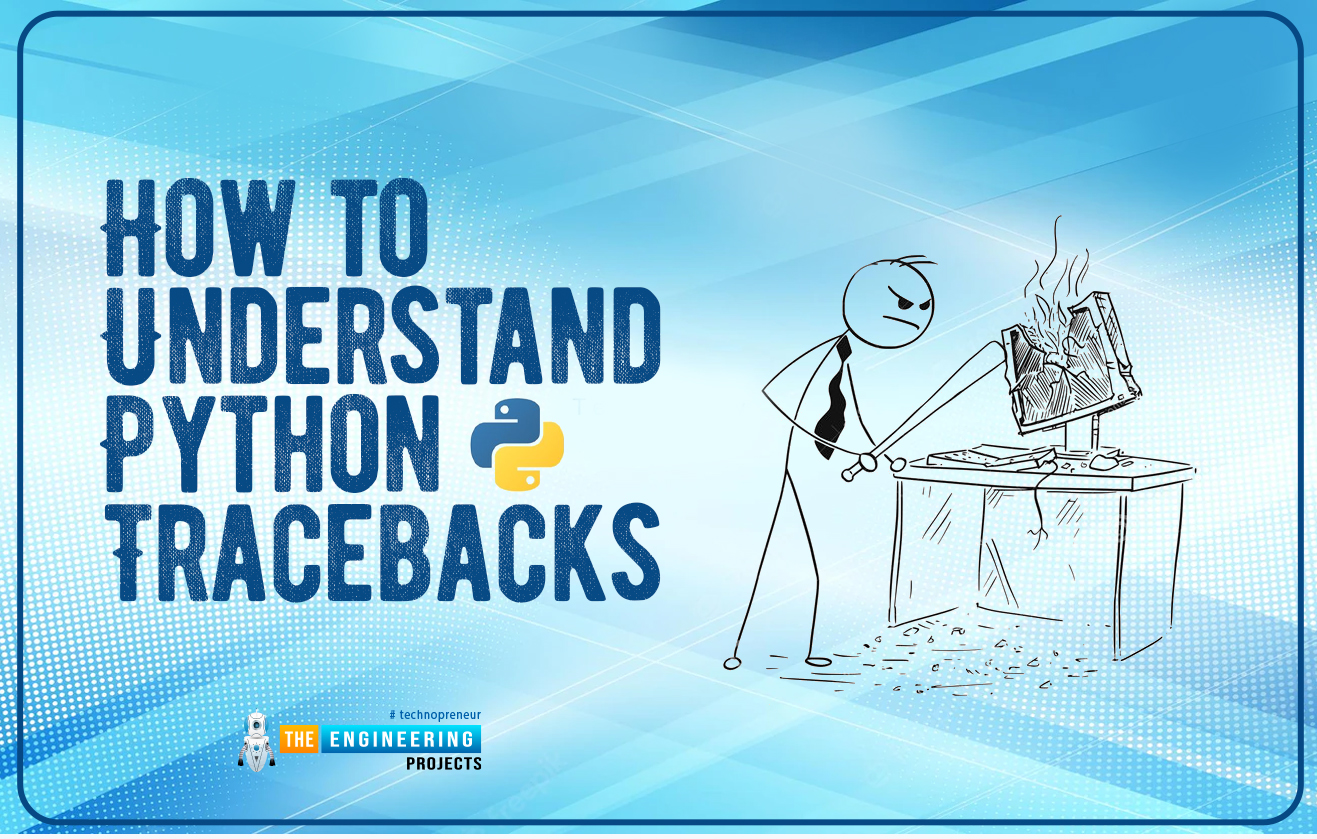
Any time an exception is thrown in your code, Python shows you the stack trace. If you don't know what the traceback output is showing you, it can be a little overwhelming. Python's traceback, on the other hand, provides a goldmine of information that can assist you in figuring out why an exception was triggered in your code and fix it. It's essential to learn how to use Python traceback to become a better coder.
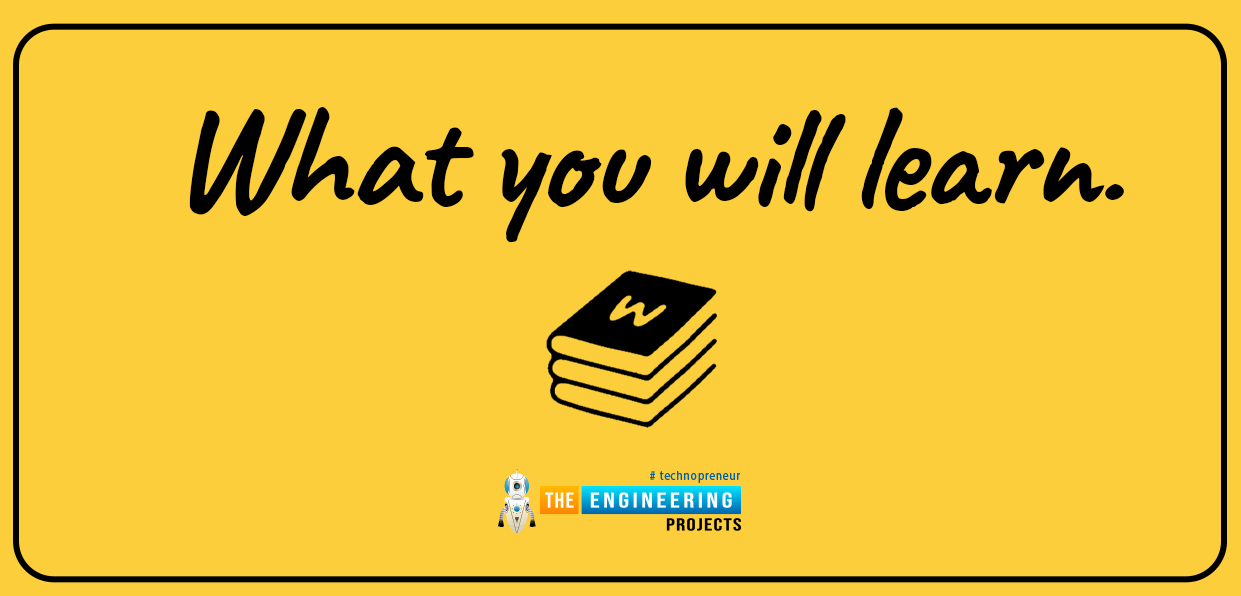
After completing this session, you will be able to do the following:
- Interpret the next error message you come across.
