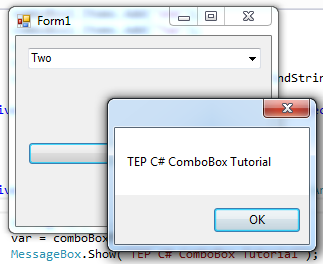
You can add the combo box from the toolbar and drag it on the form. When you drag that you can set the height and width by just moving the coordinates of combo box field. In other words, ComboBox is a combination of textbox and list box to show the list data as the text.So,now let's have a look at How to control C# ComboBox:
C# ComboBox Control
- As I have mentioned earlier, drag your ComboBox from the toolbar and drag it on the Main Form.
- If you have dragged the C# comboBox then add the following code in the main function to add the demo values in your C# combobox list.
- By using the following code, you can easily insert the values in your combobox, you can do it manually or you can take inputs from the C# TextBox too, it all depends on the situation and development scheme.
comboBox1.Items.Add("The"); comboBox1.Items.Add("Engineering"); comboBox1.Items.Add("Projects");
- Here's the screenshot of our C# ComboBox having three items which I have inserted using above c# code:
- ComboBox1 is the default name of the first combo box which you will drag on the windows form. You can change this name from the property panel which is on the right side. Even that you can insert the values from that too inside the combo box.
- Every value which you want to insert is considered as an item. You have observed in the above code we used the default.items.add("DataValue") this is the basic syntax for insertion and CRUD is also applied by this syntax.
- Now move on some advanced techniques which you can be used to make your application more interactive. Like you can be retrieved the selected items from the combo box as the value or input. You just have to take the string type variable and passed the selected item's value to it, you can do this by the following code.
var item = this.comboBox1.GetItemText(this.comboBox1.SelectedItem);
- If you want to show a message box which will be notified you that, what the item you have selected from the combo box. Then you just have to add the message box and used its attribute show to express the values, here is the code below which is working fine and you can test it in your application too.
var item = this.comboBox1.GetItemText(this.comboBox1.SelectedItem); MessageBox.Show(item);
We have inserted the values in above codes, now move on to how we can delete or remove the inserted values. As we used the Add attribute to inserted the values now we will use the Remove and RemoveAt to delete the values. These are the two attributes which we can be used for the same purpose. The first attribute will remove the values directly like you have added the value "Name" then you can delete is as Remove("Name") and the second method is RemoveAt which will remove the value by index like RemoveAt(1) if you don't get the concept, then check the example code below.
comboBox1.Items.RemoveAt(1); comboBox1.Items.Remove("The Engineering Projects");
You can even change the properties of dropdown by using the DropDownStyle property. It's used to determine that what you actually wanted, mean, are you wanted to show the list always or the data is displayed in a list. You can also edit the text alignment inside of the list.
There are three values which you can be used for the DropDownStyle Property.
- Simple
- DropDown
- DropDownList
You can check the following code where I have shared how you can use these properties, don't copy all the properties to run them at once. Because the last property will get override on all above two properties. So always do code with precautions.
comboBox1.DropDownStyle = ComboBoxStyle.Simple; comboBox1.DropDownStyle = ComboBoxStyle.DropDown; comboBox1.DropDownStyle = ComboBoxStyle.DropDownList;
You can set the default selected item in the combo box by declaring the SelectedItem property. There are two ways, one you can set it by SelectedItem or you can do by passing the value as FindStringExact. Let's move on code and understand the concept behind these two properties.
comboBox1.Items.Add("The"); comboBox1.Items.Add("Engineering"); comboBox1.Items.Add("Projects"); comboBox1.SelectedItem = "Projects";
In the above code we have inserted three values and by SelectedItem we passed the value which we want to set as the default in a combo box. Let's view the below code which is the second method.
comboBox1.Items.Add("The"); comboBox1.Items.Add("Engineering"); comboBox1.Items.Add("Projects"); comboBox1.SelectedIndex = comboBox1.FindStringExact("Projects");
In this method, we have used the SelectedIndex property and passed the string value by parsing them from FindStringExact method. This is the alternative technique to set the combo box selected item and difficult to remember. You can use them both but the first is easy to remember and understand.
