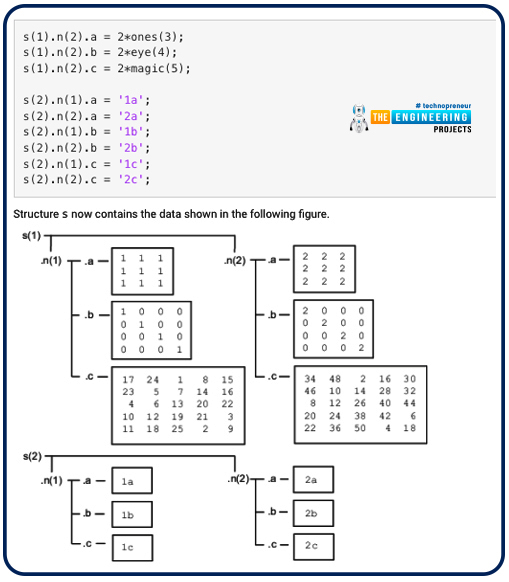
Hello friends. In this lecture, we are going to have a look at the different kinds of MATLAB data types.
As we have already seen in previous lectures, MATLAB stands for MATrix LABoratory and allows us to store numbers in the form of matrices.
Elements of a matrix are entered row-wise, and consecutive row elements can be separated by a space or a comma, while the rows themselves are separated by semicolons. The entire matrix is supposed to be inside square brackets.
Note: round brackets are used for input of an argument to a function.
A = [1,2,3; 4,5,6; 7,8,9];
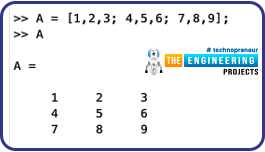
An individual element of a matrix can also be called using ‘indexing’ or ‘subscripting’. For example, A(1,2) refers to the element in the first row and second column.
A larger matrix can also be cropped into a smaller matrix, as we can see in the example below.
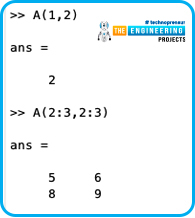
A scalar is just a special case of a matrix and its element size is 1x1. Square brackets are not needed to create a scalar variable. Also, a vector is a special case of a matrix with a single row or column. Square brackets without an element inside them create a null vector. We see examples of this in the code below:
- u = [1 2 3]; %Produces a row vector
- v = [1;2;3]; %Produces a column vector
- X = []; %Produces a null vector
Elements of a matrix can be all kinds of numeric datatypes, whether they are floating-point, integers, or imaginary numbers, as we will see in the next section. They can even be symbolic.
DataTypes of MATLAB
Every variable that is stored in the workspace of MATLAB has a datatype. It can be an in-built or default datatype or users can build their own datatypes depending on the purpose of the code.
You can always find a datatype in MATLAB by using the 'class’ function.
Numeric datatypes:
Double:
- This datatype takes 64 bits to store a number. This essentially represents a floating-point number, i.e., a decimal number with double precision, and can be positive or negative. You can use the function ‘double’ to declare the datatype when creating a variable, but double is the default datatype in MATLAB and whenever you call a command such as the one shown below, the number is stored as a double.
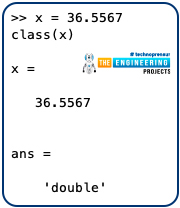
- These variables can store values varying from between -1.79769 x 10308 and -2.22507 x 10-308 for the negative numbers and the range for positive numbers is between 2.22507 x 10-308 and 1.79769 x 10308 In situations where such large values of numbers are not needed, single-precision floating-point variable can be used.
Single:
- When storing smaller numbers, it is better to use the single-precision floating-point numbers which vary between-3.4x10^38 and 3.4x10^38. This stores a variable in only 32 bits and helps to speed up the code. We use the function single in order to create this kind of variable.
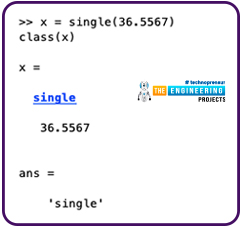
Integers:
- MATLAB supports four types of signed integers and four types of unsigned integer datatypes, given by, int8, int16, int32, and in64 for the signed integers, and uint8, uint16, uint32 and uint64 for the unsigned integers. The number refers to the number of bits required to stored these integers and the range of allowed numbers is decided accordingly.
