Now, in today's tutorial, I am gonna introduce you to a new concept which is variables. Variables are used in every programming language and without variables we can't design even a simple code. There are different types of variables available in C# language and today among these variables we are gonna focus on C# Int variables. If you are doing engineering then you must have the idea about integers. :P So, let's get started with C# Int variables. Before discussing the C# Int variables, let's first get an overview of variables.
What are Variables ???
- In any kind of programming projects, there's a need for storing some data in temporary memory spaces. these temporary memory spaces are named as variables.
- For example, I have a simple project in which I need to add 2+2 and then I need to add 2 more in the output.
- So, in that case what I am gonna do is first of all, I am gonna do the simple addition operation and will add the 2+2 and then I need to save the output which is 4 somewhere.
- So, I am gonna save this output 4 in a variable and then I am gonna add 2 more in that variable and again I will save the output which is 6 in another variable and then I can print it on the output.
- I think now its quite clear from the above example that variables are used to store data for a short time.
- Now data can be of any type, it could be an integer or some decimal value or it could be characters.
- So, that's why we have a long list of variable types in C#, which are as follows:
- Int (Integer)
- Double
- Float
- Char
- String
- Byte
- sByte
- Decimal
- Boolean
- You can read more about the variables from Microsoft Variables Page.
How to use C# Int variables ???
- C# Int variables are used for storing integers values. Int is actually a short form of Integer.
- So, for example, you have to save 2 in some variable then as 2 is an integer so you can save it in C# Int variable.
- But if you have 2.05 value then its not an integer because it has a decimal part so you can't save this value in an integer so C# Int variable will only save integers in it.
- Let's design a simple example on C#, as we did in Introduction to C# Windows Forms.
- Now in this project, I am gonna add a Button and a Text Box and on pressing that Button I will print a variable in this Text Box.
- So, design a simple project, as shown in below figure:
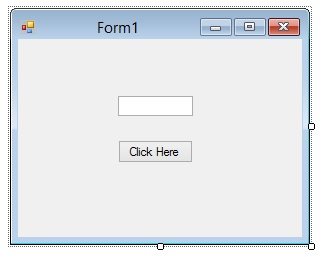
- I have changed the name of the Button to "ClickHere" and the Text of the Button to "Click Here".
- I have also changed the Name of the Text Box to "txtClick".
- Now add some code in the Button as shown in below figure:
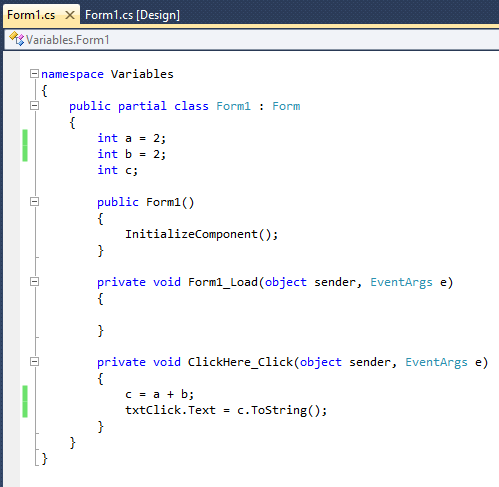
- In the above code, you can see first of all I have declared the C# Int variables, which are a, b and c.
- Then I have also assigned a value to a and b, which is 2.
- Now in the Button Click function, I have added both the C# Int variables and then saved their result in another C# Int variable named as c.
- Finally, I have printed that c variable in a text box.
- You must be wondering why I have used toString() because text boxes can only print strings but c is an integer so that's why I have converted that integer into string.
- I will discuss this string conversion in more detail later.
- Here's the code for you to use:
using System; using System.Collections.Generic; using System.ComponentModel; using System.Data; using System.Drawing; using System.Linq; using System.Text; using System.Windows.Forms; namespace Variables { public partial class Form1 : Form { int a = 2; int b = 2; int c; public Form1() { InitializeComponent(); } private void Form1_Load(object sender, EventArgs e) { } private void ClickHere_Click(object sender, EventArgs e) { c = a + b; txtClick.Text = c.ToString(); } } }
- Now run your project and when you click the button then 4 will appear in the text box as shown in below figure: