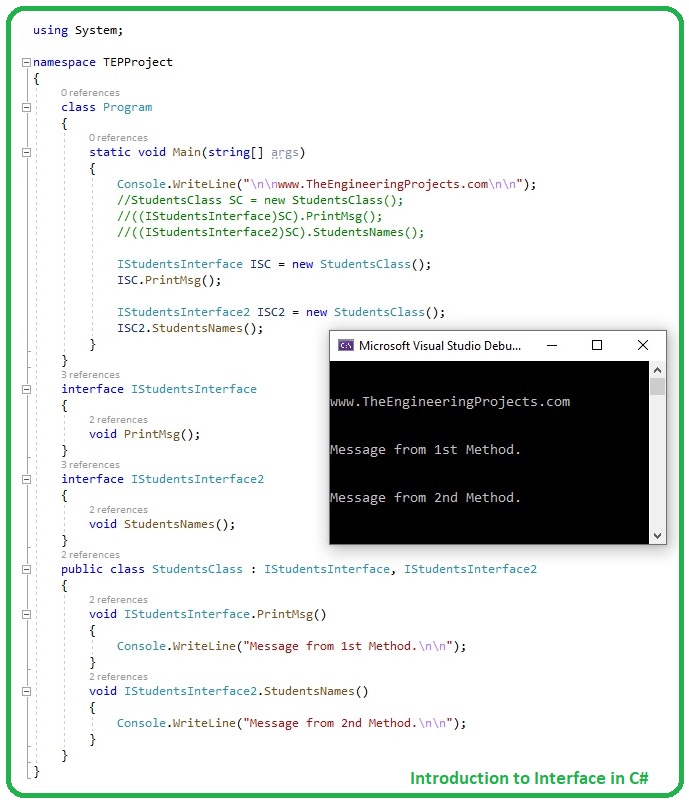
Introduction to Interface in C#
- Interface in C# is created using Interface Keyword and is used for the declaration of Methods, Properties, Events etc. but we can't add any implementation in it. ( we can't declare Fields in Interface )
- You can think of Interface as a signature, you look at the interface and you will know all Methods, Properties etc. used in your project.
- Interface members don't use access modifier in their definitions, by default they all are public.
- It's a naming convention to use capital ( I ) as the first character of interface name, so that you know, just by looking at the name, that it's an Interface.
- Let's have a look at the syntax of Interface , before exploring it any further:
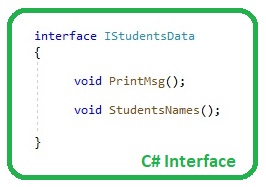
- In the above figure, I have created an interface named IStudentsInterface and it contains declaration of two C# Methods.
- We can't implement these methods in Interface, as it will generate a compiler error.
- Now you must be wondering, what's the benefit of using Interfaces, when we can't add the Implementation, and here comes Inheritance:
Interface Inheritance in C#
- Interfaces are normally used as Parent Node in Inheritance, while C# Classes and C# Structs are inherited from Interfaces.
- We can inherit a class or struct from multiple Inheritances, separated by commas. (We can't inherit a class from multiple classes)
- When we inherit a C# Class or Struct from an Interface, then it must provide the implementation for all the Members of that Interface.
- If we miss implementation of any single member, then compiler will generate an error.
- We can't create a new instance of Interface as we can do for Classes & Structs, although we can create an Interface Reference variable that will point to its derived class object.
- So, let's inherit a C# Class from our interface IStudentsInterface and see How it works:
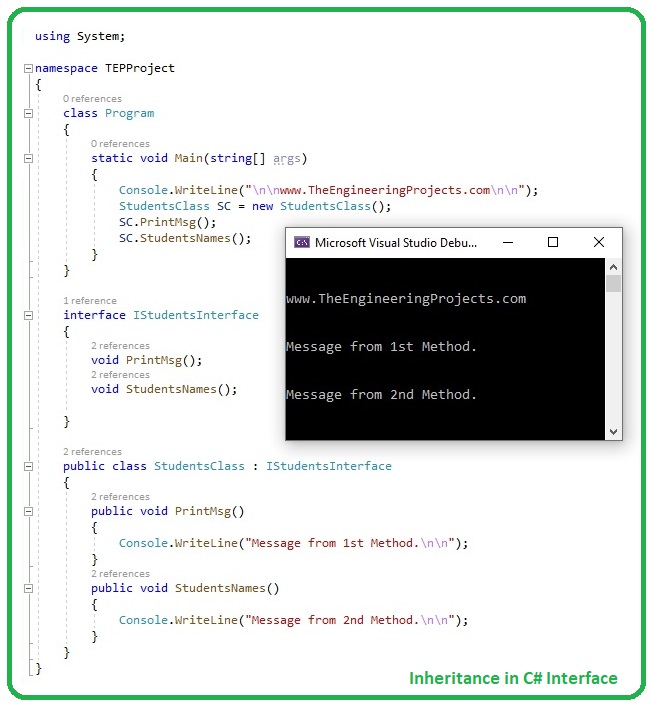
- In the above figure, I have created an Interface named IStudentsInterface and it has declaration of two Methods i.e. PrintMsg() & StudentsNames().