Complex numbers:
- You can create a complex number by using the ‘complex’ function or using the default symbol ‘i’ for the imaginary part of the complex variable. We can also extract the real and imaginary parts of the complex number using the ‘real’ and ‘imag’ functions on the complex number.
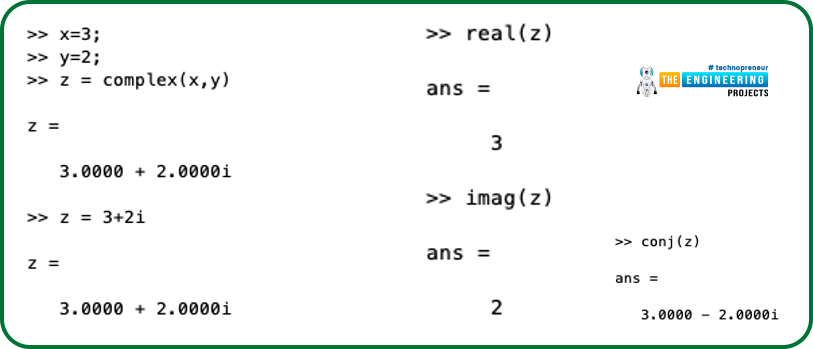
Infinity and Nan:
- Any number larger than the range given above is represented in MATLAB by the value ‘Inf’. Such a number can result when we divide by zero, which leads to results too large to represent as floating-point values and they end up being outside of the ranges discussed above. We can check if a variable is an infinity or not by using the function ‘isinf’ on it. Some numbers that can’t be represented by a real number such as the result of calculating ‘0/0’ or ‘inf/inf’ are called NaN, i.e., “Not a Number”. We can check if a variable is NaN or not by using the function ‘isnan’ on it.
Logical:
- These are variables that only have values of 0 and 1, and are the result of a comparison operation. An example of a result of comparing two matrices that gives a logical matrix as an output is shown below:
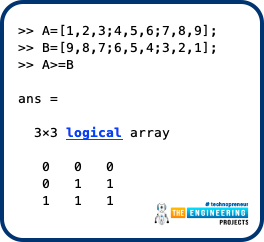
Representation
We can represent the output of a double in a shorter format which is easy to read or in a longer format which will help with learning about the accuracy of those double variables, using the commands below:- format short
- format long
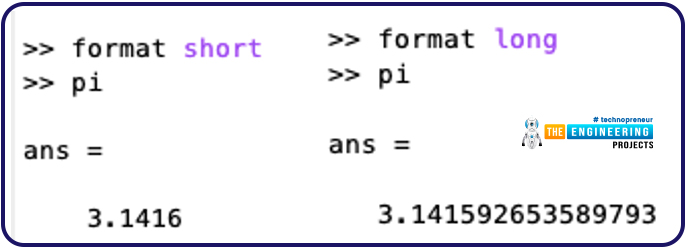
Conversion of numeric datatypes:
We can convert between double or single-precision numbers, as well as from floating-point to integers or vice versa using the functions described above which we use while declaring the numeric data type.Operations on numeric datatypes:
Following is a list of operations on the numeric datatypes.
- abs: to determine absolute and positive value of a signed or complex number.
- Fix: round-off a floating point number towards 0. For example, fix([-2.1, 2.9]) = [-2, 2]
- floor: round-off a floating point number towards –Inf. For example, floor([-2.1, 2.9]) = [-3, 2]
- ceil: round-off a floating-point number towards +Inf. For example, ceil([-2.1, 2.9]) = [-2, 3] round: round-off a floating-point number towards the nearest integer. For example, round([-2.1, 2.9]) = [-2, 3]
- Rem: Remainder after division of first argument by second argument. For example, rem(10,3)=1
- Sign: signum function returns the sign of a number.
Other datatypes:
Characters/strings:
- The character or string array is used to store text data in MATLAB.
Single quotes are used to declare a character array. For example,
A = ‘Hello World’ is a character vector.
However, double quotes are used to declare a string. String is different from a character because individual parts of a character array can be accessed using indexing but the same is not true for strings.
You can carry out the exercise shown below to understand this subtle difference.
