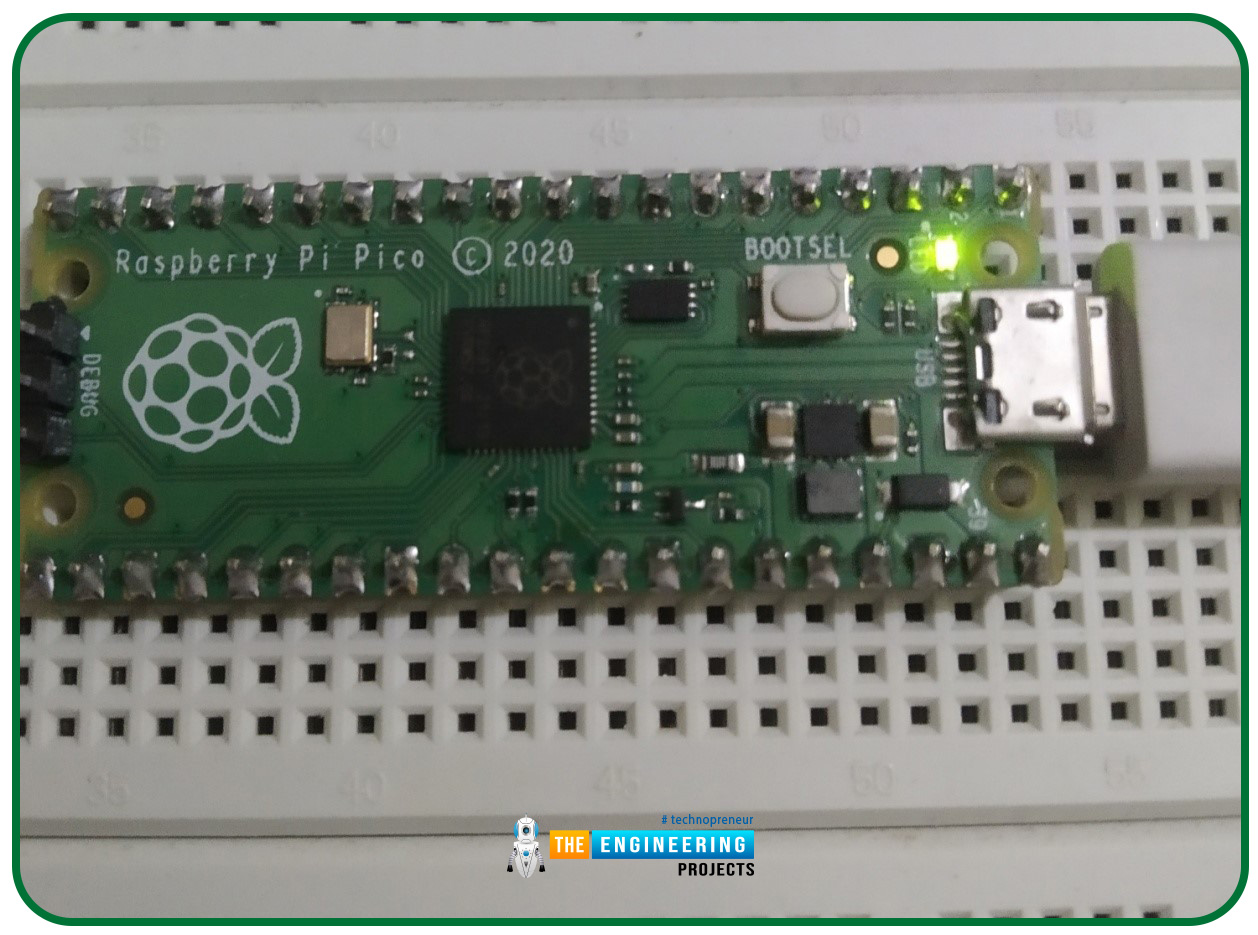
Hello readers, I hope you all are doing great. In our previous tutorials on raspberry pi Pico, we discussed the basic features, architecture, download and installation of development environments for raspberry Pi Pico programming. In this tutorial, we will learn how to access and control Raspberry Pi Pico and its GPIO pins to implement LED blinking using MicroPython.
Introduction
Raspberry Pi development boards are quite popular and frequently used among students and hobbyists. But the drawback of Raspberry Pi is their cost i.e., around $35-$45. So when cost of the development board is of prime importance the users prefer to use ESP8266, ESP32, Arduino, PIC etc. over Raspberry Pi. To overcome this drawback, Raspberry Pi foundations designed a new board i.e., Raspberry Pi Pico which is a cost effective solution for costlier Raspberry Pi boards.
Raspberry Pi Pico is a cost-effective development platform designed by Raspberry Pi which has enough processing power to handle complex task. It is a low cost and yet powerful microcontroller board with RP2040 silicon chip.
