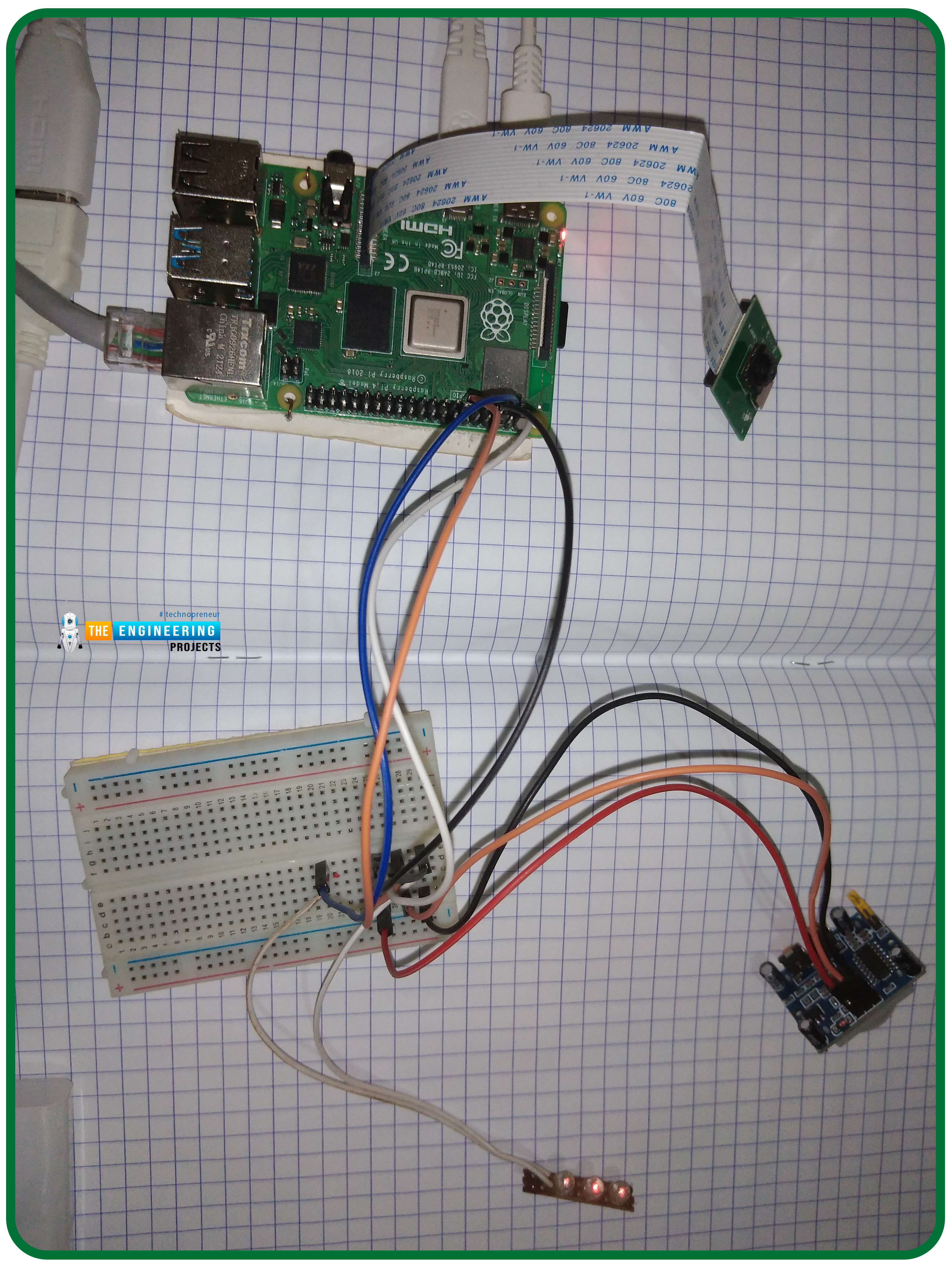
Thank you for joining us for yet another session of this series on Raspberry Pi programming. In the preceding tutorial, we constructed a personal Twitter bot using Tweepy, a Py framework for querying the Twitter application programming interface. We also constructed a Response to robot mentions that would post a response to everybody's tweet mentioning it with a certain keyword. However, in this tutorial, we will implement a security system using a motion sensor with an alarm.
