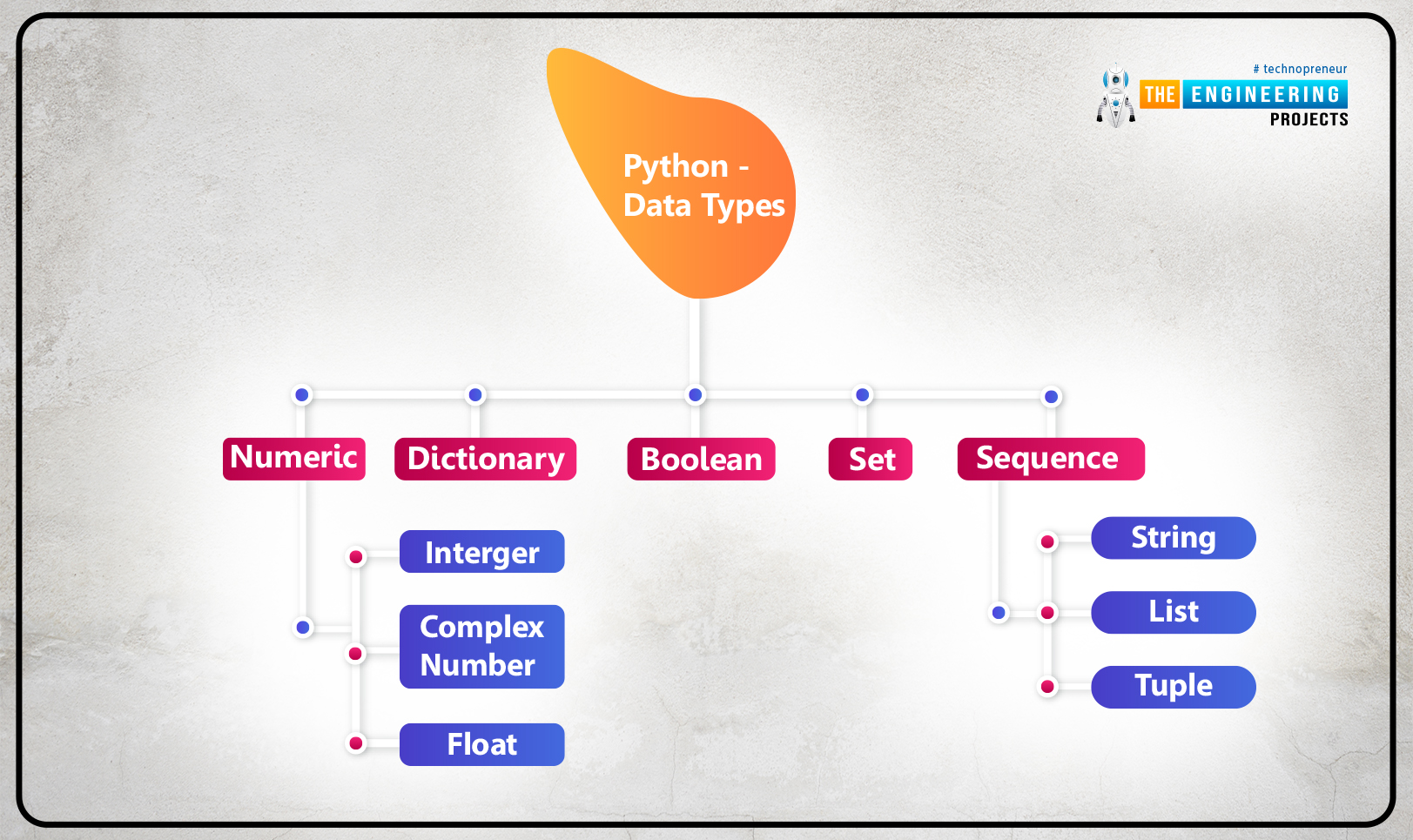
Welcome to the next tutorial of our python course. We learned about python numbers in the last tutorial, and in this tutorial, Data types in Python include, dictionaries, sets, and Boolean, among others. We'll give a quick overview of the above data types in this section but later in this course, we'll go over each of them in-depth.
Introduction
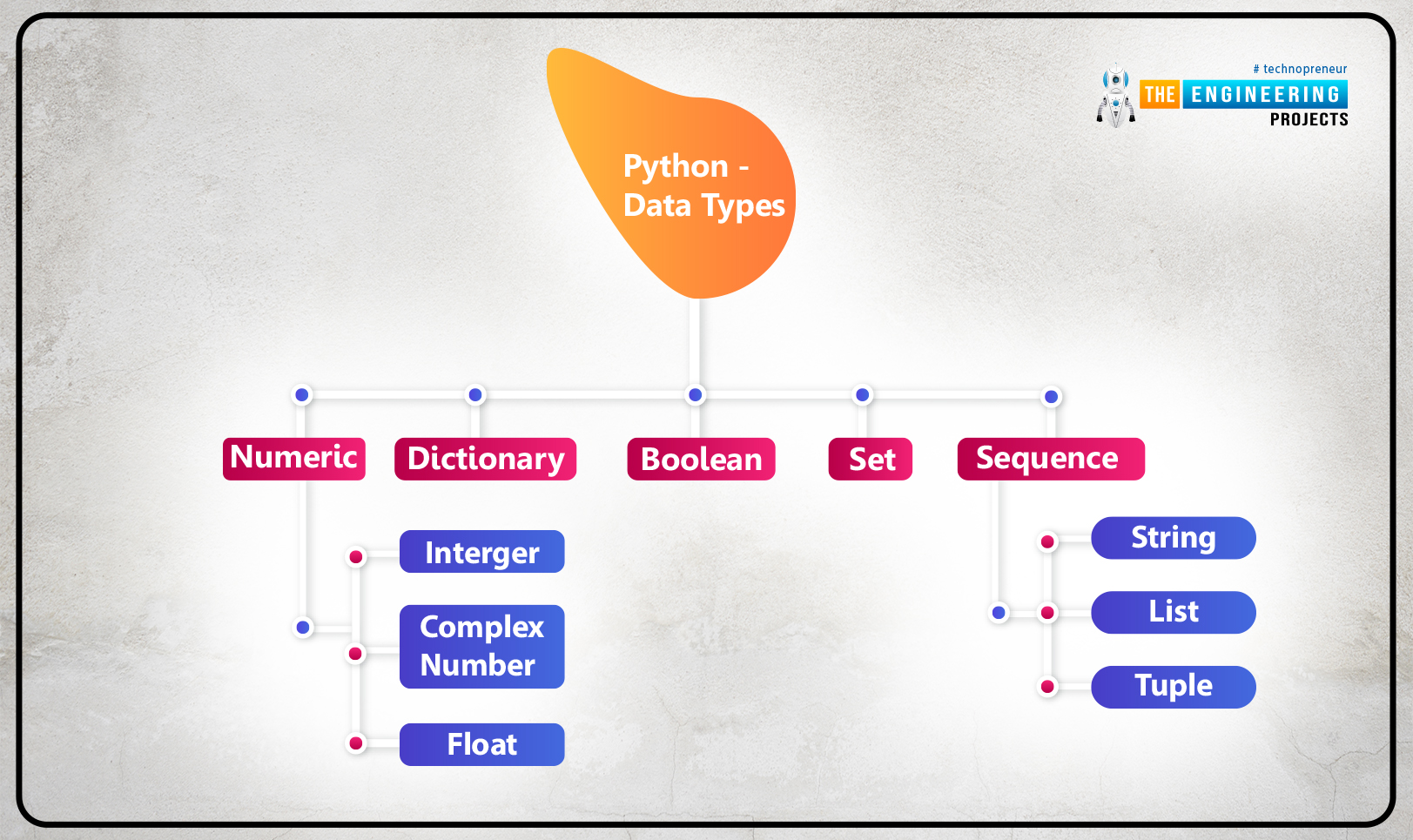
In the Python programming language, data types are a necessary concept. Python assigns a data type to each value. Data Types are used to classify data objects or to assign a value to a data category. It aids in comprehending the many operations that can be applied to a value.
