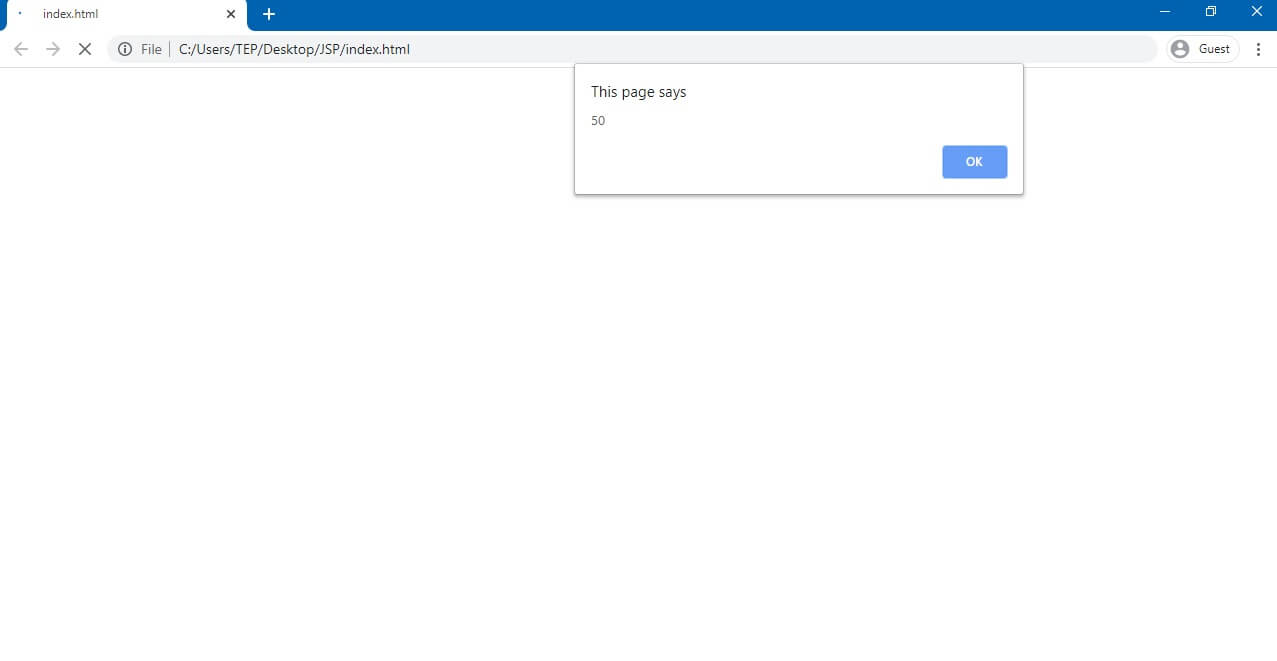
Divison Operator in JavaScript
Output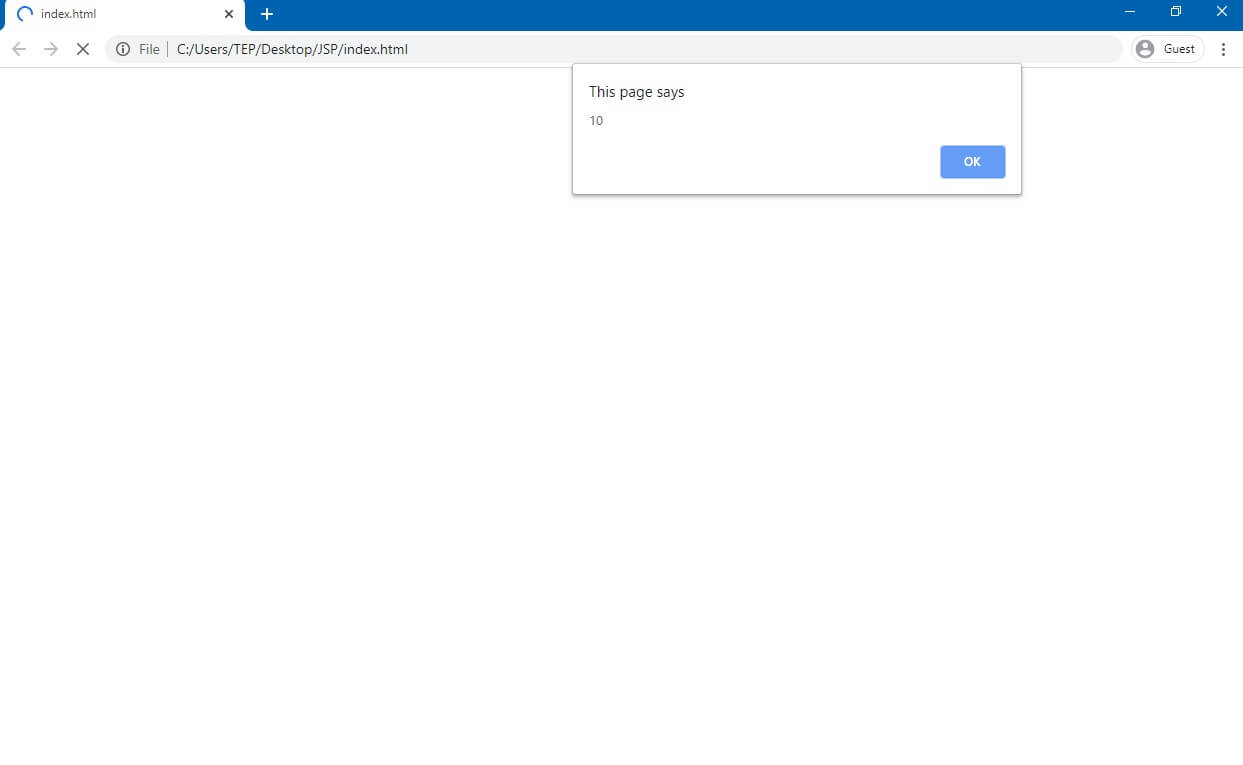
Modulus in JavaScript
Modulo (%) is used to find the remainder of a quotient after division. It can help us find in that even if the number is even or odd. It is less familiar operator than others. It