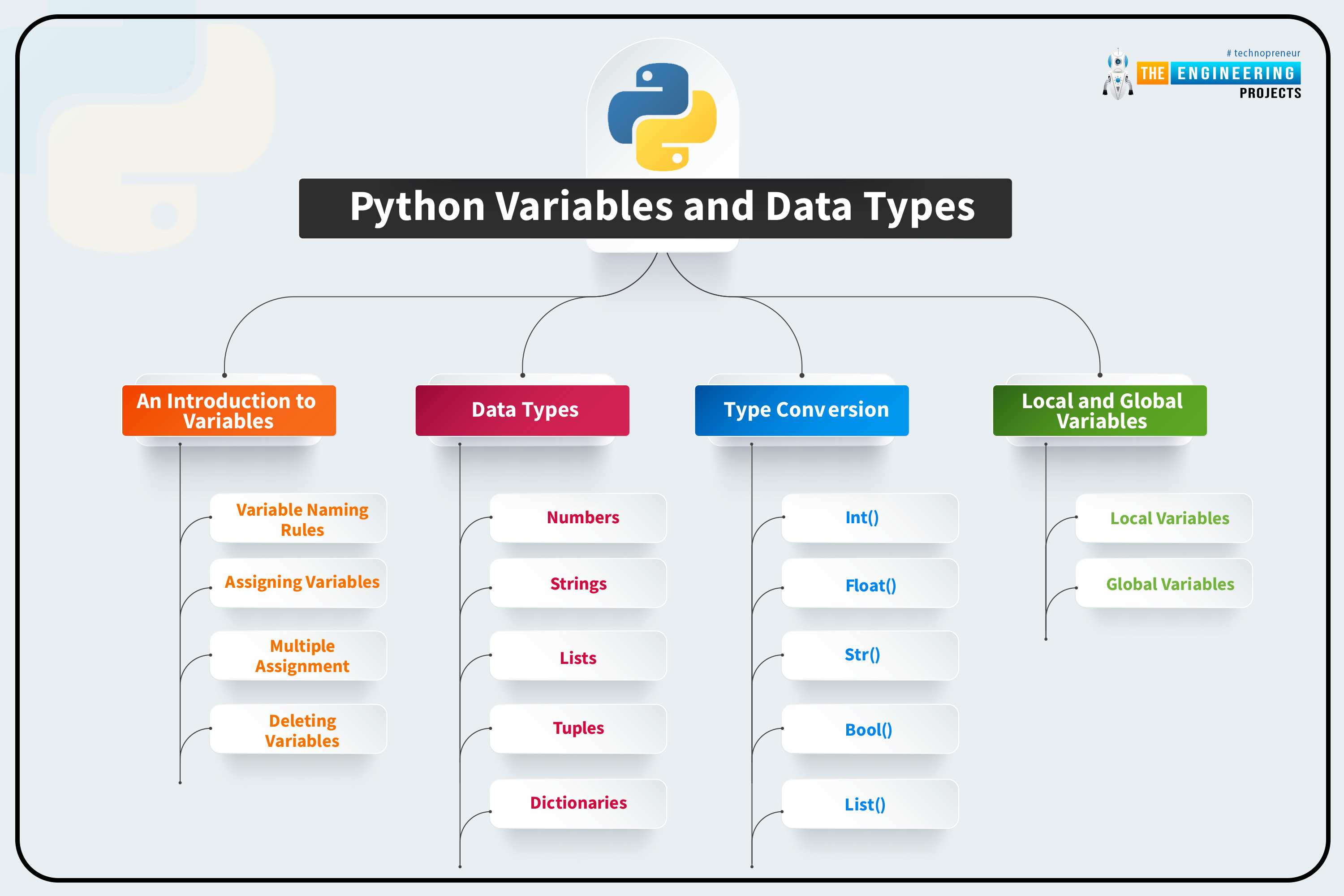
Welcome back! This is the fifth lesson in our Python programming course. In the last chapter, we discussed how string data types are used in Python. In this tutorial, we’re going to discuss variables in python and the rules for naming them in Python. In addition, you'll learn the fundamentals of working with numbers and strings.
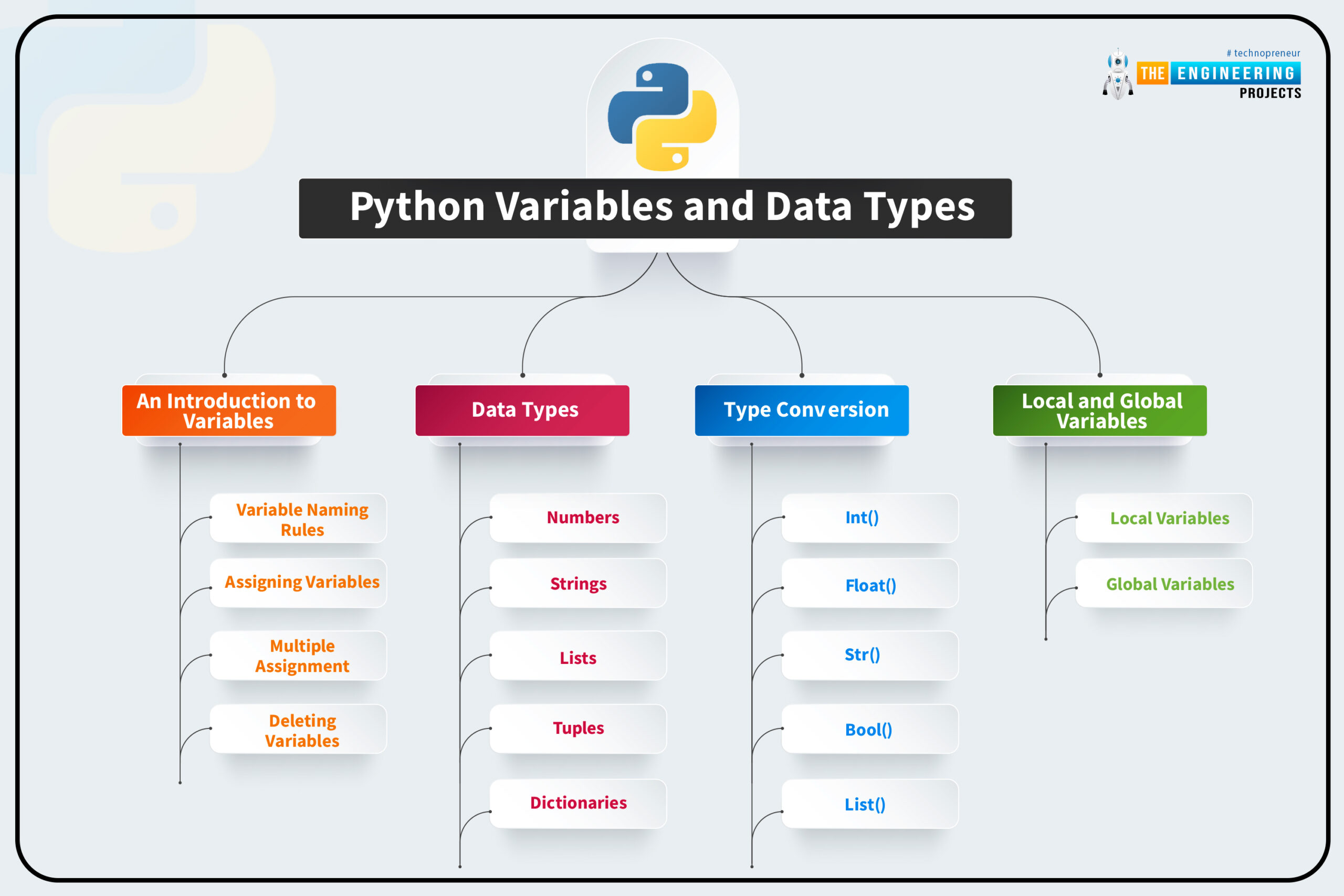
What are variables?
All programming languages use variables as a fundamental building block of their language. It is the allocation of memory that is dedicated to data storage and manipulation. Variables are program elements that keep track of data. The following is an example of a variable.
x = 100
It's called x in the diagram below, and it has a value of 100 in it. In this case, the variable name is x, and the data it contains is the number.
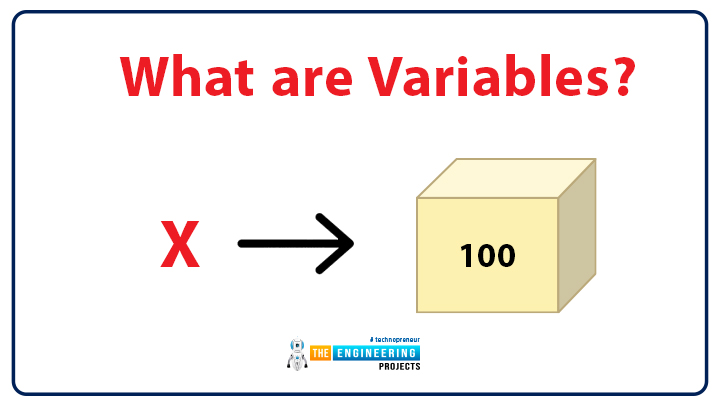
For a variable, its data type is just the type of data it contains.
Data types - what are they?
If a variable has a certain data type, it can be used with any mathematical, relational, or logical operation without resulting in an error; this is known as the data type in programming. There are many different types of data, such as strings and integers, that are used to categorize different kinds of information.
How do computers store Python variables?
As a Python programmer, we don't have to do anything to free up space. Garbage collection handles this.
There are two types of memory:
Stack memory
Stack memory is used to hold all functions and their calling references. The lifo rule governs how a stack operates. Continuous blocks make up the stack memory. When a function is called, the variables it returns are kept in the program's call stack, where they can be freed upon function completion and the program's exit successfully.
Heap memory
Heap memory refers to the memory allocated at run time when each instruction is performed. A heap is used to store global variables, which can be shared by all functions in the program, rather than the local variables defined within a function. Here, x = 23 is an example.
Number types in Python
Integers, floats, and complex numbers are all available as numeric types in Python. In this tutorial, we'll learn about integers because we'll be using them to show how variables work.
For simplicity's sake, an integer is a number that does not include a decimal point or fraction. They might be both positive and negative at the same time. For example, the numbers 1, 2, 3, 500, and 10000000.
The int class contains all integer variables. The class name can be found using the type () method, as illustrated in the example below.

Python's Rules for Variable Naming
- When naming a variable, it should begin with a letter or an underscore (_). For example, Age, _age, and Age.
- There are no special characters allowed in the variable name, except for the underscore (_). There are several examples of this: age_, _age
- Variables are case-sensitive. Therefore, age and Age are two different things.
- However, the variable name can have numbers at the end, but not at the start. Age1 is a good illustration of this.
- Python keywords should not be used in the name of a variable. Reserved words are another term for keywords. True, False, None, and Def.
Techniques for naming a variable with numerous words.
- Camel Case: As a visual aid, the second and following words are capitalized. For example, pythonTutorial
- Pascal Case: Similar to Camel Case, except that the initial letter is capitalized instead of being lowercase. For example, PythonTutorial
- Snake Case: Underscores divide each word. For example, python_tutorial
Variable Assignment
A variable refers to an item's specific name. Instead of having to declare or specify a variable like in other programming languages, Python simply uses the equals (=) sign to create a variable and assign a value.

As stated, "n is allocated the value of 300" in the example above. You can then use n in a statement or expression, and its actual value will be substituted for n.
Just like with a literal value, variables can be shown directly from the interpreter prompt in a REPL session, without the usage of print ().

In the future, if you use n again and alter its value, the new value will be used:
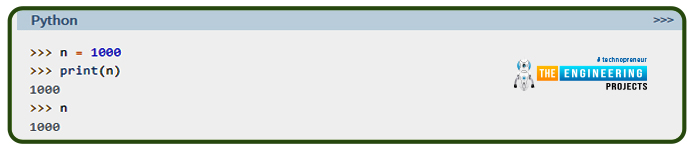
Chained assignment in Python allows the same value to be assigned to many variables at the same time:
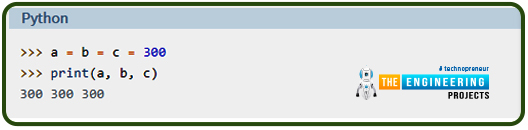
The above-chained assignment gives the variables a, b, and c the value 300 at once.
Python's Variable Types
Python has two types of variables: global variables and local variables. To utilize the variable in other parts of your program or module, you need to declare it as a global one. It is common practice in Python to use local variables when creating new variables.
Using the following program, you can see how Python variables function by comparing the local and global variables.
- Using Python, let's create a global variable called "f" and assign it the value 101, which is printed in the output.