- After that, I have inherited a class named StudentsClass from this interface IStudentsInterface .
- This class must have the implementation of those two methods we declared in our Interface IStudentsInterface.
- So, I have just created two simple methods with Console ouput, so we remove any one of them, the compiler will generate an error.
- Let's inherit our class from multiple interfaces, shown in below figure:
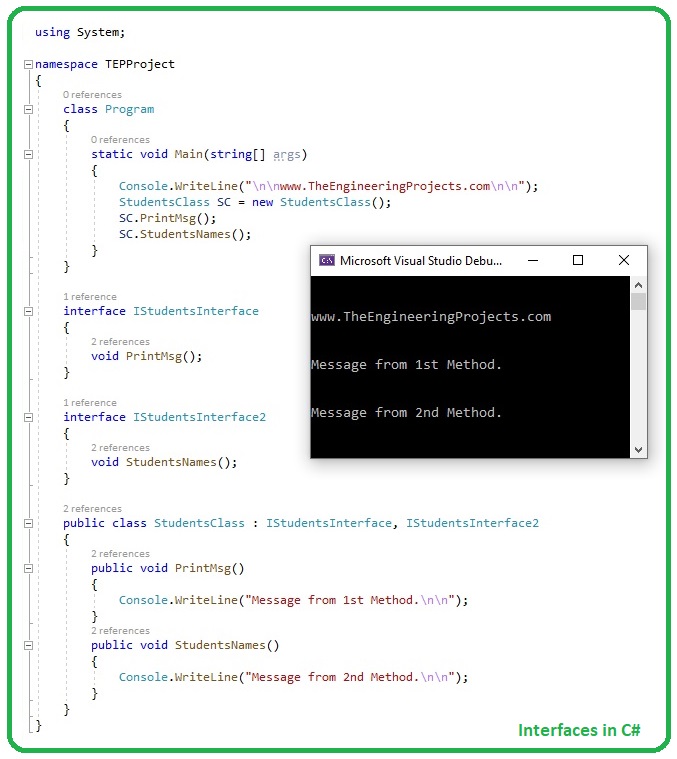
- In the above figure, I have created two C# Interfaces having 1 Method each.
- Moreover, the class is inherited from both of these interfaces, separated by commas, so it must have the implementation of both of these methods.
- Similarly, if an interface is inherited from another interface and you are inheriting your class from Child Interface, then still you must have the implementation of all Members of these two Interfaces in your class.
- Let's have a look at this multiple level inheritance of Interfaces:
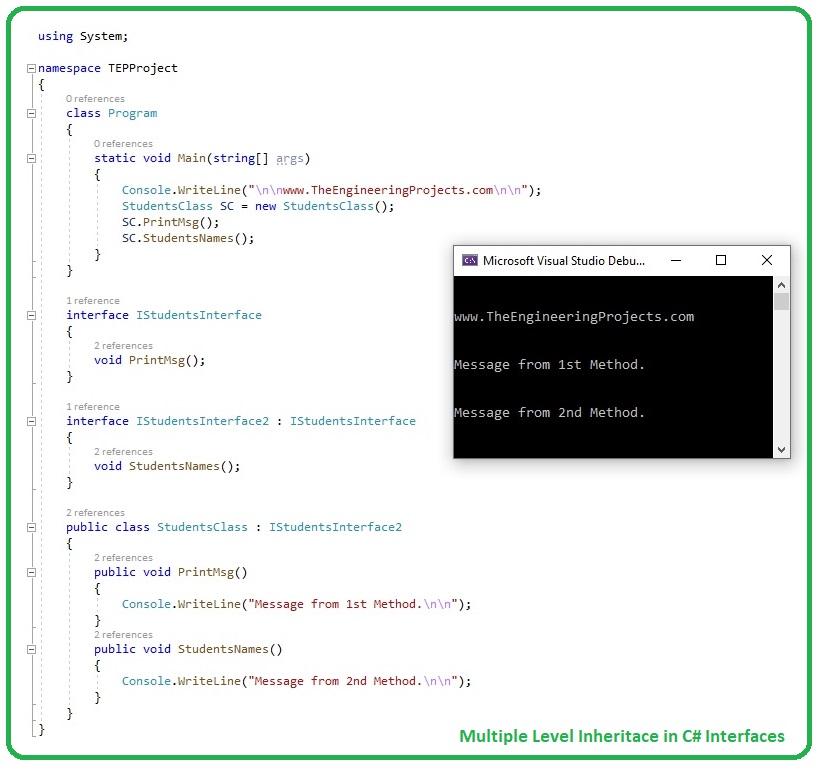
- You can see in above figure, that IStudentInterface2 is inherited from IStudentInterface and our class is inherited from IStudentInterface2. (Like a chain)
- So, in this case as well, our class must have the implementation of both these methods, otherwise we will get compiler error.