You can also retrieve the database values in a combo box, by using the DataSource property. Let's check the code below how we retrieve the database values in Combo Box.
comboBox1.DataSource = datasetVariable.Tables[0]; comboBox1.ValueMember = "databaseTable_ID"; comboBox1.DisplayMember = "databaseTable_NAME";
We have to use the DataSet variable to retrieve the values from a database, then we will pass that values to DataSource property of C# ComboBox. ValueMember will store the ID values and DisplayMember stored the values of Names, just suppose a SQL query it's same like that. Such as "SELECT databaseTable_ID, databaseTable_NAME FROM StudentsTable".
If you want to manipulate the data between two C# comboBox then you can do easily. Suppose you have taken two combo box and wanted that when you select any value in the first combo box then the value of second combo box gets changed according to the first combo box. Then you have to use the SelectIndexChanged Event. Let's create the code to demonstrate the concept.
using System; using System.Windows.Forms; namespace WindowsFormsApplication1 { public partial class Form1 : Form { public Form1() { InitializeComponent(); } private void Form1_Load(object sender, EventArgs e) { comboBox1.Items.Add("TEP Arduino Projects"); comboBox1.Items.Add("TEP C# Tutorials"); } private void comboBox1_SelectedIndexChanged(object sender, EventArgs e) { comboBox2.Items.Clear(); if (comboBox1.SelectedItem == "TEP Arduino Projects") { comboBox2.Items.Add("Stepper Motor Direction Control"); comboBox2.Items.Add("Stepper Motor Speed Control"); } else if (comboBox1.SelectedItem == "TEP C# Tutorials") { comboBox2.Items.Add("How to use C# Comments"); comboBox2.Items.Add("How to add C# Control in Windows Form"); comboBox2.Items.Add("How to use Button in C# Windows Form"); } } } }
Before using this code, you have to drag two C# combobox on your form and don't change their name. Then go to the main code and replace that with the above code and execute. The first C# combobox gives you two options in the list as TEP C# Tutorials and TEP Arduino Projects. When you selected any of these values the second combo values get changed. Such as if you selected TEP C# Tutorials then the second combo box will show the values as below.
- How to use C# Comments
- How to add C# Control in Windows Form
- How to use Button in C# Windows Form
But if you selected the TEP Arduino Projects then the second combo box value is as follows.
- Stepper Motor Direction Control
- Stepper Motor Speed Control
By this, you can create innovative software and give maximum utilities to a user. This is the simple example of multiple combo boxes to give the better understanding of the concept, you can make more advanced code than the above.
You make your combo box unable for a user to give input. Sometimes we need to block the combo box on some conditions. Like combo box will not become enabled until a user will not fill some pre-check statements. There are two ways by which we will change the combo box to read-only. The first way is by changing the DropDownStyle to DropDownList. It will allow users to just read the data but a user will not able to insert any kind of data. The second method is to completely disable the combo box for a user by make the Enable property to false.
First Method:
comboBox1.DropDownStyle = ComboBoxStyle.DropDownList;
Second Method:
comboBox1.Enabled = false;
Let's revised all above concepts once again and summarize then in one code. There are much more functionalities which we don't share right now. Because that each function is the whole new concept, such as the OLED or different types of databases which you can connect with your application and the different method of retrieved the data.
using System; using System.Drawing; using System.Windows.Forms; namespace WindowsFormsApplication1 { public partial class Form1 : Form { public Form1() { InitializeComponent(); } private void Form1_Load(object sender, EventArgs e) { comboBox1.Items.Add("one"); comboBox1.Items.Add("Two"); comboBox1.Items.Add("Three"); comboBox1.Items.Add("Four"); comboBox1.SelectedIndex = comboBox1.FindStringExact("Two"); } private void button1_Click(object sender, EventArgs e) { string var; var = comboBox1.Text; MessageBox.Show(var); } } }
In the above code first, drag the combo box and button on your application and copy the code for execution. When you will execute the code it will allow you to select any item from the list and by default Two is selected. When you click the button it will show you the message with the value of selected item from C# combobox.
Here is the screenshot of C# comboBox Code. You can observe which libraries you have to use for testing the codes.
- The below video will give you better explanation of C# ComboBox: