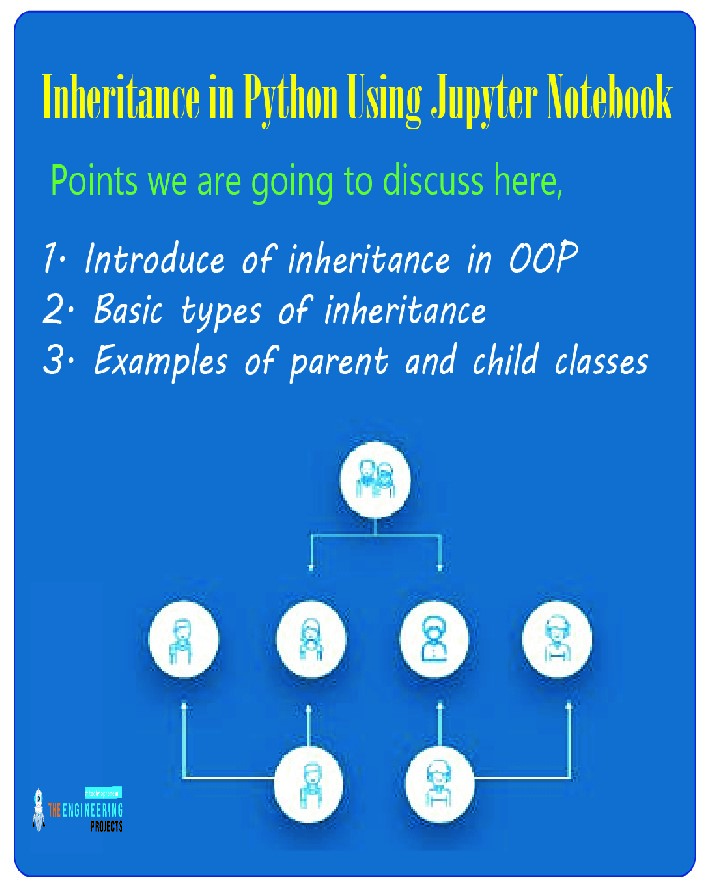
Hola students! Welcome to the new Python tutorial, where we are learning about OOP. We all know why OOP is important in programming. In the previous lecture, a detailed overview of classes and objects was discussed. We are moving towards the next step of learning where our focus will be on inheritance. Yet, programming also contains inheritance. We have all read about inheritance in real life and are aware of the concepts surrounding it. This time, we will discuss these concepts according to the workings of the classes and related entities. The concept of inheritance is the same in both scenarios, and with the help of interesting examples, the practical implementation of inheritance will be shown to you, but first of all, it would be interesting to know about the main headings that will be shared in detail with you later.
How do you introduce the inheritance in OOP and how do you compare it with the biological inheritance?
What are the basic types of classes that are used in inheritance?
Give some examples of parent and child classes.
How do you explain __init__() function with inheritance?
What do you know about the self keyword?
How to override the parent class in inheritance?
What is Inheritance in OOP?
Inheritance is the fundamental concept in OOP, and with the help of Python, the programmers can easily get the required outputs with minimum effort. Keep in mind, OOP is applicable because of its feature of reusability of the code in the perfect way. This helps to curtail the redundancy while working on the development projects. There are different ways of recycling the code in Python, and inheritance is one of the most prominent ways to do so. As in biological inheritance, where the characteristics of the parents are transferred from parents to children, so is it with inheritance in object-oriented programming. The whole concept is about the relationship between different classes and this is important to learn for the large and complex projects of Python.
Types of Classes in Inheritance
We have discussed in the previous lectures that different classes can be used at the same time in the same code, and this is the time to utilize that concept. When there is more than one class, it is important to make connections between these classes. Inheritance is a relationship between different types of classes, and this is the basis of the concept of inheritance. The following are the main classes used in inheritance:
Parent class
Child class
Parent Class
The parent class is also known as the base class, and it has the main characteristics that can be inherited or copied by the other classes. Any class can be the parent class, and therefore, there is no special syntax for it. The way to form the simple class is considered the parent class. So, we can think in our mind that all classes are parent classes until they are derived from other classes. Here is an example of a parent class.
Example
Let us take an example from the real world so that you may understand it well. We all know about bank accounts. If we consider the bank account as a parent class, then the types of the bank accounts such as business accounts, saving accounts, etc have many attributes that the “bank account” class may have. So we can consider the savings and business account as the child class so whenever we declare them, we do not have to write all the attributes of other types of bank accounts.
Code for the Parent Class:
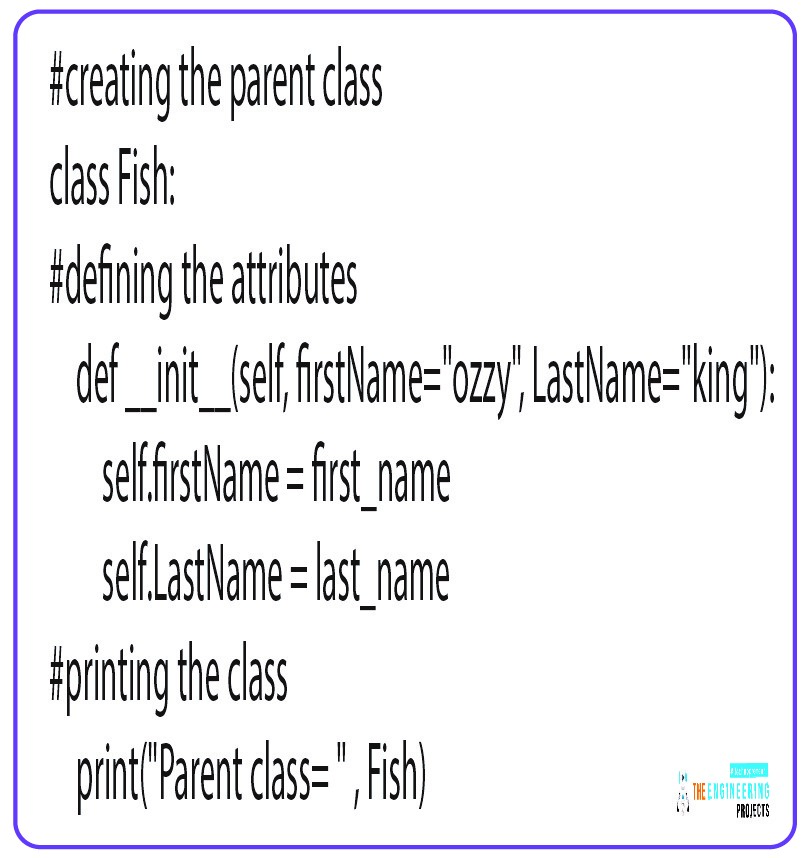
#creating the parent class
class Fish:
#defining the attributes
def __init__(self, firstName="ozzy", LastName="king"):
self.firstName = first_name
self.LastName = last_name
#printing the class
print("Parent class= " , Fish)
Output:
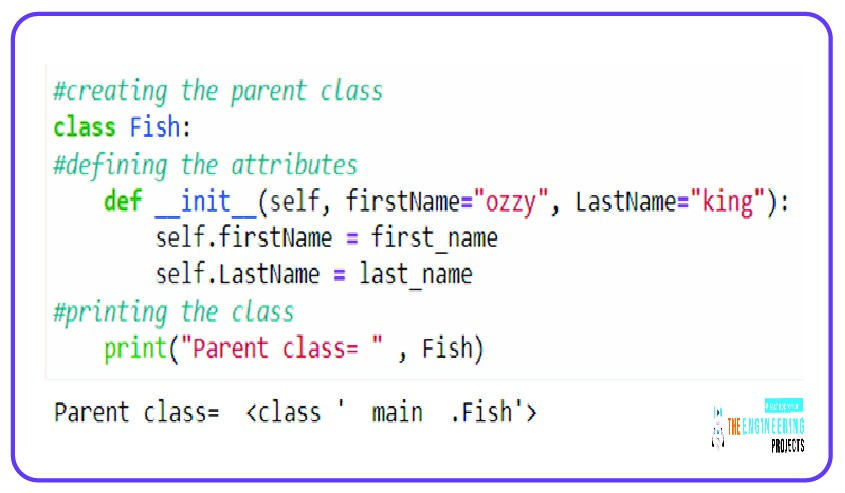
In this code, we have just made a simple class with some attributes and considered it the parent class. As you can see, we can make more objects of this class and use it in different ways, but for now, we are not moving forward because the concept here is clear and one that we want to discuss.
Child Class
As you can guess, the child class is the one that is derived from the parent class and has the same attributes as the parent class. It is a subclass and has a slightly different syntax than its parent class. Let’s say you are talking about bears, All bears can walk, run, eat, and sleep. So, these attributes remain the same, and the parent class, in this case, is "bear,” whereas, the polar bear and brown bear are the subclasses. The same is true for all the animals that have the different kinds of the speicies in them because of the same attributes but have many subclasses. The following code will be a little bit confusing, but the discussion will clarify the concept:
Code For Child Class
#creating a new class with the name penguin so the animal bird specie is used
class Penguin:
#declaring the attribute and method of the parent class
name = ""
def swim(self):
print("I love swimming")
# a child class that inherits the message from a parent
class tag(Penguin):
# new method in a subclass
def display(self):
# access name attribute of superclass using self
print("My name is ", self.name)
# creating an object of the subclass
pengName = tag()
#Using the superclass attribute and method
pengName.name = "tag"
pengName.swim()
# calling the subclass method created above
pengName.display()
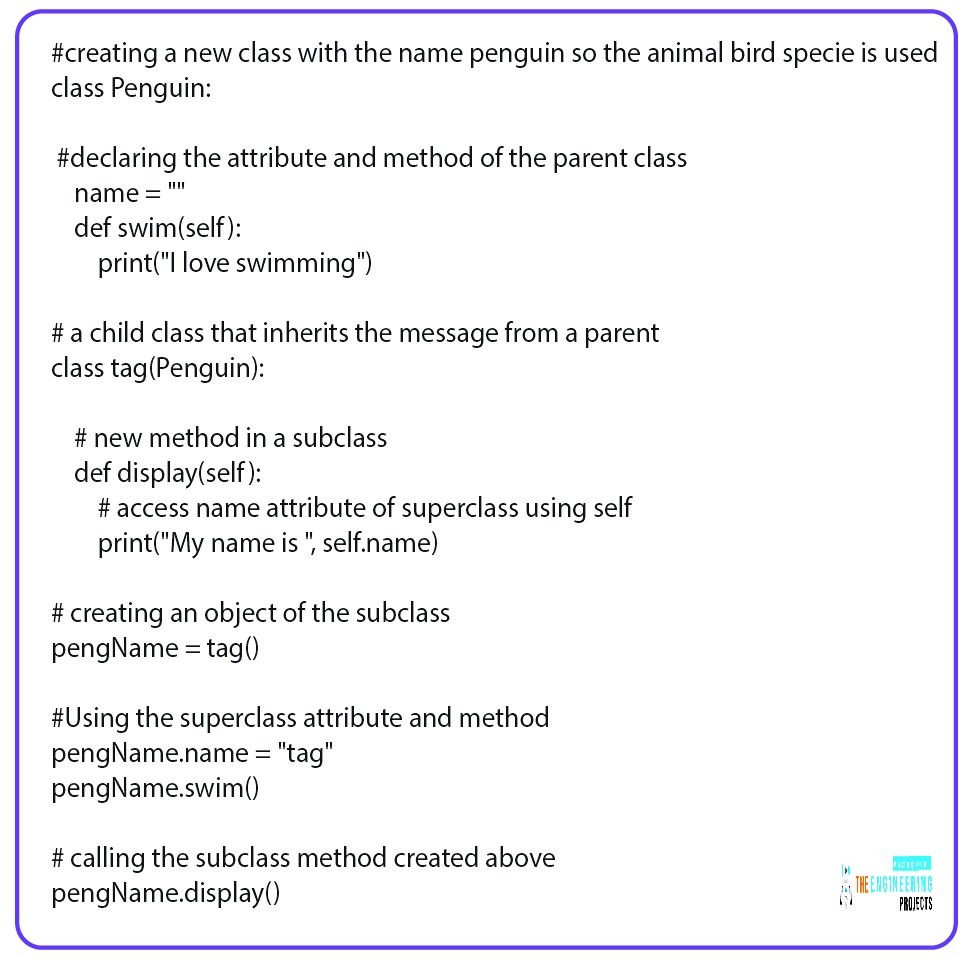
Output:
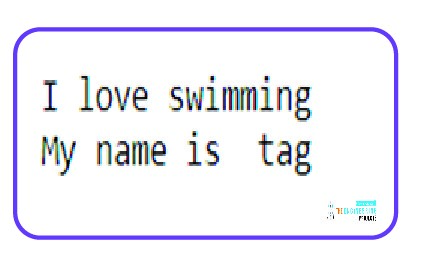
Discussion:
In the beginning, a parent class is declared with the name "Penguin.” This class has two things declared in it. First of all, it tells us that the “name” is in the form of a string. The second thing is the attribute of swimming. This also gives us the string message that the tag can swim. We have written it here just to show that messages from the child and parent can both be used in the output.
