I am gonna use the same project which we have designed in our first lecture on C# named as Introduction to C# Windows Forms. So, you should also use the same project and as we know this project has one Button and one Text box. Just a recall that I have changed the Text of the button to Click Here and the name of the button to ClickHere. Similarly, I have changed the name of the text box to txtClick. Here's the image of that form:
So, let's continue with How to use C# ArrayList. :)
How to use C# ArrayList ???
Before going into the details of using C# ArrayList, let's first have a look at its definition i.e. what is C# ArrayList and why we have to use it.
What is C# ArrayList ?
- C# Arraylist is like a bucket which is used to store data in it just like C# Arrays but there's a slight difference between the two. In C# ArrayList you can add or delete data at any time and the ArrayList adjusts itself automatically.
- Addition or deletion of data in C# ArrayList is done by using indexes of those datas. So, when you add some data in it then the ArratList automatically got stretched and welcomed the incoming data in a new index. :) Similarly when you delete some data from the ArrayList then it shrinks and adjusts the data accordingly.
- We are shortly gonna have a look at How to add or delete data in c# ArrayList and how it behaves.
- Now you can see C# ArrayList is quite similar to Array with a slight difference.
- C# ArrayList is used in old C# Programming but its kind of neglected in new C# Applications because in complex codes it becomes quite messy so the coders normally neglect it and use the alternative but if you have an encounter with any old C# Project then there's a big chance that you find C# Arraylist in it.
- Now, let's have a look at how to initialize an ArrayList:
Initializing a C# ArrayList
- In order to initialize a C# ArrayList, you have to use the below code:
// .... Initialization of C# ArrayList ..... ArrayList TEP = new ArrayList(); // .... Ends Here ......
- Now in the above code line you can see I have used the ArrayList as a datatype and then I have given my variable a name, which is TEP.
- So, in simple words, I have created a ArrayList named TEP.
- One important thing is you have to add using System.Collections; in the top section of your code otherwise system won't recognize the keyword ArrayList.
Adding Data to C# ArrayList
- Now, let's add some data in our C# ArrayList, which we just created in the above section.
- In order to add the data, we have to use the command TEP.Add(), where TEP is the name of our ArrayList while our data comes in the brackets.
- So, let's add some data in our C# ArrayList by using the below code:
// .... Adding Data in ArrayList .... TEP.Add("The"); TEP.Add("Engineering"); TEP.Add("Projects"); // .... Data added in ArrayList ....
- So, I have added three values in my TEP ArrayList.
- Now, let's print them out.
- So, in your Button Click function add the below code:
// ... Displaying values ..... txtClick.Text = TEP[0].ToString(); txtClick.Text += " , "; txtClick.Text += TEP[1].ToString(); txtClick.Text += " , "; txtClick.Text += TEP[2].ToString(); // ... Values Displayed .....
- In the above code, you can see I have called those values via their indexes.
- Now your complete code will look something as shown in the below figure:
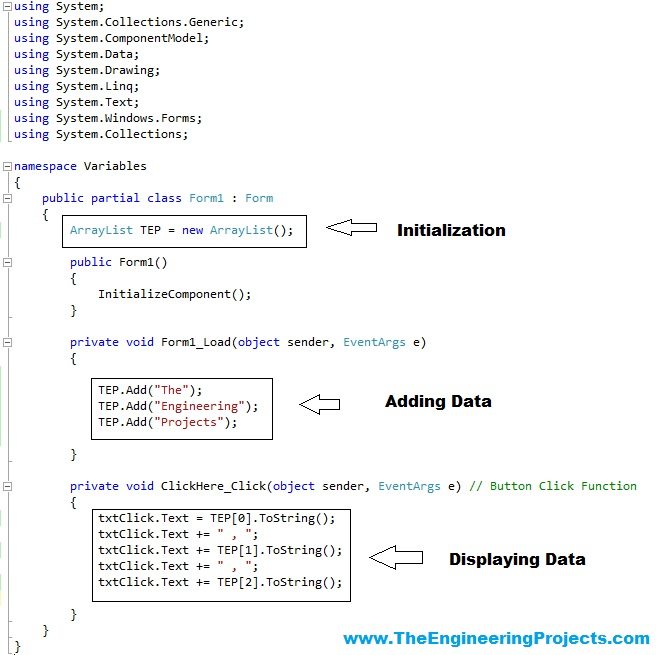
- Now, in the above figure, you can see all the three steps i.e. initialization of C# ArrayList, then adding values in that ArrayList and finally displaying those values.
- Now, when you click run your program and then click the button you will got your C# ArrayList in the text box as shown in below figure:
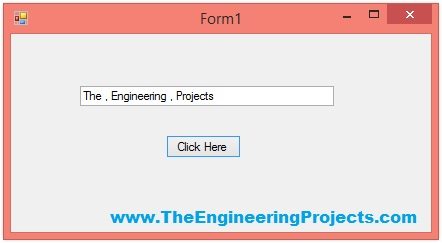
- And you can see in the above figure that our TEP ArrayList elements are printed in the text box.
ArrayList Elements Count
- Now, let's have a look at how to count the total number of elements in an ArrayList.
- In order to do so, you have to add a small code in your button click function as given in below code:
// .... Display Values ..... txtClick.Text = TEP[0].ToString(); txtClick.Text += " , "; txtClick.Text += TEP[1].ToString(); txtClick.Text += " , "; txtClick.Text += TEP[2].ToString(); txtClick.Text += " , "; txtClick.Text += TEP.Count; // ..... Values Displayed .....
- You can see in the above code, I have added a small function to display the TEP Count and when you run it, it will show you the total values in your ArrayList as shown in below figure:
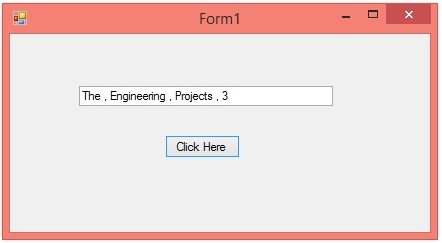
- Now you can see the last figure is showing the total number of elements in that ArrayList.
Clearing a C# ArrayList
- Now suppose you have created a C# ArrayList and now you wanna clear its values then you have to use a simple clear command as shown in below code:
txtClick.Text = TEP[0].ToString(); txtClick.Text += " , "; txtClick.Text += TEP[1].ToString(); txtClick.Text += " , "; txtClick.Text += TEP[2].ToString(); txtClick.Text += " , "; txtClick.Text += TEP.Count; TEP.Clear(); txtClick.Text += " , "; txtClick.Text += TEP.Count;
- In the above code, first of all, I have displayed the values of ArrayList and after that I have displayed the total elements in that ArrayList and then I cleared the ArrayList using TEP.Clear(); and then again displayed the total elements and because I have cleared the ArrayList that's why it will give you 0 this time because now there's no element in it as shown in below figure:
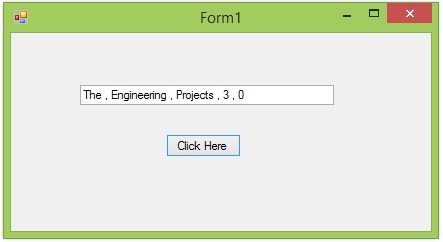
Now, I hope that you are well aware of ArrayList and can easily use it in your program if you have to. So, that's all for today, will see you guys in the next tutorial. Till then take care and have fun !!! :)