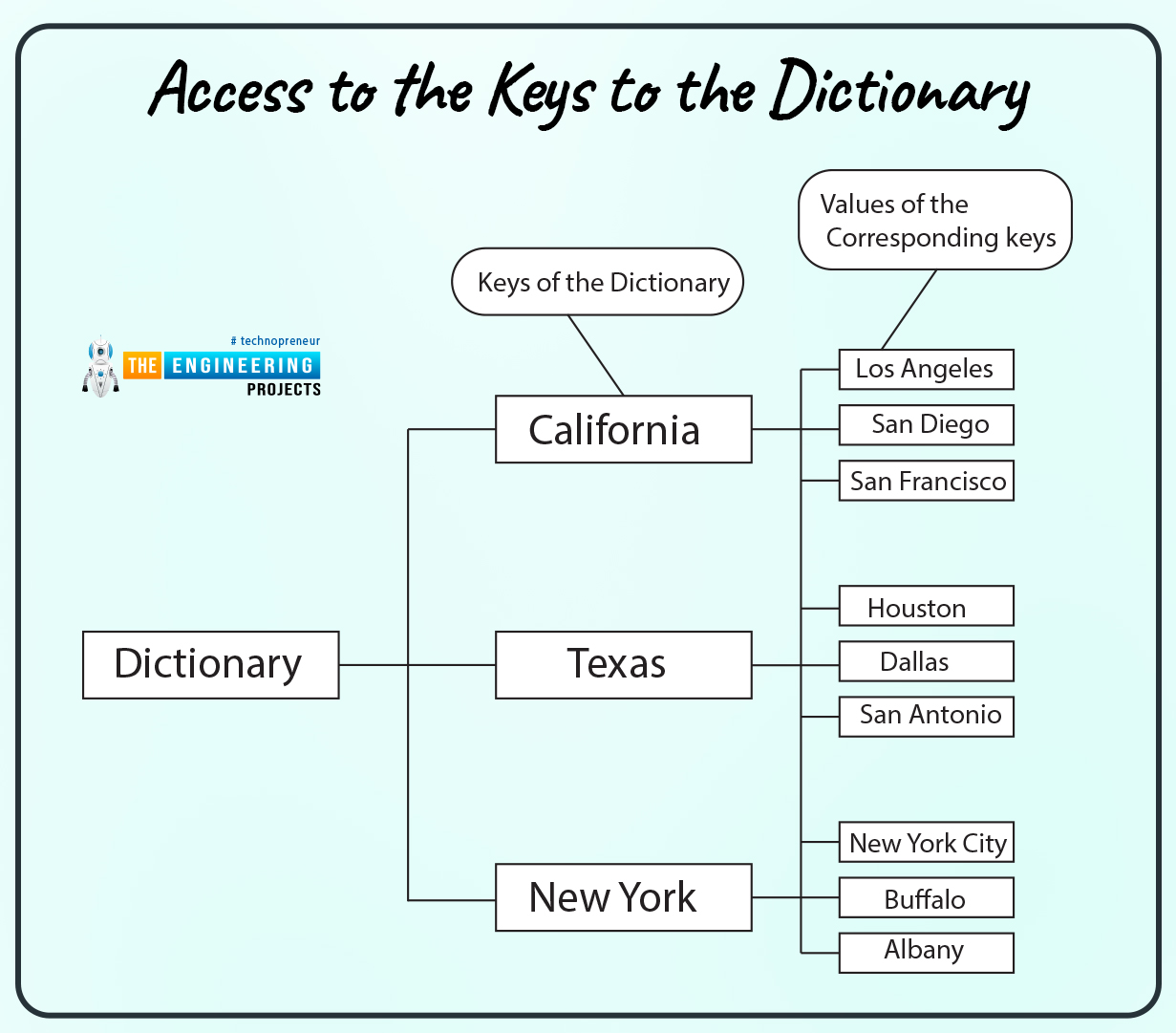
Hello! Welcome to the next lesson in this Python course. In the last session, we learned about python tracebacks and how to deal with them. A dictionary, like a list, is a collection of items, and we'll look at that in this tutorial as well.
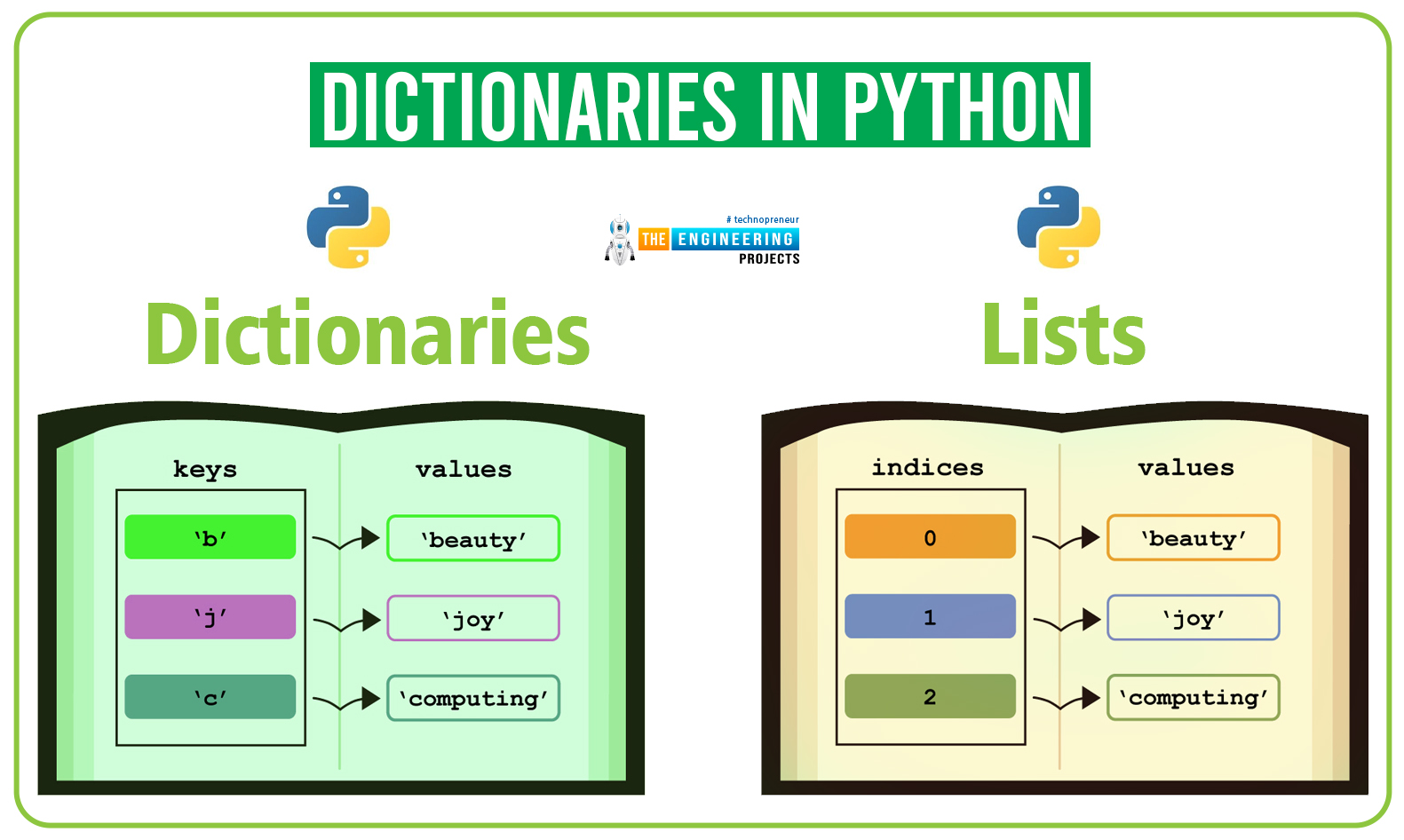
What will you learn?