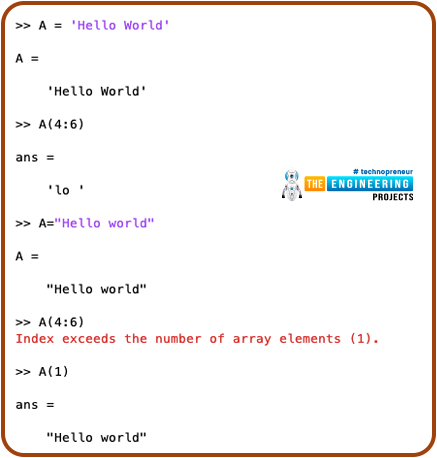
The various operations that can be performed on character array include:
Cell matrix:
- Just like matrices, a cell array is an indexed data container with each element known as a cell and can contain any type of data, including other cells and arrays which can be numeric or non-numeric types. Cells can be declared with curly braces, { } to let MATLAB know the input is a cell array. Individual cells inside a cell array can be called using round parentheses whereas contents at a location can be accessed by curly braces again, as shown in the example below:
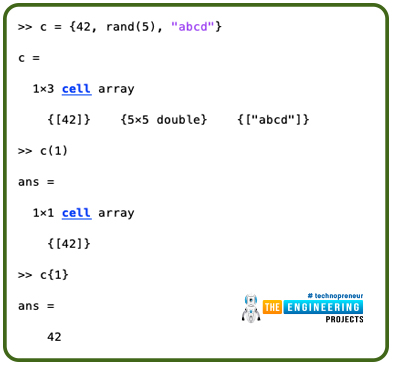
The function ‘cell2mat’ takes a cell as an argument and outputs a matrix. However, for this, all the elements of a cell array must be the same data type. This is what distinguishes Cell arrays from Matrices.

Tables:
- A table is a convenient data structure to write column-based or tabular datasets that can be stored in a text or spreadsheet file, or plotted easily as well. Tables let you access individual columns with variable names. Each variable can be a different datatype. The only restriction for different columns is for them to have the same number of rows. You can load the inbuilt tabular dataset inside MATLAB as shown below.
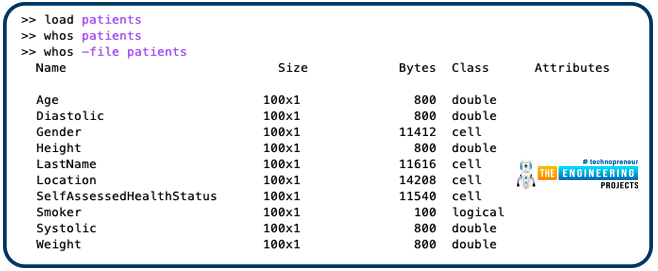
Structures:
- Structures are the most generic datatype in MATLAB which consist of indexed elements and each index can store a different datatype or in fact, a structure itself. Such structures are known as nested structures and the various branches of a nested structure can be accessed using the dot notation.
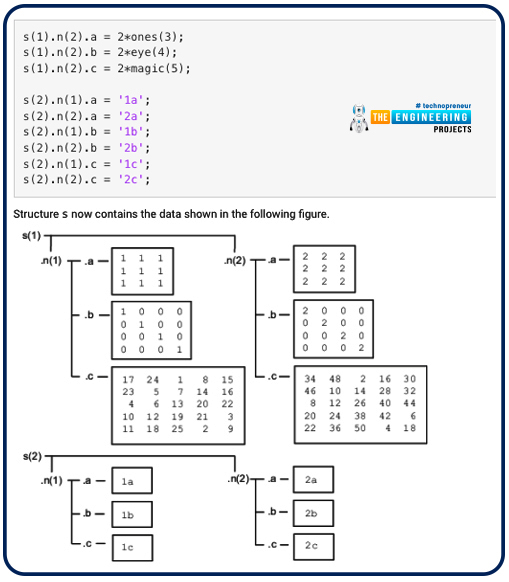
Non-numeric datatypes also include function handles, symbolic variables and anonymous functions but they are a topic worth a separate lecture for discussion and will come up in the upcoming lectures.
In further chapters, we will look at some of the applications of MATLAB in Linear algebra, look at different kinds of matrices inside MATLAB that are commonly used in a linear algebra class, and also work with input and output of data and functions using ‘m’ files as well as ‘mat’ files. We will also read about saving and loading operations, for input and output of data from MATLAB, and we will look further at making GUI in MATLAB, plotting linear, polar, 2D and 3D graphs with data sets.