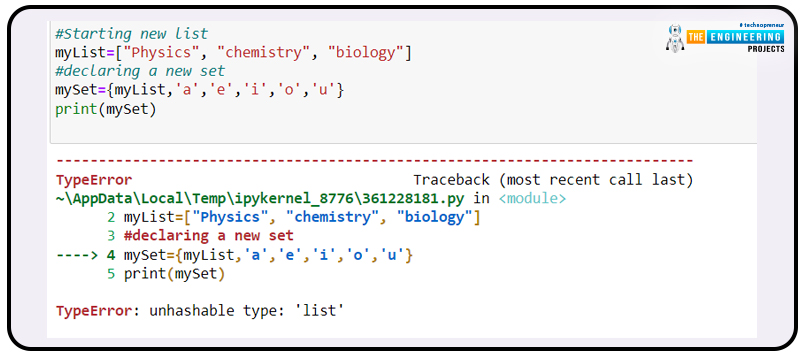
Hello students! Welcome to the new tutorial on Python. We all know that Python is one of the most popular programming languages, and there are hundreds or thousands of developers that are earning a handsome amount with the help of this easy programming language. In the previous lecture, we studied the range in the sequence, and in the present class, our concern is having the command on the sets in Python. We know you are curious about the set's details, but before this, I want to share the list of topics that will be covered in this class.
What is a set in the Python programming language?
What are some properties that distinguish the set from other data types?
What is the mutable data type, and how is it related to the set?
Introduction of the Jupyter notebook.
Can we have duplicate elements in the set?
How to add, remove, and update the elements in the set while using the Jupyter notebook.
How can we access the elements using a loop?
Give an example of how to use the length function with sets and why it is important.
All of these are important interview questions, and we will not only find the answer to them but also elaborate on them with the help of simple but understandable examples taken from daily life routines. Your duty is to perform each and every code, not only by copying it from the lecture but also test your knowledge and practising more and more by making your own examples.
Introduction to Sets in Python
Since the last few tutorials on Python, we have been studying a lot about the sequence, which is basically the representation of a collection of data types with homogeneity or heterogeneity in the elements. If we talk about the sets, these have the same properties and procedures as their other group, such as list and range, but a slight difference in their property makes them a different data type. This can be elaborated with the help of its basic definition:
“The set is the type of sequence that contains the group of different data types, and it is the collection of unordered or unindexed data types together.”
Until now, the sequence discussed had been represented exactly as it was written by the programmers in the code. Yet, in the sets, the order is not exactly the same all the time. If you are thinking it is strange, then you must know, in the higher level of programming, this property of the set works great because we get the elements in random orders.
Another difference between the set and the other sequences is the usage of the bracket, or, in other words, the declaration of the sequences. To tell the compiler that we want a set in the sequence, the programmers use curly brackets. You must have noticed that it is very rare to use curly brackets in Python, and therefore we can say that the representation of the set in Python is unique.
Properties of Set Sequence in Python
As we have a lot of information about the sequences, we can openly discuss the properties of the set, and the reader will easily understand them by comparing them with others. So, here are some of the properties that can be compared:
Sets are represented with curly brackets.
The elements of the set can not be duplicated; that is, all the elements are uniquely defined, and no element should be repeated; otherwise, the compiler will show the output in which the duplicate values are shown only once.
The set is a heterogeneous collection of elements, and therefore, the programmers can add one or more data types to a single set according to their choice.
The set can be empty, that is, declared with zero elements.
The set can be updated after its formation if the programmer wants to make some changes to it afterwards.
There are certain built-in functions of the set that, when used with the sets, have great applications in Python programming.
Each of these properties can be explained well with the help of TensorFlow. We have been using the Jupyter lab of TensorFlow since the start of this tutorial, and now, I want to tell you a better and more professional way to run the code with the help of TensorFlow. For this, you do not have to install any other software but the Jupter notebook already installed on your PC. Simply go to your search bar and run the Jupyter notebook. It will add a new tab with the label "home." Here, go to the “New” dialogue box and select Python 3. This will add the new project to a new tab. You can name it, but by default, it is named "untitled."
If you are practising all the codes with us by hand, you will observe that the Jupyter notebook has a better user experience, and it adds the ending of common syntaxes such as the double quotation and parentheses by itself when the programmer starts them. We will talk more about it in later lectures, but for now, we are moving towards the codes and properties.
Sets and Mutable Objects in Python
The first thing that we want to revise here is the definition of mutable elements:
“In programming languages, mutable objects are those that are used to group different items and can change their value according to the instruction of the programmer.”
