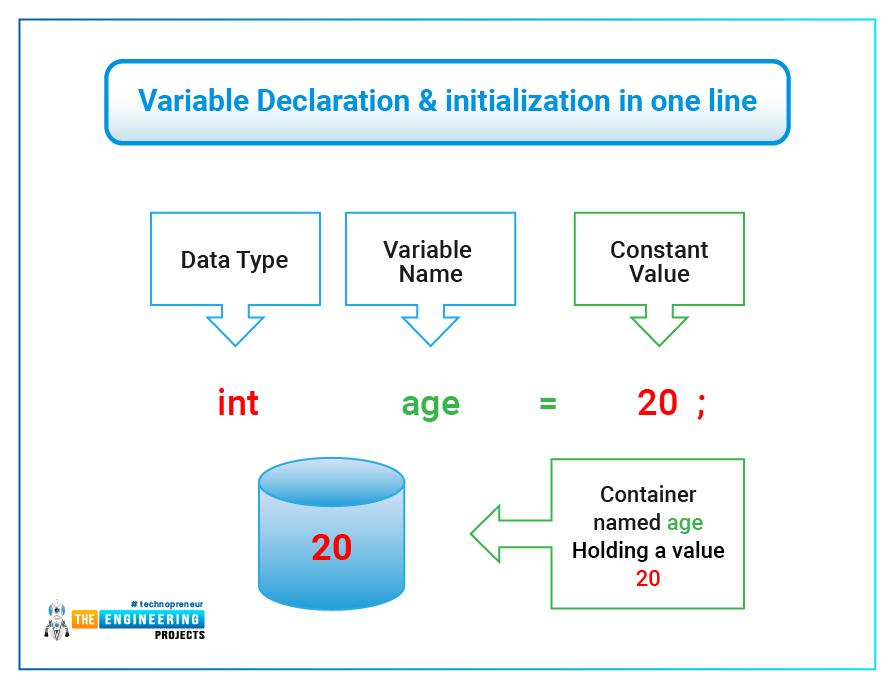
In the previous topic, we have discussed data types in C++ in detail. Today we will discuss variables and constants in C++.
A named memory location or memory cell is called a variable. The program's input data and its results are stored in variables during execution. During the execution of the program, the value of a variable can be changed but its name can not be changed.
Variables are created in random access memory. As we know that RAM is a temporary memory so data stored in it is also temporary. It can only be used during execution. When the program ends, the data stored in the variable is automatically removed.
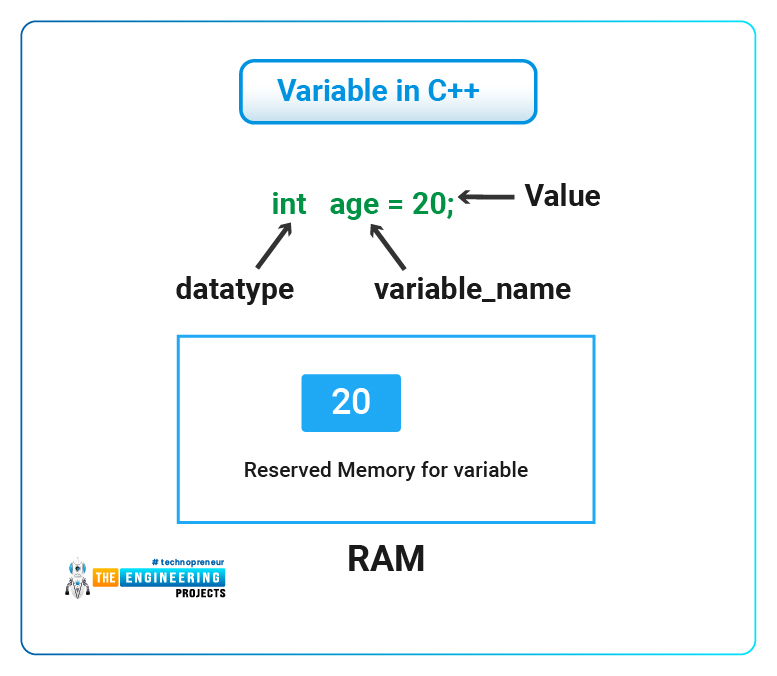
- Name of variable: an identifier that represents a memory location.
- Address of variable: memory location of the variable.
- Contents of variable: the value stored in a memory location.
Variable Declaration:
- Variable declaration is the process of specifying the name and the type of variable.
- Before a variable is used, it can be declared anywhere in the program before its execution.
- Information is provided to the compiler about the variable by variable declaration.
- The compiler determines the memory required for each variable.
- After the declaration, the required memory of bytes is allocated to variables.
- Once the variable is declared then its name and data type can not be changed but its value can be changed during execution.
