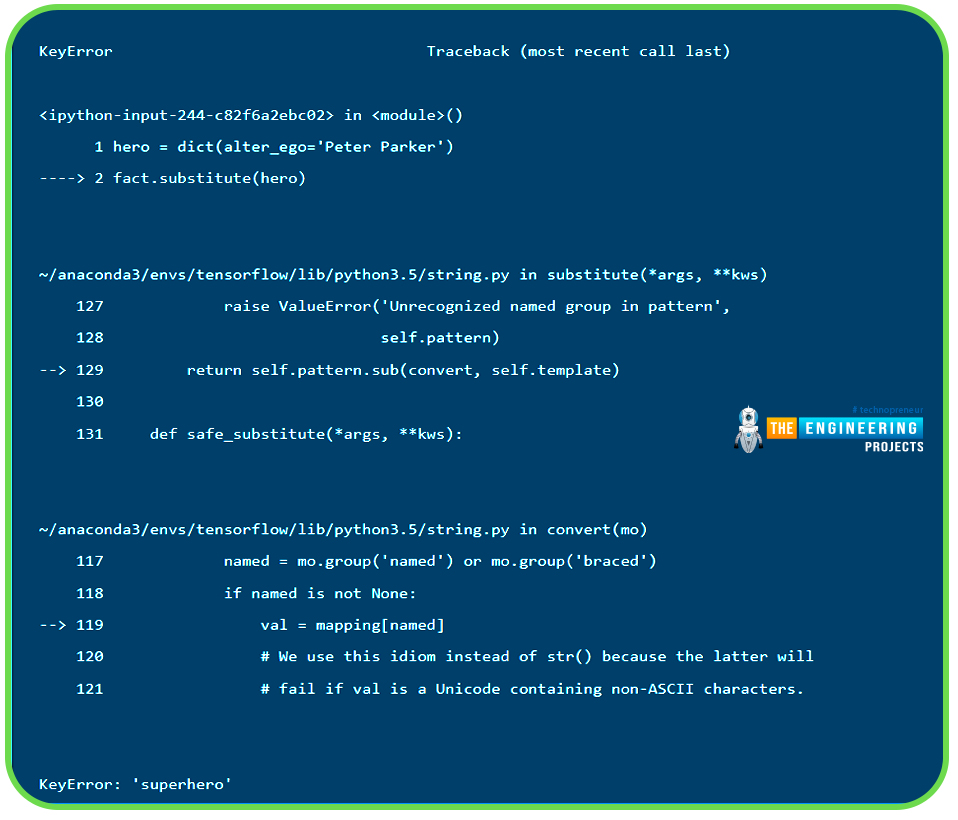
Welcome to the fourth lesson of this python course. Our previous session taught us how to utilize the print function in python, so we have a firm grasp of the terminology and the functions themselves. In this lesson, we'll cover a few more Python terms, such as:
- Strings
- Operators
- Input function
Also, we'll build a simple program to print out an imagined dog so that we may better grasp how these concepts are employed. So, let's get started now.
Why do we need to understand these terms?
Programming is a lot like building a structure out of blocks. Even with just a few types of children's toy blocks and some time and imagination, you can build anything. Because we'll be utilizing these phrases all the time in programming, it's critical that you know what they mean and how to use them.
What exactly are the strings?
