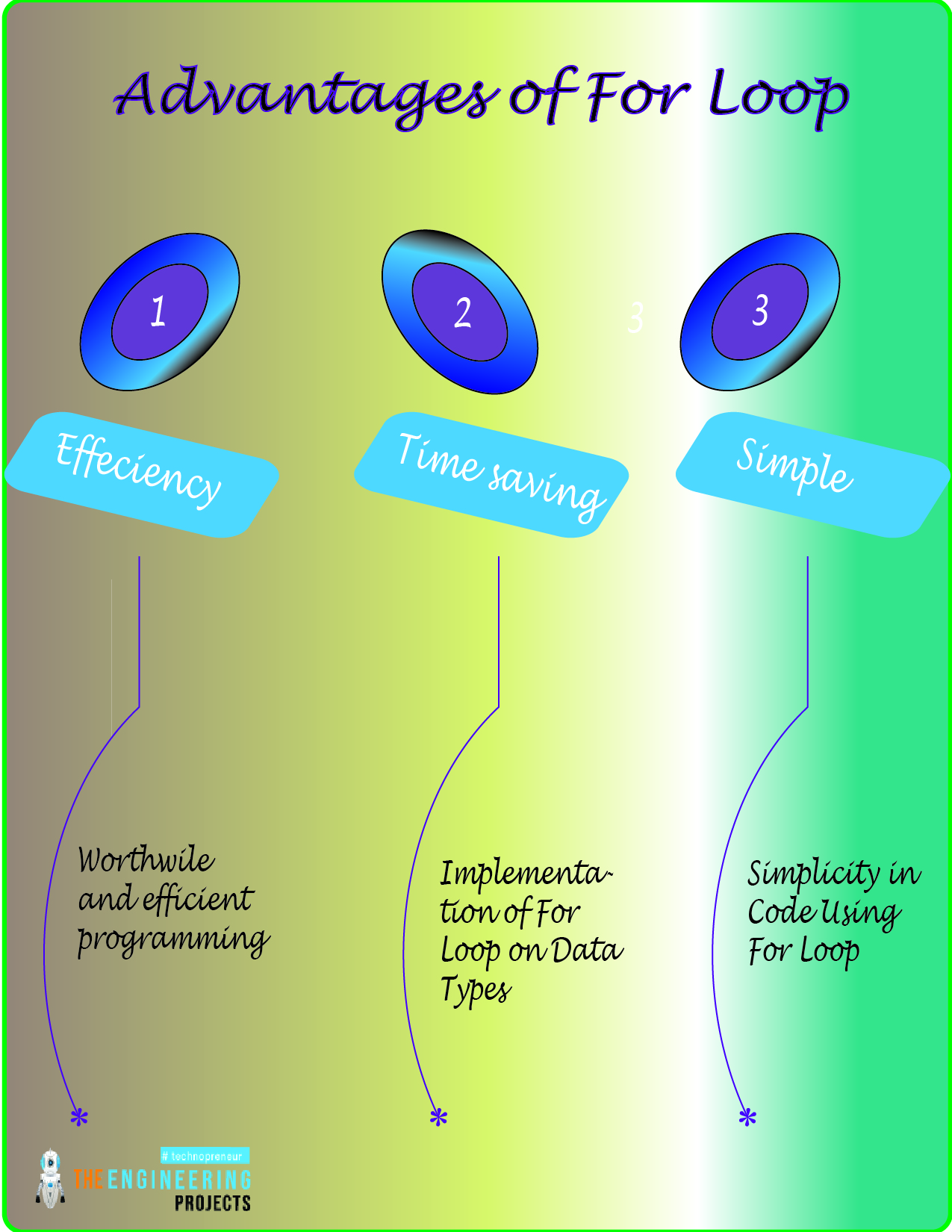
Hey peeps! Welcome to the new tutorial for the Python programming language, where we are at the point where we are going to learn about the loops. Loops are fundamental concepts in programming, and if we talk about Python, there is a smaller variety of loops in it, but the most important ones are available to practice in Python. In the last session, there was a long discussion about the while loop in detail. We had seen some examples and the details of the statements that can be used within the while loop. In the present lecture, we are going to discuss the second type of loop in Python, that is, the for loop. You will go through the training of every important and basic step about this loop, but first of all, you have to look at the basic list:
Why do we prefer for loop over some other concepts in Python programming language?
What are some basic concepts that every programmer must know about the for loop in Python?
Describe the flow chart of the for loop.
What are some examples that explain the for loop in Python?
How do we use different sequences in the for loop?
What is the range function explain it in detail by using the for loop in Python?
There are other ways to get the same output as we will get in this lecture but using the for loop is one of the easiest and most fundamental ways to get the best results. Have a look at the detail of each question.
What are The Advantages of For Loop?
The for loop is the basic looping process in Python that is extremely useful in making the basic to higher level codes in simpler way. We all had the introduction of the For loop in the previous lesson, and now, have a look at the following positive points about this loop:
Simplicity in Code Using For Loop
The for loop makes the code very simple and the programmers do not have to pay much attention to make the logic. You can get the idea of its simplicity that the user can initiate the loop in just a single line if all the logic is clear in it. After that, the body of the loop starts. Just like other loops, the For loop gives us the independence to adjust the flow of the code so that we do not have to write the same block of the code again and again.
Implementation of For Loop on Data Types
Different data types can be used in the for loop and the best thing about it is, it becomes super easy to use the For loop to manage the sequences. It would not be wrong to say that For loop makes the working of the sequence best therefore, for loop is perfect for the sequences such as lists, tuples, and arrays. Moreover, the traversing of the sequences is easy with the For loop.
General Loop
The for loop is not a new concept but in the old higher-level programming languages such as C and C++, for loop is used therefore if a programmer is moving from such basic programming languages to Python, the concept will be cleared before they start it. In other words, the for loop is a generic type of loop that once understood, can be useful as well when they switch to the new programming language.
Basics of For Loop
We know the introduction of the for loop but this time, we are going to discuss the detail of every process that will be useful in the practical coding using the for loop. Keep in mind, loops are the statements and not the whole code. This helps to manage the code in a better way and to reuse the same block by merely inserting it in the body of the loop.
Syntax of For Loop
We are discussing the generalized form of the For loop and therefore, all the concepts are very general that we have discussed till now. Yet, the only difference in the coding is the syntax of the loops and therefore, we are discussing it at first to get an idea of what actually is going on.
for iterator in sequence:
statement(s)
It seems very simple here but the statement is not that much simple. The programmers, then using the for loop add multiple statements in different ways to get the required output. More things will be cleared when you will see the flow chart.
Flow Chart of The For Loop
The best way to get the concept of the for loop is to check for the flow chart of this loop. Have a look at the picture given next and then we will move towards its discussion.
Here, you can compare the syntax and flow chart and get an idea of what happens when data is input in the for loop. At the start, when the condition is applied on the for loop, the compiler checks for the condition if it is true or not and as you can guess, if the condition is true, the compiler will check for the body code
Examples of Data Types with For Loop
Different data types can be used with the for loop and we will move from basic to complex ones. We all know about the string; therefore, here is the first example with the string message.
String with For Loop
#initializing the message
message= print("Enter the string")
#taking input from the user
str=input(message)
#using for loop to print the message from the user
for i in str:
print(i)
Output
Here, when we run the program, we get the dialogue box it says the “none” word with the dialogue box. This will look strange but it makes sense. With some functions in Python, the return value is mentioned in the output. Since this function will not return any result but will only give us the same string that we are going to input in it, therefore, there is the word “none” mentioned in the dialogue box. We can solve it in different ways but the point I want to make here is to clarify the reason behind this indication.
When the string message is typed by the user, the same message with the same format is shown on the screen but this time, as we are using the for loop, each character is picked by the compiler one after the other and therefore, the result will be obtained in the vertical form with each character at the new line.
Here, you can see the “none” is added by default without a string message and once it is printed, the for loop picked each character and printed it in a separate line. Even the spaces between the words are counted, and we get a clean and understandable output.
Have you tried the code given above? If yes then there must be a question in your mind that what happens if we input any other data type instead of string? Actually, this is not a precise code yet, if we add the restriction to add only the string as we have done in the previous lecture, the compiler will not accept any other data type other than string. Yet, to keep things simple, I am not changing the code as the main focus is on the for loop till now.
Sequences in Python Using For Loop
Here comes the practice that is specialized in the for loop. Since the beginning, you have been noticing that we are emphasising the sequences. We all have practised them and in some examples, the for loop was used before but since this is a pure practice of the for loop, I have made a program that contains all important sequences in a single program.
Code of Sequences:
#initializing the List to use with the For loop
print("\b Names of the fruits")
fruits= ["strawberry", "cherry", "blueberry", "apricot", "tomato"]
for i in fruits:
print(i)
#initializing the tuple to use with the For loop
print("\b Price list of mentioned fruits")
priceOfFruits= (34.8, 11.7, 126.9,11.6,11.5,22.0,1)
for i in priceOfFruits:
print(i)
#initializing the array to use with the For loop
print("\b Random things and numbers")
items= {"lipstick",3,"papers", 3, "pen", 1, "bread", 3.4 }
for i in items:
print(i)
Output:
Hence, the program is clear as we have practised it before. The type of bracket represents each sequence and it has its own characteristics. For loop access each and every element of the sequence and print it on the screen. The arrangement of the items in the array is random and we can not suggest the sequence of the elements because it randomly picks the elements and print them. Hence, every time we run the code, the value stored in the variable i is different and the one, printed on the screen, is never repeated at that particular run time.
Range Function in For Loop
The next topic here is the range function that has interesting applications in the fields like gaming. It is because it can be used in different ways as it has the independence of the number of parameters. The syntax of the range function is the same with the for loop as it is with other statements.
x=range(start, stop, step)
Where,
x=name of the variable in which the value of the range is being stored.
start=starting point of the series
stop=ending point of the series
step=The interval between the range member.
Keep in mind, you can use one, two, or three parameters at the same time according to your easiness. Instead of using separate examples for each case, we have designed a program that describes the all. Have a look at the code and then we will discuss it more.
Code of Range Function
#using the range function to print the table
num = int(input("Enter the integer for which the table is required? "))
for i in range(1,21):
print(num,'x',i,'=',num*i)
#range function with one parameter
print("\b using the range function with only ending point")
for i in range(7):
print(i)
#range function with two parameters
print("\b using the range function with starting and ending points")
for i in range(2,9):
print(i)
#range function with three parameters
print("\b using the range function with start,end, and step points")
for i in range(1,10,3):
print(i)
Output
Discussion about The Range Function
For your convenience, we will go through the basic to the complex concepts.
The three parameters are useful to get a uniform series of numbers.
Only integers can be used with the range function.
If the start point is not mentioned, it always starts from zero.
The stop parameter is the compulsory one to use in the range function.
To make the restriction of only integers, the “int” keyword is used with the input function in a specific syntax.
You can get the table of any number with the range of any integer easily using the range function.
For loop is the best way to get the required output from the range function.
Consequently, we can say that we are not beginners and instead of learning the long theories about the basic concept, the focus of our lecture was towards the practical examples of the for loop therefore we started this lecture with the introduction and then moved towards the basics, syntax, examples, and the codes using different data types. As the specialized data type of the for loop is sequence, therefore, we had the practice of the for loop with the sequences. Using the range function with for loop gave us the interesting outputs and with the help of these examples, we learned the for loop in a better way. The upcoming lectures are interesting tooo so keep practicing and stay with us.