is also known as Modulus too. Output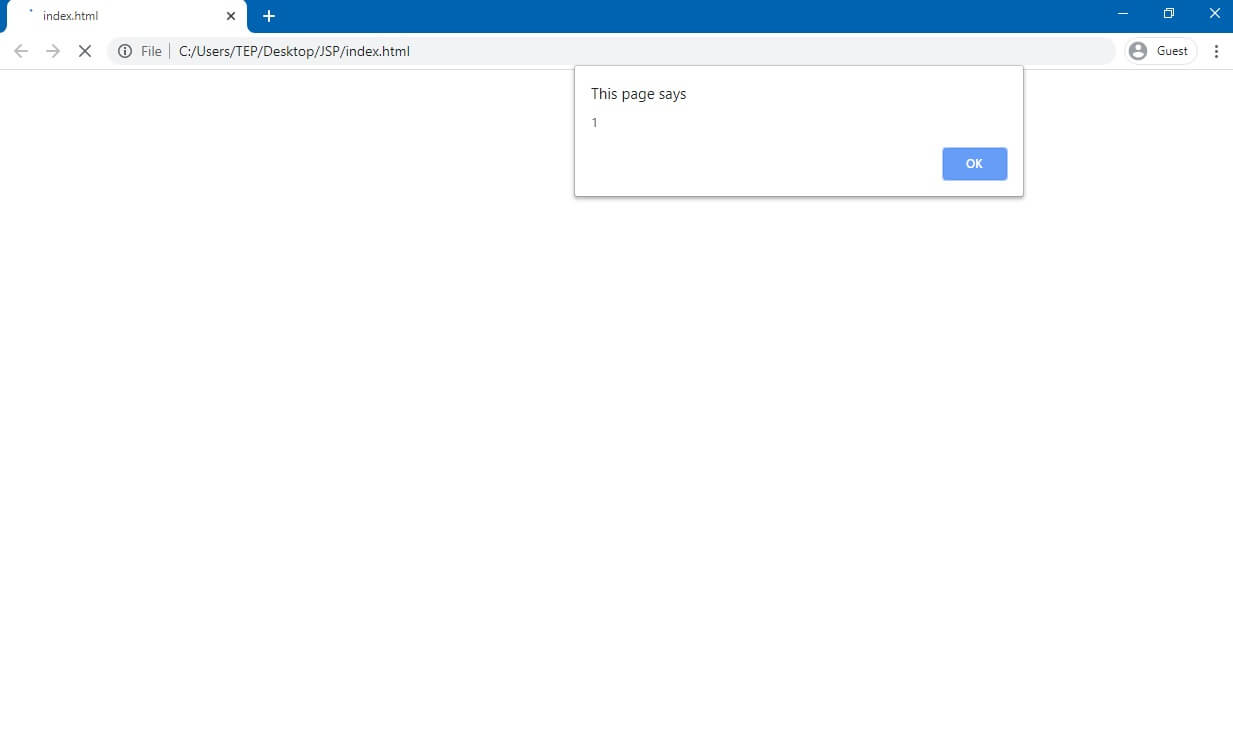
Exponentiation in JavaScript
Exponentiation (**) is the new operator in JavaScript. Its calculate the power of a number by its exponent. Its written like this 8^4 (Eight to the 4 power).
Output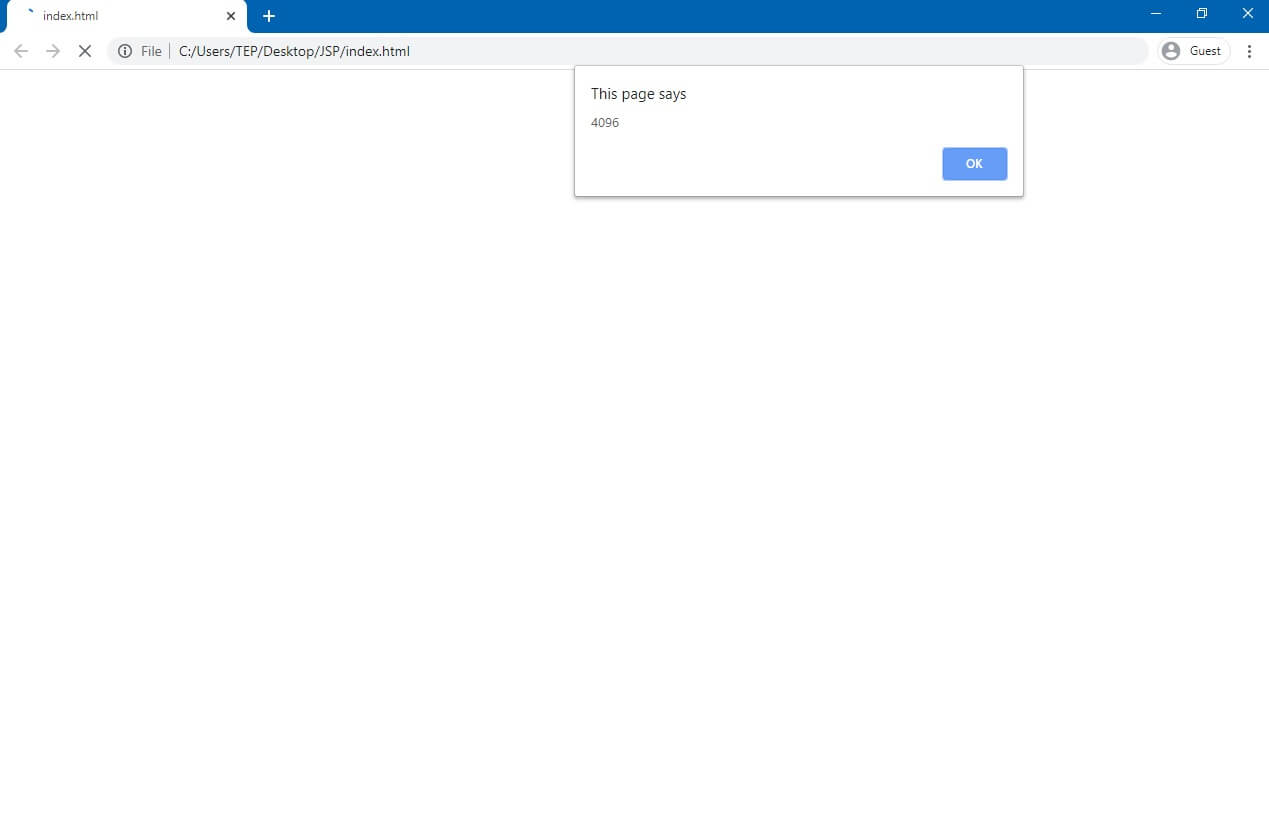
