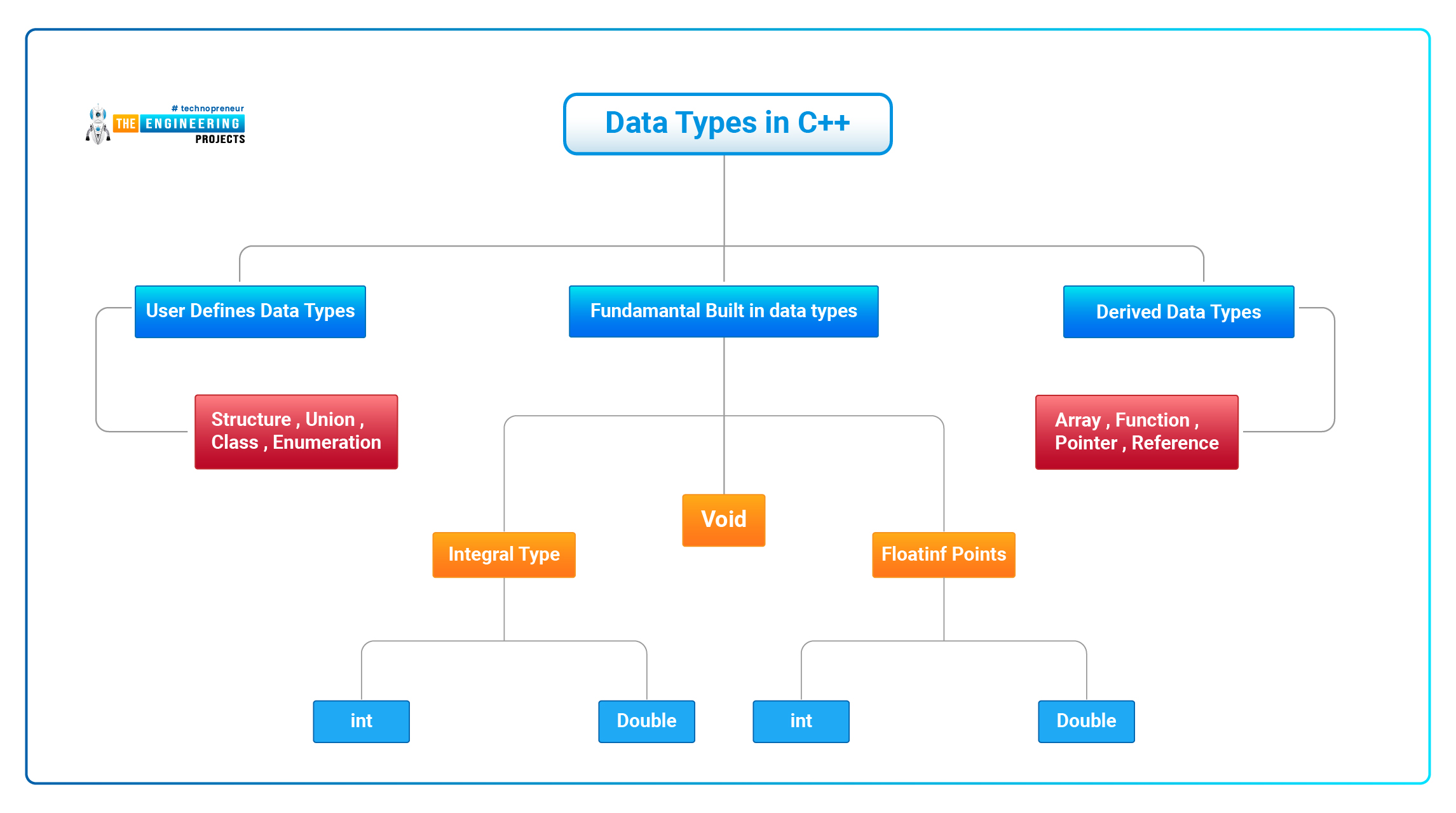
Hello friends, I hope you all are doing great. In today's tutorial, I am going to give you a detailed introduction to the C++ Programming language. In cross-platform programming languages, C++ is the most popular that can be used to work on low and high-level applications. Bjarne Stroustrup was the founder of C++. He modified C language to develop C++ language. Control over system resources and memory can be attained by using C++. In 2011, 2014, and 2017 it was modified to C++11, C++14, and C++17. C++ is a middle-level language. It is advantageous to both programming languages low-level (drivers, kernels) and higher-level applications (games, GUI, desktop apps etc.).
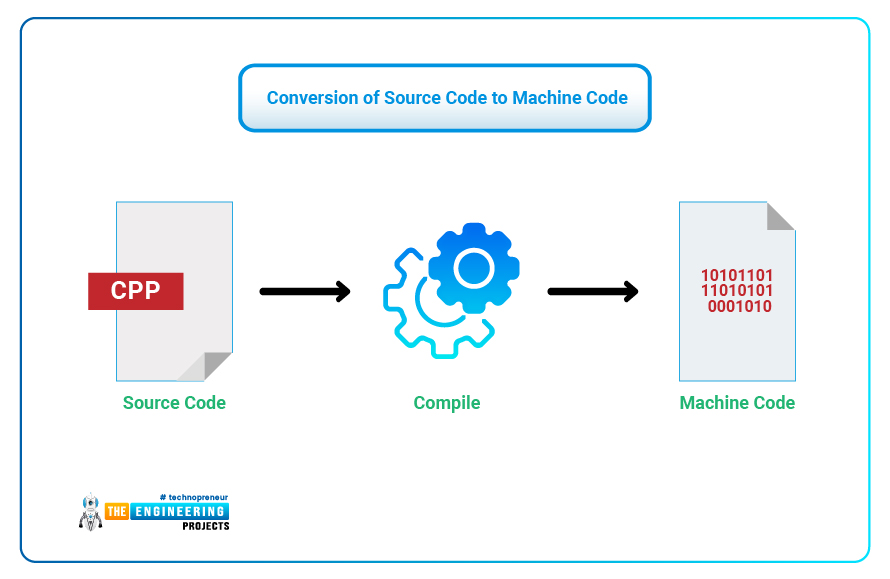
Uses of C++
C++ is one of the world's most famous programming languages. It is used in today's OS, embedded systems and GUIs. It provides a clear structure to programs, permits codes to be reused and lowering development costs as its an object-oriented language. Since it is portable and can be used to create applications that can be used on multiple platforms. It is very easy to learn. As it is close to C# and Java, so switching to C++ or vice versa is very simple.
It is used in
- Operating systems e.g. Linux-based OS
- Browsers like UC browser, chrome, opera and firefox.
- Games and graphics e.g. Photoshop
- Clouds like Dropbox
- Database engines like reedit
Features of C++
Rich library support:
- It is a simple language as programs can be split into logical units and parts. It has rich library support and many data types.
Platform Dependent and Machine Independent:
- It is machine-independent but platform-dependent. It does not run on windows but is executable on Linux.
Middle-level language:
- It is a middle-level language as we can do both low-level programmings(drivers, kernels, networking etc.) and build large-scale user applications (Media Players, Photoshop, Game Engines etc.) as a high-level language.
3rd party libraries:
- C++ has rich library support as well as 3rd party libraries (e.g. Boost libraries) for easy, smart and rapid development.
Fast execution:
- For C++ speed of execution is very fast because it is compiled and highly procedural language.
- Garbage-collection, dynamic typing etc. slow the execution of the program overall. Since there is no additional processing in C++ so it is fast than others.
Provides direct Memory-Access:
- It provides pointer support to manipulate storage addresses. This helps in low-level programming ( indirect control over memory addresses).
Object-Oriented language:
- It is better than C with respect to object orientation that helps it to maintain extensible programs so large-scale applications can be built easily.
- Its friends and virtual features violate some important rules rendering it a completely object-oriented language.
Amazing facts of C++:
Some interesting facts about C++ are listed below
- C++ name tells us that C language modified with ++ incremental operator is C++ language
- The most famous language C ++ is used in commercial software.
- Four primary features of OOP are supported by C++
- Inheritance
- Encapsulation
- Abstraction, and
- Polymorphism
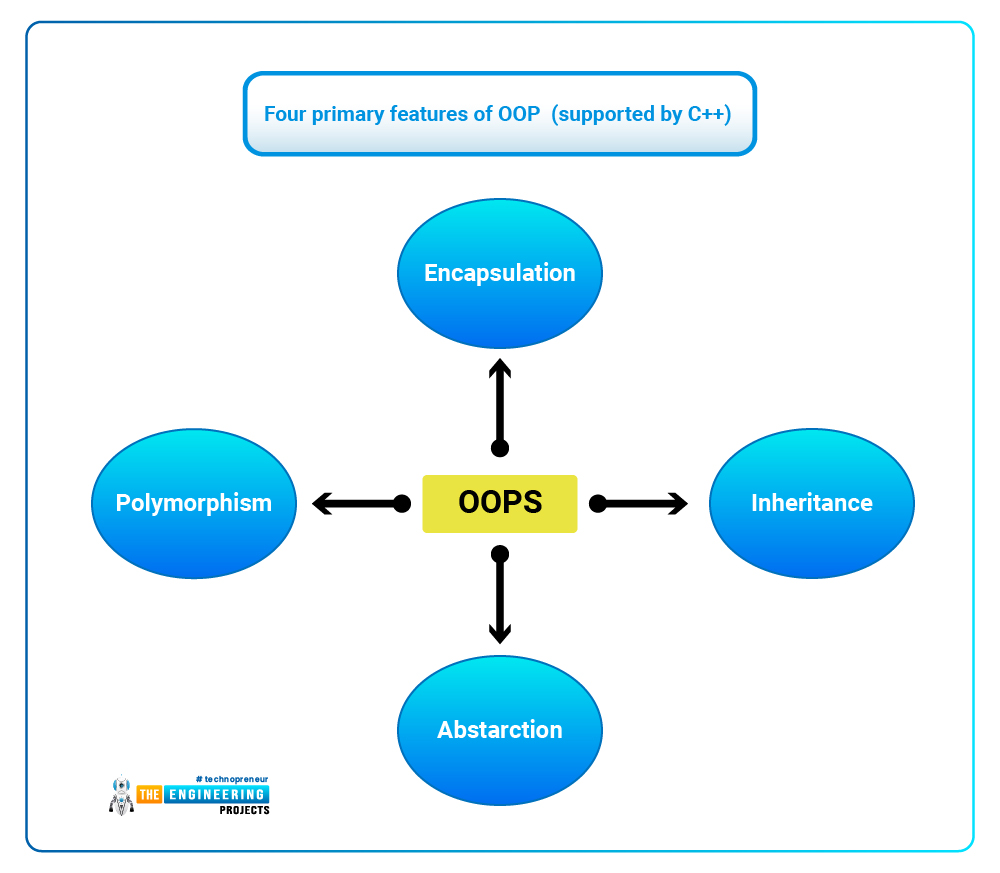
- From Simula67 Programming language C++ gained the features of OOP.
- For a C++ program to execute(at least main() function) , a function is the least requirement.
Basic concepts of C++ :
Basic concepts like syntax, variables, loop type etc will be discussed here.
Syntax of C++:
Here is the C++ basic program
#include <iostream.h> using namespace std; int main() { cout << "Hi this is C++"; }
- iostream is a header file and provides us with input & output streams.
- namesspace std tells the compiler to use standard namespace. It can be used in 2 ways.
- the return type of main () is int.
- count << is used to print anything on the screen.
Comments in C++:
- // is used to add single comment. For multiple comments /*multiple comments*/ is used
Data types in C++:
There are built-in as well as user-defined data types in C++.
- In C++, classes are user-defined data types.
- Built-in data types are int, float, double etc.
- Derived data types are Array, function, pointer and reference.
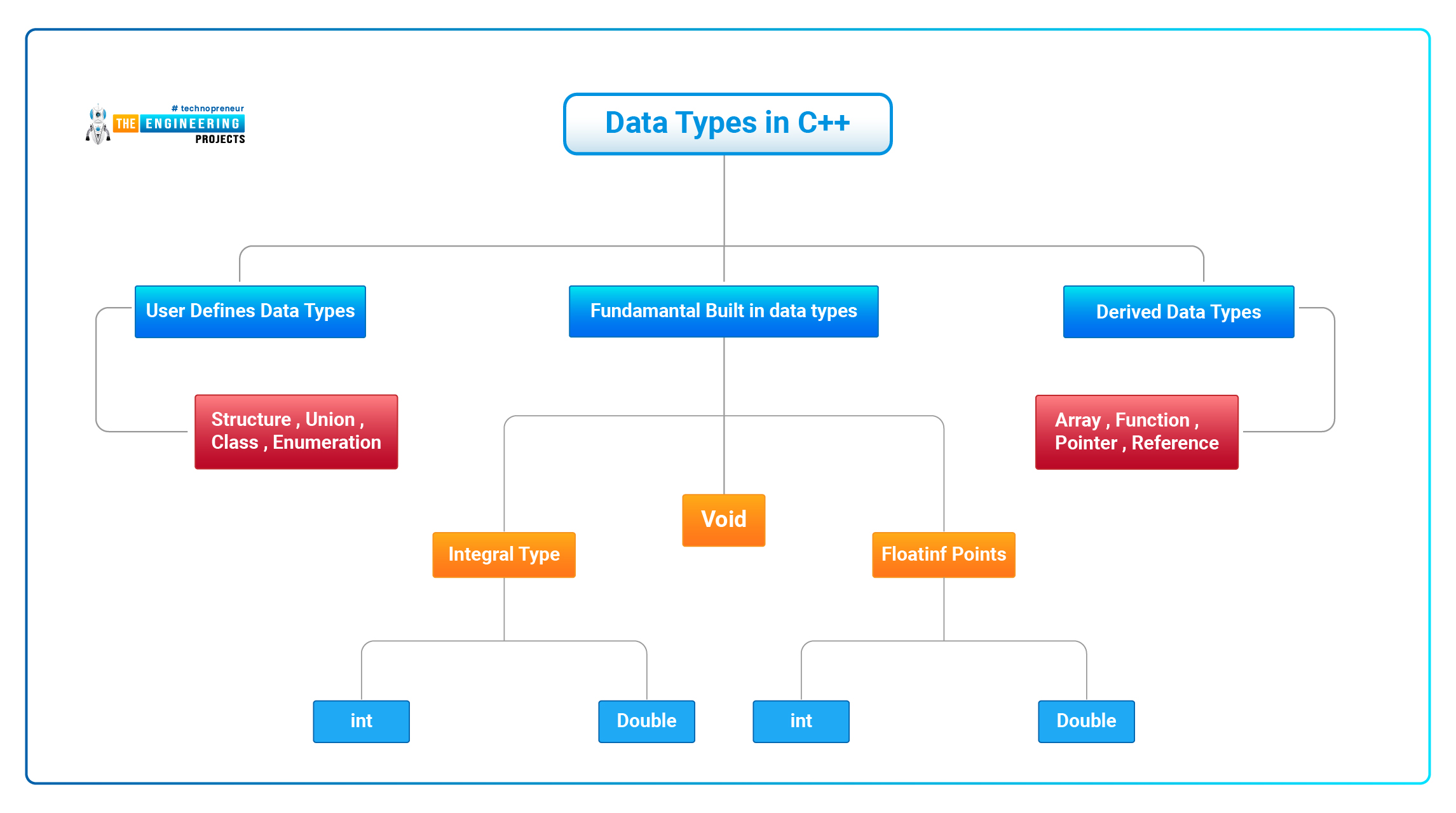
C++ Program to get a sum of 3 numbers
//Program to receive three integer numbers and display their sum #include <iostream> using namespace std; int main() { int num1, num2, num3, Sum; //variables num1, num2, num3 and sum are declared as integers cout << "\n Enter Number 1: "; cin >> num1; cout << "\n Enter Number 2: "; cin >> num2; cout)<< “\n Enter Number 3: “; cin>>num3; Sum = num1 + num2 + num3; cout << "\n The sum of " << num1 << num2 “ and " << num3 << " is " << Sum; }
Modifiers in C++
In C++, special words(called modifiers) are used to modify built-in data types. There are four main data type modifiers in C++, they are:
- Long
- Short
- Signed and
- unsigned
These modifiers are with built-in data types to make them more precise and for expanding their range.
- long and short modify the maximum and minimum values that a data type can hold.
- Signed types include both +ive and _ive numbers as is the default type.
- Unsigned, numbers do not have any sign, so they are always positive.
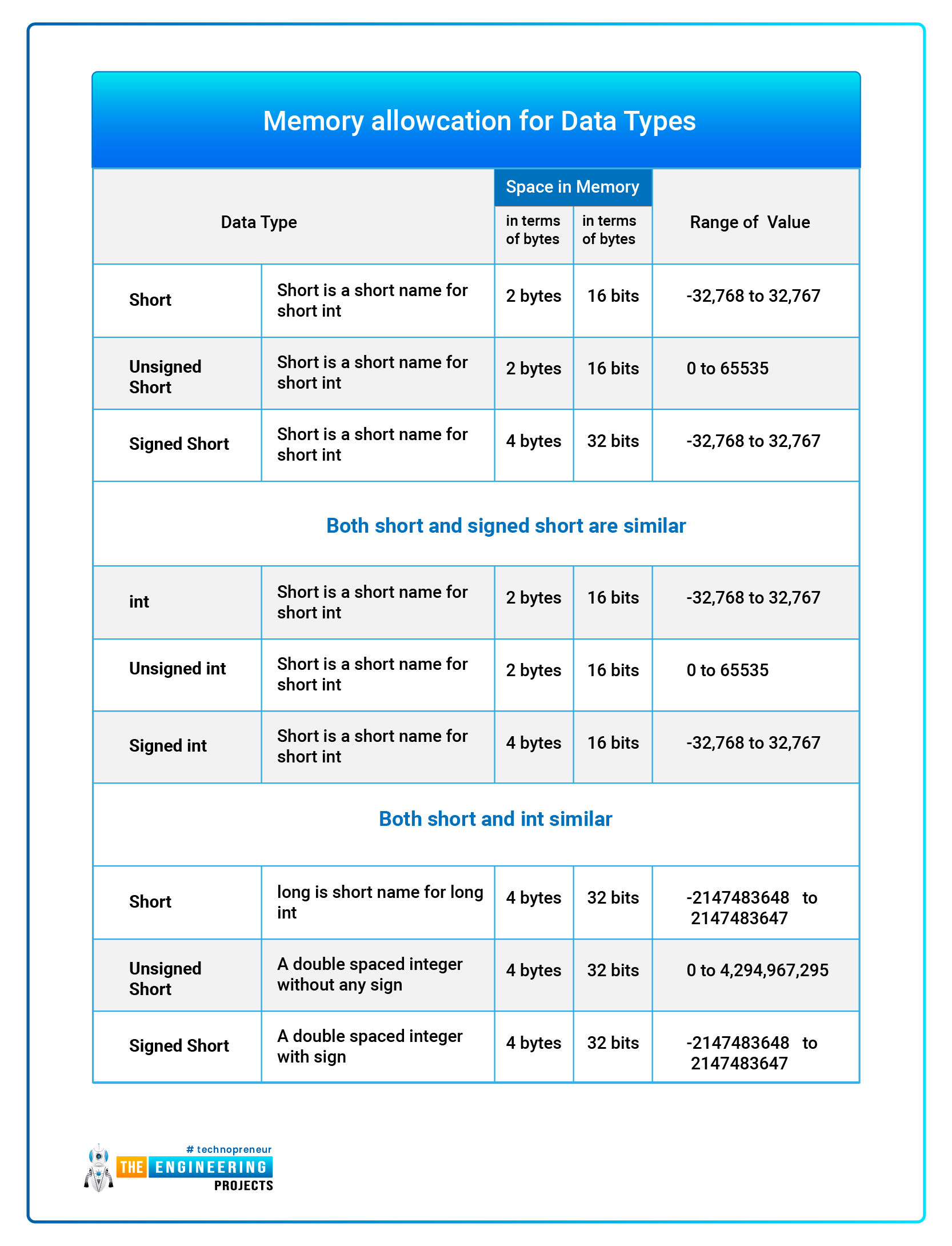
Variables in C++ :
- Variable is used in C++ to store any value, which can be changed in the program.
- Variable is declared in many ways each with different memory location and functioning.
- It is the name of the memory location allocated by the compiler to the variable.
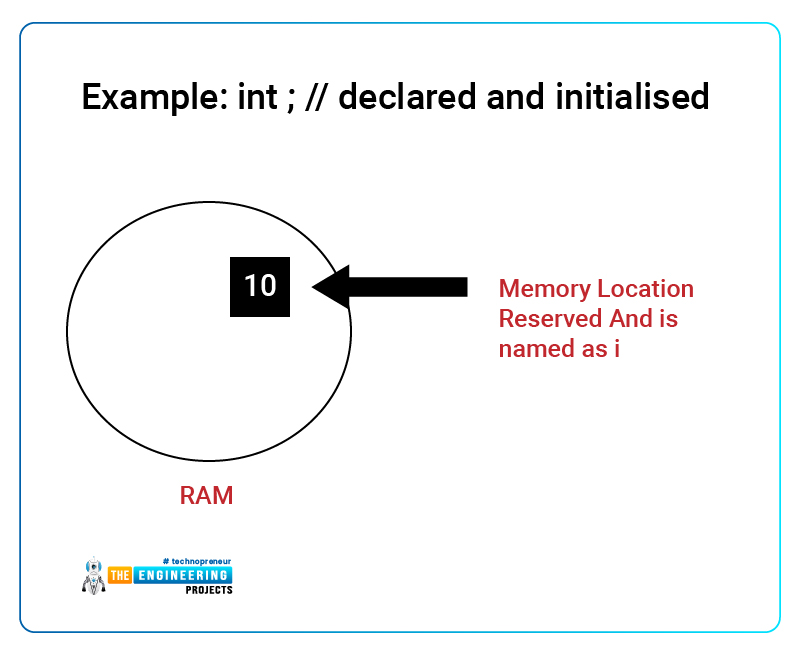
Variables are divided into two main types,
- Global Variables
- Local variables
Global variables
Global variables are those which declared only a single time and used again and again. They are declared outside the main() function. If only declared then assigned different values at different times in program lifetime. But when they are declared and initialized at the same time then they can be assigned any value at any point in the program.
For example: Only declared, not initialized
include <iostream> using namespace std; int x; // Global variable declared int main() { y=10; // Initialized once cout <<"first value of y = "<< y; y=20; // Initialized again cout <<"Initialized again with value = "<< y; }
Local Variables
- Local variables exist only between the curly braces, in which they are declared.
- Outside the curly braces, they are unavailable and lead to a compile-time error.
include <iostream> using namespace std; int main() { int j=10; if(j<20) // if condition scope starts { int m=100; // Local variable declared and initialized } // if condition scope ends cout << m; // Compile time error, m not available here }
C++ Program to find the curved surface area of a cylinder (CSA) (CSA = 2 pi r * h)
#include <iostream> using namespace std; int main() { float pi = 3.14, Radius, Height, CSA; cout << "\n Curved Surface Area of a cylinder"; cout << "\n Enter radius (in cm): "; cin >> Radius; cout << "\n Enter height (in cm): "; cin >> Height; CSA = (2*pi*Radius)*Height; system("cls"); cout << "\n radius: " << Radius <<"cm"; cout << "\n height: " << Height << "cm"; cout << "\n Curved Surface Area of a Cylinder is " << CSA <<" sq. cm."; }
Output:
- Curved Surface Area of a cylinder
- Enter radius (in cm): 7
- Enter height (in cm): 20
- radius: 7cm
- height: 20cm
- The curved Surface Area of a Cylinder is 879.2 sq. cm.
Operators in C++ :
- Operators take one or more arguments and generate a new value.
- For example : addition (+), subtraction (-), multiplication (*) etc, are all operators.
