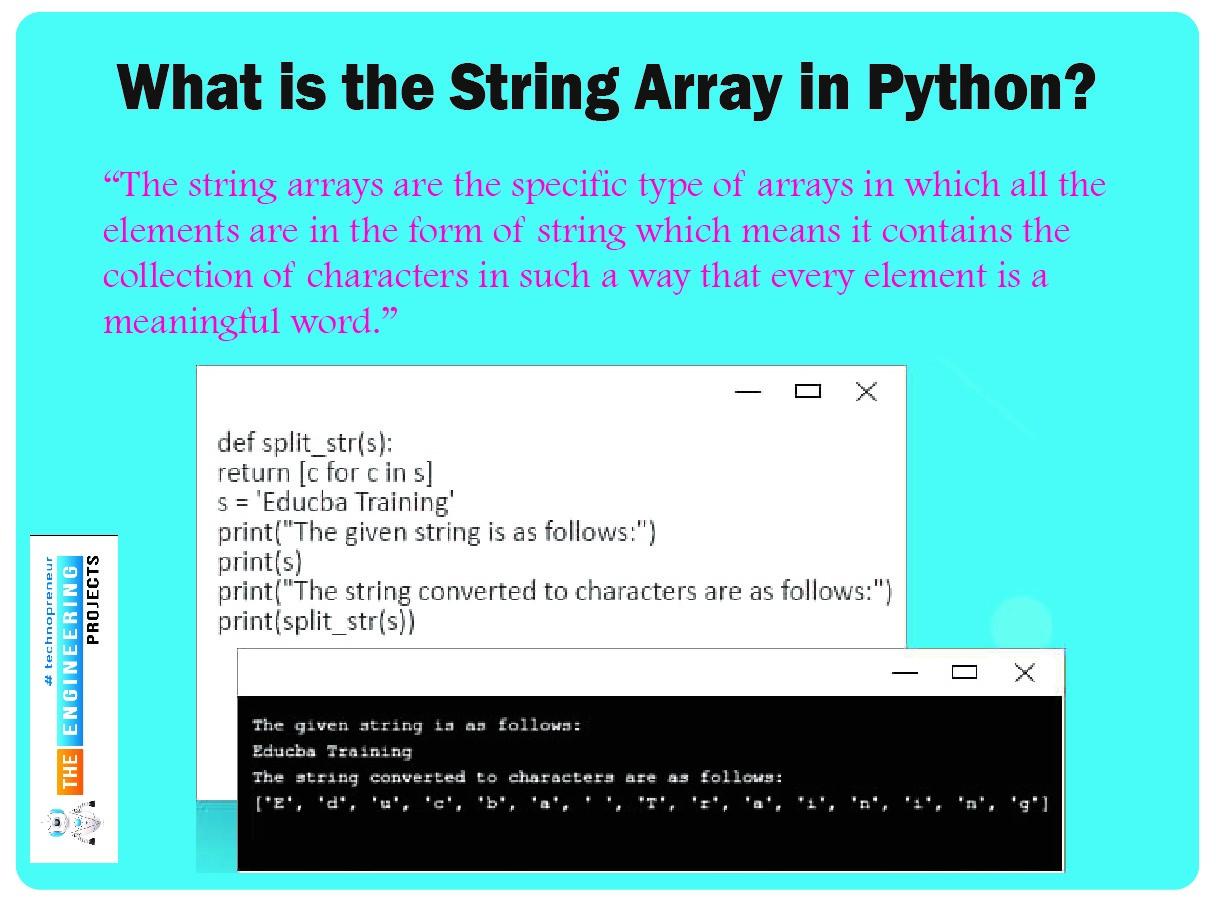
Hey peeps! Welcome to the Engineering Projects where you are learning the basics of Python in detail. We are working a lot with the arrays as these are important concepts in most of the high-level programming languages till now, the numeric data was used in the arrays but for our practice, we are moving towards the next step where the focus will be on the string data type. The practice is done on the Jupyter notebook and every step is going to be explained well without skipping any concepts but to keep things easy, we are not emphasising unnecessary details and concepts. So, before going deep into the topic, have a look at the list of the contents that will be discussed in this lecture:
What are the strings?
How do we use the string in arrays?
What is the list?
How do we extract the characters from a simple string through indexing?
How do you relate the string array with the list?
How can we get more than one character from a string message without using the array?
How do we add more than one message together by using the concatenation?
What is the best way to access the elements of the string array through indexing?
Can we add more elements of the string array into the already declared array?
How do we remove the elements from the string array?
What is the relation between the string and character in Python and how do you extract the single alphabets from the string using the list method?
All these concepts will be discussed in detail and will be understood by using the coding in Jupyter Notebook.
What is the String Array in Python?
Here is a quick revision of the concept we have learned so far in this series but it is important to understand the basic definition so that we may understand the difference between all of some basic data types. The string is the sequenced order of the characters. It means that all the meaningful conversations between people and even this lecture are in the form of string. So, in this way, the array, containing all the elements in the form of the string is called the string array. We define it as:
"The string arrays are the specific type of arrays in which all the elements are in the form of string which means it contains the collection of characters in such a way that every element is a meaningful word."
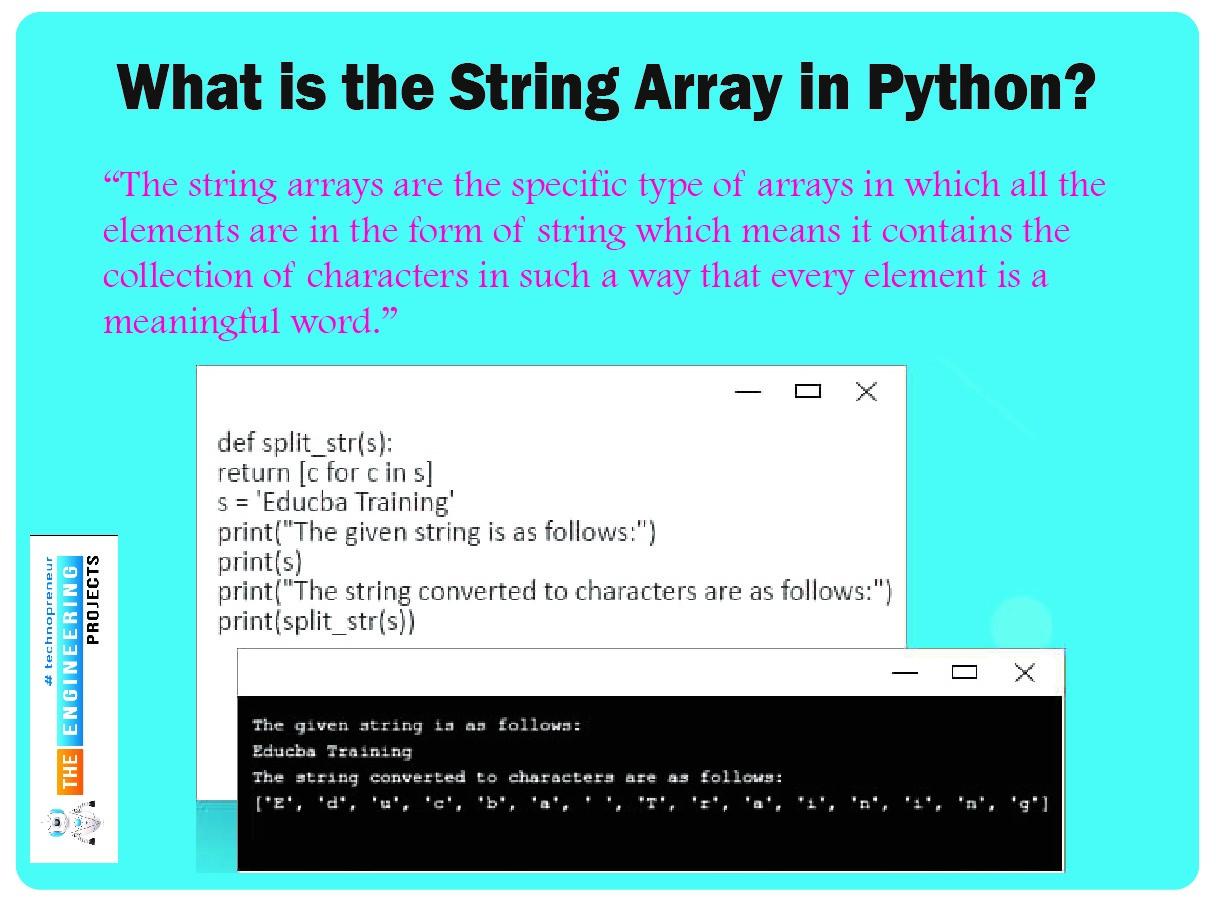
We use the string in our daily life during the conversation and there is no need to explain more because we have used the string many times in our series of these lectures.
