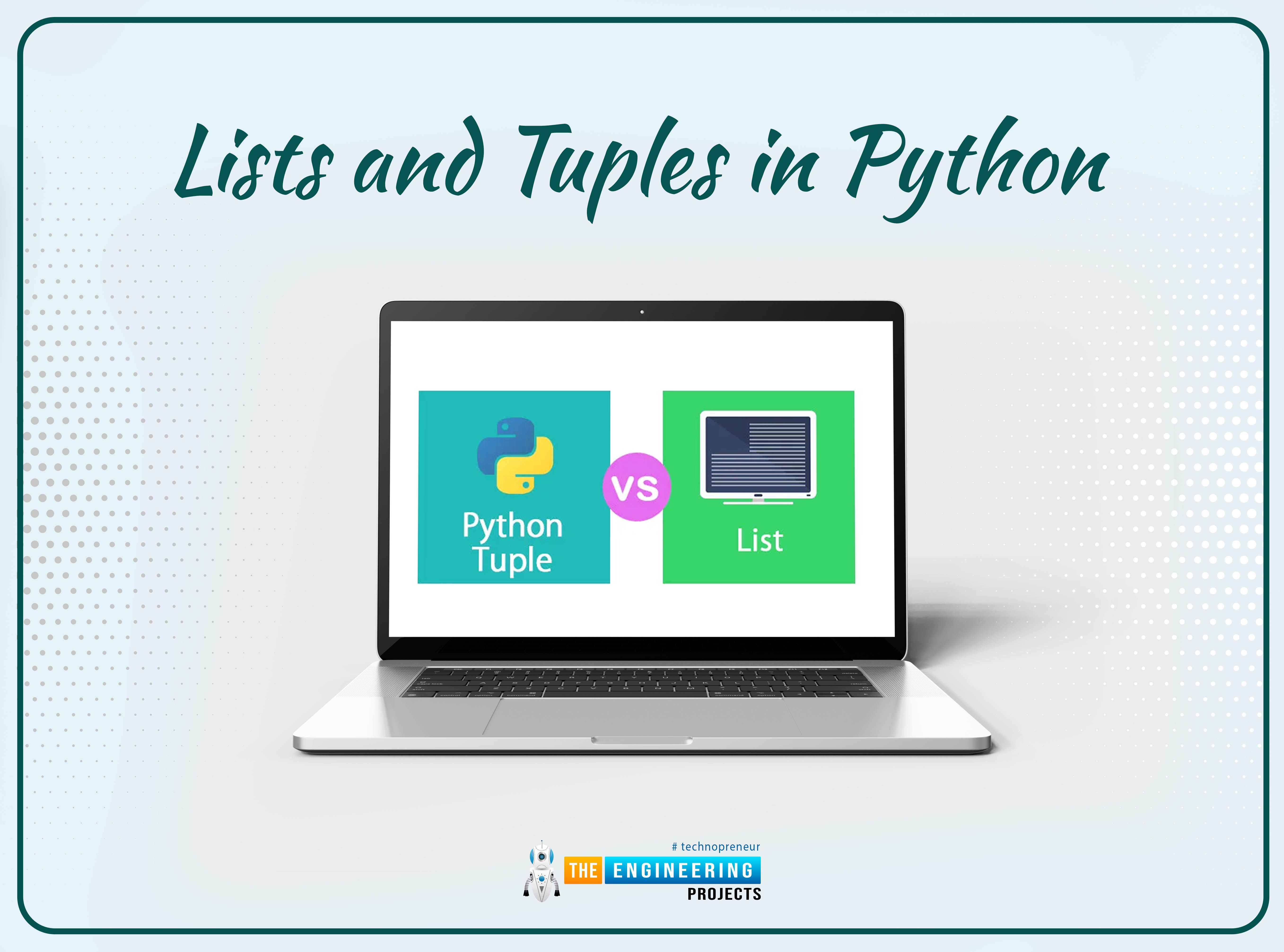
Welcome to the fourteenth chapter of our python tutorial course. In the last lesson, we looked at sets and operations done to sets, including union and intersection. In this tutorial, we'll take a closer look at lists and tuples to see how they're used. Python's most versatile and useful data types are lists and tuples. A non-trivial Python application will nearly always have these.
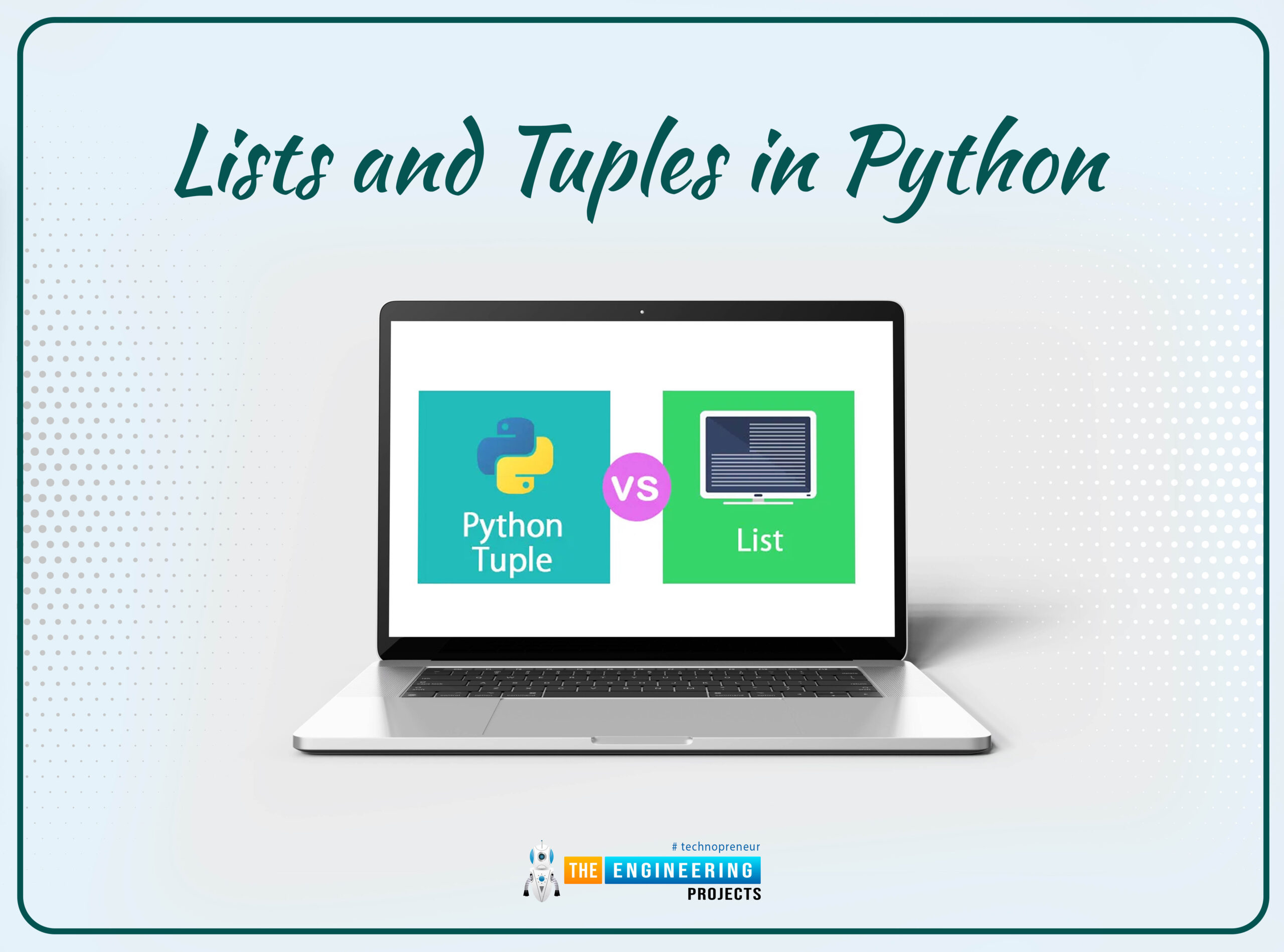
What will you learn?
Lists and tuples have a number of significant features that you'll learn about. In this course, you'll understand the definitions and applications of these terms. By the time you're done, you'll know when and how to employ different Python object kinds.
What are lists?
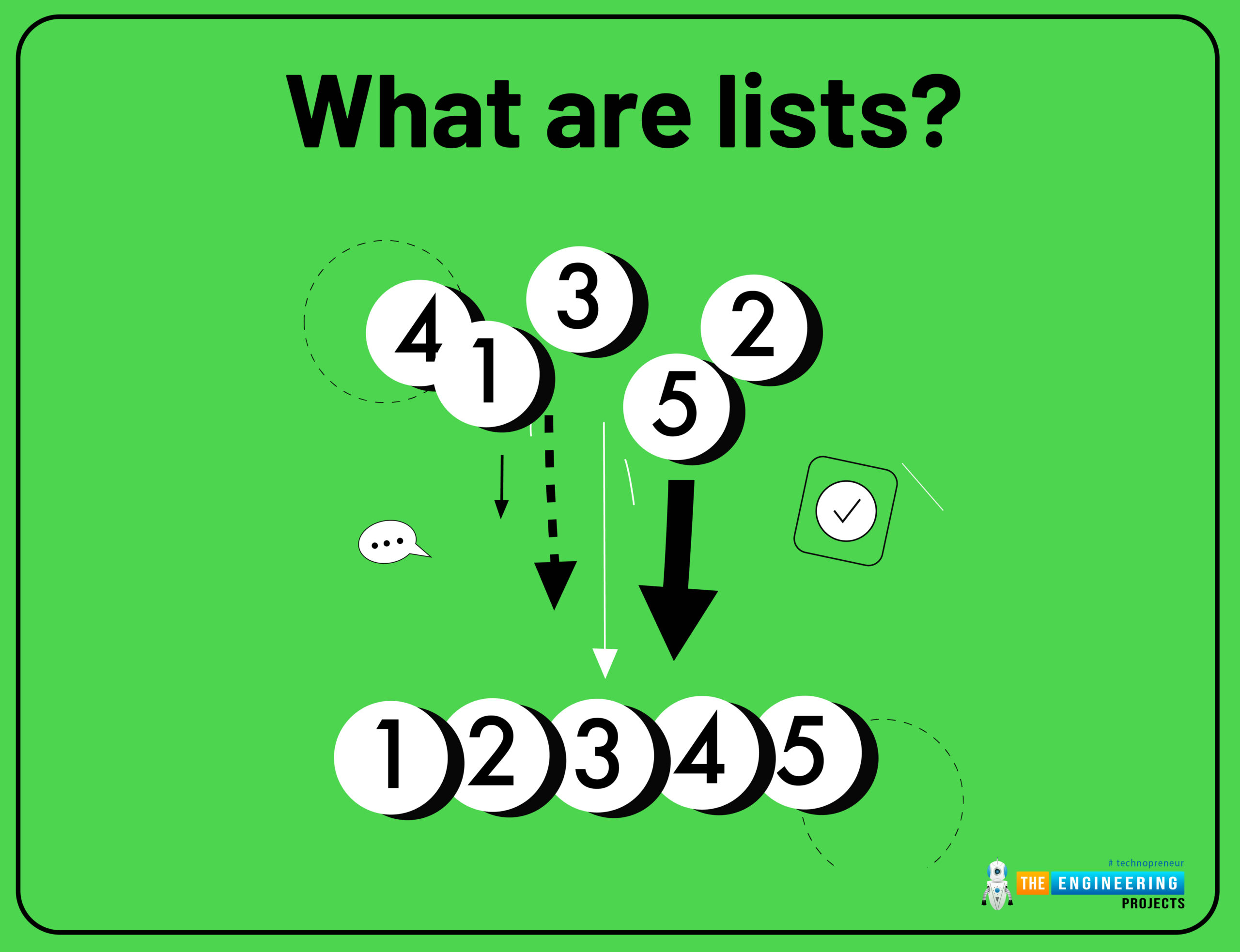
In other words, Lists are similar to arrays in many other programming languages because they allow you to store any number of arbitrary elements within them. For a list to exist in Python, an object sequence must be enclosed in square brackets ([]) as seen in the example below:
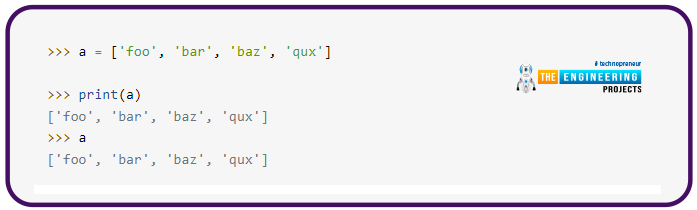
What are the characteristics of Python lists?
