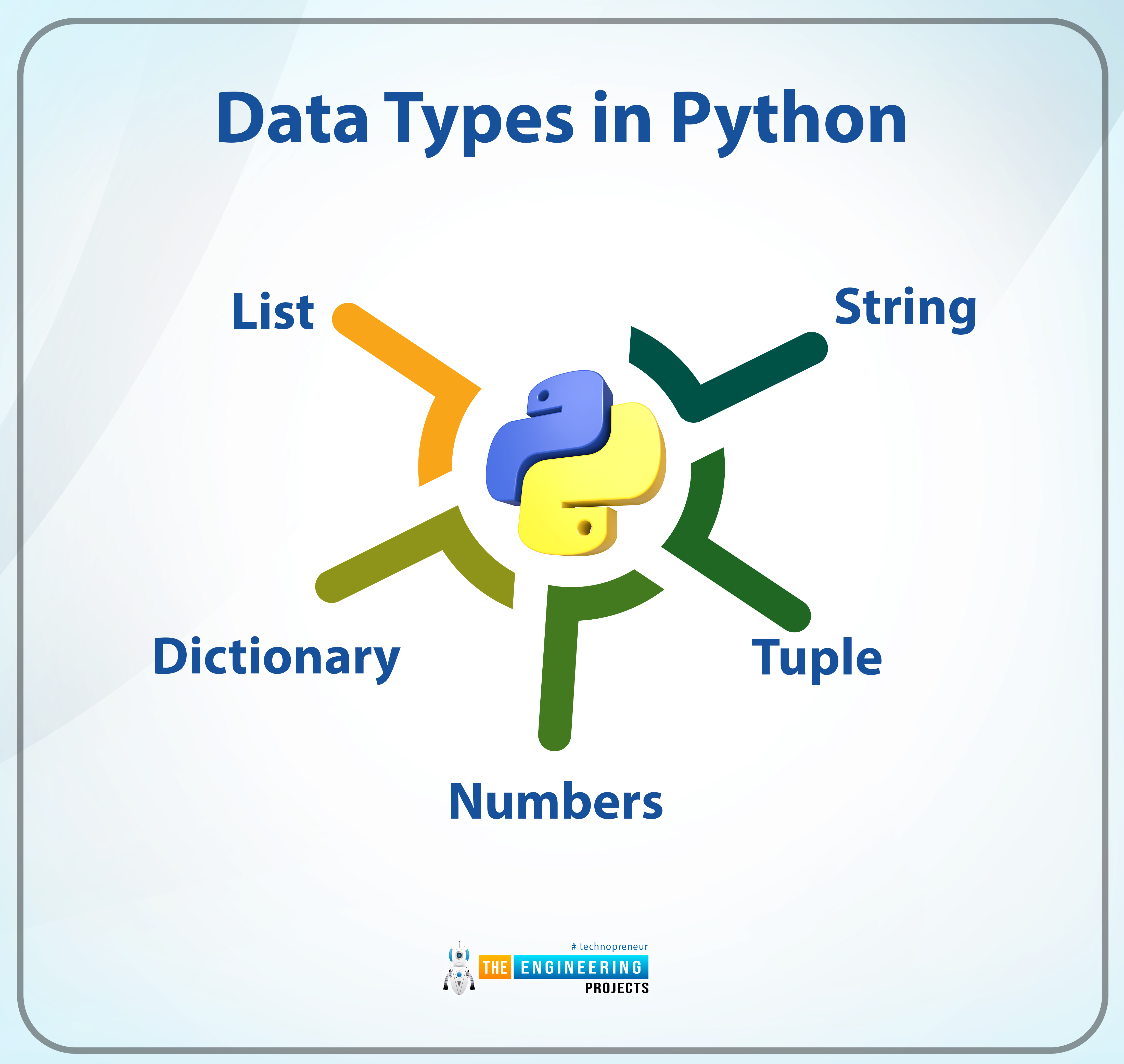
Hello learners! Welcome to the engineering projects where we are working on deep learning. In this series, we are at the part where Python is under our observation. In the last session, we saw the Python built-in functions and the practical implementation of some important pre-defined functions of Python. In the current lecture, you will learn about the fundamental concept of Python. It is not wrong to say that if you want to work in any high-level programming language, you have to understand its data types; otherwise, you will not be able to code complex or long programs using it. More details will be discussed in the next section, but before this, you should have a glance at the concepts you will learn in this tutorial.
What are the data types?
How are Python data types different from other programming languages?
What is the syntax to use the data types in Python?
What is the difference between floats and integers?
How can you practice other concepts along with the complex number in TensorFlow?
What is the casting process in the Python programming language and how can you use it for changing the type of data you enter?
What is the “type” function in Python and how do you use it?
For your convenience and detailed learning, we are just discussing the numeric data types in this lecture, and you will learn more types in the next lecture because I want to discuss each type with the practical implementation of different operations on these data types.
What are Data Types?
Consider the case where we use different types of numbers in mathematics and apply the operations to these different types of numbers. We know that only numbers can be added, subtracted, multiplied, etc. The same is true for data types. When dealing with programming languages, we have to deal with different kinds of objects, and it is important to use them wisely because of the memory storage. Have a look at the basic definition of the data types in the programming languages:
"In programming languages, the data type is the basic concept that declares the type of object being used in a specific way, and based upon the data types, the memory space is occupied by the compiler."
In simple programs, such as the ones we used in our examples, we don't notice much of a difference when we occupy more space in the program, but this is not the best practice because, in long and complex programs, we need to use the exact data type. Hence, the compiler runs perfectly and we can apply the specific operations to the particular data type.
The declaration of the data type is always required in most programming languages so that the compiler can fully understand the data type. So, you can declare the integer as a float and vice versa according to our will, but it causes problems in the compilation.
Data Types in Python:
As we always say that Python is an easy programming language, and here is one of the main reasons why. In Python, the compiler itself is intelligent enough to understand the type of data you are putting into it, so your program may run well if the code is well-written. It feels like a relief that you do not have to remember the names of different data types, and there is no need to memorize the space occupied by the data type so that you may compare and choose the perfect data type for your code. The name of the data types is almost the same as the other programming languages that we have mentioned before, but the way these work with different operations may seem a little bit different. The declaration of different data types in the Python programming language is given in the next section.
Syntax of Data Types in Python
The best way to learn any concept in programming is to learn the syntax first instead of going into the example and understanding how that concept works. When we talk about the syntax of data types in Python, this section is very important. You have to keep in mind that unlike some other high-level programming languages such as C++ and C#, you have only two items:
Variable name
Value of the data type
Now it's time to go over the various data types one by one. The syntax of all of these appears to be the same, but the examples will demonstrate the differences in how each of them works. For your convenience, the different data types are divided into six categories:
Numeric
String
Sequence
Mapping
Boolean
Set
These categories also have sub-categories, and the same category works in the same way, but you have to keep the differences in mind, especially if you are a beginner because it becomes easy to understand the code and the way you work with it. Other details will be clear when you see the implementation in the tensor flow. For this, I want to share the procedure for starting code in TensorFlow in a straightforward way:
Fire up your Anaconda navigator.
In the environment sector, you have to search for “Jupyter Lab” and hit the launch button there.
A new local host will appear in your browser. Go to this local host.
In the new cell, begin coding.
Numeric Data Types in Python
We all know what the numbers are, and there is no need to provide you with the details of the numbers, but to refresh the concept in your mind, we have to tell you that there are three classes of numeric data types that are introduced in Python:
Integer
Float
Complex
A short description of each of them is given next:
Integers in Python
The first and simplest data type that you will see in every tutorial is an integer. These are whole numbers and do not contain any extra parts. These are the signed integers that have a non-limited length. It means that on the positive side of the numbers, you can have the length of an integer as long as you want. One thing that has to be considered here is the memory of the system you are using, but when purely talking about the integer, it does not have any limit in length.
