In the previous tutorial, we have seen the basics of C++ and have also discussed a small code. We have discussed various programming terms in the previous tutorial and from now on, we are going to discuss those terms in detail. So, today's lecture is on Data Types in C++, let's get started:
C++ Data Types
A data type defines the type and the set of operations that can be performed on the data. Various types of data are manipulated by the computer. The data is given as an input to the program. According to the program's set of instructions, data is processed and then output is returned on the screen. Before designing the actual program data, its type and operations performed on it are defined and used to process the data. At the start of the program, the type of each data value is identified. Various data types are provided by C++ and the computer’s memory allocates each data type differently.
Types of data types in C++
- Built-in data types
- derived data types
- user-defined data types
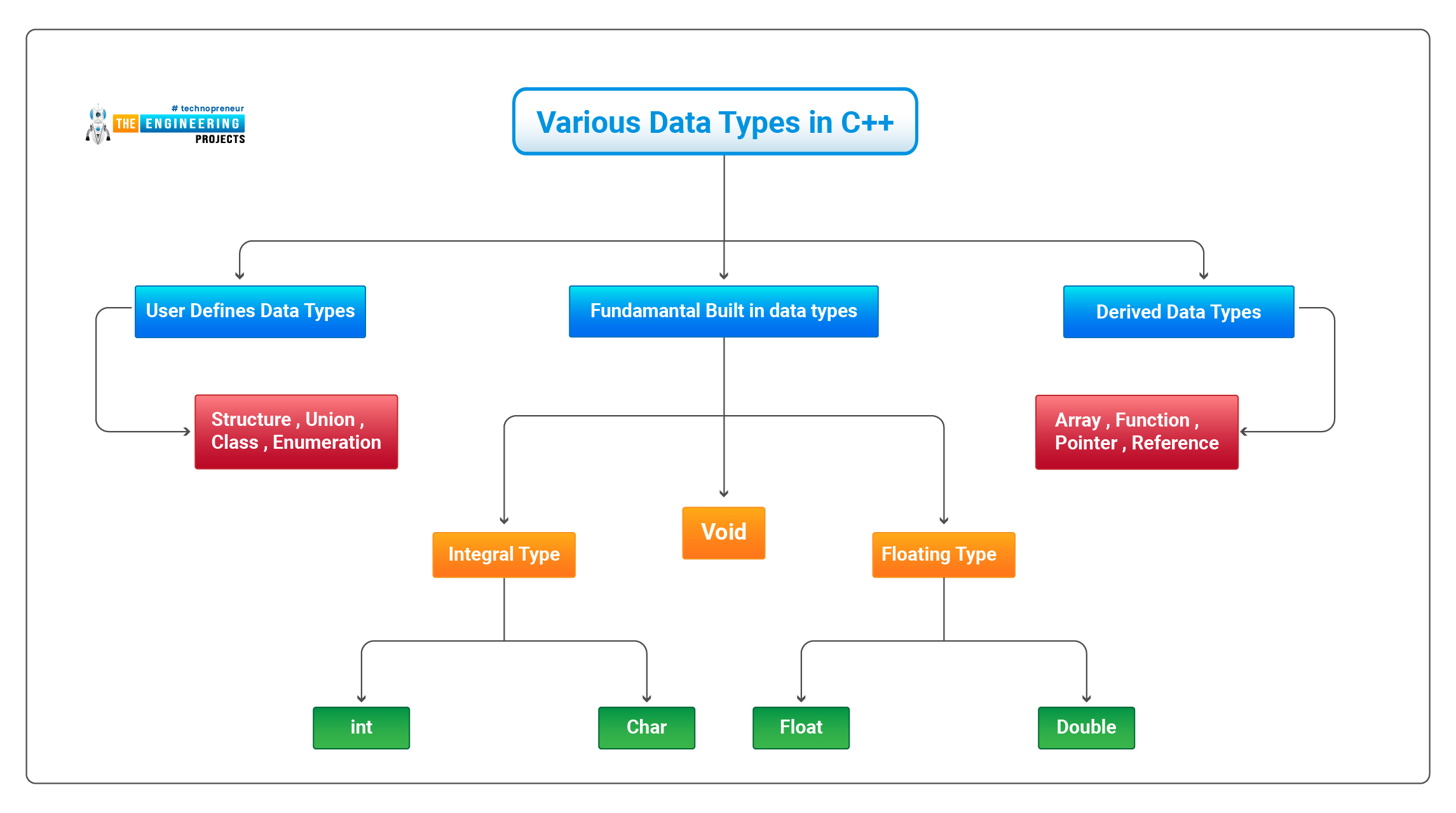
Built-in Data Types in C++
- Integral, floating-point and void data types are provided by C++ as built-in data types.
