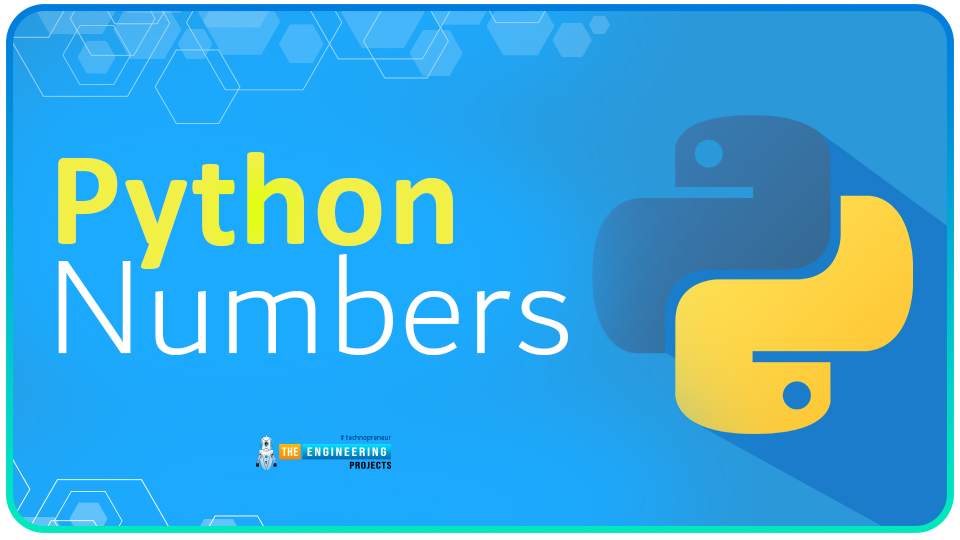
What you'll learn in this tutorial is how to:
- Add, subtract, multiply, and divide numbers.
- Work with modular.
- Use exponents.
- Use expressions.
- Use a predetermined number of decimal places to round numbers
- Use strings to format and show numeric data.
With this in mind, let`s start.
How are integers created?
Integers can be created by simply inputting a number. For example, the tutorial variable is assigned the integer 6 in the following way:
>>>Tutorial = 6
In this case, the integer literal is 6 since it is written into the code exactly as it appears. Using int () and str (), you can turn a string containing an integer into a number (). Commas or decimal points are commonly used to separate digits in huge quantities written by hand. When compared to 1000000, the value 1,000,000 is easier for reading. Use underscores (_) instead of the commas (,) if you want to separate digits in an integer literal. Integer literals of one million can be expressed in one of the following ways:

There is no limit to the size of an integer, which may seem unexpected given that computers have a finite quantity of storage. Atom`s interactive window may be used to enter in the largest number you can think of and Python will be able to run it with no problem.
Floating-Point Numbers
Numbers having decimal places are called floating-point numbers. -1.75 is a floating-point number, just like 1.0. float is the name of the data type for floating-point numbers:
>>> type (1.0)
<class 'float'>
A floating-point literal or a text converted to a float using float () may be used to construct floats, much like integers.