Python Integers in TensorFlow
Are you ready with your TensorFlow to practice all the data types and apply the different operations on them? Ok, we hope your TensorFlow is launched, and have a look at the simple representation of the integer in it.
integer=1257859304284756327
a=3824374269873874
print("integer= ", integer)
print("a = ", a)
add=integer+a
print("integer+a = " ,add)
Here, you can see that:
We can name the variable anything we want, whether it is a special name or any combination of the alphabet, but it must follow the rules that we specified in the previous lecture. The results of the code are given next:
The length of the integer does not matter, as integers can be long or short according to the requirement.
The addition can take place with the integers. Similarly, other operations such as subtraction, and multiplication is also possible.
Float in Python
The next is the float, which in some cases looks like an integer, but if you are a science student, you must have the idea that integers and floats are not the same. The floats contain a decimal floating number just after the integer part. So, even if the number after the decimal point is zero, it will still be called the "float." Another thing to be noticed in the floats is, you can use the 15 digits in the float, and these are not unlimited as the integers are.
Python Float in TensorFlow
In TensorFlow, I want to perform the subtraction on the float this time, and I want to show you by code that when we do not add any decimal part to the float, the compiler itself adds the zero in the decimal part and provides us with the result. Moreover, you will observe that it takes the most significant number after the decimal part; therefore, in the code, when we add the zero as the last digit, it ignores it and the results are shown with the significant numbers.
Complex Numbers in the Python
We've all learned about complex numbers in math class, and according to the requirements, these complex numbers play an important role in programming. The representation of the complex number in Python is a little bit different than in mathematics class. Have a look at the code, and after that, we will discuss it in detail.
Complex Numbers Using TensorFlow
The representation of the complex number is the same as what you were expecting. We have to use the i and j for the complex part, and the sign of addition or subtraction represents the difference between the real and complex parts.
# Step 1
z = complex(a,b);
print(z)
# Step
print ("The real part of complex number is : ",c.real)
#Step 3
print ("The imaginary part of the complex number is : ", c.imag)
Here is the first step, we have assigned the duty to the compiler to make a complex number for us. For this, we have used the “complex” built-in function. We have discussed it in lecture number 10 of this series.
The step is completed when we print the complex number to show you how the functions are working here.
We are naming the complex number “c” and keep in mind, it consists of the real and imaginary parts.
In the next step, we are simply using the “real” and “img” keywords to separate these parts of the complex number.
The good thing is, we are using the same line to print the description and the result of the code. You can also do so by using the additional line of print, but I like simple and shortcodes.
So, the overall result can be observed in the following image:
The result is shown in the form of floating numbers, and in our case, the real part is 0 by default.
Casting in Python
Till now, we have seen simple declarations in the Python programming language, but now it's time to discuss another method of variable declaration, which is casting. Because of the various forms in which metals are cast, we've heard of this term before. The concept of casting is related to the same idea. In Python, this process is defined as:
"Casting in Python is a process in which the original type of the variable is changed to any other type by specifying it in the code in a particular way."
We have mentioned before that the Python compiler automatically detects the type of content, and therefore, in some special cases, we want to occupy the space from memory that does not match the value you are entering. If still it is confusing for you, do not worry because it will be clear in just a bit when you will look at a simple example to do so in TensorFlow.
a=23.56
b=type(a)
print("Here 'a' is a float", b)
c=int(23)
d=type(a)
print("After using the casting, the result is" , d)
Here is the output of this code, which tells you the details by itself.
The type function was covered in the previous lecture, so I hope you understand what it does. If not, you can review the previous lectures. Since the data types are so long that it becomes difficult to explain in a single lecture, you will get the details of other data types in the next lecture. Until then, you must continue to practice with these data types. We have seen the introduction of the data types and the ways to work with the data types in Python. We've also seen a comparison of other languages and Python on the same topic, and this lecture is heavy on numeric data types. I hope it was fruitful for you and that you will go to the next lecture for more data types.