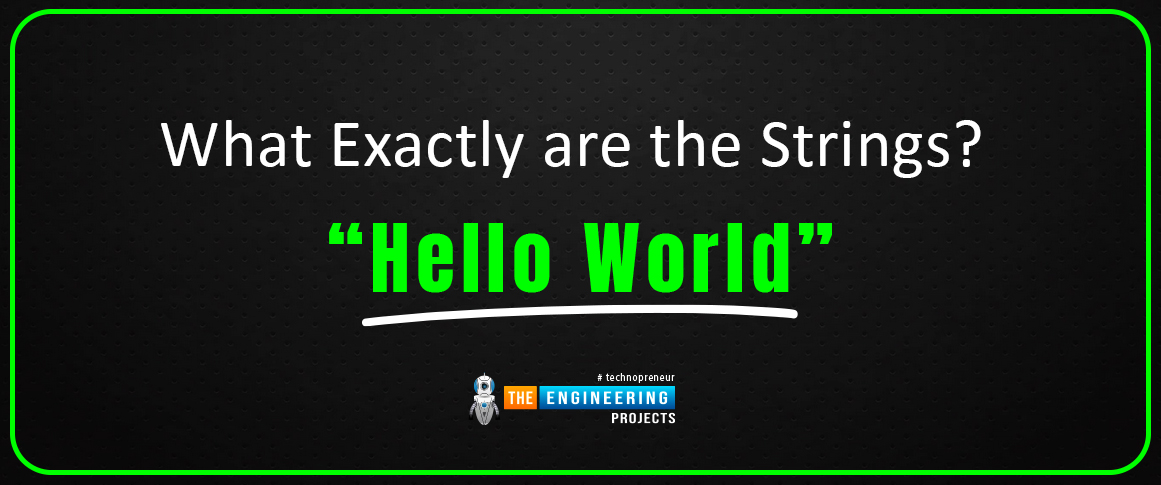
An alphabet, word, or other character collection is referred to as a "string." As one of the most fundamental data structures, it serves as a framework for manipulating data. An in-built string class called "str" is available in Python. After they've been produced, strings are "immutable," which means that they can't be rewritten. Because of the immutability of strings, we must generate new ones each time we want to represent newly computed values.
Quotes are used to denote a string. There are a variety of ways to accomplish this:
- Single quotation marks, as in the following example: For "double" quotations in your string, use "single" quotes."
- Use double quotation marks. Using single quotations in your string is easy with double-quotes.
"Double quotes allow you to embed 'single' quotes in your string."
- If you want to use triple quotes, you can do so in the following way: (""" """), (''' ''')
Triple quoted strings to make it possible to work with a set of multiple-line strings and include all of the whitespaces that accompany them.
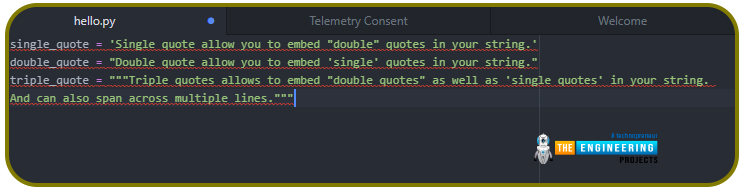
The fact that a string cannot be changed results in an error if you try to do so. The adjustments require the creation of a new string.
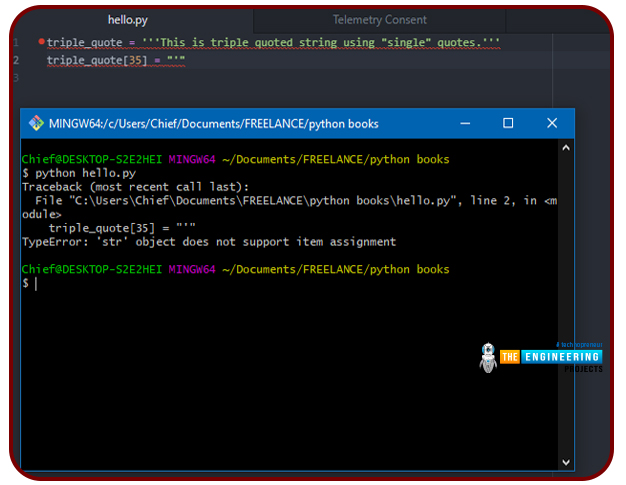
Instead, use this method.