Prerequisites
- Raspberry Pi Pico Module
- Latest version of Python
- Latest version on Thonny IDE should be installed on your system
- MicroPython setup installed in Raspberry Pi Pico
We have already published tutorials on how to download and install the above mentioned software components.
Follow the given link for detailed study of Raspberry Pi Pico: https://www.theengineeringprojects.com/2022/04/getting-started-with-raspberry-pi-pico.html
Programming Raspberry Pi Pico
To program Raspberry Pi Pico board there are various development environments available (like uPyCraft IDE, Visual Studio Code, Thonny IDE etc.) and multiple programming languages as well. In this tutorial, we are going to use Thonny IDE to program the Raspberry Pi Pico board.Installing Thonny IDE for Raspberry Pi Pico Programming:
We have already published a tutorial on installing Thonny IDE for Raspberry Pi Pico Programming. Follow the given link to install the IDE: https://www.theengineeringprojects.com/2022/04/installing-thonny-ide-for-raspberry-pi-pico-programming.html
The Raspberry Pi Pico board comes with an onboard LED which is internally connected with GPIO 25. So first we will discuss how to access the on board LED and then will interface an external one.
Controlling (Blinking) Raspberry Pi Pico’s on board LED:
- Open the Thonny IDE.
- Connect your Raspberry Pi Pico board with your system (laptop or desktop).
- To create a new project; go to Files >> New.
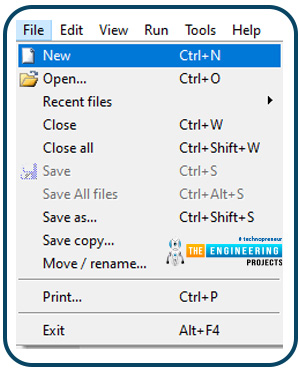
Fig.
- Write the program to blink the inbuilt LED with a particular delay.
Programming
Steps included in programming the Raspberry Pi Pico to make the onboard LED to blink are:
- Importing necessary files from machine
“from machine import Pin, Timer”
- The onboard LED is internally connected to the GPIO 25 of the raspberry Pi Pico board. So, the next step is creating LED object from pin 25 and setting the GPIO25 as output pin.
“led = Pin(25, Pin.OUT)”
- Toggle the LED: toggle() command is used to blink the onboard LED.
“ led.toggle()”
- Save the program.
- Save the program. We can save the program either on computer or on Raspberry Pi Pico board.
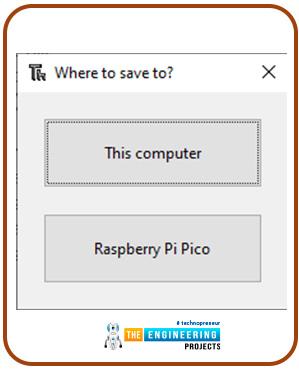
Fig. Save the program
- Run the code.
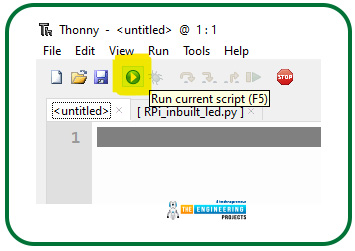
Fig. Run the code
Note: If you are already running a program on Raspberry Pi Pico board then press ‘Stop’ before running a new program.