- f is a new local scoped variable declared by function f. When this variable is set to its default value, "I'm studying Python." is printed as an output. This Python declared variable is distinct from the global variable "f" specified before.
- A function call's local variable f is disposed of once the execution has completed. At line 12, the global variable f=101 is displayed when printing the value of "f" once more.
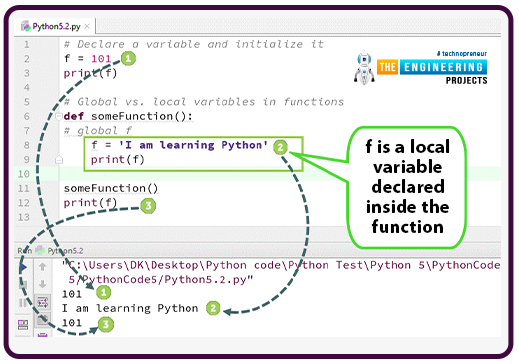
You can utilize a global variable in a function in Python if you include the global keyword in the variable definition.
- The "f" variable is global in scope and has been assigned a value of 101, which is printed in the output of the code.
- The variable f is declared to exist by using the keyword global. That which was previously defined as a globally applicable variable will be used here. When we print the value, it comes out to 101.
- Inside the function, we modified the value of "f." The new value of the variable "f" remains even after the function call has ended. Line 12 prints the value "changing global variable" when value of "f." is printed.
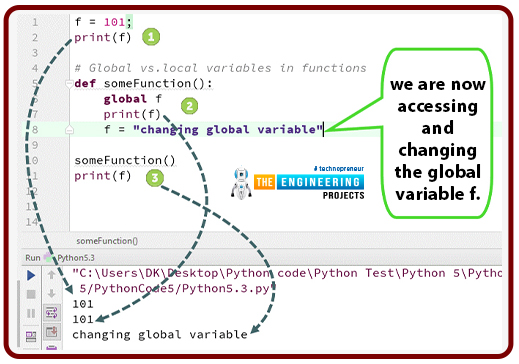
Variables are statically typed in many programming languages. A variable's data type is specified when it is created, and any value that is assigned to it must always be of the same type.
However, Python variables are exempt from this rule. It is possible in Python to give a variable a value of one type and then change it to another type later on:
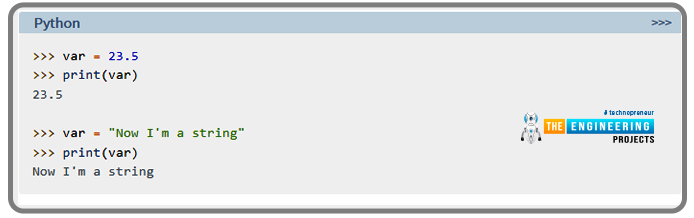
In reality, what happens when you assign a value to a variable?
This is an important subject in many programming languages, but the answer in Python is a little different.
Since Python is a highly object-oriented language, every piece of data in a program is an object of some type or class. This will be brought up again and again in the course of these tutorials.
References to objects
Take a look at the following coding:
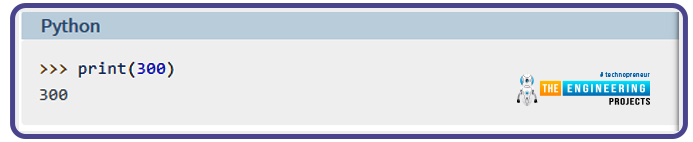
Translators are instructed to perform the following actions when provided with a statement print (300).
- Creating and supplying the value 300 to an integer object
- The console will show it.
As you can see, the built-in type () function creates an integer object.

An object can be referenced by a Python variable's symbolic name, which serves as a pointer or reference. After assigning a variable name to an object, you can use that name to refer to the object. However, the object itself still contains the data. For instance,

An integer object with the value of 300 is created and the variable n is assigned as an identifier.