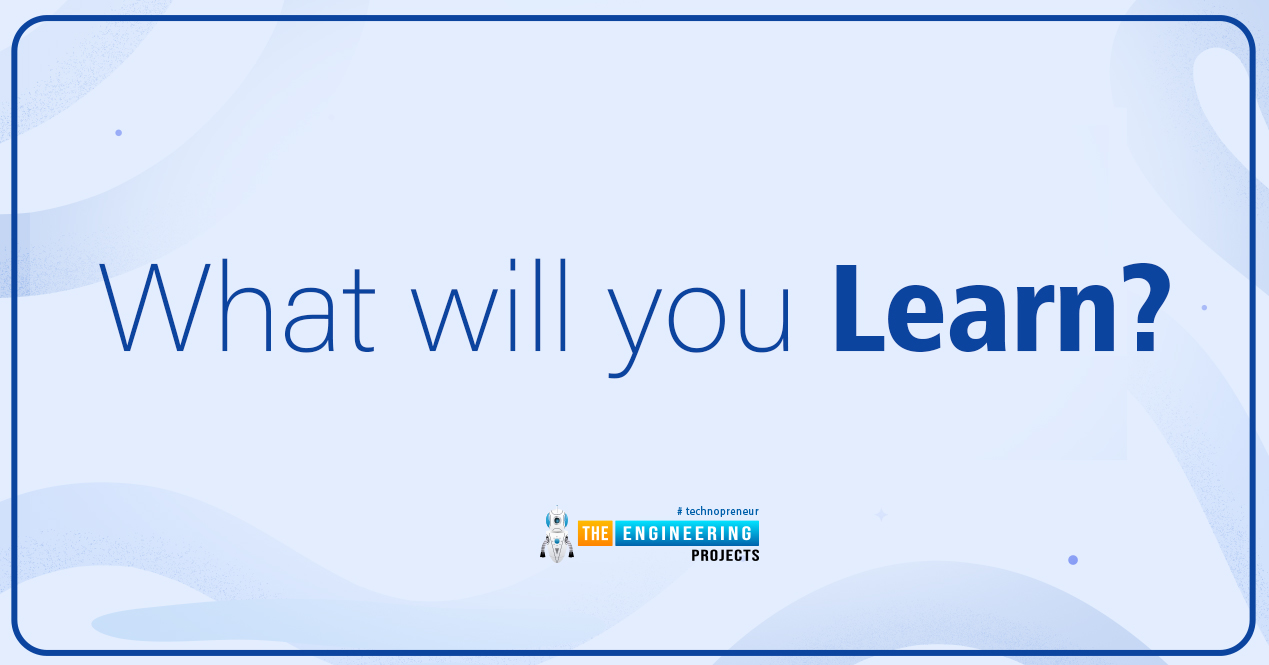
This tutorial introduces you to the fundamentals of dictionaries in Python and teaches you how to work with dictionary data. After reading this article, you should be able to tell when and how to utilize a dictionary as a data type.
Characteristics of Dictionaries and lists
- Both can be changed (mutability).
- A dynamic relationship exists between them. When needed, they're able to expand and contract.
- Both are nestable. Another list can be contained within one. It is possible to have a dictionary inside of a dictionary. A list can be found in a dictionary, and the other way around.
How do dictionaries differ from lists?
- Using indexing, list elements can be retrieved by their position in the list.
- Keys are used to access dictionary elements.
Defining a Dictionary
Data structures that use associative arrays, or dictionaries in Python, are known as dictionaries. Dictionaries store key-value pairs. Every pair of key-values has a corresponding value assigned to it.
With curly bracketed lists of value pairs, you can define a dictionary (). Each key and its corresponding value are separated by a colon (:):
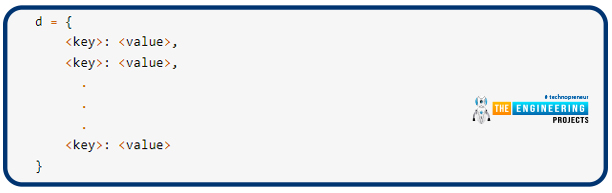
The following is a definition of a dictionary that links a place's name to the MLB team that occupies that location:
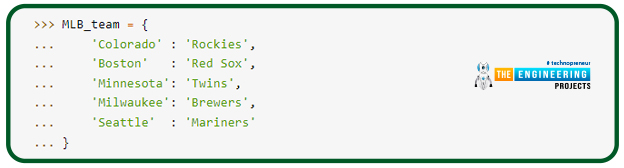
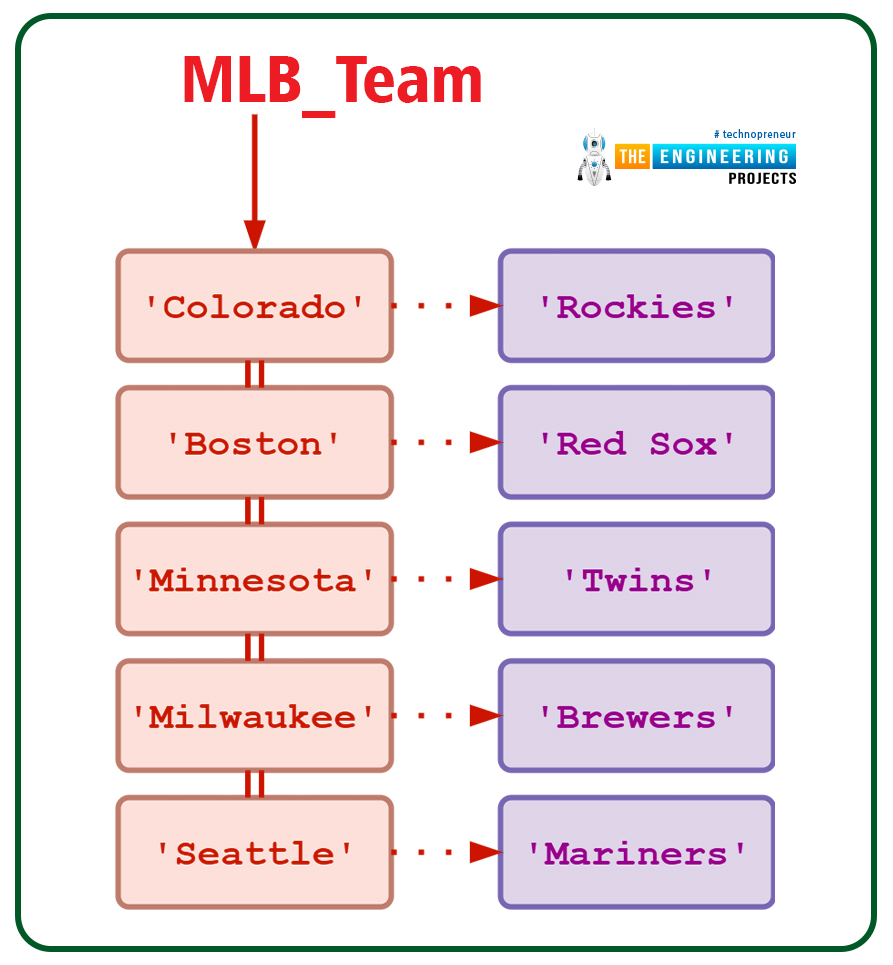
The built-in dict() function can also be used to create a dictionary. Key-value pairs should be passed to dict() as an argument. In this case, a list of tuples works well:
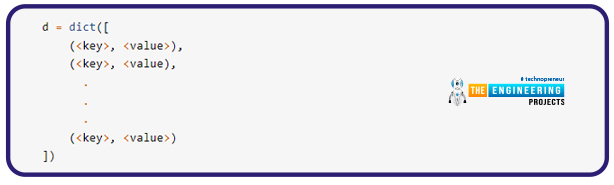