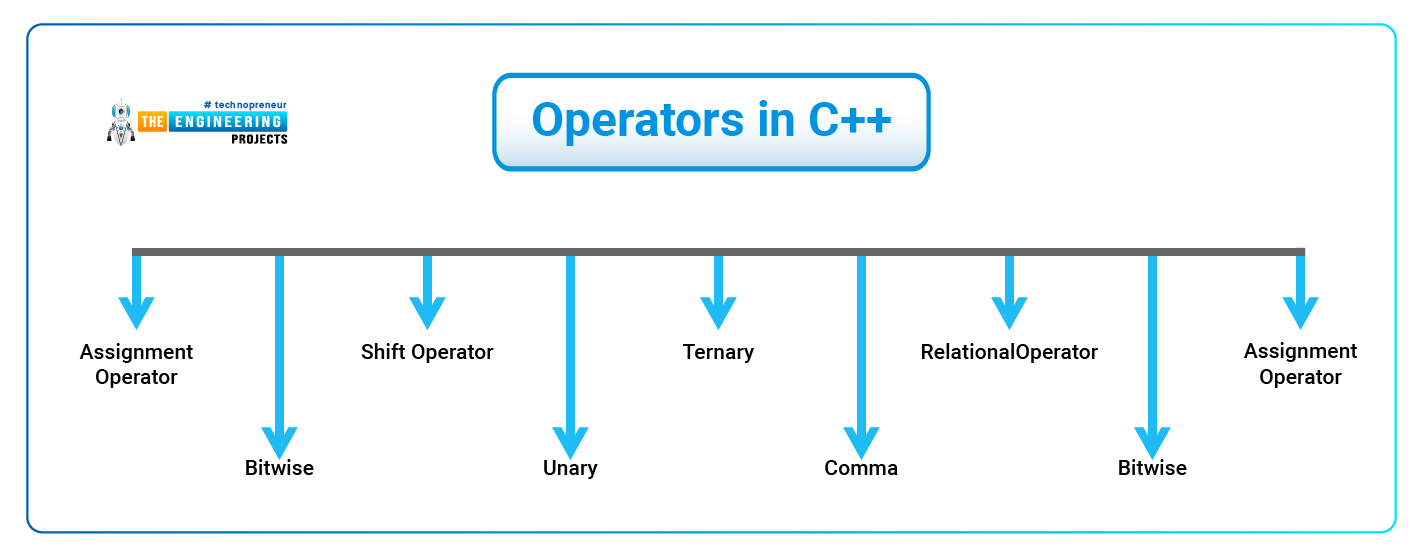
- These are used to perform different operations on variables and constants.
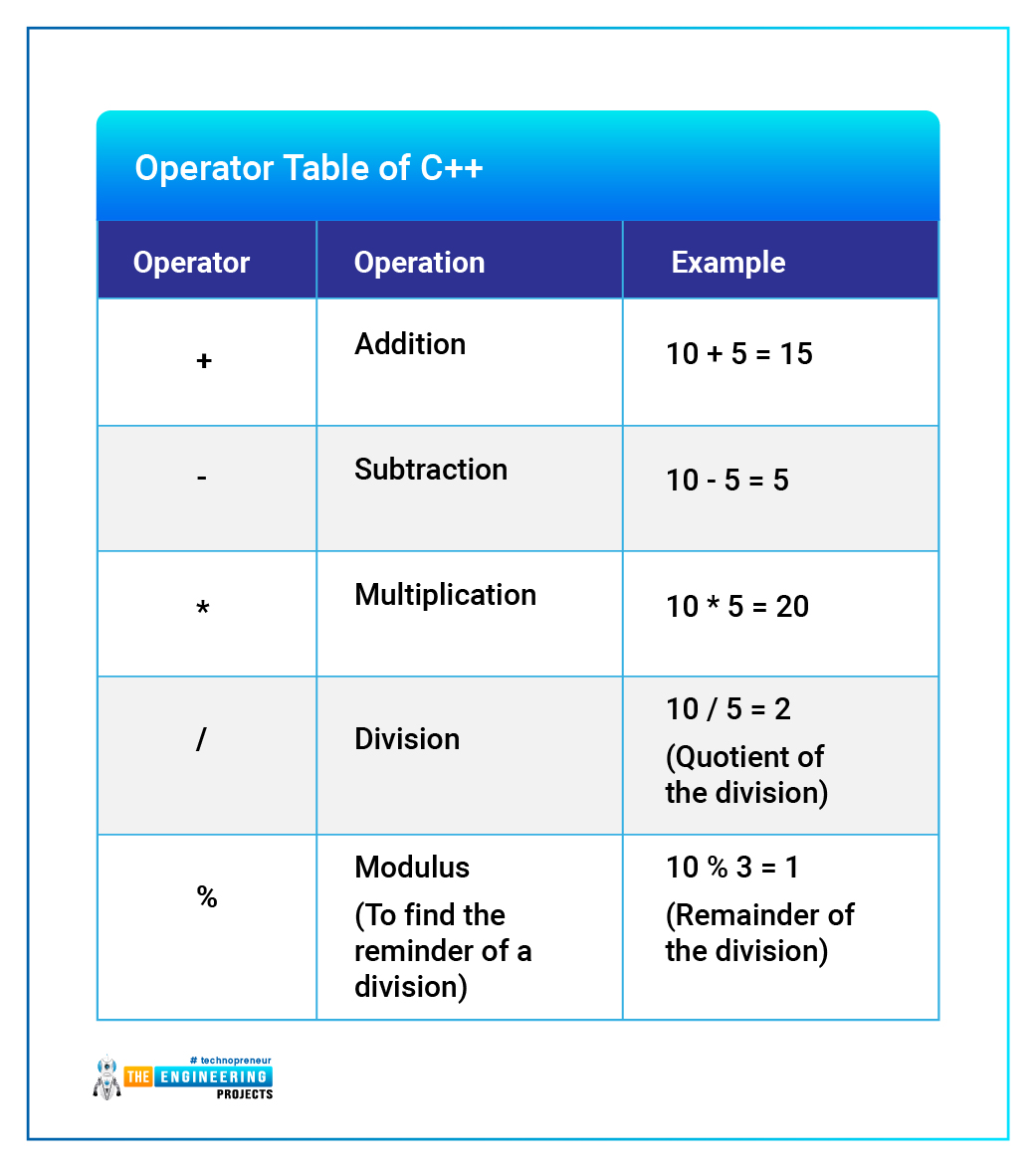
Errors in C++:
There are three types of errors that occur in C++ programming
Syntax Error:
- The syntax is a set of grammatical rules to make a program. Every programming language has unique grammatical rules.
- when grammatical rules of C++ are violated Syntax Errors occur.
- For example: if you type as follows, C++ will throw an error.
cout << “Hi welcome to C++”
- As per the grammatical rules of C++, there should be a semicolon at the end of the statement. But, this statement does not end with a semicolon so Syntax Error occurs.
Logical Error:
It may be happened by the wrong use of variable or operator or order of execution etc. This means that the program is grammatically correct, but it contains some logical errors. So, “Logic Error” is also called Semantic error.
Run time error:
- During the execution of the program when some illegal action takes place, run time error occurs
- For example, if a program tries to open a file that does not exist then it will result in a run-time error.
Control Statements in C++:
- The sequence of flow of instructions is changed by the use of control Statements.
Selection statement
- Statements can run sequentially, selectively or iteratively in a program. Sequence, selection and iteration processes are handled by every programming language.
- If the statements are executed sequentially then the flow is called a sequential flow. In some situations, if the statements alter the flow of execution then this flow is called a control flow.
