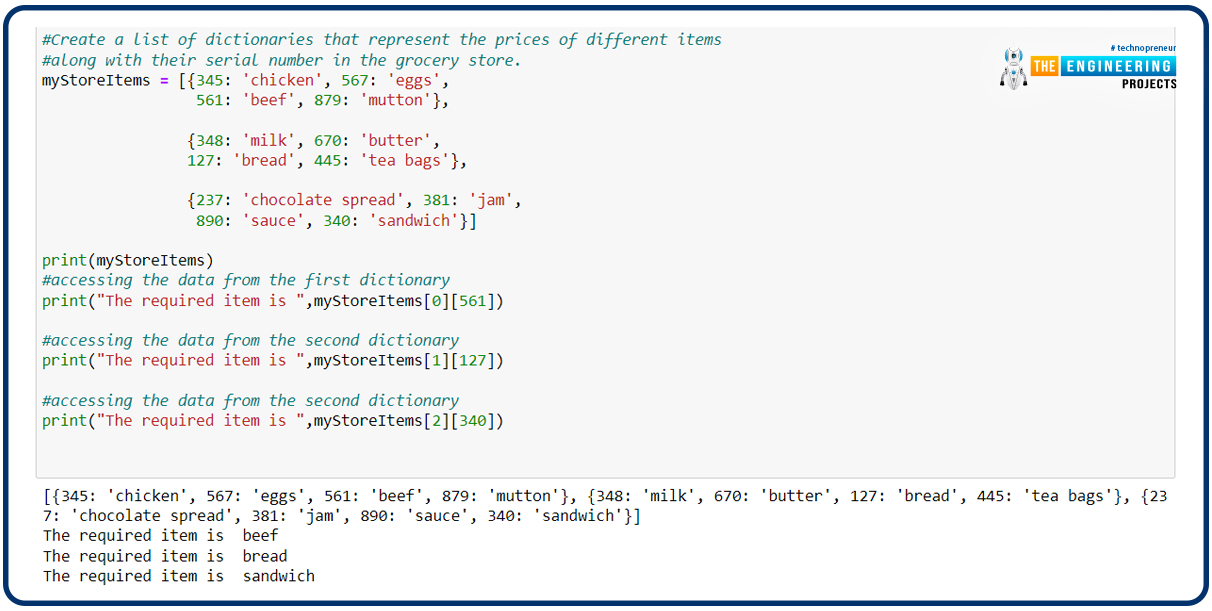
Hello students! Welcome to the new Python tutorial, where we are learning a lot about this programming language by applying the codes in a Jupyter notebook. It is interesting to see how simple codes with easy syntax can be useful to store, retrieve, and use data in different ways. Many things in Python are so unadorned and pre-defined that programmers may use them in a productive way without any issue.
In the previous lecture, we have seen the details of the sets and how different types of mathematical operations are used to get the required results. We have seen many codes, the examples of which were taken from day-to-day life. In the present lecture, the mission is to learn a new topic that is related to the concepts of previous lectures. Yet, it is important to have a glance at the points that will be discussed in detail here:
Introduction to the dictionaries in the python programming language.
Why do we need dictionaries if we have different ways to store the data?
How can we use dictionaries in Jupyter notebook?
How do you define the concept of keys in dictionaries?
What are some characteristics that make the dictionaries different from others?
How do you justify that using the list with dictionaries makes the working better?
These points are the important interview questions and all of these will be crystal clear in your mind when we will go deep into these concepts one after the other. So stay in this lecture till the end and we will discuss the basic concept today.
What is Dictionary in Python?
To understand the topic, the first question that arises in the mind is what actually constitutes a dictionary and why it is important to understand this concept. An interesting piece of information to share here is that dictionaries are not new concepts; these were defined in the older versions of Python as well, but the difference is that in the older versions, from Python 3.6 onward, dictionaries were considered unordered, and if we talk about the current introduction, we will get the following lines:
"In Python 3.6 and later, a Python dictionary is an ordered collection of data that stores data in the form of pairs in keys or values."
The word “ordered” is not new to us and from the above information, we can understand that in the older versions of Python dictionaries when displayed in the output, does not have the proper indexing and the order of the element was unpredictable but when talking about the updated version (that we are using during this tutorial) the order of the elements in the dictionaries is well defined and the elements will be arranged in the same manner always that is defined by the programmer. Here it is important to notice that usually, the students are using the updated version of Python and there is no need to mention the older versions but the reason to mention this is, the older versions are preferred by the users that have older personal computers or because of any other reason, if they are using the previous versions, then they will find the dictionaries a little bit different from this tutorial.
Why We Need Dictionaries?
We have been working with the data types in Python that all are used to have the collection of data and the question here is why we need another entity that can store the data if we have lists, sets, and others. The answer here is simply because the way to store the data is different for all of these and as the topic of discussion today is dictionaries, you must know that it uses the key values to store the data and therefore, it becomes uncomplicated to store the data using the key value mapping format. The most common advantage is, the retrieval of data from the dictionary becomes super easy with the help of these key values. If we talk about the complexity of the dictionaries, we will get the following data:
The best case of a dictionary has O(1) complexity.
The worst case of a dictionary has the O(n) complexity.
Using the Dictionaries in Jupyter Notebook
Now, that you understand the basics of dictionaries, to make the discussion clear, examples are being used in the Jupyter notebook. We have been using it in our tutorial, and therefore, we know that we simply have to start the software and get the new tab in the browser by clicking on the “new” dialogue box and clicking on “Python 3."
Once you get the worksheet now it's time to get used to all the codes that will clarify the concepts in a better way. So let’s start learning the concept and then simply use all the learning in our codes. This will be easy to understand because we have been working with such types of codes in this whole course but the difference here is the key of the dictionary.
Concept of Keys in Dictionary
You must know that dictionaries are declared by using the curly brackets in Python and if you have seen our previous tutorial, you must have the idea that the same representation was used in the sets then how can the compiler differentiate between these two and what is the reason to introduce the concept of dictionary in Python? Well, as we move towards the complex problems, we get the idea that simply storing the data is not enough, there is a need of providing the reference of the data and this happens when we use the keys in dictionaries.
“A key in Python dictionary is the specific and unique value assigned to each element and that has the immutable data type.”
The role of keys in the dictionaries will be clear by using the output of the code given next:
#declaring the dictionaries containing the grocery items
GroceryItems={"lettuce":4, "onion":34,"pepper":8,"plum":12, "frozen fruits":22}
print(GroceryItems)
Hence, the keys add more details to the dictionaries, and the data is stored in a better manner while being used in dictionaries. The usage of the keys is still ambiguous in the mind, hence, we are using different examples to express it well. There are different ways to use the dictionaries, and these procedures are given next:
#declaring the dictionaries containing the grocery items
GroceryItems={"lettuce":4, "onion":34,"pepper":8,"plum":12, "frozen fruits":22}
print(GroceryItems)
#retriving the data using the concept of keys in a dictionary
print("The required data = ", GroceryItems["pepper"])
Hence, the data stored in the key can be used easily to retrieve the data. In the example given above, when the person wants to get the number of groceries they want with the help of items.
Characteristics of Dictionaries
We have focused on the examples, but before going into the details of them, you must know some important points about the dictionaries.
The dictionaries are declared with the help of curly brackets.
The keys are used with the elements and to declare them, the colon is used along with each element.
The value of the keys can be obtained by mentioning the element.
The dictionaries are ordered and every time when the same dictionary is declared, we get the element as same as that was mentioned in the code.
The usage of any data type is allowed in the dictionaries, and therefore, the working of a large amount of data is possible in the dictionaries.
Same as the sets, duplicate elements in the dictionaries are not possible. It means when the element is repeating in the code, the compiler automatically ignores the second third, or any type of repeating of the same element and therefore, the repetition is not allowed in dictionaries.
Let us now look at some examples of dictionaries where we will use our Python concepts and some functions to interact with the dictionaries.
Using List with Dictionaries in Python
We know about the details of lists in the Python programming language, and now we want to connect things together so that we may understand why the students had to learn a lot about lists. Python provides uncomplicated ways to use different functions and concepts and get useful results. In the Jupyter Notebook, we are now inserting the list into our dictionaries in two ways, and in this way, the concepts of lists will also refresh our minds.
Inserting the List as an Element in Dictionaries
During discussion about some important characteristics of dictionaries, it was mentioned that any data type can be inserted into the dictionaries, and this point will be proved with the help of the following code:
#declaring the dictionary of tooth brush
toothBrush = {
"Name of Item": "toothbrush",
"electric": False,
"price": 60,
"available colours": ["red", "white", "blue"]
}
print("Features of tooth brush in detail: ",toothBrush)
print("Price of the tooth brush= ", toothBrush["price"])
When we input this code in the new cell of our local host, after pushing the run button, the following results can be obtained:
Hence, it is proved that any data type in Python can be inserted into the dictionary, and this characteristic gives the programmers the independence to use the collection of data and relate it to a single item in the dictionary. In the example we have just seen, there were multiple options for the same brush about its colours, and hence, in such cases, the programmers use dictionaries to get the required results.
Using the Dictionary in List
Here comes a different kind of concept that we have not learned in this tutorial until now. The list can also have the dictionaries as the element and if you are wondering why the programmers use them then you must know, the characteristics of the data types matter, and each data type has its own speciality therefore, the combination of both these data types in different ways gives us the variety of uses.
#Create a list of dictionaries that represent the prices of different items
#along with their serial number in the grocery store.
myStoreItems = [{345: 'chicken', 567: 'eggs',
561: 'beef', 879: 'mutton'},
{348: 'milk', 670: 'butter',
127: 'bread', 445: 'tea bags'},
{237: 'chocolate spread', 381: 'jam',
890: 'sauce', 340: 'sandwich'}]
print(myStoreItems)
#accessing the data from the first dictionary
print("The required item is ",myStoreItems[0][561])
#accessing the data from the second dictionary
print("The required item is ",myStoreItems[1][127])
#accessing the data from the second dictionary
print("The required item is ",myStoreItems[2][340])
In this way, we can extract the following concept from this code:
The dictionaries can be used in the lists.
There is the possibility of using different dictionaries in the same code.
The data can be accessed from any dictionary by using the index of the data.
To get the exact element, the programmers have to write the index of the element.
For using the right indexing method, first, we have to mention the dictionary number and then the key of the element.
It's a reminder that indexing starts at 0.
It becomes easy to manage the data with the keys and more data can be stored in a single dictionary in a more manageable way.
The declaration of the dictionaries resembles the sets and we see many features of these two concepts that resemble each other.
In this lecture, we started our discussion with the introduction of dictionaries. The list has many informative features, and the combination of dictionaries with the list makes many useful tasks easy to complete without facing any issues because of the smooth process. The user simply has to feed the required data, and at the appropriate time, the data can be retrieved easily. So, that was all for today. Dictionaries have applications in different ways, and therefore, it is not possible to provide students with all the necessary information in a single lecture. Therefore, in the second part, we will discuss more details about dictionaries and learn a lot through examples.