The following is an alternate definition for MLB team:
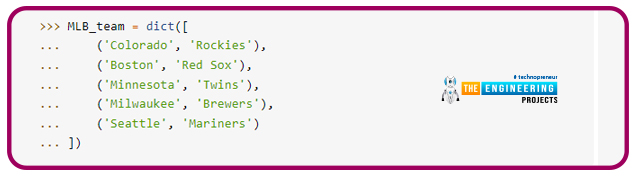
Key values can be supplied as keyword arguments if they are simply strings. MLB team can also be defined in another way.
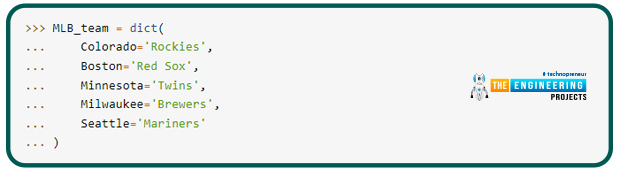
You can display the contents of a dictionary in the same way you do a list once it has been defined. When presented, the following is what each of the three definitions provided above looks like:
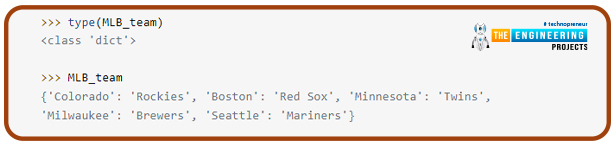
An alphabetical list of words and phrases is shown in the dictionary. When it comes to getting them back, however, none of it matters. There is no numerical index for dictionary elements:
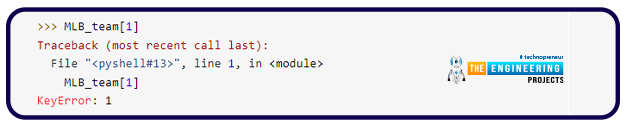
How do we query the Dictionary's Data?
Dictionary elements, of course, need to be accessible in some way. What if you can't find them using an index?
To get a value out of a dictionary, use the square bracketed key ([]):
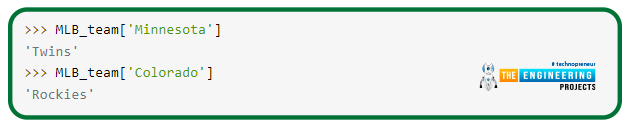
Python throws an error if you try to use a key that doesn't exist in the dictionary:
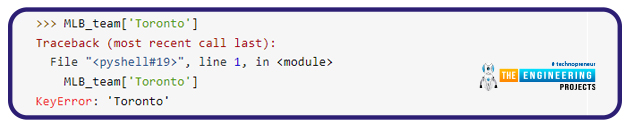
It's as simple as setting a new key and value to the existing entries in a dictionary:

In order to make a change to an existing record, you can assign different value to the current key.

A record can be deleted by issuing the del command with the key you want to delete specified:

Comparison of List Indices to Dictionary Keys
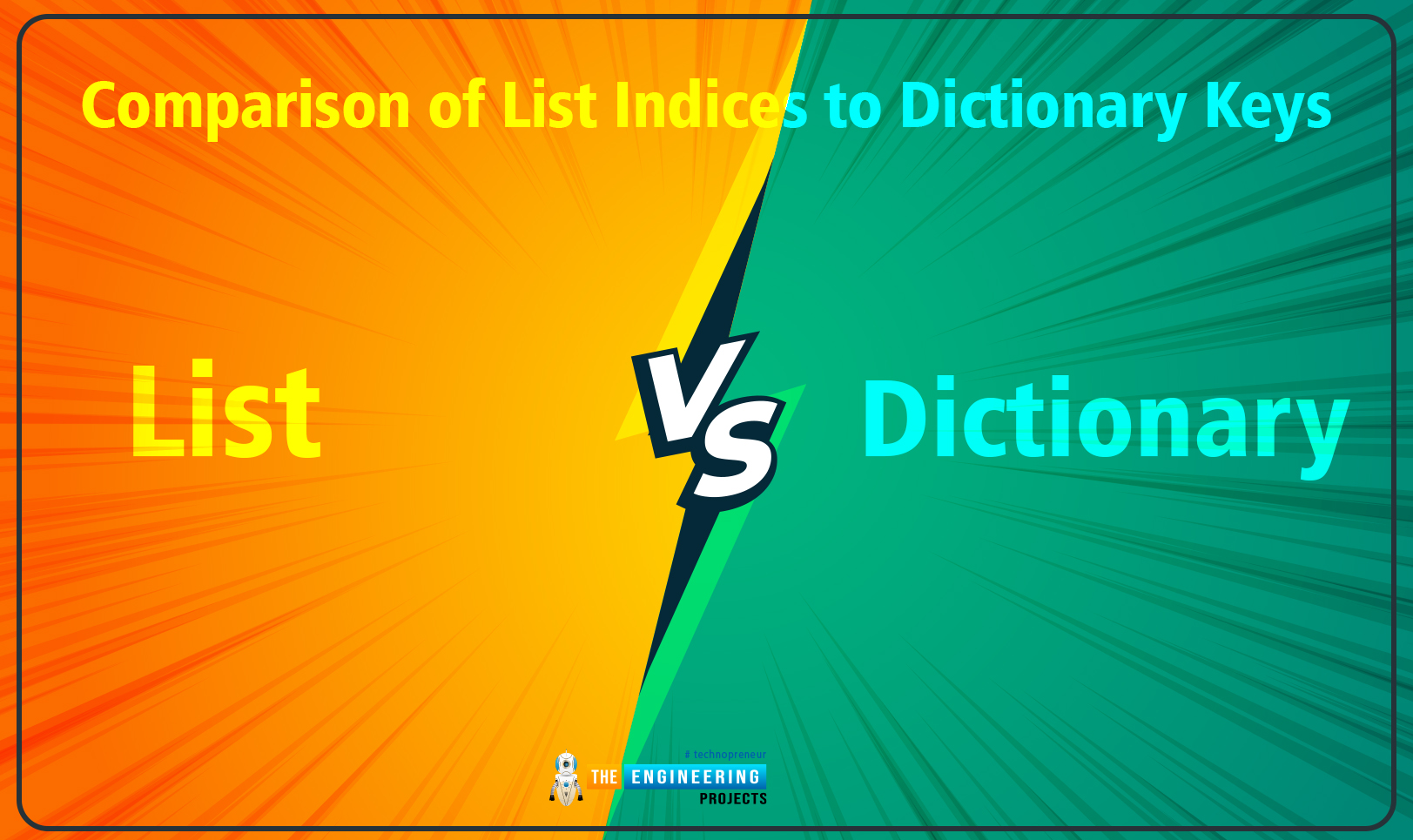
For some reason, the interpreter throws a Key Error whenever an undefined key or numeric index are used to retrieve a dictionary's keys.