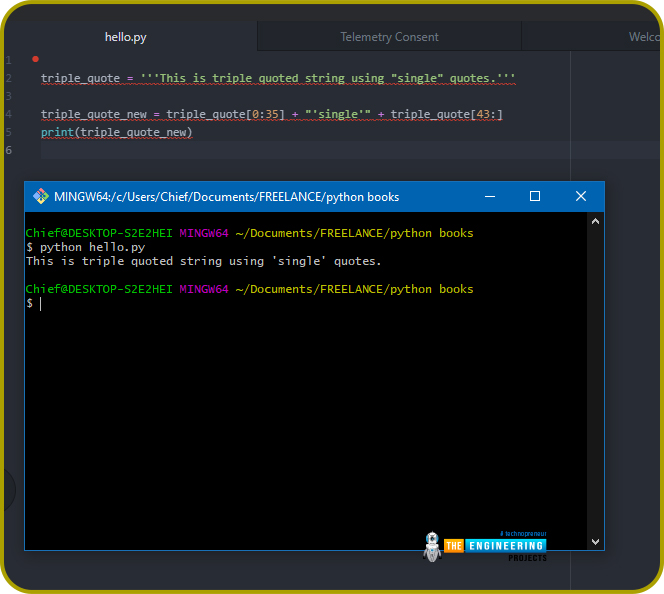
The built-in len() function can be used to determine the length of a string:
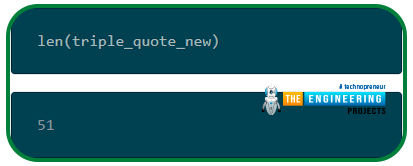
String slicing in python
Strings can be sliced and indexed since they are a sequence of characters. A string's indexing starts at 0 and is based on each character in the string.
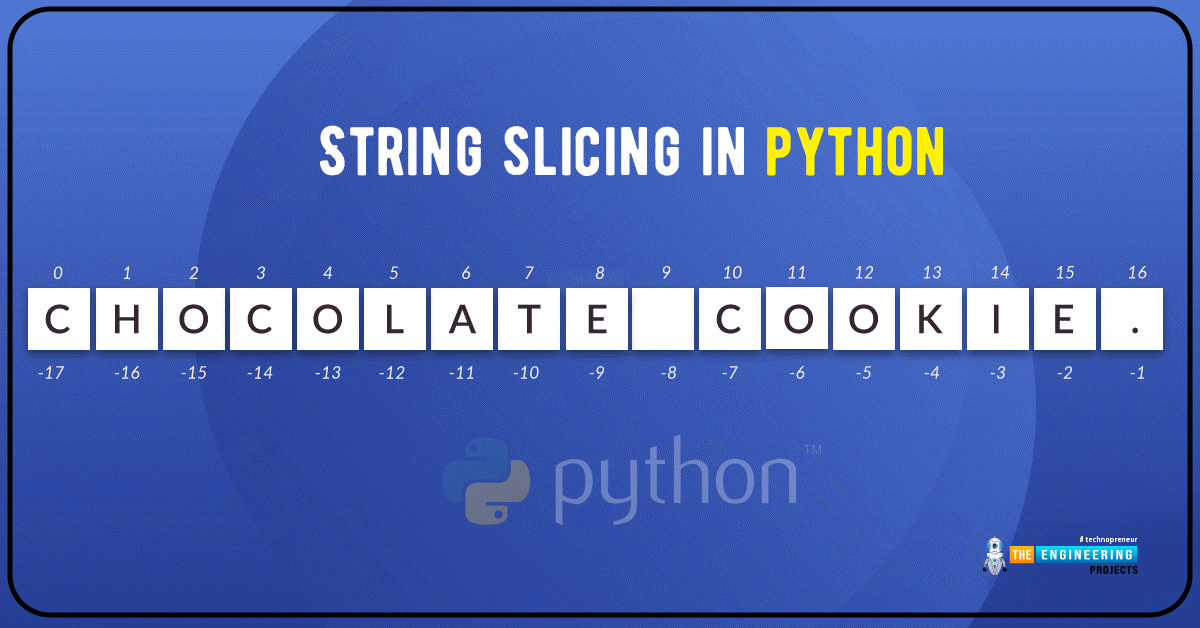
The initial character in the string is C, which is located at position 0 of the index. The final syllable is a period, which is the string's sixteenth character. When you want to access characters in the opposite direction, you can use -1 as an index. when it's strung together, Chocolate and cookie are separated by a whitespace, which has its own index, 9 in this example. Slicing is a good way to verify this.
