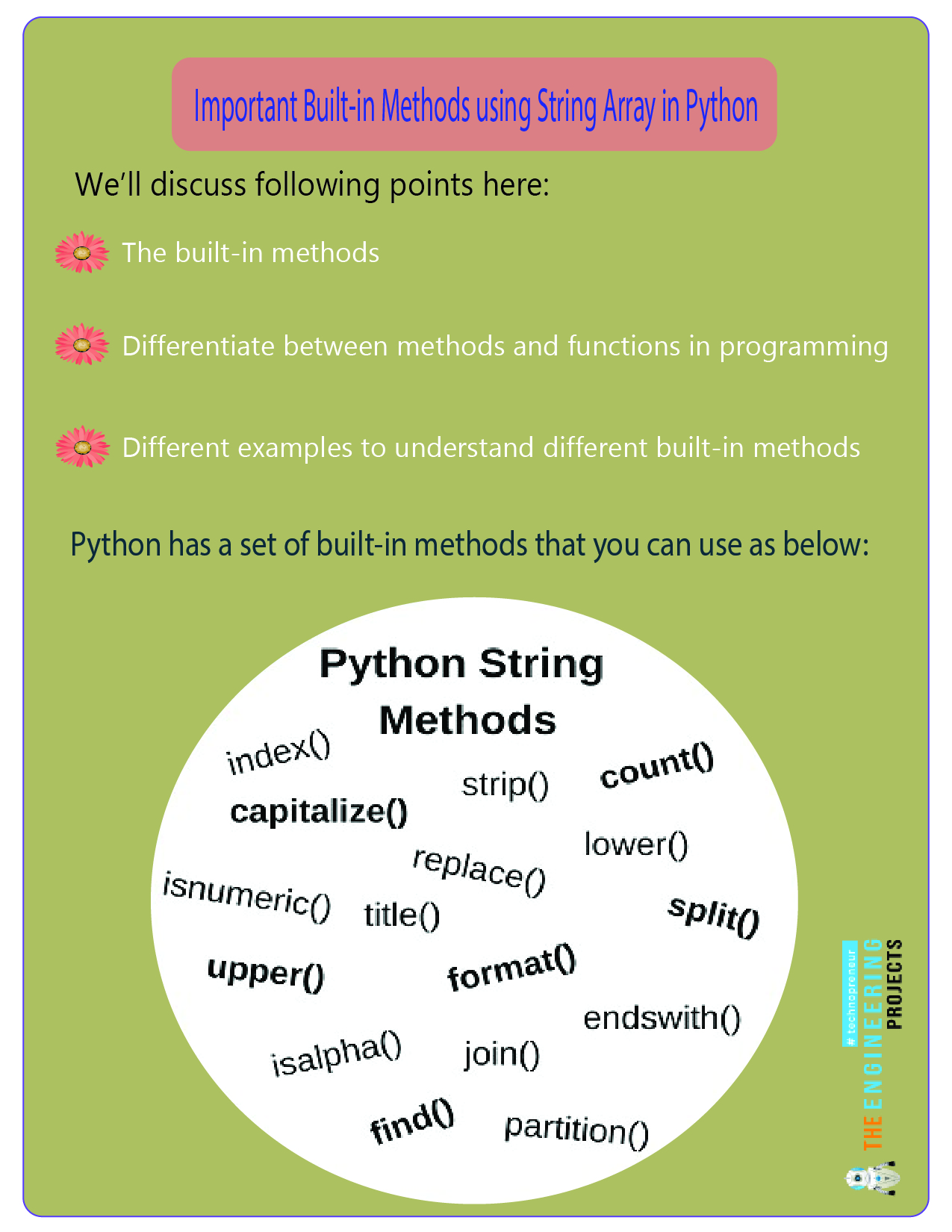
Hi learners! Welcome to the next episode of learning the arrays using string data. We have been working with the arrays and in the previous lecture, we saw the interesting characteristics of the string arrays. In the present lecture, our target is to get knowledge about the built-in functions and we will take the strings in the array so that we may know about both of them together. String arrays are used in many officials uses and therefore, we want to use the built-in functions that every Python programmer must know. Here is the list of the concepts that will be polished in this lecture:
What are the built-in methods?
How do you differentiate between methods and functions in programming?
Give different examples to understand different built-in methods to be used in the same code.
How do Jupyet notebook use the copy(), clear(), Insert (), Index(), reverse(), range(), and sort() function in Python on the string array?
How do these functions are related to the working of simple string messages?