We have learned many mutable sequences, such as lists, and here, the point is to revise it to a set and not use the mutable sequences as the elements. Only data types such as strings, integers, etc. can be used as the elements in the set; otherwise, the programmer will face an error. This can be explained with the help of the code given below:
#Starting new list
myList=["Physics", "chemistry", "biology"]
#declaring a new set
mySet={myList,'a','e','i','o','u'}
print(mySet)
As a result, it is demonstrated that programmers can combine simple data types into sets, but it is not possible to create collections of mutable objects or collections of collections within sets.
Duplication is Not Allowed in Set
In the properties, we have mentioned that the process of feeding the duplicate elements into the set is not useful because it checks for each and every element while providing the output, and if the element is being repeated, the sets ignore them. As a result, if we have the element more than once in our input, the number of elements in the input and output are not the same.
#Declaring the set
MySet={21,23.6,55,'Peach', 'Almond', 23.6,21,'Almond'}
#using iteration to print the set
for item in MySet:
print(item, end=" ")
print()
#calculating the length
length=len(MySet)
print('Numbers of elements = ',length)
This property will be more clear with the help of the following screenshot:
Hence, out of eight elements, the two duplicate elements are removed by the compiler, and we only get five elements that were calculated by the length function.
Addition Method in Set
This is an interesting method that is compatible with the set in Python. Consider the situation where the programmer has declared a set and then needs to add an element to the same pre-defined set. In such cases, the addition method is useful, with the help of which the programmer simply uses the syntax of the add method and there is no need to recreate the whole set again.
NameOfSet.add(element to be added)
If the question arises about the position of the element, this will be clear with the help of an example that we are going to check:
#Initializing the set
mySet={'eggs', 'bread', 'jam',23,67,132,55}
print('Elements of my set is= ', mySet)
#adding a new element
mySet.add("oats")
#printing the set with the added element
print('Elements of my set with new element= ', mySet)
Removing the Element From Set
Keep the scenario in your mind that we have discussed above, but this time, there is a need to remove the lament from the set, and for this, Python has another method that simply searches for the required element from the set and removes it. Afterwards, the results can be printed on the screen to check whether the task is complete or not. The keyword to remove the element is "discard,” and it is used in the same way as the add keyword.
#Initializing the set
mySet={'eggs', 'bread', 'oat','jam',23,67,132,55}
print('Elements of my set is= ', mySet)
#removing the element "oat"
removeValue=mySet.discard('oat')
#printing the set with the removed element
print('Elements of my set with discarded element= ', mySet)
So, the removal process is also very simple and understandable but the syntax must be kept in mind and before using the final set in this case, always check for the results by printing the elements on the screen as we are doing here because a little mistake on the syntax results in no removal and it may cause the problem in the code. So it is a good practice to have an eye on the elements.
Updating the Elements of the Set
The updating process of the set may include different types of updates, such as increasing the size or changing the elements' sizes. For a better understanding, the best way is to learn how two or more sets can be merged into one large set. In the previous lectures, we have seen this type of process where merging is done with the help of a method. To discuss a new method with you, here we are using the update method. The process and syntax are the same as we have seen in the previous two methods.
setToBeAdded.update(setToBeUpdated)
As a result, the final set has elements from both of these sets. But it is important to notice that both sets have to be declared first, and in the third step, we get the merged or updated search with the help of the command given above.
#Initializing the first set
myFirstSet={'eggs', 'bread', 'oat', 'jam',23,67,132,55}
print('Elements of first set is= ', myFirstSet)
#Initializing the second set
mySecondSet={'Python', 'Java', 'C++'}
print('Elements of second set is= ', mySecondSet)
#Updating the sets
myFirstSet.update(mySecondSet)
#printing the final set
print('Elements of final set= ', myFirstSet)
Hence both of these are merged together and as we are using the sets, the order of the final set is different and unarranged. Well, it is a good practice to check for the numbers of elements using the length function all the time.
For Loop in Set
We hope by now you have an idea of the for loop and how we use it with different data types in Python. Similar to the list, the programmers can access each and every element with the help of iterations (loops). So, let us review the elements of a set with the help of the for loop.
#declaring our set with the name to-do list.
ToDoList={'assignment', 'coding', 'prayer', 'washing cloths', 'doing dishes'}
#starting for loop
for work in ToDoList:
print(work, end=" ")
If we look at the output, we get the following results:
Hence, it was an interesting tutorial on the sets where we learned a lot about the topic and the details were interesting and related to our daily life. At the start, we saw the basic definition and a brief introduction to the topic. We have seen some properties of the sets that were resembling the types of sequences but these were also different in many ways and we not only studied them in detail but practically proved them in the Jupyter notebook. It was nice to use the Jupyter notebook that we are going to use onward in this series. In the next tutorial, we will put light on some other features so stay tuned with us because we are preparing our next lecture on Python.