This is what it looks like:
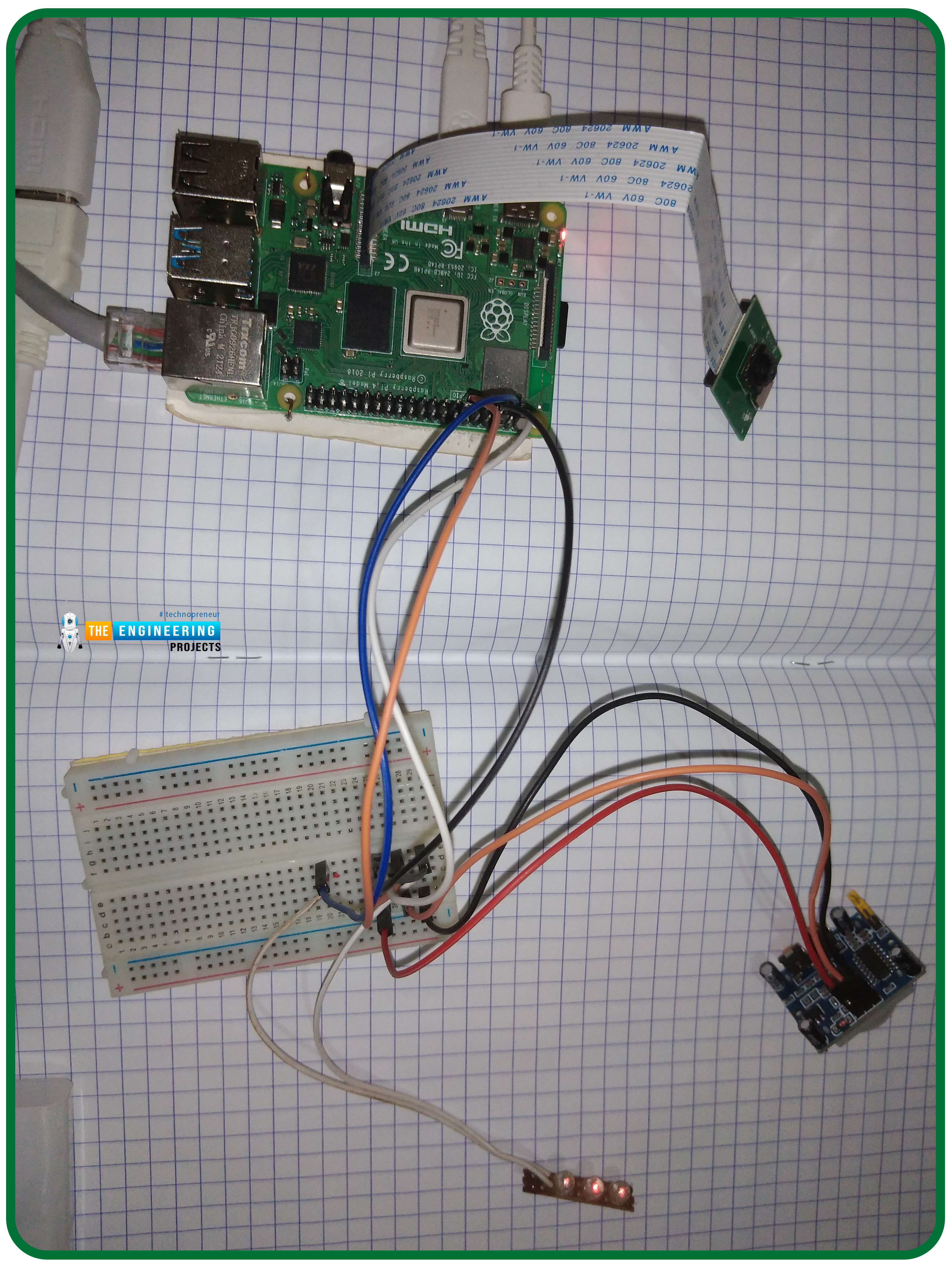
PIR Motion Sensors can be implemented with RPi by understanding how it is connected to a Raspberry Pi. Whenever the motion sensor detects human movement, an alarm is triggered in this project and the LEDs blink. You may create a simple motion-detection alarm using this interface.
Overview
Infrared Motion Detectors or PIR Sensors are Motion Sensors that use Infrared Radiation to detect movement.
Infrared rays are emitted by anything with a temperature higher than absolute zero, be it life or non-living. Humans are unable to see infrared radiation because its wavelength is longer than the wavelength of visible light.
That's why PIR Sensors are designed to pick up on those infrared rays. Due to their wide range of uses, such as motion sensors for security systems and intruder alert devices
"Passive" in motion sensor refers to the fact that it doesn't produce any radiant rays of its own, but rather detects it when other things emit infrared radiation. This is in contrast to active detectors, which perform both the generation of infrared waves and the detection of these waves simultaneously.
An Overview of Motion Detectors
For this project, we used a motion detector that included an infrared sensor, a BISS0001 integrated circuit, and other parts.