For the convenience of the students, we will refresh some important concepts in our mind side by side and whenever required, we will provide the necessary details about the working of the particular method. All these methods have been introduced in Python for a better user experience. One must notice that the same work is also done in other programming languages but Python gives us an uncomplicated way to use them.
Difference between Methods and Functions
Programming is a vast subject and if programmers start making short programs again and again when required, the programming process becomes boring and time taking. For this, the programming languages have built-in methods and functions to be used by simply mentioning them in a specific syntax. Normally, people seem confused between these two because the working and syntax of both of these look alike. So, let's discuss some minor differences between these two.
Function |
Method |
A function is a piece of code that can be reused. It can operate on input data (i.e. arguments), and it can also return data by having a return type. |
A method is associated with the objects with which it is being used. |
It is independent. |
It depends upon the object’s name and requires a reference. |
It is related to the procedural or functional programming language. |
It is related the object-oriented programming concepts. |
The data passes explicitly or at the external level. |
The data in the method is passed internally. |
The working of both of these is similar as the programmer has to input the required data and both of these, after their specific operations, provide the required output as expected.
Built-in Method Used with String Arrays
There are certain methods for the arrays and related concepts that are defined by the Python programming language, but to understand them, we are using the arrays with the string values in them. The method makes the coding easy to use and without knowing them, it is not possible to get the perfect result for a complex task. This will be clear with the help of examples of each of them. A list of such methods is given next:
clear()
copy()
extend()
count()
insert()
index()
Reverse()
Sort()
Here, we want to compare these functions with some of those that are discussed before in this series. So, let’s move towards the first example:
Copy() and Clear() in Python
If you are interested in programming, you must know to copy the code from one place to another so that you may test it more accurately and produce the better one by using your concepts. By the same token, another method that is important is clear() which is used to clear the memory area where the array is being stored. In this way, the programmer gets the empty space. For convenience, we are using these two in a single code:
Code:
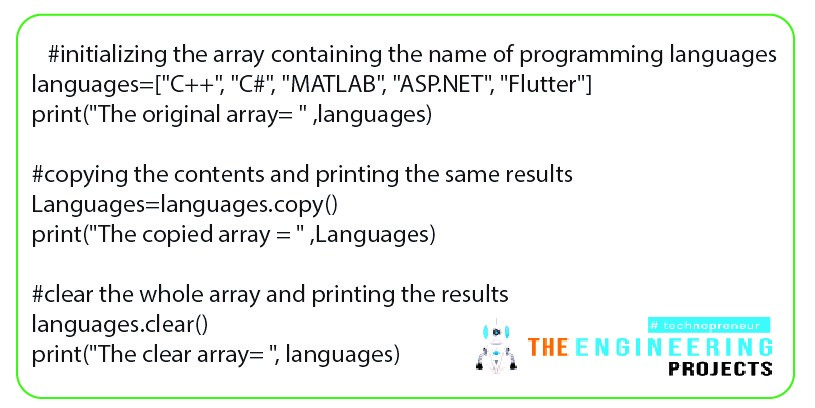
#initializing the array containing the name of programming languages
languages=["C++", "C#", "MATLAB", "ASP.NET", "Flutter"]
print("The original array= " ,languages)
#copying the contents and printing the same results
Languages=languages.copy()
print("The copied array = " ,Languages)
#clear the whole array and printing the results
languages.clear()
print("The clear array= ", languages)
Output:
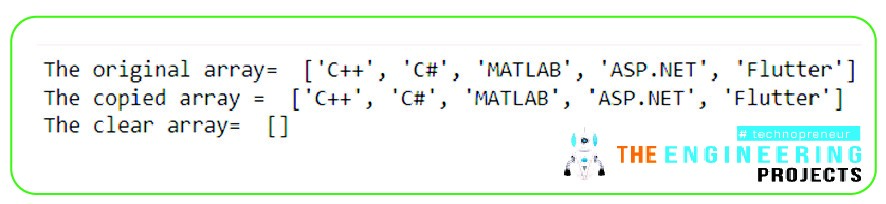
Here, it is important to notice the difference between the clear() and remove(). The clear() causes the empty locations in the array no matter what the size of an array is whereas, in the remove method, the whole array was removed and the compiler throws the error about the existence of that particular array. In other words, the compiler, after the clear() gives you the chance to add the new data of your own choice and you can think that the container of the element is empty but still, we have that container and can fill the data according to our will.
Extend() and Cout() Method in Python
The next methods that we are going to discuss are fun to perform. In the previous lecture, we saw the append method, and this time the same task will be performed with the help of another method called extend(). As the name suggests, this function extends the length of the array by adding another array or extra item to it. The modification of the array is an important feature of Python, and practically, it seems like a blessing in some cases.
The other method that will you see in the code is the count(). It counts the frequency of the mentioned item in the data type, and in our case, we are using it to compare the frequency of the “flutter” before and after the extended array. The element may be any, and the programmer simply has to input it into the method to get the frequency. In programs with a large amount of data where the array or list has 1000+ or even more items, the count() method is a blessing.
Code
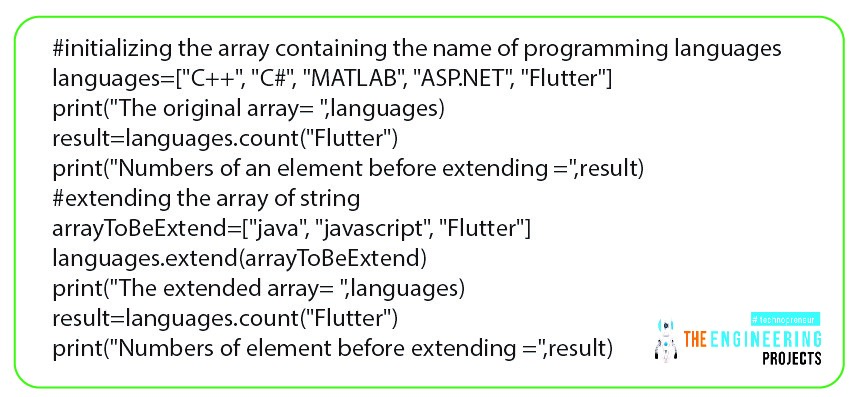
#initializing the array containing the name of programming languages
languages=["C++", "C#", "MATLAB", "ASP.NET", "Flutter"]
print("The original array= ",languages)
result=languages.count("Flutter")
print("Numbers of an element before extending =",result)
#extending the array of string
arrayToBeExtend=["java", "javascript", "Flutter"]
languages.extend(arrayToBeExtend)
print("The extended array= ",languages)
result=languages.count("Flutter")
print("Numbers of element before extending =",result)
Output
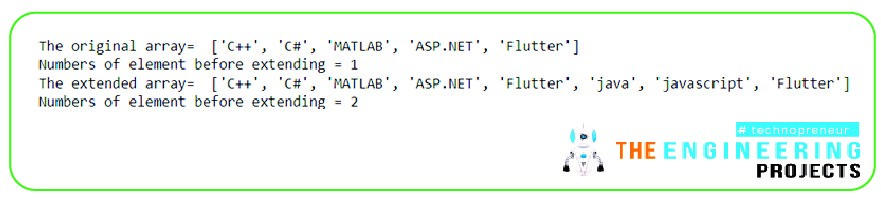
Insert() and Index() in Python
The next methods to be discussed are the insert and index methods in Python, and we are expecting that you understand the working of these methods. As in the previous cases, the name exactly matches the working name of the method.
Code:
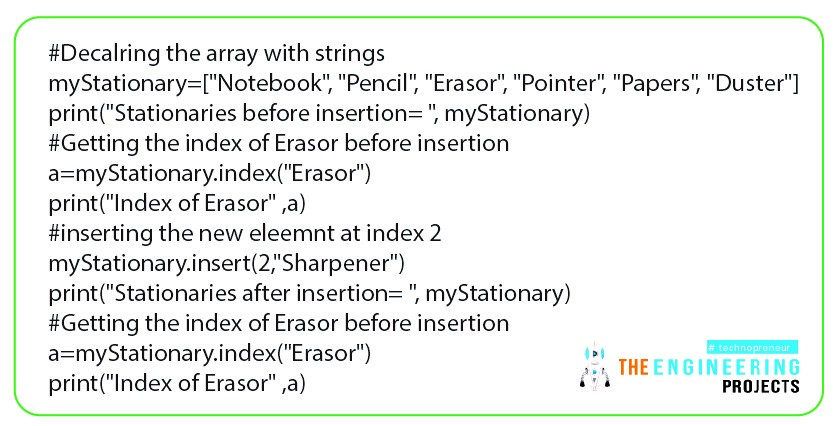
#Decalring the array with strings
myStationary=["Notebook", "Pencil", "Erasor", "Pointer", "Papers", "Duster"]
print("Stationaries before insertion= ", myStationary)
#Getting the index of Erasor before insertion
a=myStationary.index("Erasor")
print("Index of Erasor" ,a)
#inserting the new eleemnt at index 2
myStationary.insert(2,"Sharpener")
print("Stationaries after insertion= ", myStationary)
#Getting the index of Erasor before insertion
a=myStationary.index("Erasor")
print("Index of Erasor" ,a)
Output:
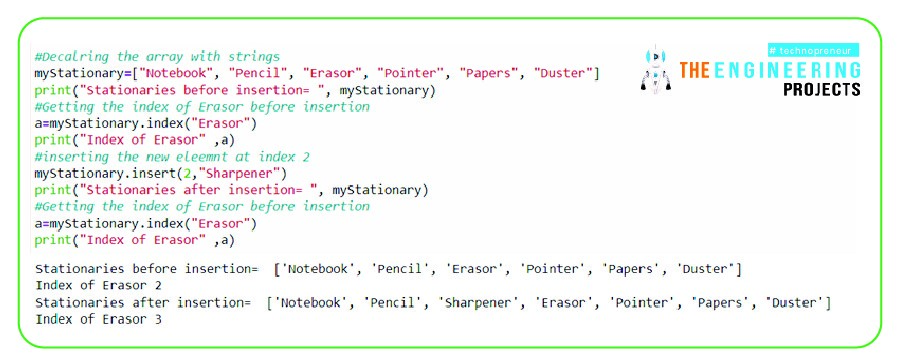
In the program given above, we have seen that the list of stationary contained the specific numbers of the elements, out of which, the index of the eraser was 2 at first, but after the addition of the new element, the index function tells us the 3 index number.
Reverse() and Range() in Python
Now, we are moving towards the situation where we will play with our array. It is interesting to notice that we can retrieve the data in different ways from a single array. Let’s say, the programmer wants results in which the order of the elements in an array is reversed. No matter what the length of the array is, the reverse() method creates the mirror image of the array and represents it. This is important in the field of image processing, where we can invert the images in a simple way.
On the other side, the range function gives us the elements in a specific length of the array according to our needs. We have been using this in the previous examples in this course, but this time, we are specifying the range() method. It is a professional way to do so. The logic behind this is not compulsory to understand; therefore, to make things simple, we are not going into detail. Have a look at the code where we are using both of these methods.
Code:
#Declaring the array
myDishes=["chow mien", "sandwiches", "rice", "butter puffs","pizza","meat balls", "rolls", "momos", "patties", "chicken", "coffee cake", "wings"]
print("\bThe array before reversing= ", myDishes)
#Reversing the whole array using the reverse method.
myDishes.reverse()
print("\bThe array after reversing= ", myDishes)
#Using the range method to get the elements
print("\bThe element from 3rd to 8th position are myDishes", myDishes[3:8])
Output:
Here, the interesting thing is, just like we used the same method in the previous lecture when we were using the same procedure on the simple string, this time, we have used it on the whole array of the string, and the outcomes are perfect. The programmer can easily get the part of the array they only want and perform different operations on that certain list.
Moreover, the reverse function is also useful in many places, as we have discussed before. The transformation of the array is not just limited to the strings, and in this way, the numbers, when used in the array, can be inverted in their prepositions and useful results can be obtained.
Sorting of String Array
We believe that you are from a programming background, and if you are from a C++ background, then you must have experience with the sorting of an array. We define the sorting process as:
"The sorting of the data is defined as the technique to rearrange the elements in a particular order or manner according to the needs of the data."
Hence, as you can see, there are different ways of sorting, but here, we are using the sorting method that arranges the elements of the string array in alphabetical order. This type of sorting follows the ascending order of the alphabet, and as an example of the reverse method, we will use the sorted array in reverse to get the new array with the descending order of the string array.
Code for Sorting String Array:
#Declaring the array
myDishes=["chow mien", "sandwiches", "rice", "butter puffs", "pizza",
"meat balls", "rolls", "momos", "patties", "chicken", "coffee cake", "wings"]
print("\bThe array before sorting= ", myDishes)
#sorting the whole array using the sort method.
myDishes.sort()
print("\bThe array after sorting= ", myDishes)
#Reversing the whole array using the reverse method to get the array in descending order
myDishes.reverse()
print("\bThe array after sorting= ", myDishes)
Output:
Hence, it is a perfect way to understand how we can use the different methods together, (in this case, the reverse method) in the same code to get the desired output. Sorting is an important concept, and in programming languages such as C and C++, sorting is done by long codes, but with the help of this uncomplicated method, the programmers can get the desired output easily in just one step.
Consequently, it was a lecture full of practical examples of the methods in Python, and we have seen many daily life problems that can be arranged in a better manner using the built-in methods. We made the pair of methods into single programs so that we could ignore the repetitions of the same data again and again. Moreover, it provided us with a better chance to understand the professional use of the methods in real-life problems. We hope you learned a lot. Stay with us for more learning.