- Find out how to identify some of the most typical repercussions
- Successfully log a traceback while addressing the exception.
Python Traceback: What Is It?
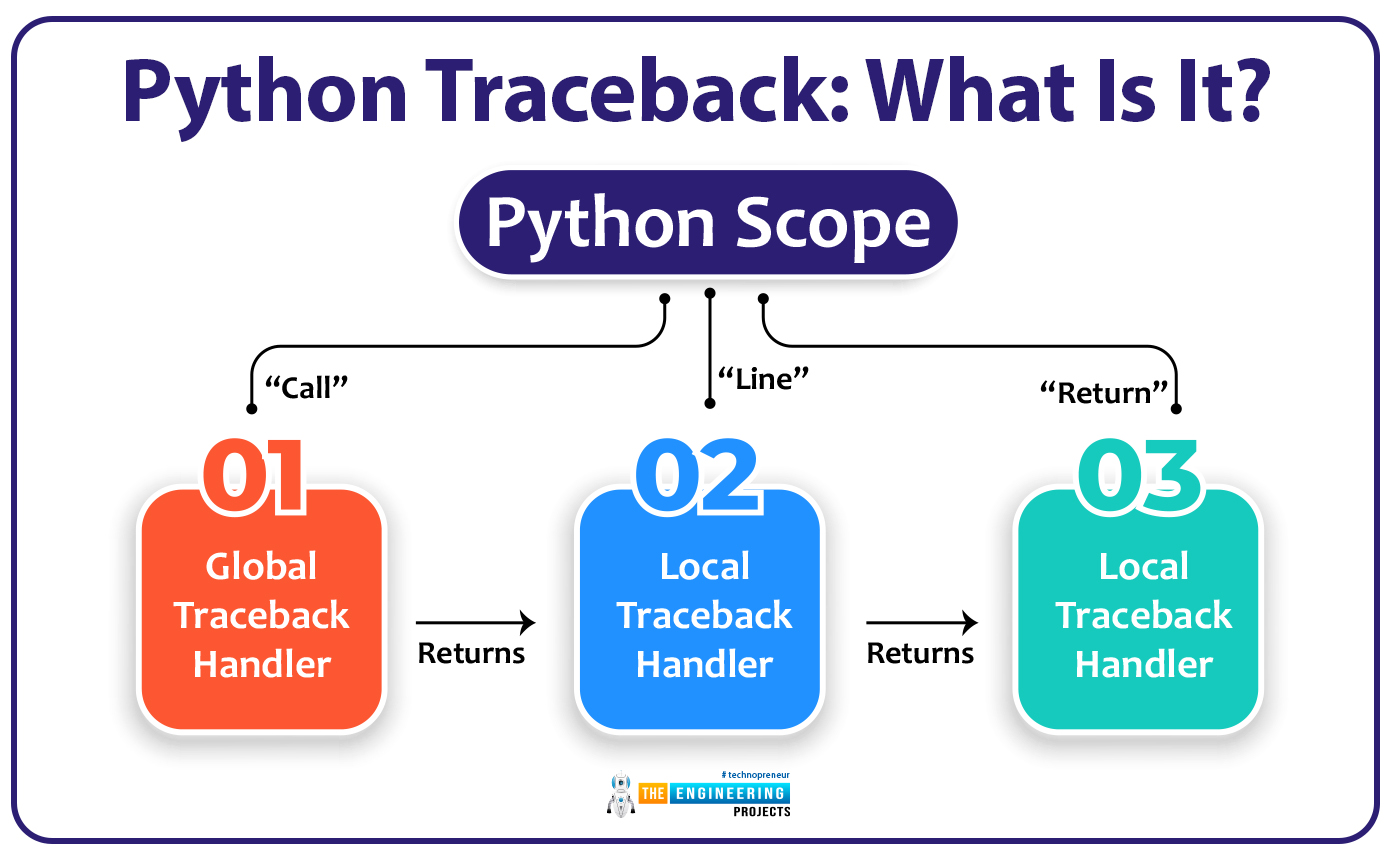
This is a list of all the function calls you made at a certain time in your code. Stack traces, stack tracebacks, backtraces, and maybe other terms refer to tracebacks. Traceback is the term used in Python. Python will report the current traceback if your program throws an exception, so you can figure out what went wrong. The following is an example of how this may play out:
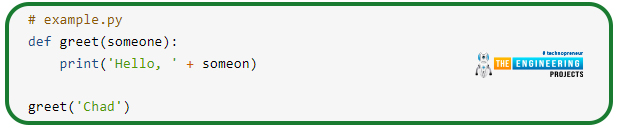
Someone is passed to greet() as an argument. But in greet(), the name of the variable is not used. In the print() call, the word someon was used instead. On startup, you'll get a traceback like this:
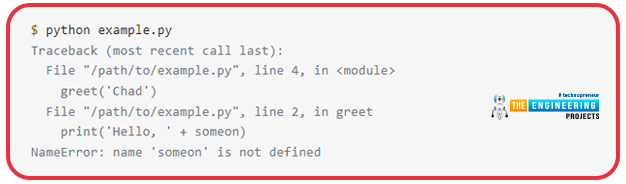
All of the details you need to investigate the problem can be found in this traceback output. What sort of exception was thrown and what information about it can be found in the last line of the traceback report? Using the traceback, it is possible to identify the code that caused the exception to be raised. When an exception like the one seen above occurs, it implies that a reference to an undefined name (variable, function, or class) is being used. Somebody is the person being referred to in this instance.