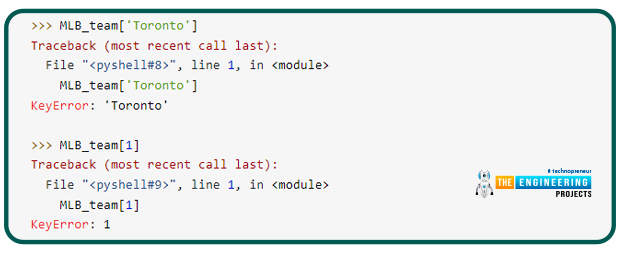
A mistake has been made in both cases. However, [1] does not represent an index in the second instance.
To utilize an immutable object as a dictionary key, you'll discover later on in this lesson that it's possible. It follows that integers are perfectly acceptable:
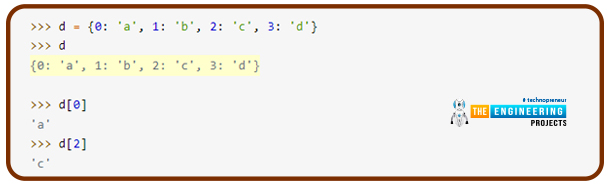
The integers enclosed in square brackets [] appear to be indexes. It's only that they don't have anything to do with the dictionary's order. They're being treated as dictionary keys by Python. The same values can be obtained by defining the dictionary in the reverse order:
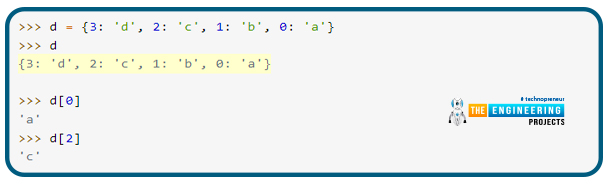
Despite the similarity in syntax, a dictionary cannot be treated as a list:
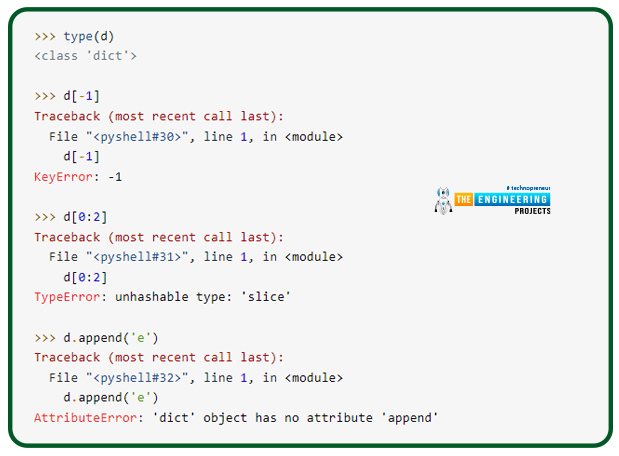
As a reminder, Python guarantees that the order of entries in a dictionary will be retained even if access to them is not required. Order matters when it comes to displaying items and iterating over the keys; this is how they'll appear when you see them. Items that are added to a dictionary are inserted in its final paragraph. Even after deletion, the remaining items retain their original order.
Adding to a Dictionary Step by Step
When all the other keys and values are known ahead of time, a dictionary can be defined as shown above by use of curly brackets and a value. It's a difficulty if you want to build a vocabulary on the fly.
Empty curly braces specify an empty dictionary, so that's where you want to start. Adding additional keys and values one at a time is possible.
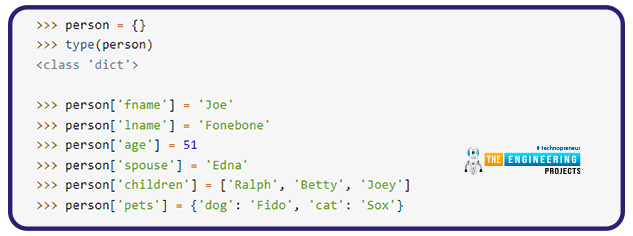
It's the same as using any other dictionary once the dictionary has been constructed in this manner:
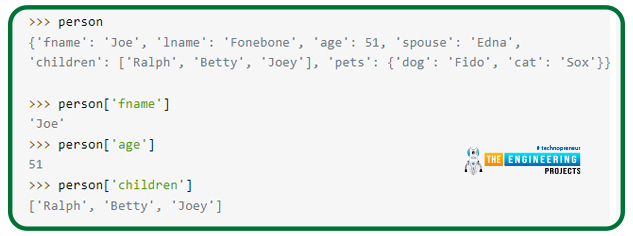
A secondary index or key is required to retrieve the values from the sublist or subdictionary:

Another advantage of dictionaries is that they don't require all of their entries to be of the same type. There are some strings, some integers, a list and a dictionary among the values in person.
The keys and values don't have to be the same.
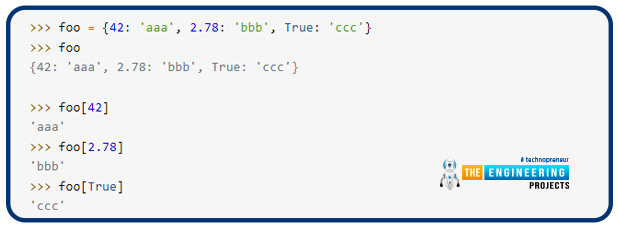
Integers, floats, and Booleans make up three of the four keys. Although it isn't immediately clear how this could be of benefit, you never know.