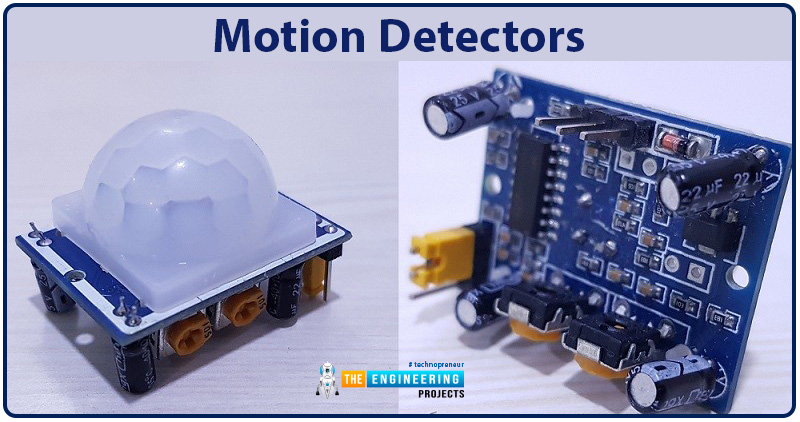
The 3 pins on the motion sensor are used for power, data, and ground. There are two potentiometers on the Motion Sensor that may be used to modify both the sensor's sensitivity and the period it remains high on sensing a body movement.
A key role in directing infrared rays onto the sensor is played by the Fresnel lens overlaying the Pyroelectric Sensor. This lens allows the PIR Sensor to detect things at an angle of 1200 degrees. The sensor has an 8-meter detection range, meaning it can pick up on human movement within that distance.
PIR Sensor Adjustments
Two potentiometers are provided for fine-tuning the sensor and output timing, as previously described.
With the aid of a potentiometer, you may modify the sensor's sensitivity. The distance can be changed between 3m and eight meters. To increase the detecting distance, spin the Potentiometer in a clockwise motion and to reduce, rotate it in the opposite direction.
The second potentiometer allows you to choose how long the motion sensor's output remains HIGH. Anywhere from 0.3s to 600s can be used. Turn the POT clockwise to raise the time and the opposite turn to decrease it.
PIR Motion Sensor with Raspberry Pi 4
A Motion Sensor based on RPi and Python language has been the goal of this project since the beginning, as stated in the intro.
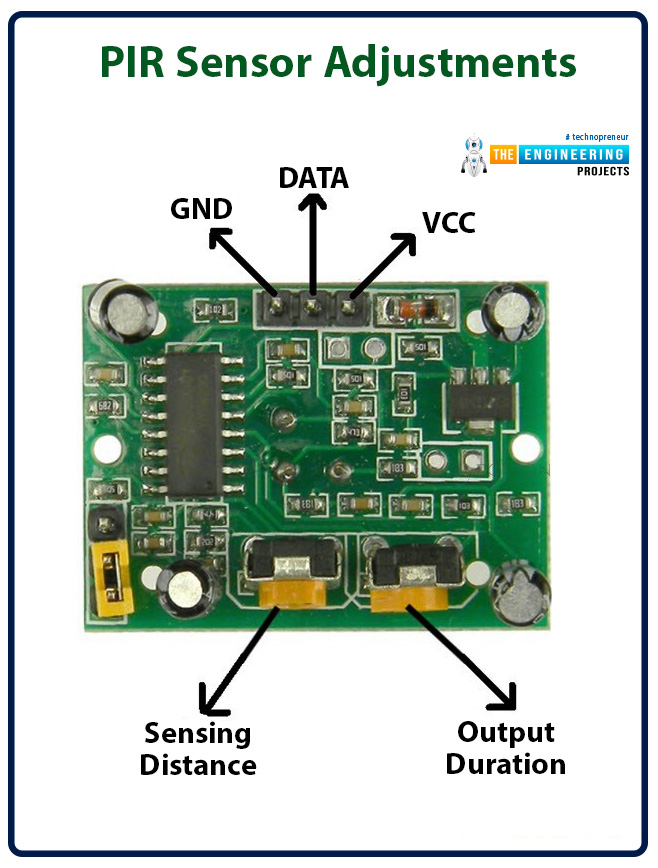
I have an Infrared Motion Sensor Component in numerous different projects like Automated Lighting using Raspberry and Various Sensors, Automated Door Opening with Arduino and a motion sensor, and GSM Home Automation Security with Pi.
The key advantage of the Infrared Motion Sensor utilizing RPi over the above-described projects is that RPi can be readily connected to the Web and allows Internet of things implementation of the project.
