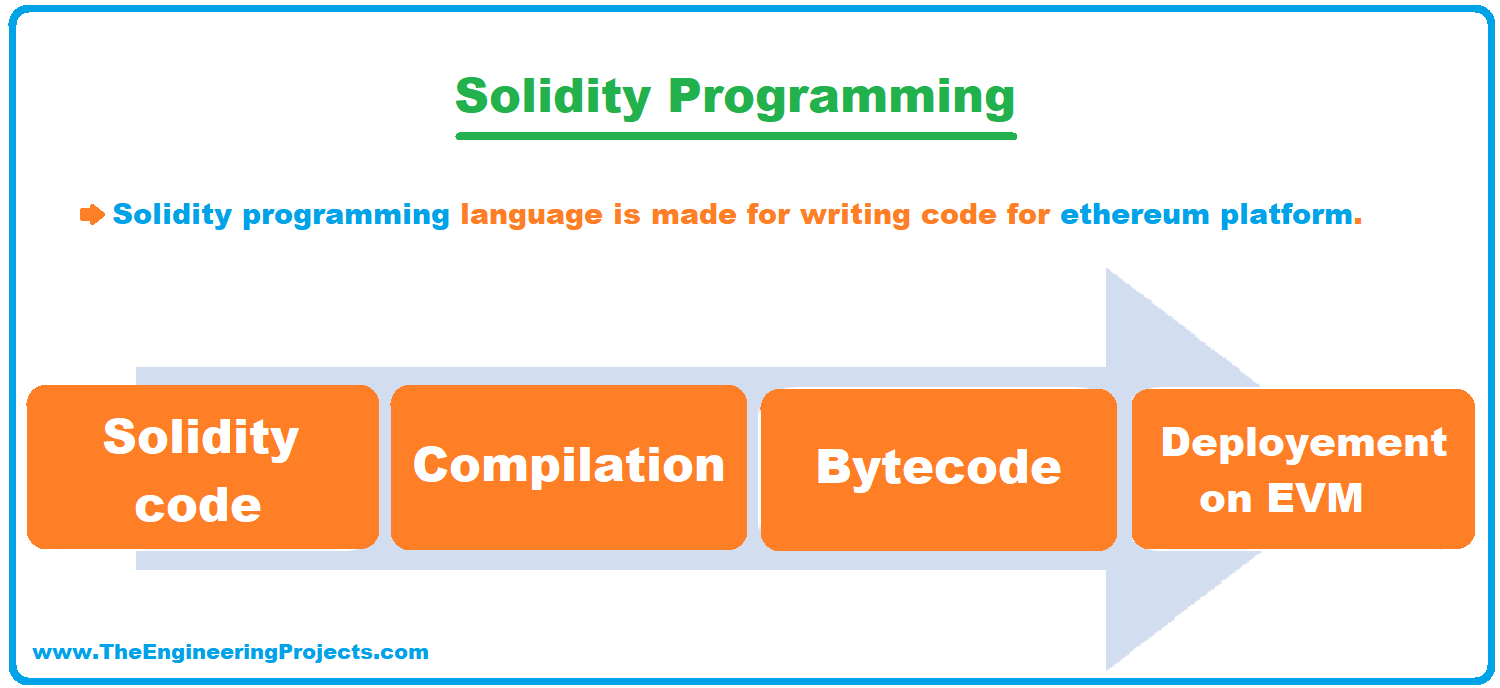
Solidity Programming
- Solidity programming language is made for writing code for the Ethereum platform. These codes (smart contracts) help in executing different functions in Ethereum virtual machine (EVM).
- It is easy to learn the language. If you are familiar with C and JavaScript, you can easily learn solidity.
- The code written in solidity is converted into bytecode by solidity compiler. The compiler of solidity language is solc.
- The extension of a solidity file is .solc.
- It is a case-sensitive language.
- Solidity is a statically typed language.
- The process of writing and compilation is shown in the figure below.