- For example, an integer required 2 bytes of memory but a character required one byte of memory. During execution int and char data types can not be changed but integer value can be changed from 5 to 9 and character can be changed from ‘f’ to ‘g’.
SYNTAX of declaring a variable in C++
- data-type: type of data that is stored in a variable.
- variable-name: tell us about the memory location of the variable.
For example:
int num1=25, num2=1000;We can also write it like this:
int num1,num2; num1=25; num2=1000;
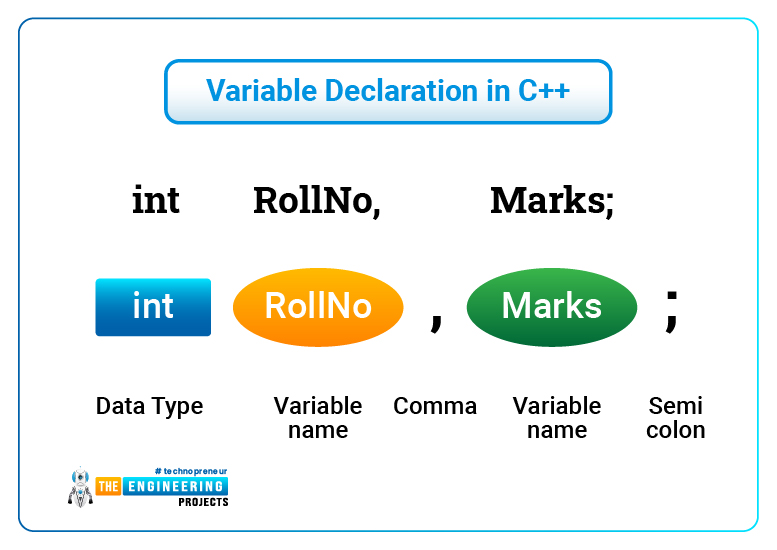
Types of Variables
Types of Variables can be categorized in two ways
- Types of variables regarding data types
- Types of variables regarding the scope
Types of variables regarding data type:
Based on the data type of variable, there are the following categories of variables in C++
- int: holds an integer value i.e. 1, 2, 3, 50 etc.
- char: holds character values like ‘c’, ‘F’, ‘B’, ‘p’, ‘g’, ‘q’ etc.
- bool: holds boolean value true or false(0 or 1).
- double: holds double-precision floating-point value.
- float: holds single-precision floating-point value.
Example
Different types of variables can be declared as follow:
- int marks;
- float average;
- char grade;
- double salary;
Variables having the same data type can be declared in a single line. Each variable in line should be separated by a comma as follows:
int a, b, c;
Types of variables regarding scope
When we discussed the Hello Program, we have seen the curly braces in the program like this:
int main { //Some code }
When variables are declared in curly braces then their scope is limited within these braces. The variable declared in main() function can not be used outside the main() function.
There are two types of variables based on their scope.
- Global variable
- Local variable
Global Variable
Global variables are always declared outside of any function.
- Their scope is in the complete program so can be accessed anywhere in the program.
- Can be accessed in the main() function, in the user-defined function and anywhere in the program.
Global variable example
Let us have a global variable myvar and it is declared outside of main. We can access the variable twice in the main() function easily.
#include <iostream> using namespace std; // This is a global variable char myvar = 'G'; int main() { cout <<"Value of myvar: "<< myvar<<endl; myVar='m'; cout <<"Value of myvar: "<< myvar; return 0; }Output:
- Value of myvar: G
- Value of myvar: m
Local variable
- They are declared inside the curly braces of any loop or control statement, main function, user-defined function and their scope is limited only to the curly braces so they can be used only within the curly braces.
Local variable example
#include <iostream> using namespace std; char myFuncn() { // This is a local variable char myVar = 'X’; } int main() { cout <<"Value of myVar: "<< myVar<<endl; myVar='H’; cout <<"Value of myVar: "<< myVar; return 0; }Output:
- As we are trying to access the variable outside its scope so a compile-time error will occur.
- Local and global variables may or may not have the same name.
- We can see an example having the same name for local and global variables.
#include <iostream> using namespace std; // This is a global variable char myVar = 'X'; char myFuncn() { // This is a local variable char myVar = 'Y’; return myVar; } int main() { cout <<"Funcn call: "<< myFuncn()<<endl; cout <<"Value of myVar: "<< myVar<<endl; myVar='A'; cout <<"Funcn call: "<< myFuncn()<<endl; cout <<"Value of myVar: "<< myVar<<endl; return 0; }Output:
Funcn call: X
Value of myVar: Y
Funcn call: X
Value of myVar: A
As the scope of the local variable is limited so the changed value of the variable is only responded for the global variable and the value of the local variable remained the same ‘ X’.