In the next step, a child class is introduced in which the particular penguin is declared to have the name “tag”.
Now, let us use the parent class and get the attribute of the name from it. In this way, the child gets the name "tag." Another thing that happened here is, that a new method was formed to explain swimming.
In the end, a built-in method display() is used to display the name of the penguin with a string message that was shown before.
Keep in mind, the display method is not limited to the inheritance only but can be used with most of the entities of the Python.
Important Terms Used With Inheritance
When dealing with the codes of inheritance, there are different concepts that must be kept in mind so that you may use them with the inheritance, and once the programmers have a grip on it, it becomes easy and comfortable to use inheritance in multiple ways. At this level, it is important to understand the following methods and constructors, and you will notice some of them have already been used in our codes of inheritance. Yet here, we are discussing it in detail so that you may use it in the practice and get the perfect results according to your requirement.
Pass Keyword in Classes
Programming is an interesting field, and when you deal with a hundred lines of code, sometimes, when the programmer does not want to use a specific function for some reason ( when you simply declare the function but there is no need to execute it but other code must be run), then to avoid the errors, the “pass” keyword is used to do so. We can define this keyword as:
"A pass keyword is a simple type of keyword that is used to tell the compiler that the line above this keyword has had no usage till now, so go to the next line during execution."
Sometimes the coders are confused between the pass keyword and the comment, but the difference is that the comment is not part of the code but is the explanation to the user. The pass keyword, on the other hand, simply causes the compiler to jump to the line above it, which may be a function that does not yet have an implementation. The syntax of this keyword is to simply type “pass” right after the line to be skipped.
Example of Pass Keyword in Inheritance
The reason why we are discussing this keyword here is, this helps a lot in inheritance. If there are many subclasses and the programmer simply wants to ignore one line to check whether my other classes are working or not, they use the “pass” keyword in such cases and it seems to be very interesting.
In the code given above, we are simply placing the pass keyword after the declaration of subclass and the compiler will jump on it and is not able to use it in the methods given in the next lines.
Code:
#creating a new class with the name penguin so the animal bird specie is used
class Penguin:
#declaring the attribute and method of the parent class
name = ""
def swim(self):
print("I love swimming")
# a child class that inherits the message from a parent
class tag(Penguin):
#using the pass keyword to jump from the line above
pass
# new method in a subclass
def display(self):
# access name attribute of superclass using self
print("My name is ", self.name)
# creating an object of the subclass
pengName = tag()
#Using the superclass attribute and method
pengName.name = "tag"
pengName.swim()
# calling the subclass method created above
pengName.display()
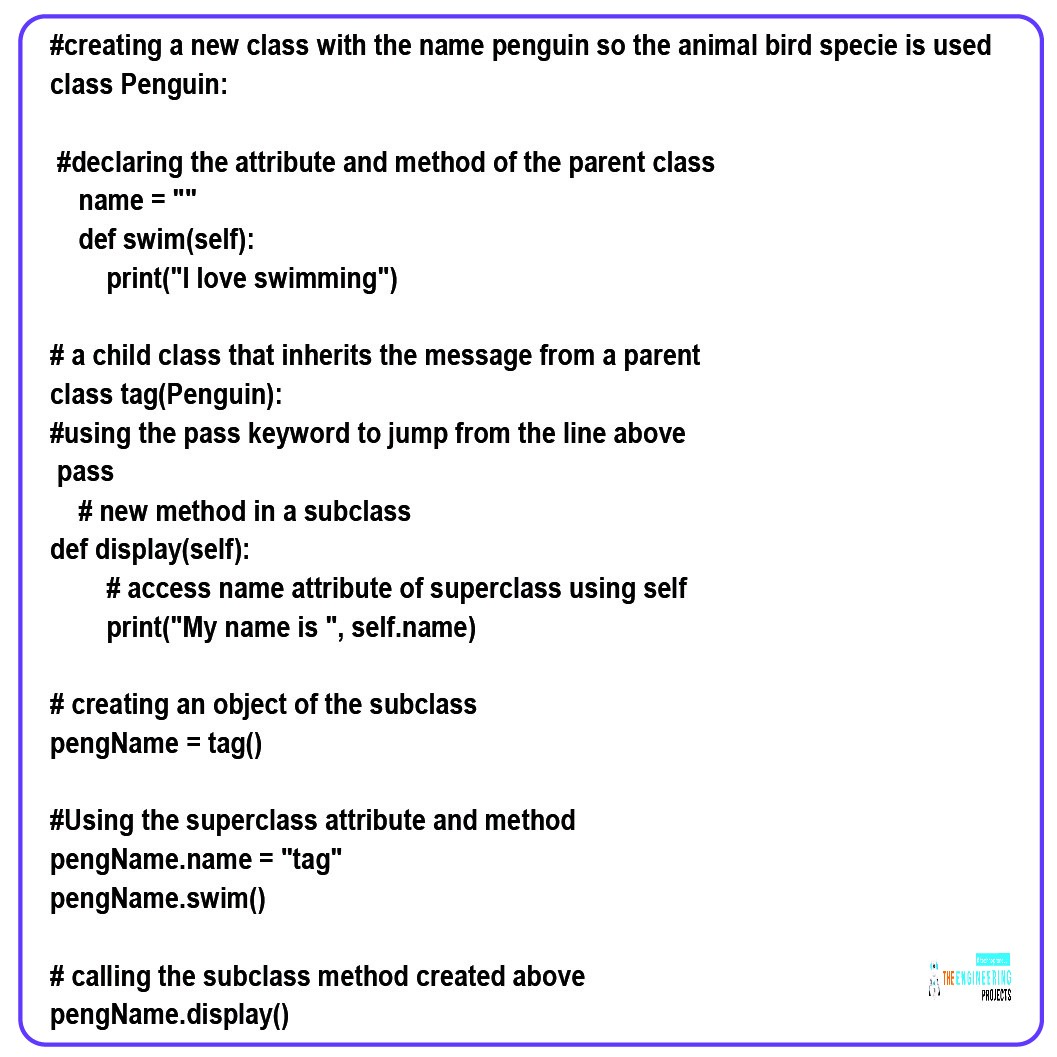
Output:
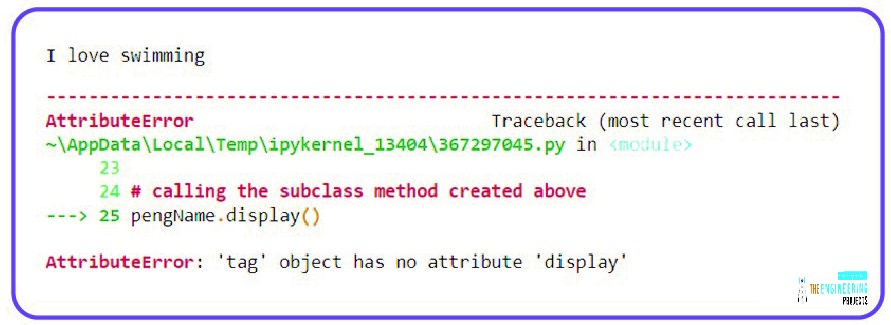
You can see only the parent class is used here, and the child class is jumped. The code is not able to be executed well because of this jump condition.
The __init__() Function
Since we are using the concepts of inheritance, have you noticed the usage of __init()? If yes then you must be wondering what is it. This is the constructor of Python and it is used to initialize the data member. That is required when the object of the class is created. At the time of the creation of the object, this function contains the collection of instructions or statements that it contains.
Self Keyword in Inheritance
In the previous lectures, we also used another keyword to be noticed, which is the “self” keyword. In simple words, we can say that when we are using the concept of classes, the “self” keyword is used to access the attributes and methods of the class. In some other programming languages, the @ is used to refer to the instant attribute.
Overriding Method in Python
Another method that is used in Python, especially with the inheritance concepts, is the overriding method. Till now, we have said that the child class gets the attributes from the parent class, but what if the programmer wants to introduce an attribute with the same name but different features in the child class? For this, we use the overriding method, which is introduced with the statement given next:
"In inheritance, the overriding method allows the child class to have the implementation of the specific method that is already declared in the parent class."
It is important to keep the flow of the code in mind while using the overriding method because incorrect overriding can cause errors or redundancy. Moreover, attributes and classes can both be overridden using this method.
In this lecture, we have learned many simple and smart concepts to deal with inheritance and have learned a lot about them. At the start, we saw the basic definition of inheritance in OOP and compared it with biological inheritance. After that, we have seen what is the child class and parent class. Some important terms about te inheritance was also discussed that were different kind of constructors and methods that we useful in their functioning. In the next lecture, we will talk more about inheritance so stay with us and happy coding.