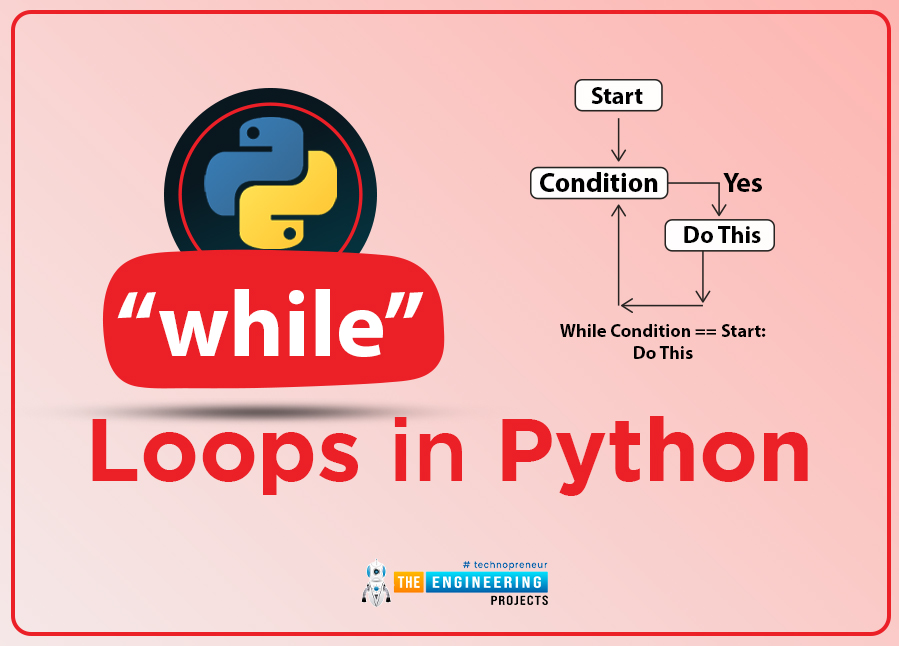
Welcome to the next lesson of our python programming class. In the last session, we looked at the use of If-else statements and created simple programs to demonstrate the concept. While loops are another type of conditional statement, and in this tutorial, we'll look at how they're used.
What will you learn?
In this article, you'll learn how to prematurely exit a while loop, a Python control structure for endless iteration. You will also learn how to write conditions in a single line to produce short and readable code.
Iteration in while loop
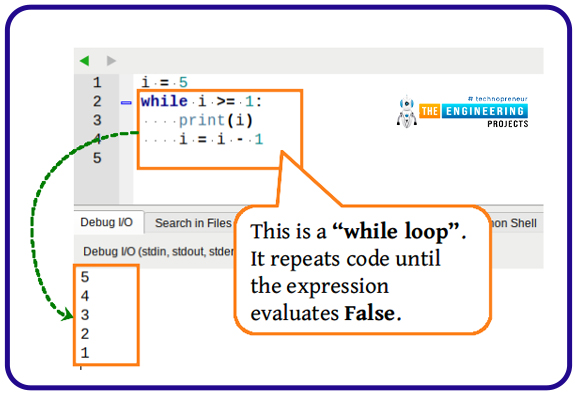
The term "iteration" refers to the practice of repeatedly running the same piece of code. A loop is a type of programming structure that implements iteration.
Both indefinite and definite iterations are recognized as valid programming constructs. Infinite iteration doesn't specify how many times the loop will be executed. As long as a condition is met, the chosen block will be executed again. An iteration that uses a definite iteration specifies how many times it will run the selected block.
What are the use cases of while loops?
- User Input: When we request user input, we must verify that the value entered is correct. We can't reasonably predict how many times the user will enter an invalid input before the software can proceed. As a result, a while loop would be ideal in this situation.
- Search: another excellent use case for a while loop is looking for an element in a data structure because we can't predict how many iterations will be required to get the target value. A while loop, for example, can be used to build the Binary Search algorithm.
- Games: A while loop can be used in a game to keep the game's primary logic running until the player loses or the game is over. A while loop is an excellent solution because we can't predict when something like this will occur.