Getting the Character of String Through Indexing
If we start from the basics then we have to mention that as the string is a collection of different characters, then extracting the characters from the strings it is quite simple and easy. Why we are associating this method with the arrays? It is because the compiler can read all the characters by indexing them one after the other and that is the reason why we can fetch the characters in Python by using the indexing method. So, have a look at the code for doing this simple task:
Code:
#Declaring and printing the string message
str="We are learning Python"
#printing the single character from the message
print("The string message = ", str)
print("The second character is " ,str[1])
print("The tenth character is ", str[11])
Output:
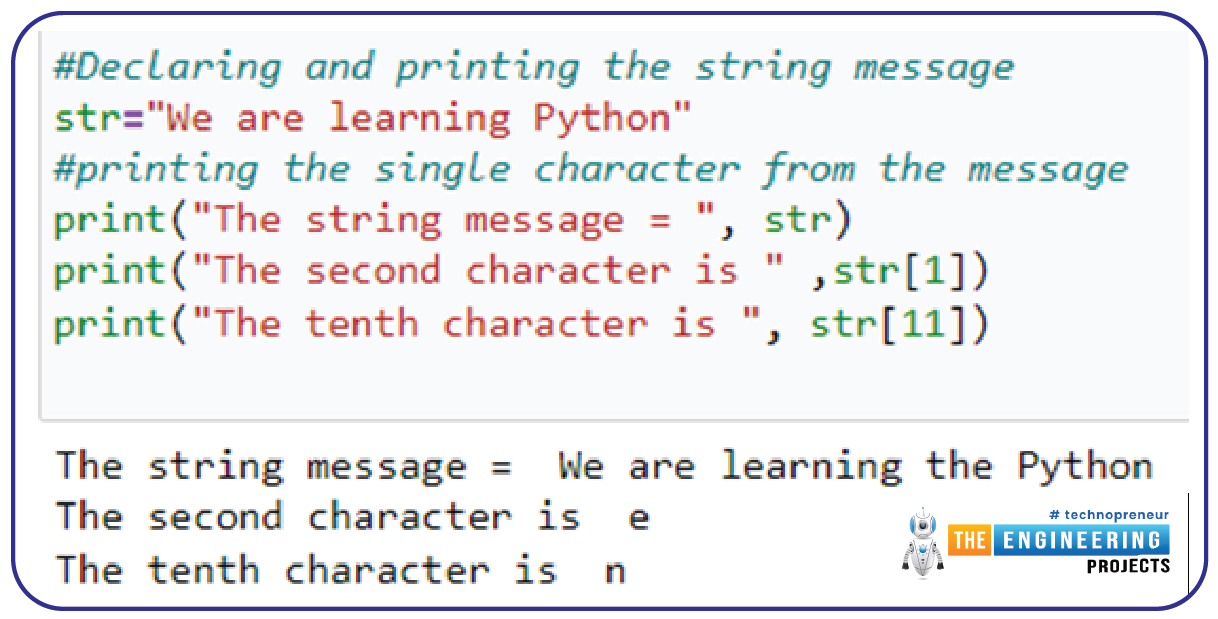
Getting More than one Character from String
Code:
#Declaring and printing the string message
str="We are learning Python"
#printing the single character from the message
print("The string message = ", str)
#More than one character
print("The characters from second to ninth index are: " ,str[1:8])
#using only semicolons
print("The first ten index characters are : " ,str[:10])
#out of the range characters
print("The out of the range characters are : ", str[:45])
Output:
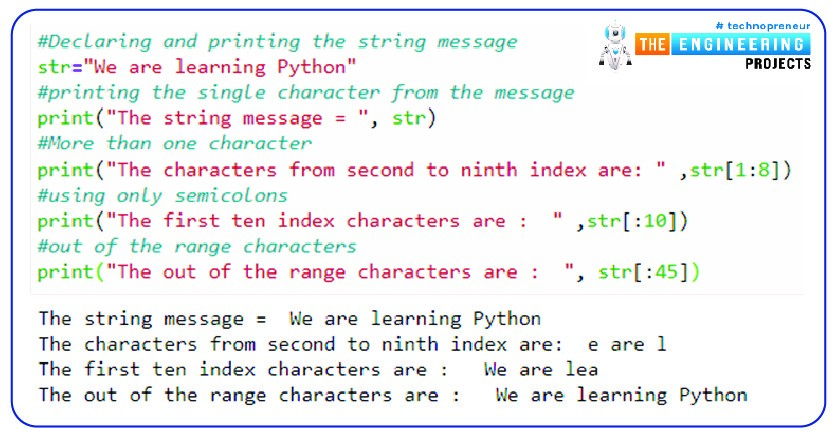
Here, the following points are to be understood:
More than one character can be extracted from the string using the index.