Python considers everything to be an object. Classes are represented by data types in Python. Variables are the names given to the objects or instances of these classes. Let's look at the various data types that Python has to offer.
Our variables can be used to store values that are of a specific data type. The type of a variable does not need to be specified when declaring a variable in Python because it is dynamically typed. The interpreter's default behavior is to tie a value to its type.
a = 5
We didn't define the type of the variable a, which has the integer value of five. Variable a will be automatically interpreted as an integer by Python.
Python can be used to determine variable`s type in a program. It is possible to retrieve the type of a variable in Python by using the type() method. Consider the following scenario for defining values for various data kinds and determining their type.
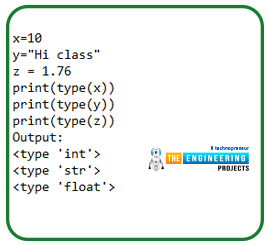
Standard data types
Different kinds of values can be stored in a variable. A name of a person, for example, must be stored as a string, while his or her identity number should be stored as an integer. Python comes with a number of standard data types, each of which has its own storage method. The following is a list of the data types defined in Python.
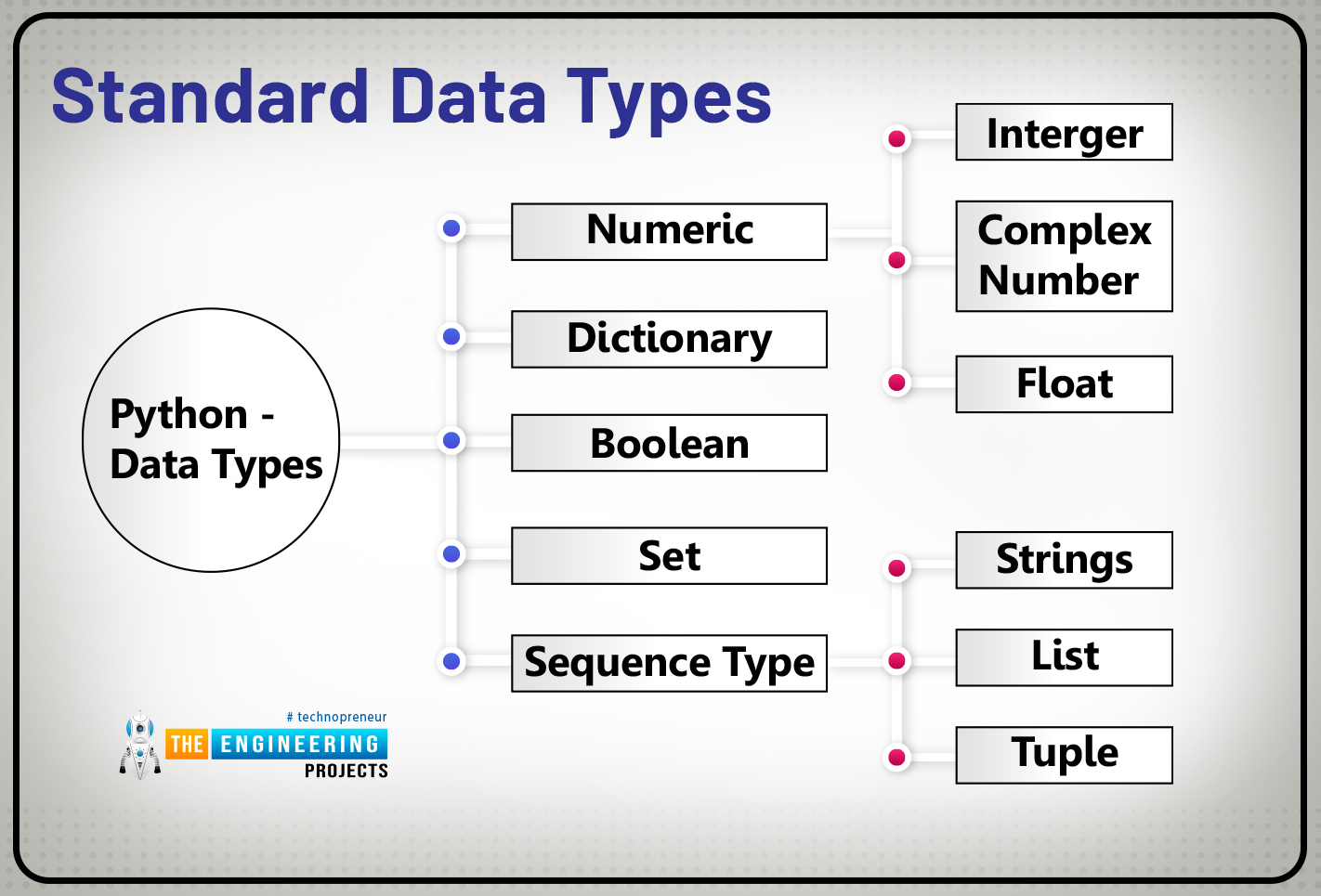
Numbers
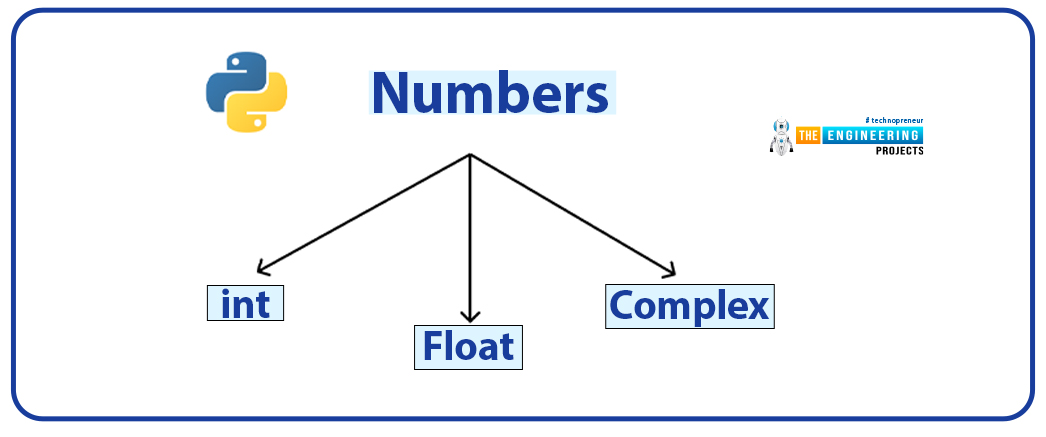
The term "number" refers to a sort of data that contains numerical values. Integer, float, and complex values are all available in the Python Numbers data type. The type() method in Python can be used to determine variable’s data type. isinstance() determines whether an object belongs to a specific class. When a variable is assigned a number, Python produces Number objects.
v = 5
print("type ", type(v))
z = 40.5
print("type", type(z))
t = 1+3j
print("type", type(t))
print(" Is this true or not?:", isinstance(1+3j,complex))
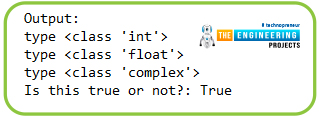
Python can handle three different forms of numeric data.
- Int - Any integer value, such as 10, 2, 29, -20, -150, can be used. The length of an integer is unrestricted in Python. It is int's value.
- Float - Float is a type of variable that stores float numbers such as 1.9, 9.902, 15.2, and so on. It has a precision of up to fifteen decimal places.
- complex – The real and imaginary components of these numbers are represented by m and v in an ordered pair, m + iv. It's 1.9 j, 2.0 j, and so forth.