Here, the final line gives you enough information to figure out how to fix the issue. You may find the correct code by searching for the misspelled name "someone" in the source code. However, it is common for your code to be far more complex.
How Do You Read Python Traceback?
When you're attempting to figure out what caused an exception to be thrown in your code, the Python traceback provides a wealth of information. Throughout this part, you'll learn about the many pieces of information that can be found in a traceback.
Overview of Python Tracebacks
It is critical to pay attention to each section of a Python traceback. As shown in the picture below, there are several different components:
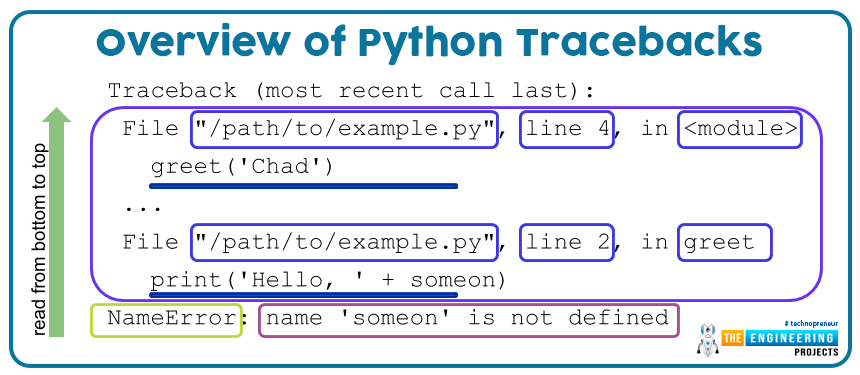
Using Python, it's better to start at the bottom and work your way up the traceback
- The error message appears in the blue box at the end of the traceback. It includes the name of the exception that was thrown.
- The error message appears in the green box to the right of the exception name. In most cases, this message provides useful information about the cause of the exception.
- Box in yellow: The function calls that have been made most recently to least recently can be seen farther along with the traceback. Each of these phone calls is represented by a two-line entry. For example, the file name, line number, and module identifiers are all included in each call's initial line.
- Red underline: This is the actual code that was run on the second line of these calls.
For example, traceback output differs between command-line execution and the REPL's execution of code. The same code from the previous section was run in a REPL and the traceback output is shown below:
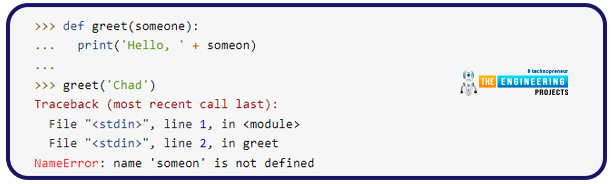
You'll see "<stdin>" in place of filenames. This makes it reasonable because you entered the code using normal input. In addition, the traceback does not indicate the executed lines of code. You may notice a big difference in the look of a Python traceback compared to other programming languages' stack traces. Other languages often begin at the top and work their way down the list, going from the most recent calls to the oldest ones, in that order.
A Step-by-Step Guide to Retracing Your Steps
You can get a better idea of what information the traceback will give you by going through some of the traceback output. In the following instances, the traceback information provided by Python is illustrated using the code below:
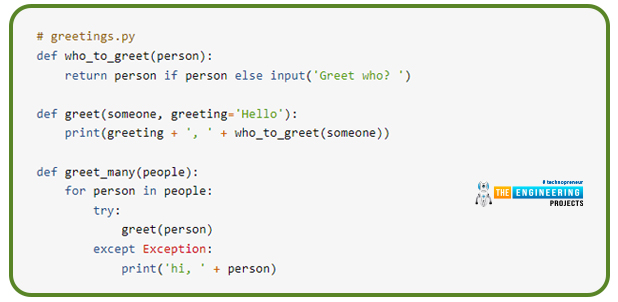
who to greet receives a value, a person, and either return it or prompts for a value to return instead. Then welcome() takes a name to be greeted, a person and an optional greeting value, and calls print() (). This function is also invoked with the passed-in value of "someone."
Finally, greet many() calls greet after iterating through the list of persons (). If welcome() returns an error, then a simple backup greeting is printed instead of the original greeting. There are no issues in this code that would cause an exception to be thrown if the correct input is provided. You'll see the following traceback if you call greet() at the bottom of greetings.py with a keyword argument that it doesn't expect (for example greet('Chad', greting='Yo’)).
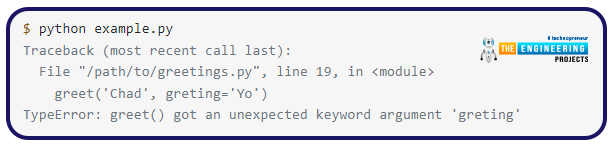
If you're dealing with a Python traceback, you should always start by tracing backward. The traceback shows that the exception was a TypeError at the end of the last line. Everything after the colon in the message after the exception type gives you a wealth of information. It informs you that a keyword argument was passed to greet() that it wasn't expecting. Greting is the name of the unknown argument. The line that caused the exception can be seen as you move up the tree. We inserted the greet() code at the bottom of greetings.py in this example.
There is a second line of information that tells you exactly where the code is, what module it's in, and how you may get to it. module> indicates that this is the file that is being executed in this situation since our code doesn't use any other Python modules. Using a new file and different input, you can see where the traceback is leading you. Remove the faulty greet() function from greetings.py and add this file to your directory: greetings.py
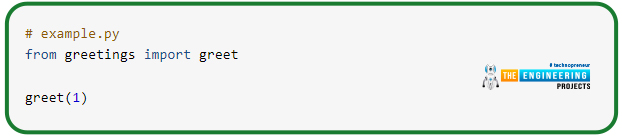
You've created a new Python file, greetings.py, which imports greetings.py and uses greet(). If you now run example.py, you'll see what I mean:
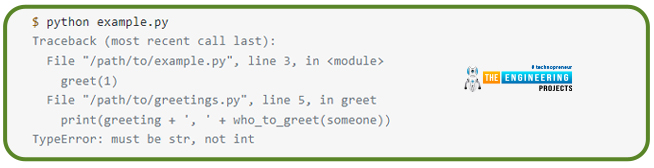
This time, a TypeError is thrown, but the message it contains is less instructive. It was expecting to deal with a string but instead received an integer at some point in the program's code. You may see the code that was performed by moving up a few levels. The code's location and filename are then provided. Greet is the function name instead of module> this time around ().
The incorrect greet() call is now passed to the next line of code to be executed. When an exception is thrown, it may be caught by another piece of code, which will then throw an exception of its own. Even if there are many exception tracebacks, Python will always print the traceback of the most recently raised one first.
Here's an example to help clear things up. The bottom of welcomes should include a call to greet many(). py:

All three people should receive printed greetings as a result of this. To see an example of several tracebacks being output, run this code.
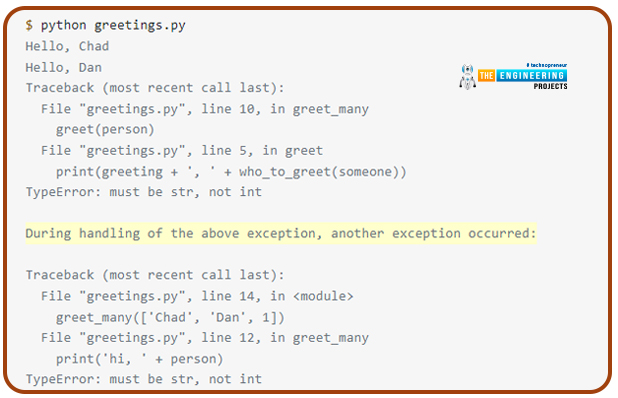
In the output above, look for the highlighted line that begins with the phrase "During handling." This line appears in the middle of every traceback. Its message is crystal clear: another exception was produced while your code was trying to handle the prior exception.
Previously, when you called greet() with an integer, you saw the same problem. We can assume the same outcome because we added a 1 to the list of individuals to greet. The welcome() call is redirected to a try-and-except block in the greet many() method. If greet() throws an exception, greet many() will output a generic welcome.
Greetings.py's pertinent paragraph is reprinted here.
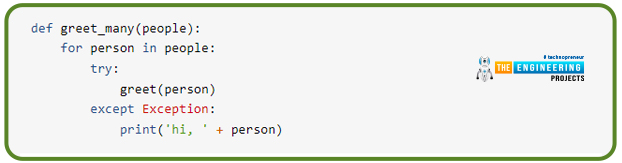
As a result, greet many() attempts to produce a simple greeting when greet() fails due to a TypeError caused by an invalid integer input. In this case, the code results in a similar exception. However, it's still attempting to combine two different types of data. Traceback output can help you identify the root cause of an exception by displaying all of the possible causes. When you view the last exception and its traceback, you may not be able to figure out what went wrong. Moving up to the prior exceptions will usually give you a clearer grasp of the underlying problem in these situations as well.