For the same reason as for other sequential data types, you can read and manipulate Python strings using their associated index numbers. It is possible to slice an object using its index values in Python to select a specific element or a subset of elements. You don't have to write a loop expression to identify or access specific substrings in a string. Slicing does this for you automatically.
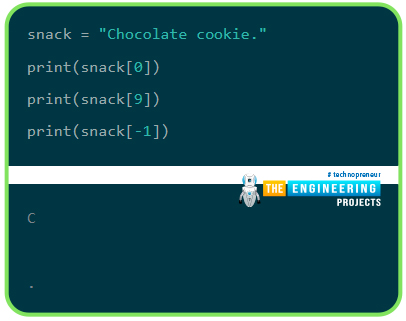
Suppose you were trying to find the cookie substring in the following string. What's the best way to go about it?
Range slicing is used in these situations. The range slicing syntax is as follows:
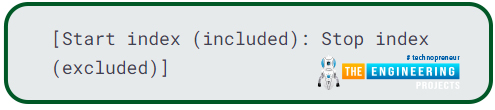
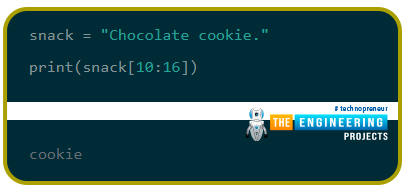
Alternatively, you might use a negative stop index:
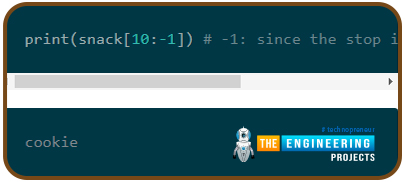
In this case, when you slice a sentence without giving an end index, you get characters from the first index to its last. In the same way, slicing a string without a starting index indicates that you begin at the beginning and end at the end.
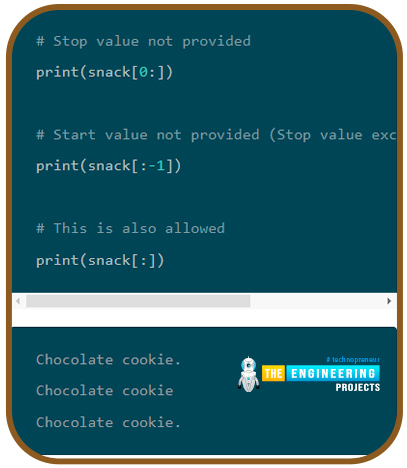
Additionally, the stride parameter can be accepted by string-slicing as a third argument, which specifies the number of characters to advance once the initial one is picked from the string. In the default configuration, stride has a value of 1.
stringnu = "1020304050"
print (stringnu [0:-2:2])
Striding allows you to reverse a string, which is a really cool feature. With a stride of -1, you can begin at the end of the string and move forward one character at a time. With a value of -2, you can start at the end and move two characters at the same time.
String operations
String operations such as slicing and range slicing are frequent. As simple as adding, string concatenation is also available.
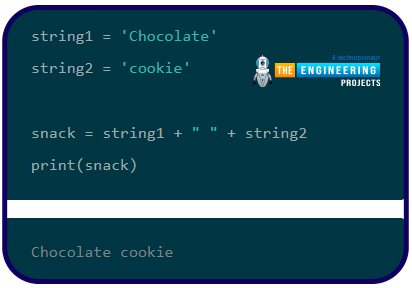
Concatenating a string with another data type, on the other hand, will fail.