- This type of Interface Members' Implementation in C# Classes or Structs is called Implicit Interfaces Implementation.
- So, now let's have a look at Explicit Interfaces Implementation:
Explicit Interfaces Implementation
- In Explicit Interfaces Implementation, we have to use the Interface Name along with dot operator and then name of the Method in its implementation.
- An example of Explicit Interfaces Implementation is shown in below figure:
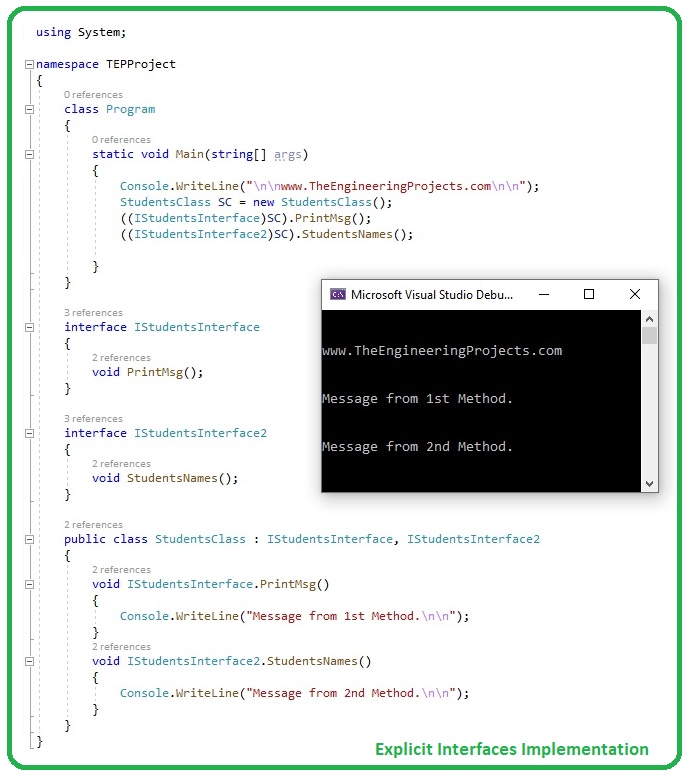
- As you can see in above figure that our class is inherited from two interfaces.
- Moreover, in this class I have implemented C# Methods explicitly i.e. now compiler won't need to find which method belongs to which interface.
- Instead the interface name is present in Methods' definition along with dot operator ( . ).
- You must have noticed that now we have removed the access modifier and its public by default as of Interface members.
- When Interface members are implemented explicitly, then we can't access them using reference variable, instead we need to use cast operator:
( ( IStudentsInterface) SC ) . PrintMsg();
- So, we need to use above method in order to invoke the Explicitly Implemented Interface Method.
- There's another way as well, which I have told you at the very top of today's tutorial i.e. we can't create a new instance of Interface yet we can create its reference variable, as shown in below figure:
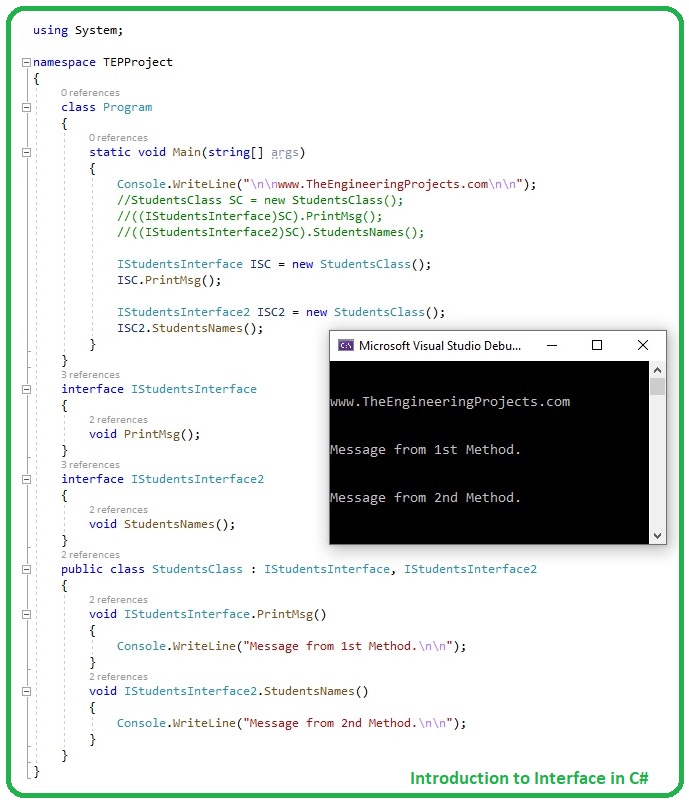
- You can see in above figure that in Main function, I have created Interface variables both pointing to its derived class object.
- Now, as they are interface variables so we can use them to invoke respective explicitly implemented method.