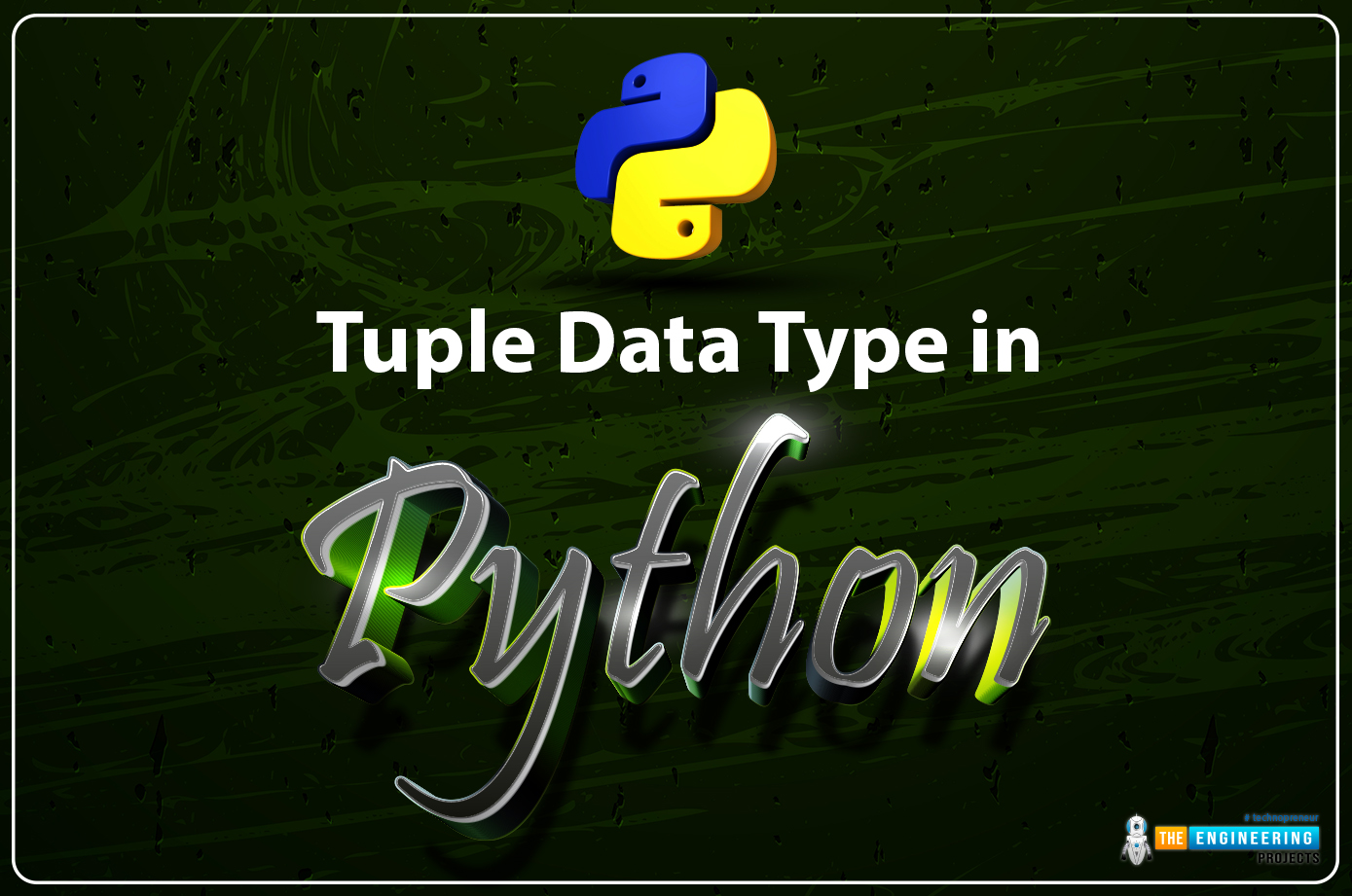
Hey peeps! Welcome to another tutorial on data types in Python. Our purpose in Python education is to get a grip on the basic concepts so that we may work on deep learning easily. In the previous lecture, we read a lot about lists as we are working on the subtypes of the sequence data type. In the present lecture, you are going to understand more types of sequences. If you know the list well, this lecture will be a piece of cake for you. We will start the introductions in just a bit, but before that, there must be a quick review of the topics that you are going to understand:
How do you introduce the tuples in Python?