The Most Common Python Tracebacks
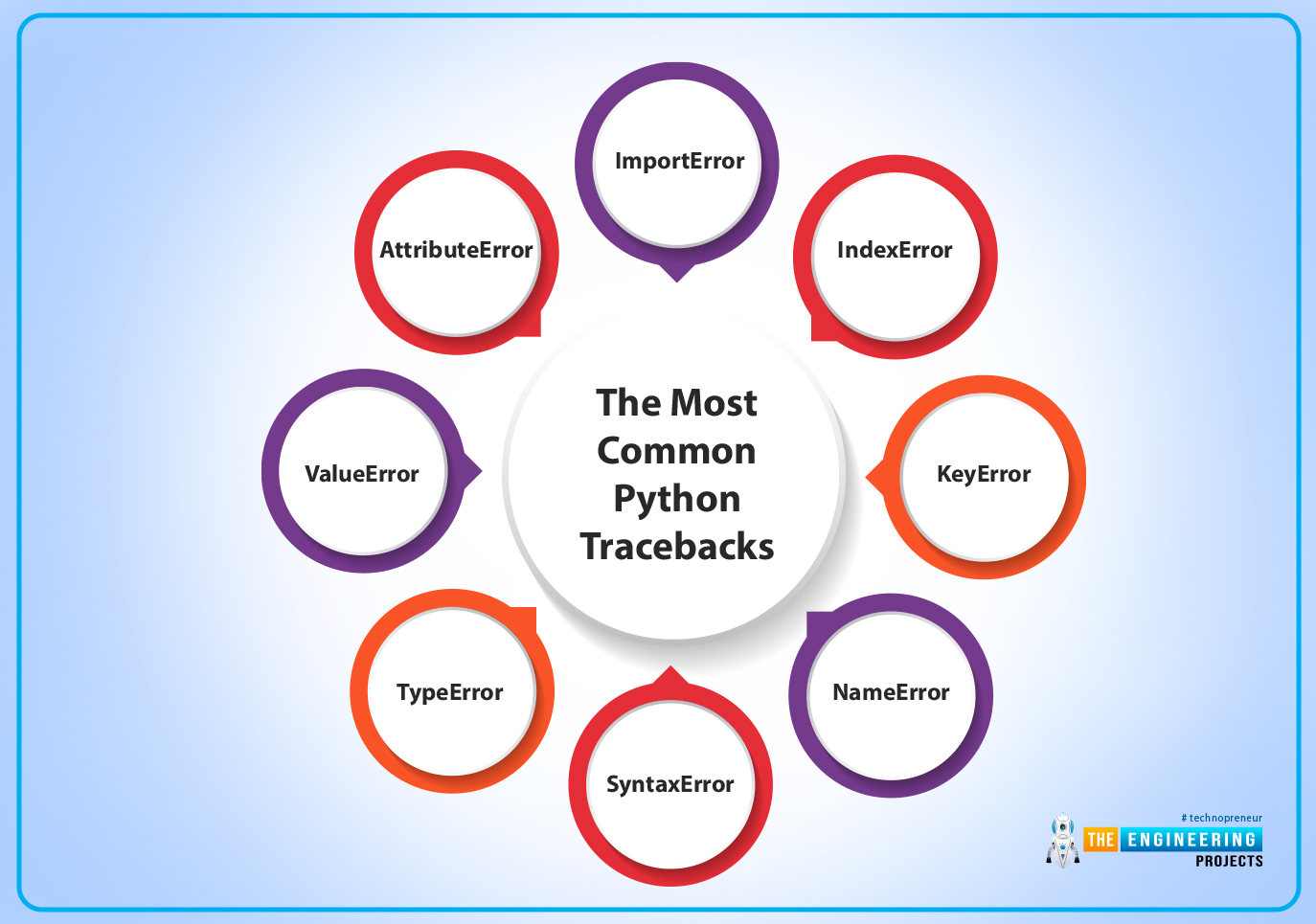
When your program throws an exception, it's helpful to know how to read a Python traceback, but it's also helpful to know some of the most typical tracebacks. Listed here are some of the most typical exceptions you'll encounter, along with the reasons they're raised and what they mean, and how to track them down.
AttributeError
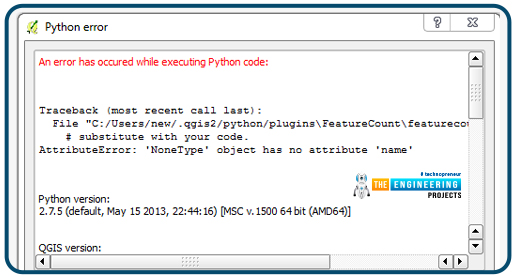
If you attempt to access an attribute on an object that does not have that attribute declared, you will receive an AttributeError exception. When this exception is thrown, according to the Python manual:
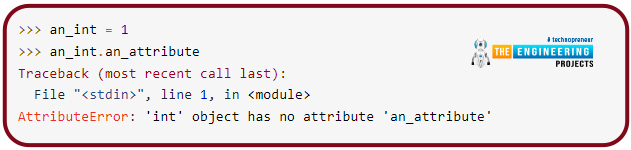
AttributeErrors have an error message indicating that a certain object type, in this example an int, does not have the attribute accessed, an attribute. If you see the AttributeError in the error message, it will assist you to identify the attribute you attempted to access and where you need to go to fix the problem.
If you get this exception, it's likely because you're working with an object that isn't what you expected.