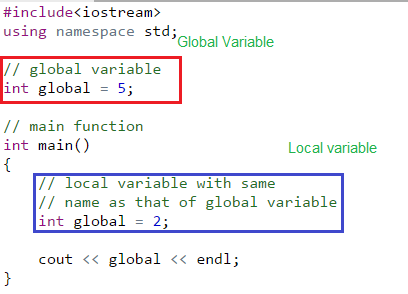
RULES FOR DECLARING VARIABLES:
There are some rules in C++ for naming variables- Variable can be a letter, number and underscore(-).
- Variable’s first letter should be a letter or underscore. The variables 6second, $6payment and 5kg are invalid.
- In the variable name, blank spaces can not be added. ‘ my var’ is an invalid variable.
- Both upper and lower cases can be used. User-defined variables are written in lower and constants are written in upper case conventionally.
- special symbols can not be used in variables as a name.
- Reserved words can not be used as a variable name. e.g int, for, while etc can not be used as a variable name.
- The no of characters in the variable should not be more than 31.
- A variable can not be declared for more data types. It should be declared for only one data type.
- The variable declaration tells us about the part where the variable was first declared before its use.
- While variable definition tells us about that part where variable assigned memory location and value
- Variable definition and declaration did at a time mostly.
#include <iostream> using namespace std; int main() { // declaration and definition of variable 'b123' char b123 = 'a'; float b; // multiple definitions and declararions int _c, _d45, e; cout << b123 << endl; return 0; }
Variable Initialization:
Variable initialization is the process of assigning a value to the variable.- = sign is used to initialize a variable
- The variable name is written on the left side of = and the value is given on the right side of =.
- Compiler allocated some memory to the variable when it is declared.
Syntax
type_ name variable=value;
- type_name: indicates the data type of the variable
- variable: name of the variable
- = assignment operator used to initialize a variable
- value: value to initialize a variable
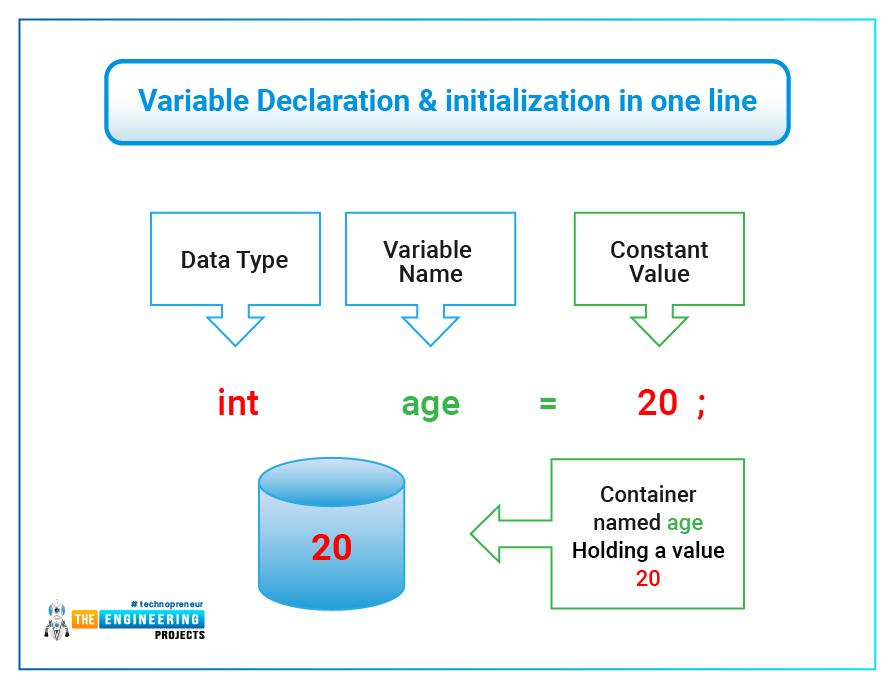
Example
#include <iostream> using namespace std; int main() { int a = 10; int b; cout << " value of variable a : "<< a; // static initialization cout << "\nEnter the value of variable b : "; // dynamic initialization cin >> b; cout << "\n value of variable b : "<< b; return 0; }
Output
value of variable a : 10 Enter the value of variable b : 18 value of variable b : 18
CONSTANTS IN C++
- Constants are those quantities that cannot be changed during program execution.
- It is used in the program wherever it is needed.