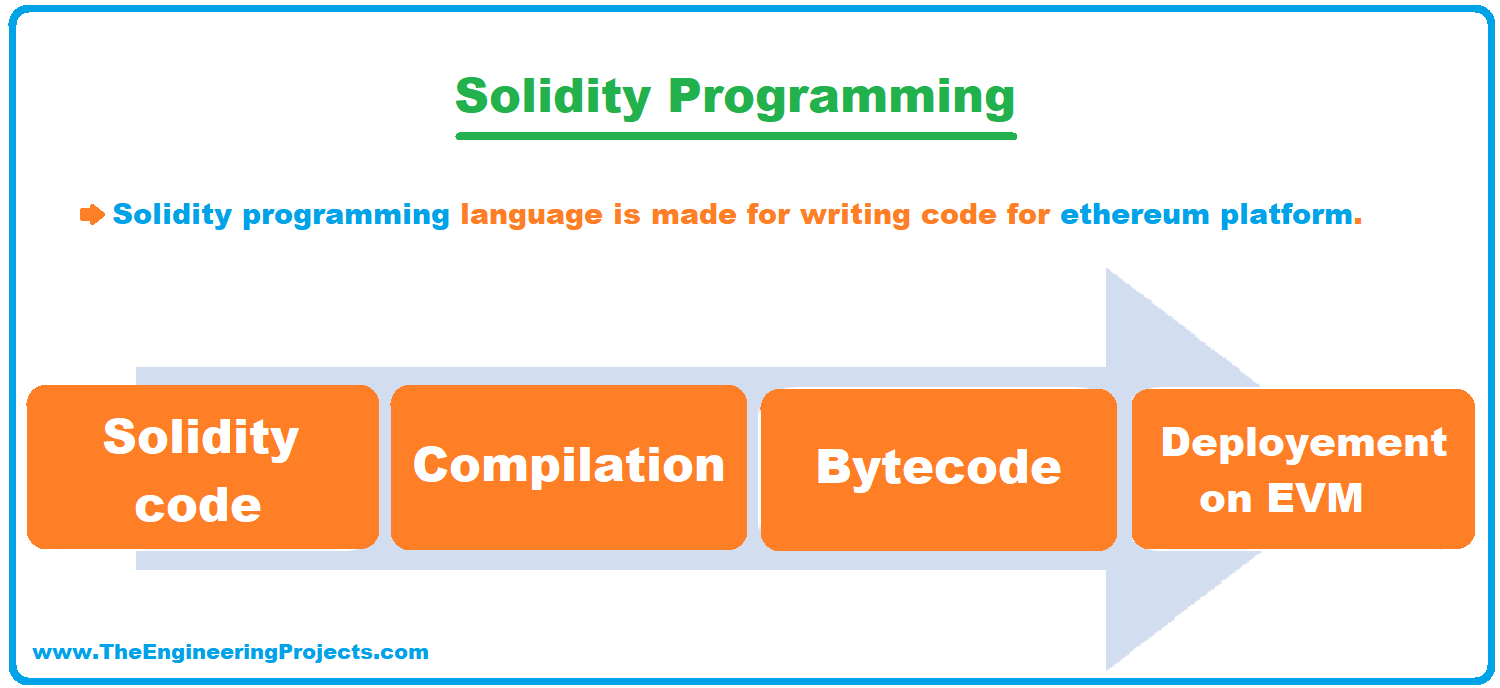
The layout of a Solidity File
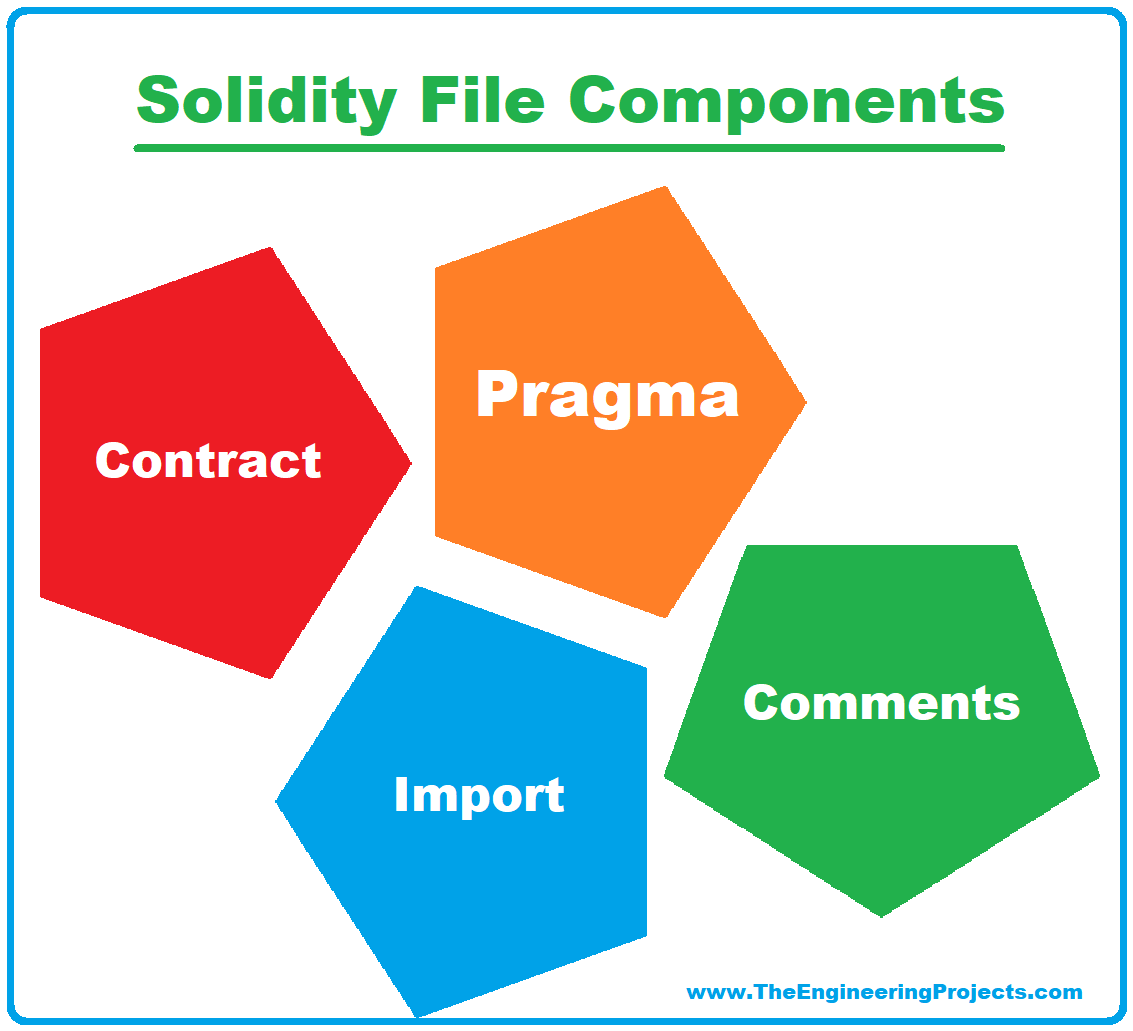
- Pragma
- Comments
- Import
- Contract / Library
Pragma in Solidity Programming
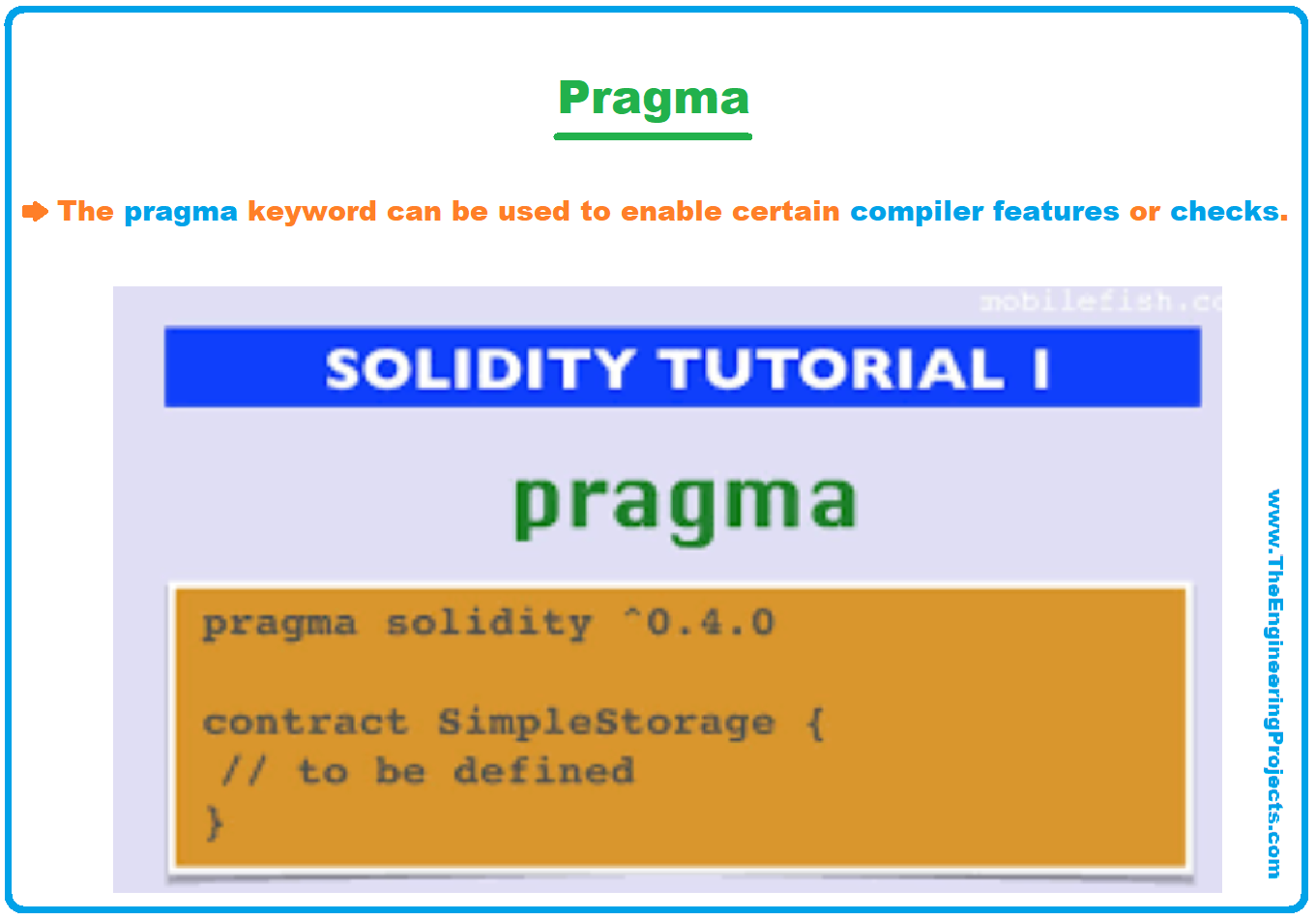
- Before writing the solidity program, you want to mention the version of solidity compiler. So that anyone using the file can choose the correct version for compilation.
- For this purpose, pragma directive is used. It specifies the compiler version of the file.
- Solidity is still growing and a lot of improvements have been made since its development. Therefore, the version keeps on improving.
- The current solidity version is 0.7.4.
- The version is mentioned by using the following syntax.
pragma Solidity ^0.7.0;
I should mention here that semi-colon is the statement terminator in solidity.- It is declared as the first statement of the program.
- The version number comprises a major build number and a minor build number. When you use ^ character with version number, it means the latest version of the major build number.
- For example, as I declared the version 0.7.0 with ^ character. Here major build number is 7. So solidity compiler of version 7 will compile it.