Python dictionaries can be used in a variety of ways. As a part of the MLB_team, the baseball team's name can be found in a number of places. There is only one entity that can be used to identify an individual: the person.
There are few restrictions on the types of keys and values that can be stored in dictionaries, making them useful for a wide range of tasks. But there are a few exceptions to this rule. Continue reading for more information!
Access to the Keys to the Dictionary
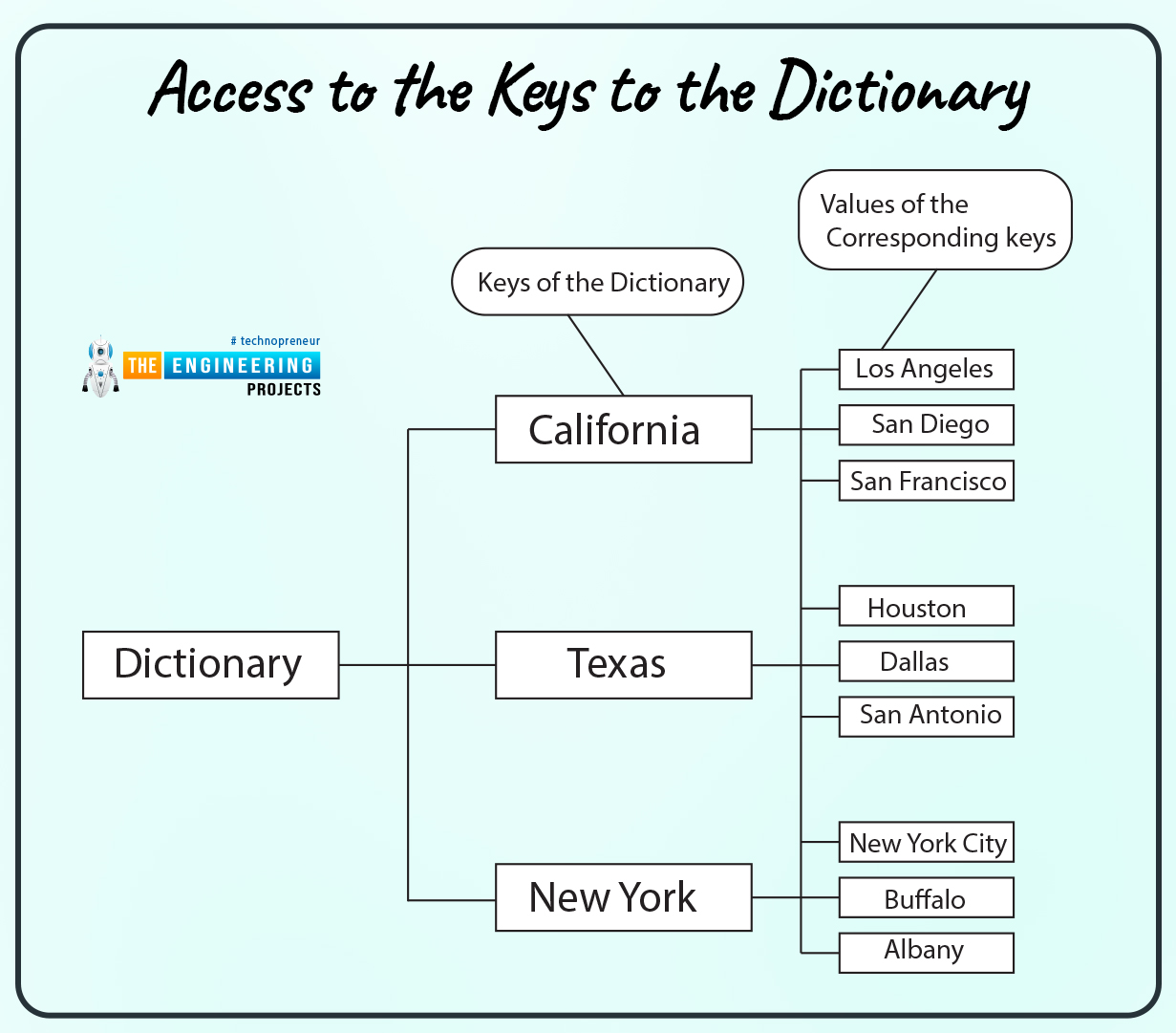
It is possible in Python to utilize almost any value as key in dictionary. You saw how to use integer, float, and Boolean values as keys like:

It's also possible to use pre-existing objects like types and functions in your code:
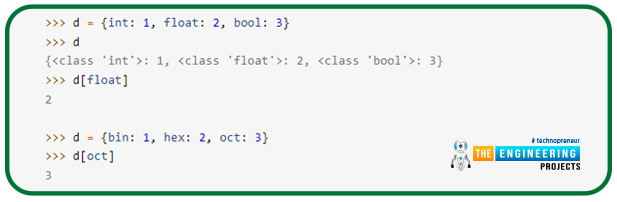
Dictionary keys, on the other hand, are subject to a few restrictions.
It is important to note that a single key can only be used in a dictionary once. It is not permitted to use two different keys. So, it doesn't make any sense to have more than one entry for the same key in the dictionary.'
You can't create a new key by introducing a new value to the current dictionary key; rather, you can simply replace the existing value.
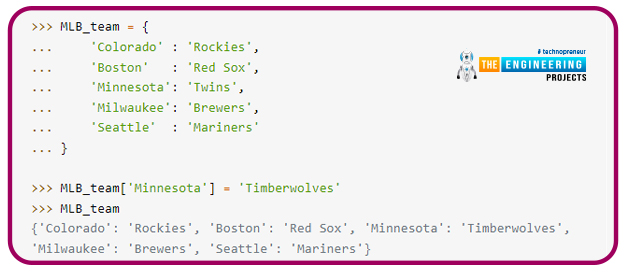
Second-occurrence key names take precedence over first-occurrence ones in the dictionary-building process.
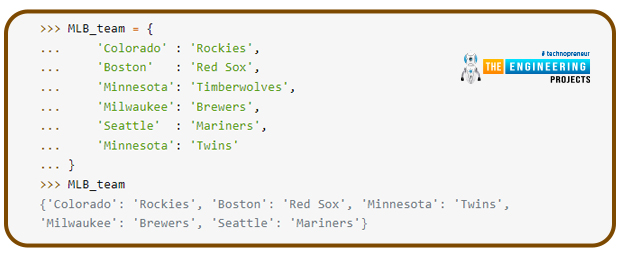
It's also important that a key in dictionary is of a type that cannot be changed. In the examples you've already seen, several of the types that are immutable, such as integers, floating points, strings, and Bools, have been used as dictionary keys.
Tuples, by virtue of their immutability, can also be used as dictionary keys:

When an immutable type is needed, a tuple is preferable to an array. One of them is this.)
Lists and dictionaries can't be used as a dictionary key because of the mutability of both:

What does "unhashable" mean in this context?