Increment and Decrement in JavaScript
The increment operator (++) increase the numerical value of a variable by one and on the other side decrement operator (--) decrease the numerical value of a variable by one. They are often used with loops. You can use these operators with variable only and using them with raw numbers can only result in an error. Look in the example.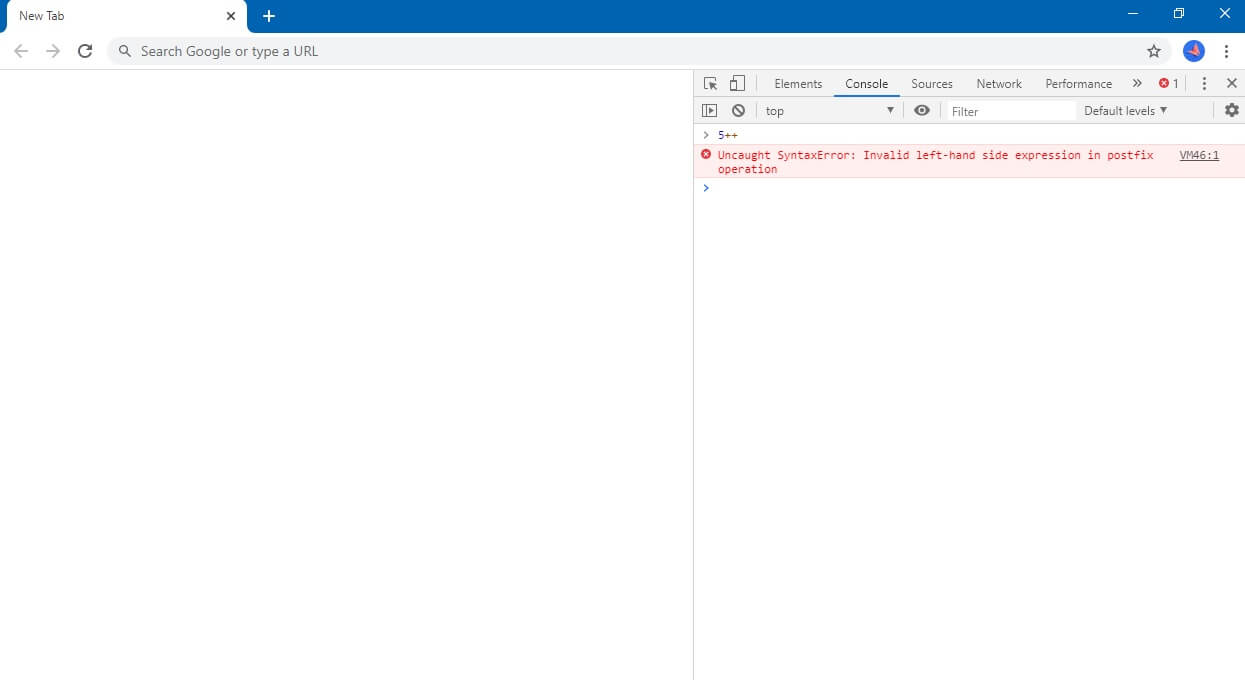
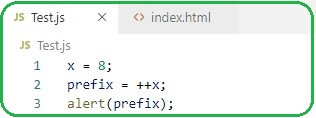
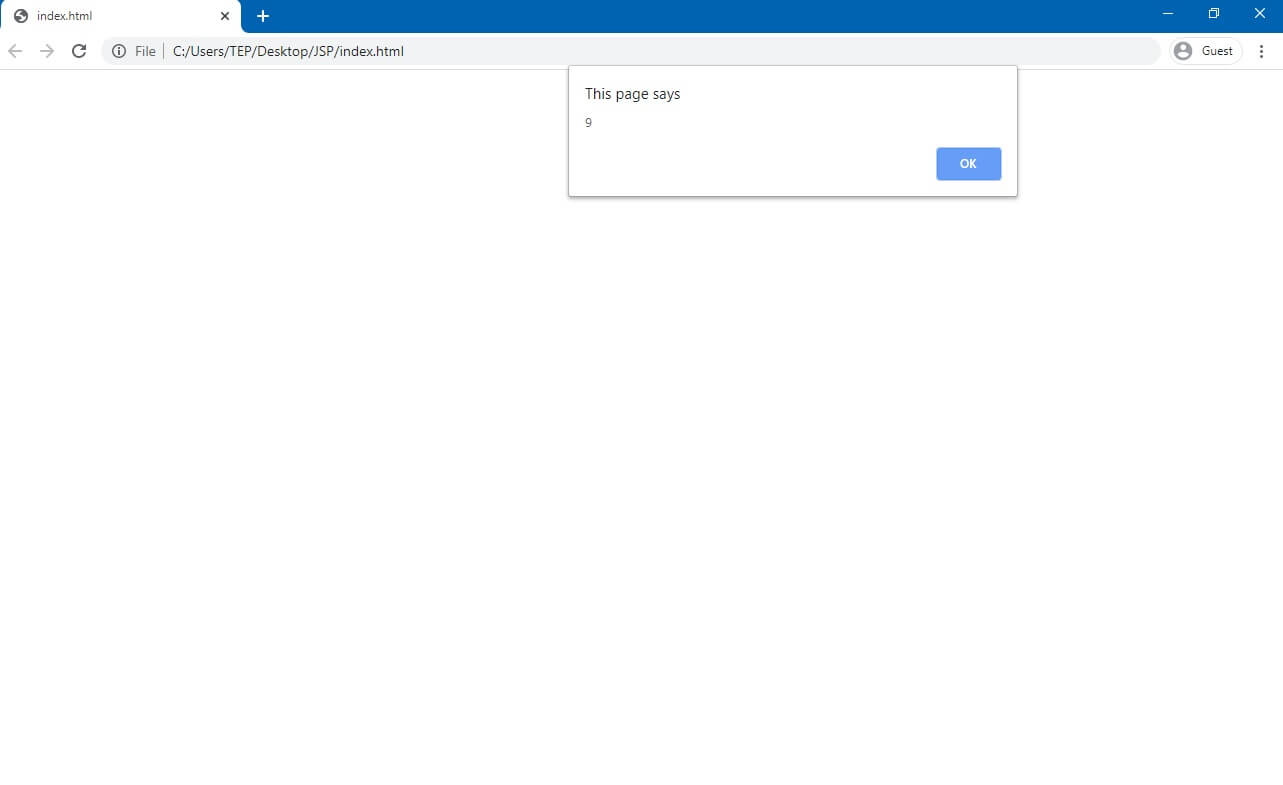
Postfix Operator in JavaScript
let x = 8;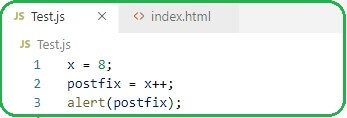
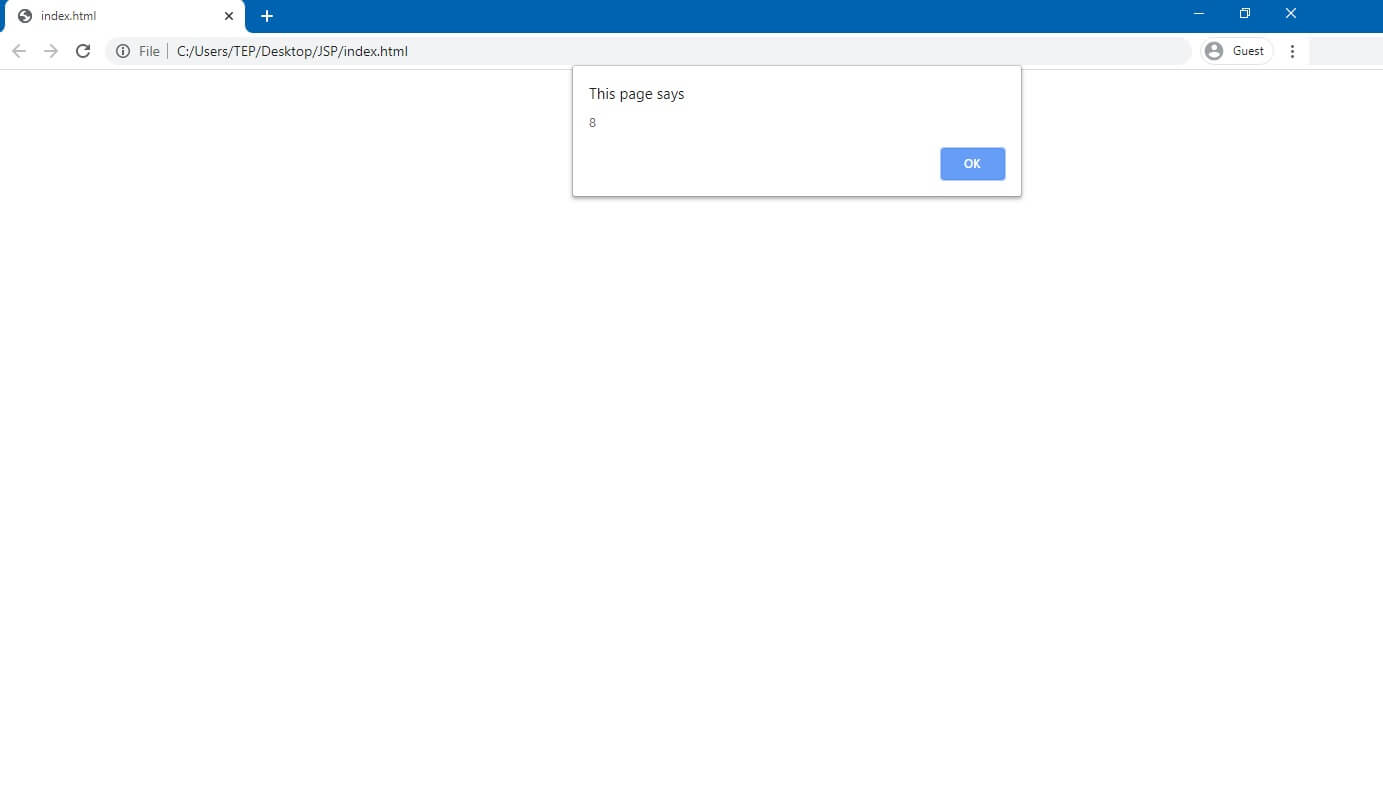