It is possible to express a floating-point literal in any one of three ways. There are various ways to construct a float literal with a value of one million.
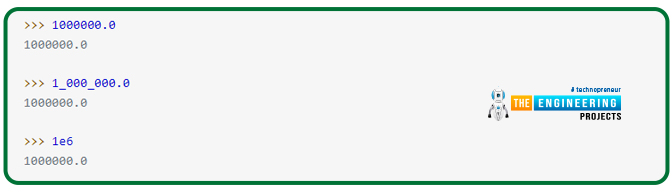
To produce integer literals, you can utilize the first two techniques. An E notation float literal is also used in the third technique.
Exponential notation - what is it?
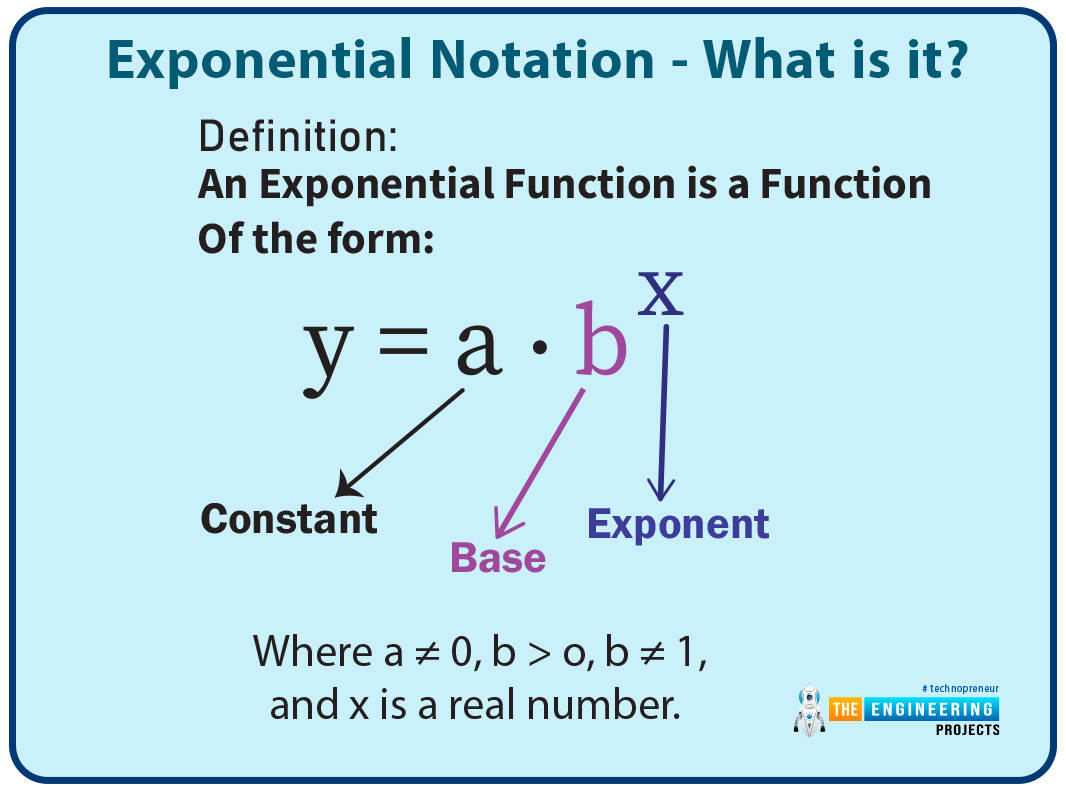
Numerical values that might otherwise result in a lengthy string of digits in decimal form can be expressed using the E notation.
You can use E notation to write a floating-point literal by starting with an integer and ending with a value. The number before e is multiplied by 10 raised to power the value that is after e. This means that 1e6 is comparable to 1×106.
Displaying very big floating-point integers with E notation is also possible in Python.

It is true that floats have a maximum size, unlike integers. Your system's maximum floating-point number will vary, but a value like 2e400 should be much beyond the capability of the majority of PCs. 2e400 is equal to 2×104°°, which is a staggeringly large digit!
When you get close to maximum allowed float value, the specialized float inf is returned by Python.

The symbol "inf" represents infinity, and it simply indicates that the number you're attempting to compute exceeds the computer's maximum supported floating-point value. Inf is still a float type:

A negative floating-point value that exceeds your computer's minimum floating-point number is represented by the -inf keyword in Python.

If you're a coder, you're unlikely to see inf and -inf unless you deal with exceedingly high numbers.
Let's see whether we grasp numbers correctly.
Exercise 1: Create two variables, num1 and num2, by writing a python program. Integer literals 25000000 should be allocated to both num1 and num2, one written with underscores and the other without. Two distinct lines should be used to print num1 and num2.
Mathematical expressions and operators
In this session, Math operations such as multiplication, subtraction, addition and division will be covered. We'll also pick up a few coding standards for expressing mathematical ideas.
PEP 8 Recommendations
Anywhere you can, keep your whitespace free of trailing spaces. A backslash, space, and newline do not constitute a line continuation indication because they are both hidden. Pre-commit hooks in many projects including CPython itself reject it, and some editors do not save it.
Assigning (=), augmenting (+=, -=, etc.), comparing (==,!=, >, =, >=), Booleans, and comparison operators (is, isn't, is, isn't), as well as any other binary operators, should always be enclosed in a single space on either side (and, or, not).
If operators of the lowest priority are used, consider separating them with whitespace. Each binary operator should have precisely the same number of whitespaces on either side.
Addition
The + operator is used to perform addition operations:
>>> 1 + 2
"Operands" refers to the values placed before and after the plus sign (+). This example uses two integers, but the type of operands does not have to be the same.
Adding an int to a float is as simple as this:

The sum of 1.0 and 2 is a float, as shown is 3.0. When a float is multiplied by another float, the output is always another float. It is always an int when two integers are added together.
PEP 8 proposes using a space to separate the operands of an operator.
Despite the fact that Python is capable of evaluating 1+1, many programmers prefer the more readable 1+1. All of the operators in this section should follow this general rule of thumb.
Subtraction
To perform subtraction, you need to use the - operator between the integers.

An int is always produced when two integers are subtracted. The outcome is always a float when one of the operands is a float.
To express negative values, the - operator can be used as follows:
>>> -3
Output: -3
Even though it may appear to be strange, subtracting a negative from another number is possible.
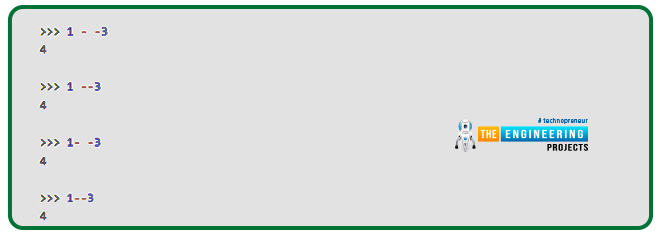
The first of the four instances are the most in line with PEP 8. It's possible to make it extra clear that the second - is altering 3 by placing parenthesis around it.

In order to make your code clearer, it is recommended that you utilize parenthesis. Despite the fact that computers are able to run code, humans are only able to read it. Your code should be as readable and understandable as possible.
Multiplication
Use the * operator to multiply two numbers:
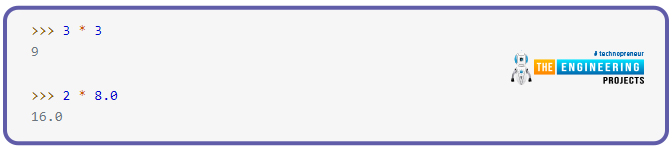
There are no exceptions to this rule when it comes to multiplication. When you multiply two integers, you get an int, and when you multiply a float by a number, you get a float.
Division
When two numbers are to be divided, the / operator is used:
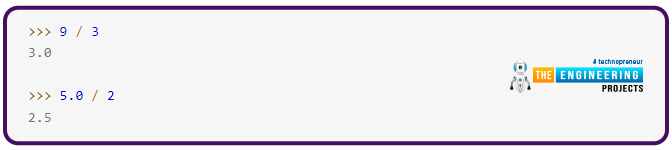
The / operator always returns a float, unlike addition, subtraction, and multiplication, which yield integers. You may use int () to ensure that the result of dividing two numbers is an integer:

It is important to note that the int () function discards any fractional parts of the number