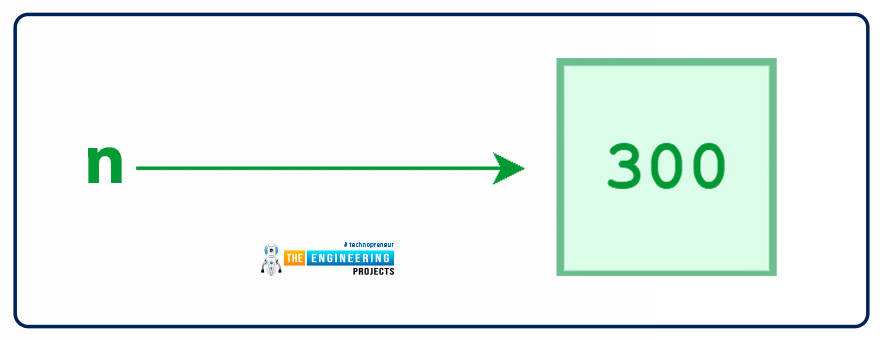
n is a pointer to an integer object, and the following code confirms that:
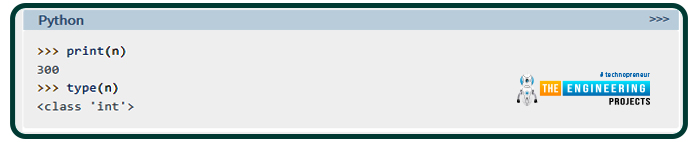
Then consider the following assertion:

What happens if it is executed? A new object is not created. Since n points to the same item, m points to a new symbolic name or reference.
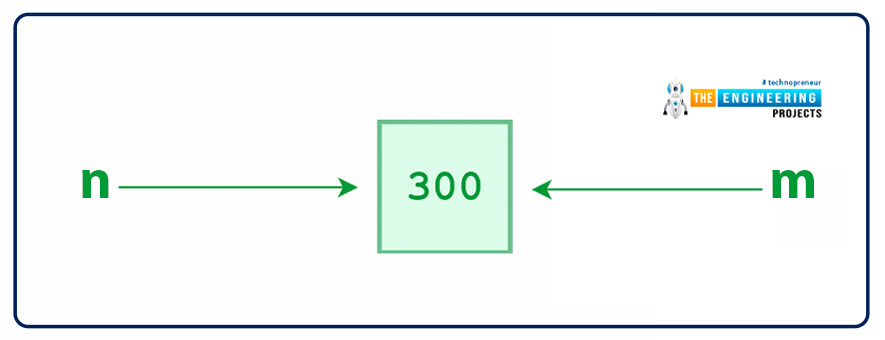
Next, you may try something like this:

Python now constructs a new integer object named m, which has a value of 400.
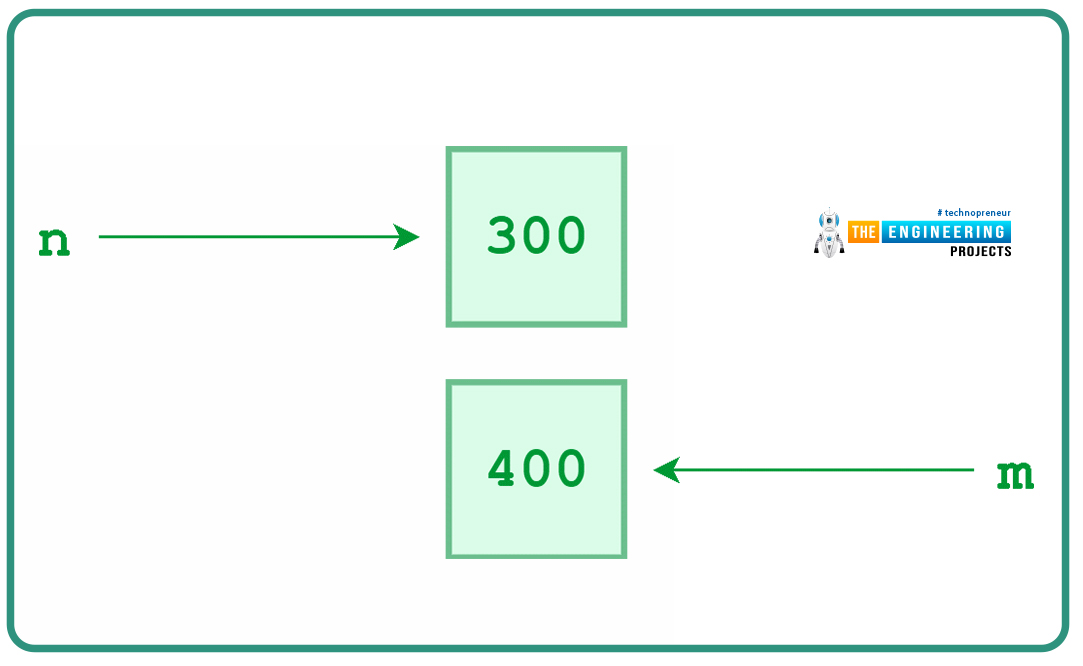
Finally, let's consider that the following statement is executed:

As a result, Python generates a string object named "foo" and uses that as a pointer in n.
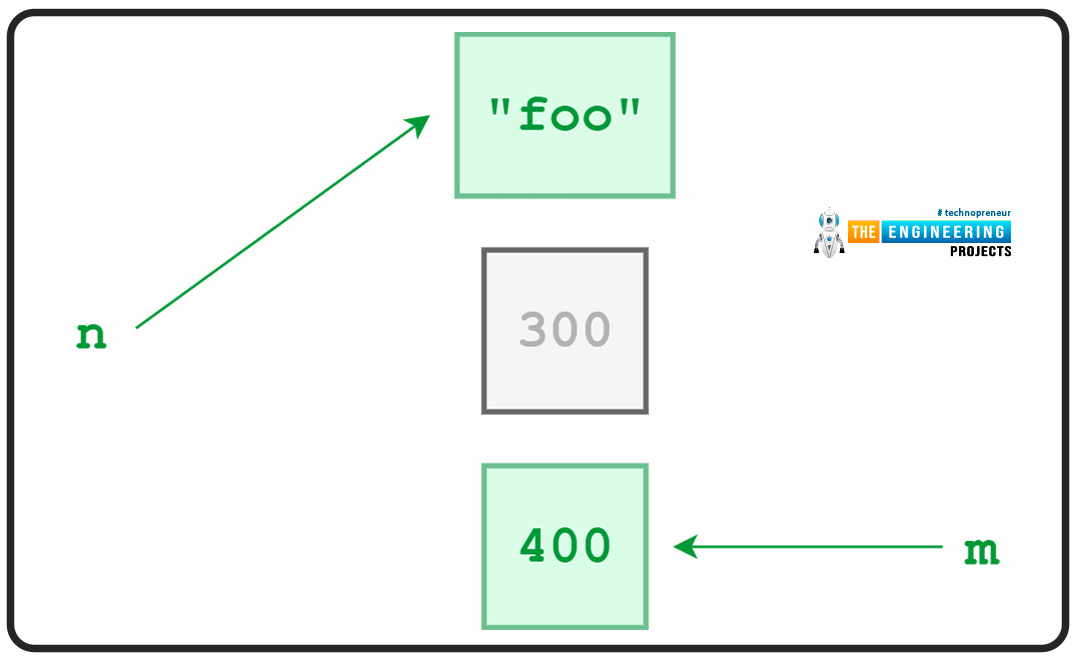
The integer object 300 is no longer mentioned anywhere. There is no way to get in touch with it.
The lifespan of an item will be mentioned at various points in this series of tutorials. When an item is first created, at least one reference to it is made, which marks the beginning of its life. A reference to an object can be added or destroyed at any moment during its lifetime, as you saw in the example above. As long as there is at least one reference to the thing, it lives on.
Objects are no longer accessible when the number of references to them reaches zero. Its time had come to an end at that moment. The allocated RAM allocated to Python will eventually be reclaimed so that it can be utilized for something else. Garbage collection, as it's called in computer jargon, is a very simple way to manage your own memory.
Object identity
Every Python object is assigned a unique identifier number when it is created. No two objects will ever share an identifier, even if their lives overlap for a period of time. It is not possible to utilize the same identifier for an object after its reference count reaches zero and it is garbage collected.
A Python object's id can be obtained using the id () function, which is part of the standard library. Id () can be used to verify that two variables are actually pointing at one another:
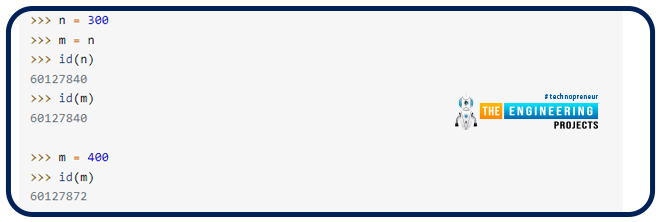
Even after an assignment such as this, which results in the same number being returned for id(n), it is clear that these two numbers point to the same thing. As soon as m is reassigned to the value 400, they no longer point to the same item, but rather to different things, take into account the following:
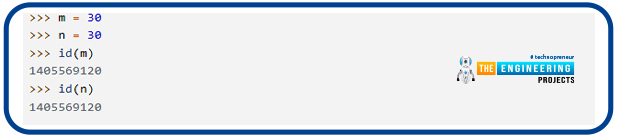
M and n are both 30-valued integers in this example. The id(m) and id(n) are identical in this example, While running the program, the interpreter makes use of previously created objects for numbers in the [-5, 256] range. As a result, if you assign different integer values in this range to different variables, they will all refer to the same thing.
Delete a variable
Python variables can also be deleted by use of function del "variable name". Python's error "variable name is not defined" indicates that you've erased the variable in the following example of the language's delete variable function.
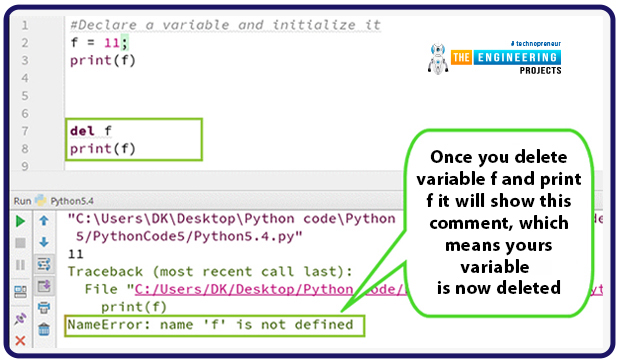
Variable type casting
Test the concatenation of several data types such as string and integer. The number "99" will be added to the word "Guru," for example, A Type Error will arise if the number is not expressed as a string when declaring variables in Python. This differs from Java, which does not require the number to be declared as a string.
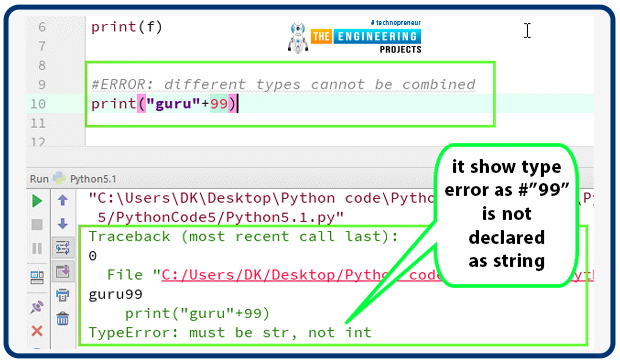
You will obtain an undefined result if you run the following code.
a="Guru"
b = 99
print a+b
Use print(a+str(b)) to concatenate both a and b into a string.
what is type casting?
This is the process of changing one data type to another. Data type conversion is necessary in some scenarios. For instance, you may want to combine two numbers where one of the variables' existing values is an integer and the other is a string. Python typecasting is required before addition, as it must transform a string's data type to an integer.
Types of conversions in type casting
- Explicit conversion
Type casting in Python can be done using a variety of functions.
- The INT () function is used to specify integer literals. A string literal is converted to an integer value.
- Use STR () to convert an integer to a string ().
- Implicit conversion
Data types in Python are implicitly converted by the Python interpreter, which means that the user is not required to intervene in the process. See the examples below for a better understanding of the subject.
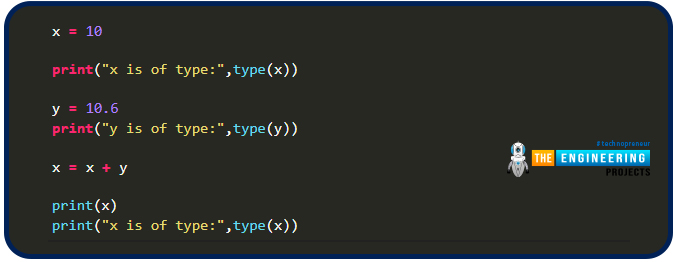
Conclusion
Congratulations! Your perseverance has paid off. The focus of this session was on Python variables, object references, and identity, as well as the naming conventions for Python identifiers and a brief discussion of integers. Let’s meet in our next tutorial as we discuss about Python data types and how they're put to use.