Circuit Diagram
The following figure illustrates the interfaces concerning the Infrared Motion Detector using RPi.
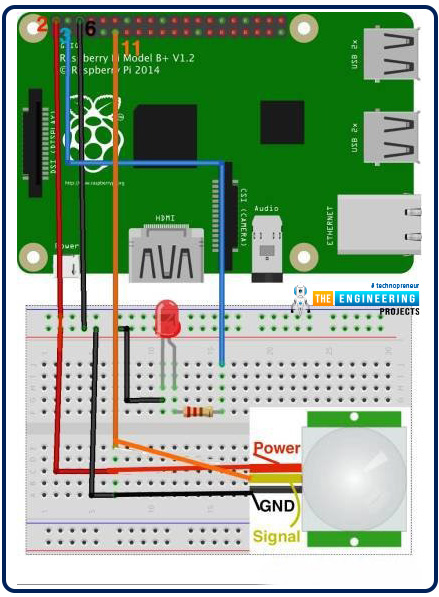
Components Required
Raspberry Pi 4
PIR Sensor
Speaker
Jumper Wires
Breadboard
Power Supply
Computer
Circuit Design
Link the Motion Sensor's Vin and GND connectors to the RPi's 5 volts and GND pins. Use pin11 to attach the Infrared Sensor's DATA Input.
Gnd and pin 3 are where you'll want to connect the led. As soon as the sensor is triggered, these LEDs will come on and go off.
Code
Python is used for the programming portion of the project. The Python program for RPi's infrared Motion Sensor is provided below. Insert the program into a new file called motion.py.
import RPi.GPIO as GPIO
import time
GPIO.setwarnings(False)
GPIO.setmode(GPIO.BOARD)
GPIO.setup(11, GPIO.IN) #Read output from PIR motion sensor
GPIO.setup(3, GPIO.OUT) #LED output pin
while True:
i=GPIO.input(11)
if i==0: #When output from motion sensor is LOW
print("No intruders",i)
GPIO.output(3, 0) #Turn OFF LED
time.sleep(0.1)
elif i==1: #When output from motion sensor is HIGH
print("Intruder detected",i)
GPIO.output(3, 1) #Turn ON LED
time.sleep(0.1)
How it works
The operation of the Infrared Motion Sensor with Raspberry Pi is pretty straightforward. If the Infrared sensor senses some body motion, it sets the Data Input to HIGH.
RPI on identifying a 1 on the associated input gpio, will trigger the alarm.
If the PIR sensor is not working:
When you purchase a new sensor, it doesn't work. The Trim port is in the default setting, so it's not a sensor issue. Sensitivity of the sensor and trigger duration port if you modify these settings. It's going to start working as planned. Make sure the trigger duration port's knob is on the left as a low trigger duration and the sensitivity port is in the middle.
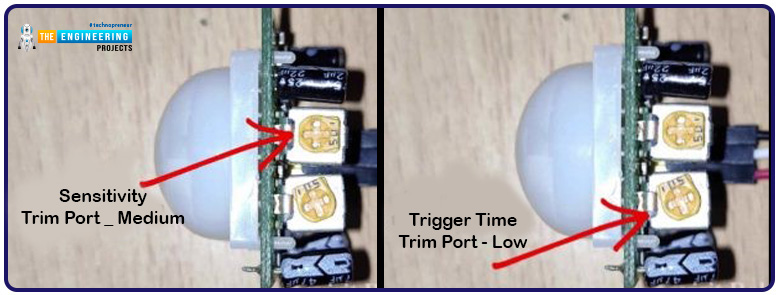
Applications
Infrared Motion Sensor with Raspberry Pi has already been discussed. They include:
Automated house lights
Motion sensing
Intruders notice
Automated door open
Home security systems
When motion is detected by the PIR sensor on the raspberry pi, we will look into how to record video and transmit it to Whatsapp as an alarm. So that we can tell who's in your room right away thanks to the photo.
Capture an image with the pi camera
Enable the camera by going to the Preferences menu and selecting the Raspberry Pi configuration option.
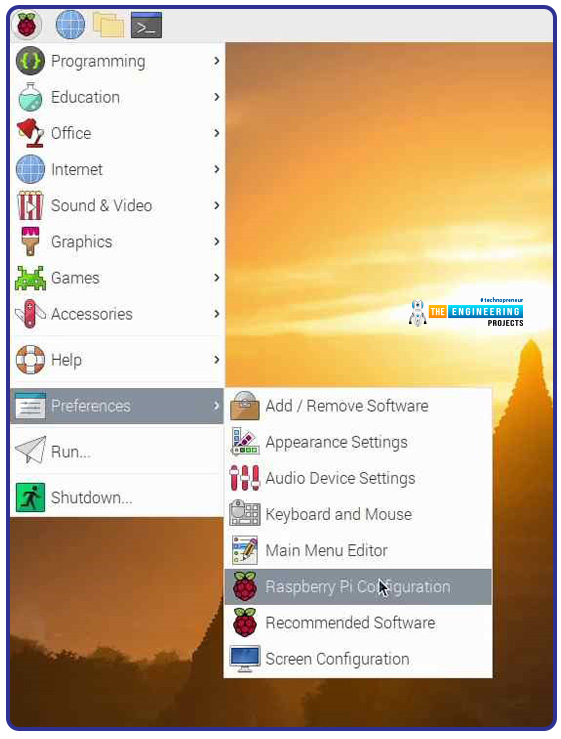
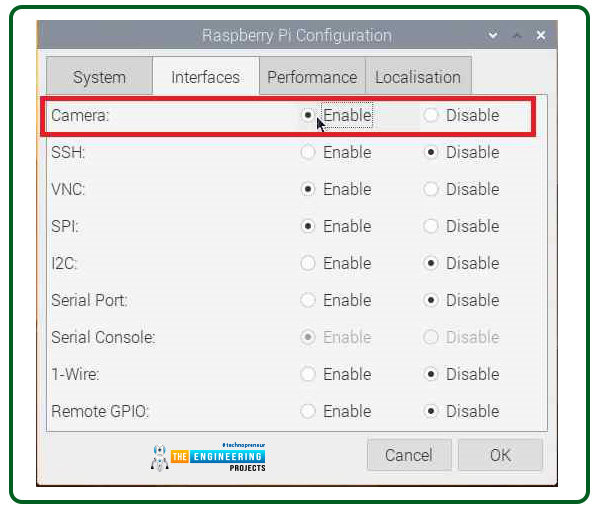
Activating the camera and saving the image will allow us to identify who or what triggered the alarm.
Python code
import picamera
from time import sleep
camera = picamera.PiCamera()
camera.capture('image.jpg')
When we run our software, the preceding code will take a picture and put it inside the root directory of the script. This image will be used to identify the intruder that has been detected.
Sound alarm
When an alarm system is triggered, there is an alert that must sound. We'll use a loudspeaker instead of a buzzer for our alarm system in this scenario. When the motion sensor is activated, we will play an alarm sound.
Code:
import pygame
pygame.mixer.init()
pygame.mixer.music.load("alarm.mp3")
pygame.mixer.music.play()
while pygame.mixer.music.get_busy() == True:
continue
As a bridge python software for video game design, Pygame is an excellent choice. Additionally, it provides sights, sounds, and visualizations that can improve the game that is being created.
Graphics for video games can be generated using a variety of libraries that deal with visuals and sounds. It streamlines the entire game workflow and makes it easier for newcomers who wish to create games.
Copy the code above and save it to a file named alarm.py then run it in the terminal.
python alarm.py
Send an image to Whatsapp using Twilio
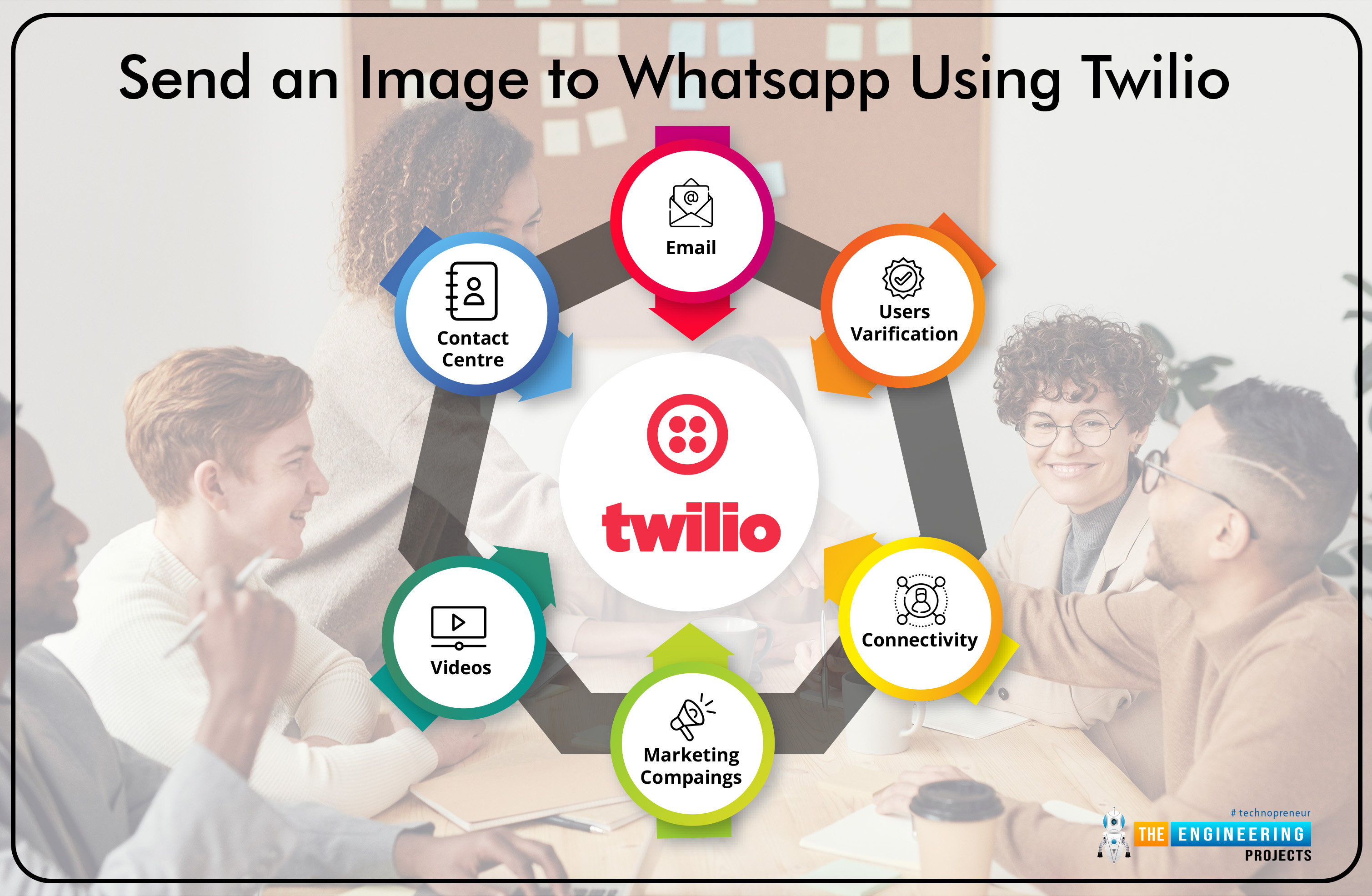
Any internet or mobile app's compatibility with several platforms was a major hurdle to overcome when designing it. It used to be possible to build a link between two pieces of software using Bandwidth or Podium or Telnyx or Zipwhip or similar before Twilio was invented. In recent years, though, Twilio has dominated the competition. Twilio has become the preferred communication API for programmers. Twilio will become clearer to you if you stick around for a time.
What is Twilio
Developers can use Twilio's API to communicate with each other in a modern way.
When it comes to creating the ideal client experience, developers have a wealth of tools at their disposal in the form of Twilio's APIs, which the company describes as "a set of building blocks."