- Literal constant
- Symbolic constant
Literal constant
It is typed directly in the program and used when needed. Example- cout<<” Hi guys”;
- int age = 19;
TYPES OF LITERAL CONSTANTS:
There are 5 common types of literal constants are used in C++- Integer constant
- Floating-point constant
- Character constant
- String constant
- Bool constant
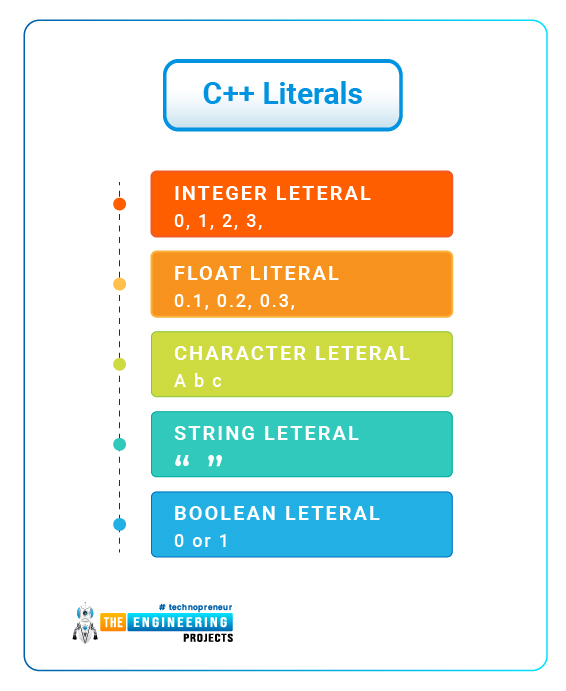
Integer literal constant
It can be a decimal, hexadecimal or octal constant. Examples:- 85 // decimal
- 0213 // octal
- 0x4b // hexadecimal
- 30 // int
- 30u // unsigned int
- 30l // long
- 30ul // unsigned
Floating-point literals
Floating-point literal constant contain four parts- Integer part
- Decimal part
- Fractional part
- Exponent part
Examples
- 3.14159 // Legal
- 314159E-5L // Legal
- 510E // Illegal: incomplete exponent
- 210f // Illegal: no decimal or exponent
- .e55 // Illegal: missing integer or fraction
Character constants
- These constants are enclosed in single quotes
- Upper case letters are stored in wchar_t type and lower case letters are stored in char type
- A character literal can be a simple character (e.g., 'x'), an escape sequence (e.g., '\p’), or a universal character (e.g., '\u0C0').
- The characters with backslash have special meanings. These characters with a backslash are given below.
Escape sequence | Meaning |
\\ | \ character |
\' | ' character |
\" | " character |
\? | ? character |
\a | Alert or bell |
\b | Backspace |
\f | Form feed |
\n | Newline |
\r | Carriage return |
\t | Horizontal tab |
\v | Vertical tab |
\ooo | Octal number of one to three digits |
\xhh . . . | Hexadecimal number of one or more digits |
Example
#include <iostream> using namespace std; int main() { cout << "Hello\tWorld\n\n"; return 0; }
Output
Hello WorldString constants
They are enclosed in double-quotes. It may contain- Characters
- Escape sequence
- Universal character
Examples
"hello, dear" "hello, \ dear" "hello, " "d" "ear"
Program for defining a constant
#include <iostream>
using namespace std;
#define VAL1 20
#define VAL2 6
#define Newline '\n'
int main()
{
int tot;
tot = VAL1 * VAL2;
cout << tot;
cout << Newline;
}
Programs
#include <iostream> using namespace std; #define LENGTH 5 #define WIDTH 20 #define NEWLINE '\n' int main() { int area; area = LENGTH * WIDTH; cout << area; cout << NEWLINE; return 0; } Program # 2 #include <iostream> using namespace std; int main() { const int SIDE = 40; int area; area = SIDE*SIDE; cout<<"The area of the square with side: " << SIDE <<" is: " << area << endl; return 0; }
Bool constants
Three keyword literals exist in C++: true, false and nullptr:- For Boolean variables, we have true or false literals/ constants.
- nullptr indicates the null pointer value.
1 2 3 | bool foo = true; bool bar = false; int* p = nullptr; |
Symbolic constants
- It is the Symbolic name given to the Symbolic values that cannot be changed
- A Symbolic constant PI having the value 3.141593 is used where needed and its value can not be changed.
- const Qualifier
- define Directive
const <Data_Type> <Variable_Name>The syntax for define directive
<Return_Type> <Function_Name>() constExample
#include <iostream> using namespace std; const int a = 100; // Const Variable class TestConst { public: void display() const // Const Function/ define directive { cout << "Value of a in the const function is " << a; } }; int main () { Test int1; int1.display(); cout << a; return 0; }
Comparison table for Constants and Variables
We have discussed above the clear understanding of variables, their types and some programs to make grip on the topic. We have also discussed constants and their types and some programs to make ideas clear. At the end, we make a clear comparison between constants and variables.
In the next section, we will discuss operators, expressions and comments in C++. Till then take care and have fun. Thanks for reading.