ImportError
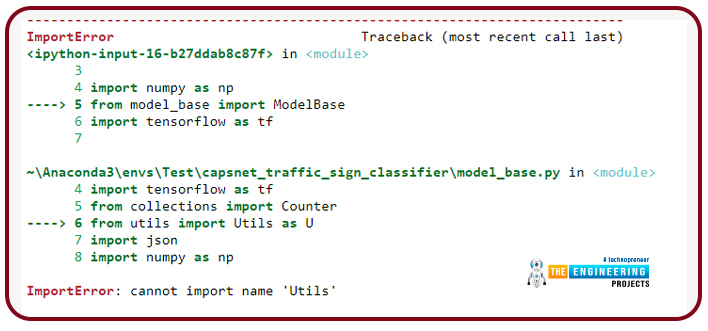
An ImportError is thrown when an import statement fails. The ModuleNotFoundError exception or a subclass thereof will be thrown if you try to import anything from a module that does not exist in the module you are trying to import it from. When this exception is thrown, according to the Python manual:
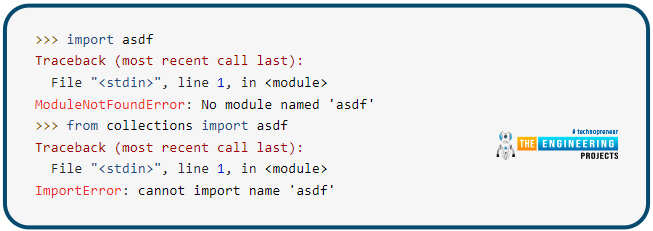
The ModuleNotFoundError occurs when an attempt is made to import a module that does not exist, like in the above example. An ImportError is thrown if you try to import something that doesn't exist from a module that does exist. Both asdf and asdf can't be imported because of the error message lines at the bottom of the tracebacks.
NameError
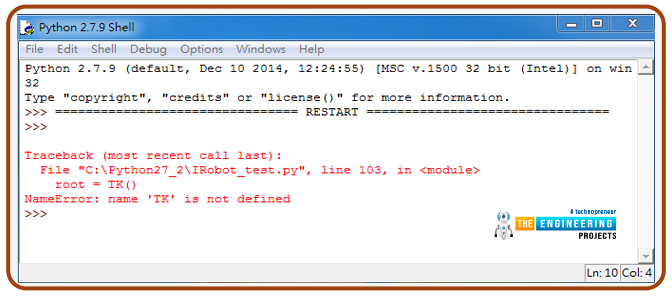
What causes the NameError to be raised is the fact that you've used a name that hasn't been specified in your code. When this exception is thrown, according to the Python manual:
Greet() in the following code accepts a person as a parameter. Persn, on the other hand, is the incorrect spelling of this parameter in the function itself:
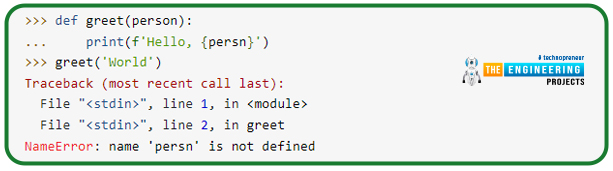
Your missing name can be found in the NameError traceback error message. In the example above, a misspelled variable or parameter was passed into the function.
If you misspelled the parameter, you'll get a NameError:
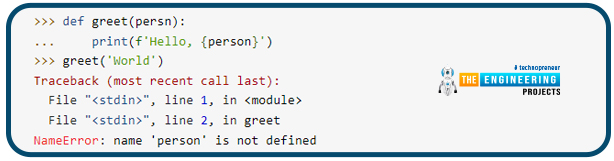
In this situation, it may appear as if you've done nothing wrong at all. A clean traceback can be seen after the last line of code was executed. Look through your code to see where the person variable is used and defined if you find yourself in this circumstance. An error in the parameter name can be easily seen in this example.
How Do You Log a Traceback?
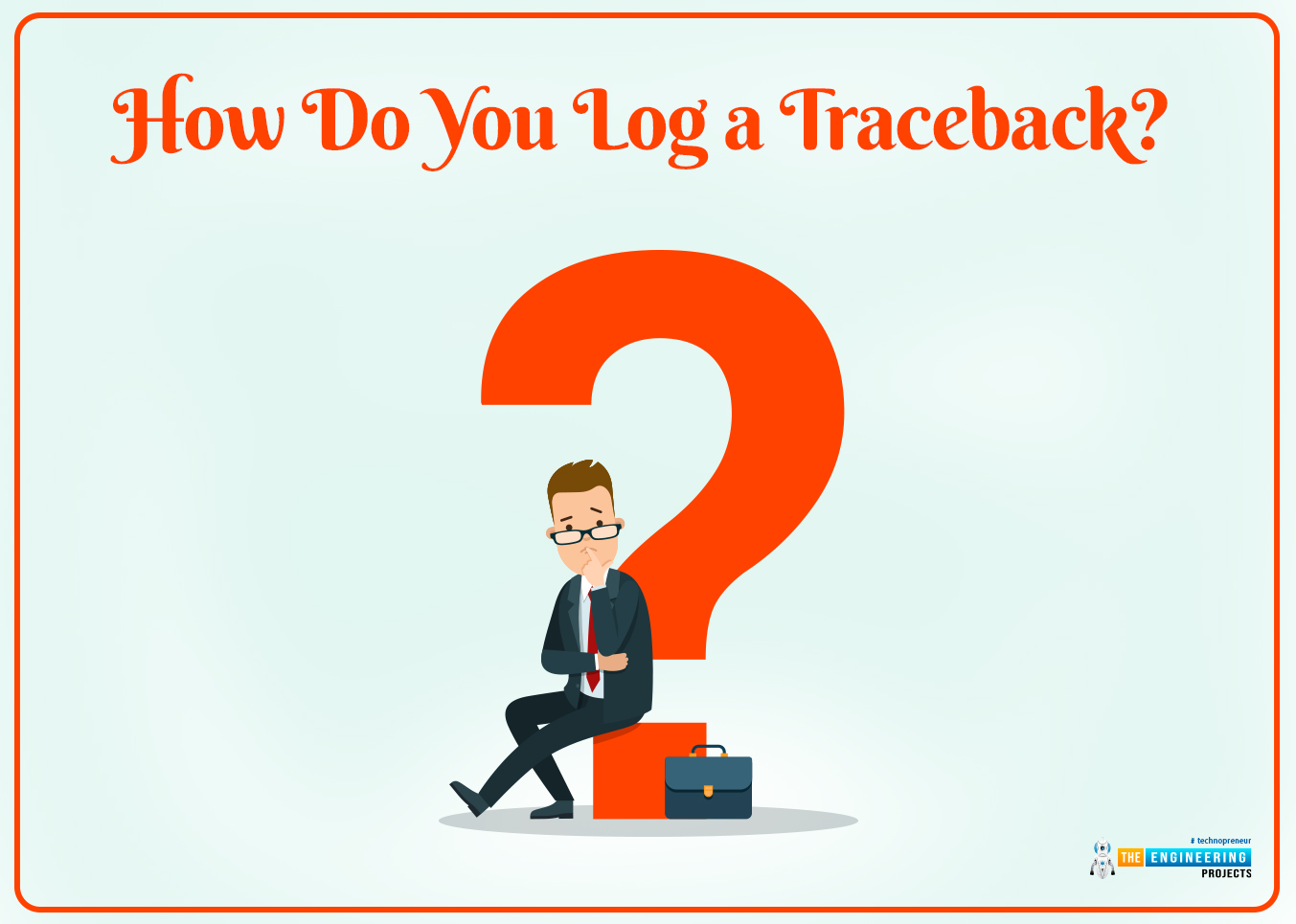
