Let's take an example for C# arrays from normal life. You can consider a classroom of a school as an array and its elements will be all the students in that class. :) So, if there's a 9th class then all the students in 9th class are the members of that 9th class array but the students of 10th class will not be members of 9th class array, instead they will be members of 10th class array. :P Now, a question arises that how to call a single member of any array then in that case for class arrays we have roll numbers. so you can call any member of 9th class by calling his roll number. Similarly, in C# array we have indexes for each member of an array and we can call individual members using these indexes, we will have a look at it below. The members of any arrary are normally called the Elements of that array. So, let's get started with C# arrays.
You may also like:
How to use C# Array ???
- As I explained earlier, C# Array is used to store similar variables in it. So, instead of using individual variables, we can simply add all those similar variables in a single array.
- So, an array is something as shown in below figure:
FirstArray[3] = {Element1, Element2, Element3};
- Now the above array has a name FirstArray and it can has maximum three members in it which is shown in these [ ] brackets.
- After that we have inserted the three members in it separated by commas.
- Now, if I want to access the individual members of this C# Array then I have to call them by their indexes.
- C# Array assigns an index to each of its elements and these indexes start from 0.
- So, the first element of each C# Array is always 0.
- So, if I call the 0th member of C# array then it will give me the first element of that array.
- Let's see how to call each of them:
- FirstArray[0] = Element1;
- FirstArray[1] = Element2;
- FirstArray[2] = Element3;
- So, that's how we can call any of the C# Arrays elements.
- Now let's design a simple projects and check it out in real example.
- So, create a simple C# project as we did in Introduction to C# Windows Forms.
- Now in this C# projects add some controls as we did in How to add C# Control in Windows Form.
- I have added two controls in it which are Button and a Text Box.
- The simple GUI is shown in below figure:
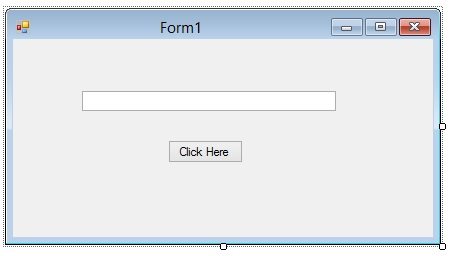
- So, now let's add an array in this GUI and then display its elements in the text box on button click.
- I am gonna add a C# string array so first of all, what I need to do is to declare the String C# array.
- In order to do so use the below code:
// C# Array Initializing String[] students = new String[5]; // Initializing Complete
- So, you can see in the above code that I have initialized the C# array and in order to do so first of all, I have given the datatype of C# Array, which is String in the above case.
- After that, I have given the name of the C# Array which is students and the I have used new word to create the new instance of C# array.
- We will cover this new word concept in later tutorials but rite now its necessary to use it while initializing the C# array.
- Finally I have given the length of array which is 5 in our case, so you can add maximum 5 elements in this C# array.
- You can also make the length variable by simply removing this 5 as shown in below code:
// C# Array Initializing String[] students = new String[]; // Initializing Complete
- Now this C# String Array is of variable length means you can add any kind of members in it.
- Now we have initialized our C# String Array, next thing we need to do is to add the values in it.
- One way of adding values to the C# array is as follows:
// Adding values to C# Array. students[0] = "Zain"; students[1] = "Nasir"; students[2] = "Kamraan"; students[3] = "John"; students[4] = "Jack"; // Values added.
- Now you can see I have added five students in our C# Array and I have also assigned the index to each of them.
- "Zain" is saved at index 0 of students array so it will be the first element of C# array.
- That's one way of assigning values to C# array, here's another way:
// Adding values to C# Array. String[] students = new String[5] {"Zain", "Nasir", "Kamraan", "John", "Jack"}; // Values added.
- In the above code, I have added the values in the initialization, both ways are correct.
- Now let's get the elements from this array and display them in the Text Box.
- In order to do so, use the below code:
txtClick.Text = students[0]; txtClick.Text += " , "; txtClick.Text += students[1]; txtClick.Text += " , "; txtClick.Text += students[2]; txtClick.Text += " , "; txtClick.Text += students[3]; txtClick.Text += " , "; txtClick.Text += students[4];
- One thing, I forgot to mention that I have changed the Name of the Button to ClickHere and Text of Button to Click Here. Moreover, I have changed the Name of Text Box to txtClick.
- Now your complete code will look something as shown in below figure:
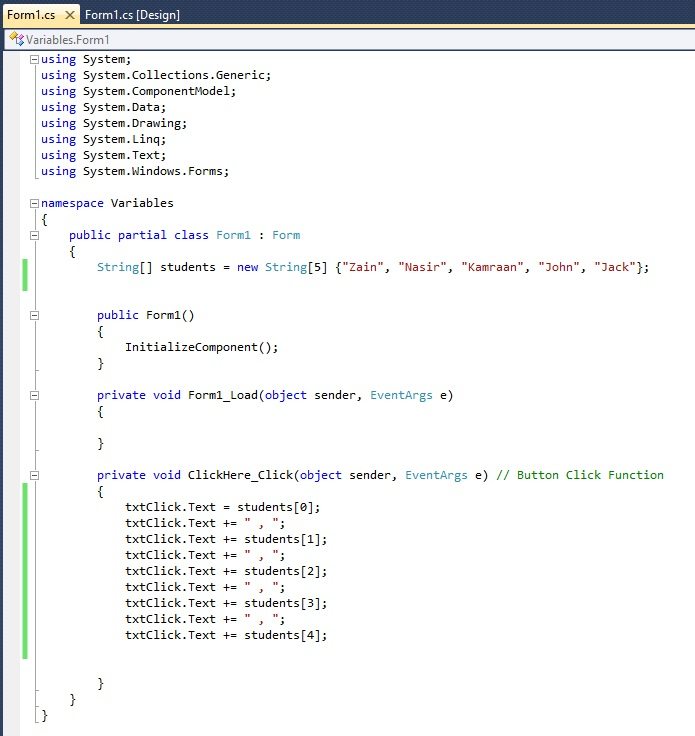
- Now run your project and click the button and if everything goes fine then you will get results as shown in below figure:
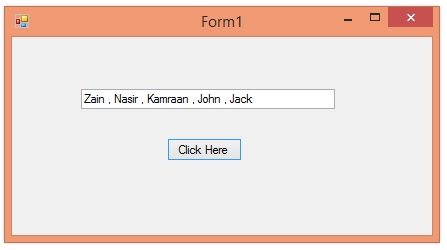
- So, you can see all the elements of our C# Array are now displayed in the Text box.