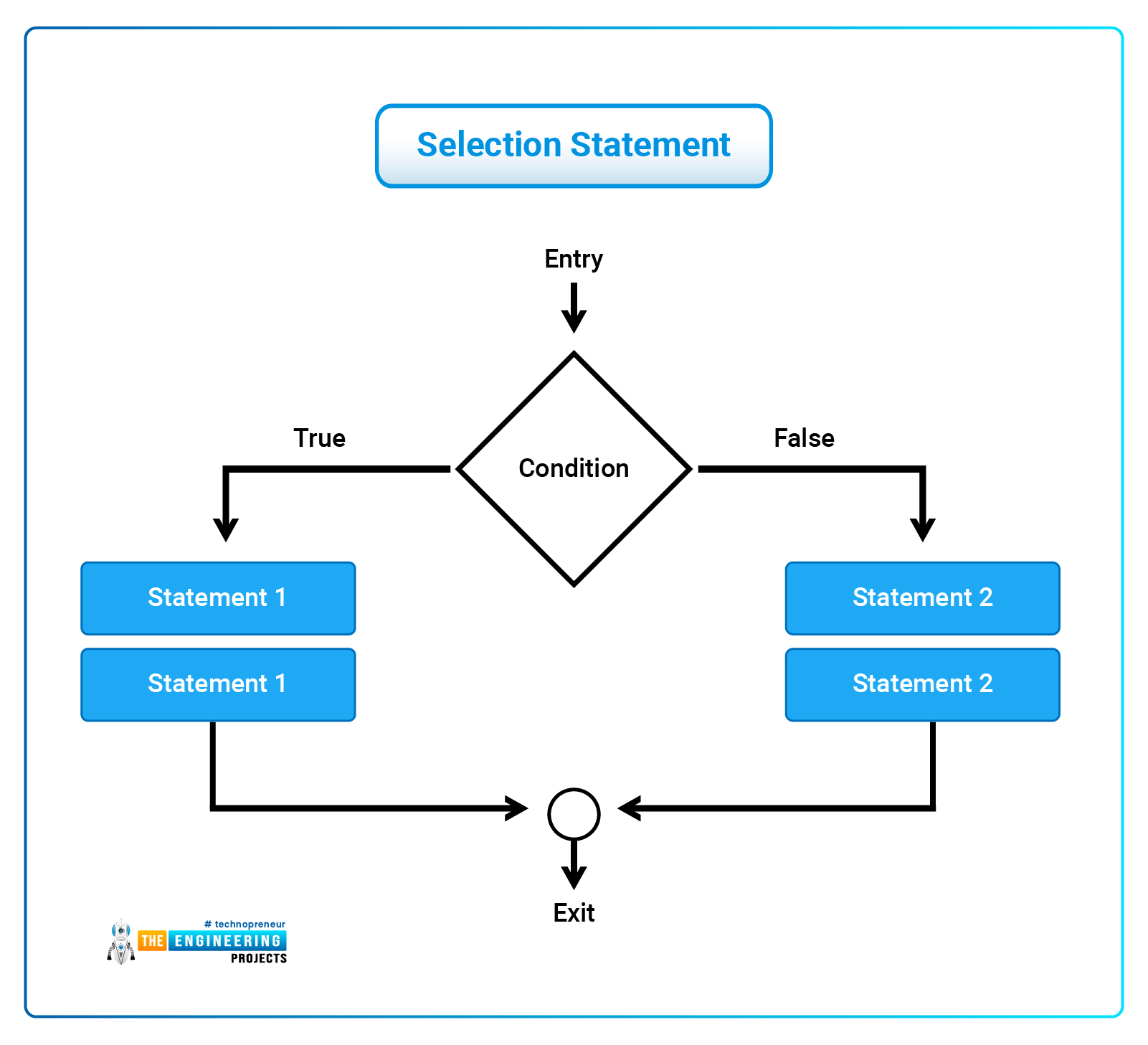
Sequence statement
- The sequential statement is executed one after the another only once from top to bottom.
- These statements do not alter the flow of execution and are called sequential flow statements. These statements always end with a semicolon (;).
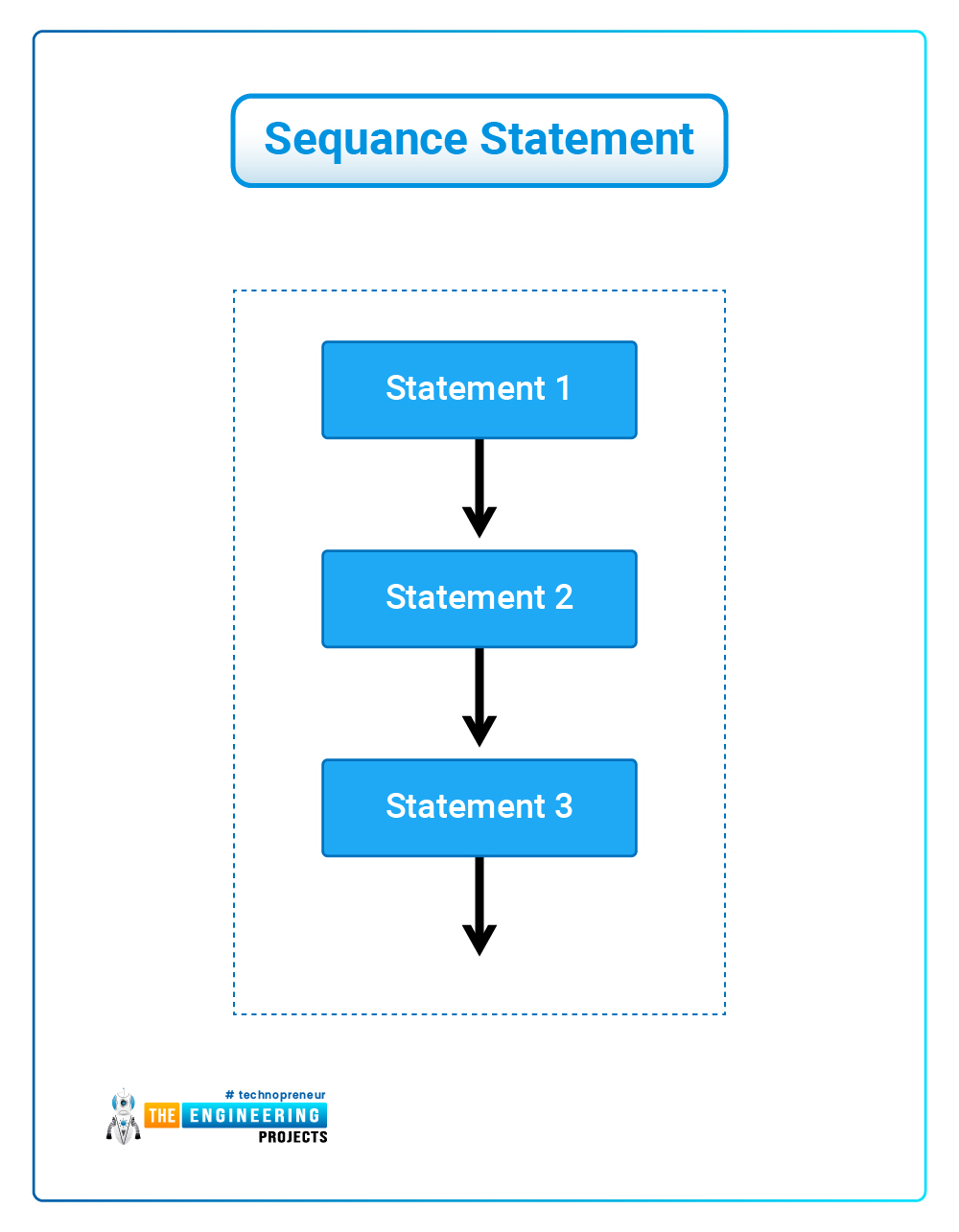
Selection statement
- When a condition is provided then selection statements are used.
- In case when the condition is true then a true block (a set of statements) is executed otherwise a false block is commanded to execute.
- This is also called a decision statement because it helps in making decisions for the set of statements to be executed.