The while Loop
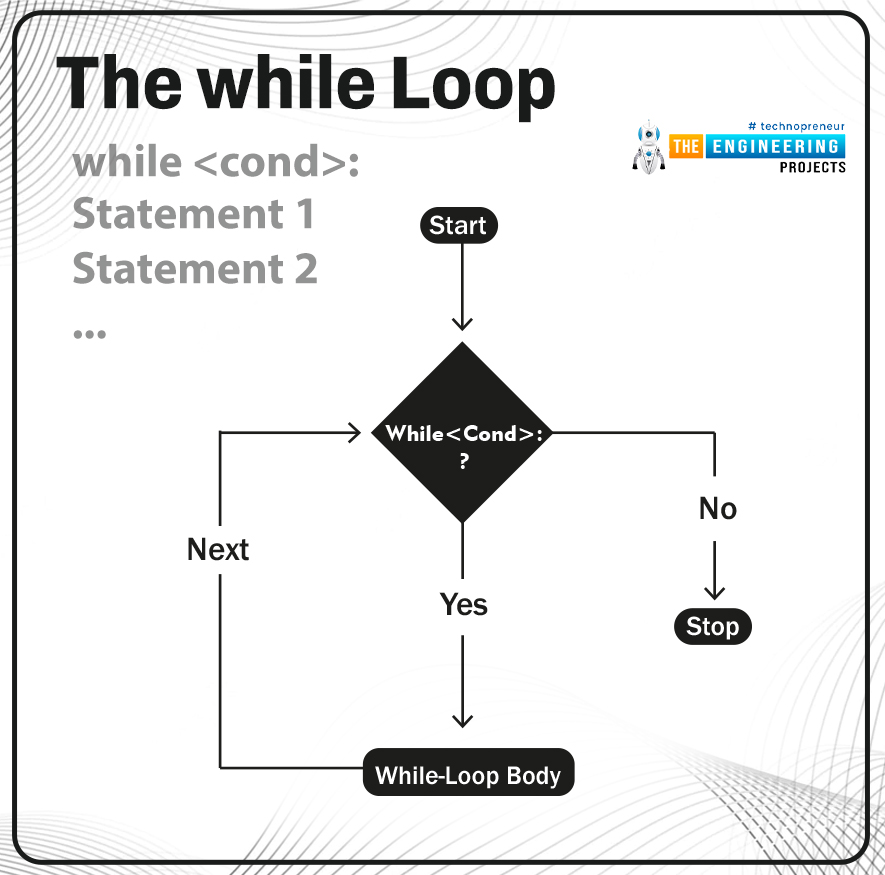
While Loops are used to iterate over the same code block for an unlimited couple of iterations until a condition is met.
While Loops are used to repeat the same code block indefinitely until a condition is met, if a given Boolean condition is completed at the end of the block, the "do while" loop will continue to run a provided block of code, or it will not.
Only one statement is required for the Do While Loop to terminate. However, the While loop may terminate with no statements executed, while the While loop may terminate with statements executed.
This type of control flow statement specifies iteration, allowing the code to be executed again and again. When we know how many times we want to run a code section, a for loop comes in handy.
Here, we'll explore how the Python while statement can be used to build loops. We'll begin with a simple design and gradually add to it.
Listed below is a basic while loop's structure:

Block denotes an execution block that will be repeated over and over again. As with an if statement, this is indicated by indentation.
Indentation is used to define blocks in all Python control structures. See the previous tutorial on grouping statements for a refresher.
In the controlling expression, expr, variables are initialized and updated during the loop's execution.
In this case, the expr parameter is evaluated first in a Boolean context. If this is true, then the loop's body is run. The body is executed when the expression returns to true. The program moves to the topmost statement following the loop body if the condition in expr is false.
Take a look at the following loop:
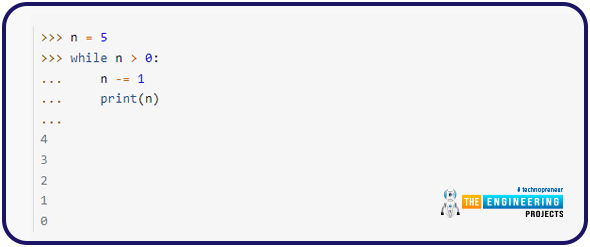
This is what we're seeing in this case. n starts as five. The loop body is run because n > 0 is true. n is greater than 0. Line 3 of the loop body prints the value of n after decreasing by 1 to 4 places.
The expression is re-evaluated from the top of the loop after the loop's body has completed. Because it's still true, the code in the body runs once again, printing 3 on the screen.
It goes on like this until n equals zero. That is when the expression is tested, and the loop is broken. The program would typically restart execution from the top most statement following the loop's body, but there isn't one here.
It's worth noting that the controlling expression of the while loop is tested first. If the loop body is false at the start, it will never be executed:

Because of this, n is set to zero when the loop is encountered. There is no need for the loop body because the controlling statement n > 0 is false.
Below is another while loop that doesn't use the numeric comparison:
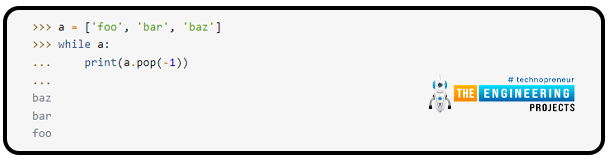
Lists evaluated in a Boolean context are either truthy or false, depending on whether or not they contain elements. As long as there are elements in it, a is true. The loop terminates when the list is empty and all items have been deleted using the pop () method.