For a dictionary key to work, an object does not necessarily need to be immutable. It must be possible to send an item via a hash function, which means it must be hashable. For database lookup and comparison, data of any size can be hashed using a hash function to produce a fixed-size result known as a hash (or simply hash).
When an object is hashable, the built-in hash() method delivers its hash value; otherwise, it raises an exception.
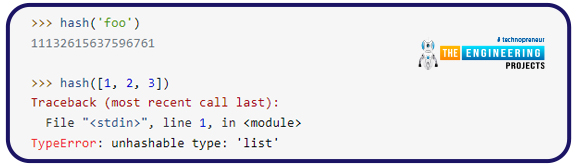
As you've learned so far, immutable types and containers (lists/dictionaries) that can be hashed are all built-in. For the time being, you can consider the terms "hashable" and "immutable" to be nearly interchangeable.
You'll see changeable objects that are also hashable in upcoming lectures.
Limitations on the use of Dictionary Definitions
Dictionary values, on the other hand, are unrestricted. In fact, there is nothing at all. For example, lists and dictionaries can be mutated and user-defined objects can be created in Python.
In a dictionary, there is no limit to the number of times a single value can appear.

Operators in Python and built-in Functions.
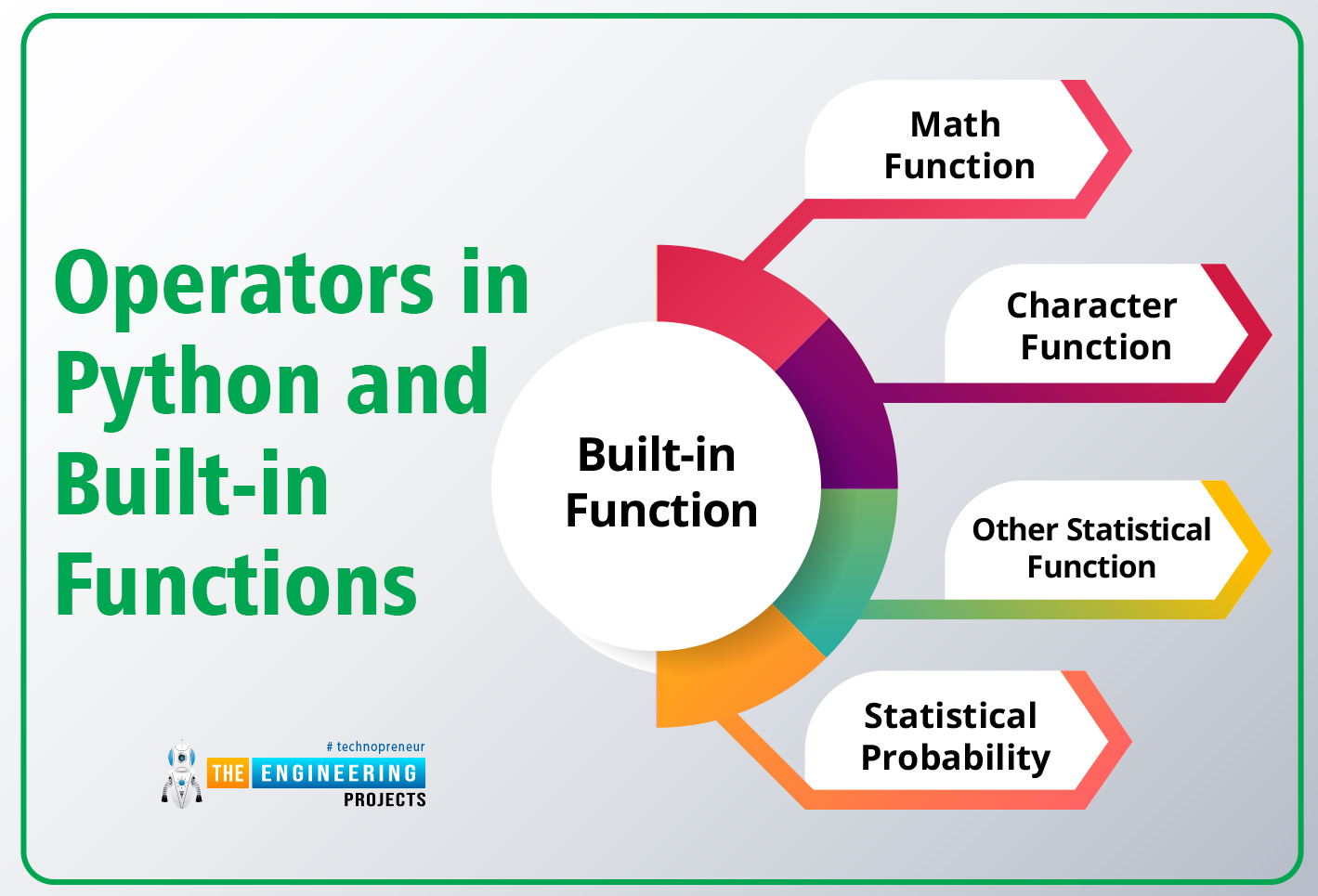
A large number of the built-in and available operators and functions for working with texts, lists, or tuples will be known to you at this point. As well as other dictionaries, several of these are useful.
Using the in and not in operators, for example, you can determine whether or not an operand is a dictionary key.
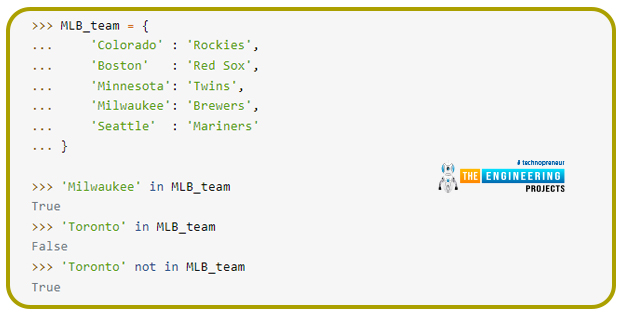
A key that is not in the dictionary can be avoided by using the in operator and short circuit evaluation:
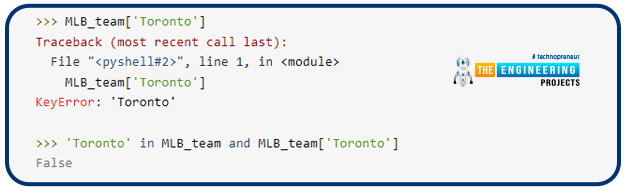
Dictionary key-value pairs are counted using the len() method.
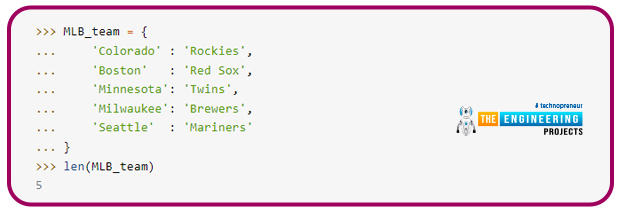
Which are the Dictionary's built-in functions?