- Selection statements are if, if-else and nested if statements.
if statement
- The general syntax of the if statement is:
if (expression) true-block; statement-x;
- and flow chart for if statement is
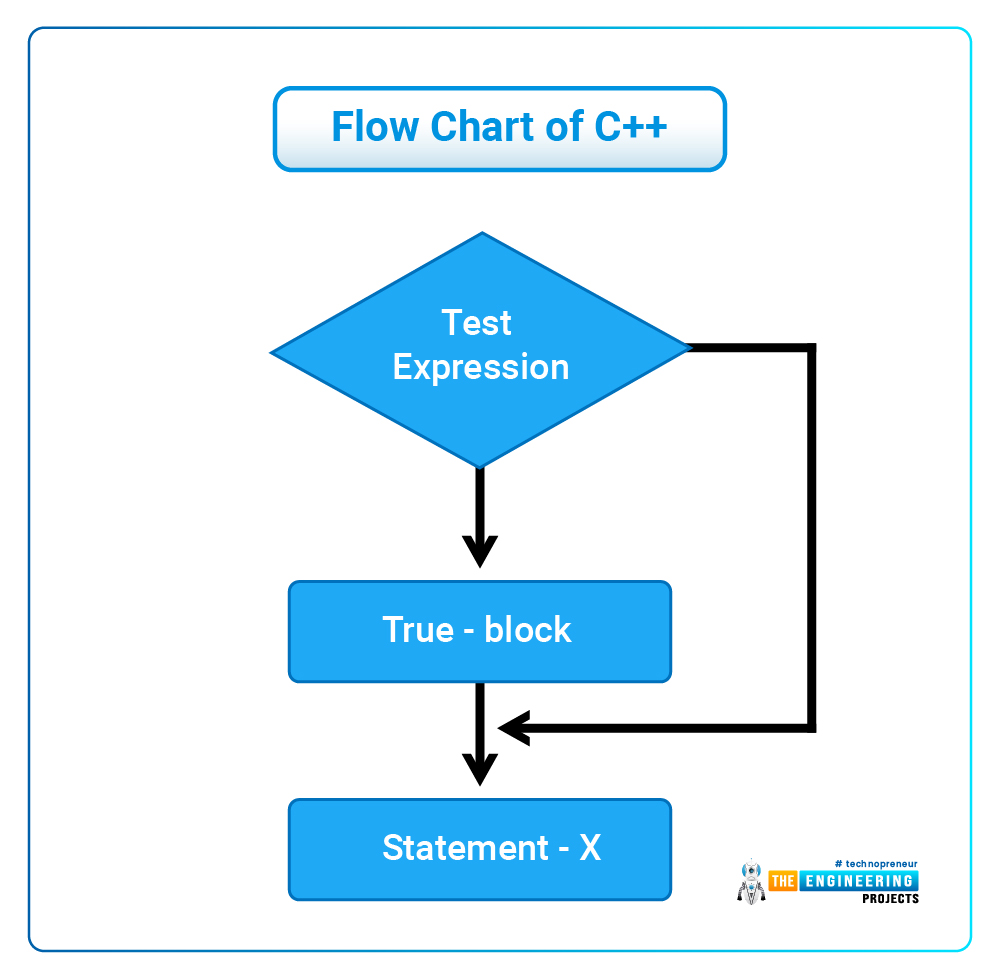
if-else statement
- The syntax of the if-else statement is given below:
if ( expression) { True-block; } else { False-block; }
- And the flow chart for if-else statement is:
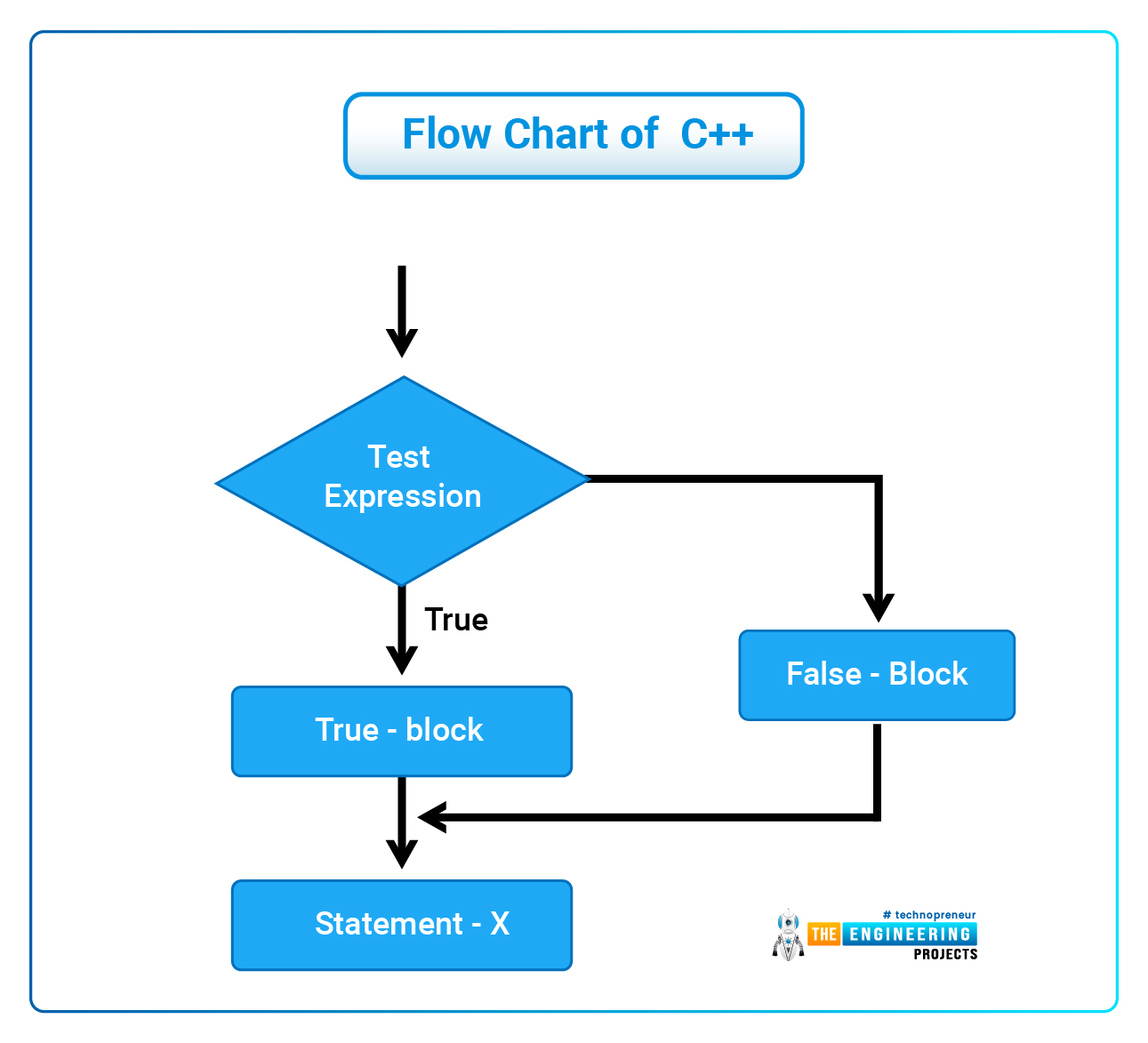
C++ program to find either number is Even or Odd
#include <iostream> using namespace std; int main() { int Num, rem; cout<< "\n enter a number: "; cin>>Num; rem = Num % 2; if (rem==0) cout<< "\n The given number" <<Num<< " is Even"; else cout<< "\n The given number "<<Num<< " is Odd"; return 0; }
Output
- Enter number: 12
- The given number 12 is Even
Nested if
It has three forms- If nested inside if part
- If nested inside else part
- If nested inside both if part and else part
Iteration statement
- The iteration statement is a set of statements that are executed again and again depends upon conditions.
- If a condition evaluates to true, the set of statements (only true) is executed again and again.
- As soon as the condition seems to be false, the repetition stops. This is also known as looping statement
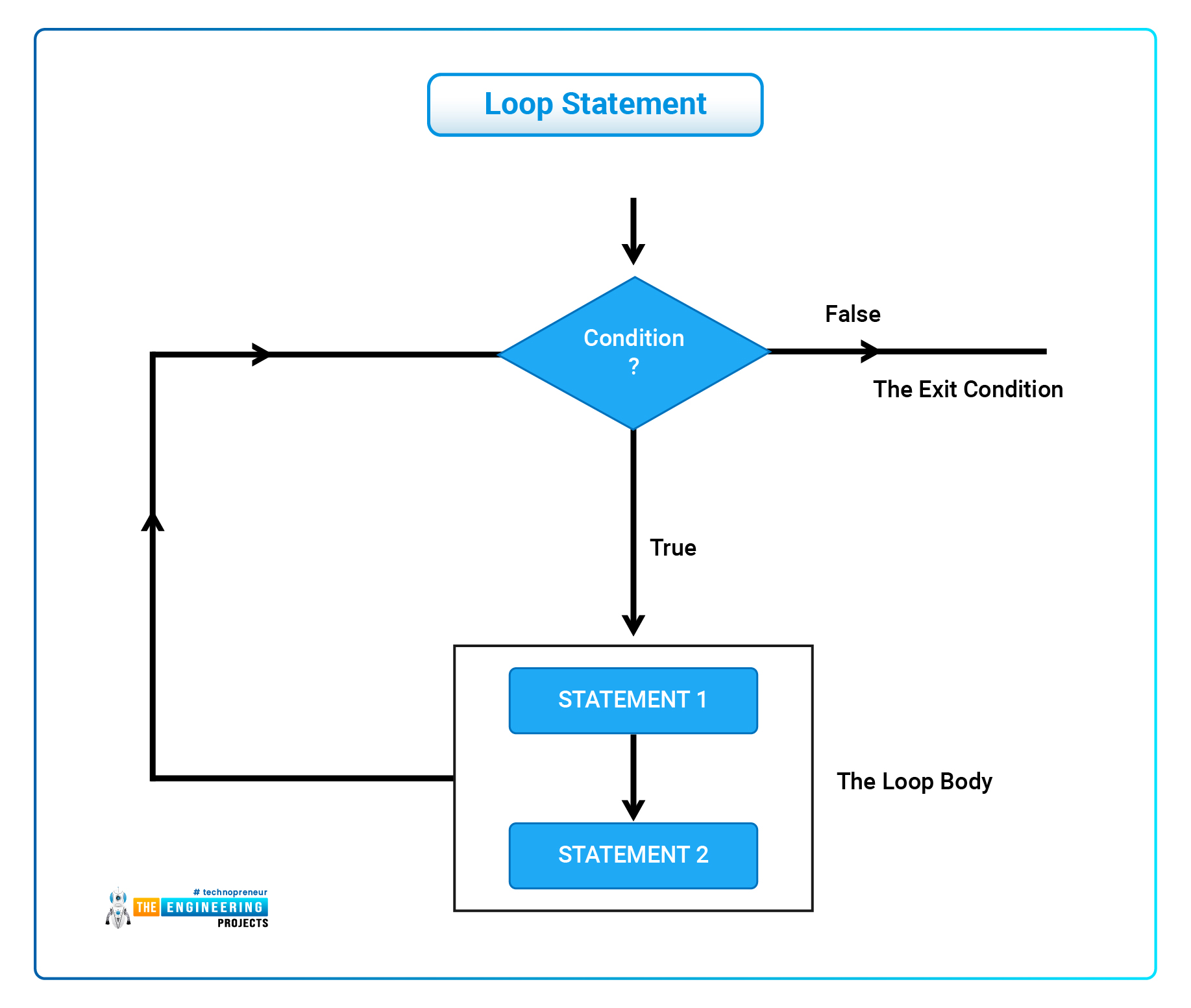
- The set of statements that are executed repeatedly is called the body of the loop.
- The condition on which exits from the loop is called exit-condition or test-condition.
- There are 3 kinds of loops in C++
- For loop
- While loop
- Do while loop
for loop
- The for loop is the easiest loop which allows code to be executed again and again.
- The general syntax is:
for (initialization(s); test expression; update expression(s)) { statement 1; statement 2; …………. }
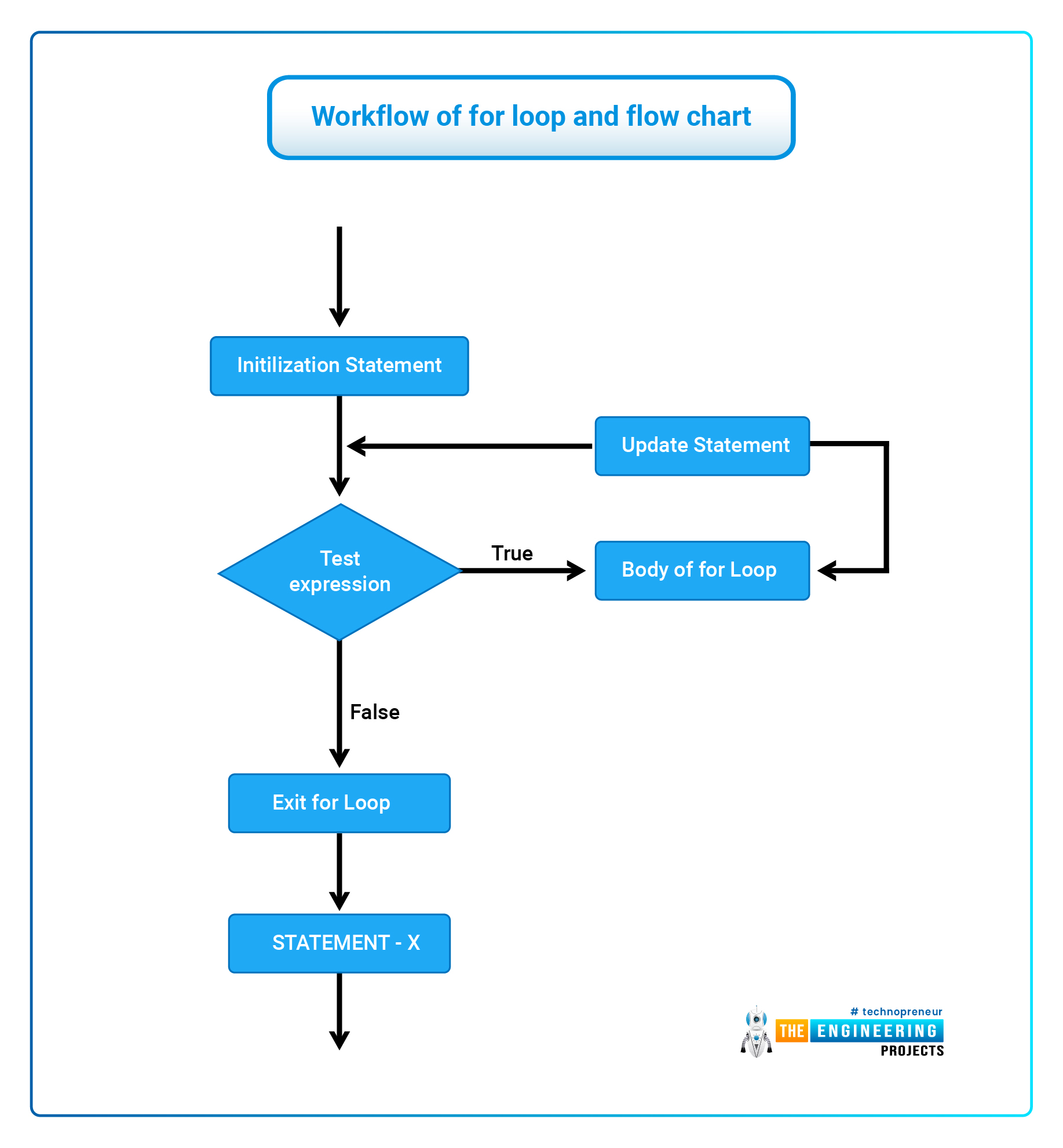
C++ program to sum from 1 to 5 using for loop
#include <iostream> using namespace std; int main () { int i,Sum=0; for(i=1; i<=5;i++) { sum=sum+i; } cout<<"The sum of 1 to 5 is "<<Sum; return 0; }
Output
- The sum of 1 to 5 is 15
While loop
- It allows the loop statements to be executed as long as the condition is true.
