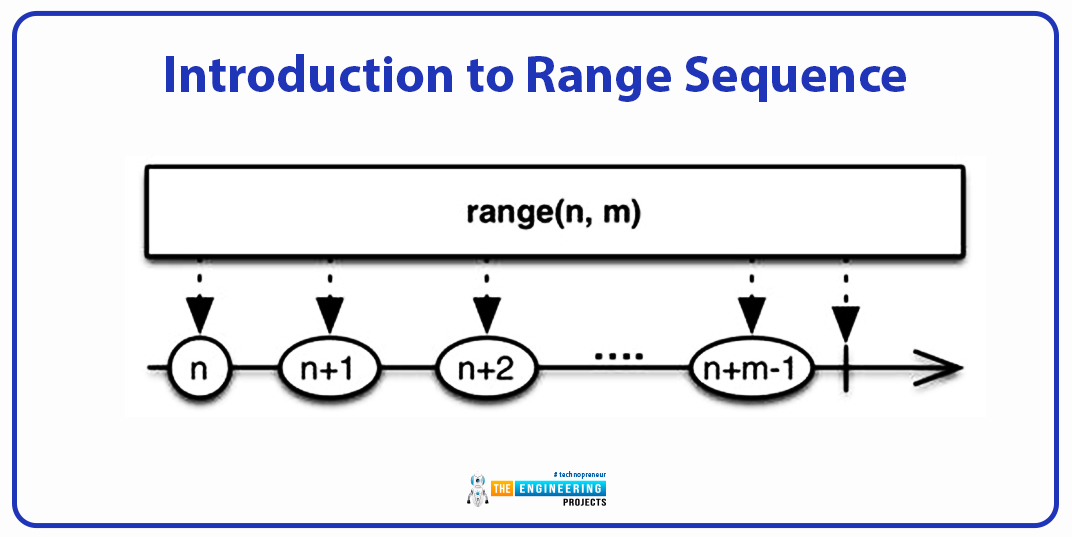
Hey peeps! Welcome to the new lecture on the sequence data type, where we are discussing the range data type. We are interested in working on deep learning, and for this, we are learning the Python programming language from scratch. If we talk about the previous episode, we saw the byte and byte array methods that were amazing for converting the different data types into bytes. The current lecture will discuss the range data type, which is slightly different from the other types of sequences, so students will learn new and interesting concepts in the lecture; however, before we get into the details of our topic, take a look at today's highlights:
What is the range function?
How can you elaborate on the syntax of the range function in detail?
What are the three types of range functions?
Give us some examples of range functions in Python.
What are some basic questions the answer of which should be kept in mind while using the range function?
The answer to each question above will be provided before the end of this lecture. All the examples will be tried on TensorFlow for better understanding.
Introduction to Range Sequence
The range is a type of sequence in the data type that also represents the group or collection of the items together in different ways, just like other types of sequences. It is also the built-in function in Python, and while using it, the programmer gets the range object. The range is one of my favorite data types because it is easy to use and, in just a few simple steps, it provides us with the sequence of the integers according to our choice. Usually, the loops play an important role while dealing with the range function. Right now, as we have not learned about loops, you will simply have an idea of the workings and output of this data type.
The good thing about using the range function is that, unlike loops, the programmer does not have to use the logic behind the series but just has to put the values in the range function, and the results are great. Keep in mind that the range function is used with the loops, and there is less versatility in the range function when compared with the simple logical loops, but for basic workings, it is important to learn about the range, and this function is great.
Syntax of Range Function
The syntax of the range function is also easy, just like its group mates. You have to know about the three parameters and will determine all of them according to your requirements:
MyRange=range(start,stop,step):
for i in range(MyRange)
print(i)
Here, the semicolon at the end indicates that the syntax of the range function is complete, and the compiler now has to calculate the range arguments. Furthermore, if the programmer wants the result to appear on the same line as the interval, he can add end=" " at the end of the print. In this way, the compiler will not jump to the next line, but the results will be printed on the same line with a space between each element. Of course, the programmer has to save the result of the range function into a variable so that it can be used in the other functions. But here, it is important to mention that all of these parameters are not compulsory, but the range function gives you the independence to use one, two, or three of them.
For loop in Python
The for loop is the iteration in the programming languages and you will learn them in detail in the coming lectures but for now, keep in mind that the range function alone can not do anything but it is fed into the for loop so that compiler can work on the iterations. The variable (usually i) is used in this loop and the results of the range function are input in this loop.
Types of Range Functions in Python
Another thing that must be mentioned here is the programmer has to choose the number of arguments according to the complexity of the series of numbers he or she wants. So here are the details of each case:
range(stop)
This is the most basic type of range function, in which the programmer simply specifies the point where the compiler has to stop making the range series. In all types of range functions, there is always a need for a stop parameter. Three things are to be mentioned here:
By default, the range starts at zero, and if the user does not have any particular choice for the start, the range function can be used with the only stop parameter.
Only whole numbers are printed on the screen.
The stop number, which is the limit of the range function, will not be printed on the screen.
When you put this value equal to zero, the result will be an empty range and you will get nothing.
for i in range(3):
print(i,end=" ")
range(start,stop)
Just think about the case where the default value, which is zero, is not to be used. Instead, the programmer has the option of printing the series of numbers without missing any of them and then specifying the start and stop ranges in the range function. But, as in the previous case, the stop number will not be printed on the screen, so you have to give the range of stops that you do not want on the screen, but the number before it is required there.
for i in range(3,34):
print(i,end=" ")
range(start,stop, step)
The third function, as expected, is the complete range function, into which the programmer feeds another step parameter. With the help of this, the programmers are able to get the series of numbers that starts from the point they want and have uniform intervals between the numbers and the ending point that is expected by the number. In short, the whole series is under the control of the programmer, but you have to notice that the steps are always uniform. The step function must not be zero and you will get the reason for this statement soon in this lecture. We can put the step value in the code discussed above and in this way, if 2 is the step value, the programmers will have half of the series as given above.
for i in range(3,34,2):
print(i,end=" ")
Range Function Examples in TensorFlow
Here comes the action because, till now, the examples you have seen are simple examples with a simple series, but now, we are dealing with some exceptional cases that will clear some related concepts in your mind. We have divided the examples into some questions, and we will try to get the answers with the help of codes:
Can we use float in the range function?
Till now, integers are being used in the range function but we know that integers and floats are the two most related data types and will try to attempt the range function with the help of floating values as the parameters.
for i in range(3.5,77):
print(i,end=" ")
As you can see, the compiler is throwing the error that it is not possible to use the float in the range function because it is designed only for integers. The same program will run when you remove the decimal part from the first value, which is the starting point.
The Intertool Chain Method
Let me tell you the interesting way to get the range series with the help of inter tool chain method. But before this, you have to look at the basic definition of this tool.
“The iter-tool iterator is the pre-define module in python that provides the complex applications of the iteration in simple ways. The methods are defined in this module, and the programmers have to import them before using them.”
So, the chain method is also saved in this method, and when the programmers need to use them in a different way, they simply use the import keyword and use it in programs. As we are dealing with the range function, the iter-tool chain function is used to connect the results of two or more results in the form of a single series. Have a look at the code given next, and then read this paragraph again to get the point.
#import the chain method from the iter-tool library
from itertools import chain
# Printing two methods in a row
print("Concatenating the result")
MyChain = chain(range(4,7), range(34,55,2))
#using the method in the range
for i in MyChain:
print(i, end=" ")
The extraction of the concepts used in this program:
We can import the chain method from the library of itertools that have the iteration tools in it.
To import the method, we use from and import keywords that are represented with the green bold colour in the program.
Concatenation is the process of connecting two or more data types into a single line.
When using concatenation, the for loop is used by making a variable and saving the results of two connected ranges together in the variable.
The independence to use the number of arguments between one to three is the same in the concatenation as in all cases.
In the for loop, when using concatenation, only a variable is used.
The other way to get the same results is by using both ranges with the for loop, but the code will not be very clear in that case.
If the programmer wants to get the results in column form, he or she can simply delete the “end” part in the code.
Can we access the Range function with the index value?
The simple answer to the question is yes, and when we go into the details, the range function simply gets the indexes the programmer wants and can provide them with the single values they require. In simple words, the programmer tells the range function its stop value, and it assumes the whole series and picks the one number demanded by the programmer. The stop range is described in parentheses when the index to be picked is mentioned in the square bracelets.
#Give the range and pick the element through the index
MyRange = range(5)[2]
print("3rd element out of 5 =", MyRange)
print()
MyRange = range(3,34)[23]
print("23rd element of this range with start and stop value =", MyRange)
print()
MyRange = range(28)[5]
print("5th element of this range with start, stop, and step value =", MyRange)
Hence, the programmer can make a range of choices and then pick one element.
What if the step is kept at zero in the range method
During the discussion of step, we saw the basic discussion of the step argument but keep in mind, if the programmer does not want the step function, he can simply ignore it. There is not need to input the step function as zero because, in such cases, the error will be shown on the screen.
for i in range(3,23,0):
print(i,end=" ")
Hence, from the above code, it is clear that the range of the stop argument is always greater than zero. Moreover, in the same code, if the value of the step argument is greater than the stop argument, it just shows the starting point of the range and does not give the other values or any errors because logically, it is true.
Truss, in this lecture, we saw many interesting concepts about the type of sequence called range function. This is a pre-defined function that is used to represent the group of numbers, and we can control the starting, ending, and interval values between the series of this number according to our wishes. This is always used with the for loop, and different cases of range functions were discussed in this lecture. Stay with us for more Python tutorials.