- The integral and floating-point data types can be treated with different types of modifiers.
- The size or range of data type is changed by using these modifiers.
- Short, long, signed and unsigned are various modifiers.
1. Integral data type
- Integer data type: keyword int is used to express integer data type.
- It normally requires 4 bytes of memory and ranges from -2147483648 to 2147483647.
long b = 4523232; long int c = 2345342; long double d = 233434.56343; short d = 3434233; // Error! out of range unsigned int a = -5; // Error! Used for storing only +ive numbers and 0
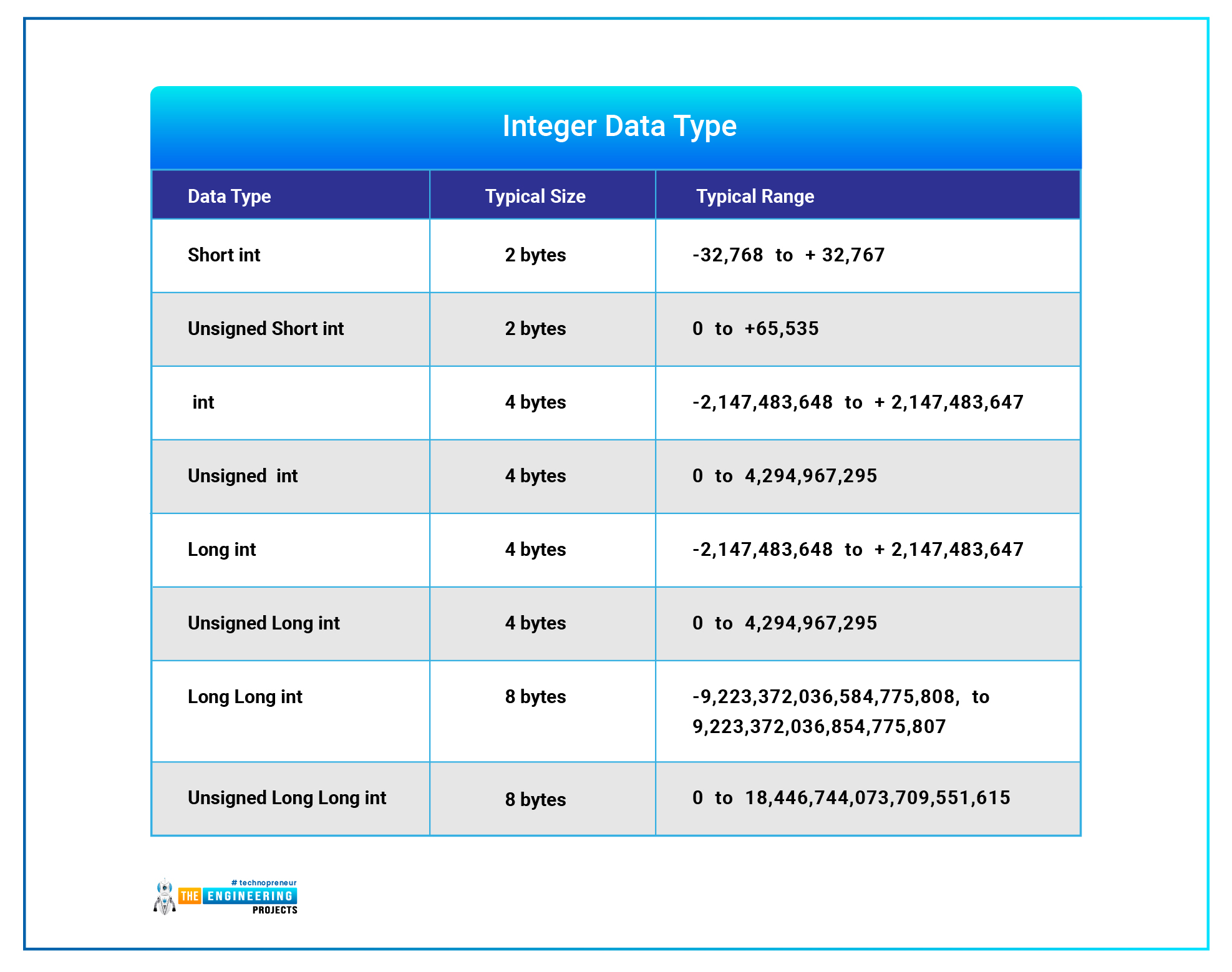
Example


Character data type in C++
- Characters are stored by using character data type.
- Char is used to representing character data type.
- It normally requires 1 byte of memory.

- char test = 'h';
- As each character is assigned by a unique ASCII code which is an integer so that’s why characters are included in integral data type.
- When we store a character in memory, we actually store a unique numeric code associated with that character in memory.
- And when the computer is instructed to print characters, it basically prints characters associated with the numeric code.
- Here's the chart showing ASCII codes of alphabetical characters:
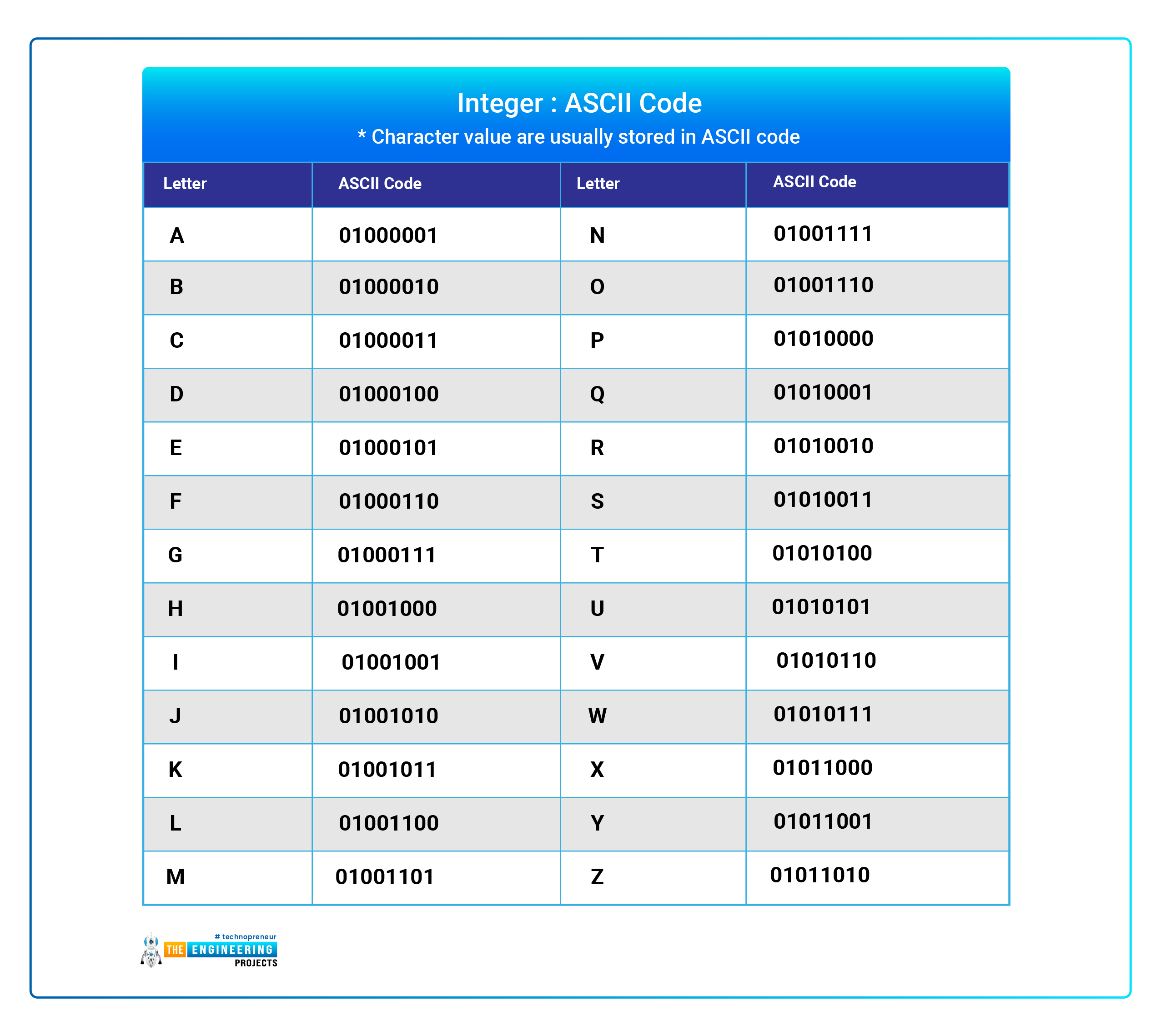
2. Floating data types in C++:
- Variables holding real numbers are defined by using floating-point data type. In C++ three data types are used to represent floating-point numbers.
- float
- double
- Long double
float area = 64.74; double volume = 134.64534;
- Values having floating-point types are expressed in terms of precision and range.
- The accuracy in the fractional part of the value is precision.
- Range: it is for the range of fractions and numbers.
- The floating point number is stored as mantissa E or the power of 10.
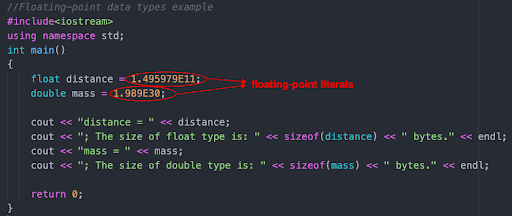
3. Void data type
- The meaning of void is without any value and is used where functions do not return any value.
- A function does not take any argument when the void is used to define an empty parameter list and a function does not return any value when it is used to return value to the function.
- 0 bytes of memory is allocated to it and hence it cannot store anything. As a result, it cannot be used to declare simple variables but it can be used to define generic pointers.
C++ Void Pointer
#include <iostream> using namespace std; int main() { void* ptr; float f = 2.3f; // assign float address to void ptr = &f; cout << &f << endl; cout << ptr << endl; return 0; }Output
0xffd117ac 0xffd117ac
Bool and wcha_t:
- In C++, Boolean and wide-character data types are also used.
- Boolean values are defined by bool data type.
- Bool data type contains only 2 integers 0 and 1.
- False is represented by 0 and 1 is used to represent true.
- Only 1 bit of storage is required by bool data type.
- As it is stored as an integer so it can be considered as an Integral data type. The results of logical operations performed on data are represented by the bool data type.
bool cond = false;
- In addition to char data type, wchar_t is used to store 16- bit wide characters in C++. Large character sets are allocated with non-English languages so wchar-t is used to store wide characters.
wchar_t test = $# // storing dollar and hash character;
User-Defined Data Types in C++
C++ provides various user-defined data types- structures,
- unions,
- enumerations and
- classes.
Structure, Union and class
Important features of the C language are structures and unions. Similar or dissimilar data types assigned a single name are grouped by structure and union. However, in C++ the concept of structure and union is modified by adding some new features to provide support to object-oriented programming. Class is defined as a new user-defined data type by C++ and it forms the basis of object-oriented programming. A class functioned as a template that can be used to define the data and functions that are included in an object of a class. Classes are declared using the keyword class. The object of the class can be created when the class is declared.Enums in C++
A set of integer constants that are named and specify all the values that can be assigned to variables Enumerators are the set of these permissible values. For example, consider the statement.enum Colour{red, green, blue, white}; // declaring an enum type
- In this statement, an enumeration data-type color (Colour is a tag name), consisting of enumerators red, green, blue and white is declared.