The floating-point number 2.5 is obtained by dividing 5.0 by 2, while the integer 2 is obtained by subtracting .5 from the result of int (2.5).
Floor Division operator
The operator (//), often known as the floor division operator, can be used instead of the cumbersome int (5.0 / 2):
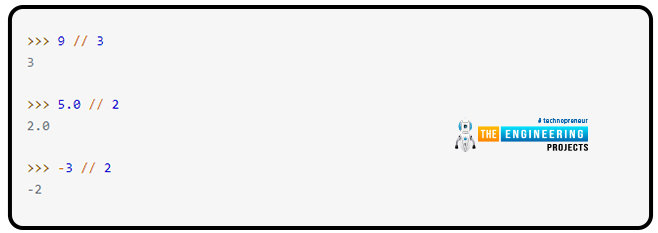
First, the / operator splits the numbers on each side of it, and then rounds down to an integer. When one of the values is negative, you may not get the expected result.
For instance, -3 / 2 produces -2. The first step is to divide -3 by 2 to get -1.5. Then -2 is rounded to the nearest -2. -1.5. 3 / 2 returns 1, on the other hand, since both values are positive.
A float number is returned if one operand is a float value, as seen in the preceding example. And that's why 9// 3, and 5.0// 2, respectively, return the integers 3 and 2.0, respectively.
When you divide a number by 0, the following happens:
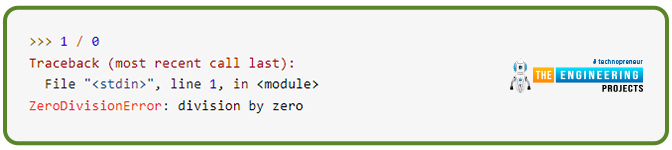
If Python encounters a ZeroDivisionError, it warns you that you've just attempted to violate a universal law.
Exponents
When you use the ** operator, you can multiply an integer by a power.
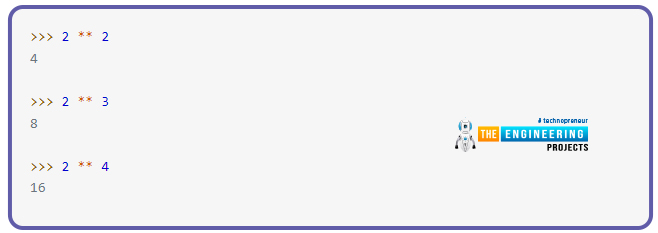
Integers are not required to be used as exponents. Floats are another option:
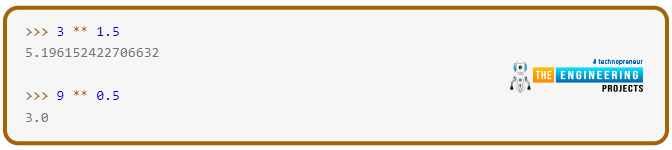
Raising a number to power 0.5 implies taking the square root of that number. The square root of nine is a float, thus even though nine`s data type is an int, Python generates a float 3.0. If both operands of an expression are integers, the ** operator returns an integer and if one of the operands is floating-point number it returns a float.
It's also possible to raise numbers to negative powers like shown below:
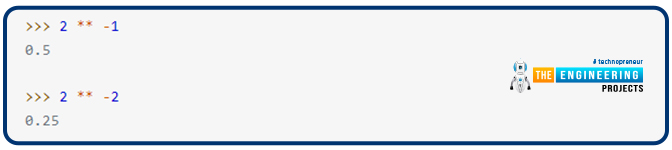
If you divide a number raised to a positive power by 1, then you have raised the number to the negative power. As a result, 2 ** -1 equals 1 / (2 ** 1), which is equal to 1 / 2 or 0.5. It's easy to see how 2 ** -2 may be represented as 1 / (2 ** 2), 1 / 4 or 0.25.
Using the Modulus Operator
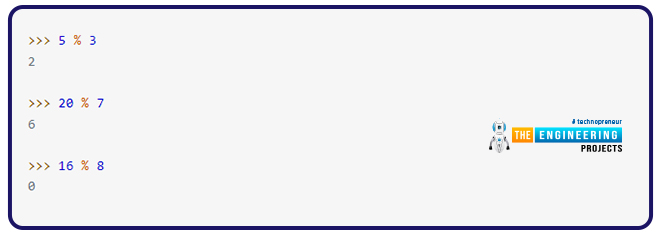
5% of 3 is 2, so 3 divides 5 once and gives a remainder of 2. Seven also divides 20 twice, leaving 6 as a remainder. In the previous example, 16 divided by 8 is 0, thus 16 % 8 equals 0. The outcome of dividing the value to the right of % by the number to the left is always 0.
Determining the divisibility of two numbers is a typical use of percent. Number n, for example, is only an odd number when n % 2 is zero. What do you suppose is returned by 1% 0? Let's give this a try.
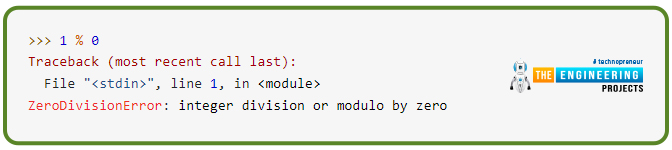
When you divide one by zero, you get 1 which is the remainder of 1 % 0. Nevertheless, Python throws a ZeroDivisionError since it is impossible to divide 1 by 0.
An error like ZeroDivisionError has little impact on your project in interactive window of IDLE. Even if a prompt window will pop up, you can continue to write code in your editor until the error is fixed.
The execution of a script is halted if Python discovers an error while running it. The software crashes in other words.
When you use the percent operator with negative values, things get a little more complicated:
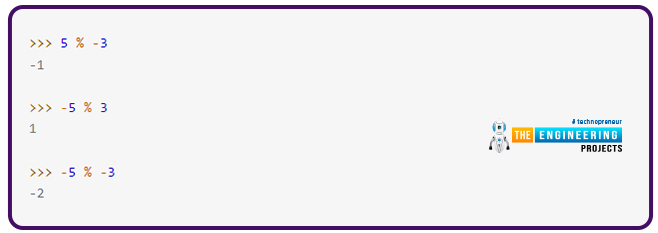
Python has a well-defined behavior that produces these outcomes, even if they appear surprising at first. To find an integer's residual after multiplying it by another number, Python utilizes the formula r = x- (y * (x / y)).
Arithmetic Expressions
Complex expressions can be created by combining operators in new and interesting ways. Python can calculate or evaluate an expression to yield a result made up of integers, operators, and parentheses.
An example of an arithmetic expression is shown below.
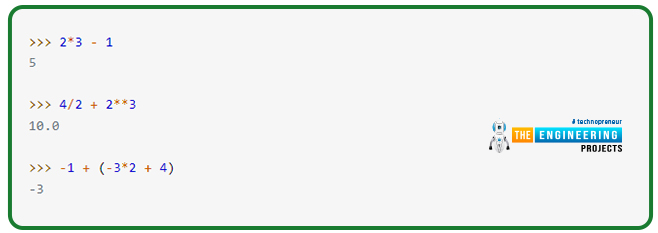
Evaluating expressions follows the same set of guidelines as performing standard arithmetic operations. These rules were presumably taught to you as the sequence of operations in school.
Among the operators that have equal precedence are these operators "*," "/," "%," and "%". Thus, 2*3 - 1 yields 5, rather than 4, when divided by 3. Because the * operator takes precedence over the - operator, 2*3 is evaluated first.
In the above example, you may have noticed that the requirement requiring a space before and after each operator was not followed. Whitespace in complex expressions is addressed in PEP 8:
If the operators with the lowest priority are being used, then whitespace should be added around them. Using a single space and the equal number of whitespaces on both sides of a binary operator is perfectly acceptable.
Even if parenthesis isn’t essential, it's always good to include them to indicate the order in which steps should be taken.
Conclusion
In this lesson, you've learned how to use Python's numbers with expressions. In the next chapter, you'll learn how to employ math functions and number techniques and learn how to avoid typical mistakes that might lead to program failures.