What are some important characteristics of a tuple that must be kept in mind when we are dealing with it?
How can you practically perform the tuples in TensorFlow?
How do you associate tuples with the list?
Can you delete, update, add, or delete the elements in a tuple? If yes, then how?
Which is the way to delete the tuple entirely?
All of these are important interview questions about the tuple and if you are dealing with these data types, you must know and perform these by yourself in TensorFlow. All the information about tuples will be discussed with you and you have to practice more and more in the code to understand the concepts.
Tuple in Sequence
For the concept of a list, we have given you the reference of an array. This time, we will be mentioning the name of the list for better understanding. If we talk about the topic of today, a tuple is also a group of items that are arranged when required in Python. The working and the definition of the tuple seem like a list because both of them are types of a single data type, which is the sequence. But there are some differences that make them ideal for different kinds of situations. The following points will make this more clear:
The tuple is represented by enclosing the data in parentheses.
A tuple is immutable, which means that once you declare the items in the tuple, you can not change them as you can in a list.
When you try to change the value of the element in the tuple, an error is shown on the screen, so while declaring the new tuple, you have to be very clear about what is on your mind and what you want to do with the tuple.
In a tuple, you can also use the function in the tuple and it becomes easy to perform different tasks through codes.
A single element in the tuple is necessary to build the tuple. In other words, unlike the strings, the tuple must contain at least one element in it.
In the tuple, any data type can be saved whether it is boolean, int, or string. Moreover, there is a possibility to add different types of data in a single tuple. So we can conclude that we can have a homogeneous or heterogeneous tuple according to our choice.
Order of The Tuple
As we have mentioned, when using the tuple, we get the ordered list of objects, which means we get the specific order, and this order, once mentioned, can not be changed, unlike other data types of the sequence.
Unchangeable Tuple
Not just the order, but the size, sequence, and entries are unchangeable, so during the initialization and declaration of the tuple, the concept must be clear about what you want from the particular tuple.
Duplication in Tuple
Another thing that must be kept in mind is, the tuple has the index form, and therefore, every element has a specific number of indexes. This is the reason, the elements are easily accessible, and hence, you can also have duplication in the tuple. The elements are recognized by the index number, and therefore, the compiler knows when which element is being called.
Length of the Tuple
The length is the number of elements in the tuple, and we have read about the length function in the previous lecture. Similar to the list, a tuple can also be used in the length function, and the programmer gets the length of the tuple. Right now, this function is not looking very attractive because we are dealing with small tuples. But take the case in your mind when the tuple contains hundreds or thousands of elements and get the length of the tuple in just a few moments.
Tuple in TensorFlow
It is now time to go over some basic tuple-related code using TensorFlow. The steps for starting it are the same as those we always follow; have a look at these steps:
Open your Anaconda Navigator by browsing it from your window panel.
Search for the Jupyter lab in the environment section.
Wait for the PC to open the new tab in your browser.
Go to the new cell.
Start coding.
With the help of code, we will try to create a tuple, and then, by using the function on it we will try to retrieve the data from the tuple in different ways. So, have a look at the code given next and then guess the output in your mind.
print('Make a tuple with stationaries item')
myTuple=('pen', 'paper', 'eraser', 'pencil', 'sharpener', 'notebooks')
print('The tuple has following items: ',myTuple)
print()
print('print only the third item of the tuple:', myTuple[3])
print()
print('print the item by using the index: ',myTuple[1])
Now, have a look at the output. Is it exactly the same as you were expecting?
You can see how simple it is to get the item from the tuple when it is declared in the tuple. This is the same as what we did with the list. But what if we want more than one element from the tuple at the same time?
print('Make a tuple with fruits and prices in dollars')
myTuple=('strawberry','kiwi','banana','orange','apple', 2.5,3,12, 4,6)
print('The following items in the fruit shop are available: ',myTuple)
print()
print('print only the forth fruit of the fruit shop:', myTuple[-7])
print()
print('print the name of only fruits: ',myTuple[0:5])
Looking at the code, you will observe that the negative number is used in the index. This is the way to tell the compiler that we are counting from the end of the index. Moreover, the index on the end side starts at 1 instead of zero. Another point to notice is that the start and end limits are specified by separating them with the colon, and as a result, we get the whole tuple in the output.
A new concept that is to be shared is that when you are providing the limits to the tuple, you have to take care that the right numbers are being used because it will then not work properly. You must be thinking that the compiler will throw the error when you feed the wrong limits in the tuple, but instead, the output will be empty, and you will get the parentheses with nothing in them.
Another thing that must be mentioned here is that you can check whether the tuple has a specific element or not. For this, we get the help of a statement. We know that, until now, we have not learned about the statements, and therefore, we suggest just looking at the code and output to understand the concept.
print('Make a tuple with fruits and prices in dollars')
myTuple=('strawberry','kiwi','banana','orange','apple', 2.5,3,12, 4,6)
print('The following items in the fruit shop are available: ',myTuple)
if "apple" in myTuple:
print("Yes, 'apple' is in the present in the fruit shop")
This program searches for the elements specified in the “if condition” and then prints the result on the screen.
How to Make Changes in A Tuple
If you are reading this lecture from the beginning, you must be thinking we have mentioned again and again that a tuple is immutable and unchangeable. Yet, programmers have the logic and solutions to every problem, and if the programmers have specified the tuple and want some changes made to it, they can do so with the help of the list. It is one of the reasons why we have discussed the list before in this series. Have a look at the following code and output, and then we will discuss it in detail.
print('Make a tuple with fruits and prices in dollars')
myTuple=('strawberry','kiwi','banana','orange','apple', 2.5,3,12, 4,6)
print('The following items in the fruit shop are available: ',myTuple)
myList=list(myTuple)
print(myList)
myList[2]='pear'
print(myList)
myTuple=tuple(myList)
print(myTuple)
Copy this code and run it on your TensorFlow, you will get the following output:
First, look at the brackets carefully and check the output in a sequence with the points given next:
At the start, the string message is shown, which shows the main title of the tuple.
The name of the tuple here is “myTuple” and it contains the fruits’ names and prices.
To make the changes in the tuple, we have to use another approach, and we know the reason why. For this, we are using the list. It is possible to convert the list into a tuple and vice versa, and we are doing the same in this. We are just using the name of the data type as a function and inputting the name of the data type to be changed. So, we changed the tuple into a list and then made the changes according to our choice.
By using the index number and feeding the value into the list, the list is updated. This can be observed with the help of square brackets.
Once we have seen the updated list, we can now easily convert it into a tuple again.
The conversion is done with the same procedure, and we get the updated tuple.
This was a simple example, other operations such as the addition of the new element, deleting the elements from the tuple, removing the single elements from the tuple, etc. are done with the help of lists.
Deleting the Tuple Entirely
Now that you know all the ways to initiate, use, and update the tuple in detail, a last method for the tuple is ready for you. In some cases, when you do not want to use a particular tuple for any reason, such as if you are no longer using it, you can do so in just a simple step. This is used in the programs when long calculations and collections of data types are needed for a particular time and then there is no need for them anymore. So for this, we use the delete operation. The programmers have to simply use the del keyword before the name of the tuple to be, and the compiler has to do its job well. Have a look at the example given next:
Tuple = ("Artificial Intelligence", "Machine Learning", "Deep Learning")
print(Tuple)
del(Tuple)
print(Tuple)
So, when the compiler was on the second line, it showed us the results, but on the next line, when we deleted the declared tuple, the compiler was not able to show us the result because it had been removed from its memory.
So, it was an informative data types lecture in which we clarified many concepts about tuples. These are the data types that belong to the class of sequences. We read a lot about it and started the discussion with the introduction. The tuple characteristics were thoroughly discussed, and then, with these in mind, we tested the functions of the tuple using TensorFlow examples. We attempted similar examples and carefully observed the operation of tuples, which also involved the list. In the next lecture, you will know more about the data types in Python, so stay connected with us.