- These enumerators represent only integer values, so any arithmetic operation can be performed on them.
- By default, the first enumerator in the enumeration data type will have the value zero.
- The value of the next enumerators is increased by one. Hence,
- the value of red is 0,
- the value of green is 1
- the value of blue is 2 and
- the value of white is 3.
enum country {US, UN=2, India, china} ;
- Value for the US is 0 by default in this declaration
- the value of UN is 2
- Russia is 3 and
- China is 4
country country1, country2;
For example, consider these statements.- Country1 Pakistan; // valid
- country2 China; // invalid
Derived Data Types in C++
Derived data types are derived from built-in data types. C++provide 4 major derived data types that are- arrays,
- Functions,
- references and
- pointers.
Array
- The set of elements having the same data type and same name formed an array.
- In memory, all the elements are stored one after another location.
- An index or subscript is used to access an element.
- The position of the element is indicated by the subscript value.
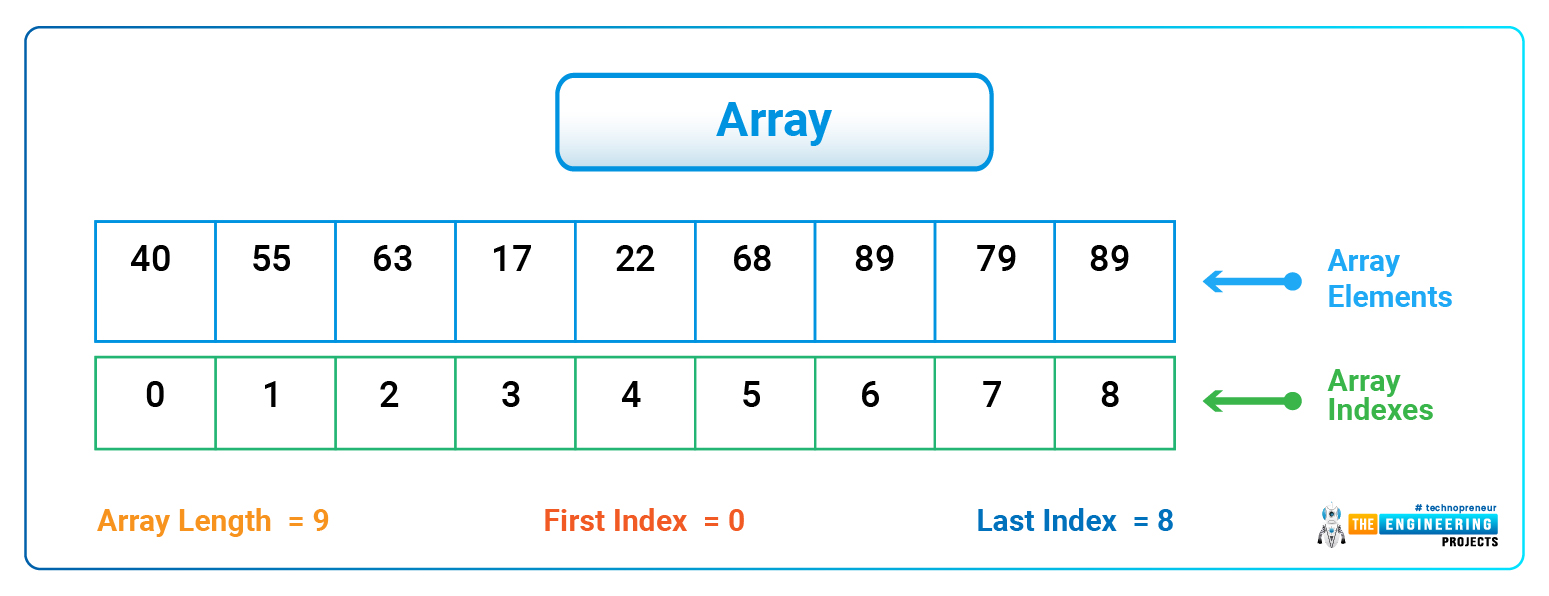
Declaring Arrays:
- Syntax to declare a one-dimensional array:
//intExp indicates number of elements dataType arrayName[intExp]; //declares array num containing 4 elements of type int: //num[0], num[1], num[2],and num[3] int num[4];
Accessing Array Elements:
- Syntax to access array element:
// intExp is used to specify position array Name[intExp] //fourth element in array num num[3];One dimensional array example
- Initialize
- Input
- Output
- Sum and Average
- Find largest element value
- Find the smallest element value
// name of the constant is initialized const int arraySize=5; double list[arraySize]; //initialize 7 variables int i=0; double smallest=0.0; double largest=0.0; double sum=0.0; double average=0.0; int maxi=0; int mini=0; //1. Array us initialized for (i=0; i < arraySize; i++) list[i]=0.0; //2. Give value for each element in array list, beginning w/first element