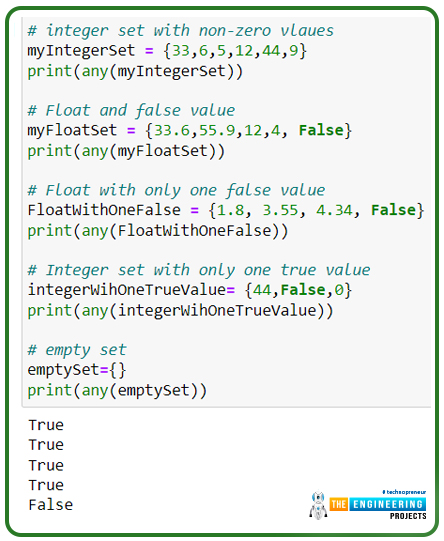
Hey people! Welcome to another tutorial on Python programming. We hope you are doing great in Python. Python is one of the most popular languages around the globe, and it is not surprising that you are interested in learning about the comparatively complex concepts of Python. In the previous class, our focus was to learn the basics of sets, and we saw the properties of sets in detail with the help of coding examples. We found it useful in many general cases, and in the present lecture, our interest is in the built-in functions that can be used with the sets. Still, before going deep into the topic, it is better to have a glance at the following headings:
What are the built-in functions?
How do we perform the all() function with examples?
What is the difference between any() and all()?
What are the parameters of sorted()?
Built-in Functions with Sets
From the beginning of this course, we have been using the built-in functions for different purposes, but here, the main idea must be cleared, so for the purpose of revision, have a look at the basic definition of these functions:
"Built-in functions are the pieces of code that have a particular result all the time and are pre-defined in the programming languages so that the programmer does not have to type the same code but simply put the values in the functions and get the required output as expected with minimum effort."
For a better understanding of the sets and the practice of these in codes, the best way is to use the built-in functions so that whenever you are coding these built-in functions arise instantly into your mind and the effort to code the same line again and again in different programs. There are certain types of built-in functions, the details of which were covered in the previous lecture, so for now, we are trying to introduce the new functions and repeat just the most common ones with the sets.
all() Function with Sets
