We'll go over a couple more arithmetic functions and complex numbers in this lesson. I will try my best to keep it simple. Let's get started!
Python round function
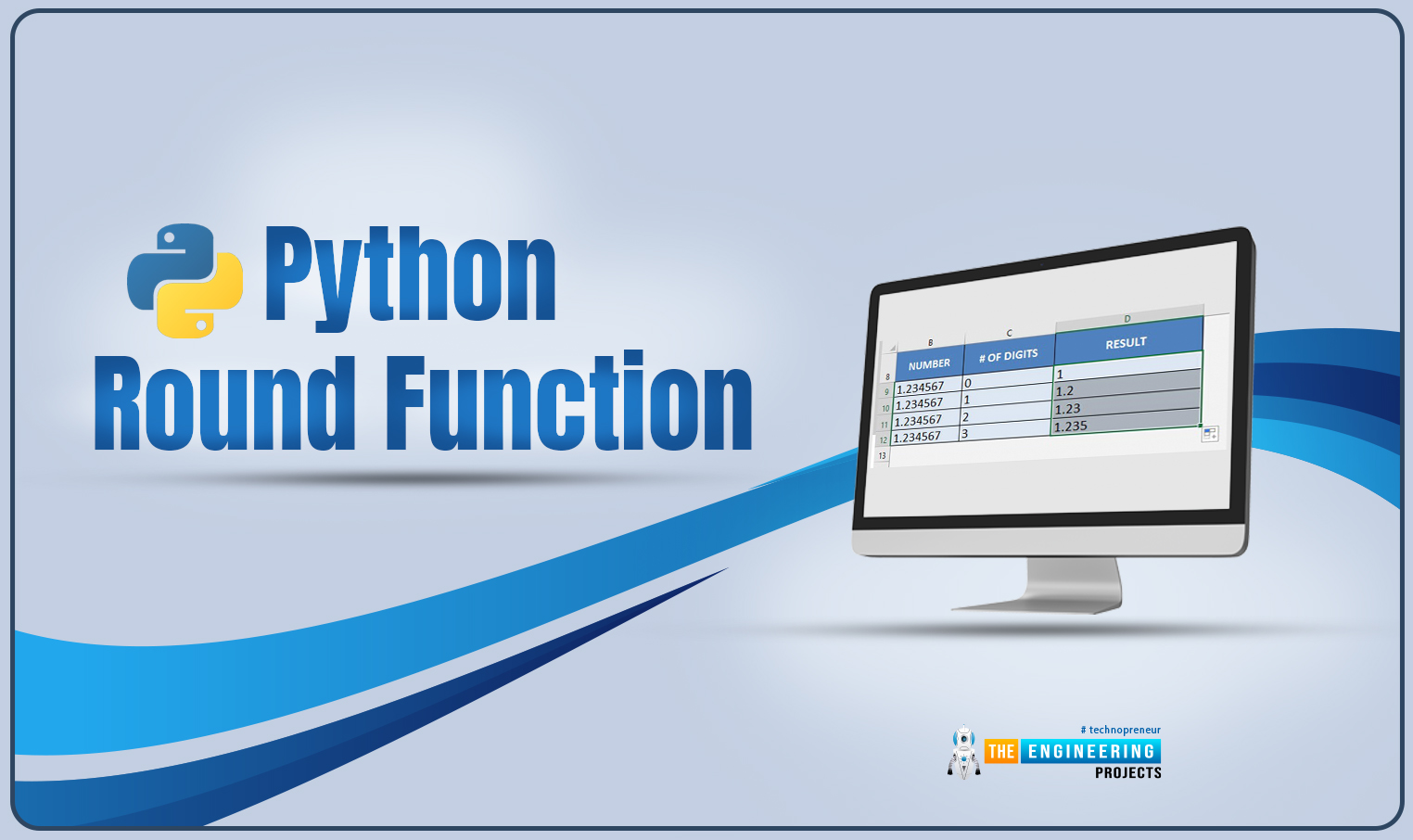
- Rounding to a specific number of decimal places can be done with round().
- abs(), which returns a number's absolute value.
- pow(), which raises a number to a certain power
As an added bonus, you'll discover how to test for the existence of an integer value using a floating-point number.
It's possible to round a number with round():
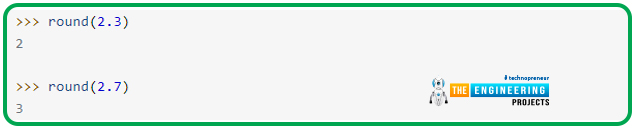
Round() acts strangely when the integer ends in .5.
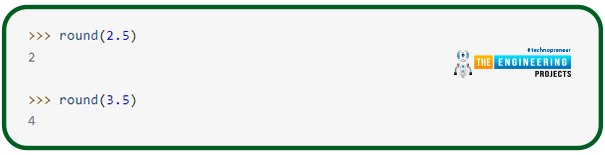
2.5 is reduced to 2, and 3.5 is increased to 4. We'll dig a little deeper into this because most people assume that a decimal number ending in .5 is rounded up.
Python 3 uses a mechanism known as rounding ties to round numbers. The term "tie" refers to any number with a fifth digit. 1.37 is the only one that is not a tie.
One decimal place left of the last digit is all that is needed to break even integers down into their constituent parts. You round to the nearest whole number if the digit is even in this case. When an odd-numbered digit is entered, you round up. Hence, a reduction of 2.5 rounds to 2 and a rise of 3.5 rounds to 4.
When dealing with floating-point numbers, the IEEE advises against using anything but rounding ties because of their reduced influence on procedures involving a large number of values.
IEEE – what is it?
With over 350,000 members from over 150 countries, the IEEE is the biggest technical professional society in the world. An organization committed to the advancement of electrical and electronic engineering and computer science theory and practice has been established.
IEEE 754 is an IEEE-maintained standard for using floating-point integers on computers. It was first published in 1985, and is still widely used by hardware manufacturers today.
In order to round a value to the desired number of decimal places, a second argument to round() might be utilized.
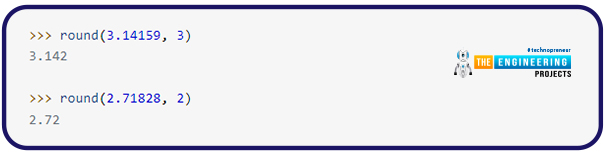
3.142 and 2.72 is the result of rounding the numbers 3.14159 and 2.71828 to three decimal places, respectively.
There must be an integer as the second argument to round() Python raises a TypeError if it isn't.
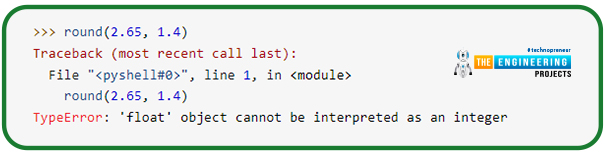
In some cases, round() does not get the answer quite right:

Because it is exactly halfway between 2.67 and 2.68, the number 2.675 is a tie. The expected result of round(2.675, 2) would be 2.68, but Python produces 2.67 instead since it rounds to the nearest even value. Errors in floating-point representation are to blame, not a rounding problem ().
Floating-point numbers are irritating, but this isn't a problem specific to Python. C/C++, Java, and JavaScript are all affected by the same flaws in the IEEE floating-point standard.
Although floating-point numbers have a small amount of error, the outputs for round() are still useful in most cases.
How do we find the absolute value using abs()?
If n is positive, n`s absolute value is n, and if n is negative, it is -n. Examples include 3 and 5, which each have their own distinct absolute values.
In Python, abs() is used to get the number's absolute value.
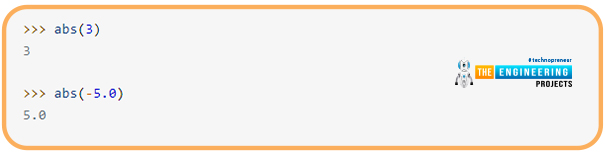
A positive integer of the same type as its input is returned by the abs() function every time it is invoked. To put it another way, when it comes to absolute values of integers and floating points, they are both always positive integers.
How do we use pow() to raise a power?
The ** operator was previously used to raise a number to a power. If you want, you can use the pow() function instead.
There are two arguments to pow().
In order to raise 2 to its exponent 3 in the following example, we can utilize the pow() function.