for (i=0; i < arraySize; i++) cin >> list[i]; //3. Resulted value for each element in array list, beginning w/first element for (i=0; i < arraySize; i++) cout << list[i] << " "; //4. Sum and Average elements in array list, and display for (i=0; i < arraySize; i++) sum = sum + list[i]; average = sum / arraySize; cout << "Sum = " << sum; cout << "\nAverage = " << average; //5. find largest element value in array list, and display for (i=0; i < arraySize; i++) if (list[maxi] < list[i]) maxi = i; largest = list[maxi]; cout << "\nLargest = " << largest; //6. find smallest element value in array list, and display for (i=0; i < arraySize; i++) if (list[mini] > list[i]) mini = i; smallest = list[mini]; cout << "\nSmallest = " << smallest;
Function in C++
- The function is used to carry out a specific well-defined task. It is a self-contained program segment.
- In C++ one or more functions can be used in a program and can be invoked from the other parts of the program when needed.
#include <iostream> using namespace std; int fact(int n); // function prototype int main(){ int nv,ans; cout<<" Input n:"; cin>> nv; ans = fact(nv);// calling a function cout<<"Answer is:"<<ans<<endl; }
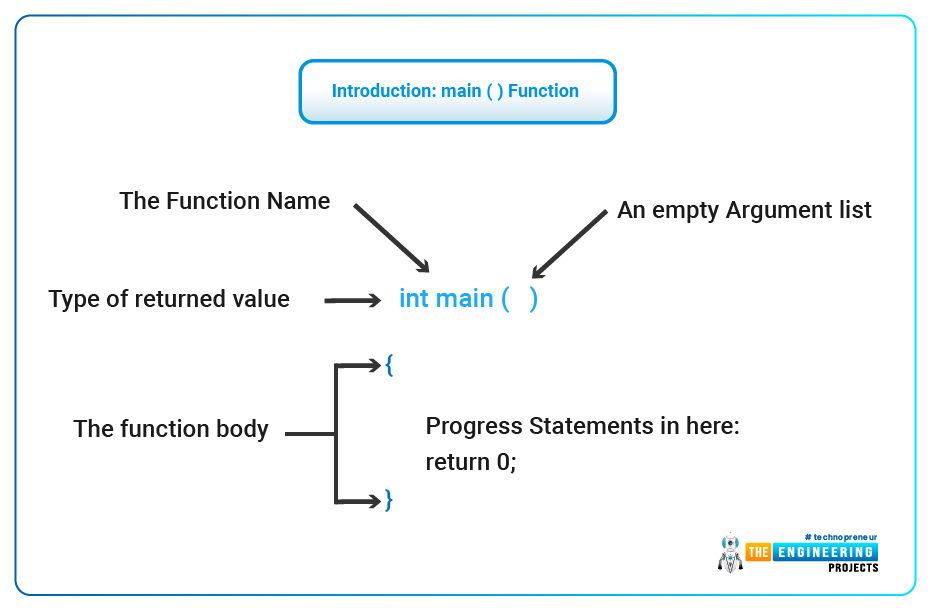
int fact(int n){ // int to another int int ans =1; for(int i= 3; i<= n; i++) ans *=i; return ans are; // function providebanswer to the caller }function prototype, calling main program and the complete function definition shown in the above program
C++ Reference
The alternate name of the variable is a reference. So we can say that a reference is an alias for the variable in the program. Both variable and reference allocate the same memory location so they can be interchangeably used in a program. When we make changes in variables, these changes are also made in reference and vice versa. – reference type variable in C++ is treated as a constant pointer that can be dereferenced.- Constant reference type variable must be initialized first and can not be used as a reference to other variables.