It is possible to utilize Twilio to communicate with customers via text message (SMS), WhatsApp, voice, video, and email. Your software only needs to be integrated with the API.
What does Twilio do?
Twilio is a provider of end-to-end solutions for integrating voice and data communication. Twilio is already used by over a billion developers and some of the world's most well-known businesses. The Twilio Communication application programming interface enables web and mobile app developers to integrate voice, message, and video conferencing capabilities. This makes it easier for app developers to communicate with one another.
The API provided by Twilio makes it simple and accessible to communicate across the web. Mobile and web applications can use this service to make phone calls as well as send text messages and multimedia messages (MMS).
How Does Twilio Work?
You might want to learn more about Twilio and how it works. As a result, Twilio allows enterprises to better understand their customers than any other service. Twilio's primary concept is to acquire clients, get to know them, provide for their needs, and keep them coming back.
Twilio has a worldwide operations center that keeps an eye on carrier networks around the clock to ensure that they are operating at peak efficiency. To keep up with the ever-changing traffic patterns, Twilio's skilled communications engineers are on the job all the time.
They employ real-time feedback from several provider services to make smarter routing decisions based on real-time data on the availability of handsets. The key distinction between Twilio and other application programming interface integration networks is that Twilio's data-centric strategy provides customer engagement service.
Key Areas Of Twilio
Contact Center
Managing a contact center in today's business environment is critical to the success of the company. Businesses can use Twilio to manage their interactions with clients and consumers through a central contact center platform.
Messaging
Before Twilight, sending mass SMS was a difficult task. Now, the Twilio Message application programming interface is widely used to transmit and receive messages, MMS, and OTT communications worldwide. Users can verify whether or not messages have been delivered using the intelligence tracking services.