- The while loop syntax is:
while ( test expression ) { body of the loop; }
- Flow chart for while loop is given below
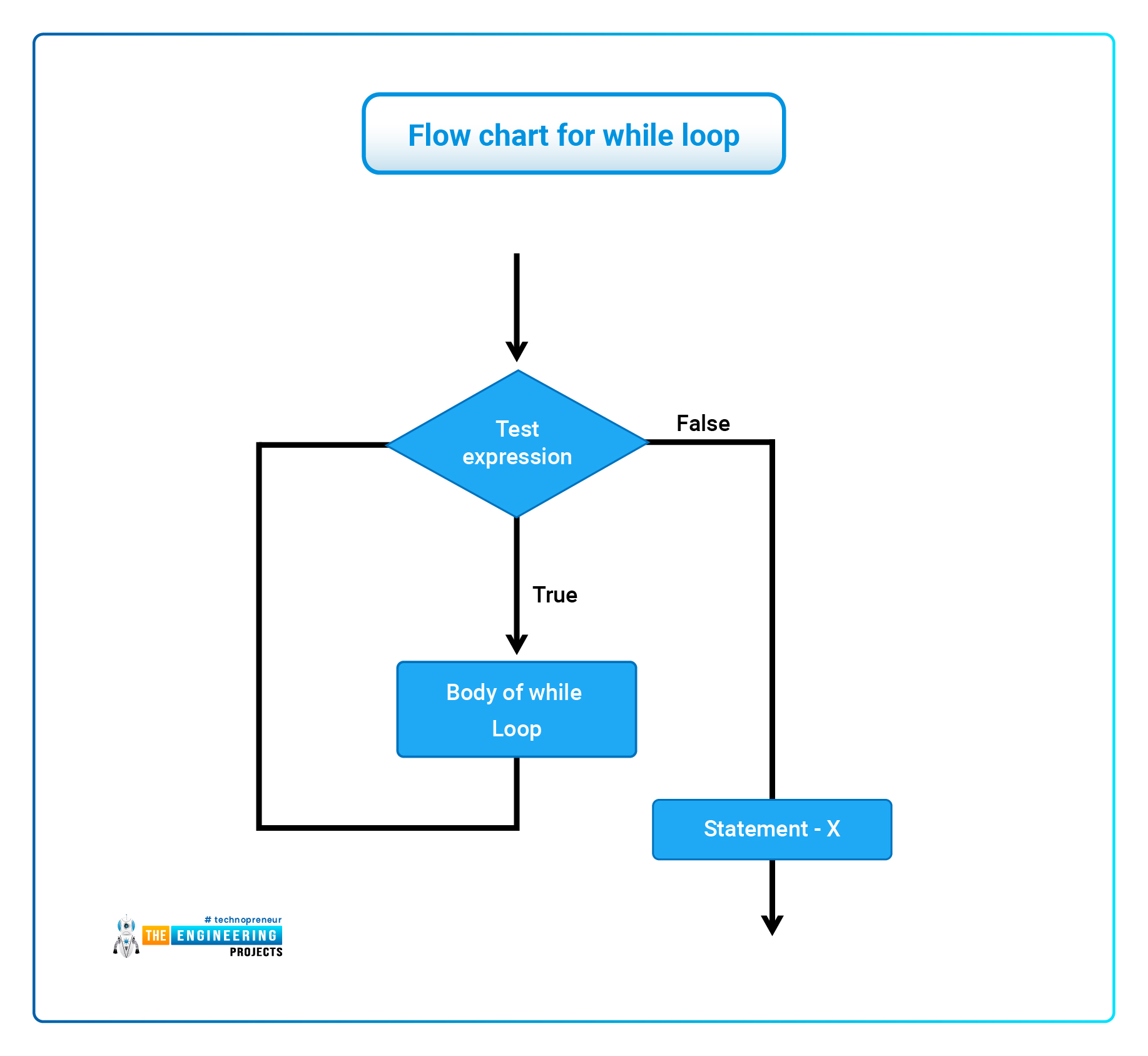
C++ program to sum from 1 to 6 using while loop
#include <iostream> using namespace std; int main () { int i=1,SUM=0; while(i<=6) { SUM=SUM+i; i++; } cout<<"The sum of 1 to 6 is "<<SUM; return 0; }
Output
- The sum of 1 to 6 is 21
do-while loop
- The do-while loop is used as an exit-controlled loop. In a do-while loop, after executing the body of the loop, the condition is evaluated.
- The do-while loop syntax is:
do { body of the loop; } while(condition);
- The flow chart of the do-while loop is shown below
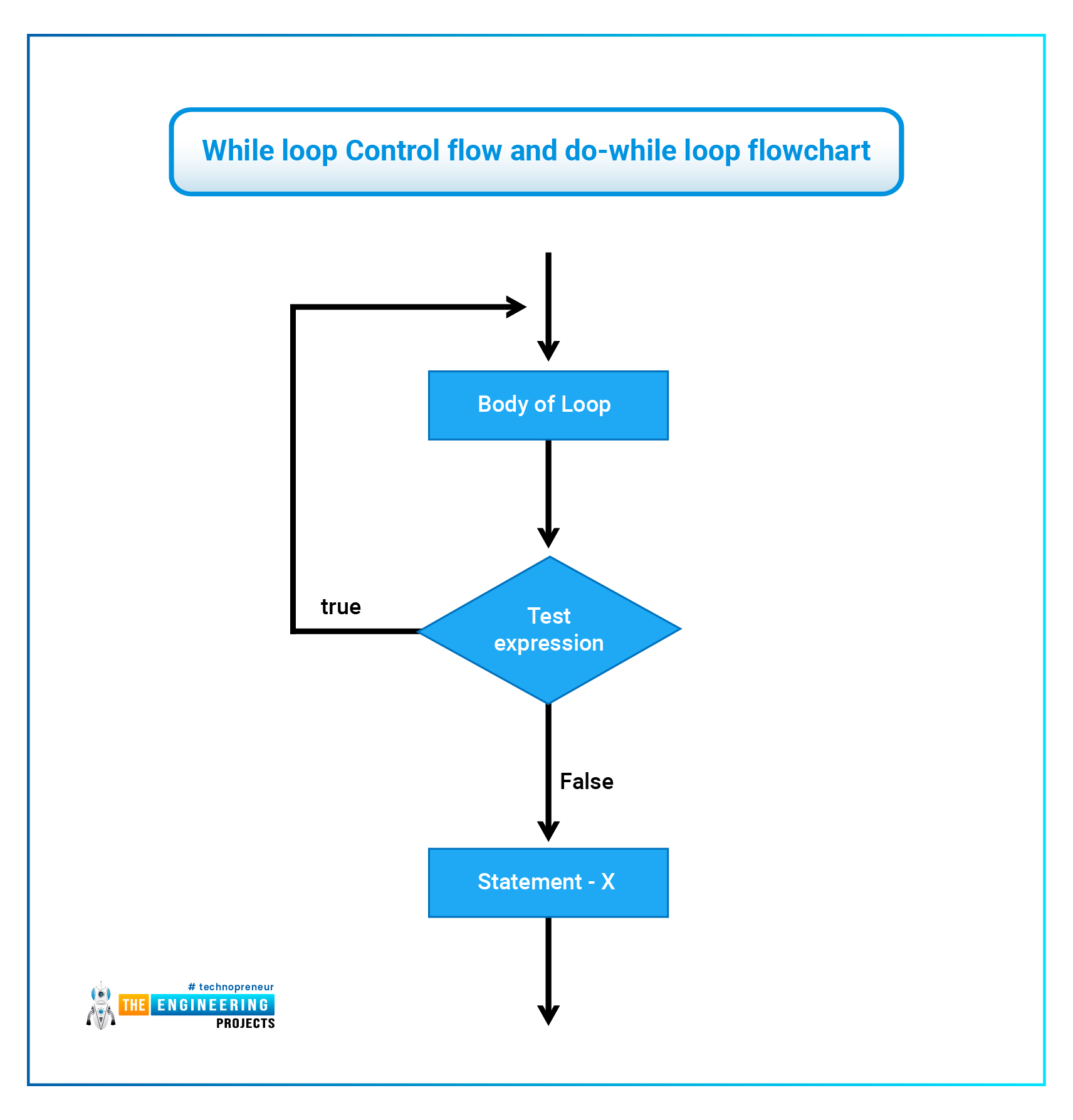
Examples of C++ programs:
1. C++ Program to find the Total marks of three subjects
#include <iostream> using namespace std; int main() { Int n1, n2 , n3, Sum; cout << "\n Enter Mark 1: "; cin >> n1; cout << "\n Enter Mark 2: "; cin >> n2; cout << "\n Enter Mark 3: "; cin >> n3; Sum = n1 + n2 + n3; cout << "\n The sum = " << Sum; }
2. C++ program to find the Area of a Circle
#include <iostream> using namespace std; int main() { int Radius; float Area; cout << "\n Enter Radius: "; cin >> Radius; Area = 3.14 * Radius * Radius; cout << "\n The area of circle = " << Area; }So, that was all for today. In the next tutorial, we are going to discuss the Data Types in C++ in detail. If you have any questions regarding this tutorial, ask in the comments. Thanks for reading !!!