Comments in Solidity Programming
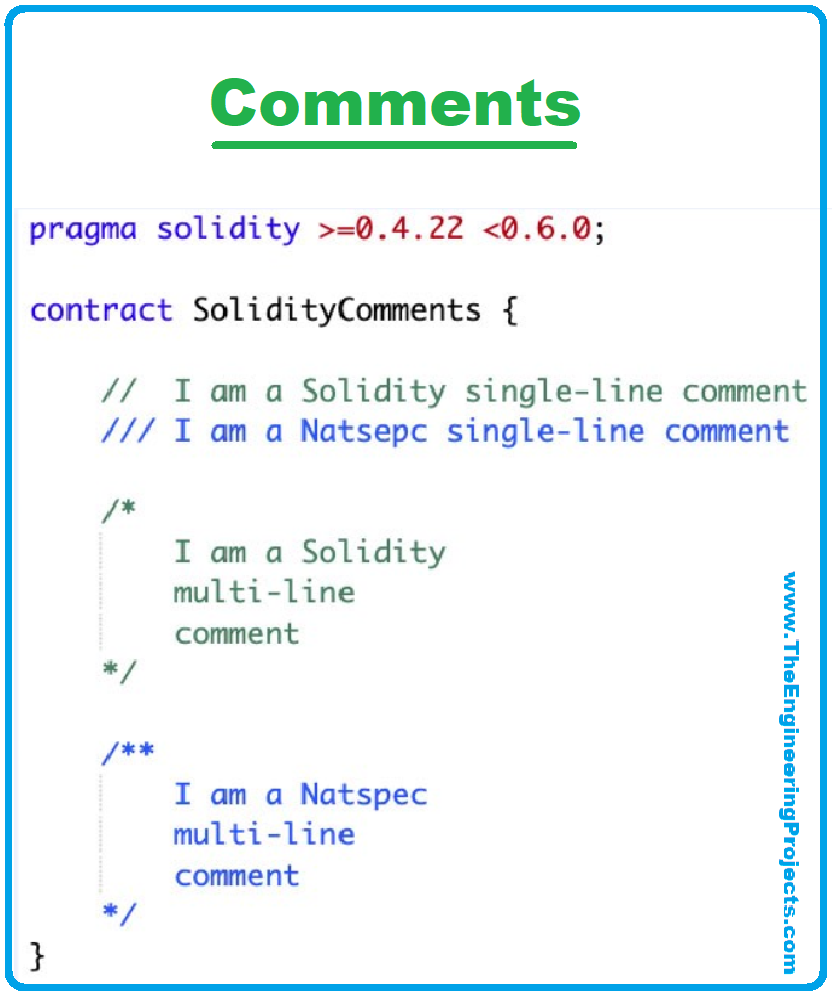
- Guys, if you are familiar with programming, you must know that programming languages support the option of commenting. Solidity also provides this facility.
- There are two types of comment in solidity:
- Single-Line Comments: single-line comment means a comment comprising of one line only.
- Multi-Line Comments: multiline comments consist of more than one line.
- Forward slashes are used for denoting comments. Single line comment is denoted by double forward slash //. While multiline comment starts with /* and ends with */.
- It is a good practice to add comments to your program. This improves readability.
Import in Solidity Programming
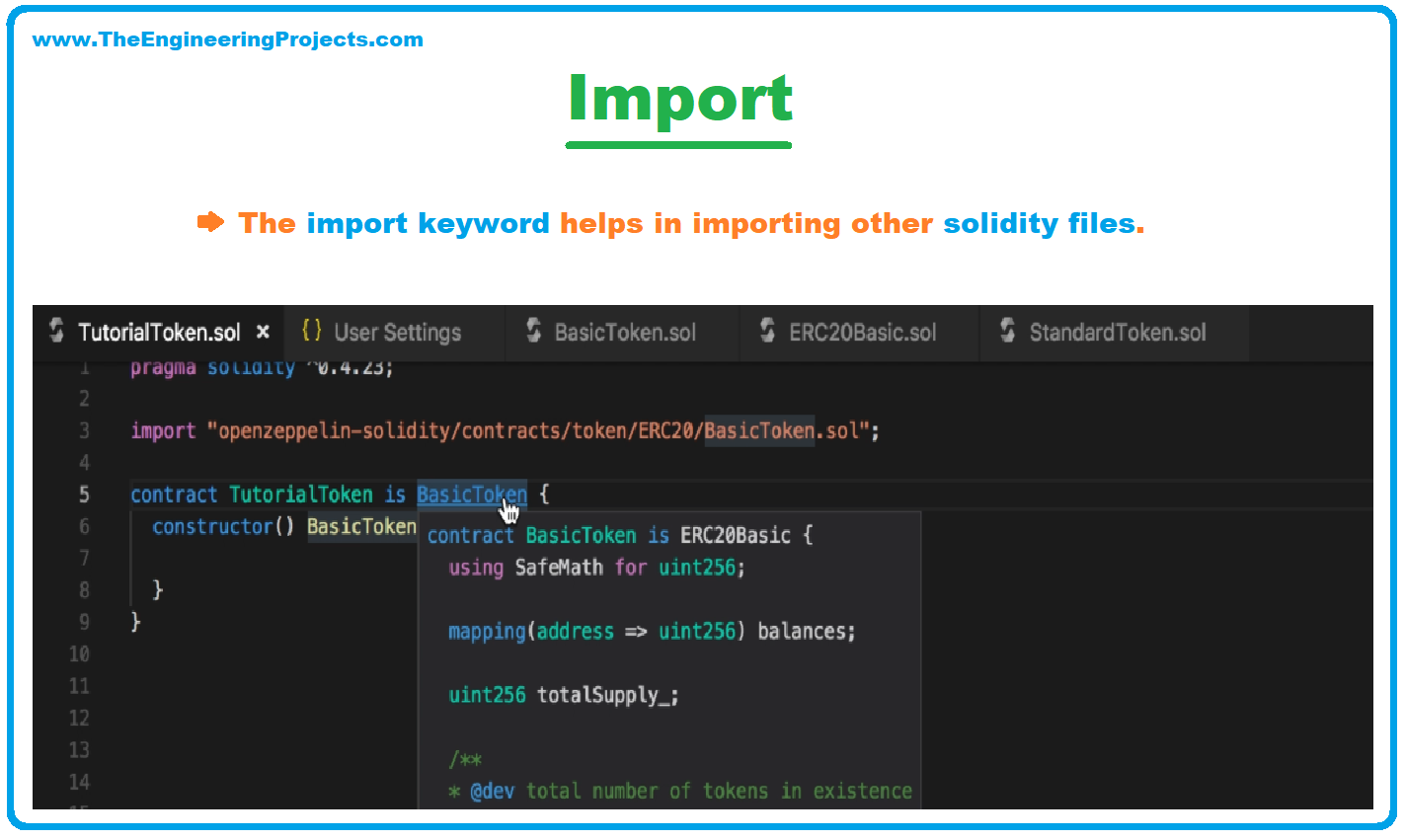
- The import keyword helps in importing other solidity files. These files can then be used in code.
- The functions of the imported file can then be used in a solidity file.
- This functionality helps us in writing code in the form of modules. In this way, lengthy code can be converted into smaller readable ones.
- The syntax for using the import keyword is given below:
import filename;
- The address of the file is mentioned within the import statement.
Contract / Library in Solidity Programming
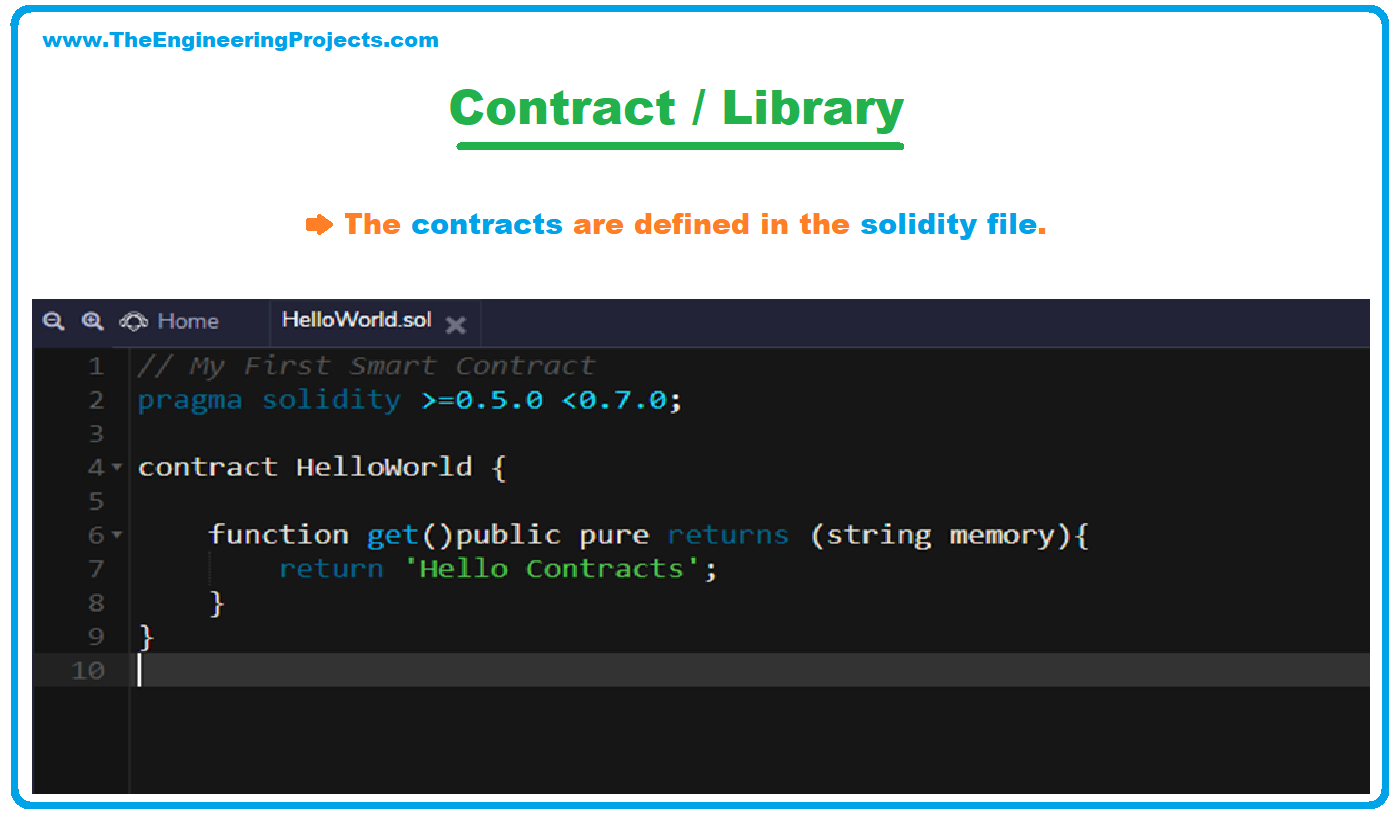
- The contracts are defined in the solidity file.
- You can define more than one contract or library in one file.
- Contracts, libraries and interfaces can be defined one after the other in a file.
Structure of a Contract
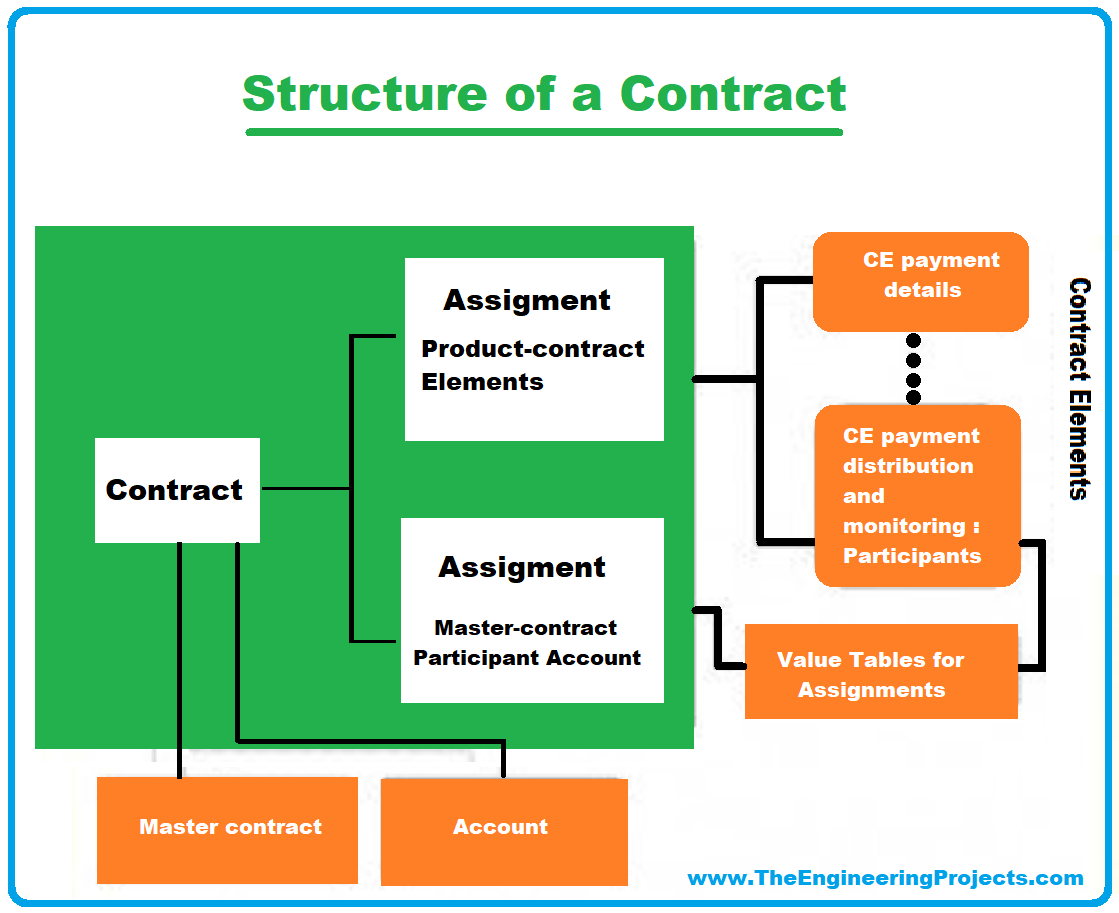
- State Variables
- Structures
- Modifier Functions
- Event Declarations
- Enumerations
- Functions
State Variables
- Variables store values in storage locations. The value saved in a variable can be change or update while programming execution.
- Once a variable is declared, you can use it anywhere in your program, at multiple locations.
- State variables are stored in Ethereum blockchain permanently. The changes in their value and their current value is secured.
- The data type of state variable is declared while writing the program.
- Other qualifiers, used while declaration, are listed below:
- Internal
- Private
- Public
- Constant
- The following data types can be used in solidity:
- Bool for boolean
- Uint / int for unsigned / signed integer
- Bytes for 8 bit signed integer
- Address for addresses of accounts
- Mapping for mappings
- Enum for enumerations
- Struct for structures
- String for character strings
Structure
- With the help of structure, you can define a data type of your own.
- A structure is a composite data type and that means it can contain different variables having different or same data types.