It is possible to have a negative exponent in pow():

** and pow() are two different functions, so what's the difference between them?
With a third optional input, the pow() function takes the first number as a modulo, and then computes the second number's power. If (x ** y)%z is what you're looking for, then Pow(x, y, z) is the same thing. To illustrate, consider the following scenario:

Eight is the result of raising two to the power of three. 8 % 2 returns reminder 0 since 2 divides 8 by itself.
Check if a Float Is Integral
Functions like .lower(), .upper(), and .find() may be familiar to you. There are also ways for converting between integers and floating-point numbers, as well.
There is a handy number approach that isn't utilized very often .is_integer() method of floating-point numbers. In this case it returns True, otherwise it returns False.
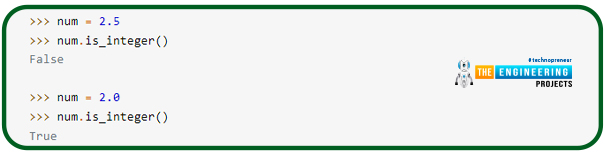
.is_integer() can be used to verify user input. When placing an order for pizza, you'd need to make sure that the customer entered the correct amount of pizzas in the order form.
Using the built-in functions round(), abs(), and pow() does not require any additional imports. But these are just three of the many functions available in Python for manipulating numbers.
Check Your Understanding
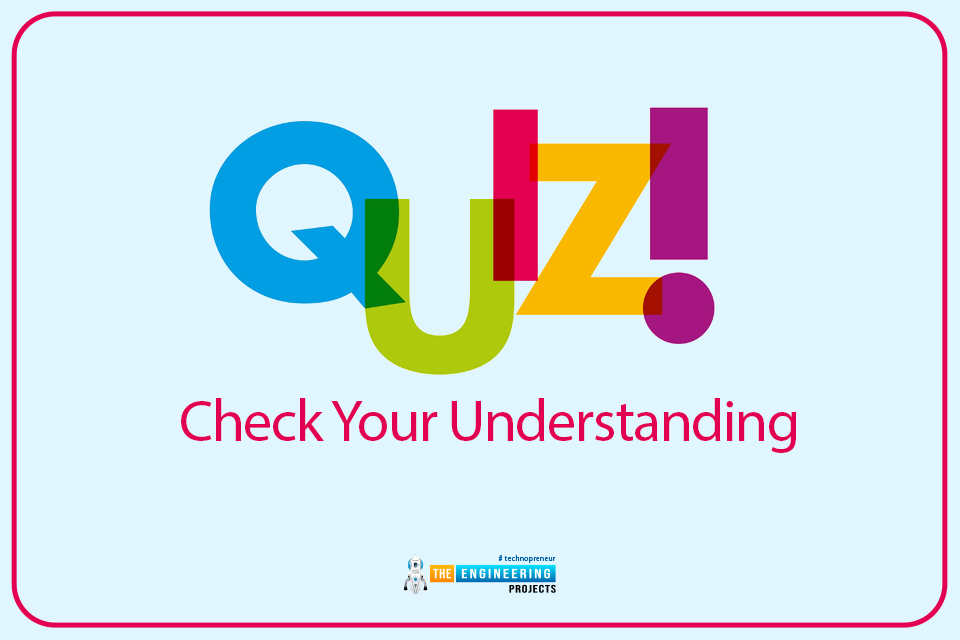

Solution:
In order to get user input, use input():

A last blank space can be seen at the conclusion of the prompt string, for your convenience. This guarantees that the colon in the prompt is separated from the user's input when they begin typing.
It is necessary to first convert the input() value to float before rounding it:

If the user input string does not contain a numerical number, the above code thinks that it does.
The value can now be rounded to two decimal places using the round() method.

To round an integer, pass it as the first parameter to the round() function. You can choose how many decimal places you wish to round to in the second input field.
Using an f-string, enter the rounded number to print the result.

Even though round() is fantastic, if you're only interested in rounding numbers for display purposes, you'd be better off utilizing the methods mentioned below.
Formatting language.
Languages for document formatting determine how printed text and visuals should be organized. Text formatting notation, page description languages, and, most broadly, markup languages are all subclasses of markup languages that specify the intended purpose of a document.
How to print python numbers in style.
When a user requests a list of numbers, they must first enter those values into a string. To do this using f-strings, you can use curly brackets to surround the variable assigned to a number:

A simple formatting language is supported by those curly brackets, which can be employed to change the appearance of the final formatted string.
Instead of using curly brackets to format n to two decimal places, use n:.2f instead.

