We have already discussed the variables in detail so I hope that you must have the idea of what variables are and if you don't then you must read the previous tutorials first. The code is also given below in the tutorial. So, let's get started with How to use C# String Variables:
How to use C# String Variables ???
- C# also supports string variable, a string variable can save anything in it in the form of characters.
- C# String variable can be of any length.
- So, let's design a small code to show how C# String works.
- First of all design a simple C# Project and add one button and one text box in it, as shown in below figure:
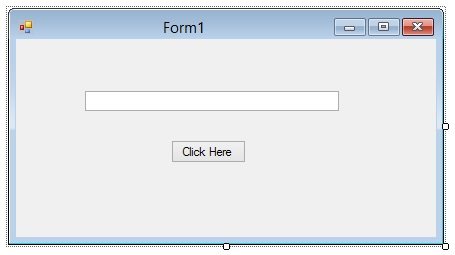
- Now change the Text of your Button to Click Here and Name of the button to ClickHere.
- Similarly change the Name of the text box to txtClick.
- We have already designed a similar project in How to use C# Int variables.
- Now that our project is added so now add the below code into it an your code will look something like:
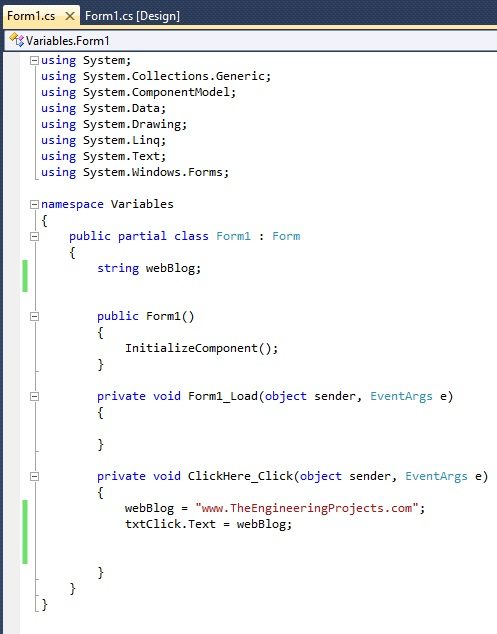
- Now you can see in the above figure, that first I have created a string variable named webBlog.
- After that I have assigned it a value, because the variable is a C# string variable that's why it can save this string easily in it.
- After that I have simply displayed it in that text box.
- You must have noticed that this time we didn't have converted the value to string as we did in C# Double variable.
- Because our variable is already a string.
- Here's the complete code for copying:
using System; using System.Collections.Generic; using System.ComponentModel; using System.Data; using System.Drawing; using System.Linq; using System.Text; using System.Windows.Forms; namespace Variables { public partial class Form1 : Form { string webBlog; public Form1() { InitializeComponent(); } private void Form1_Load(object sender, EventArgs e) { } private void ClickHere_Click(object sender, EventArgs e) { webBlog = "www.TheEngineeringProjects.com"; txtClick.Text = webBlog; } } }
- Now let's have a look at the results.
- So, run your project and then click on the button and the string will appear in the text box as shown in below figure:
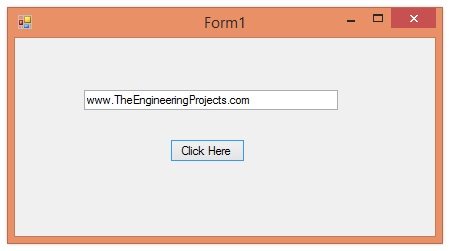
- So that's how C# string variable works.
- Now you can save any kind of long strings in C# string variable.
- Now let's have a look at few of the string properties.
C# String Comparing
- Suppose you have two strings then How to compare them.
- So write your code as shown in below figure:
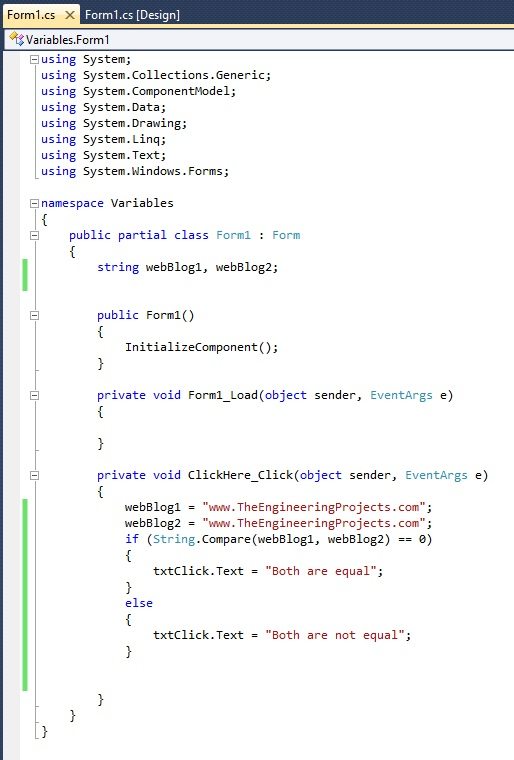
- Now you can see in the above code, I have used two C# String variables webBlog1 and webBlog2.
- I have given the same text to both of these C# string variables.
- Now after that I have used a small function called String.Compare() and it takes two C# string variables.
- After that it compares these two C# string variables and if they are same then it give 0 so that's why I have compared it to 0 means if the two C# string are equal then I have printed Both are equal otherwise not equal.
- So, that's how you can compare two C# String Variables.