Break and continue Python
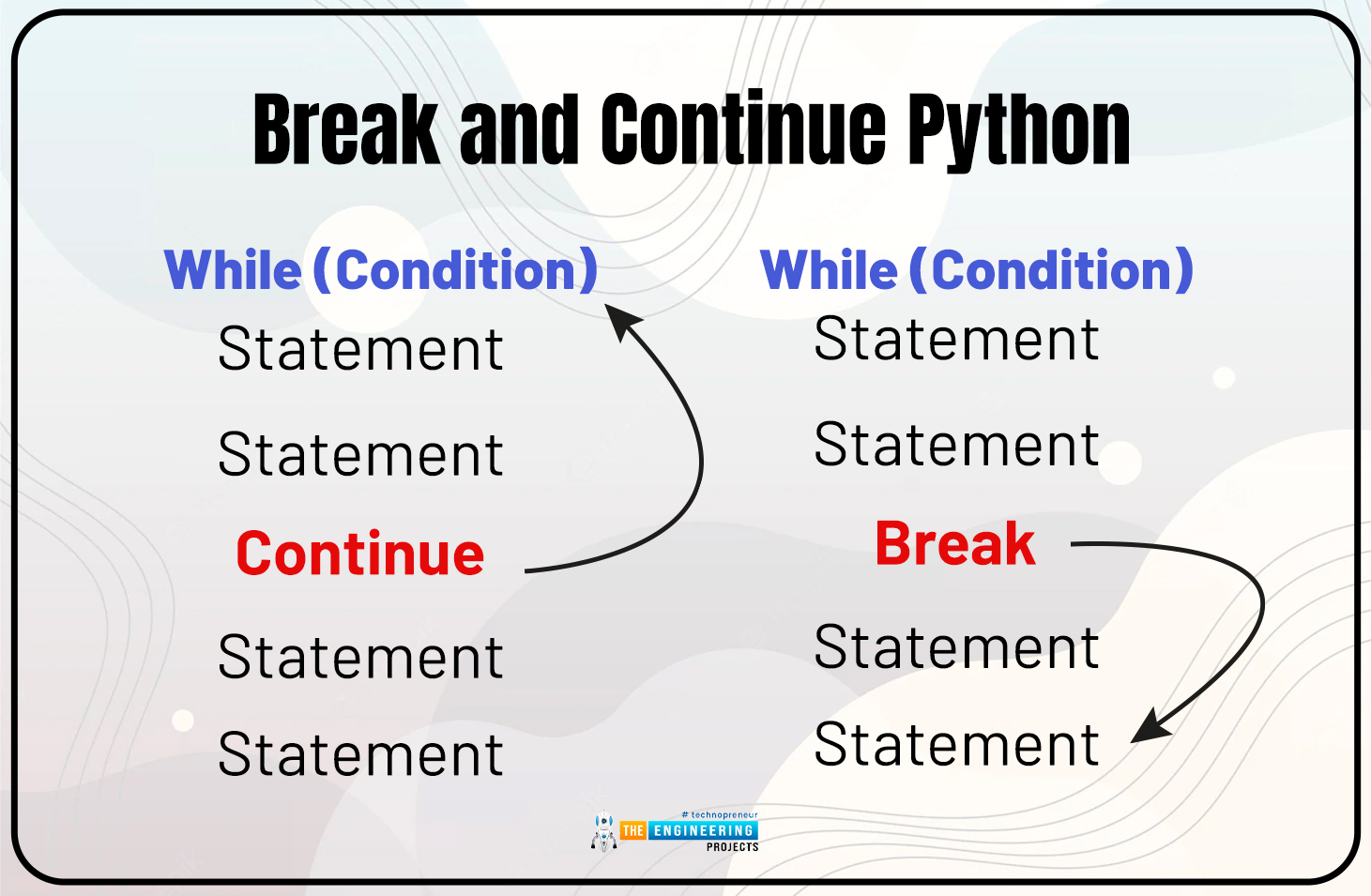
The whole body of the while loop is executed each time it is executed. Python has two keywords, break and continue, to prevent a loop from repeating itself.
This statement breaks a loop completely and immediately in Python. Python performs the next statement in the program after the loop body, which ends the current loop iteration. As soon as an expression is evaluated, it is evaluated again to see if the loop will continue or end.
The following diagram illustrates the difference between the statements "break" and "continue":
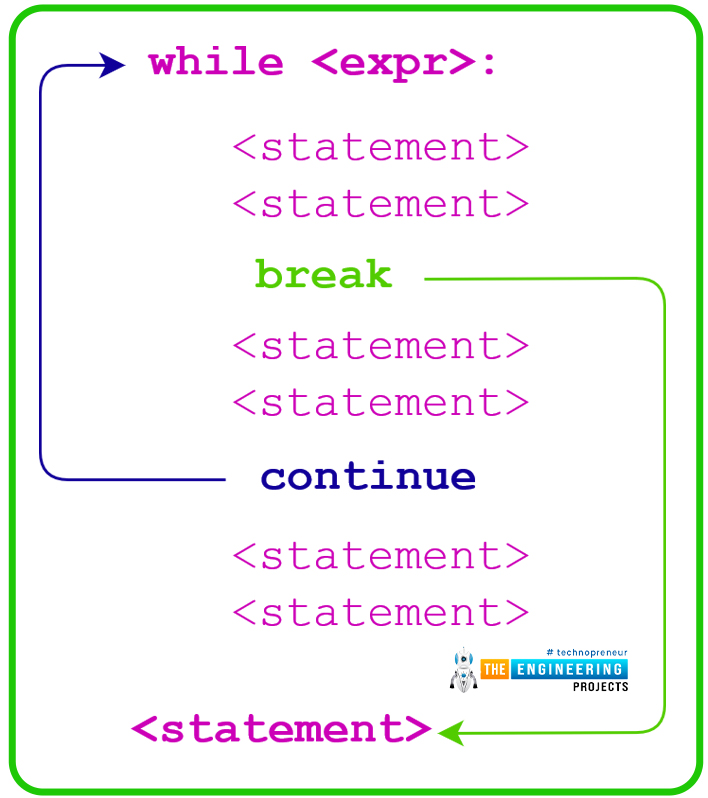
Break.py is a Python script that demonstrates the use of the 'break' statement:
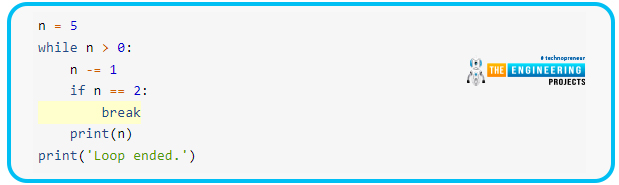
Using a command-line interpreter to run break.py yields the following results:
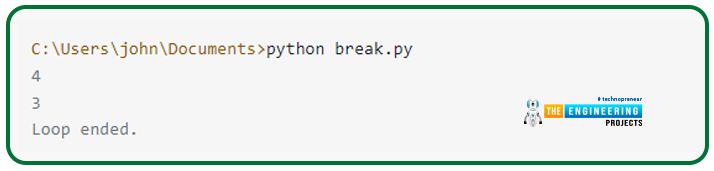
The break code is executed when n reaches the value of 2.0. Execution immediately moves to the print () call on line 7 after complete termination of the loop.
A continue statement replaces the break in the next script, continue.py.
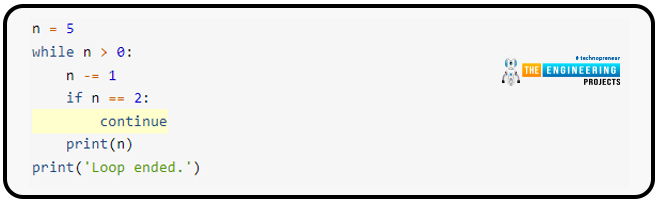
The output is something like this:
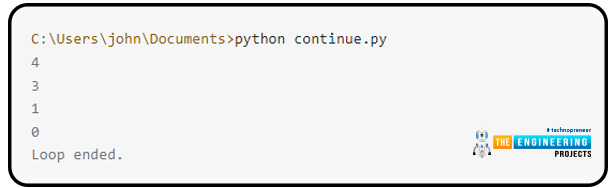
When n is 2, the loop is terminated by the continue statement. Thus, the number 2 is omitted. The condition is re-evaluated at the top of the loop, and it is still true. As before, the loop terminates when n equals 0.
Else condition
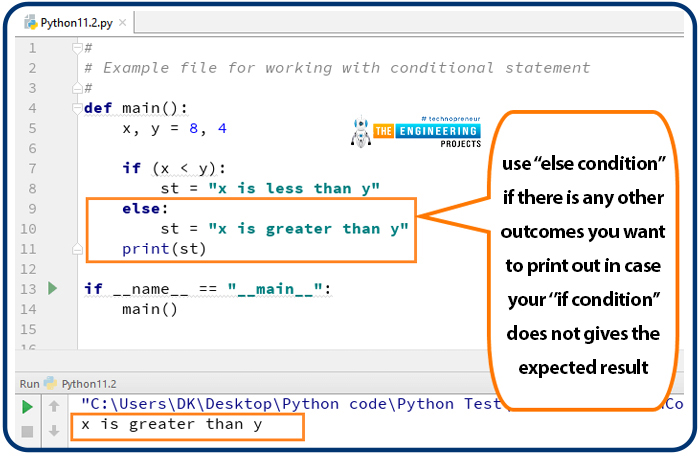
While loops in Python can have an optional else condition at the end. Unlike most other programming languages, Python has this unique functionality. Syntax in the form of a diagram:

The other clause's further statements will execute when the while loop completes, as shown in this example.
You may be wondering, "How is that useful?" at this point. After the while loop, you could insert the following statements directly after it:

What’s the difference?
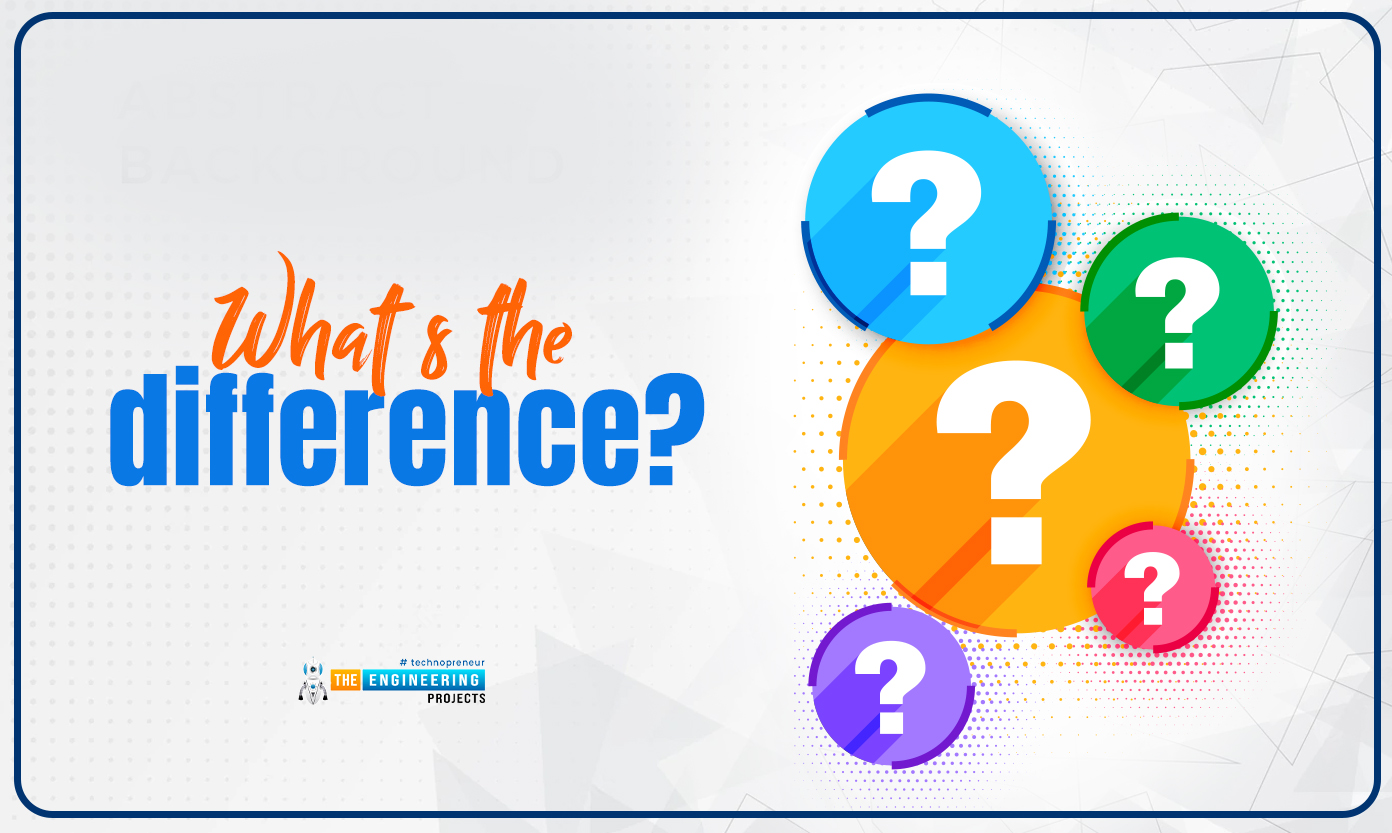
Additional statements will be run regardless of whether or not the while loop is terminated.
An else condition will only execute if the loop stops "by exhaustion"—that is until the controlling condition is false; otherwise, no subsequent statements will be executed. If a break statement is used to break the loop, the else condition is not executed.
Think about the following scenario:
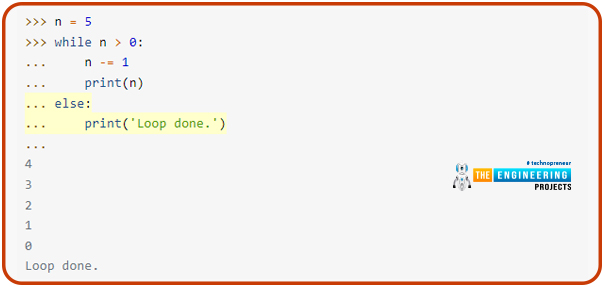
Variable n becomes 0, and therefore n > 0 became false. The loop's block runs until the condition is exhausted. The else clause is executed since the loop is allowed to run its course.
Consider the following example to see the difference:
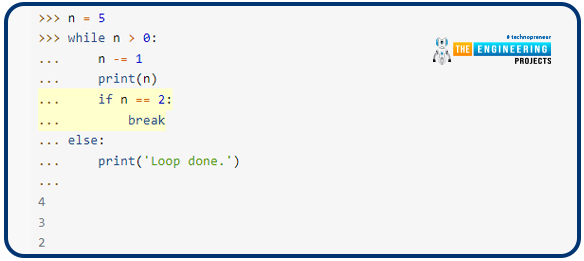
The else condition is not executed because the loop is prematurely interrupted by the break.
The term else may appear to have a different meaning in the while loop then it does in the if statement. In order to make it more understandable, you may try one of the following:
- You can see it as an "if" statement that repeats itself over and over, with the "else" clause ultimately being performed when the condition fails.
- It's best to think of everything else as if it were a break so that the following block is executed if there was no break.
You are free to disregard either of these interpretations if you don't find them helpful.
In what situations is else condition useful?