- Ampersands (&) are used with reference type variables. For example,
int result = 0; int &ref_result = result; … ref_result = 100;
C++ Pointers
- The variable which can be used to store the memory address of another variable is called a pointer.
- Memory is used dynamically by pointers.
- In run time, memory can be allocated and de-allocated with the use of pointers which increases the efficiency of the program.
- Assignment and dereferencing are two major operations performed by pointers. Assignment operation assigns a value to the pointer and dereferencing takes reference by indirection technique.
- If ptr is a pointer variable with the value 7080, and the cell whose address is 7080 has the value 206, then the assignment j = *ptr sets j to 206.
- The assignment operation j = *pta
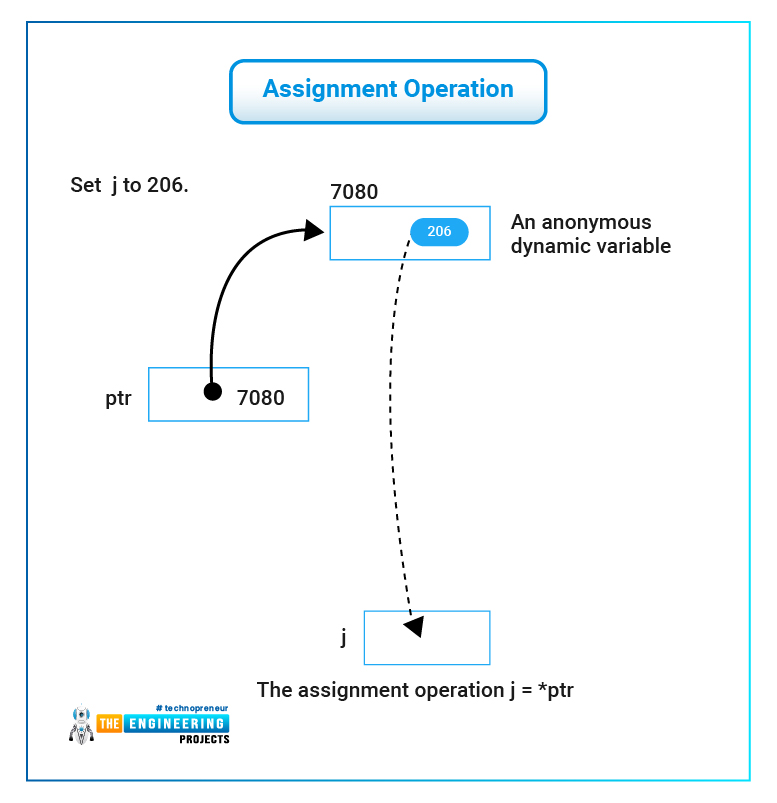
- Pointers should be used with care because they are flexible.
- Used for management addressing and dynamic storage.
- Pointer arithmetic in C++ makes them more interesting than other programming languages.
- The asterisk (*) is used for dereferencing operation, and the ampersand (&) for producing the address of a variable. For example, in the code
int *ptr; int count, init; … ptr = &init; count = *ptr
- single assignment statement can take place the value of two assignment operators.
count = init;
Example: Pointer Arithmetics in C++
int list[10]; int *ptr; ptr = list;
- *(ptr+5) is equivalent to list[5] and ptr[5] *(ptr+i) is equivalent to list[i] and ptr[i] –
- Domain type is not needed to be fixed.