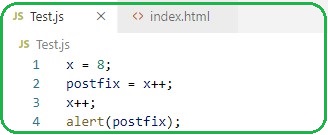
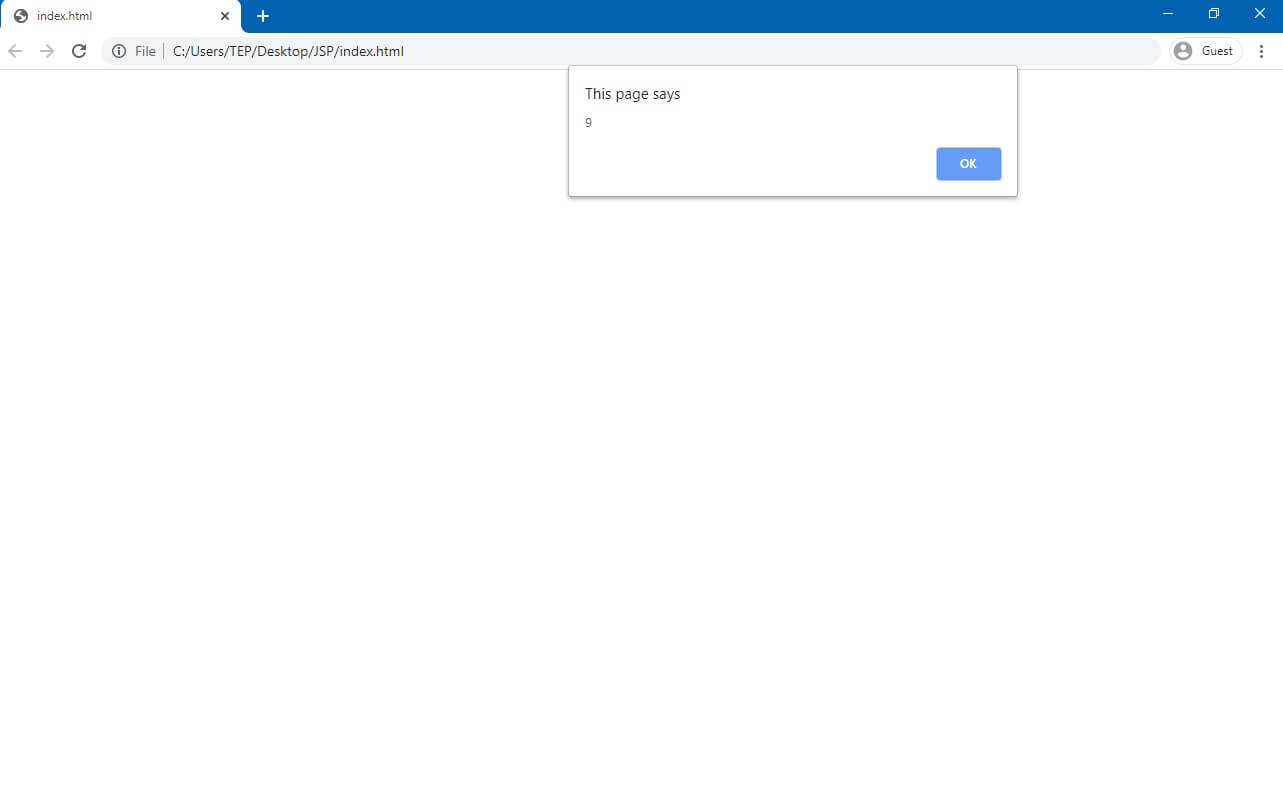
Assignment Operators in JavaScript
The assignment operator (=) is the most used operator, which we have used already. We used the assignment operator to assign the value to the variable.//Assign 50$ to price variable Price = 50$JavaScript also contains compound assignment operators. Let's discuss a compound assignment operator example.
//Assign 50$ to price variable Price = 50$ Price += 5$; alert(price);The output of this result will be 55$. In this case, price += 5$ is same as price = price + 5$. The arithmetic operator can be combined with the assignment operator to make a compound assignment operator. Down below is a table of the assignment operator.
Description | Operator |
Assignment | = |
Addition assignment | += |
Subtraction assignment | -= |
Multiplication assignment | *= |
Division assignment | /= |
Exponentiation assignment | **= |
Remainder assignment | %= |