- Lists are sorted.
- There is no limit to what can be included in a list.
- The index can be used to get at list items.
- You can nest lists indefinitely.
- Lists can be edited.
- Lists are dynamic.
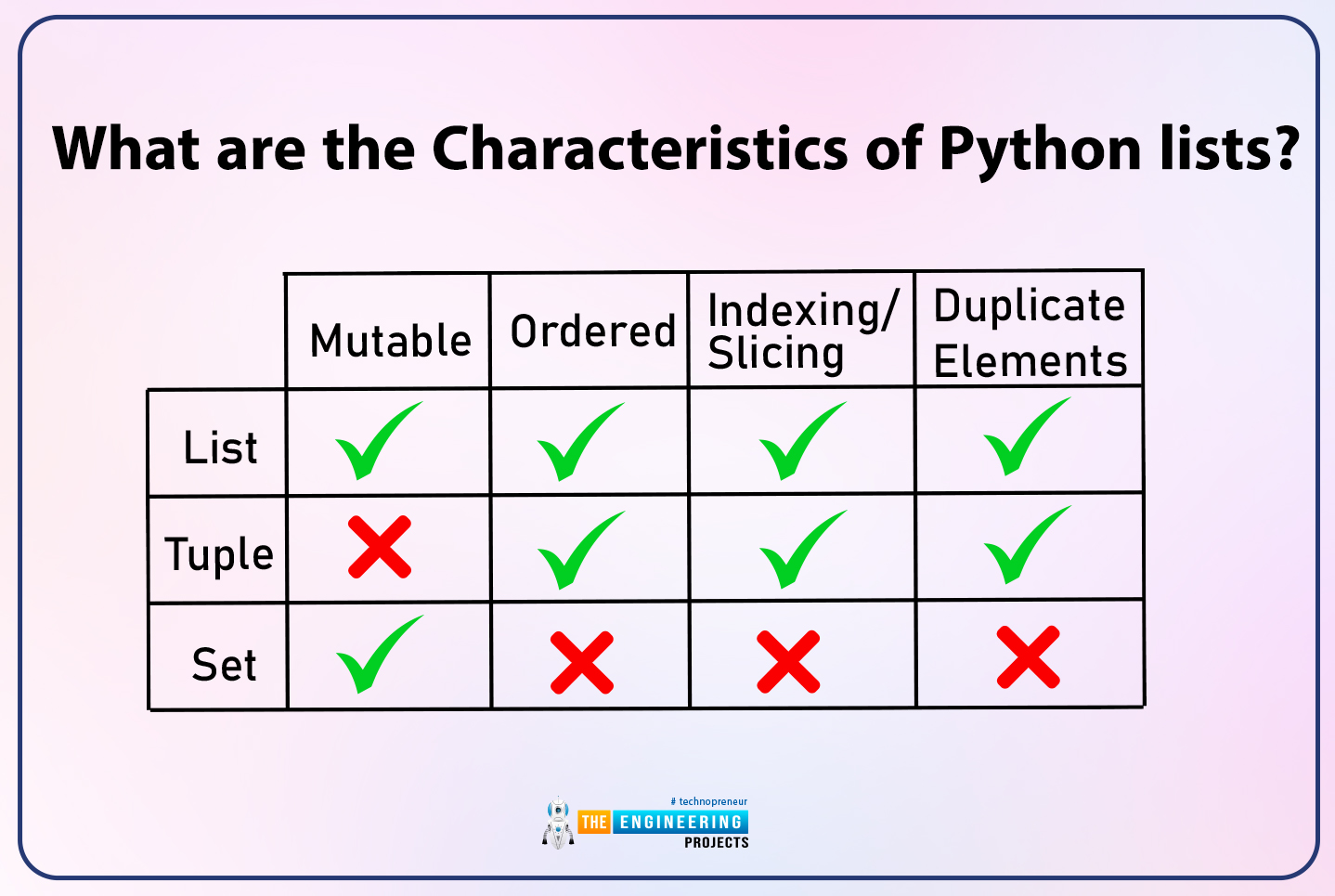
What do we mean by lists are sorted?
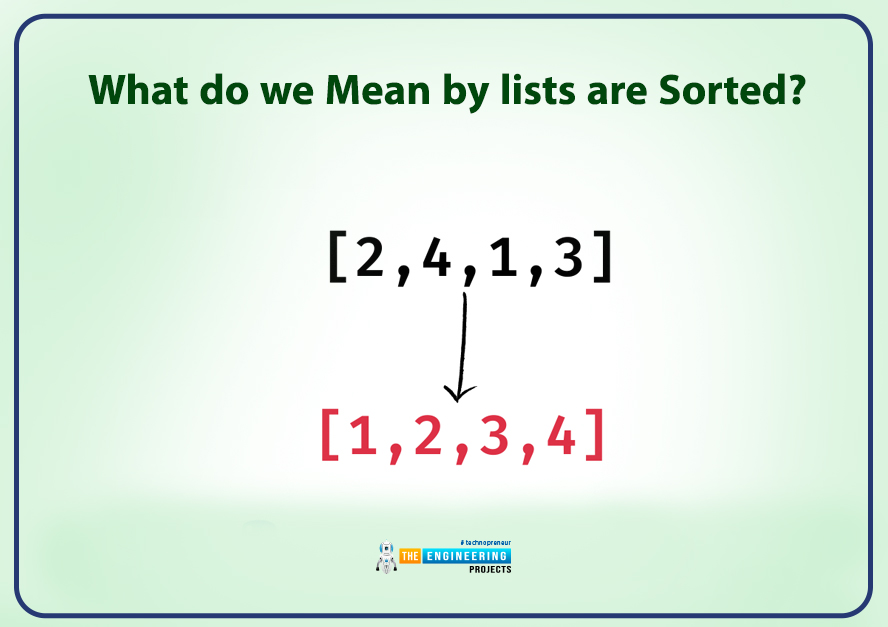
In other words, a list is more than a collection of things. Collections of things are organized in this way. Lists are defined by the order in which their elements are listed, and this order is maintained throughout the life of the list itself. For more information on Python data types, check the dictionaries tutorial (coming soon).
A comparison of two lists that contain the same contents but are organized differently is impossible:
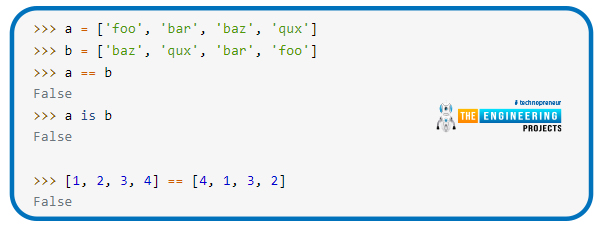
Can any object be included in a list?
A list can be made up of any number of items. A list can have all of its elements of the same type:

Different kinds of elements can be used.
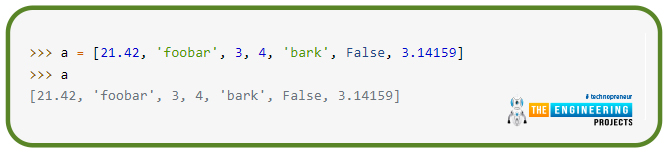
What more can be included in a list?
Complex objects such as functions, classes, and modules can also reside in lists, as you'll see in forthcoming tutorials:
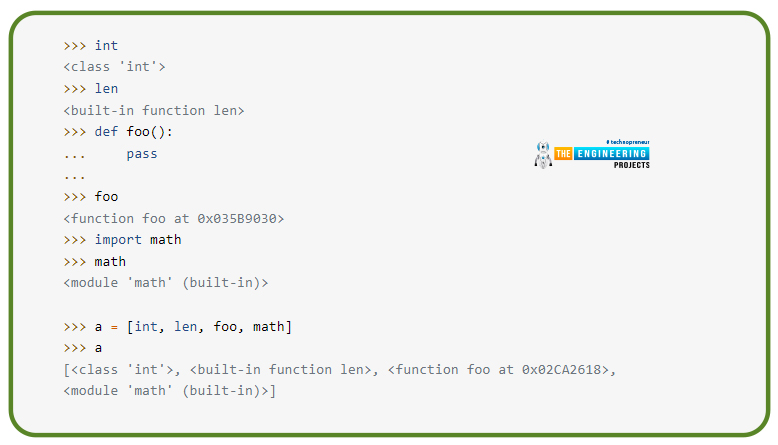
From 0 to the limit of your computer's RAM, a list can contain any number of items.