If you need to find a certain item on a list, this is a common scenario. If the item is discovered, you can use the break to leave the loop. Also, else condition can contain code to be run if the item is not found:
Infinite Loops
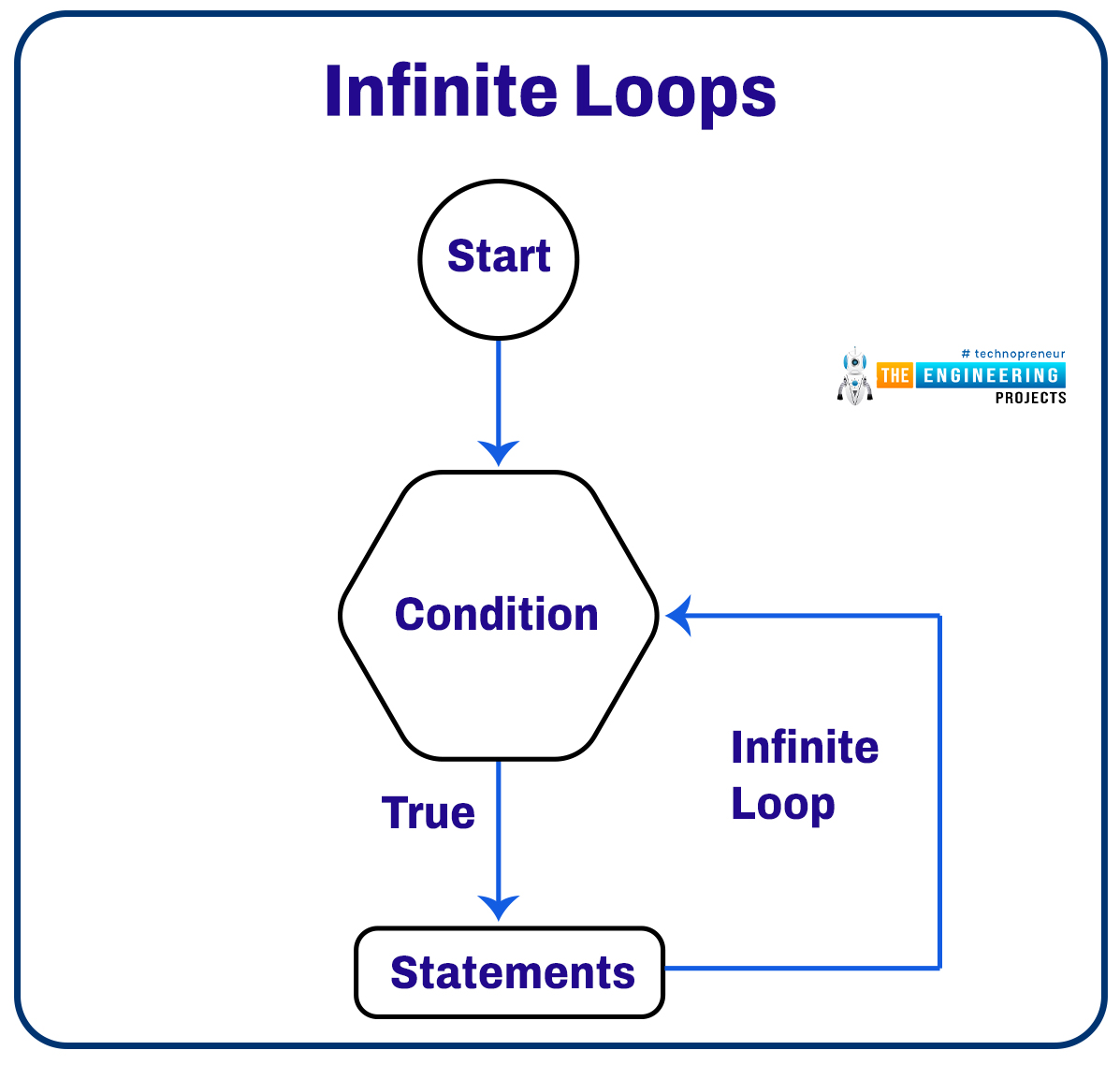
Assume you've written an infinite while loop. This may sound weird, right?
As an illustration, consider the following:
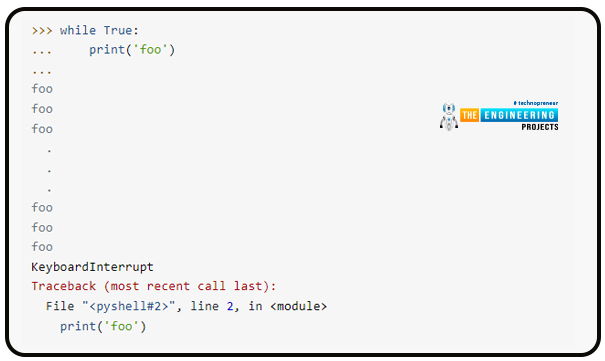
Ctrl+C, a keyboard interrupt, was used to end this code. There was no way this could have ended. The vertical ellipsis represented in this output has taken the place of several output lines that were omitted.
We're all going to be in a lot of trouble if True can ever be false. On the other hand, False creates an infinite loop that might conceivably run indefinitely.
However, this is a typical pattern, and it may not sound like something you would want to do. Code for a service that accepts service requests and operates indefinitely is a good example. In this situation, "forever" implies until you turn it off or the universe reaches the point of no return.
Alternatively, keep in mind that the break statement can be used to exit a loop. Instead of evaluating a condition at the top, conditions recognized within the loop body may make it easier to terminate the loop.
If you'd rather, you can use pop () to delete each item in the list in turn, as illustrated above:
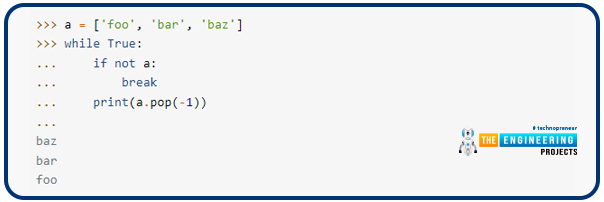
Not a is true when a is empty, and the break statement terminates the loop.
Multiple break statements can be specified in a loop:
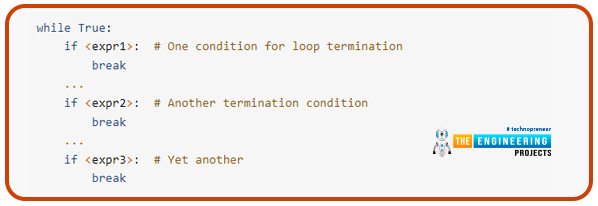
Breaking out of the loop at various points rather than trying to define every possible termination condition in the loop header is often preferable in situations like this.
Infinite loops can be convenient in some circumstances. It's important to remember that the loop must be stopped sometimes, or it will become limitless.
Nested while loops
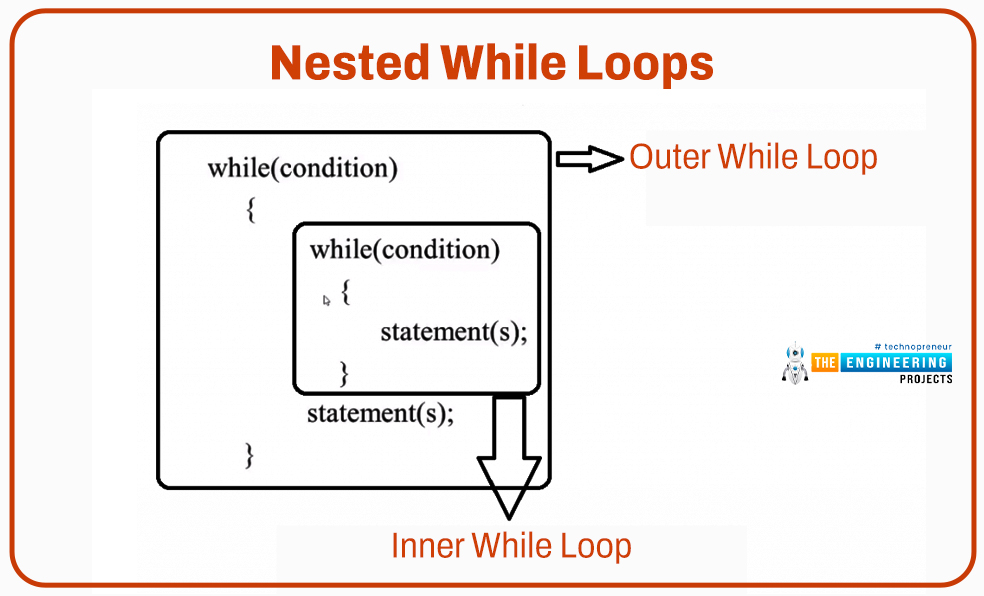
Generally, it is possible to nest Python control structures inside one another. Nested conditional statements, for example, are possible: if/elif/else.
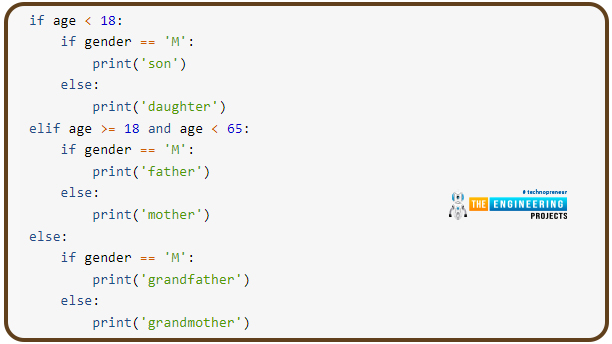
The following code shows how a while loop can be nested inside of another while loop:
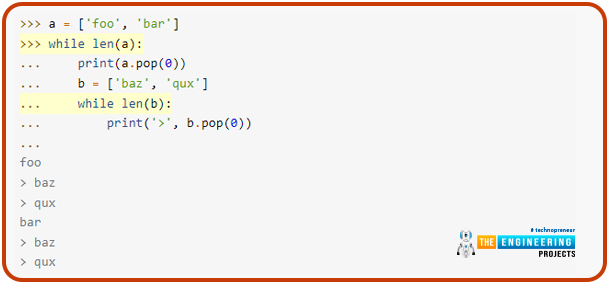
Nested loops are affected by statements like "break" or "continue" since they are nested within each other.
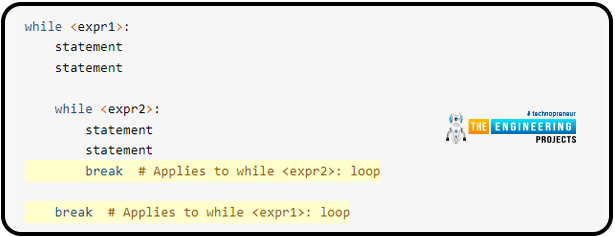
Loops can be nested in and out of the if/elif/else statements and the other way around.