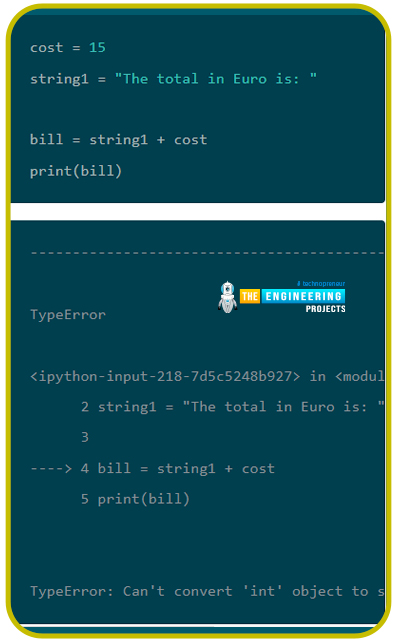
You attempted to concatenate an integer value with a string, which is not permitted. Integer addition or string concatenation is not understood implicitly by the interpreter. However, give this a try:
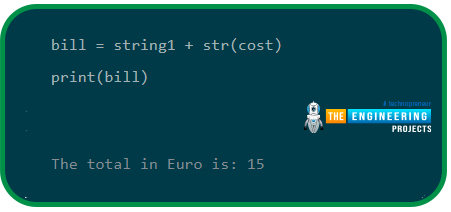
The reason for this is that you used concatenation after you turned the integer into a string.
A string can be repeated using the * method.
wordsig = 'hip '
line1 = wordsig * 2 + 'hurray! '
print (line1 * 3)
To manipulate strings, Python comes with several built-in methods and utility functions. It is possible to use these built-in techniques to replace substrings, to put some words in a paragraph in capital letters, and to locate the position of a string within another text.
- capitalizes the first character in the string returned by capitalize().
- islower(): will return true/false when all characters in the string are lowercase/uppercase.
- If a substring is passed to find(substring), it will be returned at the string's lowest index. When searching for substrings, you may optionally specify a start and end index for each location in the string that you want to search for. If the substring is not found, it returns -1.
- count(substring) returns the number of times a substring appears in the string. The string's start and stop indexes are also programmable.
- isspace() When the string has a whitespace character, the value is true. Otherwise, it is false. A space, a tab, and a new line are all whitespace characters. When working with real-world datasets, this can come in handy because the proper spacing may not be encoded properly during format conversion.
- A function called lstrip() eliminates all leading whitespace from a string. It's a feature that comes in handy when dealing with real-world datasets.
- If the string contains just digits, isdigit() returns true; otherwise, it returns false.
- All instances of the substring are replaced with new using replace (sub-strings, new). A third argument, max, can be specified to replace as many of the substring occurrences as the value of max allows in the string. This is not an in-place replacement, so the immutable attribute of the string remains intact.
- split(delimiter="") provides a list of substrings based on the specified delimiter (or a space if none is provided).
Python string formatting
Multiple string formatting options are available in Python. To better understand these formatting strings, let`s dive right in.
% Format
Python has a built-in modulo percent operation. The interpolation operator is the name given to it. There is a percent followed by the data type that must be prepared or transformed. This operation then replaces the word "percent datatype" with one or more components of that type:
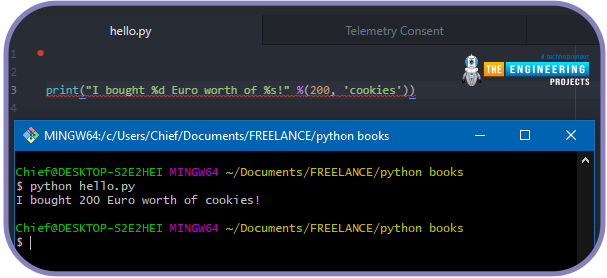
Percent d is used for integers, whereas percent s is used for strings; you've seen both. Octal values can be converted to octal equivalents with this type of conversion, as can Hexadecimal values with this type, and Floating-Point Decimal Format with this type.
Python string formatter class
One of the built-in string classes is the formatter class. The format () method can be used to perform sophisticated variable substitutions and value formatting. Rewriting public methods such as format () and vformat () allows you to build your own string formatting techniques (). There are a number of methods that are designed to be replaced by subclasses, such as parse (), get field, get value, check unused arguments, format field, and convert field ().