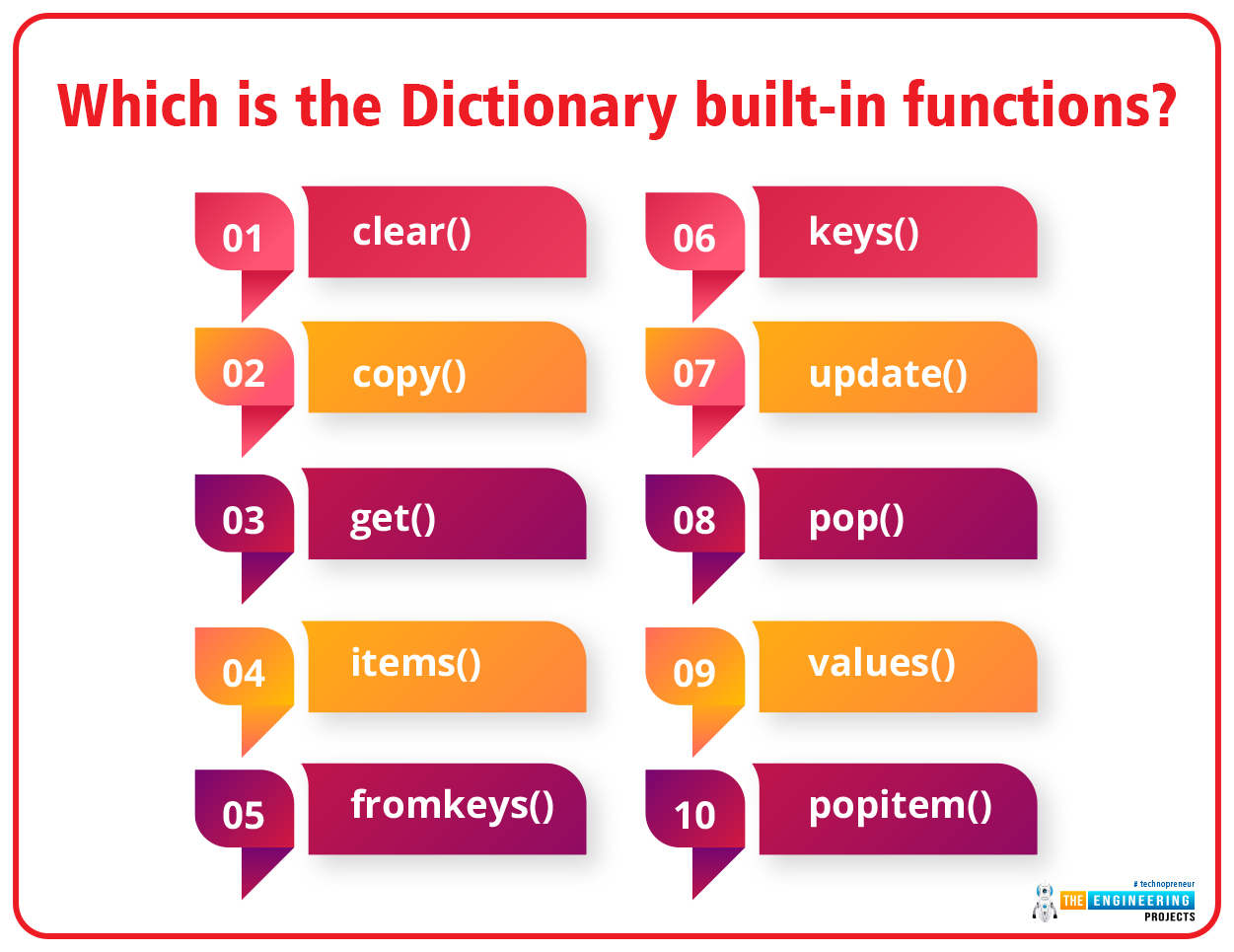
Similarly, to strings and lists, dictionaries include built-in methods that can be used. Although lists and dictionaries share names in some circumstances, it is not always the case. Since various kinds might have methods with the same names, this is totally appropriate when discussing object-oriented programming.)
There are a few approaches that can be used for dictionaries:
d.clear()
Clears elements in the dictionary.
The function d.clear() removes all key-value pairs from the dictionary d:
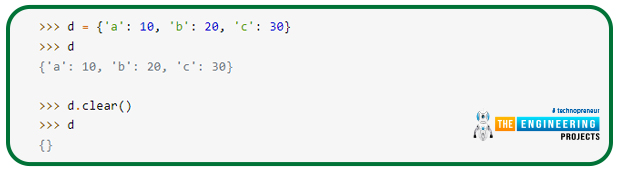
d.get(<key>[, <default>])
Whether or not a key exists in the dictionary is checked, and the value associated with it is returned.
This method can be used to retrieve the value of any key without having to verify that the key exists.
If a value matching the supplied key (<key>) is discovered in dictionary d, Get (<key>) returns it. If it is unable to locate the appropriate key, this procedure returns 0.
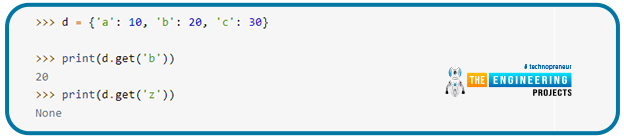
We're going to use -1 instead of None in case <key> could not be located and the <default> argument had been provided.

items(“value”)
The key-values` list in a dictionary is returned by this command.
items(‘value’) will return a tuple`s list containing the values in d. Key value tuples consist of two items: the key and the value.
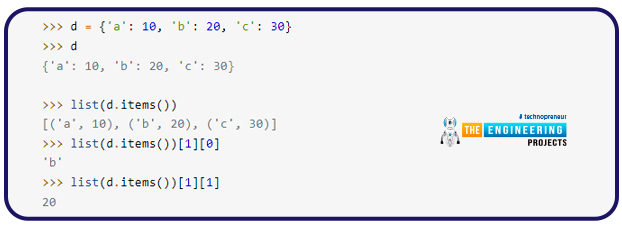
d.keys()
This function will return a list of the dictionary's keys.
A list of all d keys can be found using d.keys()
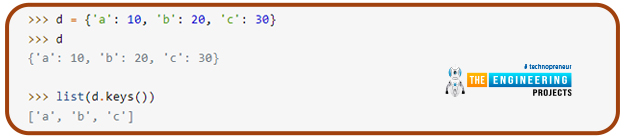
d.values()
Values of dictionary are returned in response to this method.