An extra colon (:) indicates that everything following it is part of a special formatting rule. As you can see, the.2f standard is used in this case.
A fixed-point number is displayed in Python using .2f since the .2 will truncate the result to the nearest tenth of a decimal place. If the number is less than two decimal places, there will still be two decimal places displayed.
The answer to n:.2f is 7.12 when n is 7.125. Python rounds to the nearest integer, just like round() does, when it comes to formatting integers in strings. If you substitute n = 7.126 for n = 7.125 in n:.2f, you get 7.13:

Replace .2 with .1 to get the decimal rounded up to one place:

Your chosen decimal place count is always displayed in the fixed-point number.
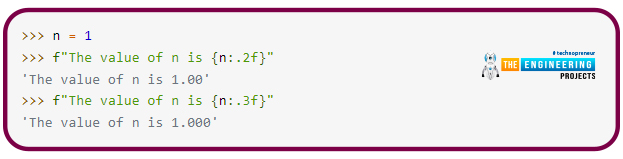
The , option allows you to use a comma for separating the integer portion of huge integers by thousands:

The , should be included before the .in in your formatting specification when rounding off or grouping by thousands.

Currency values can be displayed by using .2f.
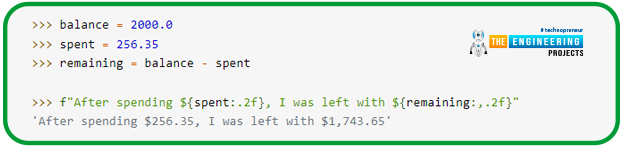
% Is a useful option for displaying percentages.
Using the percent option at the conclusion of your formatting specification is mandatory, and you cannot use the f option with it. As an illustration, .1% shows a number with one decimal place exactly:
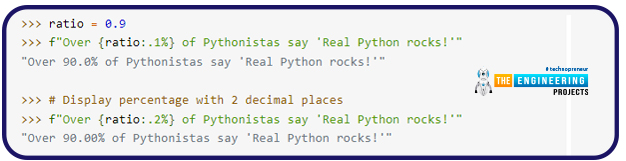
Check Your Understanding

Group thousands by commas when printing the number 150000. There should be two decimal places on all currency displays, and the dollar sign should always appear first.
Solution:
One step at a time, let's build up our F-string.
F-string 150000 without any formatting looks like the following:

Set yourself prepared to add the formatting specifiers by putting this in place first.
It is possible to display the value as float by using a colon (:) after both 150000 and letter f.

A precision of six decimal places is the default setting in Python. There should only be two decimal places in your currency, so you may just add . 2 between the : and the f:

Make sure the number is shown in its entirety by including a colon (:) after the number and before the period (.).

There should also be dollar signs ($) to indicate that the price is in US dollars.

Complex Numbers
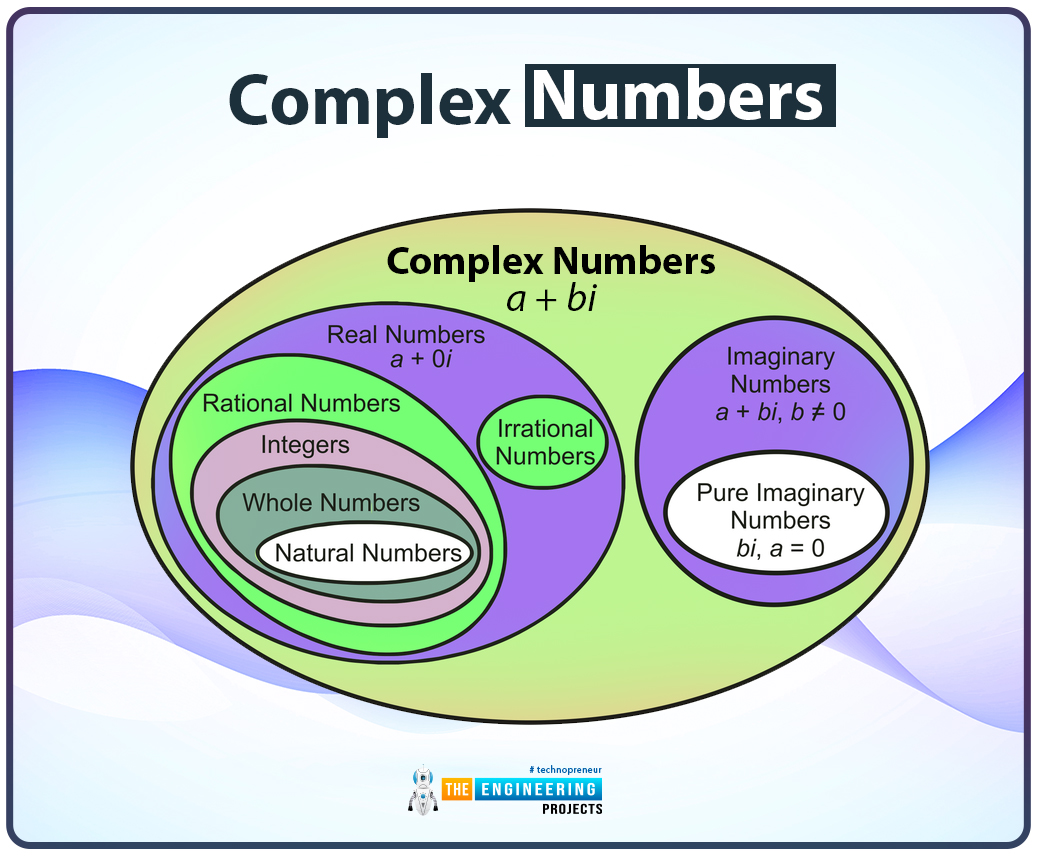
Because it is so uncommon in other programming languages, Python has support for complex numbers right out of the box. Python's support for complex numbers, while uncommon outside of scientific computing and computer graphics, is a major plus for the language.
It is common knowledge that a complex number contains two components: a real component and an imaginary component.
When writing complex numbers in Python all that is required is to write the real component, the plus sign, and then the imaginary section with the letter j after them.

This is what we see when we look at the number n in Python's code:

Thus, the output is no longer misinterpreted as a mathematical expression when it is shown in this way.
The real and imagistic components of an imaginary number can be retrieved using the .real and .imag characteristics:

Even though the real and imaginary components were defined as integers, Python nevertheless delivers them as floats.
The conjugate of a complex number can be found using the .conjugate() method.

Find the complex number whose real part and imaginary portion are the same as the opposite-sign complex number's conjugate.
Unlike .conjugate(), the .real and .imag properties do not require parentheses following their names.
Instead of calculating a complex number, the .conjugate() method returns data about the number, while the .real and .imag methods just provide information.
In object-oriented programming, the distinction between methods and properties is critical.
Float and integer arithmetic operators, with the exception of the floor division operator (//), all function with complex numbers. For the sake of keeping things simple, complex arithmetic's mechanics are outside the scope of this article. Rather, consider the following arithmetic examples that demonstrate the use of complex numbers and operators:
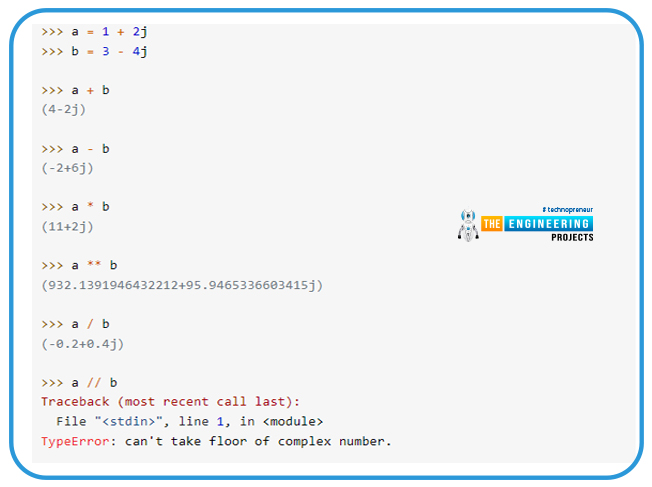
The .conjugate() method for int and float objects is interesting, but not surprising, from the perspective of mathematics.
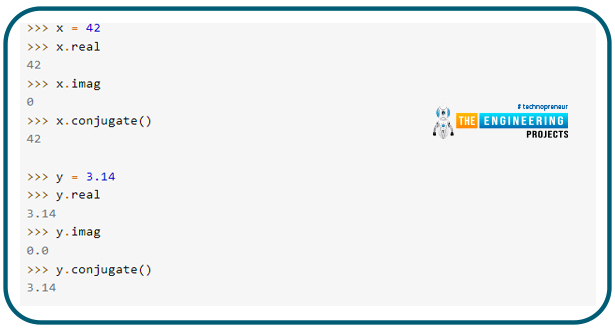
Conjugated numbers are returned when using .real and .conjugate(). However, while using .imag, it always returns 0. As long as the number is an integer, .real and .imag will return integers; if it is an unsigned int, they will return floats as long as it is an integer.
It's possible that you're wondering when you'll actually need to employ complex numbers. In Python, you may never have to deal with complex numbers unless you're doing data analysis or web development.
Science and computer graphics necessitate complex numbers for computation. Because of this, when dealing with complex numbers, Python's built-in support is handy.
Conclusion
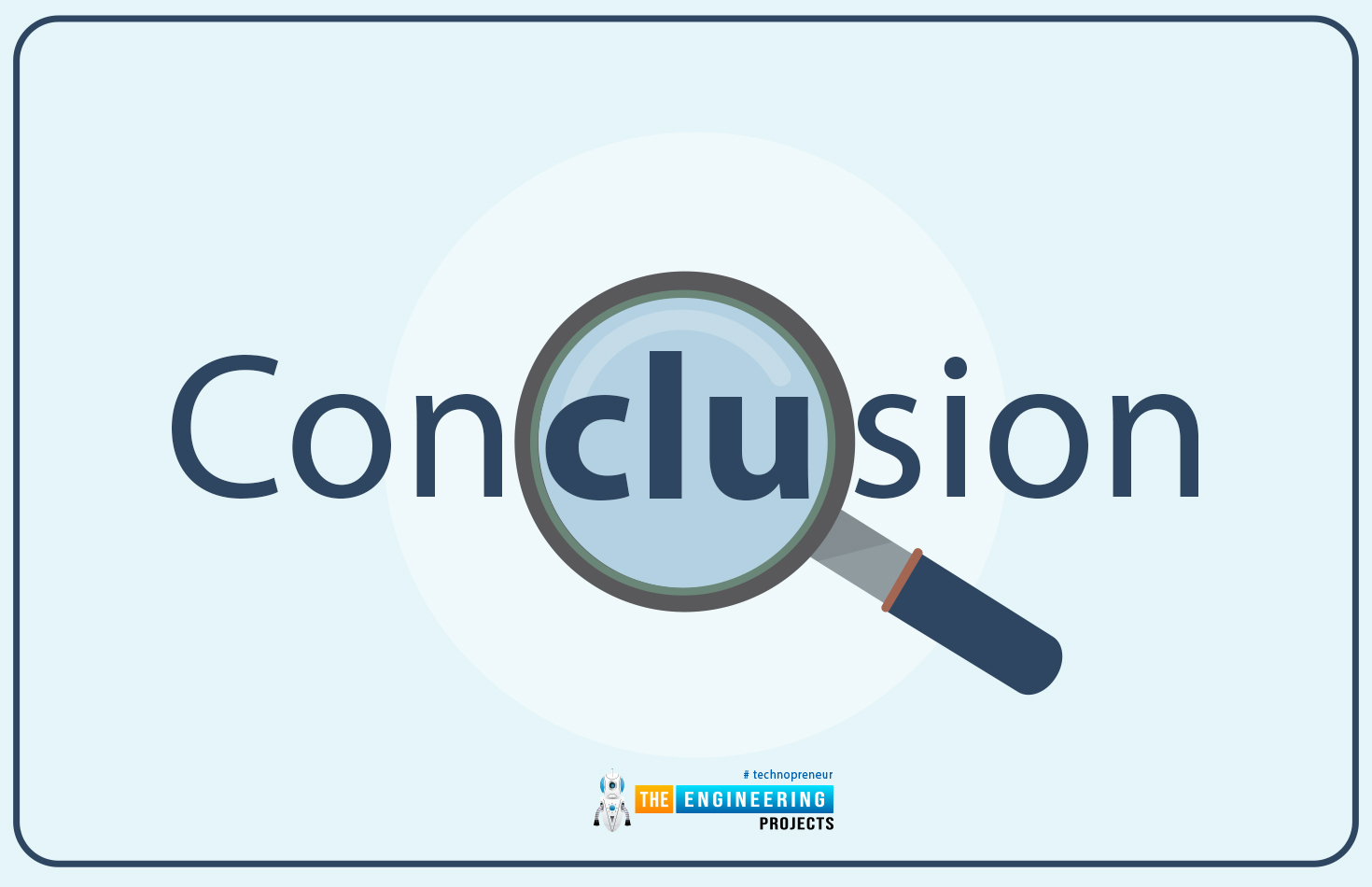