Template strings
Templates allow substitutions based on dollars rather than percentages. A major reason for template syntax creation in Python Version 2.4 was that, despite the strength of percent string formatting, errors are easy to make, because the forms that follow '%'are extremely restrictive. This is a common blunder when it comes to percent formatting: forgetting to include the e in percent (variable).
substitution () and safe_substitute() are two methods specified within templates (). You can use them in the following ways:
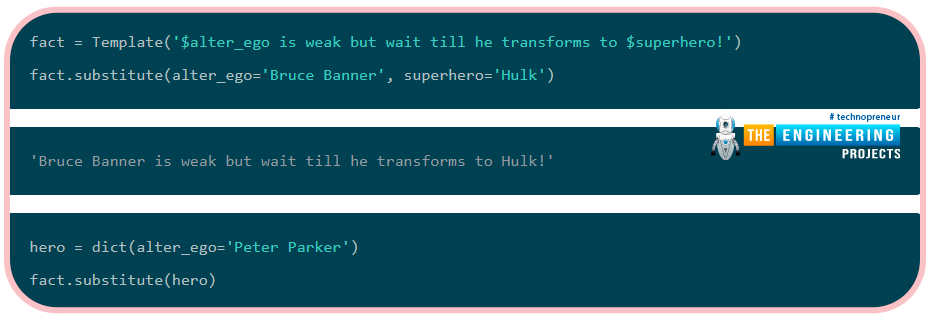
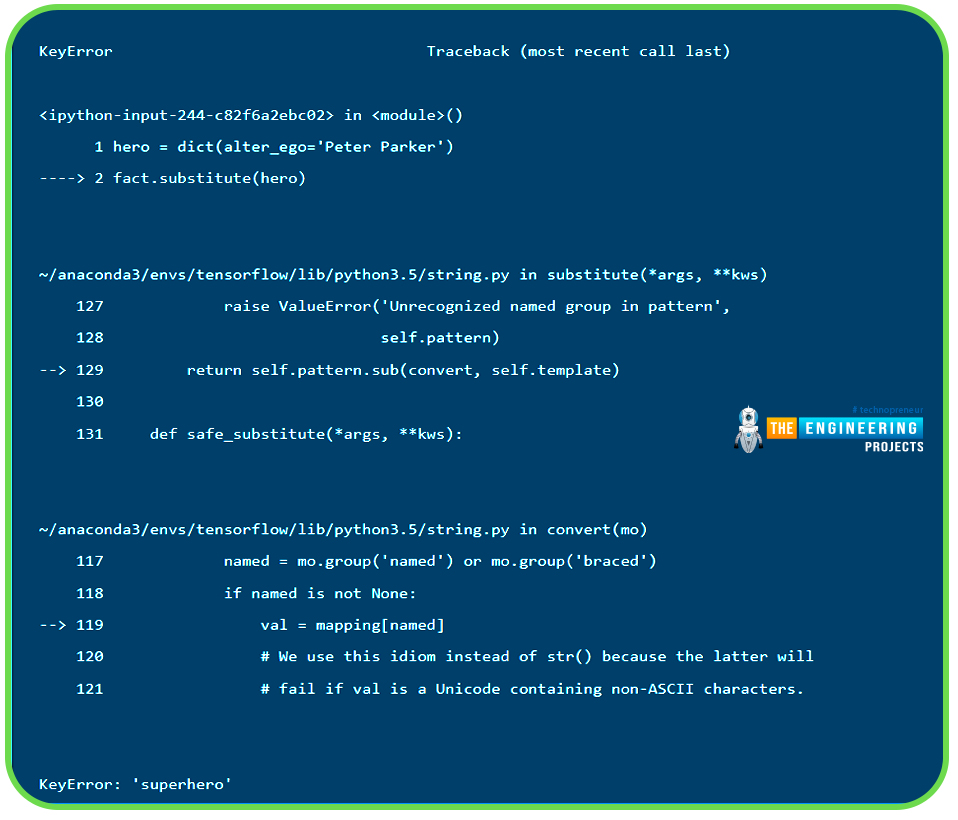
Safe substitution () is an advantage to employing a template, in addition to other advantages.
String literal formatting
In Python 3, this is yet another way to format strings. A prefixed 'f' or 'F' string literal is known as a formatted string literal or f-string. Within curly brackets, you may include identifiers that will be utilized in your string.
What's the point of adding another string formatting option? well, this is because practicality and simplicity are appealing.