Making a decision after receiving a Python exception and associated traceback can be difficult. It's always a good idea to address your code first, however, in certain cases, the problem is caused by inaccurate or unexpected user input. There are instances when silence or hiding an exception by logging the traceback and doing anything else is more appropriate than providing for those situations in your code.
The following code needs to suppress some Python tracebacks in the real world. The requests library is used in this example.
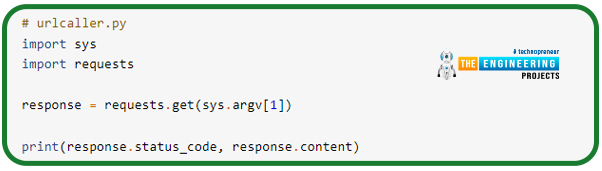
This code works perfectly. A command-line argument is required to launch this script, which will call the URL and print its HTTP status code and response content. Even if the answer contained an HTTP error status, it still works:

Your script may be unable to obtain a URL because it does not exist or the host server is unavailable. Uncaught ConnectionError exceptions and tracebacks will now be raised and printed in those circumstances.
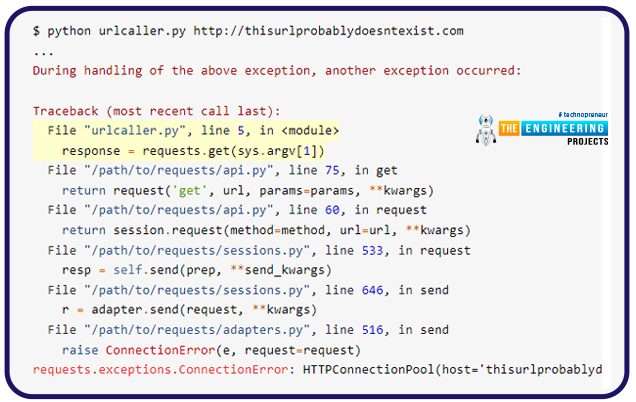
Many other exceptions may be raised before the ConnectionError is raised by the requests themselves in the Python traceback. Line 5 of urlcaller.py is where the trouble began, as you can see in the final exceptions traceback.
For example, if you put the offending line in a try and except block, you can catch the proper error and continue to work:
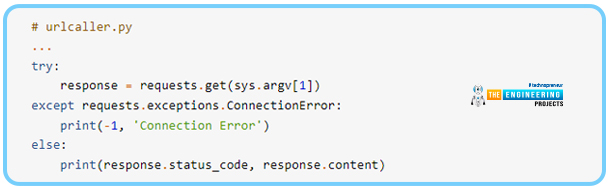
Instead of using a try/except block, the above code uses an else clause. To learn more about this feature of Python, see the section on else clauses in Python Exceptions: Introductory remark
You'll now see a -1 for the status code and the text "Connection Error:" written when the script is run with a URL that raises a ConnectionError.