Videos
For healthcare, virtual classrooms, recruiting, and other uses, Twilio's WebRTC and cloud infrastructure components make it easy for developers to create secure, video, and HD audio applications.
Marketing Campaigns
Twilio's ability to run and manage marketing campaigns is another noteworthy but still-evolving feature. Users can examine performance numbers, run campaigns, and view design concepts.
Voice
As a result of this trend, Twilio has also seen an increase in voice traffic. Any app, website, or service can use Twilio to make phone calls over the PSTN or SIP. It's easy to use Twilio Programmable Voice to make and manage digital calls for any campaign.
The Twilio SendGrip application programming interface eliminates the issue of emails that never make it to their intended recipient's inbox. Customers and clients will receive your emails with Twilio, so you won't have any worries about them not getting them.
User Verification
You'll never have to worry about online scams or fraud again using Twilio's verify feature. It is continuously validated by SMS, Voice, email, and push alerts continuously.
Connectivity
Advancing solutions and services provided by Twilio allow for global connectivity. As a result of this connectedness, your company can grow with ease.
Obtain Twilio credentials
The Twilio WhatsApp sandbox
Developing and testing your app is made simple using Twilio's WhatsApp Sandbox. Your Twilio mobile number must be approved by WhatsApp before you can seek production access.
You'll learn how to connect your phone to the environment in this section. Select Messaging in the Twilio Console and then Take a look at the WhatsApp section by clicking on it. On the webpage, you'll find the information you need to join our sandbox.
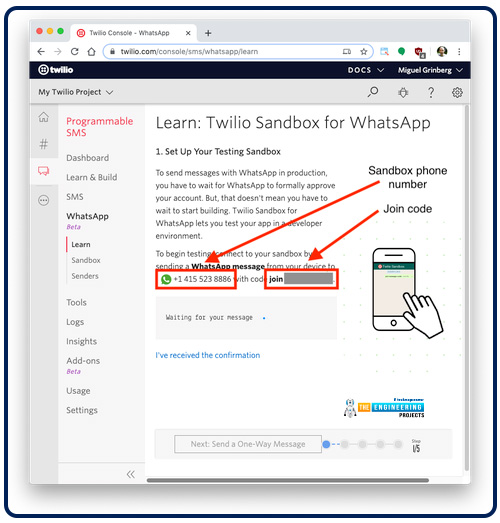
The word "join" will be the first character in the code, followed by a two-word phrase chosen at random.
As soon as Twilio receives your message, you should be able to send and receive text messages on your cell phone without any issues.
Please repeat the sandbox application process for each additional mobile device that you wish to use to test the application
Configuration
Set up a new Python project in the following section.
mkdir python-whatsapp-pic
cd python-whatsapp-pic
We'll need a virtual space for this project because we'll be installing several Python packages.
Open a terminal on your RPI machine and type:
python -m venv venv
source venv/bin/activate
(venv) $ pip3 install twilio
When using a PC running Windows, execute these commands from a command line.
python -m venv venv
source venv\bin\activate
(venv) $ pip3 install twilio
Python's Twilio library will be used to deliver messages via Twilio.
Authenticate against Twilio services
To authenticate with the Twilio service, we must safely store a few critical credentials. To use Twilio we need to register for an account at the official Twilio website. Create a new account with your email and password. They will send a confirmation message to your email inbox for you to confirm the registration. Go ahead and confirm it. You will also have to verify your WhatsApp phone number to proceed.
Setting environment variables can be done by entering the code below into your terminal:
ssh auth token
export TWILIO_ACCOUNT_SID="your account sid"
export TWILIO_AUTH_TOKEN= "your auth token"
after we have exported the credentials in our environment, the next step is to activate the WhatsApp sandbox to receive messages. Go to the develop mode, then select messaging and send a Whatsapp message.
You will see a message directing you to deliver a text to your phone and if Whatsapp is connected to the computer, it will be easier to click on the link that will be provided below to send the message. Send the message that will be displayed on the chat box on your Whatsapp application.
If it works you will see a message shown below:
This number that will be displayed here is the “from” number that we will use in our code and the “to” number is your Whatsapp number.