To demonstrate why f-strings are the best way to format strings in Python, check out the examples below.
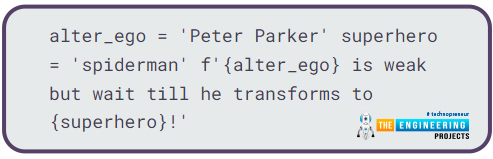
Please note that the preceding code is only compatible with Python 3.6 and above. With f-strings, Python expressions can be used inside curly braces, which is a significant benefit of using them.
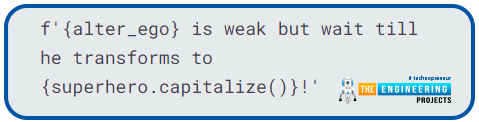
What's the point of having so many string formatting options?
Syntax is the most important consideration here. For the most part, it boils down to the trade-off between simplicity and the amount of verbosity you're willing to sacrifice. People with a C programming background will find it easy to use the percent sign to format strings, for example. Using the format () function can be more verbose, but it provides a wider range of options.
Input function with strings
While your application is running, you can utilize input routines to get data from the user. A key benefit of this approach is that it does not rely on preexisting values or file content to function. The syntax for the input function is as follows.
input([prompt])
Input functions will cause our application to pause. After the user inserts the text into the Python shell or command line, the application resumes.
input(message)
In order to prompt the user for text, you'll need to provide a message. It's important that a user understands what they need to do by reading this message. As a result, a user may wonder why the software isn't progressing. For example,
input ("Enter email address: ")
print ("Confirm it is your email address:")
In order to request an email address from a user, we've implemented the input () method. Messages in brackets are displayed on the same line where a user is expected to enter text in the command line.
Note that as soon as a user inputs data into the input () function, it is automatically converted to a string.
How to draw an imaginary dog.
Using the fundamentals of strings that we've learned in this lesson; we'll construct a simple program that prints out an image of a dog.
Let's open up our favorite coding editor, Atom, and get started. Before looking at the solution, I advise you to give it a shot on your own.
- Step 1: In the code editor where the hello.py file is loaded, write the print function () to print out the upper body of our dog image.
- Step 2: Insert double quotes or single quotes inside the print function. This shows the output will be a string.
- Step 3: Write "o" in the speech marks.
- Step 4: Close the speech marks and reopen the speech marks. This is because we want to add another string to the same print function. Add hyphens to represent the neck and upper body.
- Step 5: Add a plus sign between the strings to concatenate the strings.
- Step 6: Create another function to print the legs. Same way we did the upper body, we will now print the legs. Inside this function, we create a string and write four slashes to represent legs. Now our dog program is complete, save the file and run the code using one of the methods we learnt in the previous tutorial. The results should be:
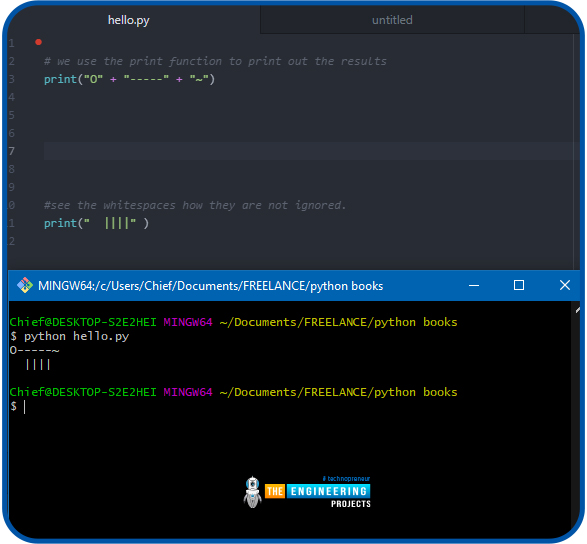
Conclusion
Congratulations! You've made it this far. You have learned about string slicing, what strings are, and explored a variety of string-related processes. Many approaches to formatting strings have also been discussed. But don't forget that practice is the key to mastering any skill! I'll see you in the next tutorial.