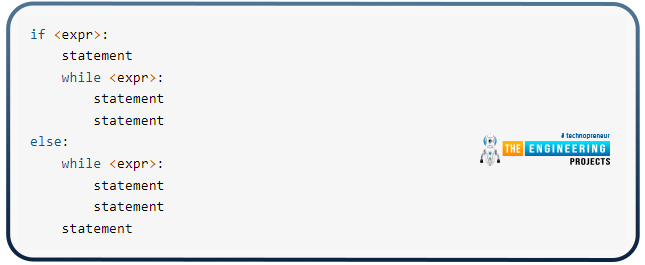
All Python control structures can be mixed and matched to your heart's content. That is precisely how it should be. You can only nest while loops a maximum of four deep, which would be unpleasant if there were unforeseen constraints like these: 'A while loop cannot be embedded within an if statement.' Trying to recall them all would be nearly impossible.
Poor programming language design is evidenced by the presence of seemingly random numerical or logical constraints. Python, fortunately, does not have any.
One-Line while Loops
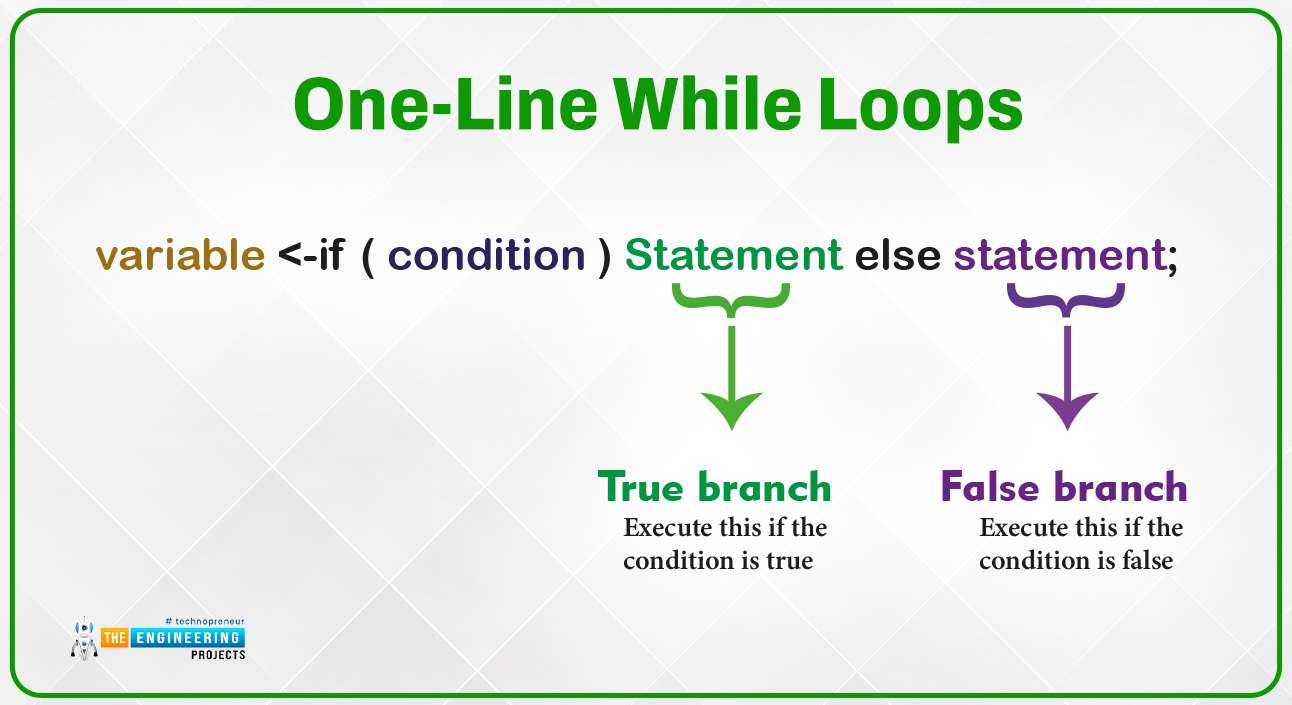
You can specify a while loop in the same way you do an if statement: on a single line. If the loop body has many statements, semicolons (;) can be used to divide them:
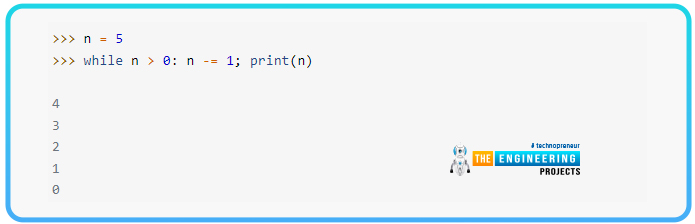
To be clear, this technique only works with simple declarative. Compound statements cannot be combined in a single line. As a result, you can write an if statement on the same line as a while loop:

You can also do this:

Note that PEP 8 forbids the use of numerous assertions on a single line. "Since this is a bad idea anyway, you generally shouldn't do it too often.
Conclusion
The Python while loop was used in this tutorial to demonstrate indefinite iteration. You can now create sophisticated and straightforward while loops, thanks to the knowledge you've gained thus far. The else clause can be used in conjunction with a while loop to handle endless loops. If you've followed along, you should understand how to run the same code repeatedly. The next lesson will cover iteration with for loops with an explicit limit on the number of iterations.