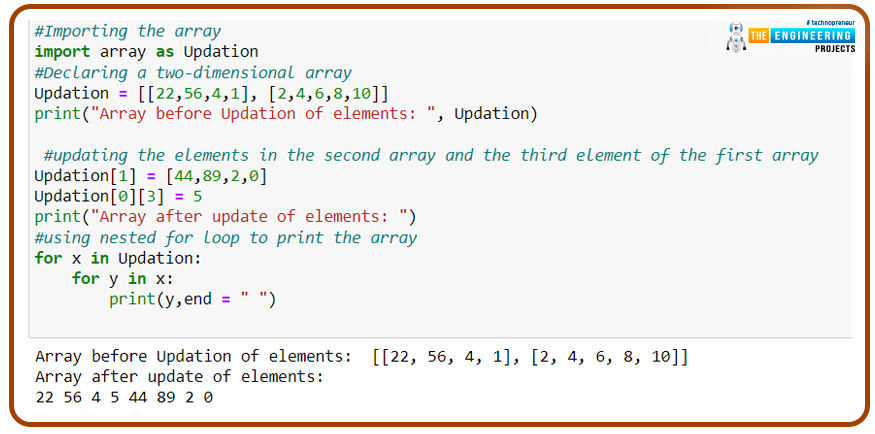
Hello learners! Welcome to the next episode of the arrays, in which we are moving towards the details of the arrays at an advanced level. In the previous lecture, we covered the introductions and fundamentals of arrays, dimensional arrays, and array operations. One must know that the working of the arrays does not end with simple operations, and there is a lot to learn about them. Arrays and their types are important topics in programming, and if we talk about Python, the working and concepts of the array in Python are relatively simple and more effective. The details of the advanced types of arrays will prove this statement. We have a lot of data to share with you, and for this reason, we have arranged this lecture. It is important to understand the reasons behind the reading of this lecture.
What are two-dimensional arrays, and how can we perform them in a Jupyter notebook?
How can we access all the elements of the two-dimensional arrays?
Can we insert the elements in the two-dimensional arrays?
How do we update our 2D array?
Is the appending process easy in the 2D arrays in Python?
Explain three-dimensional arrays and provide an example of how the array is updated.
All of these are important interview questions, and you will learn the concept through its practical implementation, so stay with us till the end of this lecture to get all the answers.
Two-Dimensional Array in Python
We all know a lot about one-dimensional arrays and have seen these concepts at different levels. In this type, we are dealing with the arrays at an advanced level, and to understand them, we will work on the Jupyter notebook. The second type of array is a little bit different, and we can say it is twice as difficult as the one discussed before. We all know the concept of dimensions from physics classes, and these concepts are also true for arrays. These are also referred to as "multi-dimensional arrays," and we define them as:
“The 2-D arrays are the type of arrays that are shown by two indices, and these contain rows and columns to save the data in the form of matrices.”
As you can guess, these are comparatively more complex than the one-dimensional arrays, and storing the data in them is more useful and has many applications in real life. While performing the example of a 2D array, we are going to use the simpler way where the user just has to mention the numbers of rows and columns and an array is ready.
Syntax of a 2D Array in Python
array-name = [ [d1, d2, .... ,dn], [e1, e2, .... ,en] ]
Here,
d1,d2,...,dn=number of elements in the first dimension
e1,e2,...,en=number of elements in the second dimension
The numbers of the elements vary from zero to infinity. For more detail, have a look at the example given next:
#Initializing the two-dimensional array
array_input = [ [10.9,78.9,111.90] ,[8.0,771.2,2.0] ]
#showing the elements of each dimension separately
print("The elements in the first dimension = " ,array_input[0])
print("The elements in the first dimension = " ,array_input[1])
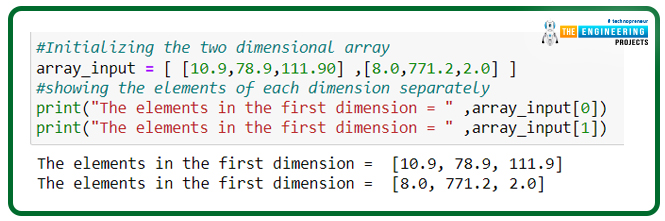
So you can see that the initializing of the array with the floating elements is done in the first step. Here, the point to notice is the usage of square brackets, and you have to remember that no other type of array is used in this case.
Accessing the All Elements of Array
In the previous case, you have seen that if we wanted two or more elements as output, there was a need for more than one print function. Yet, there are also cases where the whole elements of an array are required on the screen. For this, the programmers use different types of iterations according to the requirements and get the results. We have seen the working of the iterations many times in this course and therefore, there is no need to explain why we use it. Arrays are one of the best examples to check the working of the iterations. In all the examples of the arrays, you will see the use of for loop in the nested form because we are dealing with two-dimensional arrays.
Inserting New Elements in 2D Arrays
One must be wondering how we can add more elements to the two-dimensional arrays because of the matrix-type arrangement of the lament. For this, the method is simple, and by discussing it, we will learn the “input” method of Python. Have a look at the code given next, and we will discuss the details in just a bit.
#importing the array from the Python
import array as twoDArray
#declaring our array with two dimensions of the order 2 by 2
twoDArray = [[2,2,2,2,2,2,2], [6,6]]
print("Array before insertion of elements: ", twoDArray)
#Inserting the elements in the matrix at position three
twoDArray.insert(3, [4,7,4,2,9])
print("Array after insertion of elements: ")
#Using nested for loop to inset the elements one after the other at the required position
for x in twoDArray:
for y in x:
print(y,end = " ")
print()
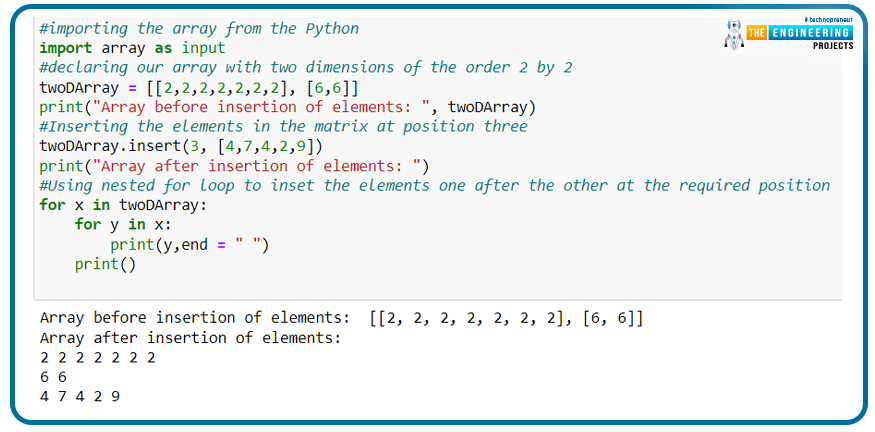
The following points are to be discussed according to this concept:
Importing the array makes the code easy and effective.
The two-dimensional array is nothing but the arrangement of elements in the form of rows and columns.
The number of rows and columns is not restricted to each other, that is, there is no restriction on using the square array all the time, and the number of elements in rows and columns may be different from each other.
The square brackets are used in the two-dimensional arrays in every case, whether it is the outermost bracket or dimensional bracket.
Special functions such as insert, delete, etc. can be used by using the dot operator with the name of the array.
To insert the elements in the two-dimensional array, a nested for loop is efficient where two loops are being used. The syntax is important in this case.
Other rules of the code are discussed in different lectures in this course.
Updating of the Elements in an Array
The next case that we are going to discuss is the updating of the elements in the arrays. Think about the situation when you first declare the array and work with it, but after some time, you have to change some of the elements of the arrays. In such cases, the insertion and deletion operations that must be done are time-consuming. The best way to do this is to update the elements directly. The reason why I mention this detail is that arrays are unchangeable data types in other programming languages such as C++, and once declared, the arrays can not be altered in such languages. Yet, Python gives us the ease to update, delete, insert, and change the arrays in the programs. We know programming is a vast procedure and there are several ways to do the same task, but for you, we have picked the simplest and most interesting way to do so that is given in the code next:
#Importing the array
import array as Updation
#Declaring a two-dimensional array
Updation = [[22,56,4,1], [2,4,6,8,10]]
print("Array before Updation of elements: ", Updation)
#updating the elements in the second array and the third element of the first array
Updation[1] = [44,89,2,0]
Updation[0][3] = 5
print("Array after update of elements: ")
#using nested for loop to print the array
for x in Updation:
for y in x:
print(y,end = " ")
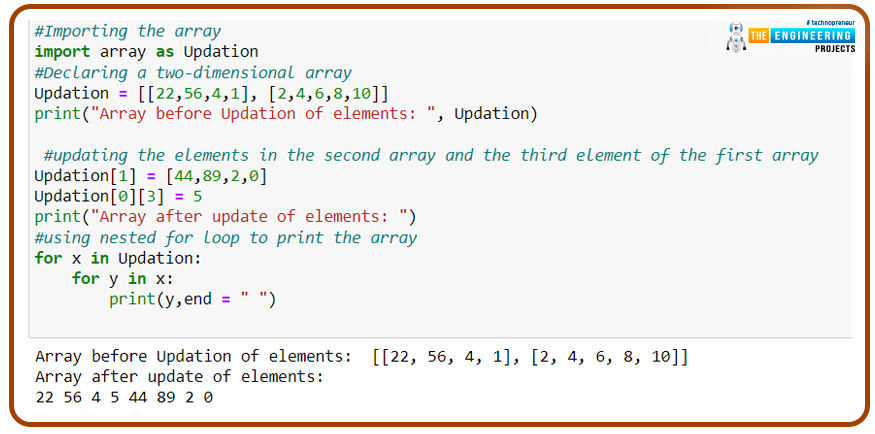
We are now able to understand such codes; therefore, there is no need for long descriptions. But, the point here to notice is, in the same code, merely by declaring the elements at certain positions, the array can be updated and the previous values are then ignored.
Appending the values in the Array
In the previous lecture, the element at the end of the one-dimensional array was appended easily. Yet, you must think that more detail is required for the appending of the data because it has to be mentioned where the exact place is where you want to append the data. So, if you remember the previous lecture then you will find it different to append this type of array.
#importing the array
import array as myArray
#initializing our array
myArray = [[1.4,66.90,234.0], [009.6,127.8,34.0,11.34], [0,2]]
print("Elements of my array = ", myArray)
#Using the length operator to check the length before appending the elements
length=len(myArray)
print("Length of the array before appending: ", length)
#The process of appending
myArray.append([7.9,334.90,2303])
#calculating and printing the length after appending elements
length=len(myArray)
print("Length of the array after appending: ", length)
#printing the results after appending
for x in myArray:
for y in x:
print(y,end = " ")
print()
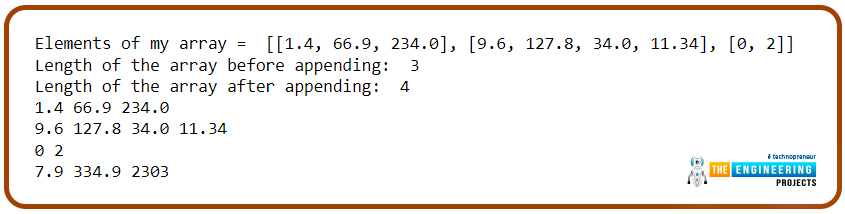
The difference is clear; the number of elements can be easily compared with the help of the length function. We have used the length function many times in this course, and here you can see a better example of how to use it.
Three Dimensional Array
The three-dimensional array is the most complex form of the array discussed so far, and usually, at this level, the learner does not go into the details of the three-dimensional arrays to avoid complexity. The 2D array has many interesting applications and has the ability to store a lot of data in a cleaner way; therefore, 3D arrays are not used much for learning purposes, but if we talk about complex applications such as gaming and other fields, the 3D arrays have a great scope.
The form of the 3D array can be understood by keeping the matrix of the order 3x3 in mind. Here is a little information about the initialization of the three-dimensional arrays in the Jupyter notebook.
#initializing my three-dimensional array
ThreeD=[[[22.5,[67.9],[44.4]],[5],[23.8]],[[12,[123],[893]],[0],[78],[126]],[[70],[11]]]
print("The three dimensional array = ", ThreeD)
#Simply updates the elements by mentioning the locations and values.
ThreeD[0][0][0] =11
ThreeD[1][0][0] =21
ThreeD[0][0][1] =111
#Printing the results
print("After updating the elements the array = " ,ThreeD)

Hence, this picture gives us information about the shape and form of the three-dimensional arrays. Do not think that 3D arrays have the form of a 3-by-3 matrix. I suggest you take a deep look at the brackets of the array that we have mentioned here. The complexity of the three-dimensional array is much greater than in previous cases. To make it simple, we have used the single element in the single brackets, but there are more options for the same work that will be unnecessary to learn right now.
Another thing to be mentioned here is that, for simple operations such as displaying and updating the elements, there is no need to import the array every time. Yet, we have used it to display the proper professional coding of the array. We are not talking about the three-dimensional arrays in more detail because they are very difficult to understand and because there are some other options that work better than the three-dimensional arrays. You will learn it in detail in the next lectures.
Therefore, we can say that we have learned a lot about the advanced level of arrays in Python which are two-dimensional arrays and three-dimensional arrays. It is interesting to note that dealing with arrays is much easier in Python than in any other programming language. Therefore, we always say that Python is interesting and easy to learn. The topic ends here, but the detail of data type is not limited to just the types of array. You have to learn a lot about iit,and we are here to share every single and necessary detail in a simple way. So, stay with us in this learning phase.