- A group of variables is defined in a structure. Each one is given a name and a data type.
- A structure is declared by using the struct keyword.
Modifier
- A modifier is a function that can change the behavior of the code.
- In solidity, modifiers are used with functions, so they can change the actions of a function.
- If you are calling a solidity function that has a modifier associated with it, then the modifier function will execute first. After its execution, the called function would be executed.
- You will define modifier function only once in the program but you can use it anywhere with multiple functions.
Events
- If you are familiar with programming, you know that different programming languages support events.
- Solidity also supports events. Events tell about the change in the state of a contract.
- With the help of logs, the caller can view the state of the contract after execution.
- Events are declared outside functions, at the global level. Then these events can be called in any function.
- The keyword for event declaration is an event. After that, the identifier is declared followed by a parameter list. The parameter list contains the data type of the variables.
Enumeration
- Enumeration provides us with an interesting facility. With the help of it, you can define a data type of your own choice.
- It contains a list of constants, from which the variable can take values.
- Each value is given an integer starting from 0 for solidity.
- The enumeration is declared by the enum keyword. After the keyword, the identifier is defined followed by the list of constant values in brackets.
- There is no semi-colon at the end of the enum declaration statement.
Function
- Just like any other programming language, a function is a key component of solidity.
- Whenever a transaction is initiated, a function of a smart contract is called.
- Functions can read and write state variables. When a function is executed and a transaction is processed, the state variable may change its state.
- A function can take input parameters, perform operations on them and can return values. The return values can be more than one.
- A function has a name that is its identifier. This identifier can be used anywhere in the program for the execution of functions.
- You can use various qualifiers with a function declaration that decides the visibility of a function. These qualifiers are listed below:
- Public
- Private
- Internal
- External
- Other than visibility qualifiers, some other qualifiers are also used with functions. These show the ability of the underlying function in terms of changing values of state variables. The qualifiers are given below:
- Constant
- View
- Pure
- Payable