The list of all values in d is returned by d.values()
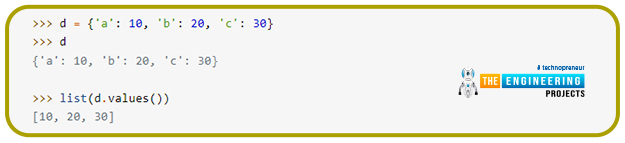
If d contains any duplicate values, all of those values will be returned:
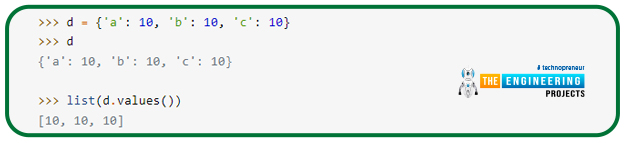
A "view object," which is a collection of objects, keys, and values, is returned by these functions. The keys and values of a dictionary can be seen through a similar window in a dictionary view object, the keys and values of the dictionary are returned as an array by these methods.
pop(key)
A key is removed from the dictionary and its value is returned if it exists.
pop(key) will remove key and return its related key when <key> is in d:
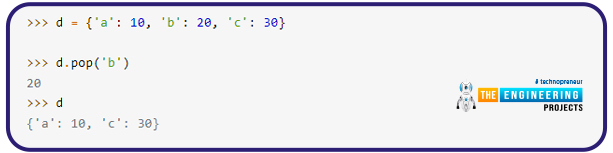
d.popitem()
In a dictionary, this function removes one key-value pair.
d.popitem() will remove and return any last key pair added to d.
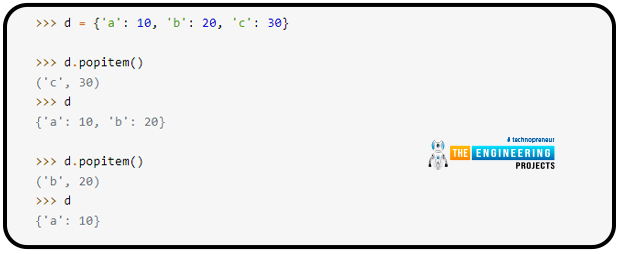
d.update(<obj>)
Merges the dictionary and iterable key-value pairs.
A dictionary can be updated with update(<obj>), which merges all of the items from obj>. For each key in obj>:
If the value pairs from obj do not already exist in d, they are added to d.
Changes to the value of the key in d are made by use of values from <obj>.
The following is an example of how two dictionaries can be combined:
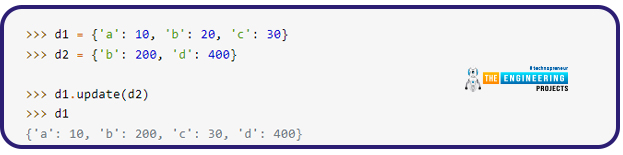
Therefore, its value is set to 200 because b is contained in d1, which is derived from d2's entry for that key. D1 does not include the key d, hence the key-value pair is taken from d2.
Key-value pairs can also be utilized to define a dictionary using the dict() function. As an example, a list of tuples, such as obj:

It is also possible to specify the values to merge in the form of a list of keywords:

Conclusion
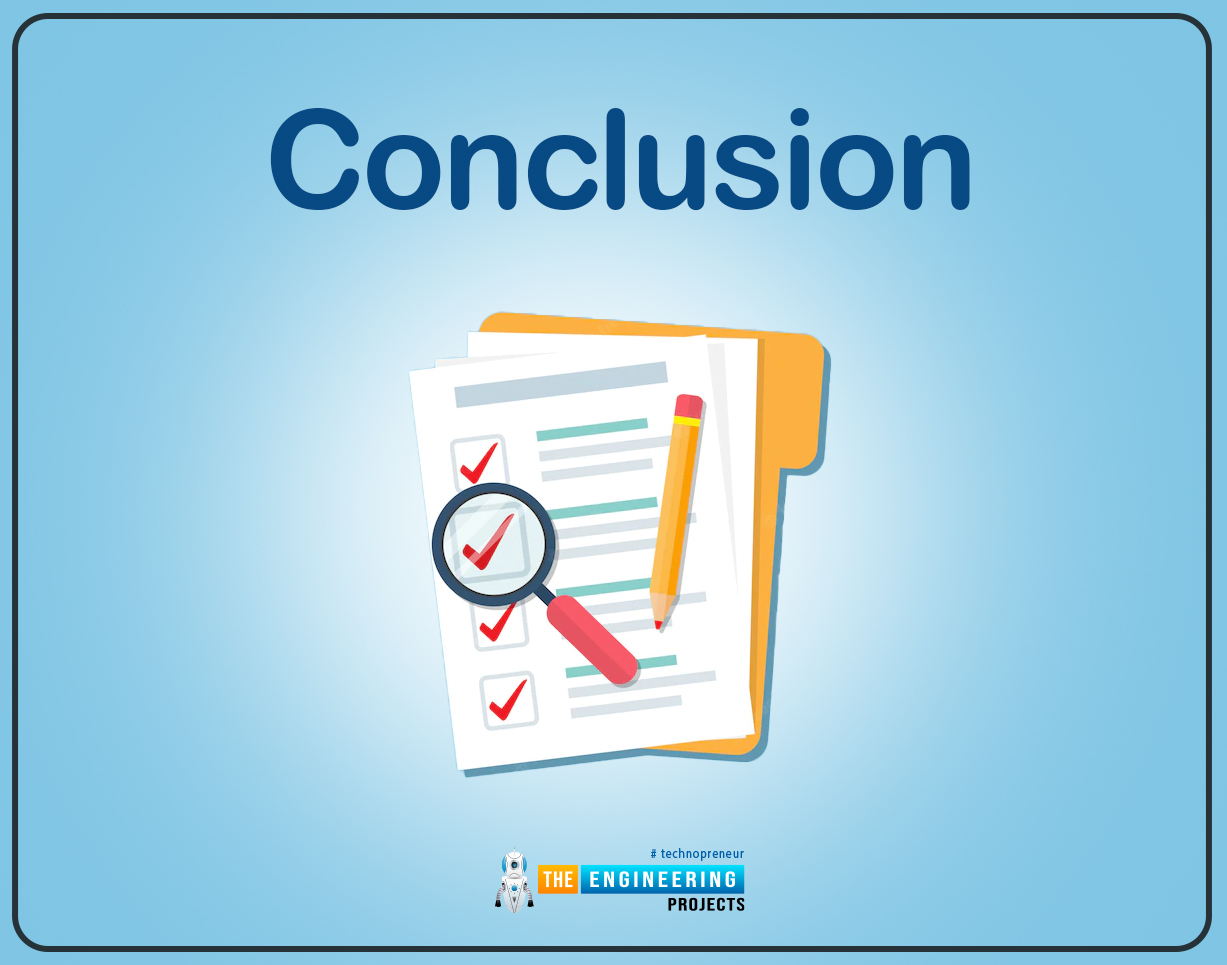
Throughout this course, you learned how to read and manipulate the data in a Python dictionary.
Lists and dictionaries are two of Python's most commonly used data structures. It is clear from the comparison that they have many commonalities yet are different in how their elements can be found. Index numbers are used to locate items in lists, and keys are used to locate items in dictionaries.
Therefore, lists and dictionaries are appropriate for different circumstances. You should now be able to tell whether one or the other is the best option in a given case.
Python sets are the next topic you'll study. Unlike lists and dictionaries, the set is not a simple collection of elements.