Code to blink onboard LED
from machine import Pin, Timer
led = Pin(25, Pin.OUT)
timer = Timer()
def blink(timer):
led.toggle()
timer.init(freq=2.5, mode=Timer.PERIODIC, callback=blink)
Result
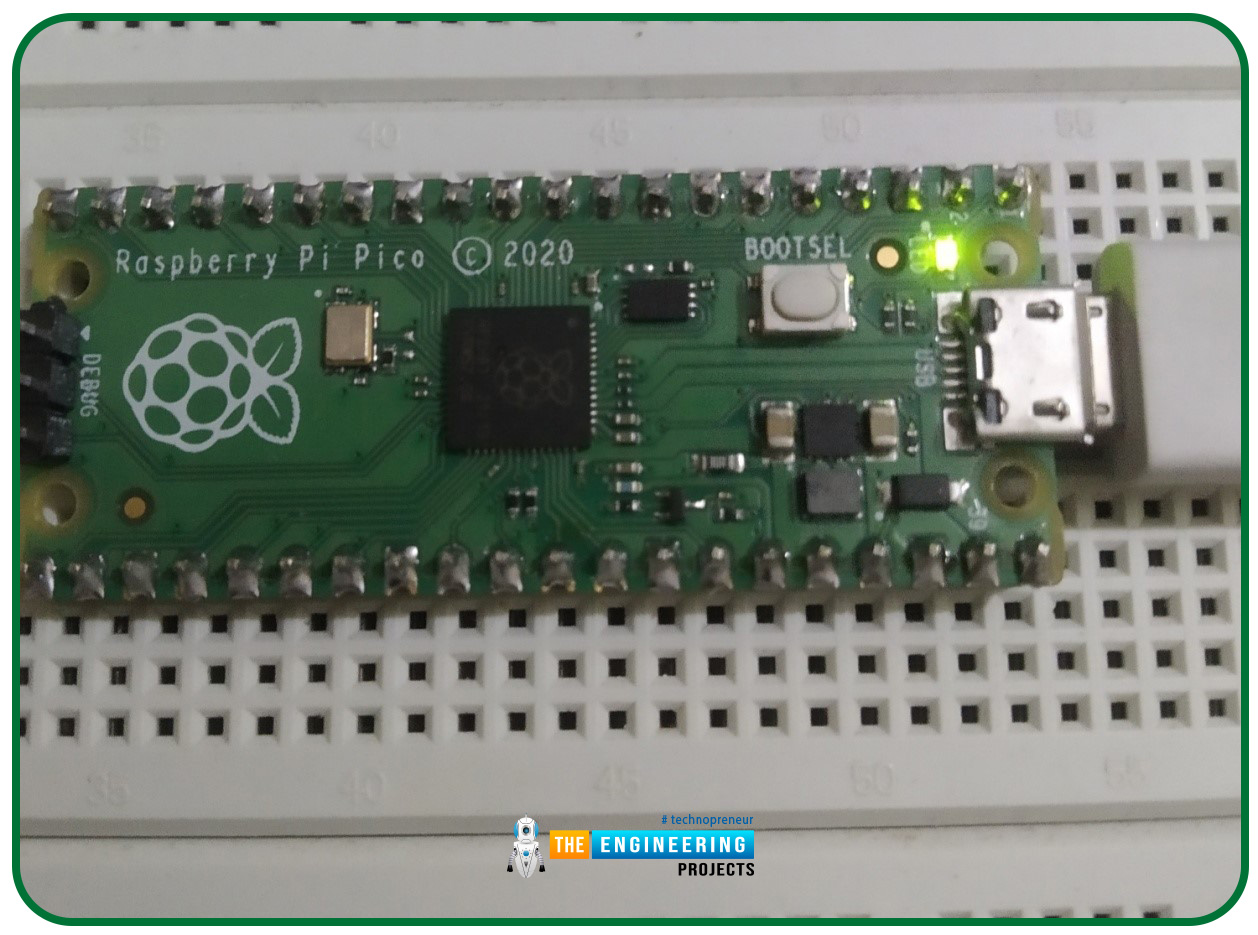
Fig. Blinking On-board LED
Interfacing peripherals (LED) with raspberry Pi Pico
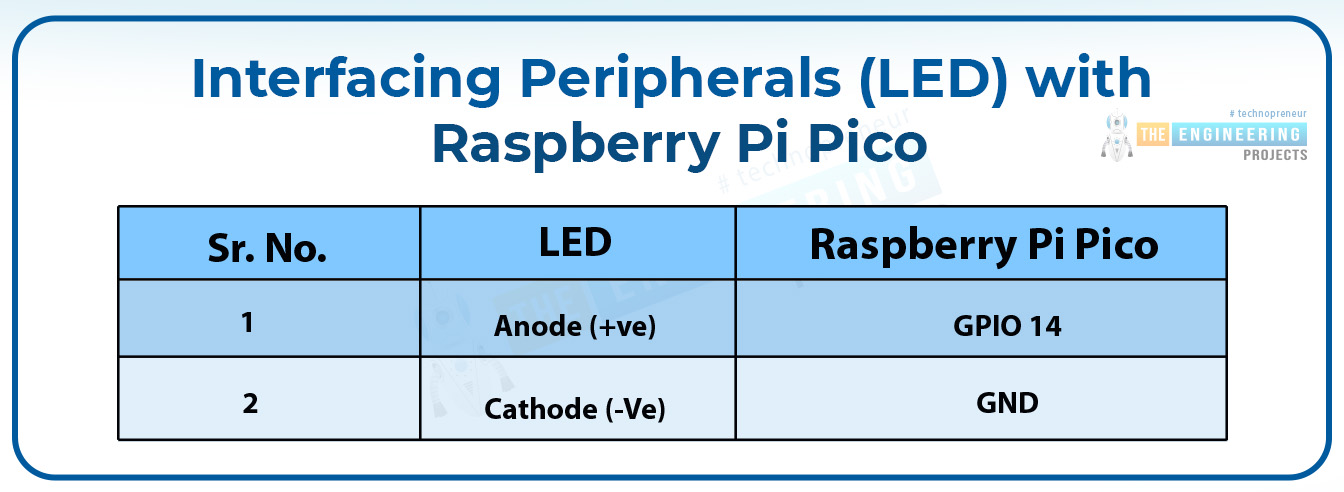
Table 1
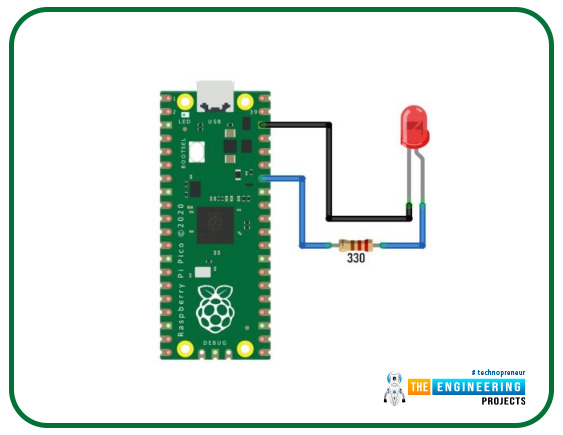
Fig. Iterfacing LED with Raspberry Pi Pico
Programming
Steps included in programming the Raspberry Pi Pico to make the peripheral LED to blink are:
- Importing necessary files from machine: We are importing two files one is to access GPIO pins and another for accessing timer functions.
“from machine import Pin”
“import time”
- Create LED object: We have multiple GPIOs available where we can interface peripheral LED. So we are using GPIO_14 of Raspberry Pi Pico board.
Create an object ‘led’ from pin14 and set GPIO pin14 to output.
“led=Pin(14,Pin.OUT)”
- Making the onboard LED to continuously blink: We are using the ‘while loop’ to make the inbuilt led blink continuously.
“while True:"
- Setting LED high: The ‘value()’ command is used to set the peripheral LED as high where we are passing ‘1’ as an argument for HIGH output.
“led.value(1)”
- Adding delay: A delay of 1 second is added to make the led blink.
“time.sleep(1)”
- Setting LED Low: Argument ‘0’ is passed inside ‘led.value()’ command to set the led as LOW.
“led.value(0)”
- Save the program either on Raspberry Pi Pico board or on your system..
- If you are already running a program on Raspberry Pi Pico board then press ‘Stop’ before running a new program.
Result
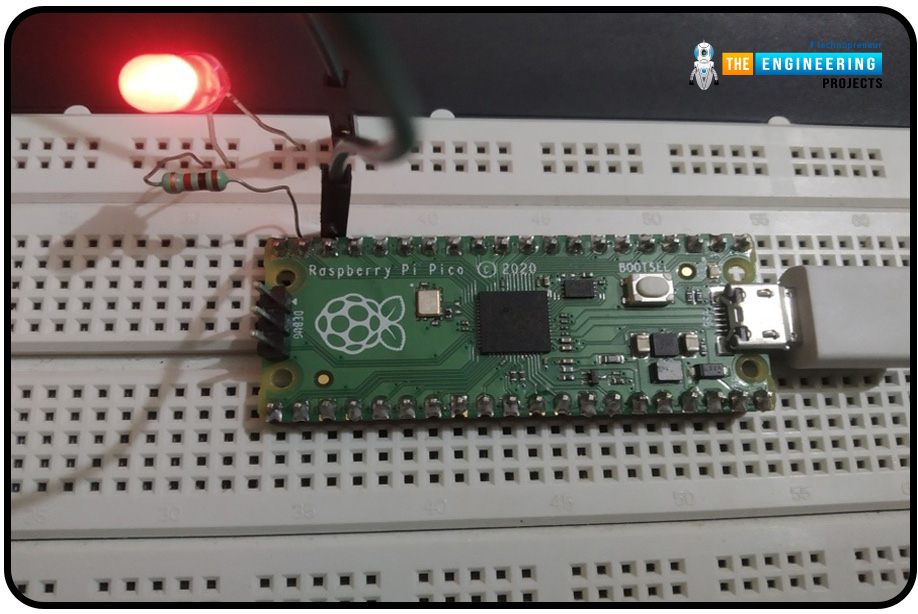
Fig. Interfacing and Blinking LED with raspberry Pi Pico
Code
from machine import Pin, time
led=Pin(14,Pin.OUT) #Creating LED object from pin14 and Set Pin 14 to output
while True:
led.value(1) #Set led turn ON
time.sleep(1)
led.value(0) #Set led turn OFF
time.sleep(1)
Conclusion
This concludes the tutorial, we hope you find this of some help and also hope to see you soon with a new tutorial on Raspberry Pi Pico programming.