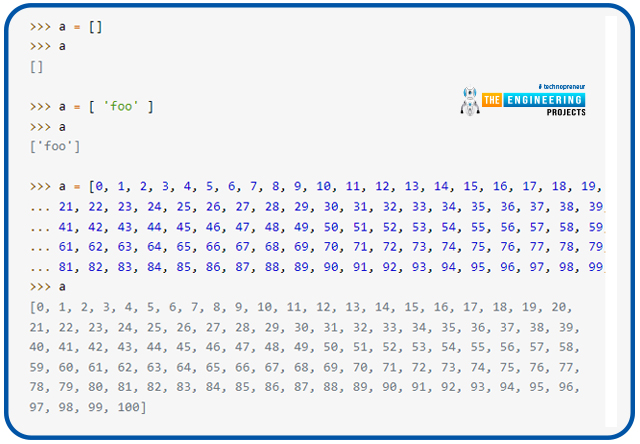
Uniqueness isn't required for list objects. There is no limit to the number of times an object can be listed:
Can list elements be accessed by index?
This is a question you could ask yourself whenever you need to access items in a list, and the answer is yes: an index in square brackets can be used to access items in a list. In other words, it's the same as looking up individual characters in a string. As with strings, the indexing of lists is zero-based. The following is a sample list:

Here are the indices for the items in a:
How is slicing applied in lists?
Slicing is another option. For lists, the formula a[m:n] retrieves only the part of a that is between m and but not containing n in the list a.

What other features are available for slice?
- You may discover some negative values in slice operation, therefore both positive and negative indices can be used. The following is an example:
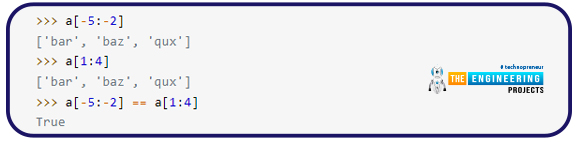
- One way to get around this problem is to just leave out the first and second indexes of the list, respectively:
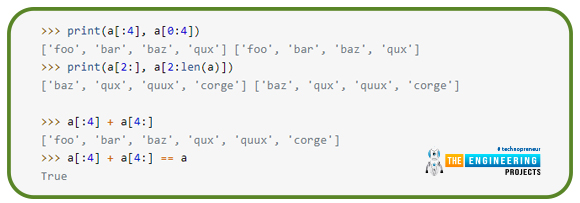
- A positive or negative stride can be specified:
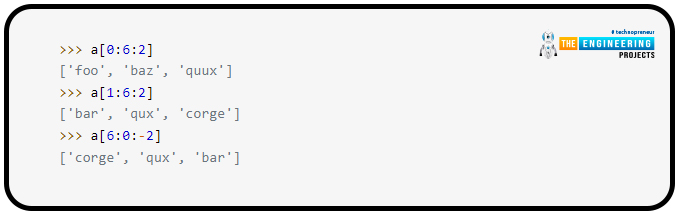
- Use the same syntax to reverse lists as you do strings:
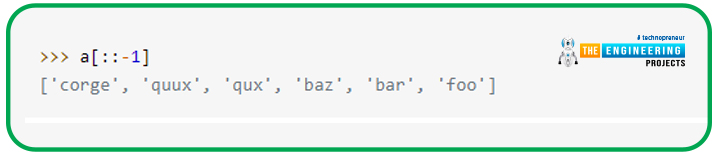
Lists can also be nested
As you learned before, an item in a list can be of any type. Another list is included in that. You can have as many sublists as you want within a single list.
As an illustration, consider the following (obviously fabricated) scenario:

x refers to an item structure depicted in the image below:
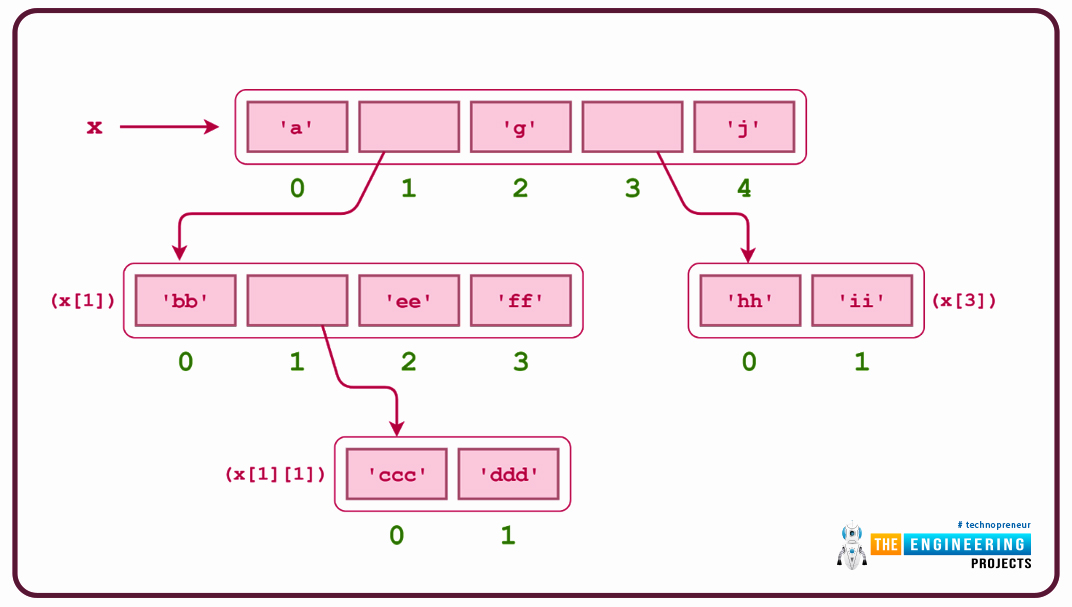
These three strings below, are all one character in length:

Example of sublists are shown below:
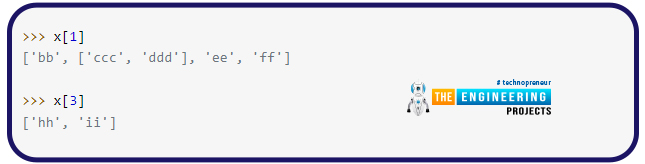
Simply add an additional index to have access to the items in a sublist:
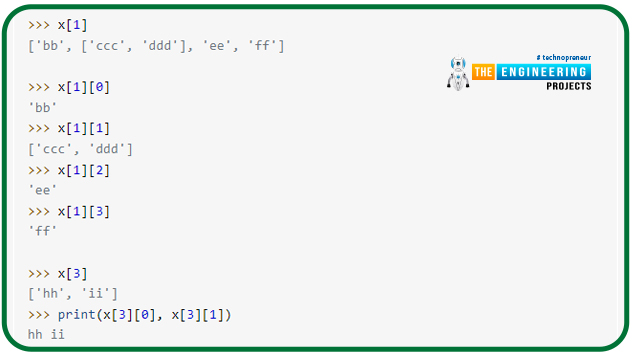
To the degree that your computer's RAM allows, there is no limit to the depth or complexity of nested lists in this manner.
Mutability of lists
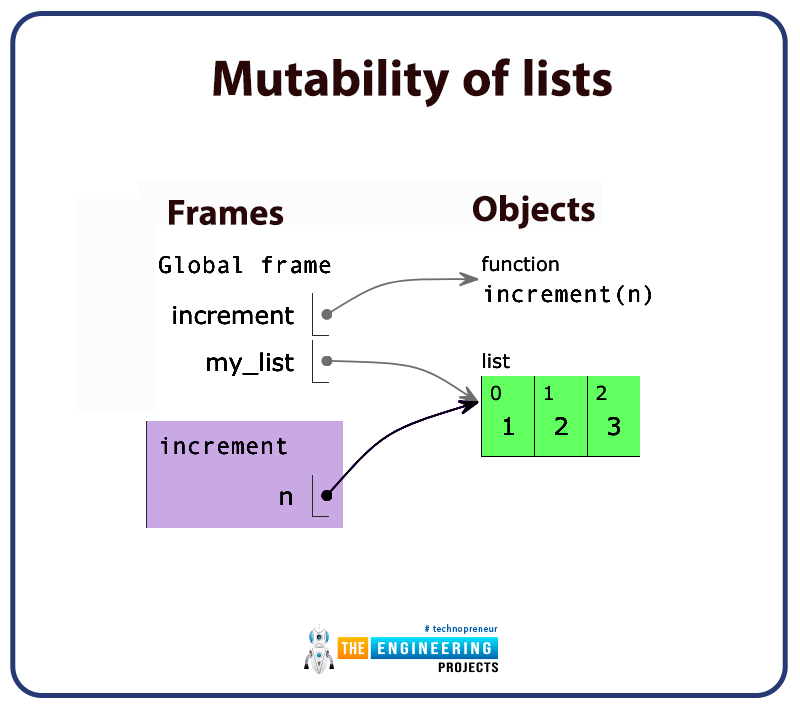
A lot of your experience so far has been with atomic data types. Primitive units, such as integers and floats, are those that cannot be decomposed further. Once they've been allocated, these types aren't able to be modified. Changing the value of an integer doesn't make sense at all. If you prefer a different integer, simply change the one you've assigned.
The string type, on the other hand, is a complex type. Strings can be broken down into their constituent characters. Think of a string of characters and how they might be rearranged. However, this is not possible. Strings are also immutable in Python.
This is the first time you've met a mutable data type, the list. It is possible to add or remove items from a list at any time after it has been created. Lists can be modified in a variety of ways in Python.
Modifying a Single List Value
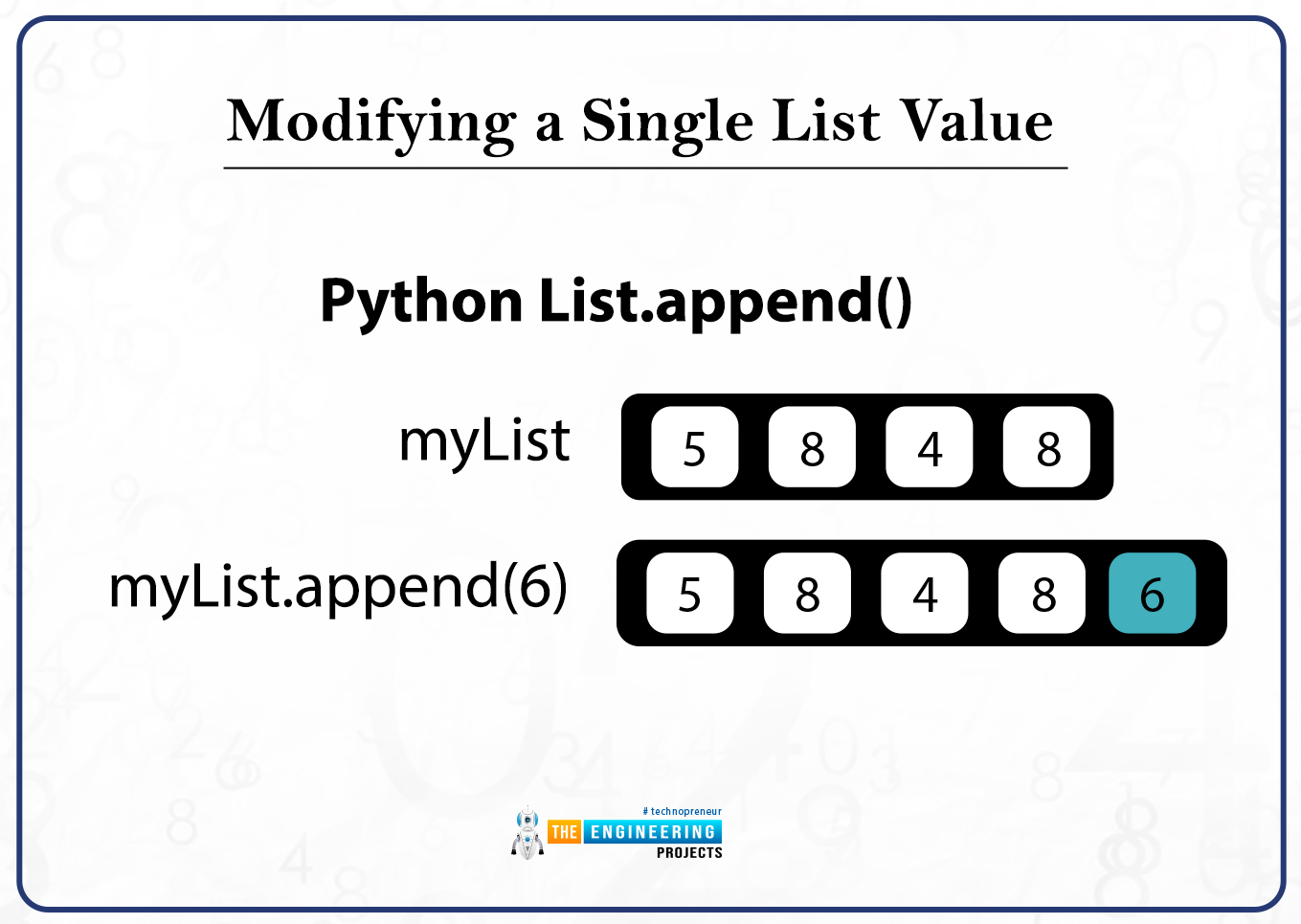
A single value can be replaced in a list using indexing and simple assignment.
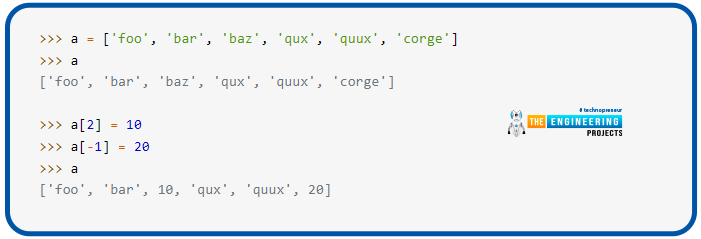
A string can't be used to accomplish this, as demonstrated in the Python tutorial Strings and Character Data.
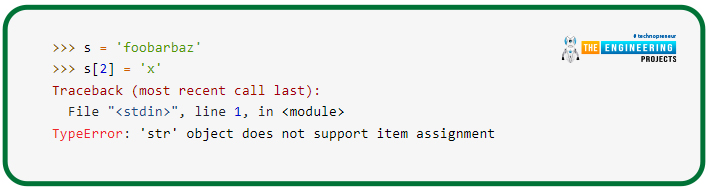
How to delete from a list
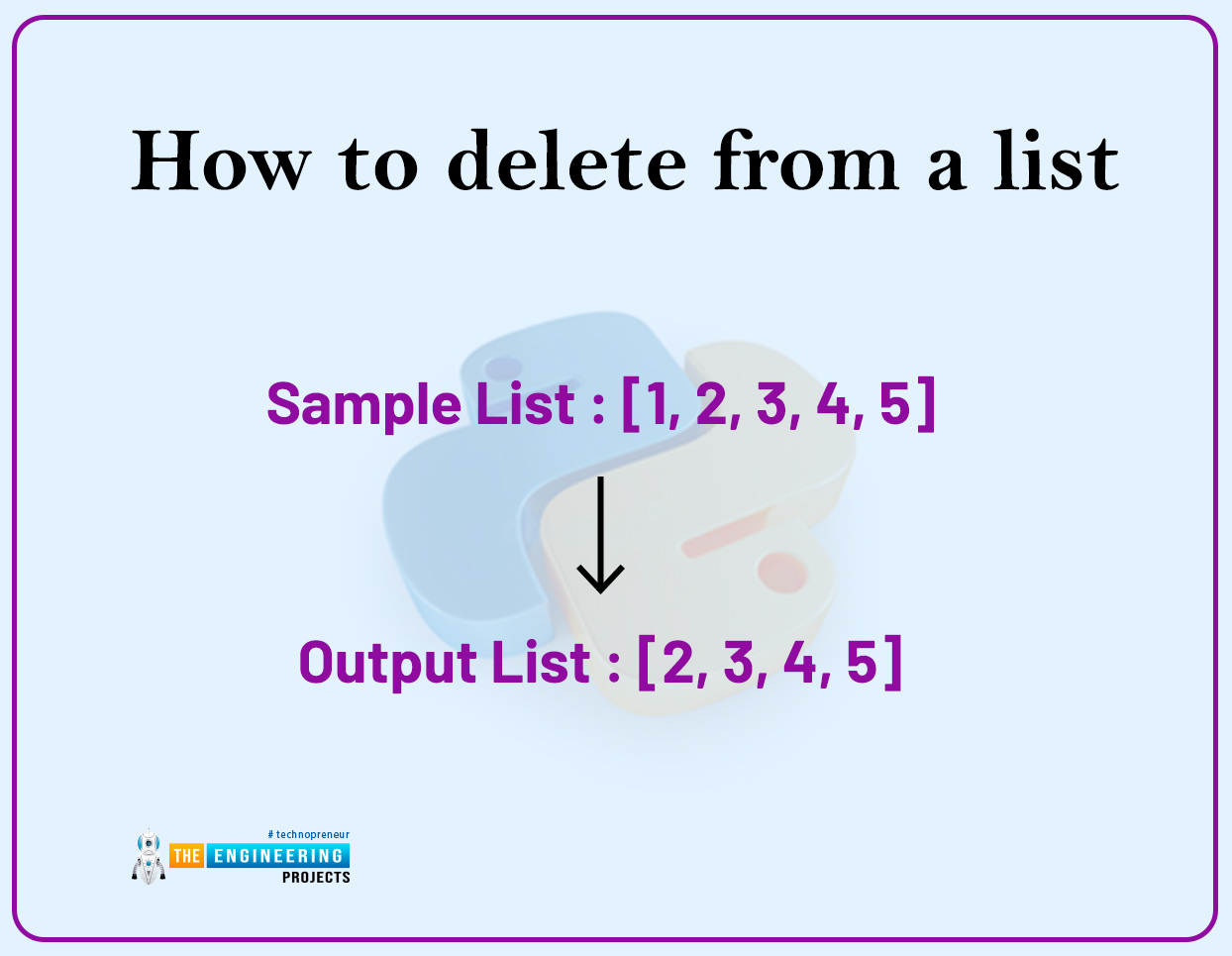
In order to remove a list item, use the del command:
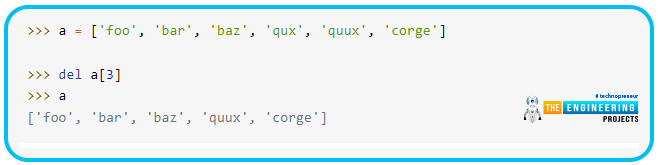
Changing the Values of Multiple Lists
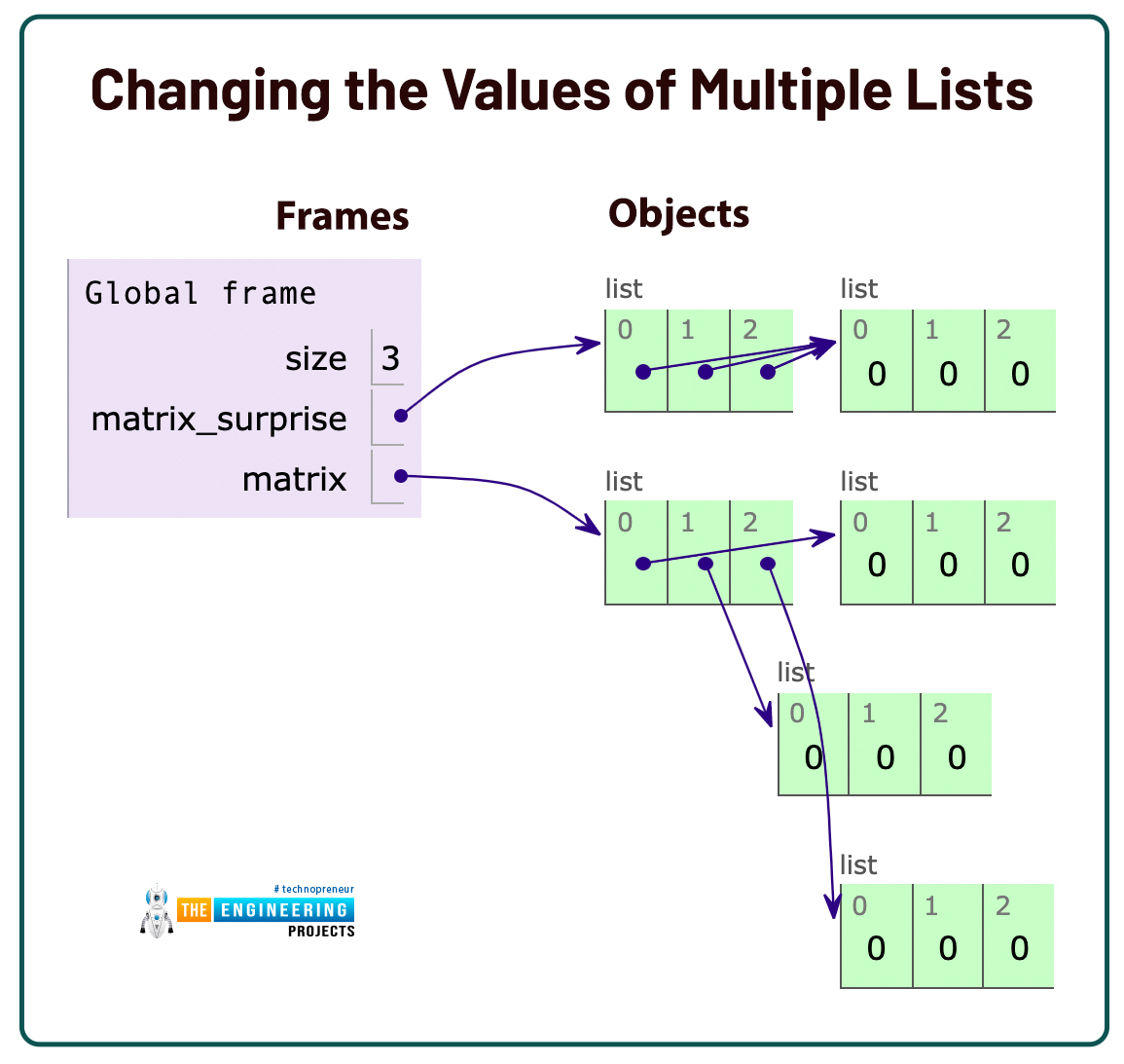
Suppose you'd like to change several neighboring items in a list at the same time. The following Python syntax for a slice assignment makes this possible.

Consider an iterable list at this point. iterable is substituted for the slice of a specified here:
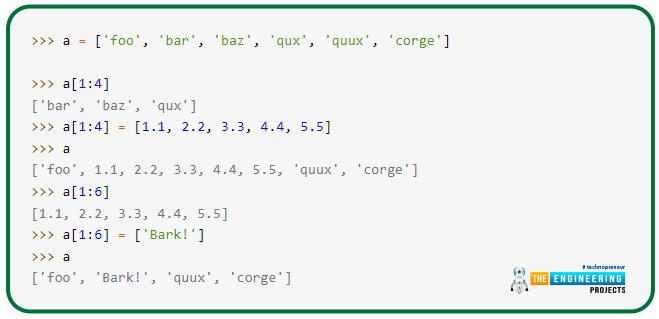
It's not necessary to have the same number of new elements as the number of old ones. Python simply increases or decreases the list based on the task at hand. Utilize a slice which only refers to one element when you wish to replace a single element with multiple ones:
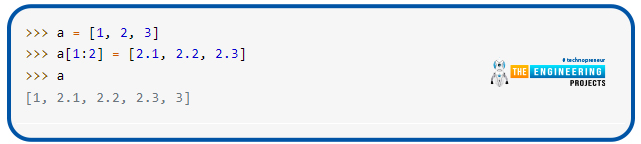
Inserting elements to a list
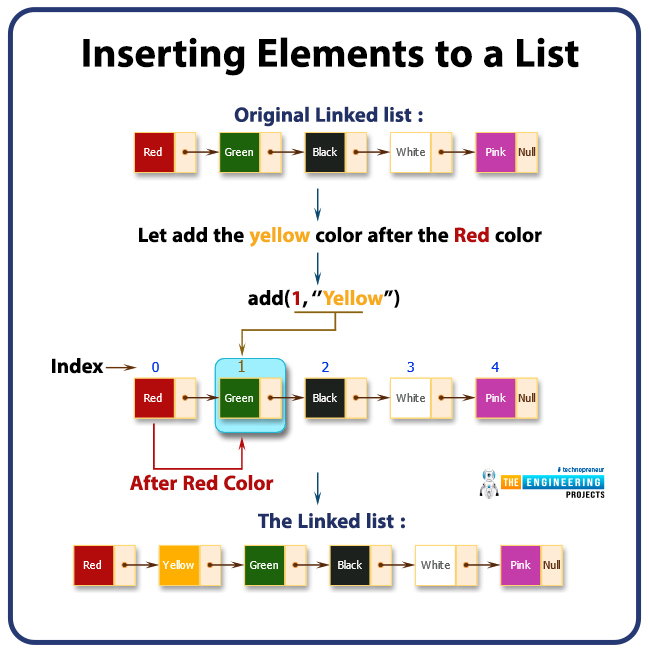
You can also add items to a list without having to remove anything from the original list. Simply type [n:n] to produce a zero-length slice at the requested index.
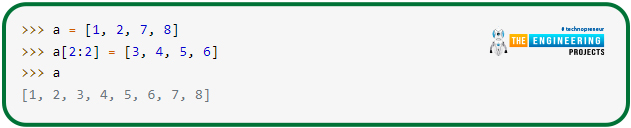
Deleting multiple elements from lists
You can remove a large number of items from a list by assigning the correct slice to an empty list. It is possible to use the del statement with the same slice:
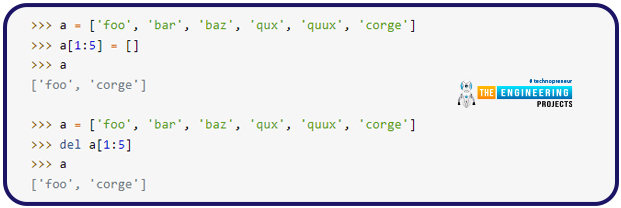
Incorporating Items into a List by Adding Them At The End
To add more items to the beginning or end of a list, you can use the + concatenation operator or the += augmented assignment operator:
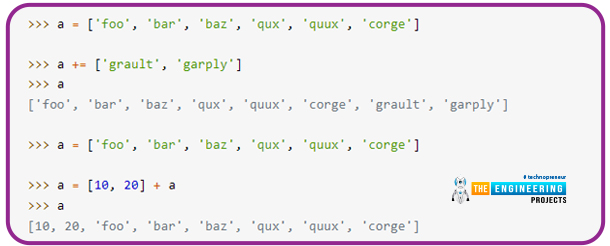
For example, a singleton list can only have one item in it, hence, it must be added to a different list:
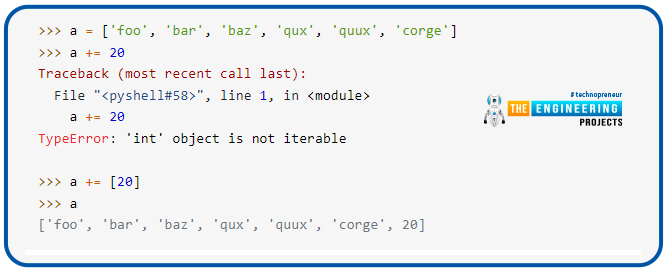
Methods That Modify a List
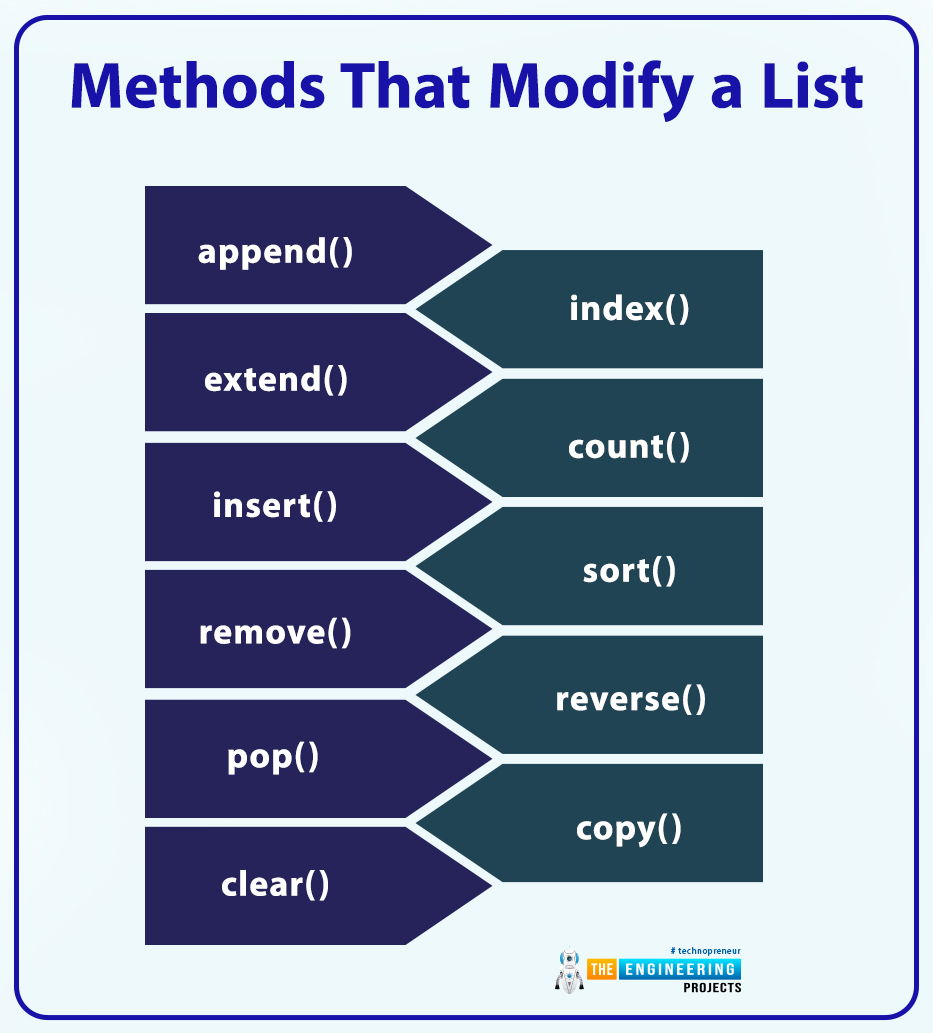
Python provides a number of built-in methods for modifying lists. Below, you'll find more information on these methods. The target string was not directly modified in the previous tutorial's string methods. Strings are immutable, so this is why. String methods, on the other hand, give you back a completely rewritten string object. They don't change the target string at all:
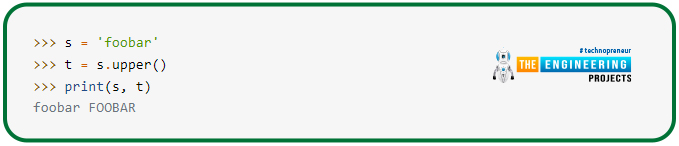
List methods differ from other approaches. Lists are changeable, therefore the target list gets modified while the list method is running.
Append()
Adds a new item to the end of a collection.

List functions change the target list on the fly. They don't give you a new one:
extend()
Adds items from an iterable to a list.
Yes, it's most likely what you're expecting. Additionally, an iterable is required as an argument to extend(). iterable> elements are inserted one at a time:
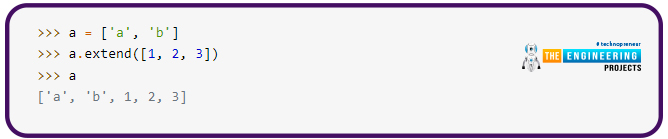
To put it another way, extend() functions similarly to the plus sign (+). Because it alters the list while it's still in place, it's equivalent to the += operator:
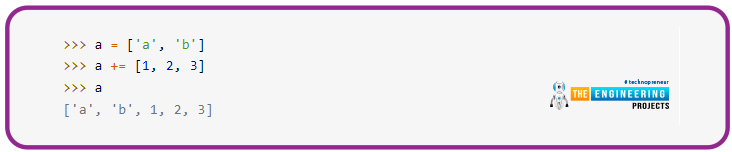
insert()
A new element is added to a collection with the help of this method. Object obj> is inserted into the list an at the index indicated by insert(index>, obj>). It's a[index>] obj, and the remaining list items are moved rightward after the function call.
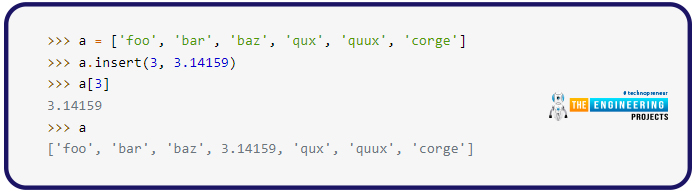
remove()
In a list, this function removes one item. remove(<obj>) list an is cleared of obj. An exception is thrown if obj> is not in a:
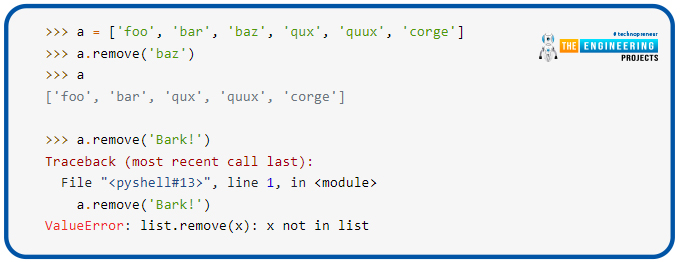
pop(index=-1)
In a list, this function removes one item. There are two key differences between this method and remove():
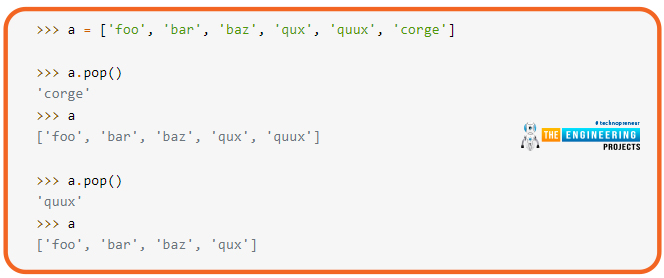
- Instead of removing the actual object, you specify its index.
- It returns a value: the item that was deleted.
The last item in the list is simply removed by calling pop():
Specifying an index in the optional index parameter causes this command to remove and return the given item. Like string and list indexing, index can be negative.
Are lists dynamic?
Python lists are described in this course by a set of six qualities. Finally, lists can be reordered. Sections above have shown many instances of this. A list expands as new things are added:
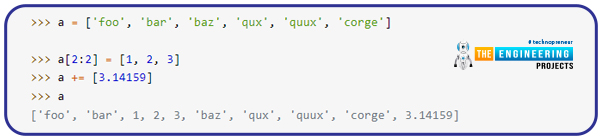
Similarly, as things are removed from a list, the list gets smaller.
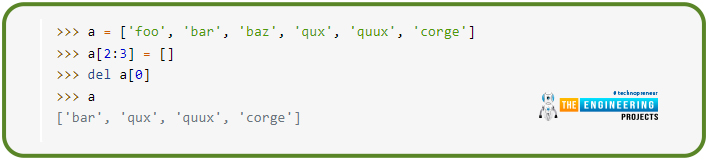
Python Tuples
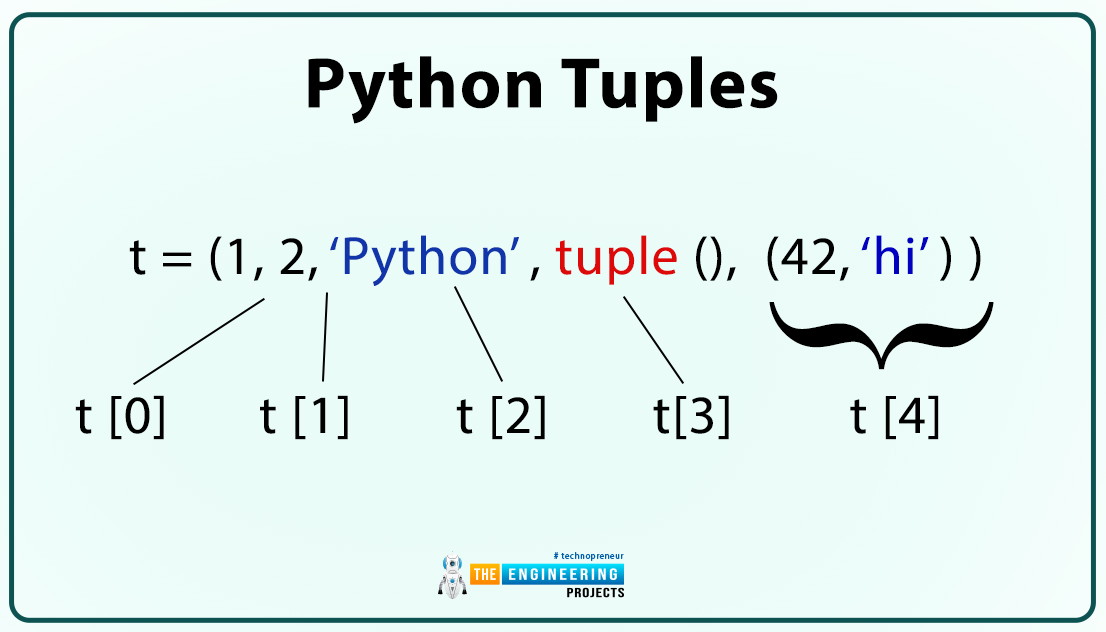
A tuple is a collection of things that are arranged in a specific order. When it comes to the pronunciation of a word or phrase, it depends on who you ask. A few people say it as if it were spelled "too-ple," while others pronounce it as "tup-ple," which rhymes with "supple." Because everyone I know pronounces "supple," "quintuple," "sextuple," and "octuple" as though they rhyme with "supple," my preference is for the latter.
Defining and Using Tuples
Lists and tuples are nearly identical, with the exception of the following characteristics:
- When creating a tuple, the elements are enclosed in parentheses (()) rather than square brackets (). ([]).
- Tuples cannot be changed.
As an illustration of tuples in action, consider the following code sample:
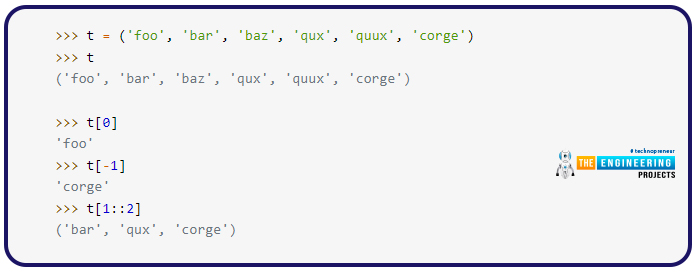
What mechanism for tuples relate to that of lists?
There's no need to worry! Reversing a tuple is as simple as using our usual string and list reversal process:

It's important to remember that although though tuples are constructed using parenthesis, you still use square brackets to index and slice them.
A tuple is a list with the same properties as a list: it's ordered, it can include arbitrary objects; it's indexable and sliceable; and it can be nestable like any other list. However, they cannot be changed:
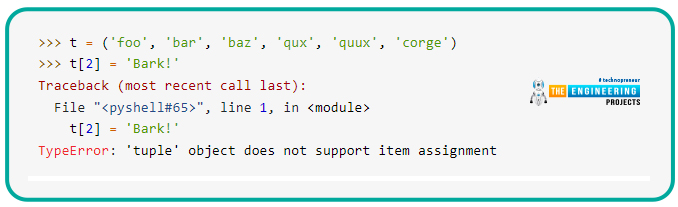
Why would you choose tuples over lists?
- A tuple is faster to manipulate than a list in terms of program execution. Assuming the list or tuple isn't too large, you may not notice this.
- Data modification is not always desired. It is safer to use a tuple rather than a list when the values in the collection are supposed to be constant throughout the program.
- Another Python data type, known as a dictionary, requires an immutable value as one of its components. This can be accomplished with a tuple, but not with a list.

There is a way to display the values of several objects at once in a Python REPL session by simply inserting them one after the other between commas:

Because Python interprets the input as a tuple, it presents the response in parentheses. The definition of a tuple has a peculiarity that you should know about. It's impossible to be vague when creating a tuple that has no items or a tuple with two or more. A tuple is defined in Python:

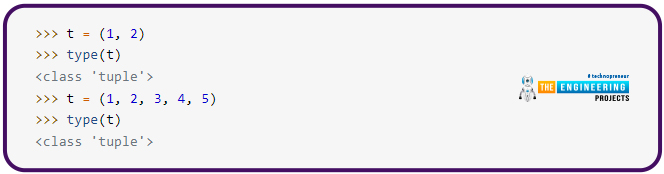
Is it possible to create a tuple with just one item?

Because parentheses are used to denote operator precedence in expressions, the expression (2) creates an int object. Before closing parentheses, you need to put in an extra comma (,): This tells Python that you plan to create a single tuple.
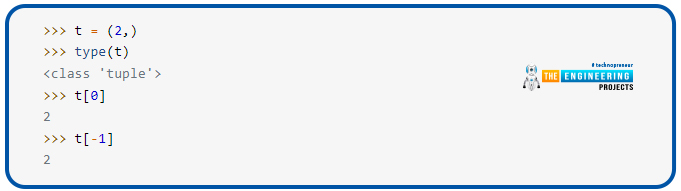
There has to be a mechanism to define a singleton tuple, even if you don't need to do it very often.
Using Python, you can display a singleton tuple by putting a comma in front of it:

Packing, assigning and unpacking of the tuples
You've seen this before: a literal tuple can be allocated to a single object.

When this happens, it's as if the tuple's contents have been "stuffed" into the object:
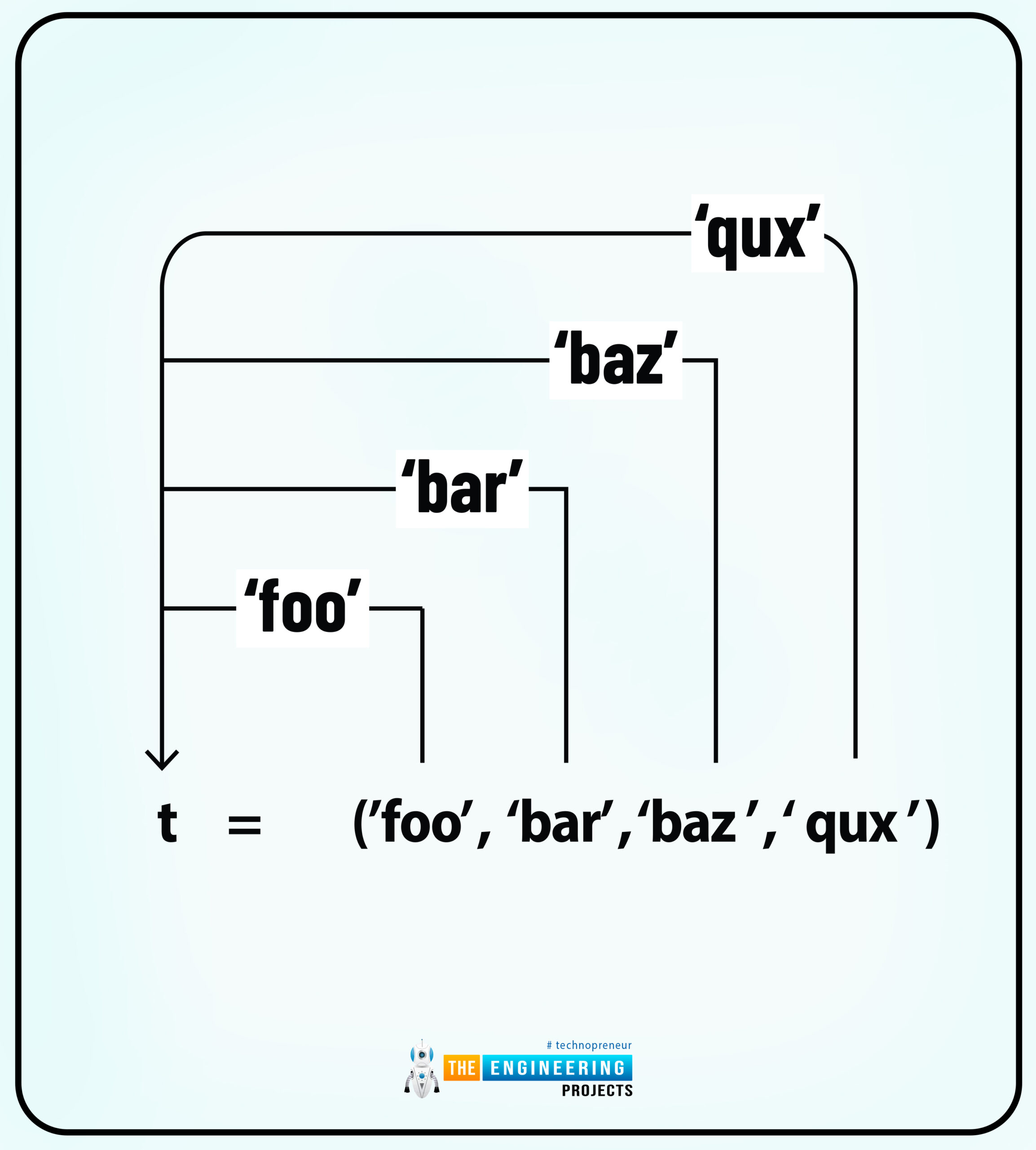
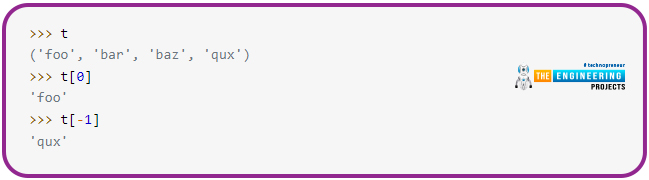
"packed" objects can be "unpacked" into a new tuple by assigning them to the new tuple's objects.
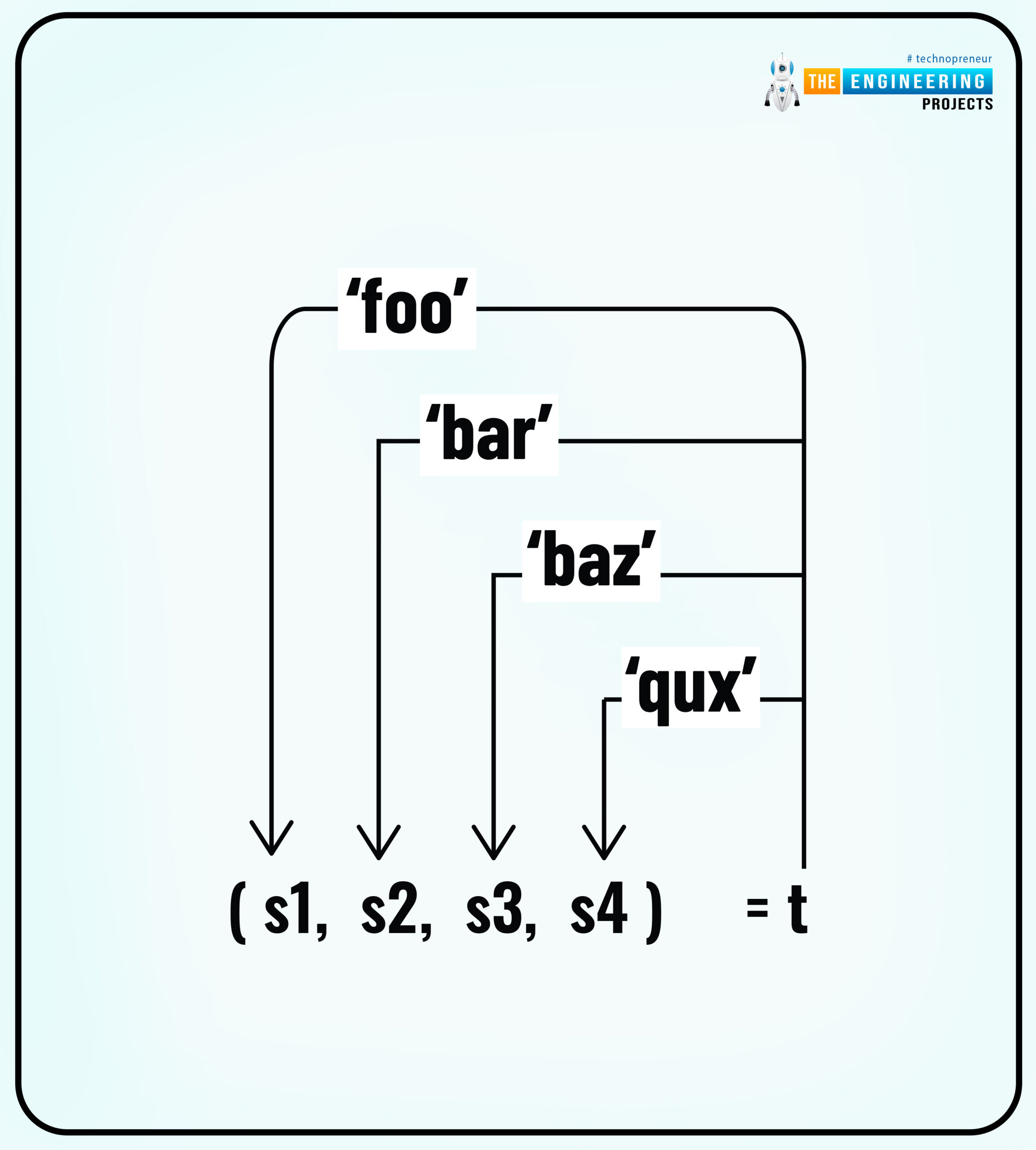
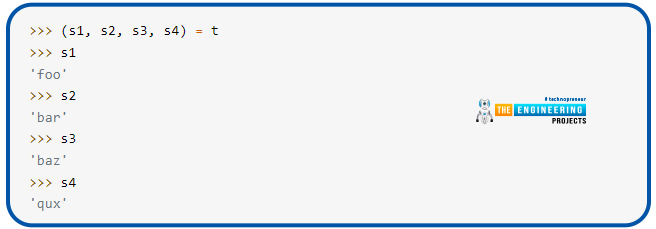
Otherwise, a problem will emerge when unpacking a tuple: if there are more variables than values, an error will occur.
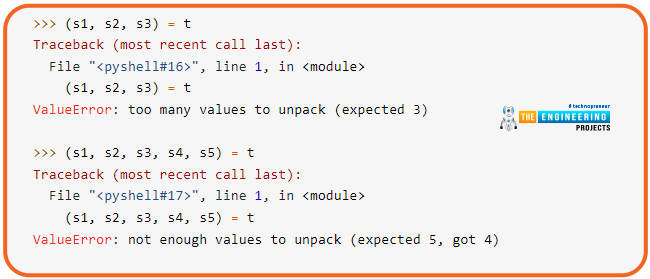
Tuple compound assignment
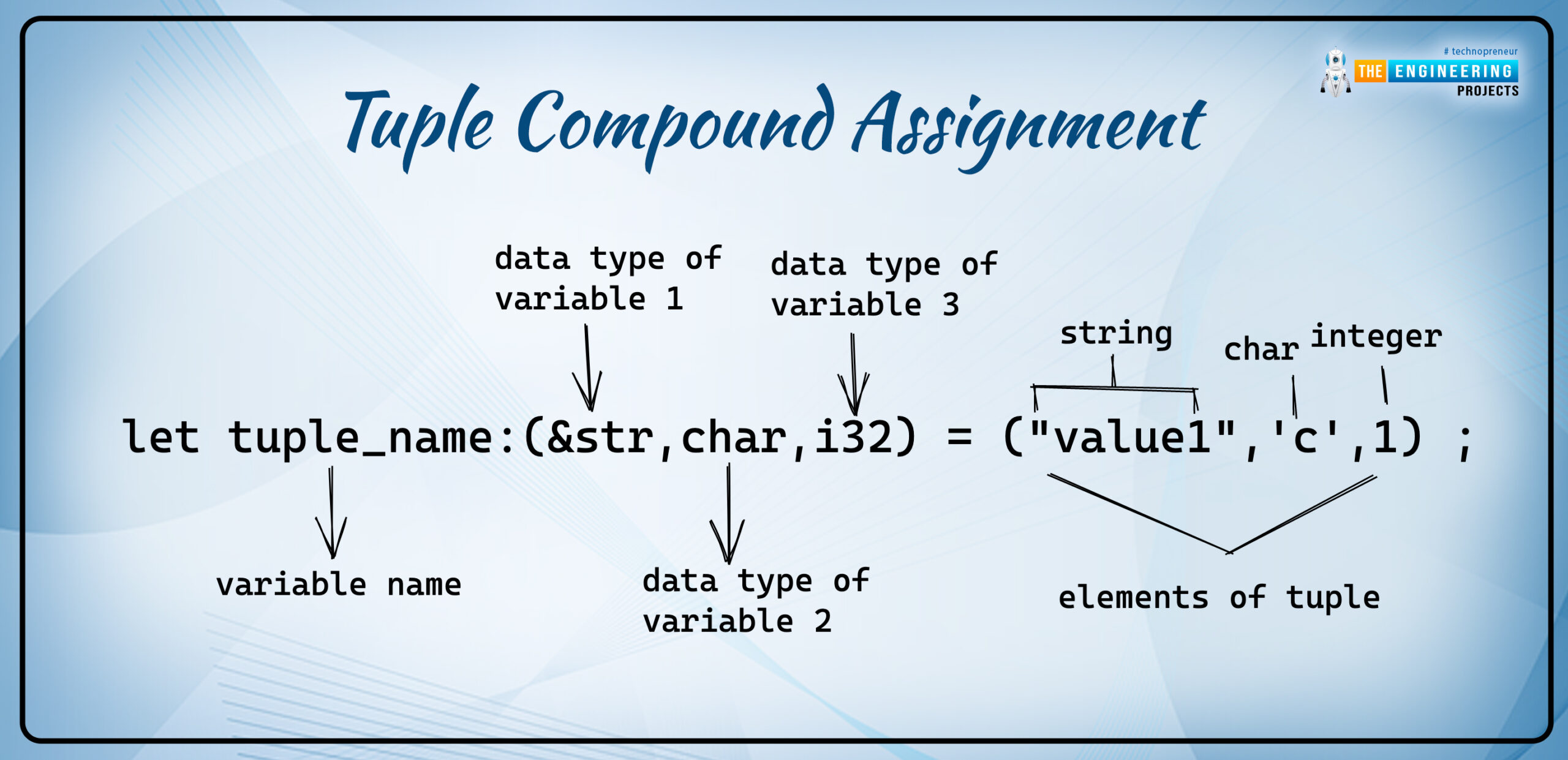
Compound assignments can be created by combining the steps of packing and unpacking into a single expression.
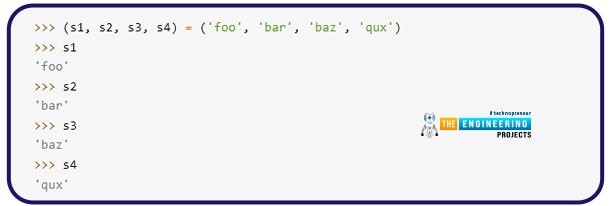
It's important to remember that in this tuple, the components on the left and right must be equal.
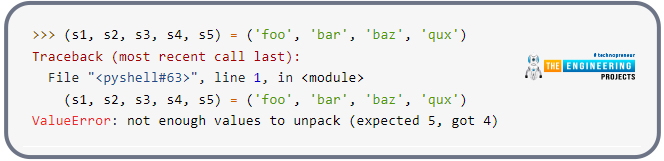
It is possible to leave off the parentheses required to denote a tuple in Python assignments like this one and a few others:
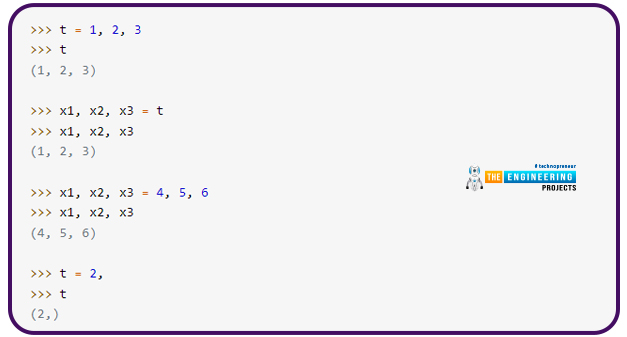
If you're unsure whether or not the parentheses are necessary, go ahead and put them in if you have any doubt. Python idioms are made possible by multiple assignment. As a programmer, it is common to have two variables whose values must be swapped. While the swap is taking place, a temporary variable must be used to store one of the values.
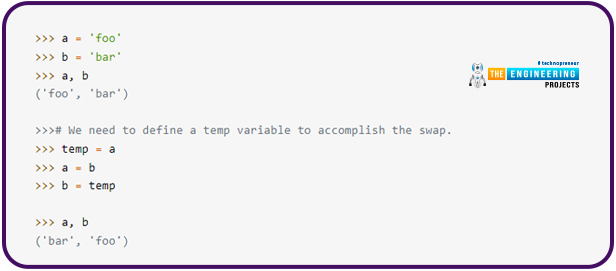
Swapping in tuples
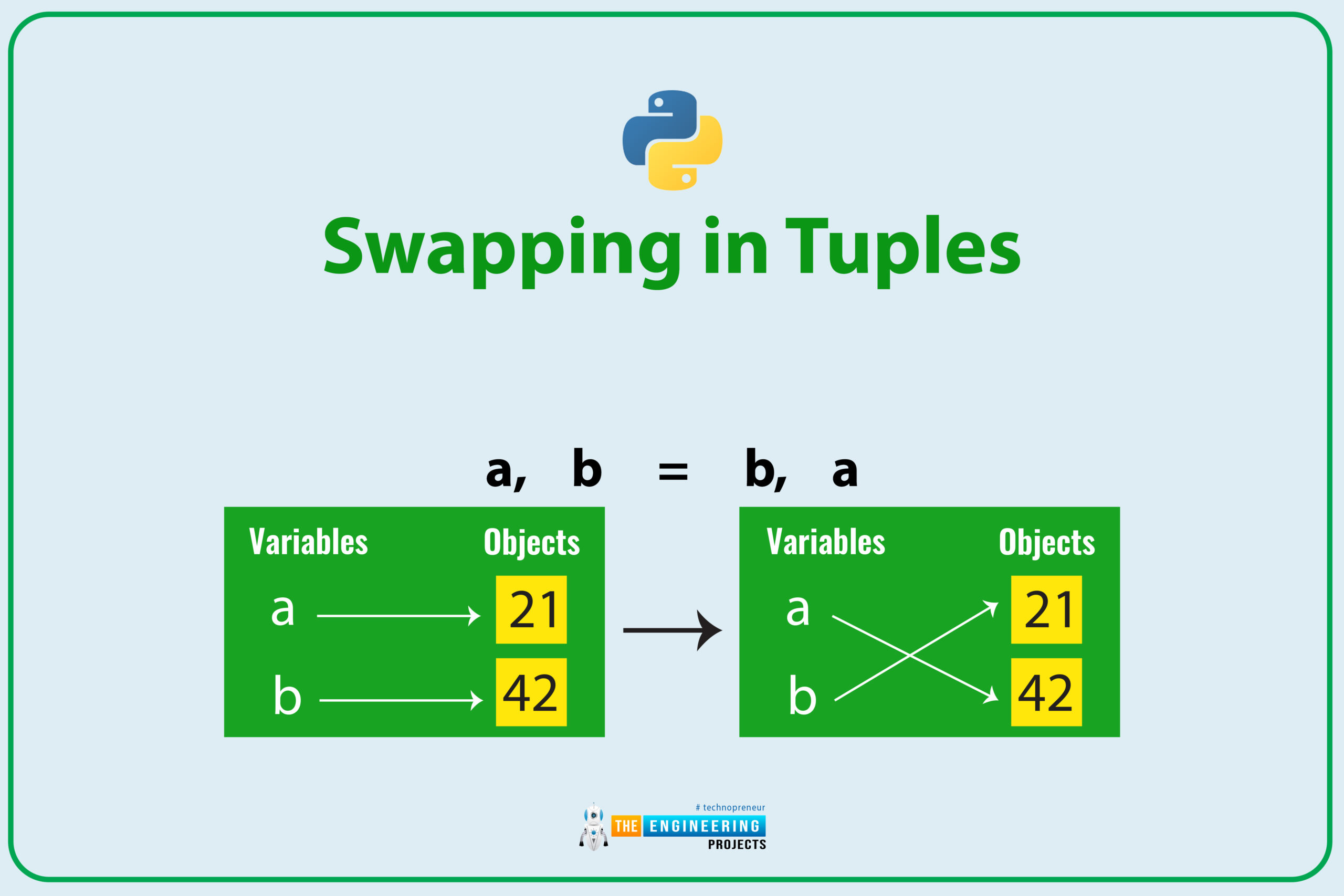
In Python, a simple tuple assignment is all that is needed to perform a swap:
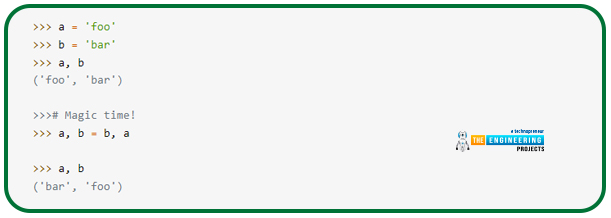
If you have ever used a Python temporary variable to exchange values, this is the pinnacle of modern technology. It's the greatest it's ever going to be.
Conclusion
Congratulations! You have now completed the list and tuple tutorial. Python lists and tuples were introduced, along with some of their basic features and operations. In Python, you'll be relying on these all the time. It is a list's primary property that it is organized. It is impossible to modify the order of a list's elements, unless, of course, the list is altered. The same is true for tuples, except that they can't be updated. Python's conditional statements will be covered in the upcoming lesson.