Sequence Type
List
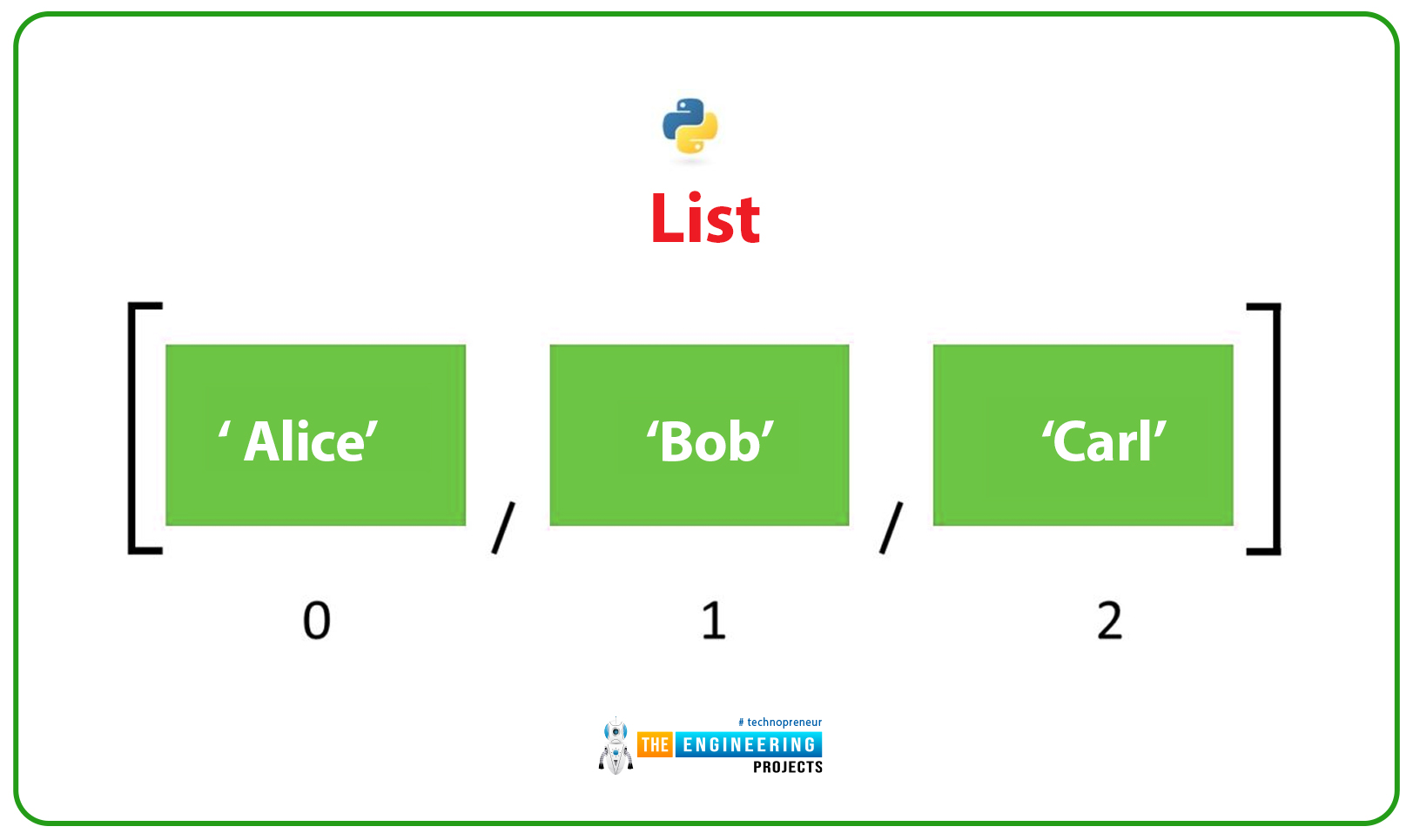
The list might include a variety of data. A comma (,) is used to divide the elements in the list, which are then enclosed in square brackets []. To access the data of the list, we can use slice [:] operators. The concatenation (+) and repetition (*) operators behave similarly in lists and strings. Consider this scenario.
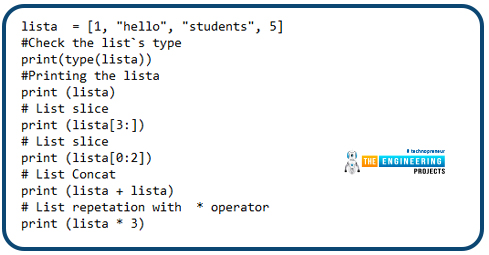
Output:
[1, 'hello', 'students', 5]
[5]
[1, 'hello']
[1, 'hello', 'students', 5, 1, 'hello', 'students', 5]
[1, 'hello', 'students', 5, 1, 'hello', 'students', 5, 1, 'hello', 'students', 5]
How do we access elements in a list?
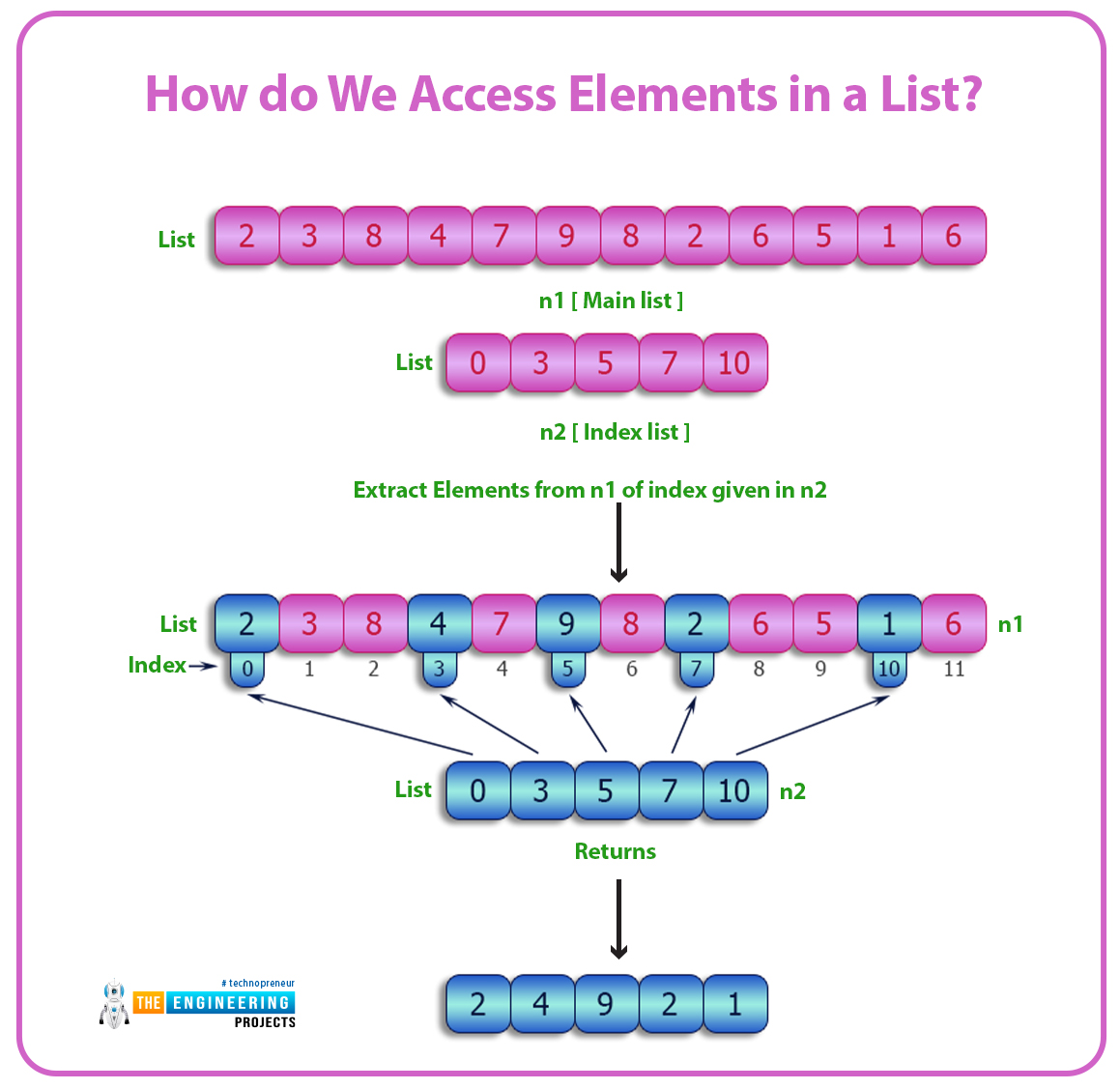
The components of a list can be accessed in a variety of ways.
List Index
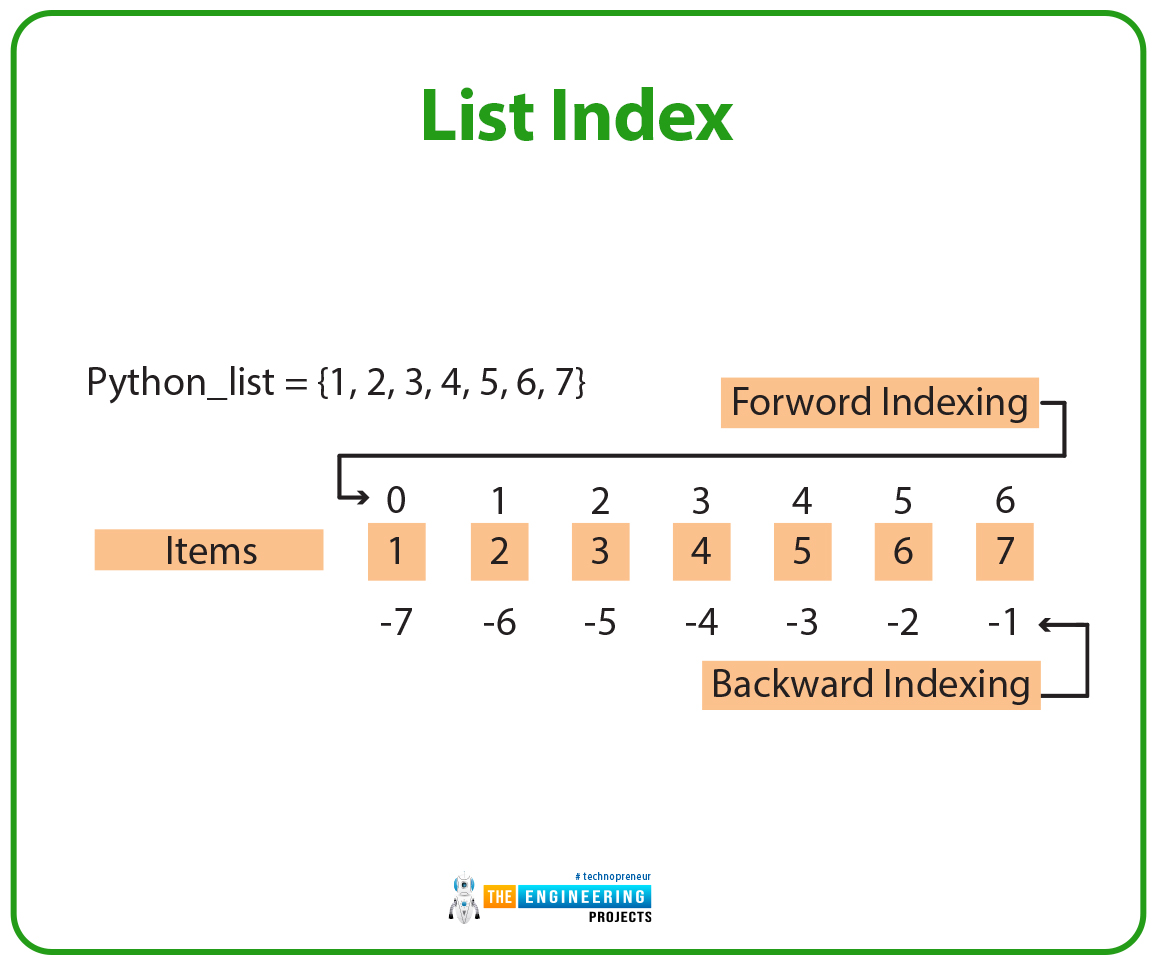
To get to a specific item in a list, we can use the index operator []. In Python, indices begin at 0 and go up from there. As a result, an index of 0 to 4 will be assigned to a list of five members. If you try to access indexes that aren't listed here, you will recieve an IndexError. An integer must be used as the index. We can't use floats or other kinds because TypeError will occur. Nested indexing is used to access nested listings.
Negative indexing
Python sequences can be indexed using negative numbers. For example, the index -1 represents the last item, and the number -2 represents the second-last item.
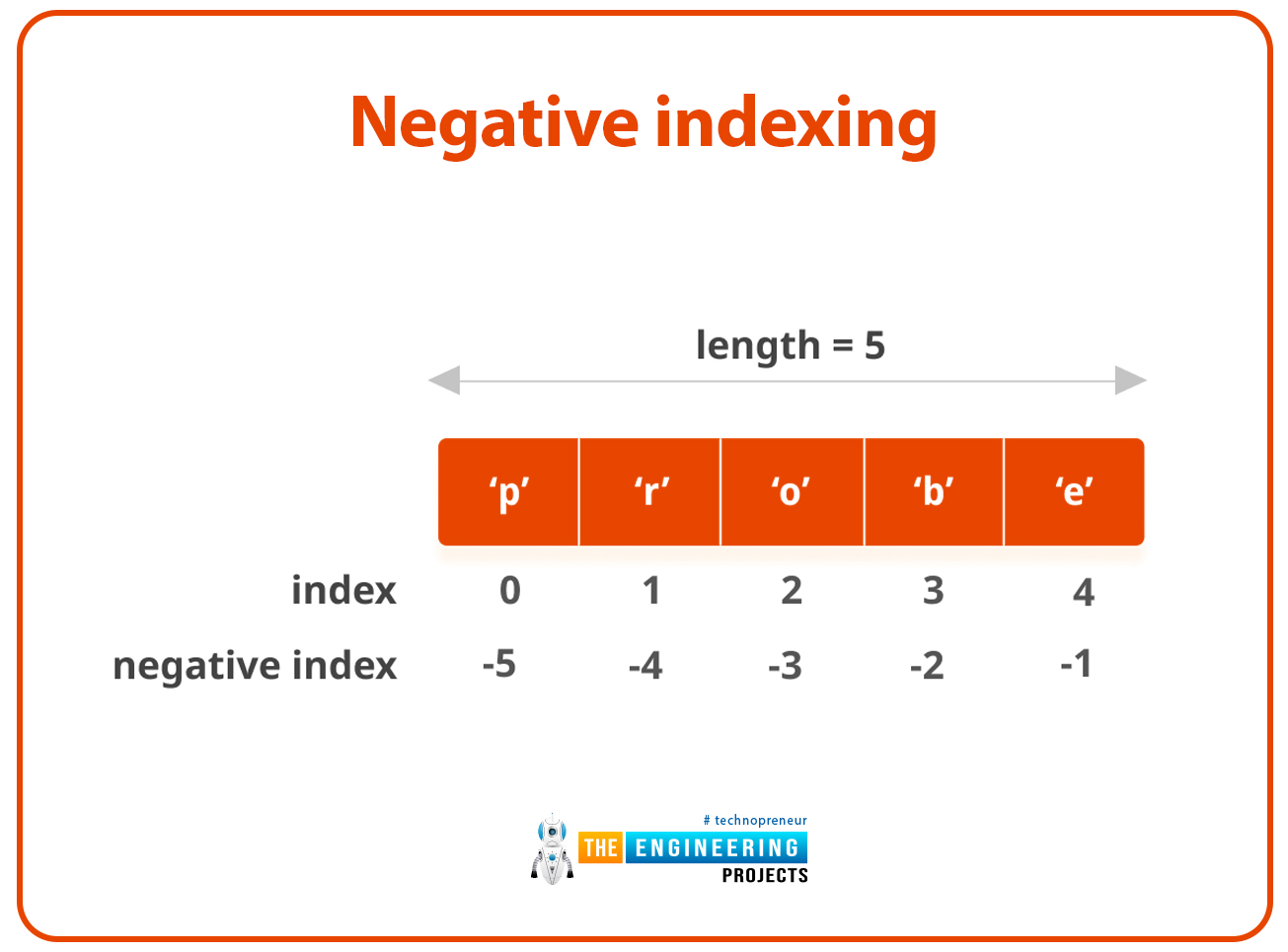
Adding item to a list
lists are mutable, meaning their elements can be changed. To update a single item or a set of objects, use the assignment operator (=).
Deleting items from a list
The in-built del function can be used to remove one or more entries from a list. It has the ability to completely remove the list.
Tuple
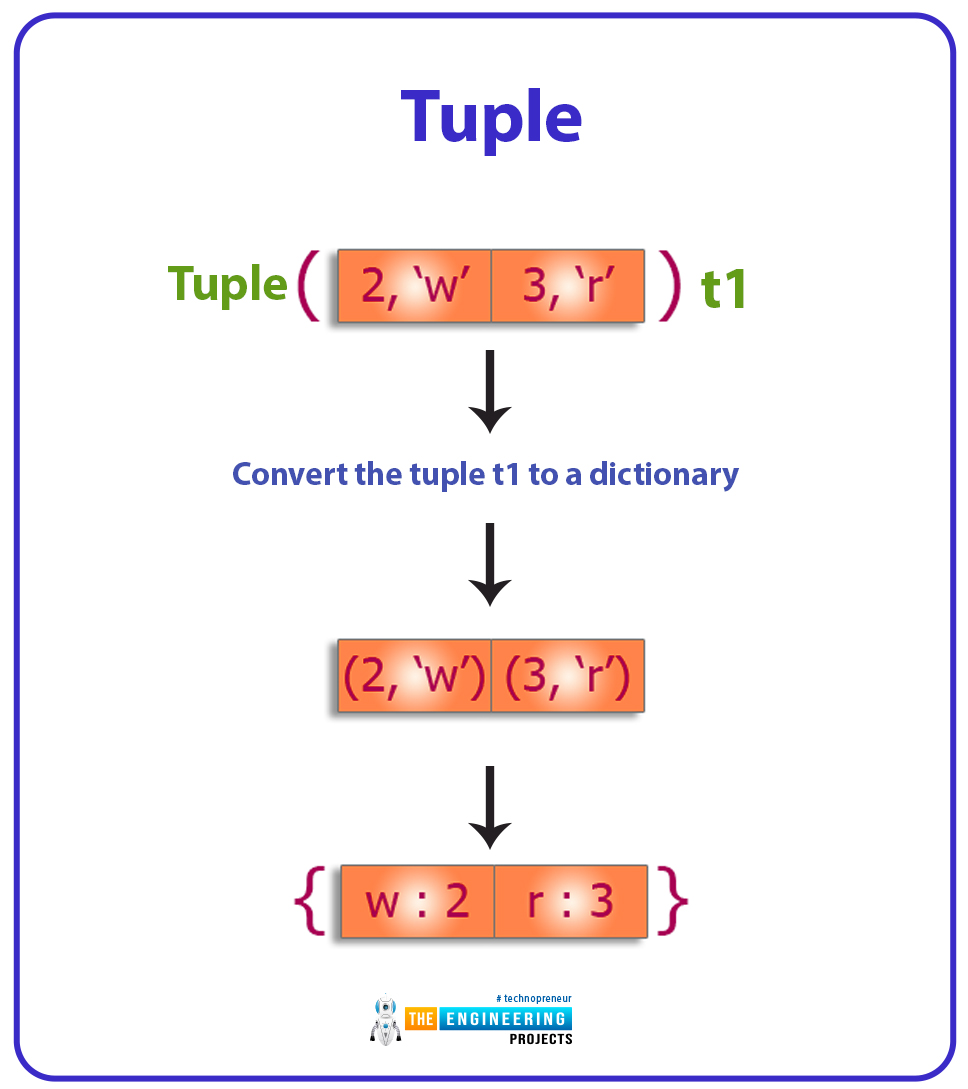
In many ways, a tuple is comparable to a list. Tuples are built up of items of several data kinds, similar to lists. The tuple components enclosed in parentheses are separated by a comma (,).
Read-only data structures, such as tuples, do not allow changes to its elements' size or value.
Let's look at a simple tuple example.
tuup = ("hello"students", 5)
# Check tuup type
print (type(tuup ))
#Print tuup
print (tuup )
# Tuup slice
print (tuup[1:])
print (tuup[0:1])
# Tuple concat
print (tuup + tuup)
# Tuup repetition by use of *
print (tuup * 4)
# Addition of a value to the tuup. It throws an error.
t[5] = "hi"
Output:
<class 'tuple'>
('hello', 'students', 5)
('students', 5)
('hello',)
('hello', 'students', 5, 'hello', 'students', 5)
('hello', 'students', 5, 'hello', 'students', 5, 'hello', 'students', 5, 'hello', 'students', 5)
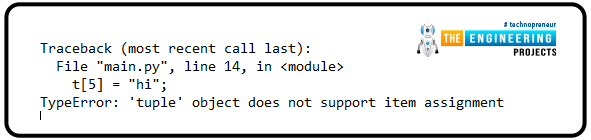
How do we access tuple items?
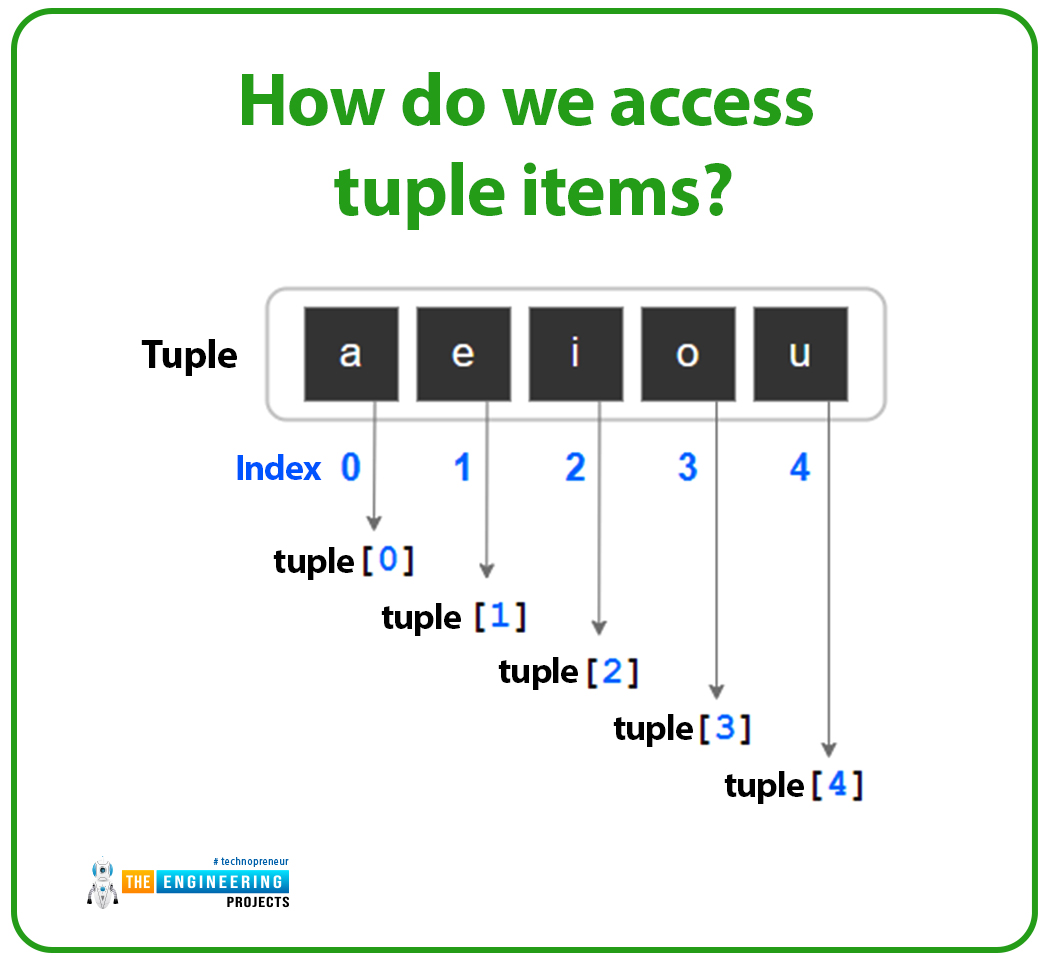
1. Use of index
The index operator [] can be used in a tuple to access items, with the index starting at 0.
As a result, a tuple with six elements will have indices ranging from 0 to 5. If you try to access an index that isn't in the tuple index range, you'll get an IndexError (6,7,... in this example).
We can't use floating or other forms of data because the index must be an integer. Because of this, a TypeError is generated.
Using the nested index feature, tuples that have been nested can be found.
2. Negative Indexing
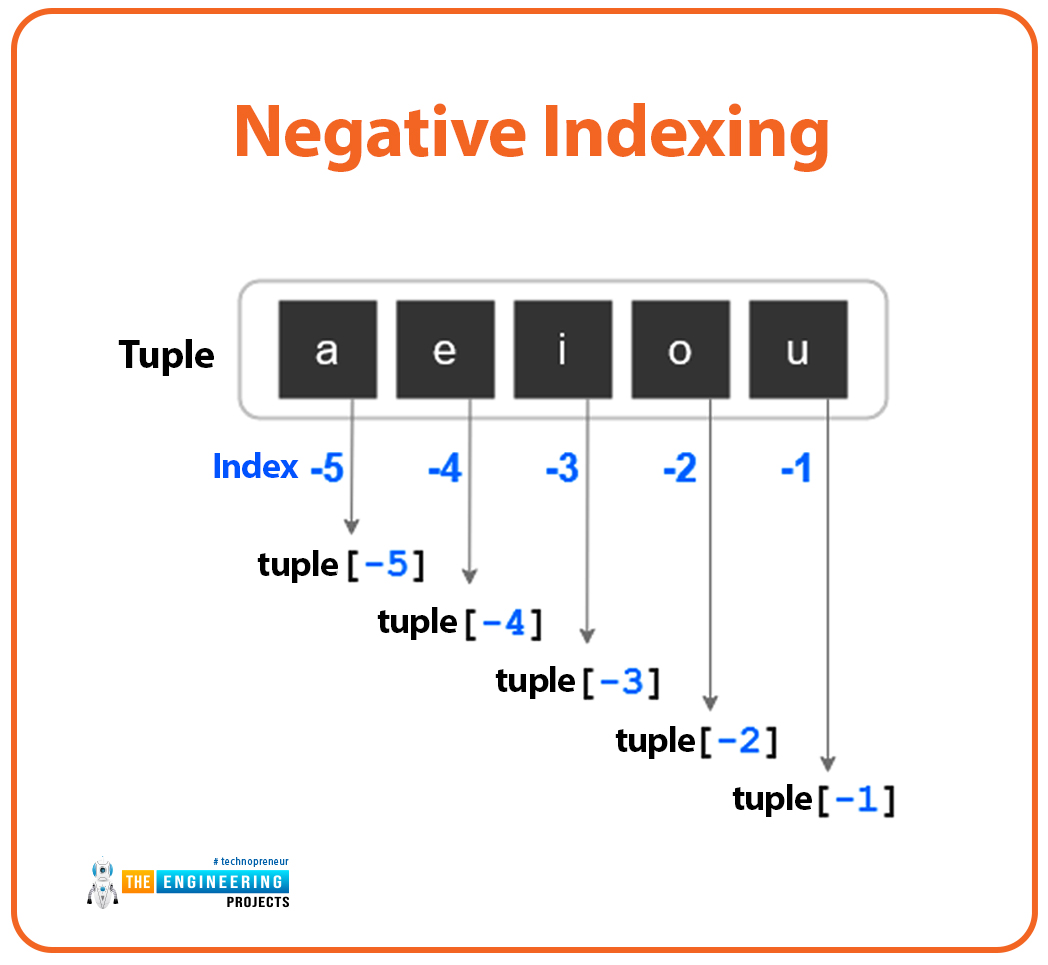
How to change a Tuple's Value
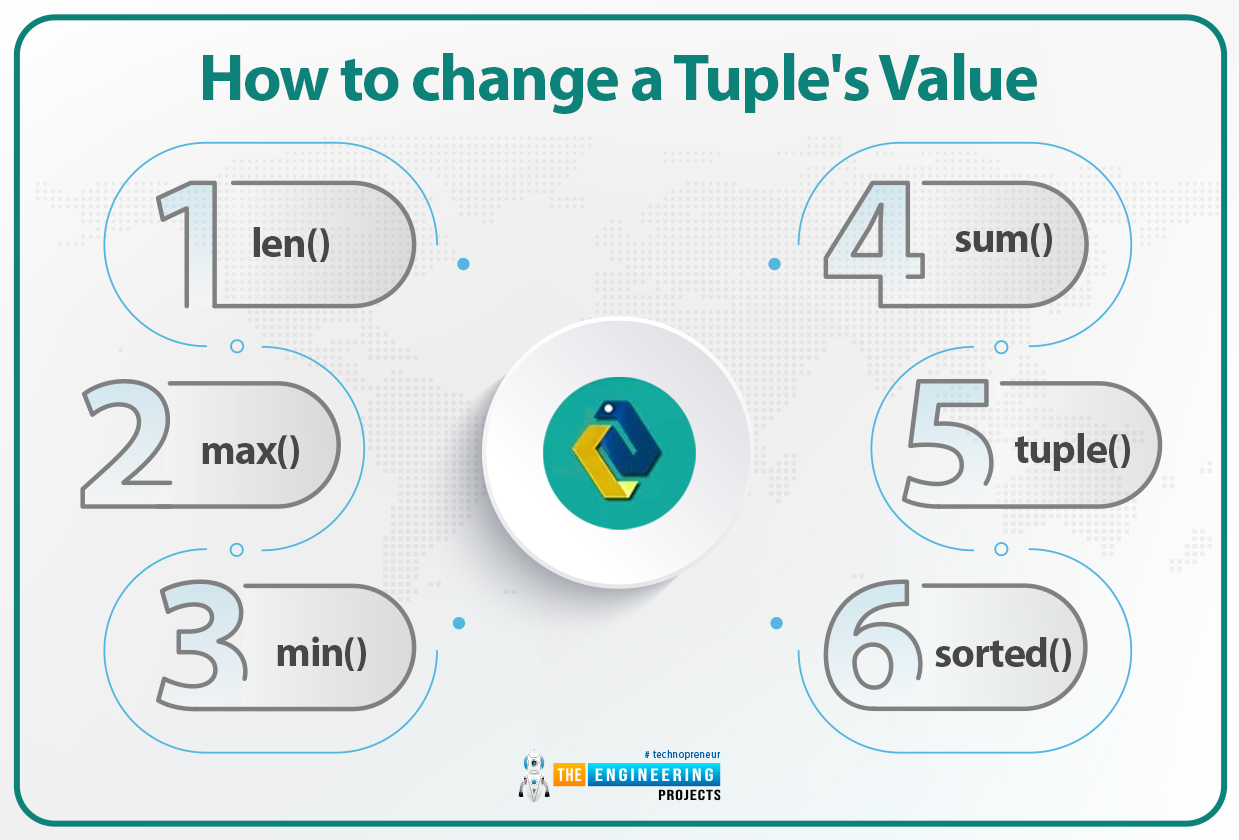
Tuples are immutable, unlike lists.
This implies that once a tuple's elements have been assigned, they cannot be changed. It is possible to alter the nested items of an element that is itself a changeable data type, such as a list.
A tuple can also have different values assigned to it (reassignment).
Deleting a Tuple
A tuple's elements cannot be changed, as previously stated. We can't delete or remove entries from a tuple because of this.
The keyword del, on the other hand, can be used to completely delete tuples.
Dictionary
A dictionary is a collection of objects that have a key and a value. It's similar to a hash table or an associative array in that each key stores a single value. A primitive data type can be stored in key, but a Python object can be stored in value.
The curly brackets contain the elements in the dictionary, which are separated by a comma (,).
Consider this scenario.
m = {1:'Jim', 2:'malec', 3:'joy', 4:'mark'}
print (m)
print ("name 1 "+d [2])
print ("name 2 "+ d [3])
print (m.keys())
print (m.values())
Output:
name 1 malec
name 2 joy
{1: 'Jim', 2: 'malec', 3: 'joy', 4: 'mark'}
Accessing elements in a dictionary
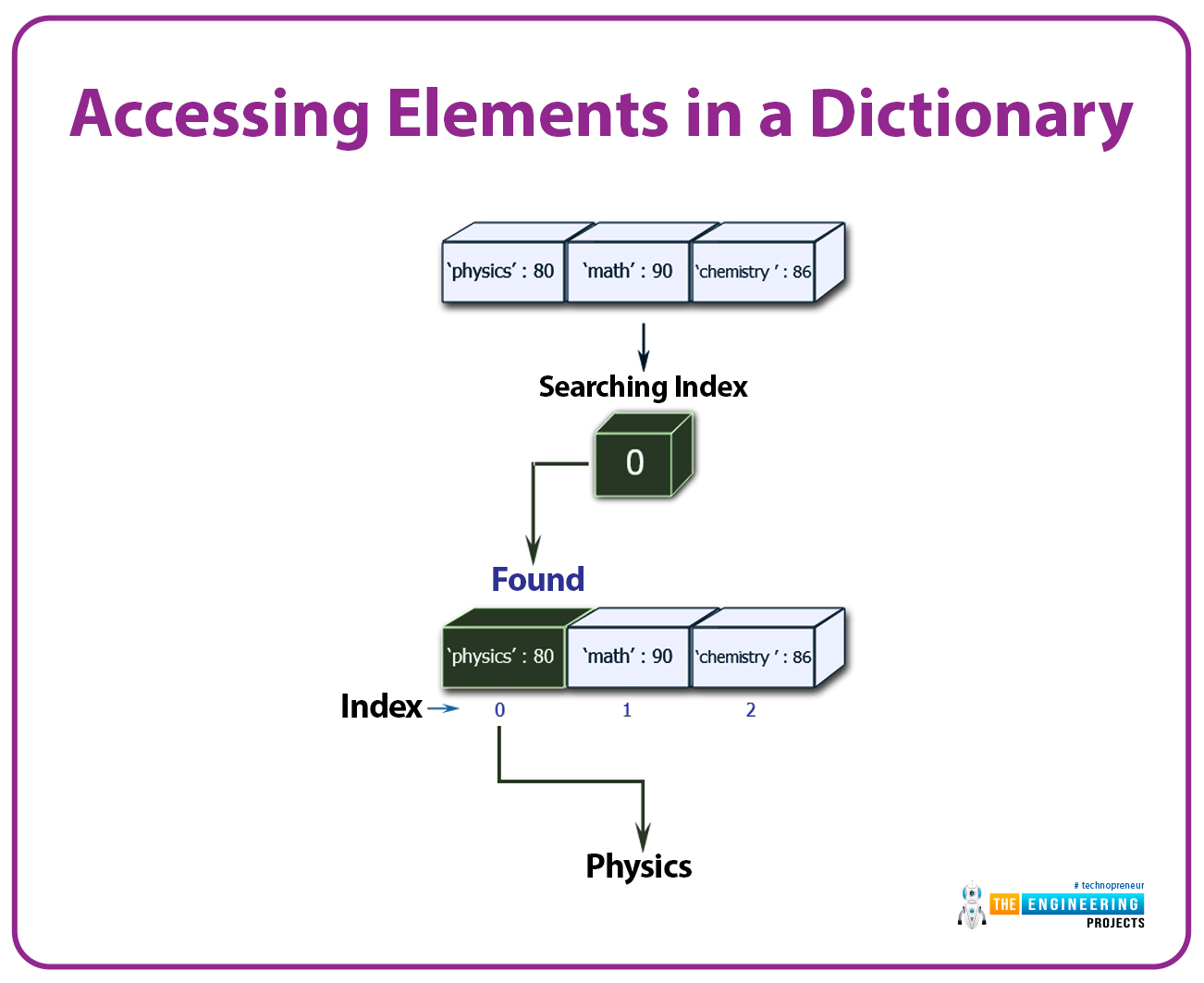
A dictionary employs keys instead of indexes to access values. Both square brackets [] and the get() function can be used with keys, if the dictionary does not have a key, KeyError is raised. In contrast, if the key cannot be retrieved, the get() method returns None.
Dictionary Elements: Changing and Adding
Dictionaries are subject to change. Using the assignment operator, new objects can be created, or existing ones' values can be altered. If the key already exists, the existing value will be changed. If the key is missing, the dictionary is updated with a new (key: value) pair. As an example,
# manipulation and addition of Dict items
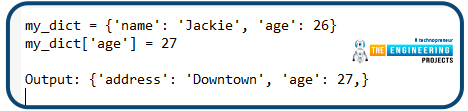
Removing elements from a dictionary
Using the pop() method, we can eliminate a specific item in a dictionary. After deleting items with any of the supplied keys, this function will return that item.
The popitem() method can be used to delete and return a key or value item pair from dictionaries.
Using the clear() method, all the items can be eliminated at once.
Deleting individual entries or the entire dictionary is likewise possible with the del keyword. Consider the following:
meters = {11: 5, 12: 8, 13: 7, 14: 17, 15: 75}
print(meters.pop(11))
Output:
{12: 8, 13: 7, 14: 17, 15: 75}
Boolean
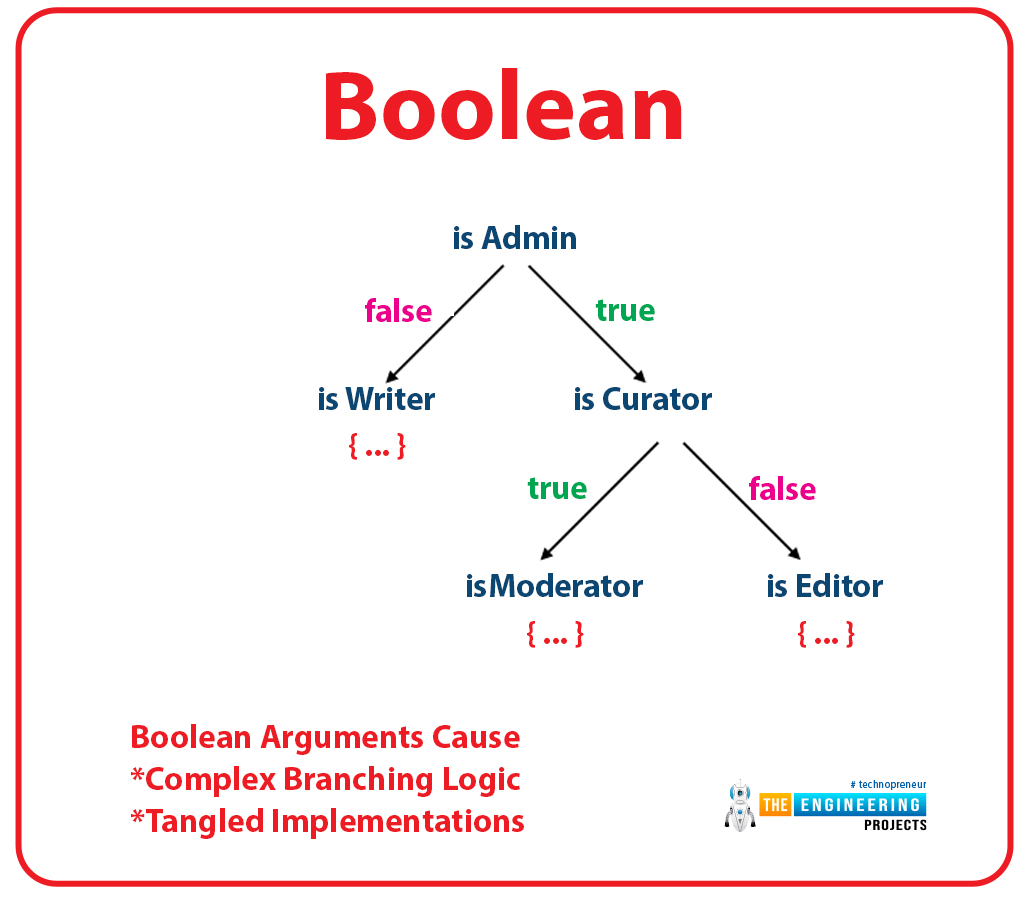
For the Boolean type, True and False are the default values. These figures are used to tell if the assertion stated is accurate. It's wrapped up in the bool class. True is any non-zero number or the character 'T', while false is any non-zero value or the character 'F'. Consider the following.
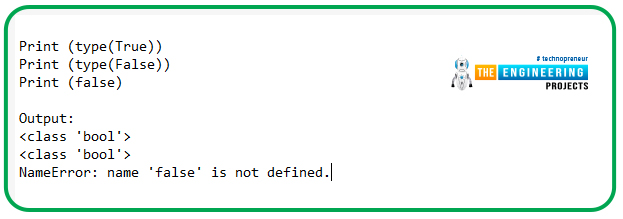
Python Booleans as Keywords
Keywords are not built-in names. They're regular variables as far as the Python language is concerned. If you assign to them, the built-in value will be overridden.
True and False, on the other hand, are not built-ins. Keywords are what they are. True and False, unlike many other Python keywords, are Python expressions. They can be used everywhere other expressions, such as 1 + 1, can be used because they're expressions.
It is possible to assign a Boolean value to variables, but not to True or False.
Because False/True is a Python keyword, you can't assign to it. True and False behave similarly to other numeric constants in this way. You can pass 1.5 to functions or assign it to variables, for example. It is, however, hard to put a value on 1.5. The Python expression 1.5 = 5 is incorrect. When processed, both 1.5 = 5 and False = 5 are invalid Python code and will result in a SyntaxError.
Python Booleans as Numbers
Python considers Booleans to be a numerical type. For all intents and purposes, that means they're numbers. To put it another way, Booleans can be used to perform mathematical operations and compared to numbers.
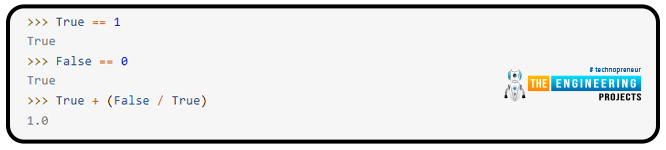
Boolean Operators
Operators With No Inputs
True and False can be thought of as Boolean operators that don't require any inputs. The result of one of these operators is always True, while the other is always False.
Sometimes it's helpful to think of Python's Boolean values as operators. This method, for example, can help you remember that they aren't variables. It is impossible to assign to True or False for the same reason you can't assign to +.
In Python, there are only two possible Boolean values. When there are no inputs to a Boolean operator, the result is always the same. As a result, the only two Boolean operators that do not take inputs are True and False.
Set
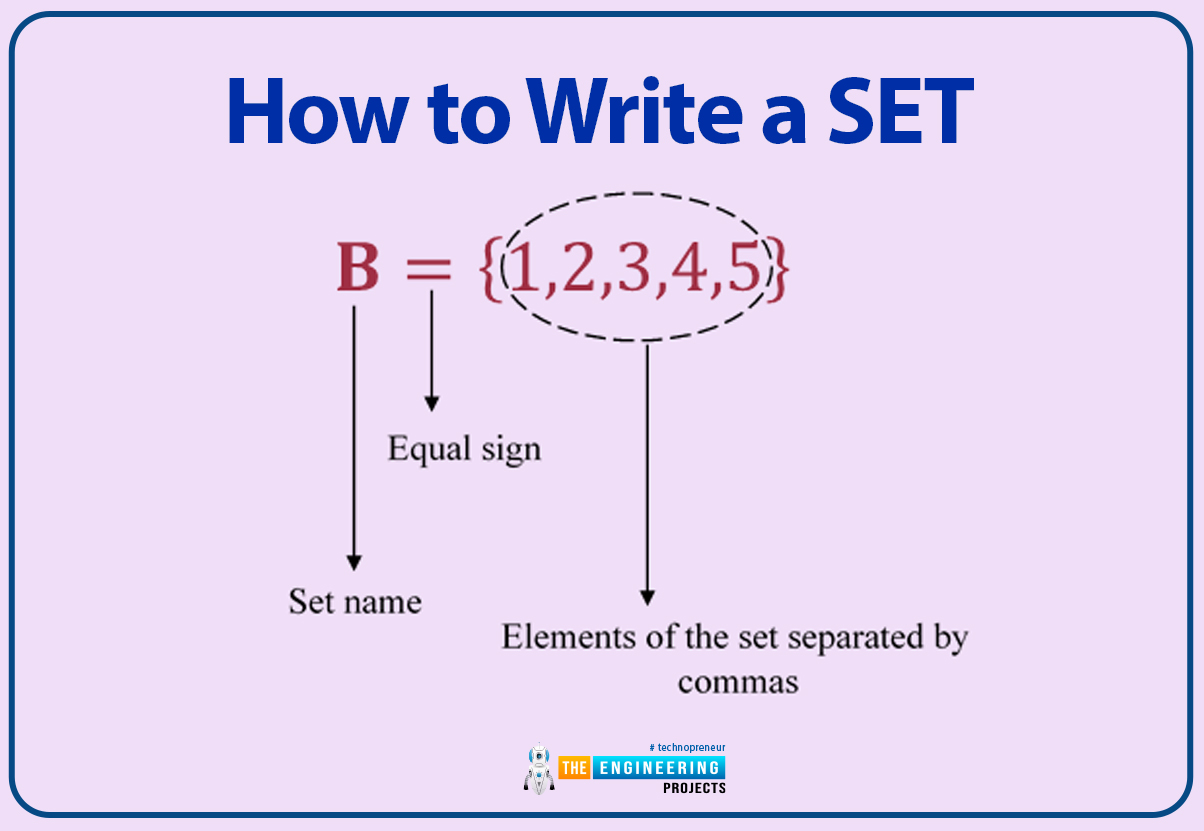
Python Set refers to the data type's unordered collection. It's iterable, changeable (meaning you may change it after you've created it), and contains unique items. To construct the set, elements` sequence is given between curly brackets and separated by a comma, or the built-in method set() is used. It can have a variety of different values in it. Consider this scenario.
seta = set()
setb = {'Jane', 2, 3,'class'}
print (setb)
setb.add(10)
print (setb)
setb.remove(2)
print(setb)
Output:
{3, 'class', 'jane', 2}
{'class', 'Jane', 3, 2, 10}
{'class', 'jane', 3, 10}
Conclusion
Congratulations on completing this introduction to data type tutorial. In this tutorial, we covered the in-built data types provided by Python.
So far, all of the examples have just altered and presented constant data. In almost all projects, you'll want to build objects which vary in value as the program runs. In the next topic, we will look at sets in depth.