The starting and the ending number of the range you want to print are to be given in the index with a separation using the colon.
If the programmer wants to print the elements from the start to a certain number, there is no need to mention the zero, and we can start directly from the colon.
By the same token, if the colon comes second and then the number is before it, the compiler will print the character at the index starting from the number till the end of the series of the characters.
In case, the programmer gives the numbers that are out of range then the compiler, instead of throwing the error, prints the whole string with its intelligent working.
Adding String Arrays in Jupyter Notebook
We all have been working with the addition of numbers in our tutorials, but today, the good thing about the string array in Python is, the addition of the arrays, even if they have strings in them, is as easy as the other types of “addition processes." The process of concatenation is used for this. We define it as:
"The concatenation is a specific process used for the string to join two or more strings together in a specific manner."
The At this level, there is no need to explain more. Have a look at the following code:
#decalring the arrays with string words
A=["hi", "we", "are", "learning", "Python"]
B=["It", "is", "easy", "with", "theengineering.com"]
#Using the concatenation for the addition
print(A+B)
Output:
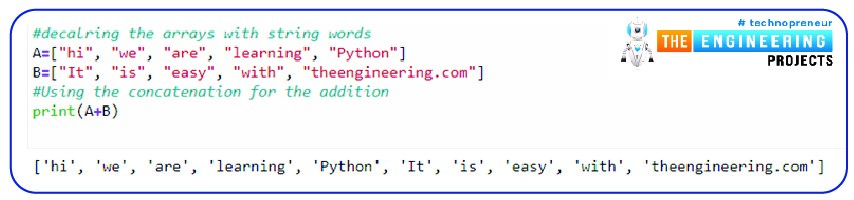
Arrays as List of String in Python
In Python, the concept of a string array can be associated with the list because it becomes easy for the programmers to deal with the string as the list has the following feature:
These contain the specific order of the elements, and for the string, if we want to have a specific message, the order is important and it makes our task easy.
It allows frequent changes in the elements so that we may change the message according to our requirements.
Duplication of the element is possible in the list, and in the passing of the message, we observe the same words in single sentences.
Similar concepts are being used here that we have seen when we were learning the numerical problems, but this time, it will be interesting to know the difference between the results with the help of shortcodes.
Accessing the Strings in Different Ways
Once you have declared your string, the extraction of single or multiple strings from the same array is fun. The programmer just has to specify the location, and the element is popped on the output. But there are different ways to do so, and some of them are shown in the code next.
Code:
#initializing the array containing the name of programming languages
languages=["C++", "C#", "MATLAB", "ASP.NET", "FLutter"]
#printing the arrays using the index in two ways.
print("\bAccessing the data through index: ",languages[3] )
print("\bAccessing the data through negative index: ",languages[-3] )
#getting the whole array of strings through for loop
print("\bPrinting the whole series using loop")
for lan in languages:
print(lan)
Output:
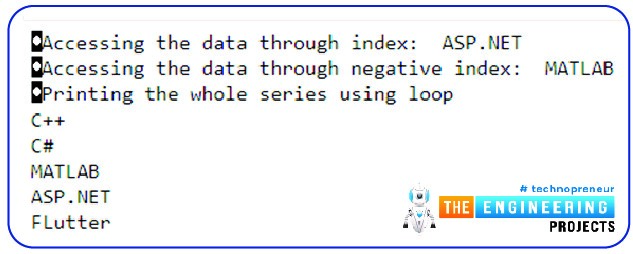
Updating the String Array
Once the array is made, the good thing is that it can be updated according to the will of the programmer. Let’s say we had an array containing the names of the students in the class; if a new student gets admission or the previous one changes the class, it can be updated easily. In other programming languages, the arrays do not allow the updating of the elements.
Code:
#initializing the array containing the name of programming languages
languages=["C++", "C#", "MATLAB", "ASP.NET", "Flutter"]
#appending the new element at the end
languages.append("java")
#getting the whole array of strings through for loop
print("\bPrinting the whole series using loop after appending")
for lan in languages:
print(lan)
#deleting the element from the array of string
languages.pop(2)
#getting the whole array of strings through for loop
print("\bPrinting the whole series using loop after deleting")
for lan in languages:
print(lan)
Output:
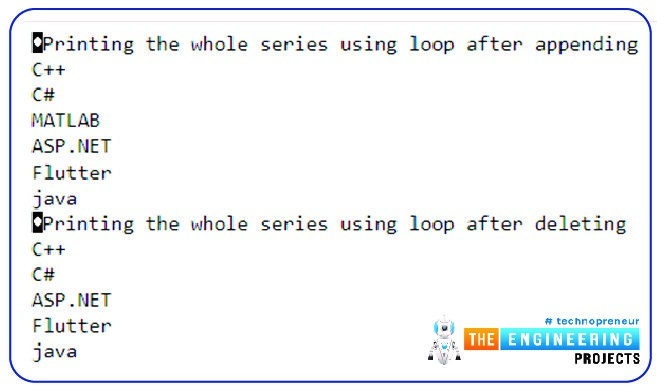
Hence, the update process is easy to use in arrays. The point that must be cleared here is, the pop function is sued to remove the element from the array and the programmer can not use a string in this place otherwise the compiler will show the error. Only the number of the element can be used in this function, and you must have noticed that it is not the position of the element that we have mentioned here but the index of that particular element.
Converting String into the List of Characters
In other programming languages, characters are separate data types that contain single alphabets and have their own identity and existence. In this way, there must be a specific way to initialize the characters and the strings. Usually, in languages such as C++ and C#, the ways to declare the string and characters are different. Strings are denoted by double quotation marks, and the characters are defined by using single quotation marks around the alphabet. The point that I want to make here is, in Python, there is no concept of single characters, but the declaration of the words or characters, when single or double quotation marks are used, means the string, no matter if it is a single alphabet or the whole message.
In case the programmer needs to convert the string into a single alphabet (the data type is still a string), he uses a simple and uncomplicated function to do so. The code and the output of this specific program with an example are given next:
Code to Convert String into Alphabets:
#initialization and printing of the string message.
myMsg = " Python is easy. "
print("The message before conversion= ",myMsg )
#conversion of the string into alphabets.
myMsgList = list(myMsg)
print("The message after conversion= ",myMsgList )
#removing the white spaces from the result.
myMsgList2 = list(myMsg.strip())
print("The message without white spaces =", myMsgList2)
#printing the message without any single space
myMsgList3 = list(myMsg.replace(" ", ""))
print("The message without any single space =", myMsgList3)
Output:
Here, the following points must be known to understand all the operations performed.
The string message is initialized simply, and after that, the programmers can convert it into a list for useful functions.
To convert the whole string message into a single alphabet, the “list” function is used, and the characteristics of the string are converted into the list. Now, you do not have the string on the screen but the list.
In this case, the compiler reads even the blank spaces between the alphabet and shows them in the form of a single character with single quotation marks.
To remove the starting and end spaces, there is a method called "stripping" that eliminates the extra spaces from the message, and as a result, we get only the alphabets with the necessary spaces between them.
Another method called “replace” is used in Python which replaces all the spaces between the message and easily converts the whole message into a series of alphabets with no spaces between them.
There are other such methods that deal with the string in different ways. For example, the conversion of the string into single words is also possible, but for now, we are not discussing it. This is all for today, and we hope you learned a lot from this lecture. The beginning of this lecture included a very basic introduction to the string, and we saw how we can use strings in arrays. Moreover, using our concepts, we have learned many built-in functions, and with the usage of the string, it was easy to understand the concepts. We have seen the concatenation and other ways through which we can modify the strings according to our requirements. We will talk more about the string in our next lecture; until then, happy coding.