How to send a photo message, using the Twilio service
Copy the following code into your python file.
from twilio.rest import Client
account_sid = os.environ['TWILIO_ACCOUNT_SID']
auth_token = os.environ['TWILIO_AUTH_TOKEN']
client = Client(account_sid, auth_token)
from_whatsapp_number = 'whatsapp:+14155238886'
to_whatsapp_number = 'whatsapp:+254706911425'
message = client.messages.create(body='The engineering project sent your this image!',
media_url='https://www.theengineeringprojects.com/wp-content/uploads/2022/04/TEP-Logo.png',
from_=from_whatsapp_number,
to=to_whatsapp_number)
print(message.sid)
With this now all we have to do is run our app.py program on the terminal.
python app.py
import pygame
import RPi.GPIO as GPIO
import time
import picamera
camera = picamera.PiCamera()
GPIO.setwarnings(False)
GPIO.setmode(GPIO.BOARD)
GPIO.setup(11, GPIO.IN) #Read output from PIR motion sensor
GPIO.setup(3, GPIO.OUT) #LED output pin
pygame.mixer.init()
pygame.mixer.music.load("alarm.mp3")
import os
from twilio.rest import Client
account_sid = os.environ['TWILIO_ACCOUNT_SID']
auth_token = os.environ['TWILIO_AUTH_TOKEN']
client = Client(account_sid, auth_token)
from_whatsapp_number = 'whatsapp:+14155238886'
to_whatsapp_number = 'whatsapp:+254706911425'
while True:
i=GPIO.input(11)
if i==0: #When output from motion sensor is LOW
print("No intruders",i)
GPIO.output(3, 0) #Turn OFF LED
pygame.mixer.music.stop()
time.sleep(0.2)
elif i==1: #When output from motion sensor is HIGH
print("Intruder detected",i)
GPIO.output(3, 1) #Turn ON LED
pygame.mixer.music.play()
capture image
camera.capture('intruder.jpeg')
#send image to whatsapp
message = client.messages.create(body='The engineering projects program has detected and intruder!',
media_url='https://external-content.duckduckgo.com/iu/?u=https%3A%2F%2Ftse4.mm.bing.net%2Fth%3Fid%3DOIP.q1z1XWRn_WAV4oM-Qr2M2gHaGb%26pid%3DApi&f=1',
from_=from_whatsapp_number,
to=to_whatsapp_number)
print(message.sid)
time.sleep(0.2)
GPIO.cleanup()
break
Captured image
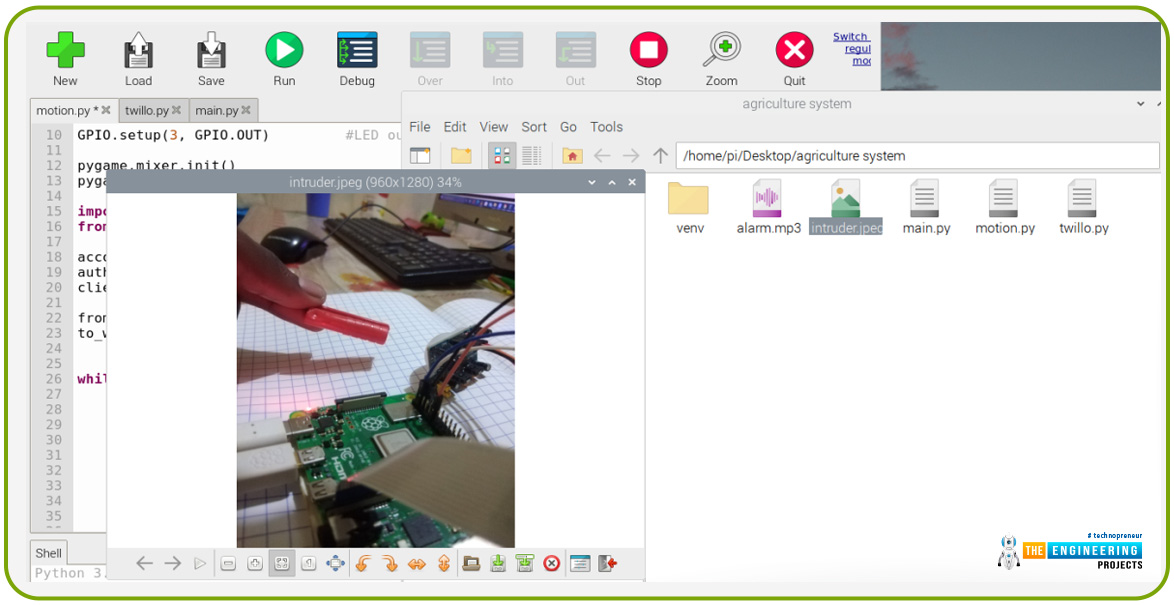
Conclusion
In this article, you learned to build a security system using a motion detector and raspberry pi. We also learned how to set up Twilio to send and receive Whatsapp messages using the Twilio API. This project can be implemented in so many areas therefore it is a good idea for you it plays around with the code and implements some extra features. In the next tutorial, we are going to build a led cube in raspberry pi 4.