The first function of discussed by us is very interesting and has application in many general cases. This function checks the element of the set one after the other and on the basis of a combination of results, it provides the output. The working of all() is a little bit tricky. Consider the case when we define a set with a particular data type and all function and then keeps that data type in the memory. Afterwards, it checks the result by following the sequence of combinations and then provides a single output in the form of true or false. The combination of all() is given in the table:
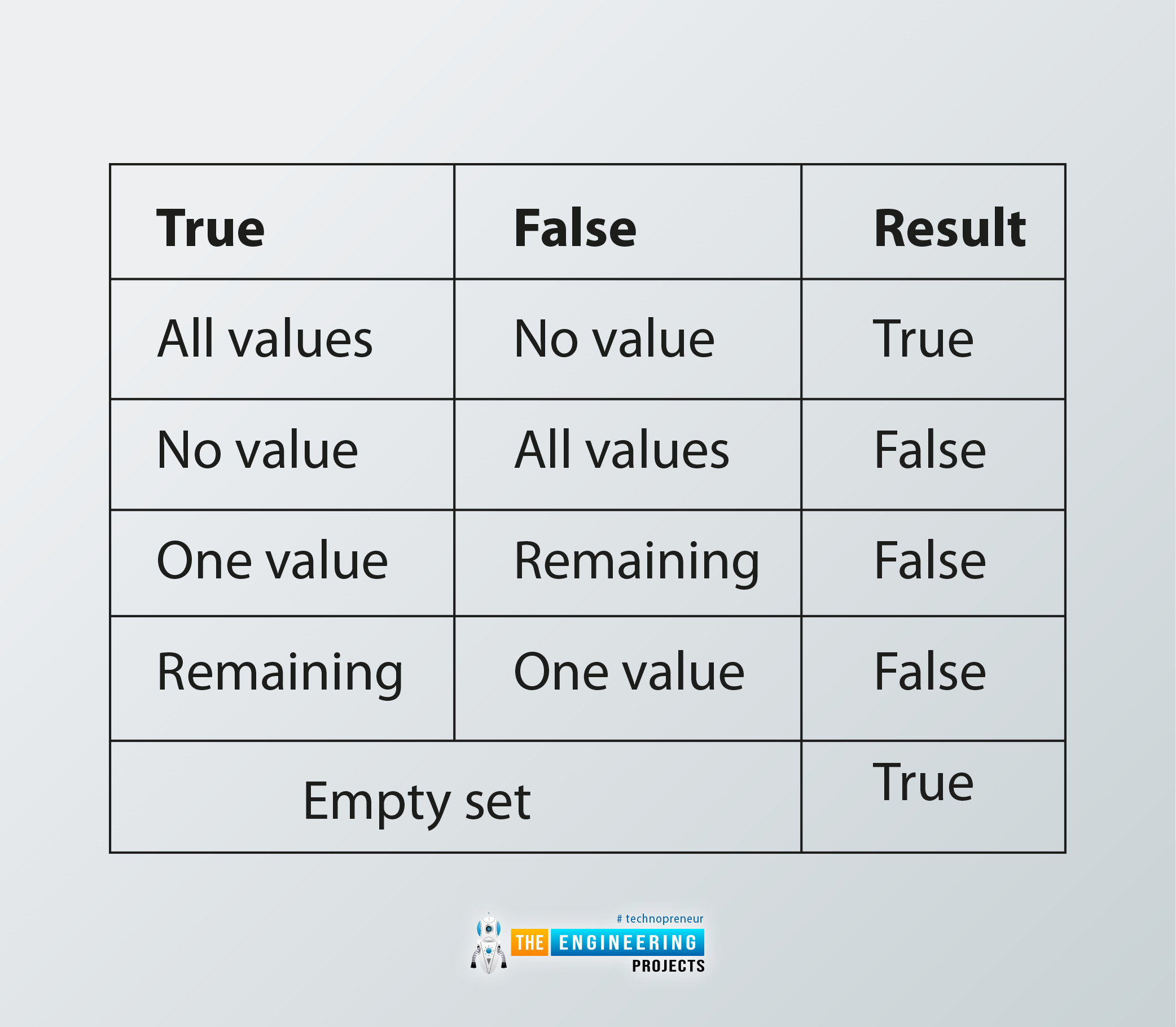
True |
False |
Result |
All values |
No value |
True |
No value |
All values |
False |
One value |
Remaining |
False |
Remaining |
One value |
False |
Empty set |
True |
Hence, just like the logical gates, the all() function works on the logics, and this will be proved when you see these examples:
all() Examples in Set
Before going into the details of the all() function, have a look at the code where only integers anf floats are used with different values.
# integer set with non-zero values
myIntegerSet = {33,6,5,12,44,9}
print(all(myIntegerSet))
# Float and false value
myFloatSet = {33.6,55.9,12,4, False}
print(all(myFloatSet))
# Float with only one false value
FloatWithOneFalse = {1.8, 3.55, 4.34, False}
print(all(FloatWithOneFalse))
# Integer set with only one true value
integerWihOneTrueValue= {44,False,0}
print(all(integerWihOneTrueValue))
# empty set
emptySet={}
print(all(emptySet))
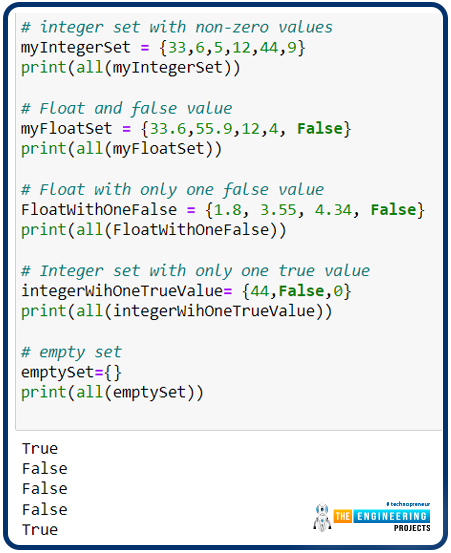
The following points are extracted from the results given above:
The digit zero is always considered false if you are using the float or integer sets.
The all() function checks each and every element and stores the results in memory, then checks for the combination and provides the results.
It is obvious that if the set contains the value "false,” then it will be considered the false function, and the compiler will not consider it a string.
The empty set is always true.
Exercise
Now, this can also be performed with the string sets, and all the strings will be considered true. Hence, any element with double quotation marks will be considered a string, and the compiler will provide the output in the form of a single result.
Your task is to design the code using the information given above and check the results by matching the table given above.
any() Function with Set
If you have understood the previous function, then this will be more fun for you because, in some cases, we can say that the any() function is the opposite of the all() function. Therefore, they are named so. So, look at the code of the any() function, where only the name of the function is being replaced, and you will see the clear difference in the results.
# integer set with non-zero vlaues
myIntegerSet = {33,6,5,12,44,9}
print(any(myIntegerSet))
# Float and false value
myFloatSet = {33.6,55.9,12,4, False}
print(any(myFloatSet))
# Float with only one false value
FloatWithOneFalse = {1.8, 3.55, 4.34, False}
print(any(FloatWithOneFalse))
# Integer set with only one true value
integerWihOneTrueValue= {44,False,0}
print(any(integerWihOneTrueValue))
# empty set
emptySet={}
print(any(emptySet))
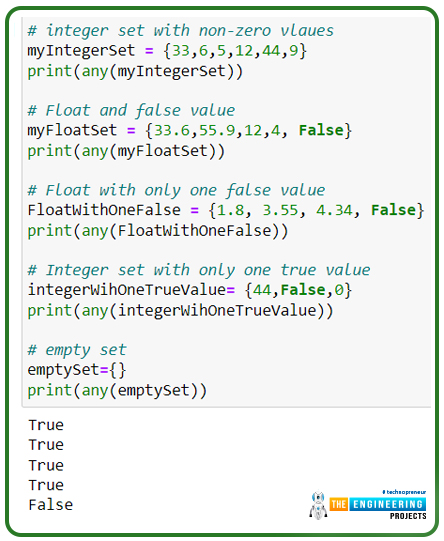
The results can easily be understood, and this time, we have extracted the table of combinations from the output of our code:
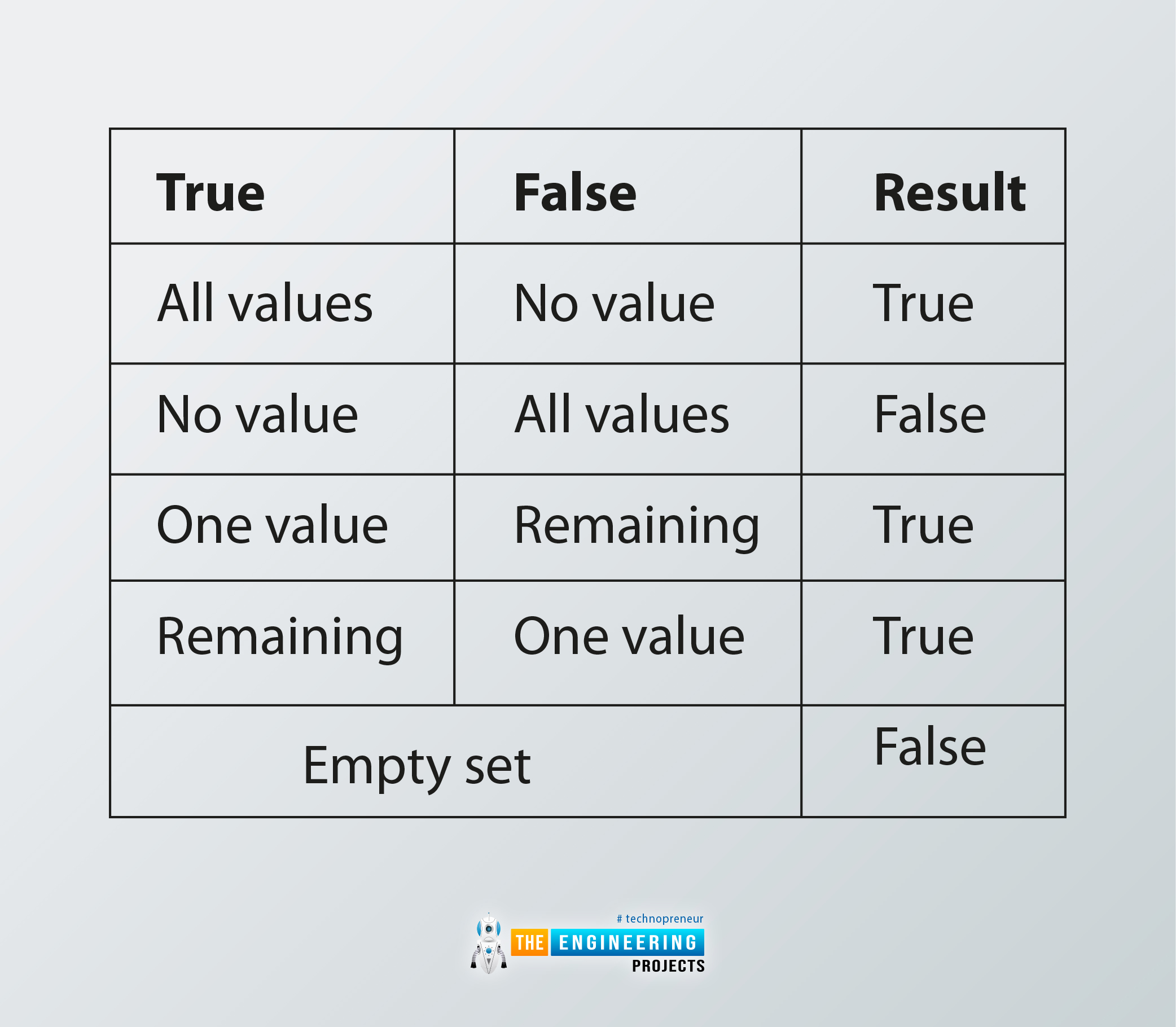
True |
False |
Result |
All values |
No value |
True |
No value |
All values |
False |
One value |
Remaining |
True |
Remaining |
One value |
True |
Empty set |
False |
So, the same task is for you as we have given the exercide with the all() function.
enumerate() Function with Set
It is a different kind of function and is meant to represent the set in a better manner. While using it with the set, the output is obtained has the number of index with it automatically. It is useful in the long sets where the number of elements matters, and by using this, the programmer does not have to do the counting manually, but the index number is shown with each element. For this, the results are saved into the variable, and then the results are fed into the list. If it is confusing at the moment, have a look at the code given next:
#Making the set of books
Books={'Biology', 'Chemistry', 'Physics', 'English', 'General Knowledge',
'geography', 'poems', ' grammer','programming', 'software', 'technology'}
#declaring the varialbe
i=enumerate(Books)
#using the varialbe in list and printing the results
print(list(i))
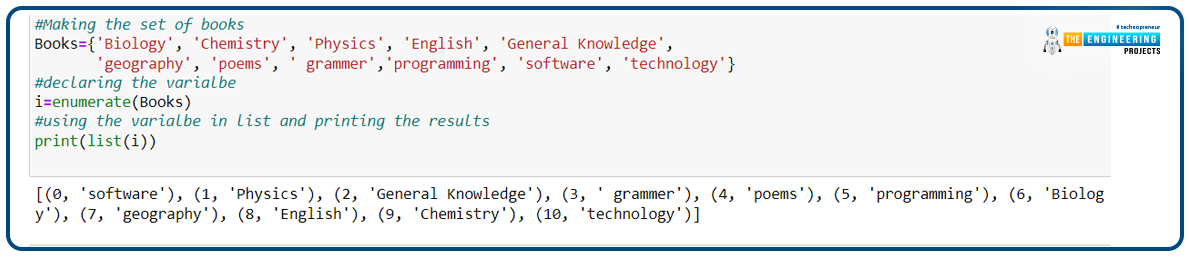
So, all the books are arranged and have an index according to their position. Take the case into your mind, where the set contains hundreds or thousands of elements, and the indexing helps us to understand the collection.
sorted() Function with Set
The next function that is going to be discussed is the sorted() function, that plays an important role in the numeric calculations. Usually, programmers feed the data in an unordered manners.
Example of Sorted() Function
If all the discussion above is not enough for you to understand the whole concept, then do not worry because these examples and their explanation will help you understand the concepts deeply.
Sorting of Numbers
It is a simple task, and the sorting is easy when you have few elements, but with the complex cases, the sorting needs logic. You will see some examples with the parameters in the same lecture, but for a start, have a look at the code below:
So, the sorting process of integers and
#Declaring the set
print("The unsorted set = ", myIntegers)
print()
#Using sorted() function on the set
print("The sorted set= ", sorted(myIntegers))
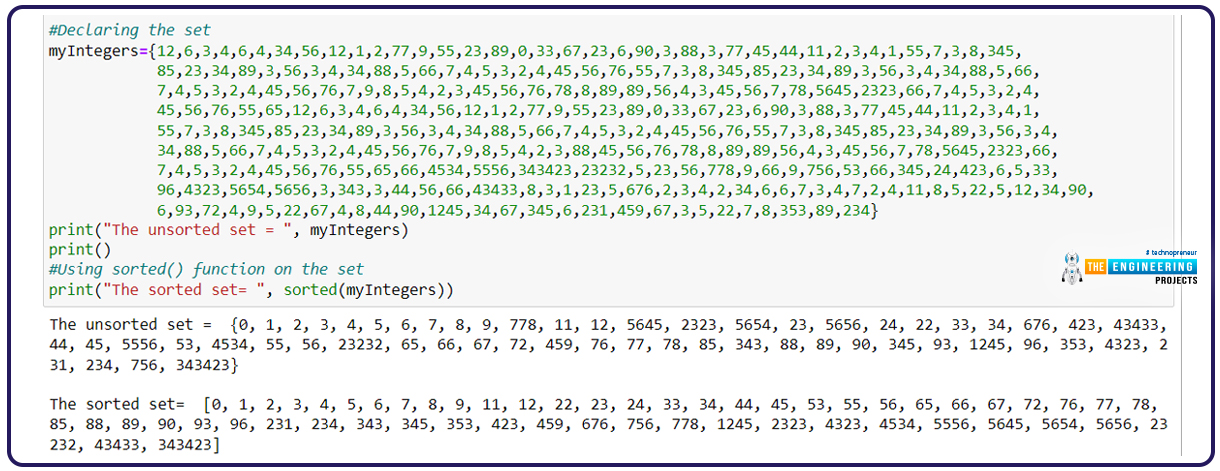
This is the big array, and it is difficult to arrange it manually; therefore, we are using sets here to eliminate the duplicate elements and to sort them with the help of a simple command.
Once the set is complete, it becomes difficult to read and understand the data of the set if it is large. In such cases, the sorted function is used, which sorts, or, in simpler words, arranges the data into a specific order. To learn about the syntax of this function, you must know three parameters:
Iterable Parameter in sorted Function:
This is the general term used to present a collection of things, groups, loops, or sequences. These are the necessary parts of the sorted function, and as “set” is the collection of data types, we can use it in this function to sort the elements according to our wishes. Without this parameter, the compiler will show the error.
Key Parameter in Sorted Function:
It is a relatively difficult parameter to grasp, but it is responsible for the function's versatility. It may be tedious to realize that the set you are entering is only sorted in ascending and descending order. In programming, the data is stored in the form of different collections of numbers, and the key is the way to tell the compiler which pattern must be followed for sorting the data. This will be clear with the help of an example, and after that, we will examine the results:
Reverse in sorted() Function:
The second parameter in the sorted() function is optional, and it is used to ask the programmer about the way to sort the data, that is if the programmer needs the iteration in an ascending or descending manner. It just has two possible inputs: true or false. As the name of the parameter indicates, if the reverse is set to true, the results will be in reverse order, that is, from the highest value to the lowest. But by default, it is always true, and if the programmer inputs true or even does not use this parameter, the output of the set will be in ascending rather than descending order.
# My string list declaration
mySet ={ ('apple'), ('red'), ('black'), ('strawberry')}
# sort set taking length as key and reversing the order
Result = sorted(mySet, key=len, reverse=True)
# print set
print('Sorted results:', Result)
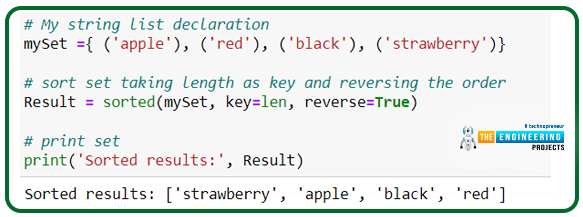
So, it was an interesting tutorial on the sets, and with the help of different examples, we have learned built-in functions such as all(), any(), and the sorted function.