- Here's the code for copy:
using System; using System.Collections.Generic; using System.ComponentModel; using System.Data; using System.Drawing; using System.Linq; using System.Text; using System.Windows.Forms; namespace Variables { public partial class Form1 : Form { string webBlog1, webBlog2; public Form1() { InitializeComponent(); } private void Form1_Load(object sender, EventArgs e) { } private void ClickHere_Click(object sender, EventArgs e) { webBlog1 = "www.TheEngineeringProjects.com"; webBlog2 = "www.TheEngineeringProjects.com"; if (String.Compare(webBlog1, webBlog2) == 0) { txtClick.Text = "Both are equal"; } else { txtClick.Text = "Both are not equal"; } } } }
Find subString in C# String
- Now suppose you have a long string and you want to find some small string whether its in the long string or not.
- Then you can use another function named as contain and the below example shows how to use this command:
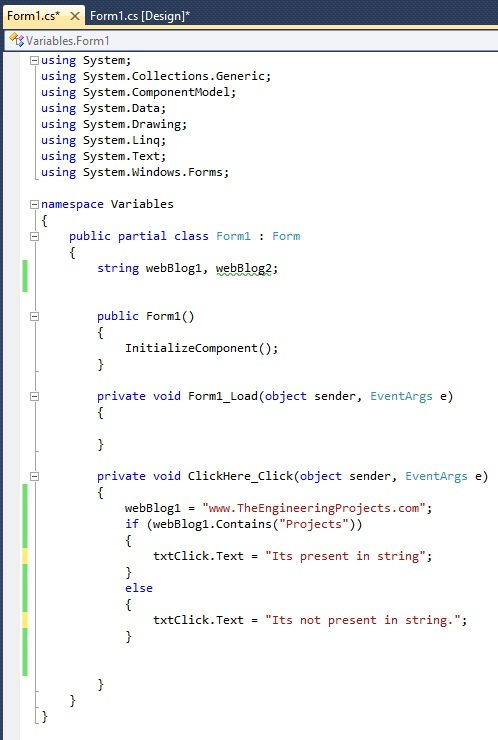
- In the above figure, you can see that I have initialized a C# String variable and given it a string text.
- After that I have used the function String.Contain and checked a part in the string.
- So you can see the String is actually in the main string so it will give Its present in the string otherwise not present.
- So, run your project and then click the button and you will get something as shown in below figure:
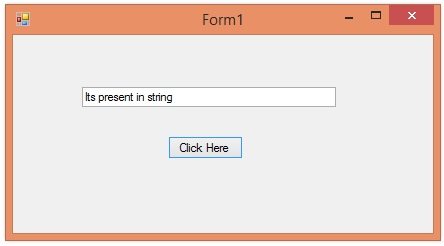
- Now, you can see it has given that its present in the string.
- Here's the code for copying:
using System; using System.Collections.Generic; using System.ComponentModel; using System.Data; using System.Drawing; using System.Linq; using System.Text; using System.Windows.Forms; namespace Variables { public partial class Form1 : Form { string webBlog1, webBlog2; public Form1() { InitializeComponent(); } private void Form1_Load(object sender, EventArgs e) { } private void ClickHere_Click(object sender, EventArgs e) { webBlog1 = "www.TheEngineeringProjects.com"; if (webBlog1.Contains("Projects")) { txtClick.Text = "Its present in string"; } else { txtClick.Text = "Its not present in string."; } } } }
Dividing a C# String
- So, now let's get something out of a string.
- Suppose in some project you have a very long C# string and you just want a part of that C# string.
- Then what you need to use is another string command which is webBlog1.Substring(4).
- So, have a look at the below code:
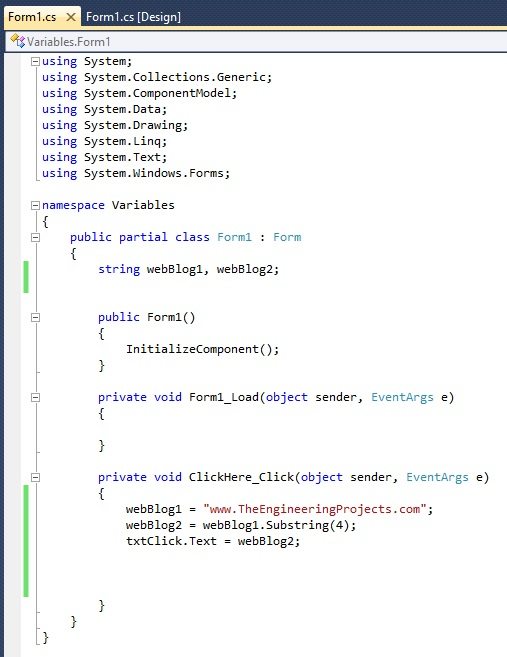
- Now in the above code you can see I have initialized a C# String variable and assigned it a value.
- After that, I have used second variable and then I have subString it by four characters and now run your project and click the button and you will get results as shown in below figure:
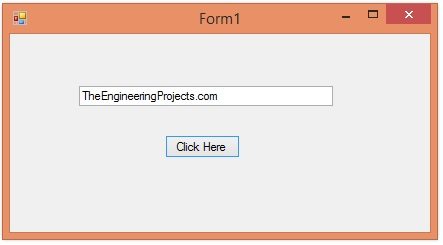
- So, you can see in the above string I have subString four characters in the initials and then the remaining is shown in the text box.
- So, that's how you can take any number of subString from the C# String.
So, that's all about C# String Variable. I hope you guys have learned something out of it. that's all for today. In the next tutorial we will learn more about C#. Till then take care and have fun !!! :)