This is fantastic. But in most real-world systems, you want to log the traceback rather than just mute the exception and its traceback. Tracebacks, help you figure out what's going wrong with your programs. It is possible to log the traceback in the script by importing the logging package, creating an exception, and calling.exception() on the logger in the except portion of the try and except block. In the end, your script should look like this:
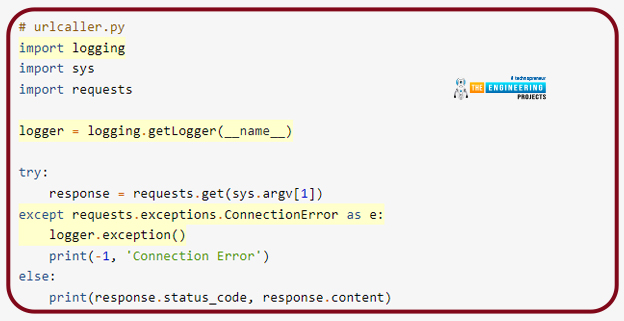
Conclusion
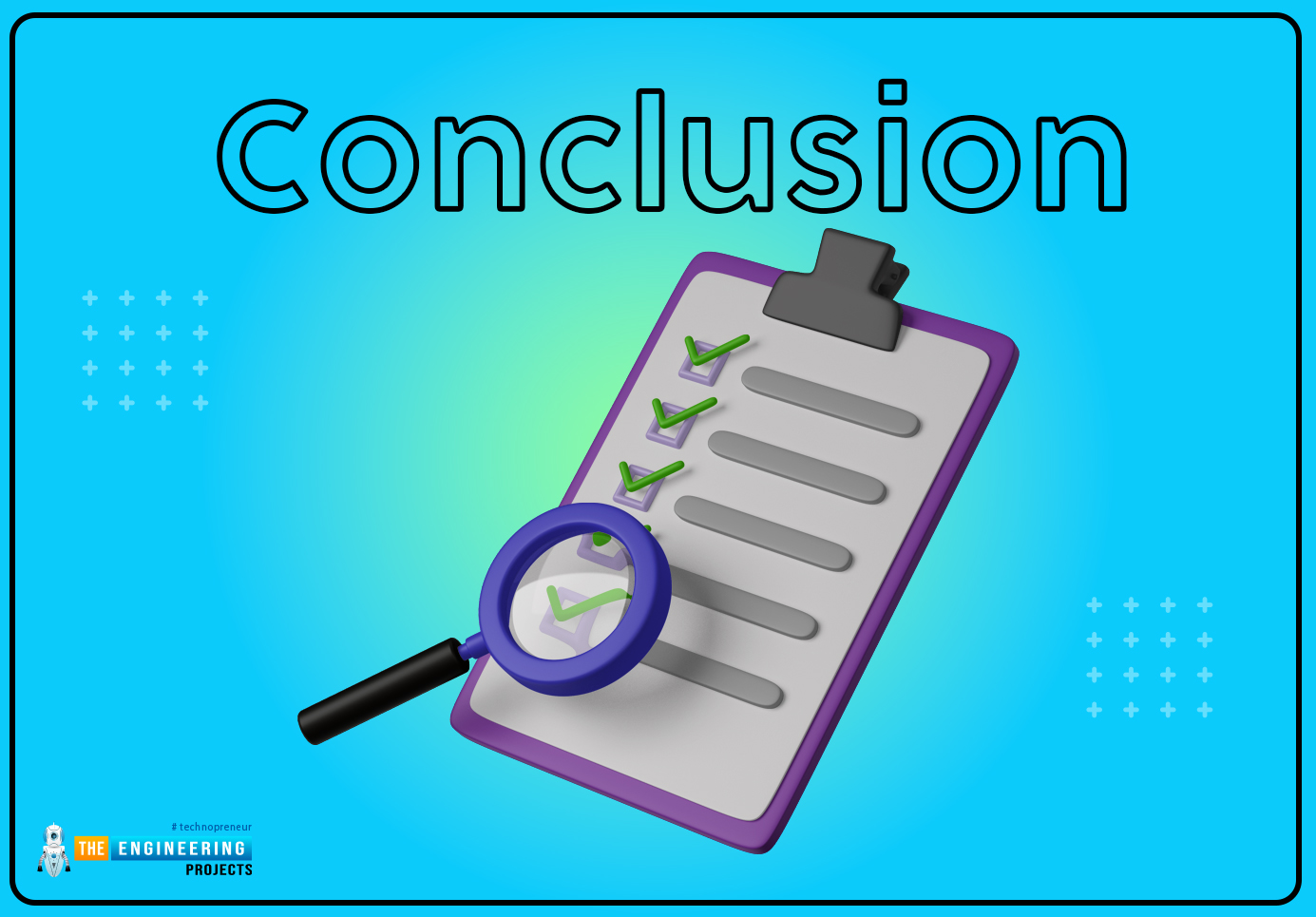
Using Python traceback is a wonderful way to find out what is wrong with your code. These tracebacks may appear frightening at first, but if you understand what they're trying to tell you, they can be really useful. You may get the most out of tracebacks if you go through a few of them line by line. When you execute your program and get a Python traceback, you have an opportunity to make improvements to your code. Python does its best to assist you in this way.
Knowing how to decipher a Python traceback opens the door to learning about other diagnostic tools and approaches that can help you figure out what's wrong with your code. Tracebacks can be seen and worked with using Python's built-in traceback module. When you want to gain more from the traceback output, the traceback module can be useful. It's also a good idea to brush up on your Python debugging skills. In light of this, we'll take a look at dictionaries in Python in the next tutorial.
awesome, nice written and come with approximately all
vital infos. I'd like to see more posts like this . Reply
since if like to read it after that my links will too. Reply
It's always useful to read content from other writers and use something from
their sites. Reply
this blog on regular basis to take updated from newest information. Reply
from. Thanks for posting when you have the opportunity, Guess I will just bookmark
this web site. Reply